Understanding Graph Algorithms in Computer Science
Graph Algorithms are a critical pillar of computer science. They serve as the core principles governing network structures, data traversals, and intricate problem-solving tactics.
Basic Principles of Graph Algorithms
To grasp the essence of Graph Algorithms, you need to understand their basic principles. The primary aspects include vertices/nodes, edges, weights, and traversal mechanisms. Essentially, a Graph Algorithm handles these components to discern solutions for specific computational problems.
A Graph is a collection of nodes (also creatively referred to as vertices) connected by links known as edges. An edge from Node A to B is different from an edge from Node B to A, especially in directed graphs.
There're also weights (numerical values) tied to the edges of these graphs. These weights augment complexities but provide a more realistic representation for certain problems.
Key Terminology in Graph Algorithms
Understanding key terminologies is instrumental in mastering the world of Graph Algorithms. This terminology includes but is not limited to:
- Node/Vertex
- Edge
- Graph
- Directed Graph
- Undirected Graph
- Weight
Different Types of Graph Algorithms
Graph Algorithms are diverse, each harboring its specialties and optimal situations where it excels. You need to know about the different types, how they work, and where to apply them to exploit their full potential.
Overview of Graph traversal algorithms
Graph traversal algorithms are designed to visit the vertices of a graph. The two conventional types are Breadth-First Search (BFS) and Depth-First Search (DFS).
BFS visits vertices layer by layer from a source vertex. Its traversal is akin to ripples spreading across the water surface when a stone is thrown.
DFS doesn't operate in layers. Instead, it dives deeply into branches, intermittently retracing steps when it meets dead ends.
Recognition of Graph search algorithms
Graph search algorithms have diverse use-cases ranging from geography to computer science. They locate shortest paths between nodes within graphs. Three such algorithms are Dijkstra's, Bellman-Ford, and Floyd-Warshall algorithms.
Purpose of Graph coloring algorithms
Graph coloring algorithms produce a juxtaposition of colors on the nodes of a graph. Honestly, it's not about basking in the spectrum's beauty. The colorings are subjected to stringent conditions, like no two adjacent nodes splaying the same color.
Graph coloring algorithms solve various issues, including map coloring, scheduling problems, and solving Sudoku games.
Importance of Graph clustering algorithms
Clustering in Graph Algorithms enables identification of closely connected groups within a graph. It's primarily used in network analysis, community detection, and anomaly detection.
Graph Algorithms encapsulate a plethora of computational solutions that can be leveraged to unravel intricacies in different spheres of life. The intrigue grows once you delve into the world of Graph Algorithms.
Deep Dive Into Graph Shortest Path Algorithm
The shortest path algorithm in graph theory is used to determine the shortest possible route from one point (vertex) in a graph to another. It's an essential 'tool kit' in fields such as network routing, where the goal is to transmit data by the quickest route possible.
Explaining Dijkstra Algorithm Directed Graph
Dijkstra's algorithm is a classic solution to the shortest path problem for a graph with non-negative edge path costs, producing a shortest path tree. Despite its elegance, Dijkstra's algorithm can be a bit complex for beginners, so let us break it down in detail.
Basics of Dijkstra Algorithm
Dijkstra's algorithm, conceived by computer scientist Edsger W. Dijkstra, operates by assigning a tentative distance to every vertex in the graph, setting the initial node as zero and the rest as infinity. The algorithm then continuously chooses the unvisited vertex with the smallest tentative distance, then recalculates the tentative distances to this vertex's neighbouring nodes.
To start, you declare two sets - settled and unsettled. Initially, all vertices are in the unsettled sets. Then, for each iteration, you pick a node with the least weight from the unsettled set and transfer it to the settled set. For this picked node, evaluate all its unsettled neighbours, and for each, calculate the sum of the edge weight and the picked node’s settled weight. If this sum is less than the current node weight, update the node’s weight.
In mathematical terms, if \( d[v] \) is the current shortest distance from the source to vertex v, and \( w(u, v) \) is the weight of edge (u, v), then the weight of vertex v can be updated to
\[ d[v] = \min(d[v], d[u] + w(u, v)) \]Application of Dijkstra Algorithm in Graphs
Dijkstra's algorithm is primarily used for routing in networking for shortest path routing protocols, where the shortest paths need to be recalculated in real time with every change in the network. In geographic navigation systems, Dijkstra's algorithm helps find the shortest path between two cities.
Practical Examples of the Graph Shortest Path Algorithm
Providing practical uses of the shortest path algorithm reveals the efficiency and applicability of the algorithm in solving various problems.
In social networks like LinkedIn, the shortest path algorithm helps find the shortest connection chain between two individuals.
In e-commerce, the algorithm aids in recommendations. For example, from a large graph of users and products, the shortest path from one product to a targeted user suggests the likeliest recommendations for that user.
Various programming languages can implement the shortest path algorithm. Typically, you create a graph, usually with an adjacency list or adjacency matrix, and apply a shortest path algorithm like Dijkstra's algorithm. Java, Python, C++, and many other languages can achieve this.
class Graph: def _init_(self, vertices): self.V = vertices # No. of vertices self.graph = [[0 for column in range(vertices)] for row in range(vertices)] def printSolution(self, dist): for node in range(self.V): print("Vertex Distance from Source") print(node, "tt", dist[node]) # Implementing g = Graph(9) g.graph = [...] g.dijkstra(0)
Ensure to assess the nature of the problem and the properties of the graph before applying Dijkstra’s algorithm. Use should be restrained where the graph is scaled to millions of nodes or when edges have negative weights, leading to the algorithm providing incorrect results.
Techniques and Examples of Graph Algorithms
Graph algorithms present a useful method for representing and solving complex relationship problems. They provide a clear structure to handle such connected data and allow efficient computation.
Essential Graph Algorithms Techniques
Graph simulation, traversal and labelling are some of the most common techniques used in graph algorithms. Dense calculations and vector computations are also prominent techniques utilised.
Graph simulation, used in monitoring large ecosystems, is a technique that treats the graph as a model of a system and cultures the model's evolution over time using computational methods. It is commonly used in areas like social network analysis, biological systems and the spread of diseases.
Graph traversal is a process that involves visiting each vertex in a graph for purposes such as exploring a maze or finding a specific node. Depth-First Search (DFS) and Breadth-First Search (BFS) are the two canonical methods for graph traversal.
Graph labelling is an algorithmic technique that entails assigning labels to vertices or edges. These labels can represent properties such as weight, capacity, or information about the associated node or edge.
In dense calculations, all pair interactions between nodes are considered. Dense calculations are used in similarity, distance, or correlation computations.
Vector computations calculate properties such as centrality or importance of nodes in a graph.
Applying Techniques in Real-world Problems
Understanding how to apply graph algorithms in real-world problems is key to mastering them. In bioinformatics, for example, graph algorithms are used to establish the similarity between different genes or proteins.
Breadth-First Search (BFS) could be used for social networking websites to suggest friends as it explores all the friends of the current node before moving to the friends of the next node.
Within journey planning and network routing, Dijkstra’s algorithm can be used to compute the shortest path between two points.
Practical Graph Algorithms Example
Practical examples of graph algorithms are abound in various domains, from social networking to airline travel and telecommunications.
How Graph Algorithms are Implemented in Computer Science
Graph algorithms play a massive role in computer science. Some key implementation areas of graph algorithms in computer science include natural language processing, artificial intelligence (AI), and database systems.
In natural language processing, graph algorithms aid in interpreting and processing human languages. These algorithms, such as BFS, DFS, and Dijkstra’s algorithm, are used to analyse the structure of sentences, co-reference resolution, and machine translation.
In artificial intelligence (AI), graph algorithms are used for searching, pattern recognising, and decision making. They're used to design intelligent systems that can perform tasks that would usually require human intelligence.
In database systems, graph algorithms are used for transaction processing, query optimisation, and managing concurrency control. They help ensure that transactions are carried out correctly and help maintain data integrity.
// Implementation of BFS in Java import java.io.*; import java.util.*; class Graph{ ... void BFS(int s) { boolean visited[] = new boolean[V]; LinkedListqueue = new LinkedList (); visited[s]=true; queue.add(s); while (queue.size() != 0){ s = queue.poll(); System.out.print(s+" "); Iterator i = adj[s].listIterator(); while (i.hasNext()){ int n = i.next(); if (!visited[n]){ queue.add(n); visited[n] = true; } } } } }
The key to implementing graph algorithms effectively in computer science is a solid understanding of the principles that underpin them. With these principles at your fingertips, you can leverage graph algorithms across multiple domains of computer science.
Advanced Topics in Graph Algorithms
Within the realm of computer science, graph algorithms open the door to solving an extensive range of complex problems. As you delve deeper into the subject, you'll encounter topics that push the boundaries of traditional applications, requiring processes like advanced understanding of algorithm complexities and specialised search algorithms. Let's give these topics proper exploration.
Complexities of Graph Algorithms
Like any algorithm, graph algorithms come with complexities that dictate the resources required for their execution. Specifically, we are interested in time and space complexity.
Time complexity in graph algorithms is a measure of the amount of computational time taken by an algorithm to run, as a function of the size of the input to the program. For a graph with \( V \) vertices and \( E \) edges, the time complexity of a graph algorithm is often expressed in terms of \( O(V + E) \) for DFS and BFS traversals, or \( O(V^2) \) for matrix representation.
Space complexity of graph algorithms, on the other hand, correlates to the maximum space needed by the algorithm. For graph algorithms, this typically revolves around the storage of the graph's nodes and edges. Much like time complexity, space complexity varies based on the graph and the algorithm applied. For example, storing a graph as an adjacency matrix would command a higher space complexity of \( O(V^2) \) than an adjacency list representation of \( O(V + E) \).
Understanding Time and Space Complexity in Graph Algorithms
Profound comprehension of time and space complexities in graph algorithms is crucial for their efficient implementation. Different types of graph representations can significantly affect both complexities, hence the need for an astute selection based on the problem at hand.
In an adjacency matrix, entering an edge or checking for its existence is an \( O(1) \) operation, whereas looking for adjacent nodes or counting the degree of a node costs \( O(V) \) time. Owing to these characteristics, an adjacency matrix can be beneficial for dense graphs, where the number of edges \( E \) approaches the square of the number of vertices \( V \), thus making its space complexity of \( O(V^2) \) more sensible.
Conversely, an adjacency list reduces space requirement for sparse graphs to \( O(V + E) \). Most operations, like inserting an edge or checking for its existence, become \( O(V) \). Adjacent nodes can be identified directly from the list, and degree counting takes constant time with appropriate data structures.
Parsing the complexities of graph algorithms, you'll come across other essentials too. Some algorithms, like Prim’s and Kruskal’s minimum spanning tree algorithms, have a time complexity of \( O(E log E) \) or \( O(E log V) \). Advanced algorithms, such as the Floyd Warshall algorithm, bear a time complexity of \( O(V^3) \) due to its three nested loops over the graph vertices.
Advanced Graph Search Algorithms
Moving beyond basic traversal techniques such as DFS and BFS, you will encounter more advanced graph search algorithms. Some of the most renowned ones include Dijkstra's algorithm for shortest path finding, Prim's and Kruskal's algorithms for finding the minimum spanning tree, and the Floyd Warshall algorithm for finding shortest paths in a weighted graph with positive or negative edge weights but no negative cycles.
The Real-life Impact of Graph Search Algorithms
Advanced graph search algorithms have a profound impact on real-world applications, solving complex problems across a myriad of domains.
- Dijkstra's algorithm, known for its efficiency and broad utility, is prominently used in routing and as a subroutine in other graph algorithms. Any time you use a GPS, you're likely utilising Dijkstra's algorithm.
- Bellman-Ford algorithm is used in distance vector routing protocol, such as the internet's RIP implementation. It's also valuable in cycle detection, particularly negative cycles which cause incorrect shortest path computations.
- Prim's algorithm and Kruskal's algorithm are used in network design. They provide a minimum cost network, making them vital tools in constructing roads, telecommunication lines, spanning trees in wheels and other similar infrastructure problems.
- The Floyd Warshall algorithm has a wide range of applications from path planning in robotics to network routing.
Implementing advanced graph algorithms comes with its intricacies, which makes understanding their structure, rule, and quirks imperative. Coding these advanced algorithms usually involves creating a graph and iterating over its vertices and edges, updating the weights, ranks, or labels of vertices or edges based on specific rules.
void BellmanFord(struct Graph* graph, int src) { int V = graph->V; int E = graph->E; int dist[V]; // Step 1: Initialize distances for (int i = 0; i < V; i++) dist[i] = INT_MAX; dist[src] = 0; // Step 2: Relax all edges |V| - 1 times for (int i = 1; i <= V - 1; i++) { for (int j = 0; j < E; j++) { ... // Step 2: Relax edge if (dist[u] + weight < dist[v]) dist[v] = dist[u] + weight; } } // Step 3: check for negative-weight cycles ... }
Mastery of advanced graph algorithms not only expands your algorithmic toolset but also equips you with the skills to apply computational principles to complex real-world problems effectively.
Improving Skills in Graph Algorithms
To stride ahead in computer science, it is essential for you to master the art of graph algorithms. This subject allows you to understand the underlying concepts important for designing efficient solutions to complex problems. Let's unravel the challenges you might encounter while learning, and delve into effective strategies and practical projects that can help you strengthen your proficiency in graph algorithms.
Challenges in Learning Graph Algorithms
As interesting as graph algorithms are, you might face a few hurdles in the learning process. These include understanding the way graphs are represented, implementing coding solutions, and dealing with space-time complexities of various graph algorithms.
Successful mastery of graph algorithms involves navigating through these unique challenges associated with each individual algorithm, all the while considering the practical implementations and constraints that could influence the choice of one algorithm over another.
Challenge | Possible Reason |
Understanding Graph Representations | Graph representations like adjacency lists and adjacency matrices can be initially perplexing due to the concept of edges and vertices. |
Implementing Graph Algorithms | Coding graph algorithms, particularly advanced ones with intricate steps, is often trickier than visualising them, given the deep programming cognition required. |
Comprehending Space-Time Complexities | The understanding of Big O notation, time complexity, and space complexity pivotal to graph algorithms mandates sound knowledge of theoretical computer science and mathematics. |
Effective Strategies for Learning Graph Algorithms
To overcome these difficulties, you need a strategic approach to learning graph algorithms. The following steps can assist you to smooth climb the learning curve:
Graphic Visualisation: A picture speaks a thousand words. For beginners in graph algorithms, visualising the graph on paper before diving into the code can offer significant assistance.
Implementation: Actively coding graph algorithms helps to cement the understanding. Try implementing common graph algorithms like DFS, BFS, Dijkstra's algorithm etc., in any programming language of your choice.
Regular Practice: The more algorithms you practice, the more adept you become in writing pseudocode and real code for different scenarios.
Analytical Thinking: Cultivate the habit of analysing the problem and the inherent graph aspects before jumping to solve the problem.
Practical Projects to Understand Graph Algorithms
Apart from theory, the real grasp of graph algorithms lies in hands-on experience. Implementing them in real-world tasks and personal projects can be an effective way to translate theoretical understanding into practical skills.
You could, for instance, consider developing a simple yet practical project like a GPS application that uses Dijkstra's or A* search algorithm for determining the shortest route between two points.
Another engaging project could be modelling a social network using graphs where nodes represent individuals and edges stand for relationships. BFS could be used to find the shortest connection (degrees of separation) between two people.
Applying Graph Algorithms in Computer Science Projects
Given the extensive applications of graph algorithms, you can incorporate them in multiple computer science projects. Here are a few examples:
Telecommunications: Communication networks are represented using graphs, with vertices representing switches and routers. Graph algorithms such as minimum spanning trees can be used to design optimal routing strategies.
Cybersecurity: For detecting intrusions in computer networks, you can use graph algorithms. Once a network topology has been mapped into a graph, graph algorithms can be applied to detect suspicious activities or anomalies.
Web Crawlers: Algorithm concepts can be used to create web crawlers. Starting from a source page, the web crawler can use a breadth-first search to find and index pages up to a certain depth.
The more engagement you have with graph algorithms, the more solutions you will find for a variety of problems. With in-depth exploration and practice, graph algorithms can become your go-to strategy for problem-solving.
Graph Algorithms - Key takeaways
- Graph Algorithms: They are methods to systemize mathematical graph structures and solve complex problems about relationships.
- Dijkstra Algorithm: It is a graph shortest path algorithm that assigns a tentative distance to every vertex in the graph and then continuously selects the unvisited vertex with the smallest tentative distance.
- Application of Dijkstra algorithm: It is widely used in shortest path routing protocols in networking and geographical navigation systems.
- Techniques in Graph Algorithms: These include Graph simulation, traversal, labelling, dense calculations, and vector computations.
- Complexities of Graph Algorithms: Time complexity is a measure of the computational time taken by an algorithm to run, and Space complexity refers to the maximum space needed by the algorithm.
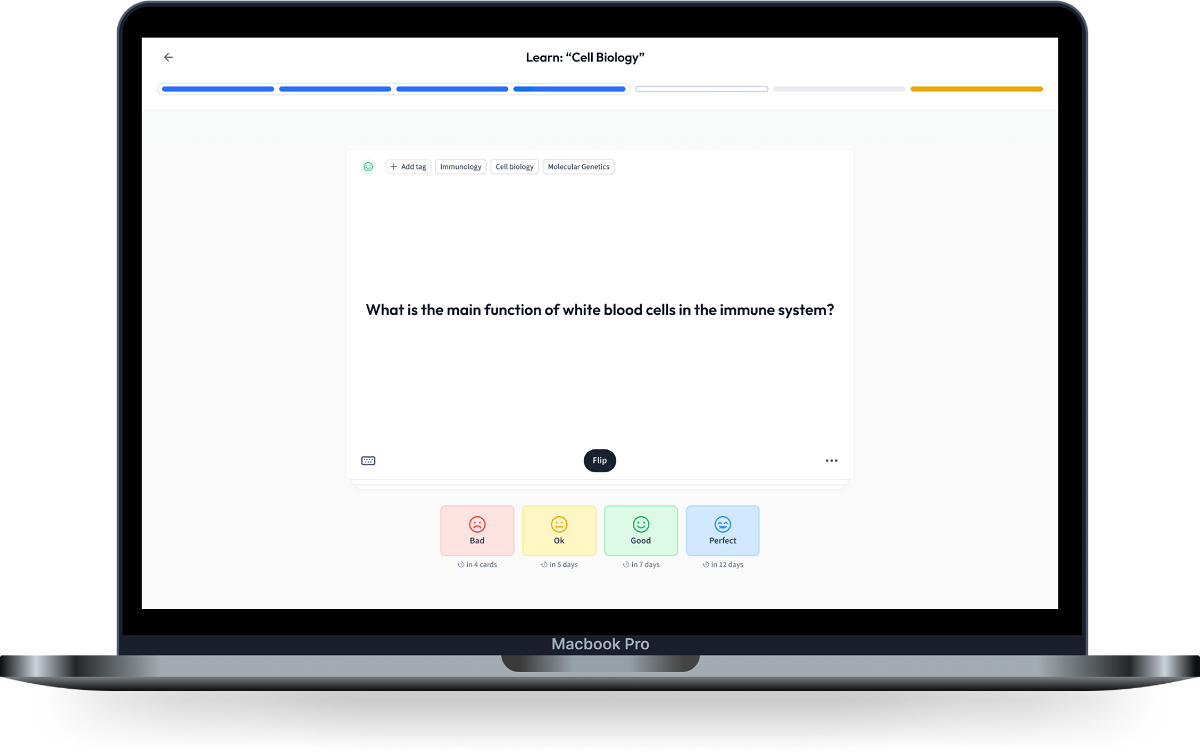
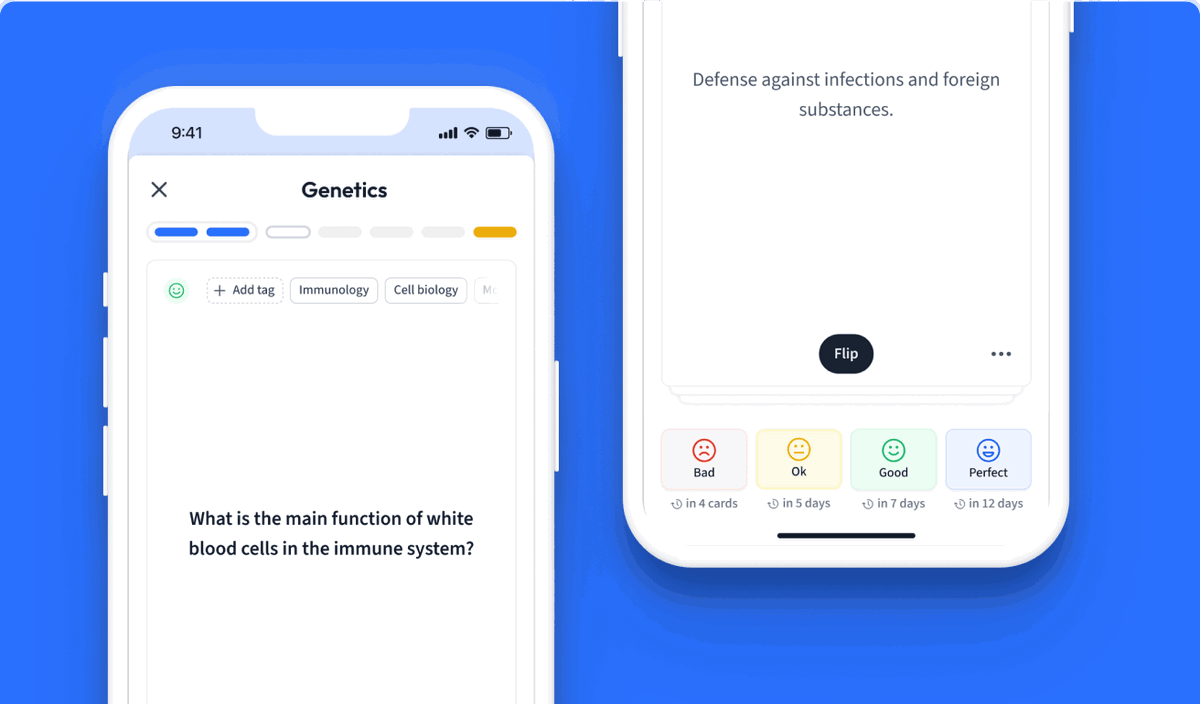
Learn with 84 Graph Algorithms flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Graph Algorithms
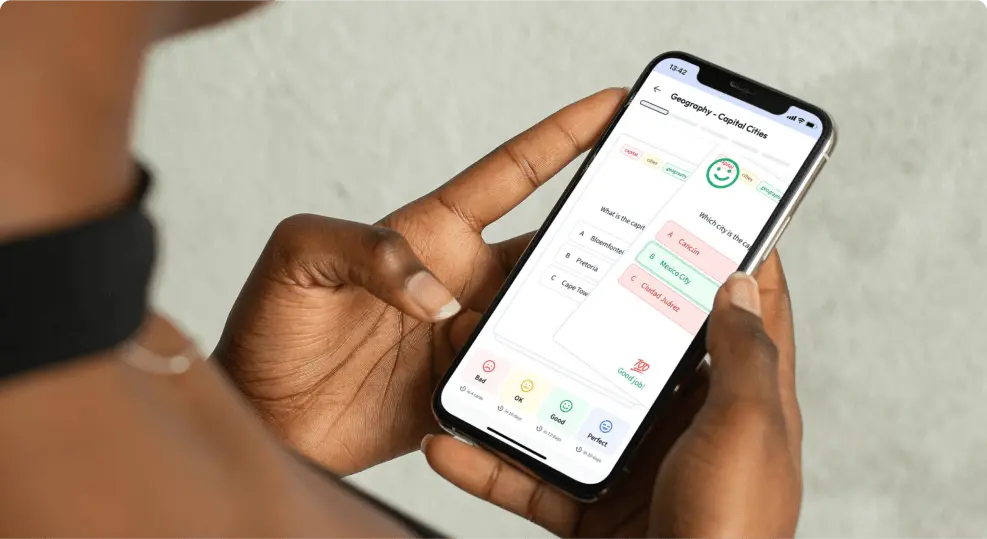
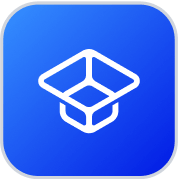
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more