Furthermore, learn about the implementation of Priority Queue in two of the most popular programming languages: Java and Python. Shed light on the intricacies of Priority Queue in Java, and get invaluable beginner-friendly insight into its usage. Then, navigate through the concept of Priority Queue Python implementation. Gain the knowledge necessary to kick-start your journey with Priority Queue in Python. This comprehensive guide affords a unique blend of theory and practical wisdom that aids in understanding and mastering the complex concept of Priority Queue in Computer Science.
Introduction to Priority Queue
Entering the world of computer science, you will encounter various forms of data structures, each with its unique functionalities. Among these structures is the Priority Queue, which plays a vital role in different programming and algorithm contexts.
Definition and Basics of Priority Queue
A Priority Queue is a particular type of data structure in which every element is assigned a priority. Elements with higher priorities are dequeued before those with lower ones. In the event of similar priorities, elements are dequeued based on their existing order in the queue.
Consider a real-life scenario: In a hospital, patients are attended to based on the severity of their conditions, not just their arrival time. This is a classic real-world demonstration of a Priority Queue.
There are two main types of Priority Queues:
- Ascending order Priority Queue (smallest element has the highest priority)
- Descending order Priority Queue (largest element has the highest priority)
The decision on which type to use depends solely on the specific requirements of the project you're handling.
Let’s consider an example. If you are designing a system for a restaurant to manage their food orders, you might use a Priority Queue. If two customers order at the same time, but one order is a single sandwich and the other is a three-course meal, the sandwich order, being quicker to prepare, should be prioritized.
Importance of Priority Queue in Computer Science
Priority Queues are vital for their efficiency in various applications. Below are a few reasons why they hold so much weight in computer science:
- Firstly, Priority Queues are used in certain types of sorting algorithms, such as heap sort.
- Secondly, they are beneficial in graph algorithms like Dijkstra's and Prim's.
- Thirdly, they are applicable in systems related algorithms like load balancing and interrupt handling.
To better understand the power of Priority Queues in action, consider an operating system that uses Priority Queues to determine which tasks to execute first. The tasks are assigned priorities; tasks with urgent needs have higher priority over less crucial ones. This priority system ensures that the most critical tasks are addressed promptly, leading to increased efficiency.
In conclusion, understanding the Priority Queue concept and how to implement it effectively offers a significant boost to your problem-solving skills as an aspiring computer scientist or seasoned programmer. Plus, it adds to your ability to efficiently handle tasks in a real-world programming environment.
Algorithm for Priority Queue
In computer science, you deal with functions and routines that require the execution of tasks in a specific order based on their urgency or importance. That's where the algorithm for Priority Queue comes into play.
Essential Elements of an Algorithm for Priority Queue
The algorithm for a Priority Queue operates on several crucial elements, ensuring that tasks with the highest priority are tackled first.
These elements include the queue, priority functions, dequeuing, and peek operation.
The Queue: This refers to the actual data structure where all elements are stored. It can hold elements of any data type, including integers, strings, or even custom objects.
Priority Functions: This assigns a priority to every element in the queue. Higher values are usually given to more important or urgent tasks.
Dequeue: This is a method for removing an element from the queue. It always removes the element with the highest priority.
Peek Operation: This method retrieves, but does not remove, the element with the highest priority.
For instance, consider a company network server managing email traffic. The server receives emails, assigns a priority to each email based on the sender’s rank at the company, then puts the email into a queue. When the server is ready to deliver an email, it uses a dequeue operation to remove the highest priority email from the queue and deliver it.
Implementing an Algorithm for Priority Queue
The steps involved in implementing a Priority Queue algorithm are as follows:
Step | Action |
---|---|
1 | Create an empty queue |
2 | Add elements to the queue, simultaneously applying the priority function to assign a priority to each element |
3 | Use a dequeue operation to remove the highest priority element when ready |
4 | Continue dequeuing until the queue is empty |
Let's denote the number of elements in the Priority Queue as \( n \). The time complexity of inserting an element into a Priority Queue is \( O(\log n) \), while removing an element is \( O(1) \).
In a programming language like Python, a Priority Queue can be implemented using the heapq module, which allows efficient insertion and deletion of elements.
Practical Examples of Algorithms for Priority Queue
Priority Queue algorithms are applied in various real-life situations:
- Traffic Management: In this case, vehicles marked 'Emergency' have the highest priority, followed by VIP vehicles, and finally, regular vehicles.
- Operating System Processes: Certain processes are crucial for the operation of the system and so are given priority over less critical tasks.
- Data Compression: Huffman coding used for data compression also applies the concept of Priority Queue.
Consider an emergency department in a hospital. Patients coming in with life-threatening conditions are considered a higher priority than those with less critical issues. The hospital staff would use a Priority Queue to ensure the right individuals get attention first, potentially saving lives.
Applications for Priority Queue
One of the fascinating aspects of computer science is the practical application of data structures, and the Priority Queue is no exception. This extraordinary data structure unwraps numerous possibilities in different fields and domains.
Practical Uses of Priority Queue in Computer Science
The Priority Queue concept is not only theoretical; it finds significant relevance in numerous computer science areas. Understanding these applications can help you gain a practical understanding of this important data structure.
A well-known use of Priority Queues is in Sorting Algorithms. Here, Priority Queues are used in the implementation of efficient algorithms:
- Heap Sort: This sorting algorithm leverages the structure of a binary heap, which is fundamentally a Priority Queue, to sort an array.
- Selection Sort: The core operation of this sort involves repeatedly selecting the minimum (or maximum) from a list of numbers and making swaps until the list is sorted. A Priority Queue can be used to speed up this process.
Consequently, Priority Queues also deliver excellence in Graph Algorithms:
- Dijkstra's Algorithm: This algorithm determines the shortest path from one node (the source) to all other nodes in a graph. It uses, you guessed it right, a Priority Queue.
- Prim's Algorithm: used for finding a minimum spanning tree for a weighted undirected graph also uses a Priority Queue.
Also, a Priority Queue is used in Systems-related Algorithms for job scheduling, load balancing, interrupt handling, etc.
A Job Scheduler in an operating system decides which of the tasks in the ready queue will be executed based on their priority. Jobs with higher priority get executed first. That's nothing but a Priority Queue in action!
Take the formula for calculating the waiting time in a priority scheduling algorithm:
\[ WaitTime = \frac{1}{n} * \sum_{i=1}^{n} \text{Time}(i) - \text{ArrivalTime}(i) \] where \(n\) is the total number of processes, Time is the time process spends in the queue and ArrivalTime is the time process enters the queue. In a well-implemented system, this process reduces wait time, increases efficiency and provides better use of resources.
Real-World Applications for Priority Queue
Priority Queues have unique applications in real-world tasks and services, demonstrating the relevance of computer science concepts in daily life.
- In the Healthcare Industry, they are often used for determining the order of care for patients. In a hospital's Emergency Room, physicians can't attend to patients based on who came first (a regular queue). Instead, they attend to patients based on the severity of their conditions, a typical example of a Priority Queue.
- Travel and Tourism is another area where the practical application of Priority Queues is profound. Imagine waiting at the airport's check-in queue where first-class passengers are allowed to check-in before economy-class passengers. The airline has created a Priority Queue, where travellers are given priority based on their ticket class.
- Also, consider Task Scheduling in real-time systems. Tasks (like data packets for transmission) are assigned priorities. For instance, in a video streaming application, audio packets can be given higher priority than video packets for smoother user experience.
Further, let's bring it down to an everyday scenario - Traffic Light Controller. Management of traffic at a signal in a smart city could also be handled by a Priority Queue. The controller can be programmed to handle the traffic based on the road's density, enabling smooth movement of vehicles in congested roads, thus using a Priority Queue.
In these complex real-world systems, the implementation of a Priority Queue can greatly improve the efficiency and overall user experience.
Advantages of Priority Queue
When dealing with complex systems that require proficient handling of data or tasks, Priority Queues provide a highly beneficial solution. From computer system tasks to real-world scenarios, the advantages are substantial and worth understanding.
Understanding the Benefits of Priority Queue
Among data structures in computer science, Priority Queues stand out due to their ability to handle data elements based on their priority. This functionality offers a range of advantages in various applications:
- Efficient Operations: Inserting elements into a Priority Queue and removing them based on their priority can be done efficiently. This is a crucial benefit in applications that require frequent insertions and deletions.
- Flexibility: A Priority Queue can be implemented with arrays, linked lists, or heaps to suit different scenarios and applications.
- Applicability in Algorithms: Priority Queues are crucial to the efficient functioning of several algorithms, including sorting algorithms like Heap Sort or graph algorithms like Dijkstra's Algorithm. The efficiency brought by Priority Queues to these algorithms is an enormous boon.
Heap sort, for example, is considered one of the best sorting methods being in-place and with no quadratic worst-case running time. The fundamental operation of heap sort involves the removal of the largest element and the subsequent adjustment of the heap - a Priority Queue operation!
Aside from these, Priority Queues also present great advantages in system-related algorithms. In scenarios that involve scheduling tasks based on priority, like in real-time computing or operating systems, implementing Priority Queues results in better resource utilisation.
They enable the system to handle high-priority tasks swiftly, thereby improving the overall efficiency and responsiveness of the system.
For example, consider load balancing, a common practice in distributed systems. The goal is to distribute the work evenly across all the systems. Implementing a Priority Queue here enables tasks to be issued to the least loaded system, consequently promoting workload balance and improving system throughput.
Consider also a system that manages print jobs in a network. Multiple users submit print jobs throughout the day, often simultaneously. A binding problem here will be deciding the order in which these print jobs should be executed. A simple FIFO queue will not be fair if some users send large jobs while others send short ones.
This is a perfect case for a Priority Queue. The print scheduler can assign higher priority to smaller jobs so that they can be printed faster, while larger jobs wait for their turn. Thus, Priority Queues help manage shared resources efficiently.
Why Choose Priority Queue Over Other Data Structures
Comparing Priority Queue to other data structures, one might question why choose it over others. The capability to deal with data or tasks based on their priority sets it apart. This characteristic is especially useful in specific situations and benefits may not be reaped with other data structures. Some reasons for choosing a Priority Queue include:
- Ordering by Priority: Unlike other queues that follow a First-In-First-Out (FIFO) policy, Priority Queues handle elements based on their priority. If any situation demands specific elements to be processed before others, a Priority Queue will be the ideal choice.
- Serving the Highest Priority: A Priority Queue consistently allows access to the item with the highest priority, making it indispensable in systems where critical tasks must be performed and prioritised first.
- Aid in Efficiency: When used in algorithms for sorting, data compression, or graph algorithms, Priority Queues can significantly enhance efficiency and decrease time complexity.
Indeed, these advantages are impressive, but the selection always depends on the requirements of your system. When the order of service depends strictly on priority, then the Priority Queue undoubtedly stands out. However, if the system operates on a simple FIFO basis or some other order, other data structures might be more fitting.
Consider a fast-food restaurant. Customers are served based on their arrival time (FIFO), not the size of their order. In this case, a simple Queue would suffice. However, in a hospital setting, where doctors must attend to severe cases first, a Priority Queue becomes essential.
Another concrete illustration can be found in event-driven simulation models - critical components of fields like network modelling or study of patient flow in hospitals. These models use a Future Events List, where events are arranged based on the event time, and events must be processed in chronological order.
Here, a Priority Queue serves the purpose well, allowing efficient retrieval of the event with the smallest event time and rapid reordering of the list when new events are scheduled.
Ultimately, choosing the correct data structure – be it a Priority Queue or something else relies on an understanding of your system, the operations you'll need to perform, and the real-world problem you're endeavouring to solve.
Priority Queue in Java
The Java programming language comes with built-in support for the Priority Queue data structure. But before using it, it's critical to understand its underlying architecture and methods available for manipulating Priority Queues.
Understanding Priority Queue Implementation in Java
Priority Queue in Java is implemented through the Priority Queue class, part of Java's collections framework. This class essentially represents a Priority Queue that is implemented as a balanced binary heap.
A binary heap is a binary tree with two additional constraints - shape property (all levels except the last have the maximum number of nodes, and nodes are as far left as possible) and heap property (every node's key is greater (or smaller in case of a min heap) than its children's keys).
Elements in Java's Priority Queue are ordered based on their natural ordering or by a custom Comparator provided during the construction of the Priority Queue. By default, the least element with regard to the specified ordering gets the highest priority.
There are eleven methods available for manipulating Priority Queues in Java. Briefly, these methods can be categorised into four groups:
- Addition : includes the add() and offer() methods to insert elements into the queue.
- Removal : includes the remove() and poll() methods for deleting elements from the queue.
- Examine : includes element() and peek() methods for retrieving but not removing the element with the highest priority.
- Miscellaneous : includes other methods to manipulate the queue, such as clear() to remove all elements, contains() to check if an element exists in the queue, and so on.
Consider, for example, a Priority Queue that holds a series of job tasks, each with a unique job ID. The job tasks can be represented as custom objects, and a Comparator can be provided to sort based on job ID. Once that queue is established, these tasks can be added using add(), removed using remove(), and the highest priority task (with the lowest job ID) can be retrieved without removal using peek().
Priority Queues in Java serve key roles in various domain areas such as task scheduling, error handling, or even in scenarios involving graph traversal and search algorithms like Dijkstra's Algorithm and Prim's Algorithm where they aid in efficiently handling and prioritising tasks.
A Beginners Guide to Priority Queue Java
Are you at the inception of your Java learning journey and wish to understand how to handle Priority Queues? No worries, a comprehensive guide is here to help you out.
To begin with, let's learn how to create a Priority Queue –
PriorityQueue<Integer> queue = new PriorityQueue<>();
You just created an empty Priority Queue with natural ordering, which means the lowest integer will have the highest priority. If you want to create a Priority Queue with a custom Comparator, it'd look like this:
PriorityQueue<Integer> queue = new PriorityQueue<>(Comparator.reverseOrder());
Now you have a Priority Queue where the largest integer has the highest priority.
To add elements to the queue, you use the add() or offer() method:
queue.add(10); queue.offer(20); queue.offer(30);
Now, your queue has three elements: 10, 20, and 30. If you want to know which element has the highest priority but don't want to remove it, use the peek() method:
int highestPriority = queue.peek();
In this case, depending on your queue's ordering, either 10 or 30 would be assigned to the highestPriority variable (30 in case of the natural ascending order, 10 for descending).
And to remove the highest priority element from the queue, you use the remove() or poll() method:
int highestPriority = queue.poll();
Polling the queue also tells you which element was removed. If the queue is empty, poll() will return null, whereas remove() will throw a NoSuchElementException.
Let's consider a real-world example to illustrate these concepts.
An Internet Cafe operates on a first-come-first-serve basis regarding new customers but gives priority to previous customers for recharges. The cafe decides to implement a Priority Queue where new customers are treated like regular integers and previous customers are represented by their negatives.
So, in essence, a new typical queue would be {5, -1, 3, -2} and when dequeued would result in {-2, -1, 3, 5}. The regular customers (-2 and -1) have higher priority than the new ones (3 and 5).
This is an overview of how to deal with Priority Queues in Java. Understanding the fundaments of creating and manipulating such queues will give you a strong foundation in dealing with real-world scenarios involving data structures and greatly aid in problem-solving within the Java programming language.
Priority Queue in Python
In Python, the concept of Priority Queue isn't explicitly provided as a built-in data structure, but thanks to Python's library heapq, it can be implemented with ease. This library offers functions to convert a regular list into a heap, find the smallest element, or pop the smallest element from the heap and push a new element, among others utilities.
Exploring Priority Queue Python Implementation
To use a Priority Queue in Python, you initially need to import the heapq module. Following that, the key functions available for implementing a Priority Queue using heapq include:
- heapify(): This function converts a regular list to a heap. In the resulting heap, the smallest element gets pushed to the index position 0.
- heappush(heap, ele): This function is used to insert an element into the heap. The order of the heap is adjusted so that it maintains its heap properties.
- heappop(heap): This function is used to remove and return the smallest element from the heap. After the removal of the element, the order of the heap is maintained.
- heappushpop(heap, ele): This function combines the action of pushing a new element and popping the smallest element into a single statement, thus ensuring efficiency.
- heapreplace(heap, ele): This function first pops the smallest element and then pushes a new element to the heap. It’s different from heappushpop() as it always pops the smallest element before pushing the new element.
While these methods ensure that the smallest element is always at the front of the queue, you can easily maintain a Priority Queue with the largest item at the head by inverting the order. You can do this by changing the values of items stored in the queue.
If you store each value as a tuple, with the first element being its priority, simply negating this number will sort by its actual priority, and the high-priority elements will be popped first.
Operation | Method | Time Complexity |
---|---|---|
Insert | heappush() | \(O(\log n)\) |
Get Minimum | heap[0] | \(O(1)\) |
Delete Minimum | heappop() | \(O(\log n)\) |
Search | N/A | \(O(n)\) |
Let’s say you have a scatter of tasks with different priorities that are routed to a central system. The system handles each task based on its priority.
Each task can be represented as a tuple, with the first element being its priority (1 for high priority, 2 for medium priority, 3 for low priority), and the second element being the task description:
tasks = [(3, 'Low Priority Task'), (2, 'Medium Priority Task'), (1, 'High Priority Task')] import heapq heapq.heapify(tasks) while tasks: priority, task = heapq.heappop(tasks) print(task)
Start with Priority Queue in Python
If you're new to Priority Queue in Python, don't fret. The simplicity and flexibility of Python make it easy to start and experiment with Priority Queue.
The first step is to set up a list of elements. Each element in this list is a tuple where the first value is the priority and the second value is the item you want to add to the Priority Queue:
queue = [(3, 'apple'), (2, 'banana'), (1, 'cherry')]
Next, import the heapq module and turn the list into a heap:
import heapq heapq.heapify(queue)
The queue is now a Priority Queue, ordered by the first element in each tuple. Now, you can pop an element from the queue with:
print(heapq.heappop(queue))
This will always remove and return the smallest element from the Priority Queue. Remember, to keep the queue as a Priority Queue, always use heapq's heappush() for adding new elements:
heapq.heappush(queue, (4, 'date'))
Imagine you want to implement a Priority Queue to manage the order of print jobs in your office. Jobs from the boss have high priority, followed by jobs from the manager, and then jobs from interns. You can denote this with a decreasing numeric priority: the boss is 1, the manager is 2, and the intern is 3. Here's how you would handle this scenario:
print_jobs = [(1, "The Boss's Report"), (3, "Intern's Presentation"), (2, "Manager's Memo")] import heapq heapq.heapify(print_jobs) while print_jobs: priority, job = heapq.heappop(print_jobs) print("Printing job: ", job)
If you follow these guidelines, you will be using Priority Queue in Python like a pro before you know it. With practice and regular use, Priority Queues can become a powerful tool in your programming arsenal, a critical component when precise task ordering matters the most in your applications.
Priority Queue - Key takeaways
Priority Queue is a data structure where every element is assigned a priority and elements with higher priorities are dequeued before those with lower ones.
Priority Queues are used in sorting algorithms (e.g., heap sort), graph algorithms (e.g., Dijkstra's and Prim's), and system-related algorithms (e.g., load balancing and interrupt handling).
A Priority Queue algorithm operates on several elements including the queue, priority functions, dequeuing, and peek operation.
Priority Queue applications include sorting algorithms, graph algorithms, and system-related algorithms. Real-world applications are found in healthcare, travel and tourism, and task scheduling in real-time systems.
Advantages of Priority Queue include efficient operations, flexibility, and applicability in numerous algorithms.
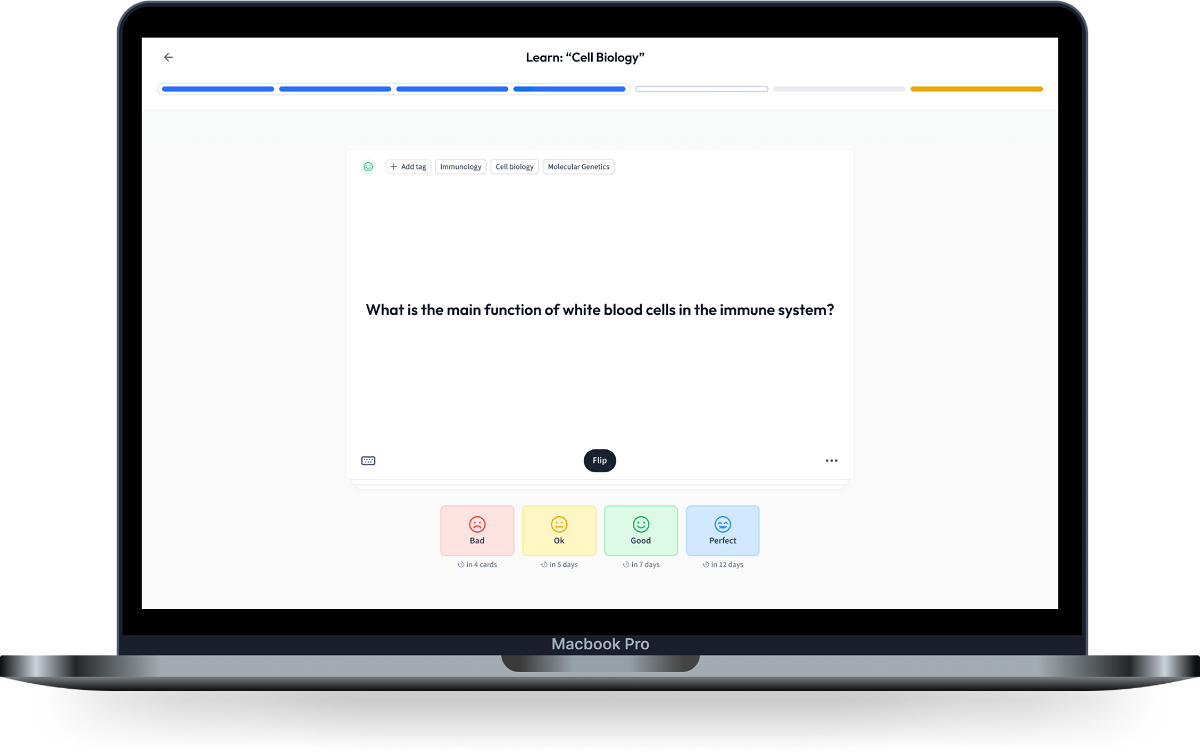
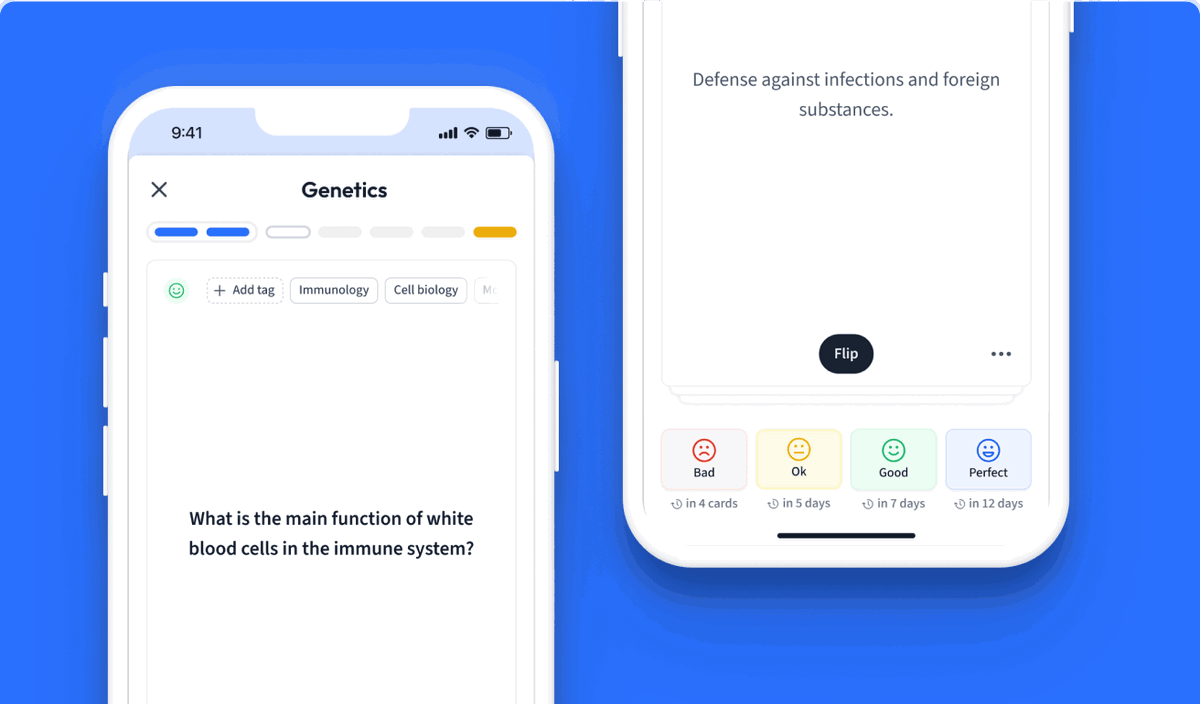
Learn with 6 Priority Queue flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Priority Queue
How to implement priority queue?
A priority queue can be implemented using an array, linked list, heap or binary search tree. The most efficient way is to use a binary heap. This is because a binary heap can fetch, remove and add items in O(log n) time. A binary heap maintains the property that a parent node's priority is higher (or lower) than the priority of its children, making it efficient to find the highest (or lowest) priority element.
What is priority queue?
A priority queue is a special type of queue in data structure where each element is associated with a priority. In this queue, elements with higher priority are dequeued before the elements with lower priority. If two elements carry the same priority, they are served as per their ordering in the queue. This is extensively used in computer systems for memory management and task scheduling.
How to create a priority queue in java?
In Java, you can create a priority queue by instantiating the PriorityQueue class. The syntax for creating a priority queue is: PriorityQueue<dataType> queueName = new PriorityQueue<dataType>();. You can optionally specify an initial capacity or a Comparator at construction. Default priority queue orders elements in natural ordering (i.e., according to their 'compareTo' method or Comparable interface), or you can customise this with a Comparator.
How to create a priority queue in python?
In Python, you can create a priority queue using the built-in module "heapq". First, import the module using `import heapq`. To initialize an empty priority queue, create an empty list, example: `pq = []`. You can add elements to this priority queue with the `heapq.heappush(pq, item)` function, while `heapq.heappop(pq)` will remove and return the smallest item from the priority queue.
How does priority queue work?
A priority queue works by giving priority to elements while inserting and removing them. When an element is inserted into a priority queue, it finds its place based on its priority, not on its insert time. During removal, the element with the highest priority is removed first. If two elements have the same priority, they are removed based on their order in the queue.
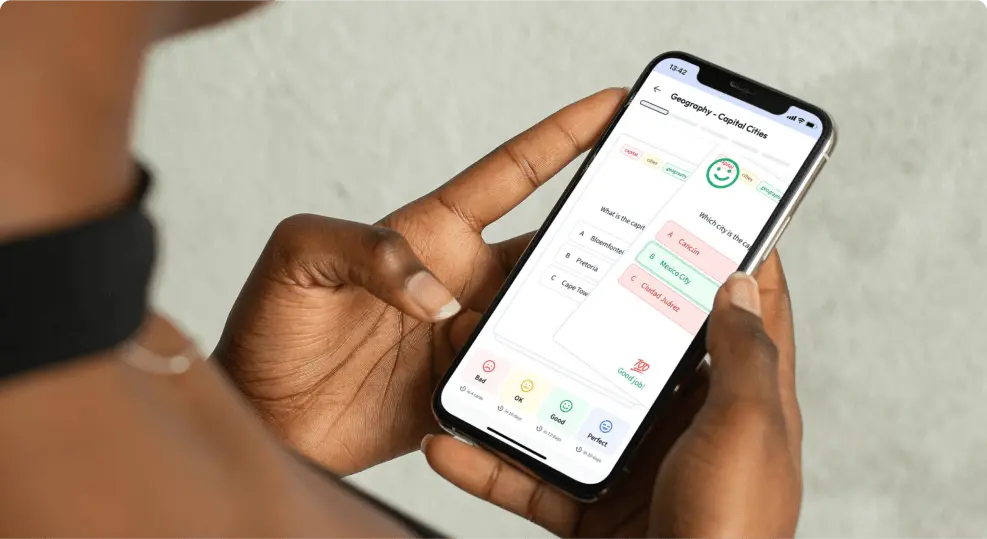
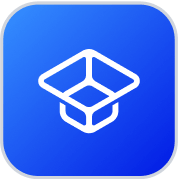
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more