Understanding the Set Cover Problem
Getting to grips with the Set Cover Problem is a fundamental aspect of computer science, particularly in relation to algorithmic theory and design. But don't worry if you're just starting out, this article will break it down for you!
What is Set Cover Problem: An Introduction
In layman’s terms, the Set Cover Problem, or SCP, is an essential issue in computational theory that you’ll encounter. It essentially involves a given set, and a list of subsets of that set, and the task is to find the smallest sub-collection of these subsets that covers the entire set.
Set Cover Problem in Computer Science: A Closer Look
In mathematical terms, given a universe
U = {u1, u2, ..., un}and a family of subsets of
U, F = {S1, S2, ..., Sm}, a cover is a subset of
Fthat covers all elements of
U.
Consider a set
U = {1, 2, 3, 4, 5}and a family of subsets
F = {{1, 2, 3}, {2, 4}, {3, 4}, {4, 5}}. Here, the set cover is
F' = {{1, 2, 3}, {4, 5}}as it includes all elements of
Uwith the fewest subsets.
Key Elements of the Set Cover Problem
To thoroughly understand the Set Cover Problem, you have to grapple with some key elements.
- Universe(
U
): The main set to be covered - Family(
F
): The list of subsets - Cover(
F'
): The sub-collection ofF
covering the universe
Importance of Set Cover Problem in Algorithms
In the realm of algorithms, the Set Cover Problem is tremendously important. It is classified as an NP-Hard problem in computational complexity theory, meaning there is no known algorithm capable of solving all instances of the problem quickly (in polynomial time).
The Set Cover Problem is often used as a benchmark for hardness in complexity theory studies, and its solutions have applications in areas such as network design, bioinformatics, and logistics.
The algorithm that is typically used to provide an approximate solution to the SCP is a Greedy Algorithm.
Here is a pseudo code for SCP using a Greedy Approach.
GreedySCP(U, F) 1 While (U is not empty) 2 Pick the subset S in F that covers the most elements in U 3 Remove the elements in S from U 4 Remove S from F 5 Return the selected subsetsThe basic idea of the Greedy Algorithm is to always select the subset that covers the largest number of uncovered elements.
Techniques and Algorithms of the Set Cover Problem
Various techniques and algorithms can be used to address the Set Cover Problem. These algorithms predominantly include the greedy approach and dynamic programming. Getting a sound understanding of these methods not only assists in tackling the problem effectively but also enhances your theoretical knowledge of computer science and algorithms.
Set Cover Problem Algorithm: In-depth Explanation
The Set Cover Problem can be tackled with multiple algorithms. Each algorithm has its unique approach, making it suitable for specific scenarios with different input parameters. Let's dive deep into how these algorithms work.
A common method used is the Greedy Algorithm approach, which works by selecting the largest unused set at each step. This method often returns good results as it tends to cover the maximum elements at every step.
A more informed approach to solving the Set Cover Problem involves using Linear Programming Relaxation. Linear programming, or LP, is a method of optimising a system that's described using linear relationships. However, transforming the Set Cover Problem into an LP algorithm and using the LP-solution to derive a solution for the SCP can be quite complex.
The Primal-Dual Algorithm is another approach utilised for solving the Set Cover Problem. The basis of this algorithm is to construct a feasible solution of the primal problem and the dual problem with an equal objective value.
A more complex way of solving the Set Cover Problem is the Dynamic Programming approach. Mostly used in situations where the universe is small, this algorithm can provide an exact solution but can also be computationally intensive.
Dynamic Programming Approach to the Set Cover Problem
Unlike the Greedy Algorithm, the dynamic programming approach can provide an exact solution to the Set Cover Problem. It is based on the principle of optimality which posits that an optimal policy has the property that whatever the initial state and decisions are, the remaining decisions must constitute an optimal policy concerning the state resulting from the first decision.
In terms of dynamic programming, the algorithm stores solutions to sub-problems, and these solutions are then used to construct solutions to larger problems. Consider a universe \( U = {u_{1}, u_{2}, ..., u_{n}} \), a dynamic programming approach would iterate over all subsets of \(U\), starting from smaller ones and gradually building up to the whole set.
Consider a pseudo code for the dynamic programming approach to SCP:
DP_SCP(U, F) 1 Create a table dp, where dp[i][S] stores minimum number of sets from first i sets following rules of SCP 2 Start by sorting the subsets according to size 3 Start filling dp[][] in bottom up manner i) For every subset 'j', find minimum nubmer of sets from first 'i' sets chosen, following rules of SCP 4 The entry dp[n][S] tells the SCP for S from subset 1 to n
Set Cover Problem Greedy Algorithm: A Comprehensive Guide
Generally, Greedy Algorithms are simple, straightforward, and short-term-goal-oriented. They follow the problem-solving heuristic of making the locally optimal choice at each stage, in hopes that these local optimums will lead to a global optimum.
When it comes to the Set Cover Problem, the short-term goal is to, at each step, pick the subset which contains the maximum number of uncovered elements. Here’s how it works:
Starting with a universe 'U' and a family of subsets 'F', at each step, select the subset that contains the maximum number of uncovered elements from 'U'. This subset is then added to the cover, and its elements are removed from 'U'. This process continues until 'U' becomes empty. Thus, the cover obtained this way is the output of the Greedy Algorithm.
Here is a pseudo code for the greedy approach to SCP:
GreedySCP(U, F) 1 While (U is not empty) 2 Pick the subset S in F that covers the most elements in U 3 Remove the elements in S from U 4 Add S to cover 5 Return the coverThe Greedy Algorithm's strategy is always to pick the choice that seems best at the moment to solve the problem. In the case of SCP, that means picking the subset that covers the maximum number of uncovered elements from 'U' at each step.
Set Cover Problem in Various Programming Languages
Like any computational problem, the Set Cover Problem can also be solved in various programming languages. Each language, with its distinct features, provides a unique approach to the problem. This section will focus on how to tackle the Set Cover Problem using Python, a powerful and highly readable language widely used in data analysis and algorithmic problem solving.
Solving Set Cover Problem in Python
Python, known for its readability and straightforward syntax, is an excellent language for learning and implementing complex algorithms. Python’s robust collection of libraries and data structures makes it an excellent choice for tackling the Set Cover Problem. Notably, you can utilise the data structures like ‘set’, ‘list’ and ‘dictionary’ to store and manipulate the information required to solve the Set Cover Problem. In Python, these data structures provide efficient ways to perform operations like union, intersection, and difference that are crucial to solving the Set Cover Problem.
Here’s the approach to tackle the Set Cover Problem using Python:
- Start by defining the universe and the family of subsets. You can define these using the 'set' data structure in Python.
- Next, create an empty set to store the cover.
- Now, you enter a loop that continues until the universe is empty. Inside the loop, at each step, use Python's built-in functionality to find the subset that has the maximum intersection with the universe.
- Add this subset to the cover, and remove its elements from the universe.
- Continue this process until the universe is empty, at which point, the set 'cover' contains the minimum sub-collection of subsets that cover the universe.
A Practical Example of Set Cover Problem in Python
For a more concrete understanding, let's take a look at a practical example of solving the Set Cover Problem in Python.
# Universe U = set(range(1, 6)) # Family of subsets F = [set([1, 2, 3]), set([2, 4]), set([3, 4]), set([4, 5])] # Set to store the cover cover = set() while U: # Find the subset with maximum intersection with U subset = max(F, key=lambda s: len(s & U)) # Add the subset to the cover cover.add(F.index(subset)) # Remove the elements of subset from U U -= subset # Print the cover print(cover)When you run this code, it returns
{0, 3}, which represents the indices of the subsets
{1, 2, 3}and
{4, 5}in the family F. These subsets form the minimum cover of the universe.
Weighted Set Cover Problem: An Advanced Technique
As you explore further into the realm of the Set Cover Problem, you will encounter more complex and advanced versions of the problem. Specifically, the Weighted Set Cover Problem is an extension of the Set Cover Problem that introduces an additional layer of complexity.
Unlike in the standard set cover problem, where all subsets are considered equal, in the Weighted Set Cover Problem each subset is assigned a weight or cost. The goal, in this case, is to find a cover that not only includes all elements of the universe but also does so with the minimum total weight.
The Weighted Set Cover Problem, despite being more complex, can be tackled using similar algorithms as the regular Set Cover Problem. The Greedy Algorithm, for instance, can be adapted to select at each step, not the largest subset, but rather the subset that has the most uncovered elements per unit cost. Other algorithms and techniques such as Linear Programming and Primal-Dual Schema can also be used for the Weighted Set Cover Problem.
Minimum Set Cover Problem: Understanding the Basics
Another interesting variation of the problem is the Minimum Set Cover problem. The terminology can sometimes be confusing, because "Minimum Set Cover" can refer to one of two distinct, although related, problems.
In one interpretation, the "Minimum Set Cover" problem is simply another name for the standard Set Cover Problem. This is because the Set Cover Problem seeks to find the minimum number of subsets that cover the universe.
In other contexts, however, "Minimum Set Cover" refers to a distinct problem: Given a set and a family of subsets, find the subfamily with the smallest total cardinality (i.e., the sum of the sizes of all subsets in the subfamily) that covers the entire set. While the Set Cover Problem focuses on covering the universe with the fewest subsets, the Minimum Set Cover Problem seeks to do so using the fewest elements.
Regardless of the variation, sophisticated algorithms and techniques, including those adapted from solutions to the standard Set Cover Problem, are available for tackling these problems.
Applications of Set Cover Problem in Computer Science
The Set Cover Problem (SCP) is a classical question in computer science, operations research, and complexity theory. It has numerous applications in various fields of computer science, such as data mining, network design, and resource allocation. Understanding SCP can, therefore, serve as an introduction to many advanced topics in computer science.
Set Cover Problem Techniques in Computer Science
The Set Cover problem is a representative of a class of problems in computer science called NP-Complete problems. These problems share the characteristic that no known algorithm can solve them quickly, and it's a major open question in theoretical computer science whether or not a quick solution exists.
Despite this difficulty, solutions to the Set Cover Problem are needed in many algorithms and applications. Therefore, a variety of techniques have been developed to find approximate solutions. The most common technique is the Greedy Algorithm, which always chooses the next option that offers the most immediate benefit. However, in the case of the SCP, the Greedy Algorithm does not always lead to the optimal solution.
Another technique is to use Linear Programming (LP), an approach where the problem is represented as a set of linear equations and then solved. However, LP is also an approximation technique when applied to SCP, as the solution it provides is fractional, while the SCP requires an integer solution.
Despite the limitations of these techniques, they have proven to be useful in various applications within computer science. This is because efficient approximate solutions are often good enough in practice.
Real-world Examples of Set Cover Problem in Algorithms
There are numerous real-world applications of the Set Cover Problem and its associated algorithms. These applications span all areas of computer science, including software engineering, data analysis, network routing, and machine learning, among others.
- Data Mining: In data mining, SCP can be deployed in feature selection. The goal is to find the smallest possible subset of features that still accurately predicts the target outcome. This falls into the category of SCP, where each feature set represents an element of the universe and covers a specific subset of the target outcome.
- Distributed Computing: In distributed computing, the SCP can be utilised in group communication system where the aim is to minimise the number of multicasts to deliver a message to a group of users.
- Wireless Networks: SCP applications in wireless networks include channel assignment and power control, where the intention is to minimise interference by connecting each device to the least number of channels or power necessary to maintain network connectivity.
Benefits and Challenges of the Set Cover Problem Approach
The primary advantage of the Set Cover Problem approach is its broad applicability in various fields of computer science and operations research. This problem is fundamental in nature and understanding it allows one to tackle a wide range of related problems in these fields.
Algorithms for solving the SCP, such as the Greedy Algorithm, are also relatively simple to implement. They require a basic understanding of data structures and algorithmic principles, making them accessible to beginners in computer science and algorithm design.
On the other hand, one of the main challenges when dealing with the SCP is the time complexity issue. As the SCP belongs to the class of NP-Complete problems, there isn't a known algorithm which can solve the problem in polynomial time. This makes the SCP quite resistant to standard techniques, and often necessitates an in-depth understanding of advanced topics in computational complexity, such as P vs NP and approximation algorithms.
Another challenge lies in finding an optimal solution for SCP. While greedy algorithms can generally provide a near-optimal solution, finding the globally optimal solution can be significantly more challenging, especially for large instances of the problem.
Despite these challenges, the benefits and wide-ranging applications of understanding and tackling the SCP makes it a worthwhile endeavour for any budding or seasoned computer scientist or algorithm designer.
Set Cover Problem - Key takeaways
- Key elements of the Set Cover Problem include the Universe (main set to be covered), Family (list of subsets), and Cover (sub-collection of Family that covers the Universe).
- The Set Cover Problem is classified as an NP-Hard problem in computational complexity theory, meaning there's no known algorithm that can solve all instances quickly.
- Common methods for solving the Set Cover Problem include the Greedy Algorithm, Linear Programming Relaxation, Primal-Dual Algorithm, and Dynamic Programming.
- The Greedy Algorithm approach selects the largest unused set at each step, while Dynamic Programming approach provides an exact solution by constructing solutions to sub-problems.
- The Set Cover Problem has many real-world applications in areas like network design, bioinformatics, logistics, data mining, distributed computing, etc.
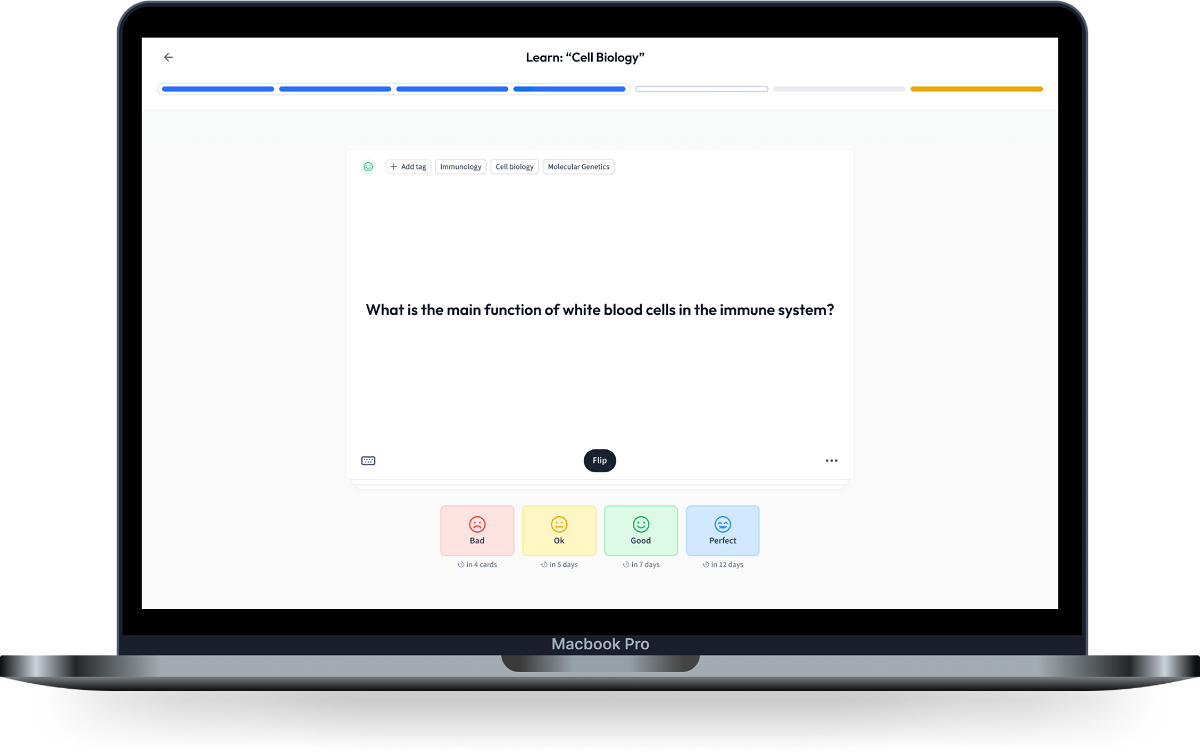
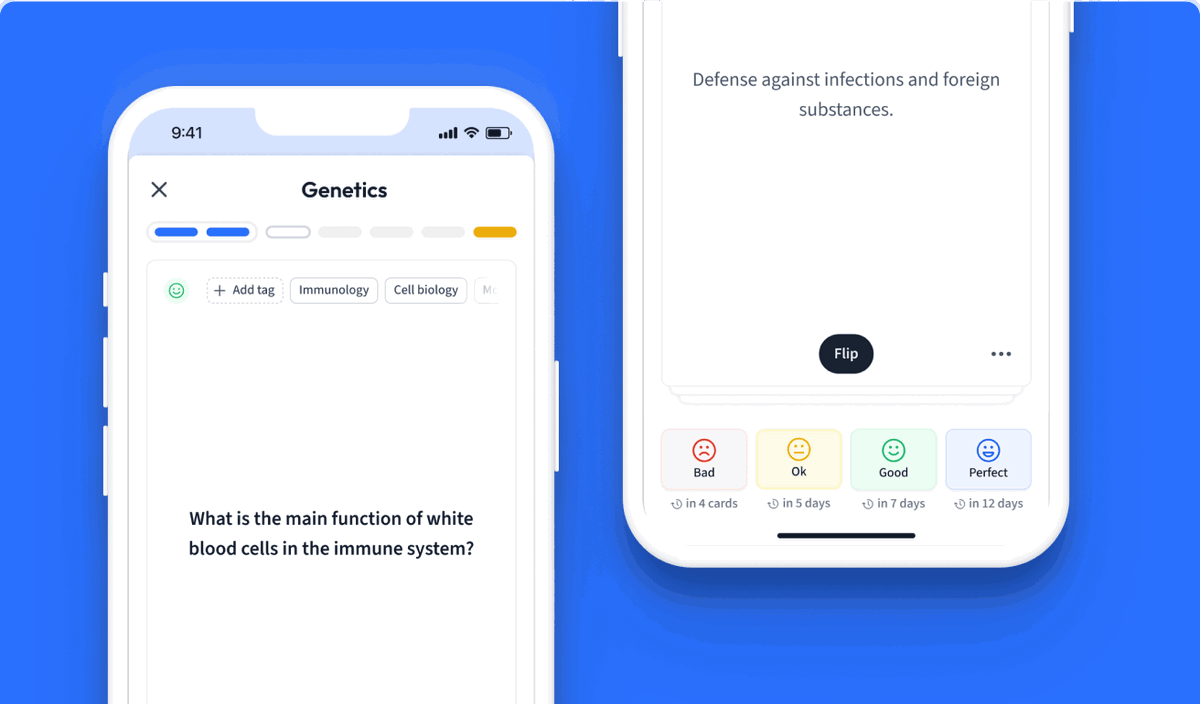
Learn with 12 Set Cover Problem flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Set Cover Problem
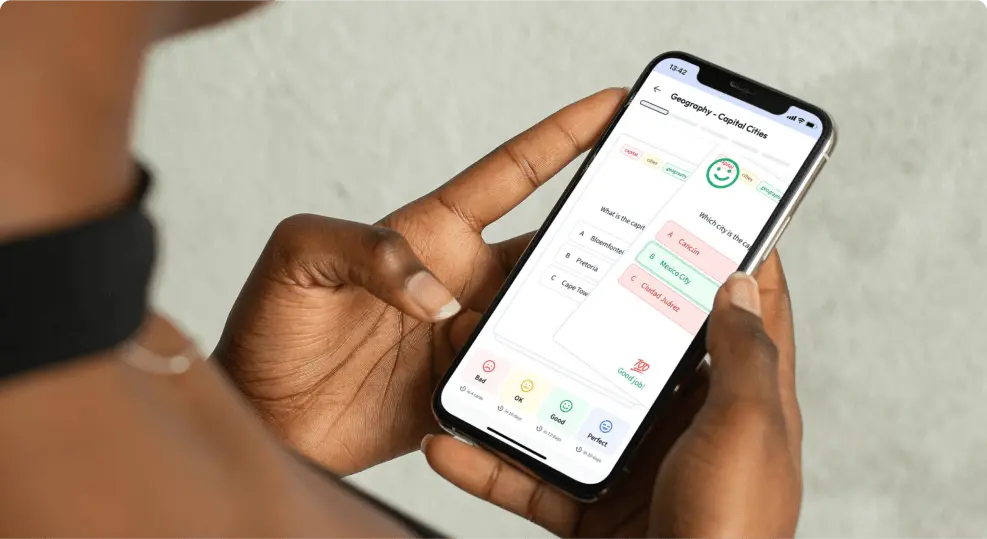
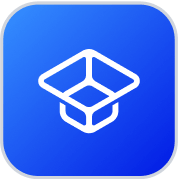
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more