Familiarise yourself with hash maps in Java, including its unique implementation methodology and specifics of its syntax. Lastly, expand your knowledge by learning about hash maps in Python. Discover the implementation details in this versatile programming language and equip yourself with a solid understanding of Python's hash map syntax. This guide ensures that you'll acquire a firm grasp of hash maps, a vital tool in your programming arsenal.
Understanding Hash Maps in Computer Science
In the world of computer science, you'll undoubtedly come across a structure known as the hash map. More than just helping to organise data, this subject no doubt falls under fundamental knowledge you need to navigate this tech-laden terrain.
What Are Hash Maps: An Explanation
A hash map is a type of data structure that implements map interfaces such as associating keys with values. In simpler terms, it's a way to store pairs of data- keys and corresponding values.
Now, to better grasp what hash maps do, it's important to understand the main components that come into play:
- The Key: A unique identifier for a piece of data.
- The Value: The specific data associated with a key.
- The Hash Function: This function takes the input (key) and returns an integer. This integer is then used as an index to store the corresponding value.
The beauty of hash maps lies in one particularly impressive quality: speed. This data structure operates much faster than most – constant time, or O(1) on average for lookups and insertions, if we're speaking in terms of algorithmic complexity.
The Basics of Hash Map Implementation
Hash map implementation refers to the steps and methods used in putting this data structure into action. It begins with creating an empty hash map, defining the hash function, inserting keys and values, addressing possible collisions, and finally accessing or removing values.
For a generic implementation of a hash map, consider this simple diagram:
Step | Action |
---|---|
1 | Create an empty hash map, either from scratch or using a pre-built data structure in your programming language of choice. |
2 | Define your hash function. Remember, a doable hash function takes the key as input and returns an integer to serve as an index. |
3 | Insert the key-value pairs into the hash map. The key is processed through the hash function, and the returned index determines where the value is stored. |
4 | Address collisions. A collision occurs when the hash function returns the same index for two different keys. To handle this, you might use chaining (where each index points to a linked list of keys and values) or open addressing (where collisions are resolved by finding the next open slot). |
5 | Access or remove values. Use the key and the hash function to find the exact index of the value you want to access or remove. |
For instance, imagine you're creating a student directory, with student ID numbers serving as the keys and the student's names as the values. You'd hash the ID numbers to get unique indices, and then store each name at its respective index in the hash map.
Dissecting the Hash Map Syntax
In the realm of code, the hash map syntax can be relatively easy to grasp, depending on the language. As computers navigate the world of binary, let's illustrate this with a pseudo-code example:
hash_map = HashMap.new() hash_function = f(x) /* some function of x */ hash_map.insert(hash_function("key"), "value")
This initiates a hash map using our predefined function, and inserts a value with a corresponding key. The inclusion of the hash function ensures our key can translate to an appropriate index within the structure. Note that the hash function isn't explicitly defined here - it could be any function designed to take the key as input and output an index.
Hence, hash maps are versatile data structures capable of storing paired information in a way that allows for rapid data access. Mastering them is an excellent move towards becoming a proficient coder.
Exploring Hash Maps in Java
Welcome to the world of Java, a popular programming language widely used for building server-side applications, video games, and mobile apps. Among the many data structures Java supports, one stands out for its usefulness and efficiency: the Hash Map.
Breakdown of Hash Maps Java Implementation
In Java, the HashMap class, part of the Java Collections Framework, implements the Map interface and uses a hash table as an underlying data structure. It lets you store elements in pairs: keys and values. What makes it unique is its high efficiency for lookup, insert, and delete operations.
Consider a simple scenario: you need to design a phone book that maps people's names to their phone numbers. With a list or an array, you'd probably perform a linear search to find a name - a computationally expensive operation taking O(n) time. But with a Hash Map, this simple lookup drops to a constant time complexity - O(1).
In Java, a Hash Map works by using a hash function to generate a unique code for each key. This code then determines where the value paired with the key should be stored. By storing values at unique positions, hash maps achieve constant time for essential operations.
Suppose you have three contacts: John, Emma, and Robert with phone numbers 9876543210, 1234567890, and 1111222233 respectively. In a hash map implementation, 'John', 'Emma', and 'Robert' are keys, while the phone numbers are values. When you hash 'John', 'Emma', or 'Robert' using Java's hash function, you get an index where the number should be stored. So, when you need to look up John's number, you just hash 'John' again and directly access his number - an operation that takes constant time regardless of the size of your contact list.
To implement a Hash Map in Java, you first import java.util.HashMap. Initiation of a Hash Map in Java gets done with:
HashMapcontacts = new HashMap ();
Adding elements (names and phone numbers in this case) to the Hash Map can simply be done using:
contacts.put("John", 9876543210); contacts.put("Emma", 1234567890); contacts.put("Robert", 1111222233);
Here, each key-value pair is enclosed in parentheses with a comma separating the key and the value. 'put' is a built-in Java method for adding elements into a Hash Map.
Understanding Syntax of Hash Maps in Java
Using Hash Maps in Java entails understanding the HashMap class's basic methods. These include the .put(Key, Value) method for adding elements, .get(Key) method for retrieving values, .remove(Key) method for deleting elements, and .containsKey(Key) and .containsValue(Value) methods for checking if a certain key or value exists in the map.
Method | Description |
---|---|
.put(key, value) | Adds a key-value pair to the Hash Map. If the key already exists, this method updates the associated value. |
.get(key) | Returns the value to which the specified key is mapped, or null if the map contains no mapping for the key. |
.remove(key) | Removes the mapping for a key from this map if it is present. |
.containsKey(key) | Returns true if this map contains a mapping for the specified key. |
.containsValue(value) | Returns true if this map maps one or more keys to the specified value. |
For instance, to check if John's number exists in our contacts, and if it does, to get and display it, we could write:
if (contacts.containsKey("John")) { System.out.println("John's number is " + contacts.get("John")); } else { System.out.println("Number not found."); }
This code first checks if 'John' exists as a key in our Hash Map 'contacts' using .containsKey(). If true, it retrieves and outputs the number using .get(); if false, it outputs a 'Number not found.' message. Such syntax helps to manipulate and use the stored data efficiently.
It's essential to manage possible hash collisions - when hashed keys end up with the same index. Java's HashMap class uses something called "Separate Chaining" for this. If a collision happens - if two 'keys' hash to the same 'bucket', each 'bucket' contains a linked list of elements, and you simply append the new
Delving into Hash Maps in Python
Hash maps in Python (commonly referred to as dictionaries) are a powerful tool that any aspiring Python programmer should master. They are especially useful because of their efficient speed and simplicity. Let's delve into how they are implemented in Python and understand their syntax.
Insight into Hash Maps Python Implementation
In Python, hash maps are implemented using the built-in dictionary data type. The dictionary, or dict for short, stores key-value pairs and provides efficient operations to access, add, or remove data. Python's dictionary is an excellent example of a hash data structure where a hash of the key is computed to provide a quick lookup of the data associated with the key.
The underlying strategy of Python's dictionary involves an array and a hash function. The keys in the dictionary are 'hashed' using this function, generating a unique integer as an array index. Then, the respective value is stored at this array index. This unique relationship allows for efficient data retrieval.
In Python, a hash map or a dictionary is a mutable, iterable, and unordered collection of key-value pairs where the key must be unique and immutable.
- Mutable: This means that you can change, add, or remove items after the dictionary has created.
- Unordered: The items in a dictionary do not have a defined order, they do not have indexes, and the order can change over time.
- Iterable: Dictionaries are iterable objects, allowing iteration over each item in the dictionary.
Suppose you want to create a dictionary in Python keeping records of student names (keys) and their respective grades (values). This can be achieved as:
grades = {'John': 'A', 'Emma': 'B', 'Robert': 'A+}This creates a dictionary where students' names are the keys and their grades are the values.
Notably, the grade book can be updated, and new students can be added easily due to its mutable property. Python also has built-in dictionary methods that enable the modification of dictionaries, like the update() method used for adding new items to the dictionary.
grades.update({'Oliver': 'B+'})
This line of code would add a new student 'Oliver' with the grade 'B+' to the dictionary. Similarly, grades can be updated for an existing student.
While Python's dictionaries provide constant-time scalability O(1), they manage any potential collision scenario using a method called 'open addressing'. Whenever a hash collision occurs - when two keys result in the same hash index - Python's dictionaries resolve this by finding the next available slot. This feature makes Python's dictionary implementation robust and fast.
Comprehending Syntax of Hash Maps in Python
In Python, dictionaries or hash maps are easy to use with a simple and straightforward syntax. A dictionary is formed with a pair of braces {} containing key-value pairs separated by a colon. A comma separates each key-value pair. The key and value can be of any data type, but the key should be an immutable type, like a string or number.
Python provides built-in methods to manipulate and access data within dictionaries. Some commonly used ones are:
Method | Description |
---|---|
.get(key) | Returns the value for the given key if it exists in the dictionary. |
.keys() | Returns a new object that displays a list of all the keys. |
.values() | Returns a new object that displays a list of all the values in the dictionary. |
.items() | Returns a new object that displays a list of the dictionary's key-value tuple pairs. |
.update({key:value}) | Inserts the mentioned key-value pairs in the dictionary. |
.pop(key) | Removes and returns an element from a dictionary having the given key. |
For instance, if you need to extract and display all keys and values from the grades dictionary, it could be done via:
print("List of students: ", grades.keys()) print("List of grades: ", grades.values())
This would print all the student names followed by their respective grades. Grasping the syntax of a hash map in Python is a worthwhile endeavour as it plays a pivotal role in data analysis, machine learning, and many other fields where Python is used extensively.
Hash Maps - Key takeaways
Hash maps are a type of data structure that implement map interfaces, storing pairs of data- keys and corresponding values.
The main components of hash maps: Key (a unique identifier for a piece of data), Value (the specific data associated with a key), and the Hash Function (this function takes the input (key) and returns an integer which is then used as an index to store the corresponding value).
Hash map implementation refers to the steps and methods used in putting this data structure into action. It begins with creating an empty hash map, defining the hash function, inserting keys and values, addressing possible collisions, and finally accessing or removing values.
Hash map syntax is the code format used to construct and operate hash maps; this syntax can vary depending on the programming language being used.
In Java, the HashMap class, part of the Java Collections Framework, implements the Map interface and uses a hash table as an underlying data structure. Hash maps in Python, also known as dictionaries, provide built-in methods for manipulating and accessing data, including .get(key) (returns value for the key), .keys() (returns all keys), .values() (returns all values), .items() (returns all key-value pairs), .update({key:value}) (inserts key-value pair), and .pop(key) (removes and returns element with given key).
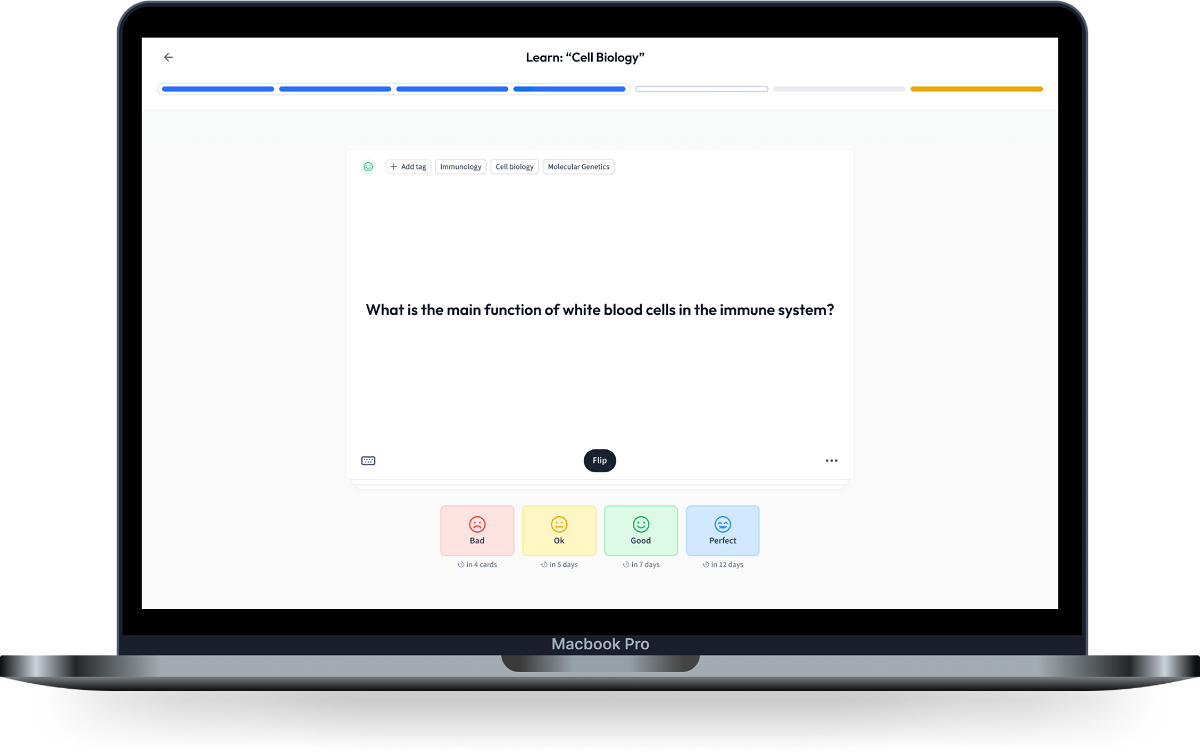
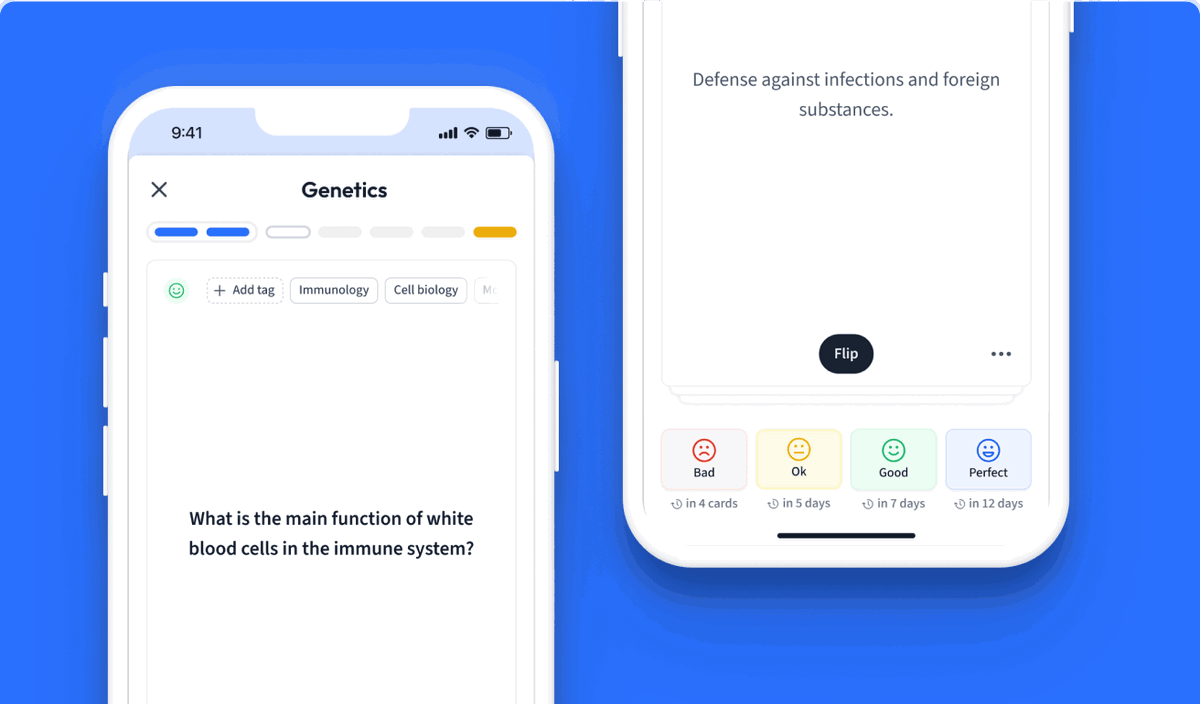
Learn with 15 Hash Maps flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Hash Maps
What is a hash map?
A hash map, also known as a hash table, is a data structure that implements an associative array, a structure that can map keys to values. It uses a hash function to compute an index into an array of 'buckets' or 'slots', from which the desired value can be found. Ideally, the hash function will assign each key to a unique bucket, but most hash table designs employ an imperfect hash. This allows it to achieve efficient constant-time average complexity for search, insert and delete operations.
How do hash maps work?
Hash maps work by using a hash function to compute an index into an array of buckets or slots, from which the desired value can be found. Key-value pairs are stored in these slots, and the key is used to generate a unique hash that indicates where the pair is stored. Consequently, searching for, inserting, or deleting data becomes a rapid process, as it can instantly navigate to a slot rather than having to check each one. However, there's a potential for 'collisions', where different keys produce the same hash, which hash maps solve using various methods like chaining or open addressing.
What is a hash map in python?
A hash map in Python, also known as a dictionary, is a data structure that pairs keys to values, allowing efficient retrieval of the value when given the key. It operates via a hashing function, which transforms the key into a hash, a unique value corresponding to its respective value in the collection. This structure is mutable, unordered and allows for fast data access. It provides a high-speed, flexible mechanism for storing and managing data in Python.
What is hash mapping in java?
Hash mapping in Java is a technique used for storing and retrieving data from a collection. It employs a hash function to compute an index into an array of buckets or slots, to locate the desired data. Hence, it allows you to store key-value pairs and access them efficiently. The Java platform provides the 'HashMap' class from its collection framework for implementing this in the form of a hash table data structure.
How to implement a hash map?
To implement a hash map, you first create an array of a certain capacity to store the values. Then, you create a hashing function that converts keys into array indices. When a new key-value pair comes in, apply the hashing function to the key to get the array index, and store the value at that index. If a collision occurs (two keys produce the same hash), resolve it using a method such as chaining, where each array element maintains a list of all elements that hash to the same index.
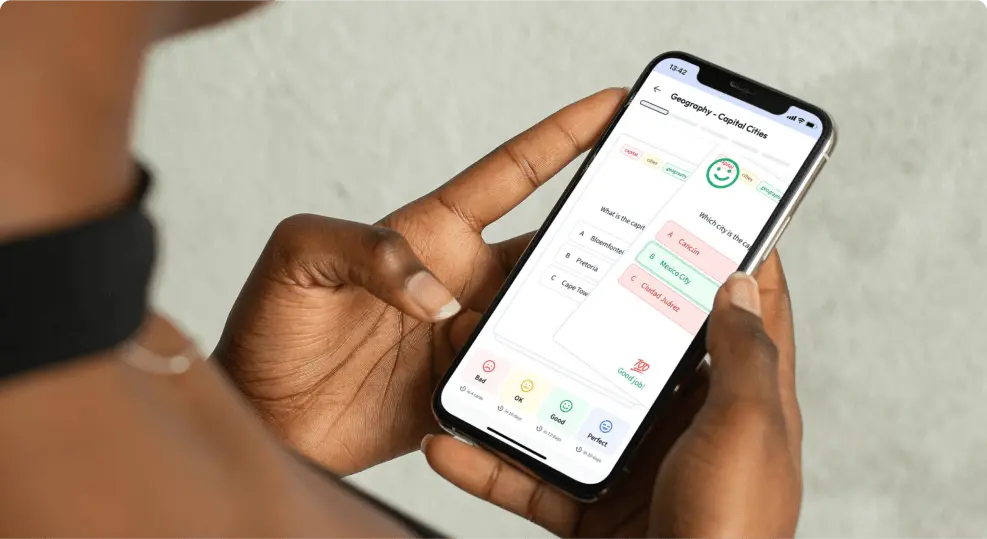
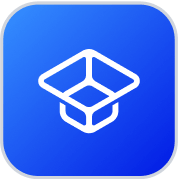
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more