Understanding Bloom Filters
Bloom Filters are an integral part of Computer Science, especially in the realm of data structure. A Bloom Filter is basically a data structure that's used to identify whether an element is a member of a set or not.A Bloom filter is a data structure designed to tell you, rapidly and memory-efficiently, whether an element is present in a set.
Fundamentals of Bloom Filters
To dive deeper into the topic, you'd need to understand that a Bloom Filter operates by using a bit vector of m bits, initially set to zero. It also uses k different hash functions, each of which maps or hashes some set element to one of the m bit positions with a uniform random distribution.Bit Vector: A bit vector is an array data structure that compactly stores bits.
Breaking Down Bloom Filters Technique
In the inner workings of the Bloom Filter technique, it treats each item or element independently. By this, you'd hash the item into several different buckets. It should be explained, of course, that every bucket is just one bit in the bit vector or array.procedure add(item) for i = 1 to k bucket = hash_i(item) bit_array[bucket] = 1 endOne interesting part about the inner workings of this technique is the checking if an item is in the Bloom Filter. The item is hashed in the same way as outlined above, but this time, if any of the buckets (the bits in the bit vector) aren't set to 1, you conclude that the item isn't in the set.
procedure contains(item) for i = 1 to k bucket = hash_i(item) if bit_array[bucket] = 0 return "no" end return "maybe"
Bloom Filters Examples
Bloom Filters are used in various applications. Here are a few real-world instances where the Bloom Filter data structure becomes extremely useful:- In Web browsers for safe browsing: Bloom filters are used to check if a URL exists in a list of malicious URLs.
- In Database systems: Bloom filters are utilised to prevent unnecessary disk reads when searching for an item.
- In Distributed systems: Nodes in the network can use Bloom Filters to check if a certain object exists in the cache of another node, which is especially helpful to reduce network usage.
Delving into Bloom Filtering
As you know, Bloom Filters are a probabilistic data structure, often wielded for checking membership within a set. They're capable of confirming that an item is not in the set, and if an item could likely be in the set. Their strength lies in rapidity and a compact use of memory, making them especially useful for operations with large datasets.A probabilistic data structure: A data structure that provides an approximate solution, under uncertain conditions, with a calculable rate of error.
Bloom Filtering in Big Data: An Overview
As Big Data continues to grow in volume, variability, and velocity, the need for efficient data processing techniques becomes paramount. Bloom Filters present a uniquely reliable and efficient approach. They are effectively applied when dealing with vast amounts of data that need to be processed efficiently, particularly when the goal is to check the membership of an element in a set. A key attribute of Bloom Filters in Big Data applications is its space efficiency. A Bloom Filter uses a small fixed space relative to the size of the data set even as the number of elements grow. This fixed size is attributed to the bit vector - the core structure of a Bloom Filter. Another significant advantage of using Bloom Filters in Big Data is the time efficiency. Querying for an element’s existence in a set takes a constant amount of time, regardless of the set's size. That's because Bloom Filters use a constant number of bit operations. However, it is equally important to acknowledge the limitation of Bloom Filters in Big Data scenarios. The possibility of false positives can escalate if not carefully managed. Remember the probability formula for false positive \( P \): \[ P = \left(1 - e^{kn/m}\right)^k \] To minimise false positives:- You can maximise the size of bit array \( m \), but it's space consuming.
- You can increase the number of hash functions \( k \), up to a certain point. Beyond that point, it increases the false positive rate and slows down the process.
Real-World Applications of Bloom Filtering
Taking you further into the world of Bloom Filters, let's explore the breadth of real-world application scenarios for this fascinating data structure. Bloom Filters are a fundamental component in database systems. The way databases utilise Bloom Filters is to avoid expensive disk lookups for non-existent rows or keys. By checking if the database row or key exists in the Bloom Filter before the lookup, they can avoid unnecessary disk I/O operations, saving processing time. Even renowned tech giants like Google make use of Bloom Filters in their applications. Google's BigTable, a distributed storage system, uses Bloom Filters to reduce the disk reads for non-existent rows or columns, reducing the latency and increasing the performance of their storage system. Moreover, network routers also make efficient use of Bloom Filters. In scalable multicast routing (SMR), network routers use Bloom Filters to encode the multicast forwarding information. This method saves memory space in the router and reduces the computation overhead for multicast forwarding. In Bioinformatics, Bloom Filters are employed in the de novo genome assembly. They come in handy to remove redundancies in a set of k-length substrings of DNA reads, reducing the memory usage for genome assembly algorithms. Bloom Filters have also found applications in blockchain-based cryptocurrencies like Bitcoin. They allow the Bitcoin client to download only a subset of the transaction set (specific to the user) in block headers rather than downloading the whole blockchain. From the breadth and variety of Bloom Filter applications, you can start to appreciate the broad capability and efficiency of this data structure in different contexts. It underlines the power of choosing the right data structures - it's not just theoretical, but practical and impactful in the real world.Exploring Compressed Bloom Filters
While traditional Bloom Filters are efficient by design, the advent of Compressed Bloom Filters takes it one step further. They are a variant of the classic Bloom filter that have been adapted to use even less memory than their predecessor, pushing data efficiency to the next level.Advantages of Compressed Bloom Filters
Beyond the benefits already offered by conventional Bloom Filters, Compressed Bloom Filters come with an added layer of advantages. - Minimal memory usage: Compressed Bloom Filters use significantly less memory than standard ones. This is particularly useful when operating with minimal storage space or transmitting the filter across a network. - Reduced false positives: The reduction in space does not compromise the reduced false positive rate of Bloom Filters. Compressed Bloom Filters maintain, and in some cases even manage to lower, the rate of false positives. - Efficient membership queries: Like classic Bloom Filters, they still provide a time-efficient means of checking for an element's membership in a dataset. However, decompressing the Compressed Bloom Filter may increase the time complexity slightly. The introduction of compressed Bloom Filters in the database and network systems has revolutionised the way data scientists and engineers handle large datasets. However, the advantages of compressed Bloom Filters do not come without a cost. A practical consideration here is that this method is more CPU-intensive because of the required compression and decompression. The compression and decompression processes can slow down membership queries slightly when compared to traditional Bloom Filters.Implementing Compressed Bloom Filters
The algorithmic implementation of Compressed Bloom Filters begins quite similarly to the basic form. You construct a Compressed Bloom Filter as you would a standard one and then you apply a compression algorithm. To add an item to the filter:procedure add(item) for i = 1 to k index = hash_i(item) bit_array[index] = 1 end compress the bit_arrayThe "compress the bit_array" step utilises a compression algorithm, which varies based on implementation. Popular choices include Run-Length Encoding (RLE) and Burrows-Wheeler Transform (BWT), among others. When querying for an item within the filter, you'll need first to decompress the filter and then proceed as with a classic Bloom Filter:
procedure contains(item) decompress the bit_array for i = 1 to k index = hash_i(item) if bit_array[index] = 0 return "no" end return "maybe"The decompression process might introduce additional latency to the query process, but it's often a reasonable trade-off for the memory efficiency gained. It's crucial to note that current modifications in Compressed Bloom Filters are mainly centred on the compression strategy, focusing on optimising the time-memory trade-off. With advances in data science, it is rational to expect that future improvements would focus on tackling the CPU-Intensive nature of compressed Bloom Filters, making them even more potent for handling large data sets.
Hash Functions for Bloom Filters
Integral to the functioning of a Bloom Filter is the use of hash functions, which serve to map the inserted data to positions within the Bloom Filter's bit array. The use of the right hash functions is critical to the efficiency and accuracy of a Bloom Filter.Understanding Hash Functions in Bloom Filters
Hash functions within Bloom Filters have a vital role. They spread the representation of data uniformly across the bit array. To put it simply, when data is inserted into the filter, the hash functions generate multiple indices, each corresponding to a position in the filter's bit array. Each element to be checked for membership or to be added to the set is passed through these hash functions, generating a specific hashed output. This output then maps onto the Bloom Filter bit array. The more hash functions used, the more bits are set for a specific element in the Filter. Factors to consider when constructing hash functions for Bloom Filters include:- The Uniformity of the hash functions: The distribution of hashed values should be as uniform as possible. Non-uniform distribution might cause the filter to have more false positives.
- Independence of the hash functions: Each hash function should be independent of the other. Correlation between hash functions may lead to clustering within the bit array, thereby increasing the chances of false positives.
- The Speed of computation: Ideally, hash functions should be quick to compute. A crucial feature of Bloom Filters is their efficiency, and slow hash functions can significantly slow this down, thereby limiting the utility of the filter.
Hash Functions: A function that takes a data (key) and returns a fixed-size set of characters, which is typically a hash code. The output is used as an index to store the corresponding key-value pair in the database.
Utilisation of Hash Functions in Bloom Filters
To further grasp how essential hash functions are to Bloom Filters, let's dissect their utilisation in more detail. Primarily, when an element is to be added to the filter, it's passed through 'k' different independent hash functions - each producing a unique hashed output. Each output corresponds to a specific index position within the Bloom Filter bit array. Every such index is set to 1. Here is a simple pseudocode to demonstrate this process:procedure add(element) for i = 1 to k index = hash_i(element) BF[index] = 1 endThe 'contains' operation also makes use of these hash functions to check an element's membership within the filter.
procedure contains(element) for i = 1 to k index = hash_i(element) if BF[index] = 0 return "no" end return "maybe"Upon querying an element, it's passed through the same set of hash functions, and the return indices are checked in the Bloom filter. If all the indices contain 1, the function will return 'maybe', otherwise 'no'. From this exploration, you can see how Bloom Filters strategically employ hash functions to optimise their space and search efficiency, while trading-off with a small and manageable error factor of false positives. The speed, independence, and uniformity of the selected hash functions, therefore, become critical in optimising the utility and impact of Bloom Filters.
The Advantages of Bloom Filters
Despite the introduction of more modern data structures, Bloom Filters continue to be highly relevant in computer science due to their unique advantages. They offer a compelling trade-off between memory usage, runtime, and accuracy, which makes them an invaluable tool when dealing with large datasets.Why Use Bloom Filters?
As a probabilistic data structure, Bloom Filters provide an efficient way to test whether an element is part of a set. At first glance, this might seem like an easily solved problem. However, when handling large datasets or working under constraints of reduced memory or limited processing power, a data structure that accomplishes this with both high speed and memory efficiency becomes indispensable. For instance, consider a scenario where you need to handle a database of several billion records. Using traditional data structures such as trees or hash tables to store this information can be cost-prohibitive in terms of memory. Sure, you could use disk storage, but this would significantly slow down your query speed. Here is where Bloom Filters save the day. They provide a memory-efficient method to perform membership queries, using a very compact representation of the dataset. The beauty of a Bloom Filter is that it handles such large datasets with relatively small amounts of memory. How? By accepting a minute trade-off - a small probability of false positives. That is, occasionally, it might tell you that an element is in the set when it actually isn't. However, it will never tell you that an item is not in the set if it truly is - so there are no false negatives. A Bloom Filter achieves this by using an array of binary values and multiple hash functions. Each element in your set is hashed multiple times. The binary positions in the array equivalent to these hash outputs are then set to 1. To then check if an item is in the set, the item is again hashed, the corresponding array positions are checked, and if all positions are 1, the filter will return that the item "might be" in the set. If any are 0, it will return that the item is definitely not in the set. In addition to saving memory and reducing the time complexity, another advantage is that deletions do not affect the performance of a Bloom Filter. In fact, Bloom Filters do not support element deletion operation. This ensures that the false positive rate remains as is over time – it does not increase with deletions.The Strengths and Benefits of Bloom Filters: An Examination
Diving deeper into the utilities of Bloom Filters, a series of key strengths emerge which truly sets them apart in the world of data structures. 1. Space Efficiency: The most noticeable strength of a Bloom Filter is its highly space-efficient solutions. When dealing with large data sets, reducing memory usage is often crucial, and this data structure provides an effective approach. The memory required by a Bloom Filter to store data grows linearly with the number of elements and hash functions, but far more slowly than the growth observed with traditional data structures like arrays or linked lists. With just a handful of bits per element, a Bloom Filter can effectively handle even vast databases. 2. Time Efficiency: Another significant benefit lies in its time efficiency. Membership queries in a Bloom filter are remarkably fast, with time complexity of \(O(k)\) where \(k\) is the number of hash functions. Because each of these can be computed in parallel, the necessary operations to complete a query happen really quickly. In contrast, the time complexity to perform queries in most traditional data structures grows with the size of the dataset. 3. No False Negatives: A crucial aspect of Bloom Filters is that they guarantee no false negatives. This means when the filter tells you that an item is not part of the set, you can be 100% sure about it. This is an essential property in situations where missing an actual member of the set could have considerable consequences. 4. Controlled Error Rate: Although Bloom filters allow for a chance of false positives, the rate of these is under your control. By adjusting the size of the bit array and the number of hash functions, you can decrease the false positive rate to a level that is acceptable for your needs. If the expected false positive rate is deemed too high for a given application, it can be lowered by using a larger bit array or more hash functions. 5. Immutable: Lastly, Bloom Filters are immutable. Once elements have been added, they can't be removed from the set. While this immutability might seem limiting, it ensures consistency over time, preserving the initial false positives rate. Through their combination of space efficiency, rapid query times, assured no false negatives, controlled error rate, and immutability, Bloom Filters demonstrate an appealing blend of strengths that make them extraordinarily practical for many computer science applications - especially those handling large-scale data sets.Bloom Filters - Key takeaways
- Bloom Filters are a probabilistic data structure used to check if an element exists in a set. They are efficient in their use of memory and time, making them particularly useful for large datasets.
- In the context of Big Data, Bloom Filters are advantageous as they utilise a small, fixed amount of space regardless of the number of elements in the dataset. The time taken to query an element is constant, irrespective of the size of the data set.
- Compressed Bloom Filters are a variant of the classic Bloom Filter, offering even greater memory efficiency. They offer reduced false positives and efficient membership queries, but they are more CPU-intensive due to the required compression and decompression.
- Hash functions play an integral role in the operation of Bloom Filters. They map the data to positions in the Bloom Filter's bit array. The chosen hash functions need to be uniform, independent, and quick to compute for the Bloom Filter to function efficiently.
- Despite the probability of false positives, Bloom Filters offer unique advantages including a compelling trade-off between memory usage, runtime, and accuracy, proving extremely useful when dealing with large data sets or under constraints of reduced memory or limited processing power.
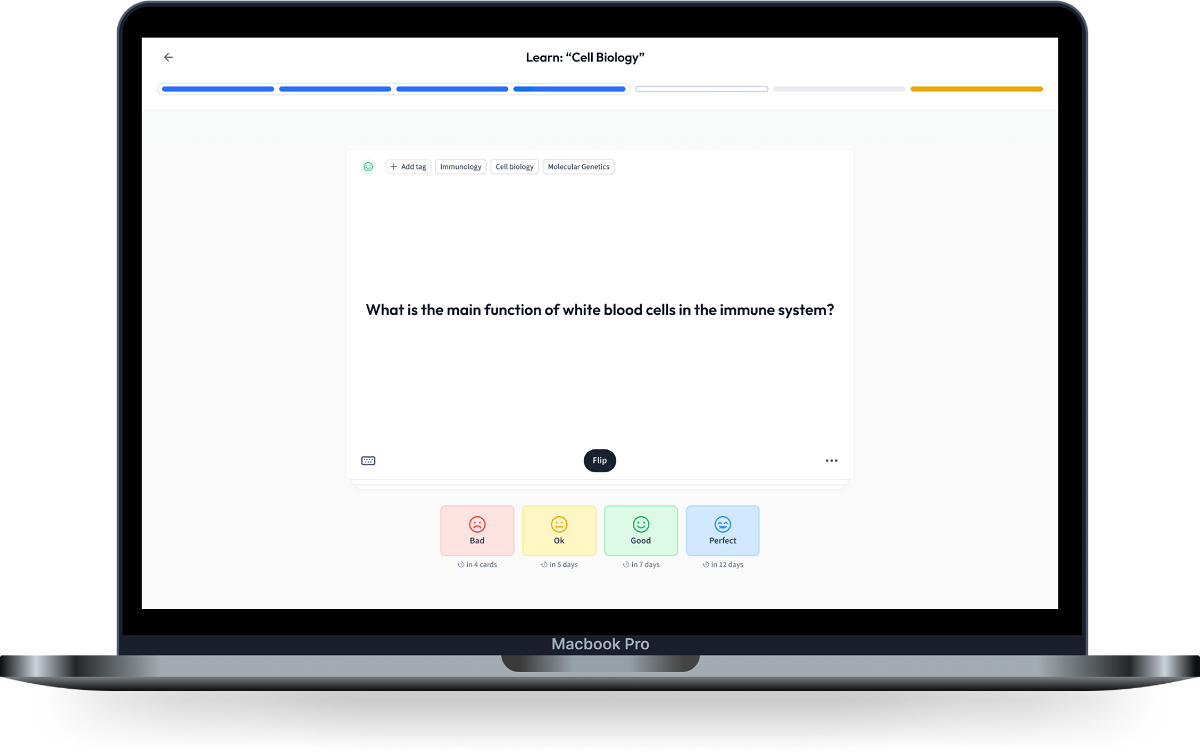
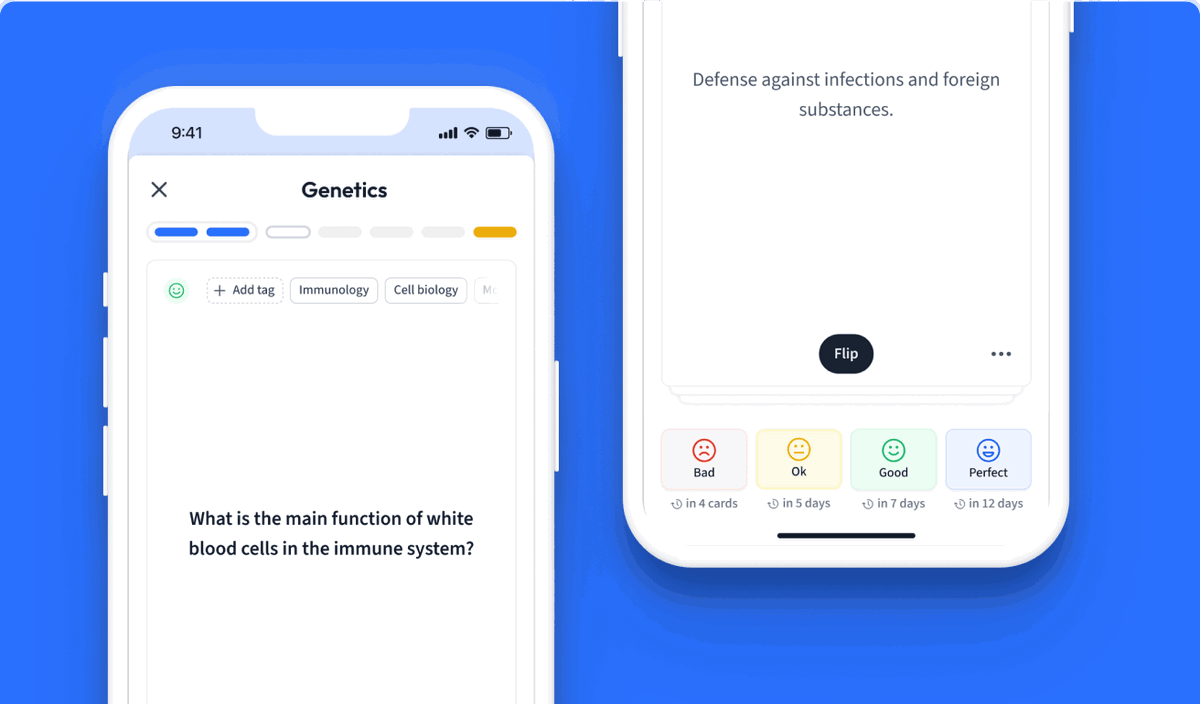
Learn with 15 Bloom Filters flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Bloom Filters
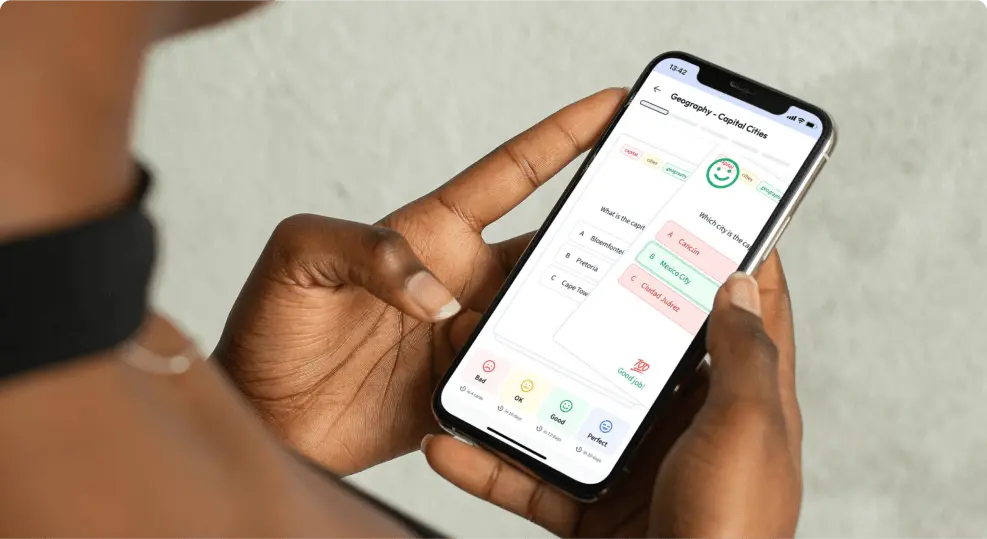
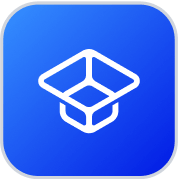
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more