Understanding the Basics of Designing Algorithms
Designing algorithms lies at the core of Computer Science, embodying the creative aspect of problem-solving and computation. An algorithm, by definition, is a set of step-by-step instructions used to solve a specific problem or achieve particular outcomes.
An Algorithm is a sequence of computational steps that convert input into output.
The Importance of Algorithm Design in Computer Science
Algorithm design plays a pivotal role in many areas of computer science and beyond. With a well-designed algorithm, you tailor solutions more efficiently and solve problems across a multitude of domains ranging from simple arithmetics to data analysis and artificial intelligence.
Here are some reasons why Algorithm Design matters in computer science:
- Algorithms facilitate automation of repetitive tasks, making computing processes efficient and consistent.
- They form the backbone of any reliable computer software.
- Impacts the performance and success of data structures, machine learning models, and information systems.
Recognising the Key Principles of Algorithm Design
Algorithm design principles are fundamental concepts that guide the construction and understanding of algorithms. They are key to developing effective, efficient, and versatile algorithms.
The following table explores some of the basic principles.
Principle | Description |
Decomposition | Breaking the problem into sub-problems |
Pattern Matching | Identifying similarities among problems |
Abstraction | Simplifying complex details |
Getting to Grips with Algorithm Design Methodology
Algorithm design methodology is about how you approach a problem, break it down, and then create the algorithm. It's a structured process that enables you to understand and solve complex problems more effectively.
How to Apply Algorithm Design Principles Effectively
For instance, in sorting a list of numbers (an array), one of the most common algorithmic solutions is the Bubble Sort method.
BEGIN FOR i = 0 to array length - 1 FOR j = 0 to array length - i - 1 IF array[j] > array[j + 1] THEN SWAP array[j] and array[j + 1] END IF END FOR END FOR END
Elevating your Skills: Advanced Algorithm Design
As you get more comfortable designing basic algorithms, you may want to delve into more advanced topics like application-specific algorithm strategies, advanced data structures, parallel algorithms, and probabilistic analysis. These concepts delve deeper into the realm of algorithm design, leading to even more efficient and effective solutions.
The Journey to Designing Efficient Algorithms
Embarking on the journey to designing efficient algorithms demands a firm understanding of the basic principles of algorithm design and an appreciation for efficiency in this domain. For an algorithm, efficiency largely concerns time complexity and space complexity. It is about how the resources consumed by the algorithm grow with the size of the input.
Why Efficiency Matters in Algorithm Design
In algorithm design, the term 'efficiency' invokes a sense of utility or functionality, focused on optimising resource consumption. It is not always just about accomplishing tasks but accomplishing them using the least possible resources. An algorithm may offer the correct solution to a problem, but if it takes a very long time or consumes a large amount of memory, it may not be efficient.
Efficiency in Algorithm Design is significant due to:
- The need to solve large problem instances: Algorithms often need to handle large inputs data sets, for which inefficient algorithms may fail, while efficient ones will succeed.
- Constrained computational resources: Especially in embedded systems or mobile devices, where both processing power and memory are limited, efficient algorithms are crucial.
- Performance requirements: Real-time systems require tasks to be accomplished within strict timelines, necessitating efficient algorithms.
Time complexity is a computational complexity that describes the amount of computer time taken by an algorithm to run. Space complexity, on the other hand, represents the amount of memory space that the algorithm needs to run to completion.
Analysing the Role of Efficiency: Design and Analysis of Algorithms
Algorithm efficiency is typically expressed using Big-O notation. This notation describes the upper bound of time or space requirements as a function of the size of the input, designated as \( O(f(n)) \), where \( f(n) \) is a function that describes how the cost grows with the size of the input, \( n \).
Thus, in the analysis and design of algorithms, the focus is on:
- Designing the algorithm to solve the problem correctly.
- Analyzing the algorithm's time and space complexity, often using Big-O notation.
- Refining the algorithm to minimise time and space complexity, if required.
For instance, if an algorithm has a time complexity of \( O(n^2) \), it means that if the input size doubles, the time for computation may quadruple. It shows a quadratic growth of computation time with the input size. So, such an algorithm could be very slow for large inputs.
Steps to Creating Efficient Algorithms
Designing efficient algorithms involves a series of systematic steps that begins primarily with understanding the problem and carries through until you refine your algorithm for efficiency. These steps make the process manageable and structured. Here are the steps involved:
- Problem Definition: Clearly understand and define the problem you are trying to solve.
- Formulating the Algorithm: Formulate the algorithm as a series of computational steps.
- Pseudocode Writing: Write the pseudocode for the algorithm, which serves as a more detailed version of the algorithm in a human-readable form.
- Analysis: Analyse the algorithm for correctness and efficiency. While correctness ensures that the algorithm indeed solves the problem, efficiency relates to the resources the algorithm consumes, such as time and space.
- Refinement: Based on the analysis, refine the algorithm to improve its efficiency, if required.
Case Study: Algorithm Design Example for Better Understanding
Let's consider a simple problem: Finding the maximum element in an array of integers.
1. Set max to array[0] 2. For each element in the array 3. If element > max Then 4. Set max equal to element 5. End If 6. Next element 7. max now holds the maximum value in the array
This algorithm is correct as it correctly finds the maximum element in any given array. Its time complexity is \( O(n) \), as it involves a single loop over the array. This complexity is considered efficient, so no refinement is necessary in this case.
The Art of Fine-tuning Algorithm Abstraction Technique
Algorithm abstraction can be seen as one of the most crucial skills to master in Computer Science. This distinctive technique transforms complex problems into manageable tasks, enhancing computational efficiency and paving a clear pathway towards solution. It involves removing unnecessary details, thus simplifying the problem-solving process.
Decoding the Algorithm Abstraction Technique
Abstraction is an indispensable principle in algorithm design, representing a strategy of managing complexity. Given its utility and versatility, understanding the way abstraction works in algorithm design might initially seem overwhelming. Below is an in-depth discussion to help unravel this fascinating subject.Abstraction in algorithm design refers to the process of simplifying complex systems by breaking them into sub-systems or components essential to the problem-solving process, ignoring unnecessary details.
- Problem Decomposition: This involves breaking down the problem into smaller, more manageable sub-problems that can be solved more easily.
- Data Abstraction: This abstraction type focusses on data manipulation, rather than data structure details. A good example of data abstraction is using data types, such as lists, queues or stacks, without considering their internal implementations.
- Procedure Abstraction: In this case, an algorithmic task is split into reusable procedures or subroutines.
The Intersection of Abstraction and Efficiency in Designing Algorithms
Although often overlooked, there is a robust linkage between abstraction and the efficiency of algorithms. The reason is simple – abstractions simplify a problem, allowing for more efficient problem-solving and algorithm design. Below are different ways in which abstraction contributes to efficiencies in algorithm design:
- Ease of Understanding: Abstraction simplifies complex problems, making them easier to understand. This clarity of thought often results in the creation of more efficient algorithms.
- Re-usability: Procedure abstraction enables the creation of reusable components in algorithms, leading to efficient code reuse.
- Modularity: Abstraction encourages a modular approach to algorithm design. Modular algorithms are efficient to maintain, debug, and update.
- Scalability: Algorithms built using the principle of abstraction are easily scalable, thus enhancing their efficiency to manage larger inputs or more complex problems.
Enhancing Algorithms with Abstraction Techniques
Heightening your command over abstraction techniques isn't a one-size-fits-all approach. It takes practice and a transformation of perspective to design abstract algorithms effectively. Here are key steps to enhancing algorithms by leveraging abstraction techniques: - **Understand the Problem Thoroughly:** Before diving into abstraction, make sure to comprehend what the problem entails at its core. This understanding makes it easier to sift out unnecessary details and focus on what is important. - **Decompose the Problem:** Break the problem down into smaller, manageable parts. This simplification often results in more effective abstraction and cleaner algorithms. - **Identify Useful Abstractions:** Determine what abstractions can be applied to the problem or specific parts of the problem. The right abstraction can often solve a problem very efficiently. - **Implement the Abstraction:** You can implement the abstraction in pseudocode or actual code, ensuring that the abstraction accomplishes the intended task efficiently. - **Validate and Refine:** Finally, validate your abstract algorithm to be sure it indeed solves the problem, and refine it as necessary.Imagine a program that calculates the distance between two points on a map. Instead of considering the full array of details inherent in a map, the algorithm abstracts the map into simply a coordinate plane, and the distance calculation becomes merely a case of applying the distance formula \( \sqrt{(x_2-x_1)^2+(y_2-y_1)^2} \).
The ultimate aim of abstraction in algorithm design is to blend efficiency, readability, and reusability, ultimately creating algorithms that are easy to understand, manage and are efficient. Effortlessly navigating between high-level problem understanding and lower-level implementation details is the true beauty of mastering abstraction in algorithm design.
Mastering Advanced Algorithm Design
In the sphere of Computer Science, algorithm design stands out as a core competency. As you delve deeper into this subject matter, one can't help but appreciate the art and science that underpin the creation of sophisticated algorithms. It's this unique amalgamation that paves the way for advanced algorithm design, a skill that holds the key to solving complex computational problems efficiently and effectively. Whether it's fine-tuning existing algorithms or innovating new ones, mastering advanced algorithm design is about leveraging complex data structures, mathematical tools and inventive thinking.Pushing Boundaries with Advanced Algorithm Design
Advanced algorithm design represents a quantum leap from the basics, heralding a new era of computational possibilities that are both complex and intriguing. This level unfolds new architectural nuances of algorithms and exposes their potential to solve advanced problems. Often, you'll find that these advanced designs are the key to optimal solutions or indispensable for addressing problems that are computationally more rigorous. To push boundaries with advanced algorithm designs, understanding the following essential aspects is vital:- Complex Data Structures: Empower your algorithms to handle large amounts of data or perform complex operations by learning to use trees, heaps, and graphs effectively.
- Greedy Algorithms: These algorithms take the best option available at each step, hoping for an optimal solution.
- Dynamic Programming: This technique is used for solving complex problems by breaking them down into simpler overlapping subproblems.
- Divide and Conquer: This approach involves dividing a problem into two or more identical subproblems until they become simple enough to solve directly.
Practical Insights: Advanced Algorithm Design Examples
Identifying theoretical aspects of advanced algorithm design is just half the journey. What breathes life into these theoretical concepts are practical applications and examples. Let's explore three examples demonstrating the practical insights of advanced algorithms:Consider an advanced application where you need to find the shortest path in a graph where edges represent cities and their costs. A suitable solution for this would be the Dijkstra's algorithm. The algorithm essentially uses the 'greedy' approach, always choosing the path with the least cost.
Another scenario is arranging a series of given tasks such that each task only starts after its dependent tasks are completed, and you want to complete all tasks in the least time possible. For this, you can leverage a 'Topological Sort' using a directed acyclic graph.
A third example is when you're provided with various items each with a specific weight and value, and you have to determine the maximum total value you can get. Here, you can use a 'Knapsack' dynamic programming algorithm to solve this problem.
How Advanced Algorithm Design Techniques Improve Efficiency
Advanced algorithm design techniques bring impressive improvements in efficiency by optimising compute power and reducing the time it takes to solve complex problems. Consider the simple 'Greedy' technique. It makes optimal choices at every stage in the hope that these local optimal solutions will result in a global optimum. This nature of greedy algorithms makes them not only efficient but also easy to implement.A Greedy Algorithm is a simple, intuitive algorithm used in optimization problems, where it makes the locally optimal choice at each stage with the hope that these local choices will lead to a global optimum.
function fib(n) if n < = 0 return n else if value[n] is already calculated return value[n] value[n] = fib(n-1) + fib(n-2) return value[n]In this 'Fibonacci Sequence' dynamic programming example, instead of calculating the same values repeatedly, these values are stored and reused, leading to significant time savings. Contrasting these techniques with naive algorithms, advanced techniques often outperform in terms of time complexity, with many capable of executing tasks in polynomial or even logarithmic time, making them the preferred way to tackle hard computational challenges.
Exploring Further in Algorithm Design
Taking your intrigue with algorithm design a step further, you can fully explore the realm of this dynamic subject area, uncovering its numerous intricacies and boundless possibilities. The landscape of algorithm design reveals a rich tapestry, woven with layers of profound concepts, principles, practical applications, advancements, and a future full of promise. It beckons you with complex challenges, imaginative solutions, and cutting-edge computational paths. The golden thread running through this exploration is the continuous advancement in algorithm design techniques. This journey of exploration is sure to broaden your logical thinking, creativity, and problem-solving skills.Future Prospects in Algorithm Design
Peering into the future of algorithm design can be fascinating and transformative. From the burgeoning field of artificial intelligence to the leaps in quantum computing, the future of algorithm design appears expansive and promising.The coming years may well witness a surge in learning-based or self-evolving algorithms, enabled by advancements in machine learning and artificial intelligence. Such self-learning algorithms can adapt and tailor their operations based on inputs and feedback, significantly enhancing efficiency and decision-making capabilities.
Algorithm | Use Case | Advantages |
Shor's Algorithm | Factoring large numbers | Exponential speedup over best-known classical algorithms |
Grover's Algorithm | Unstructured search | Quadratic speedup over classical search algorithms |
The Impact of Algorithm Designing on Future Technology
Algorithm design as a field exerts enormous influence on future technology scenarios. Algorithmic advancements can shape new horizons, transcending existing technology paradigms and introducing unprecedented capabilities. A significant facet of this impact is the development of highly efficient algorithms, which could redefine the existing benchmarks of computational efficiency and scalability. One such realm that's being revolutionised by algorithmic advancements is data analysis and processing. The proliferation of big data generated by various sectors like healthcare, banking, retail, and social media necessitates the evolution of sophisticated algorithms capable of processing massive volumes of data, extracting meaningful insights and drawing precise conclusions. On another front, machine learning algorithms are driving the surge in artificial intelligence applications, ranging from image recognition and natural language processing to autonomous vehicles and advanced robotics. These shifts hold the potential to dramatically alter how societies function — from how industries operate and governments work, to even how daily life unfolds. Indeed, the artfully designed algorithms hold the promise to turn previously unimaginable technological marvels into reality.Challenging Yourself: Problems for Advanced Algorithm Design Practice
Challenge can be the catalyst to higher learning and honing of skills. As you delve deeper into advanced algorithm design, practical problem-solving provides invaluable learning experiences. Here are some problems that you can consider for practice:- Travelling Salesman Problem: Starting at a city, you have to find the shortest path that enables the salesman to visit all cities exactly once and return to the starting city.
- Graph Colouring Problem: Determine the minimum number of colours needed to colour a graph such that no two adjacent vertices share the same colour.
- Bin Packing Problem: Pack objects of different volumes into a finite number of bins in a way that minimises the number of bins used.
Designing algorithms - Key takeaways
- Time complexity describes the amount of computer time taken by an algorithm to run, while space complexity represents the amount of memory space that the algorithm needs to run to completion.
- Algorithm efficiency is typically expressed using Big-O notation, which describes the upper bound of time or space requirements as a function of the size of the input.
- Designing efficient algorithms requires steps such as defining the problem, formulating the algorithm, writing the pseudocode, analyzing the algorithm for correctness and efficiency, and refining the algorithm to improve its efficiency, if required.
- Abstraction in algorithm design is the process of simplifying complex systems by breaking them down into components essential for problem-solving, while ignoring unnecessary details. It can aid in creating efficient and reusable algorithms.
- Advanced algorithm design leverages complex data structures, mathematical tools and inventive thinking for solving complex computational problems efficiently.
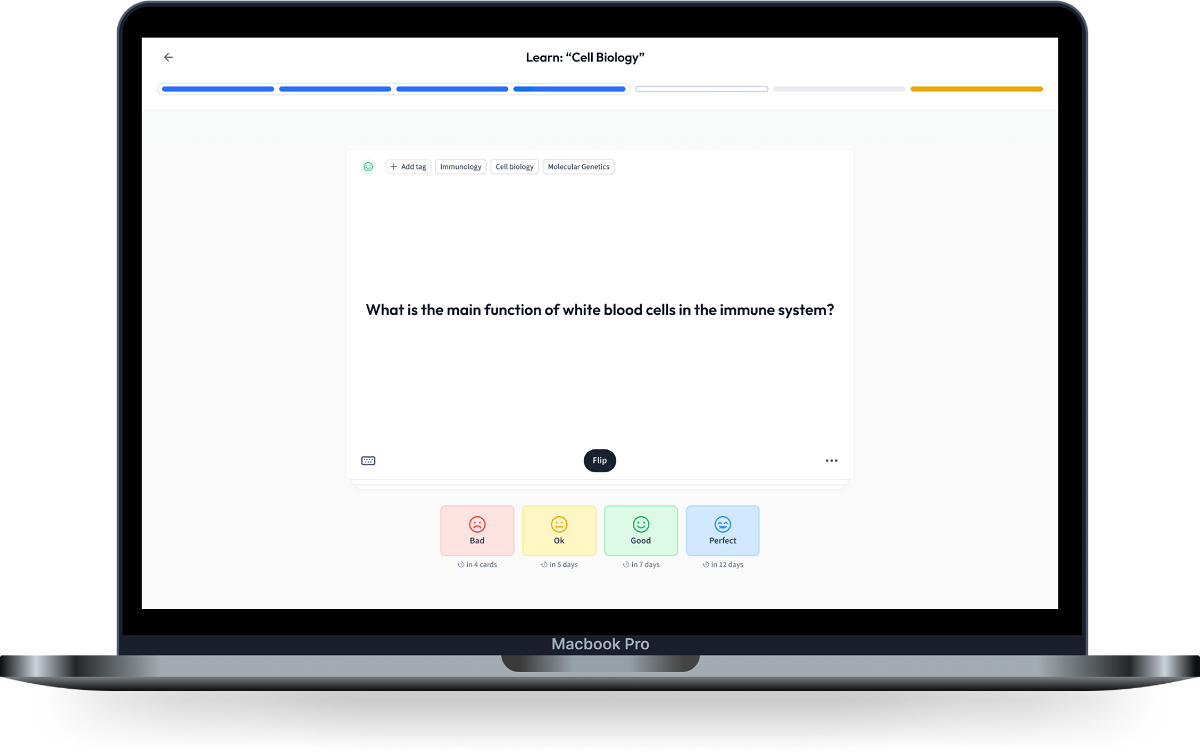
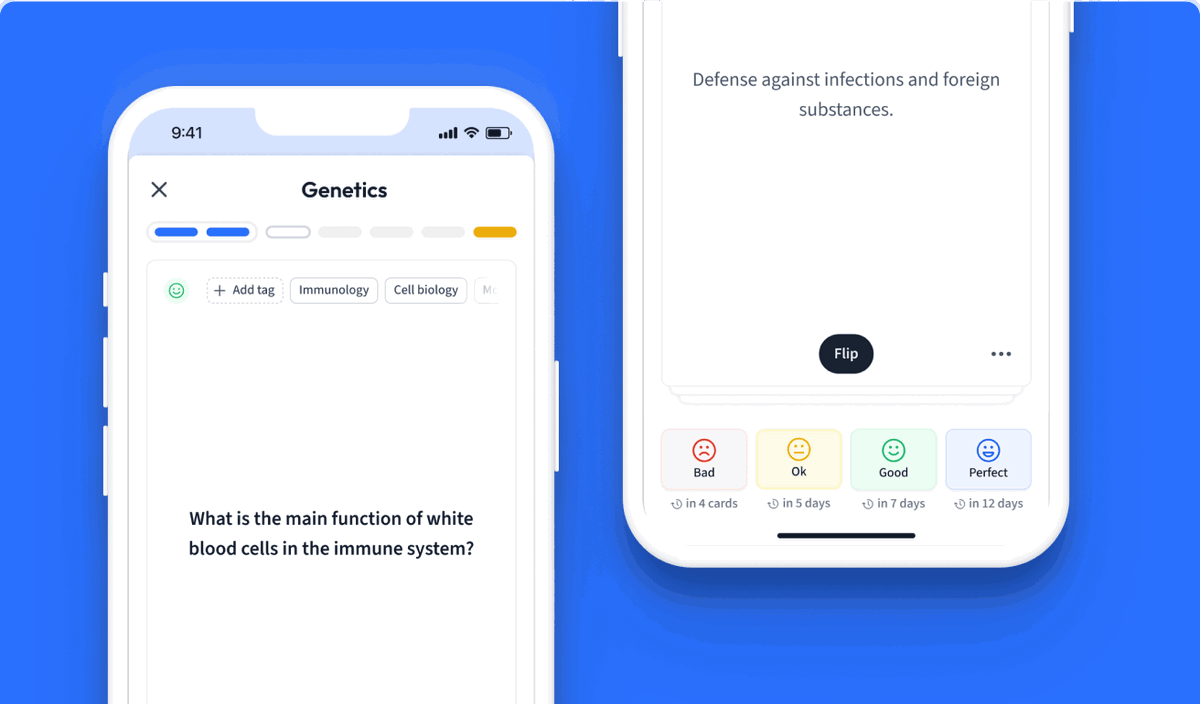
Learn with 15 Designing algorithms flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Designing algorithms
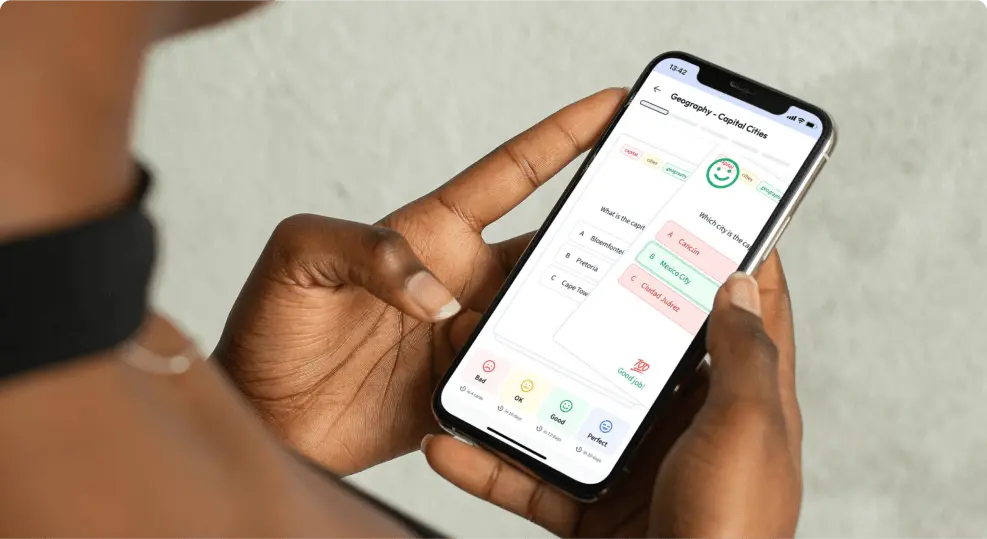
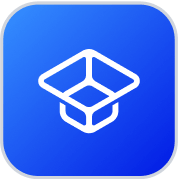
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more