Real-life examples of Binary Trees and their roles in computer science serve to highlight its widespread relevance. Following this, you'll explore the notion of an Inverted Binary Tree, understanding why and how to invert a Binary Tree. The information on Balanced Binary Trees supports your journey towards mastering this concept. Your knowledge will expand into Binary Tree implementation in Python, giving you practical programming skills. Finally, find how to handle Binary Tree data effectively using Python. All this awaits you—let's help you demystify the world of Binary Trees.
Understanding Binary Tree
To kickstart this journey into the realm of computer science, let's first delve into what Binary Tree is - a fundamental data structure used for computer science applications like image processing algorithms and efficient searches.
The Binary Tree is a tree data structure in which each node has utmost two children, referred to as the left child and right child.
Consider the English alphabet. The Binary Tree structure would place all letters ineffectively 'nested' categories. Rooted at the top with 'A', the left child could be 'B', and the right child could be 'C'. The 'B' subtree might have 'D' and 'E' as its children, and so on. This segmentation fosters efficiency in certain types of searches, akin to how an organised bookshelf directs a reader to the correct set of books without having to peruse each book individually.
Essential Properties of Binary Tree
Understanding the key properties of a Binary Tree is necessary for comprehending its significance and application in computer science. Below are some of the most important properties:- Root: The topmost node in the tree.
- Child: Any node, excluding the root, connected upward by an edge.
- Parent: For any 'child' node, the connected node above it, nearer to the root.
- Leaf: A node with no children.
- Subtree: Any node can be viewed as the root, together with children, of a subtree.
- Visiting: Executing some form of operation i.e., print, compute a function at the node.
- Traversing: Visiting the nodes in some order.
- Levels: levels are numbered from the root down. The root is at level one.
Binary Tree Structure Explained
Let's explore the Binary Tree structure more intricately. Its condition stipulates that a node can have two children at the most. Here are a couple of essential terms to expand upon:- Full Binary Tree: This type includes a tree where every node has either zero or two children.
- Complete Binary Tree: In this type, all levels are completely filled, except possibly the last level which is filled from left to right.
In computer science, this Binary Tree structure is widely utilized to structure data for quick searching, quoting and deleting of information. It’s important to note that Binary Trees are so named, not because they necessarily have two children, but because they have two (binary) choices for each child slot - occupied or unoccupied.
Consider a telephone directory. Using a Binary Tree structure, the person you're looking for can be found much faster as with each step, you exclude half the remaining names. On the other hand, a 'brute force' method would consist of scanning through each person's name chronologically, which can be extremely time-consuming.
Node | Left Child | Right Child |
---|---|---|
A | B | C |
B | D | E |
Diving Into Binary Tree Search
Binary Tree Search is a classic computer science algorithm that is used to locate a specific value within a binary tree. The algorithm operates on the principle that for each node, all elements in its left subtree are less than the node, and all elements in the right subtree are greater than the node. Binary Tree Search operates by efficiently halving the search space at each step, redefining it to either the left or right subtree, based on the comparison.
With Binary Tree Search, you could start with the root of the tree (say, the middle element of the array, if that’s how the tree is implemented). If the target value is equal to that of the root, the search is successful. If the target value is less than that of the root, the search continues to the left subtree. Conversely, if the target value is greater than that of the root, the search continues to the right subtree. The process repeats recursively in the relevant subtree until the target value is found or until the search space is exhausted.
A classic real-world example of Binary Tree Search is found in binary search algorithms used by digital dictionaries. Suppose the system has to locate a user-specified word. The program compares the word with the root of the tree (the ‘middle’ word in the dictionary). If the word is found, the search ends. If not, and the word comes alphabetically before the root, the search repeats in the left subtree; otherwise, the search repeats in the right subtree. This process significantly optimises search times in large dictionaries.
Implementing Search in Binary Tree
In the realm of computer science, Binary Tree Search is widely implemented in many programming languages. Let's now deep dive into its implementation:A Binary Search Tree (BST) could be implemented using a linked data structure in which each node is an object that contains key value, satellite data, and attributes left, right, and p, which point to the nodes corresponding to the parent, left child, and right child of the node, respectively.
For simplicity, we may consider the satellite data as a name associated with the key. Here are the steps to searching an element in the Binary Search Tree:
Binary Tree Search in Python
Now, let’s see a practical implementation of Binary Tree Search, using Python, a popular programming language renowned for its clarity, breadth, and depth.Python, like other programming languages, implements Binary Search Tree operations in a straightforward manner, which makes the process simple and easy to understand for students. It has explicit support for binary data structures and powerful native functions that allow you to navigate and modify binary trees with ease.
Here's a Python code snippet showing the implementation of Binary Tree Search:
class Node:
def __init__(self, key):
self.left = None
self.right = None
self.val = key
def search(root, key):
# Base Cases: root is null or key is present at root
if root is None or root.val == key:
return root
# Key is greater than root's key
if root.val < key:
return search(root.right, key)
# Key is smaller than root's key
return search(root.left, key)
In the snippet above, a Node class is created, and the BST search operation is implemented as a function which takes two parameters – 'root' and 'key'. The function tests whether the currently explored node's key matches the searched key. In case of a match, it returns the node.
If the searched key is greater, the function is recursively called on the right subtree, and otherwise, on the left subtree. If the search space is exhausted (i.e., an empty subtree is reached), 'None' is returned signifying the key has not been found in the tree.
Exploring Binary Tree Examples
To understand the concept of Binary Trees better, let's look into real-world applications where Binary Trees play a vital role.Binary Trees are omnipresent in today's coveted computer programs. They are commonly used for designing and implementing various software programs and algorithms. They play a significant role in optimising tasks as they help to reduce the time complexity of an operation and increase the efficiency of the system.
- Search Systems: One of the most widespread applications of Binary Trees is in search systems. For instance, in an online dictionary, Binary Tree Search allows a user to find a word much faster as compared to linear searching.
- Decision Trees: Decision trees, a popular tool in decision analysis, are also a type of binary tree. Each node represents a decision, and the tree’s structure allows for a comprehensive review of the result of each decision path.
- Sorting Algorithms: Binary Trees are also used in sorting algorithms like heapsort that are more efficient than bubble, insertion, and selection sort.
Application | Example |
---|---|
Search Systems | Online Dictionary |
Decision Trees | Decision Analysis |
Sorting Algorithms | Heapsort |
A classic example is a game of Twenty Questions. In this game, one person is thinking of an object while another person is trying to guess what the object is through a series of yes/no questions. The guessing strategy can be modelled as a Binary Tree in which each node is a question and each branch is a possible answer ('yes' on one side, 'no' on the other). By navigating this Binary Tree effectively, the guesser can usually isolate the object in question quite quickly.
Binary Tree Examples in Computer Science
In the field of Computer Science, Binary Trees find numerous applications. Let's delve deep into the core of this subject by discussing a few notable examples where Binary Trees render its benefits.Binary Trees are an essential tool for designing various algorithms and data structures in Computer Science. They prove valuable in applications involving sorting, searching, partitioning and building other data structures such as heaps and hash tables.
- File System Hierarchy: The file system in computer systems is structured in the form of a Binary Tree. Each folder (directory) and file is a node of the tree structure. The Binary Tree's hierarchical property suits naturally for this task given the inherent hierarchy of file systems.
- Expression Parsing: Expression parsing, a crucial aspect of compiler design, deploys Binary Tree. The expression is represented as a Binary Tree structure, allowing the compiler to easily evaluate the expression.
- Network Data Routing: Network routers use a binary space partitioning tree to speed up data access and foster efficient routing in the network backbone.
Applications | Examples |
---|---|
File System Hierarchy | Operating Systems |
Expression Parsing | Compiler Design |
Network Data Routing | Internet Backbone |
An illustrative example to explain Binary Trees in Computer Science can be syntax trees in compilers. In high-level language compilers, the source code's syntax must be checked for correctness. This process results in a parse or syntax tree, which is a Binary Tree.
The source code is divided into basic elements and built into a Binary Tree, which can then validate the code syntax. If the syntax is incorrect, the compiler can easily trace back to the erroneous part using the Binary Tree.
A Guide to Inverting Binary Tree
In the realm of computer science, the concept of inverting a Binary Tree can be intriguing. This process involves swapping the left and right child nodes around for all nodes in the tree. The end result is a mirrored version of the original tree.What is an Inverted Binary Tree?
An inverted Binary Tree, also known as a mirror or a reflexive tree, is a Binary Tree in which the roles of left and right subtrees of every node are reversed. This process means that every parent's left child becomes its right child and vice versa.
The inversion of a Binary Tree is a popular interview question for software development roles, partly because it requires the understanding of tree traversal methods and the application of recursive or iterative techniques.
How to Invert a Binary Tree
Inverting a Binary Tree may seem complex on the surface, but the process is rather straightforward once you understand it. To invert a Binary Tree, one would:- Swap the left child and the right child of the root node.
- Recursively invert the subtrees rooted at the just swapped left and right children.
Consider the binary tree ['A', 'B', 'C', 'D', 'E', 'F', 'G'] where 'A' is the root. The inverted/mirror version of this binary tree would be ['A', 'C', 'B', 'G', 'F', 'E', 'D'] where 'A' remains the root, however, 'B' and 'C' are swapped, then 'D'-'E' and 'F'-'G' (children nodes of 'B' and 'C') are swapped giving us our inverted binary tree.
Inverting Binary Tree in Python
One can accomplish the task of inverting a Binary Tree by using various programming languages. But for simplicity's sake, let's discuss the Python approach.Python, being a popular and versatile language with a clear syntax, is often preferred when implementing and understanding algorithms. In particular, Python's support for recursion makes it ideal for the task of inverting a Binary Tree.
class Node:
def __init__(self, key):
self.left = None
self.right = None
self.val = key
def invert(node):
if node is None:
return
# Swap left and right child nodes
temp = node.left
node.left = node.right
node.right = temp
# Recursive call on subtrees
invert(node.left)
invert(node.right)
In the snippet above, a Node class has been defined to represent Binary Tree nodes, and the Binary Tree inversion operation has been implemented as a function that accepts a node as an argument. The function simply swaps the provided node's left and right child nodes, and then makes recursive calls on both child nodes. If a 'None' node is provided, which happens when a leaf node's child is considered, the function simply returns. This recursively descends the entire Binary Tree structure, effectively inverting it. Keep in mind that an important aspect of understanding tree inversion in Python is knowing how recursion works, as it's one of the basic operational principles of the inversion process.Mastering Balanced Binary Tree
A Balanced Binary Tree is a particular type of Binary Tree that is deeply ingrained in computer science due to its unique and useful properties. The primary attribute that defines a Balanced Binary Tree is the fact that the height of the left and right subtrees of every node differs by at most 1.
This ideal balancement condition ensures optimal performance in operations like insertion, deletion, and search, and therefore, Balanced Binary Trees are used extensively in various data-lookup structures like AVL trees, red-black trees and B-trees.
This balancing constraint is root-deep, meaning it not only applies to the root of the tree itself, but also to all subtrees (i.e., to all nodes viewed as roots). Balancing optimises the tree by minimising its height, contributing to more efficient operations.Suppose you are sorting a list of numbers. Without any specific methodology, you might end up with a skewed Binary Tree, where most values are either on the left or the right, resulting in inefficient sorting or search times. But if the tree is balanced, the elements would be uniformly distributed across both sides, reducing the number of comparisons and swaps necessary, thereby speeding up the entire process.
Balancing a Binary Tree: An Outline
Balancing a Binary Tree is all about ensuring that the tree maintains its balanced state even after insertion or deletion operations. The concept behind it is simple but may become complex during its practical implementation.Typically, after performing an insertion or deletion operation, the Binary Tree is traversed starting from the modified node, moving up towards the root, checking for balance at each step. If an imbalance (difference of heights greater than 1) is detected at a node, a tree rotation operation is performed to balance the node.
- Left Rotation: Performed when the right subtree of a node is heavier (higher).
- Right Rotation: Performed when the left subtree of a node is heavier.
- Left-Right Rotation: A combination of left rotation followed by right rotation. Performed when the left subtree is heavier and its right child contains the imbalance.
- Right-Left Rotation: A combination of right rotation followed by left rotation. Performed when the right subtree is heavier and its left child contains the imbalance.
While rotations can appear complicated, they are a straightforward way to optimise the Binary Tree's structure for faster data lookups. By keeping the tree balanced, the worst-case time complexity for searching stays at \(O(log(n))\), where 'n' is the total number of nodes.
Consider a Binary Tree with root node 'D' with left child 'B' and right child 'E'. 'B' has 'A' as its left child. Now, this tree is imbalanced as the right subtree of 'B' (which is NULL) and its left subtree (rooted at 'A') differ in height by more than 1. Here, a single right rotation at 'B' would suffice to balance the tree, resulting in 'A' becoming the left child of 'D', 'B' the left child of 'A', and 'E' remaining as the right child of 'D'.
Balancing Binary Trees in Python
The balancing operation of a Binary Tree is an essential task in many applications. Implementing this task in Python allows for efficient and clean code given Python’s brevity and versatility.In the Python implementation of Binary Tree balancing, a check for balance (difference in the heights of left and right subtrees) is made after every insertion or deletion operation.
If an imbalance is found, an appropriate rotation operation is performed based on the type of imbalance. Python's support for recursion and object-oriented programming facilitates this task.
Here’s a short Python code snippet showcasing this:
class Node:
def __init__(self, key, height=1):
self.left = None
self.right = None
self.val = key
self.height = height # height of node added
def insert(node, key):
#...insertion code...
# Update height of node
node.height = 1 + max(getHeight(node.left), getHeight(node.right))
# Get balance to check if tree became unbalanced
balance = getBalance(node)
# Perform rotations (4 cases)
# ...rotation code...
In the above-mentioned code, a Node class has been defined equipped with an additional attribute – height, which stores the height of the node.
After every insert operation, the height of the node is updated, and the balance is checked. If the balance exceeds 1, appropriate rotations are performed based on whether the imbalance is in the left, right, left-right, or right-left subtrees. This iterative process thereby ensures that the Binary Tree always remains balanced.
Implementing the Binary Tree in Python
Delving into the world of Binary Trees, mastering this concept in Python is enthralling yet practical. Python, owing to its ease of use and readability, offers a seamless platform to understand, implement and experiment with Binary Trees.A Binary Tree can be implemented in Python using node-based linked data structures. Each node contains a value, along with information pointing to its left and right child nodes.
class Node:
def __init__(self, data):
self.left = None
self.right = None
self.data = dat
Each node carries data and has links to its left child and its right child. The tree starts with a root node, branching out to its children recursively. To add a node, we can create a recursive function that will track down the tree based on the value it wants to add compared to the node it is currently considering: def insert(root, data):
if root is None:
return Node(data)
else:
if data < root.data:
root.left = insert(root.left, data)
else:
root.right = insert(root.right, data)
return roo
To print out the data in the Binary Tree, there's an order known as "in-order traversal": Left --> Root --> Right. This is a method to traverse the tree and could be implemented in Python like so: def inOrderTraversal(root):
if root:
inOrderTraversal(root.left)
print(root.data)
inOrderTraversal(root.right)
With just these simple functionalities, you now have a basic Binary Tree in Python at your disposal!Suppose you want to create a binary tree to represent students' grades where the root is 'C'. A grade of 'B' would branch left, 'D' would branch right, and so on. Using the above implementation, this can be easily achieved in Python. By calling 'insert' with the tree root and the grade, you can build this Binary Tree. Then use 'inOrderTraversal' to fetch the grades in order: 'A', 'B', 'C', 'D', 'E', 'F'.
Python Binary Tree: Simple Steps to Get Started
If you're brand new to the world of Binary Trees and Python, do not fret. Here are some simple steps to implement a Binary Tree and get started:- Create a Node class: Each node of the Binary Tree will be an instance of this class, containing a 'data' attribute, and two nodes 'left' and 'right' representing the left and right children respectively.
- Design the 'insert' function: This function takes the root of a Binary Tree and some data. It inserts the data into the Binary Tree rooted at 'root', obeying the Binary Search Tree property, and returns the root.
- Create a 'traversal' function: This function prints the nodes' data in various orders. The function takes a root, traverses the Binary tree rooted at 'root' in in-order (left --> root --> right), pre-order (root --> left --> right), or post-order (left --> right --> root), and prints the data.
Handling Binary Tree Data using Python
Once you have understood the basic implementation of the Binary Tree, it opens gates to a whole lot of operations and manipulations of Binary Trees. Be it balancing, rotating, or Mirroring the Binary Tree; you can do it all. To maintain the Binary Tree as an efficient, ordered data structure, we need to ensure that the tree remains balanced, primarily when we add or remove nodes. Python provides several built-in functions to simplify these tasks.
We discussed binary tree balancing and rotation in detail in previous sections. The key is to ensure that the maximum difference in the height of the left and the right subtrees of any node is one. Observing this rule will keep the tree balanced and optimise the time complexity of searching, insertion, and deletion operations to \(O(log(n))\). To mirror or invert a Binary Tree, again Python's straightforwardness comes into play.
Here's a neat way to invert a Binary Tree:
def invert(root): if root: root.left, root.right = root.right, root.left invert(root.left) invert(root.right) return root
In this Python function, at every node encountered, its left and right children are swapped. The function then recursively inverts the left and right subtrees. Couldn't get any simpler, right?
Python's simple and consistent syntax lends itself exceptionally well to expressing Binary Trees intuitively and compactly. With Python, tasks like node representation, tree traversal, and subtree comparisons can be coded explicitly and succinctly, allowing you to focus on understanding the concept rather than parsing the code.
Binary Tree - Key takeaways
A Binary Tree is a fundamental data structure in computer science where each node has utmost two children: a left child and a right child.
A Binary Tree can organise data effectively for quick retrieval and is used in many applications. Examples of Binary Trees in real life include search systems like online dictionaries, decision trees in decision analysis, and sorting algorithms like heapsort.
In Computer Science, Binary Trees are used in file system hierarchies, expression parsing in compiler design, and in network routers for data routing.
A Binary Tree can be inverted or mirrored by swapping the left and right children of all nodes. This process is used in several computer science applications and is a common interview question for software development roles.
A Balanced Binary Tree is a Binary Tree in which the height of the left and right subtrees of every node differs by at most 1. It is used extensively in data lookup structures such as AVL trees, red-black trees, and B-trees.
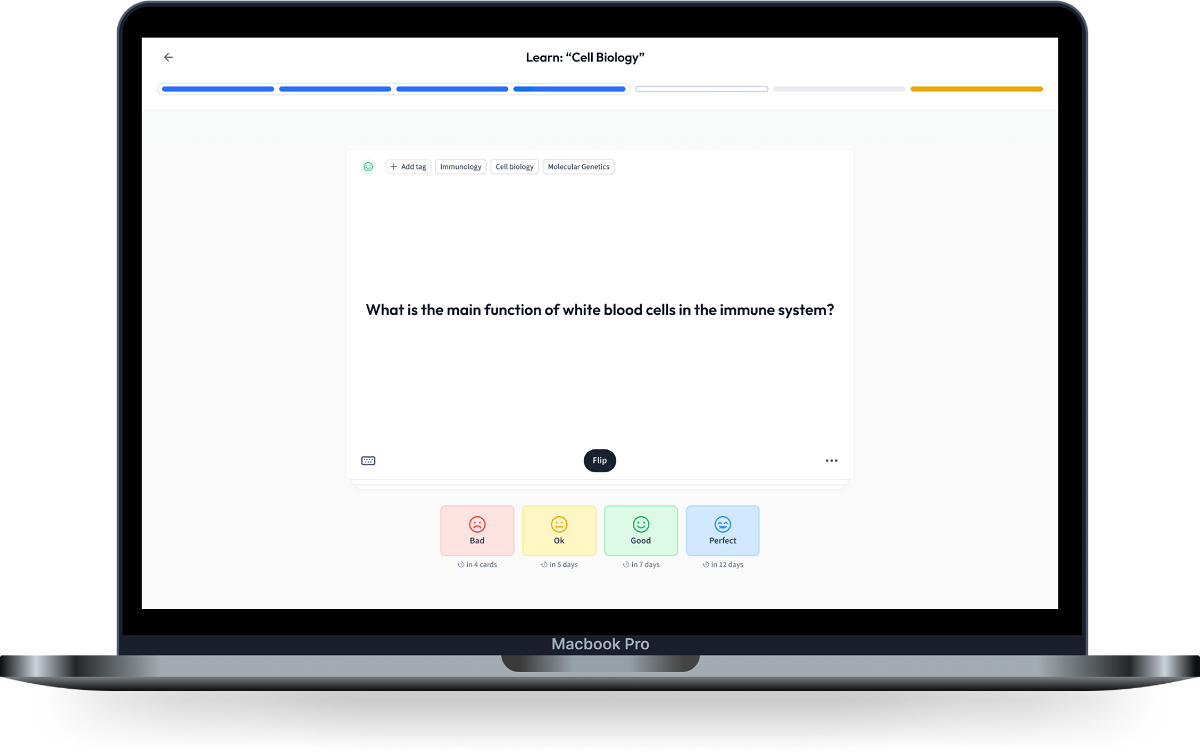
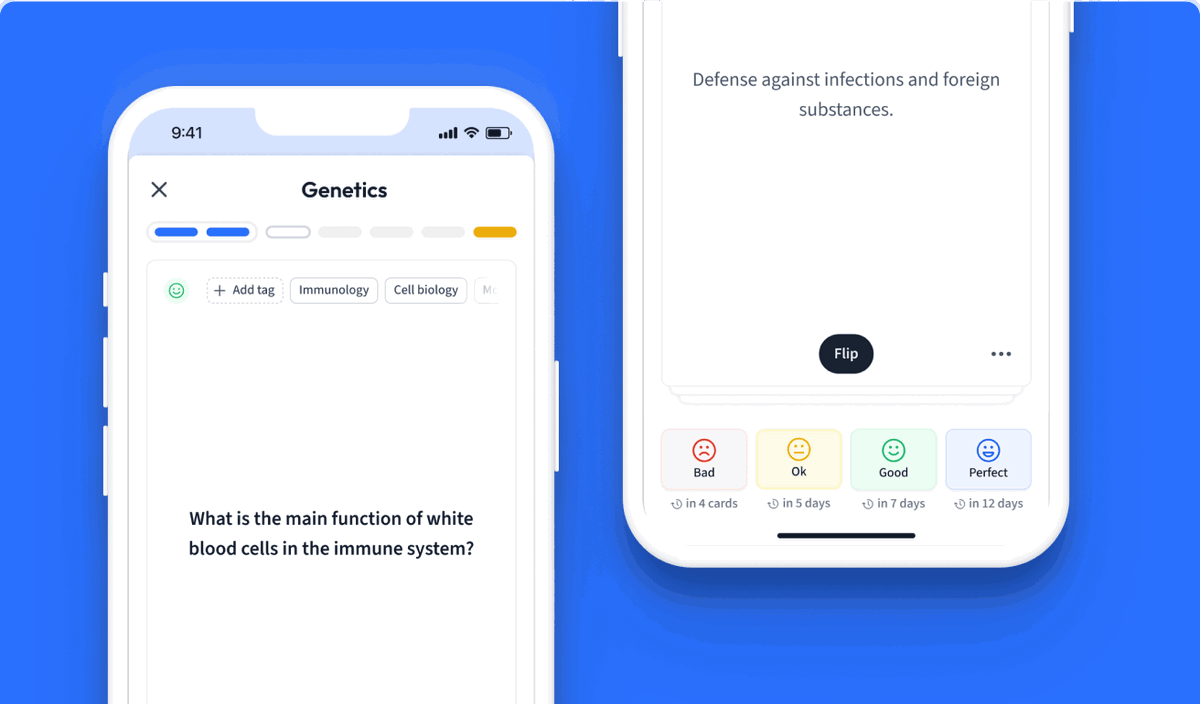
Learn with 6 Binary Tree flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Binary Tree
What is a binary tree?
A binary tree is a type of data structure in which each node has at most two children, typically referred to as the left child and the right child. It is used in many areas of computer science, including algorithm design and efficient searches. The topmost node is known as the root, while the nodes with no children are called leaves. Unlike arrays, linked list, stack and queues, which are linear data structures, trees are hierarchical data structures.
How to make a binary tree?
To create a binary tree, start by creating a node structure with a data element and two pointers that point to the left and right child respectively. The root node is the initial node. To add elements, compare the data in the node. If it's less than the current data, add the node to the left pointer. If it's more, add to the right. Make sure to check the tree is balanced to ensure efficient operations.
How do you invert a binary tree?
Inverting a binary tree involves swapping the left and right child nodes in each parent node. Start from the root, then recursively swap the left and right children of this node. Continue this process until you have traversed through all nodes of the tree. As a result, the bottom-most nodes will become the root of the subtrees.
How does a binary tree work?
A binary tree works by organising data in a hierarchical structure, where each node can have a maximum of two children, referred to as the left child and the right child. It starts from a single top node, known as the root. For every node, all elements in its left subtree are less than the node and all elements in its right subtree are greater. This structure facilitates efficient search and sort operations.
How to search a binary tree?
To search a binary tree, you typically use a traversal technique (like in-order, pre-order or post-order traversal). Starting from the root node, you compare the target value with the current node's value. If the target value is less, you navigate to the left child. If it's more, you navigate to the right child. The process is then repeated until the target is found or we have traversed all nodes without finding the target.
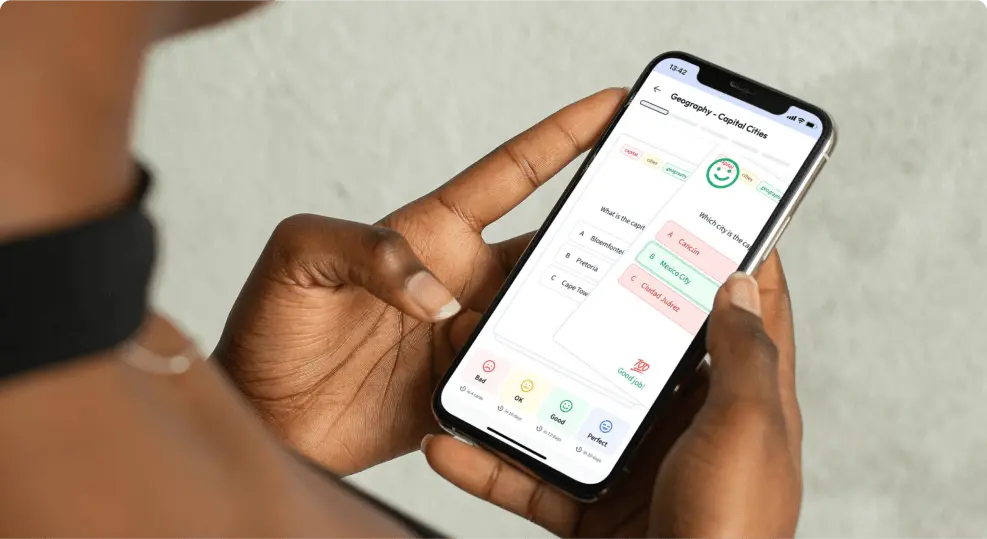
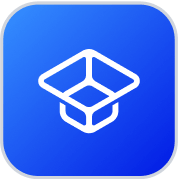
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more