Understanding the Shell Sort in Computer Science
The Shell Sort is an efficient and unique algorithm for data sorting in the world of computer science. Offering a unique twist on the insertion sort, it can provide superior performance while operating on close to linear time complexity with certain incremental sequences. The allure of Shell Sort in Computer Science lies in this very property — it achieves much better time complexity than many traditional comparison-based sorting algorithms.Precise Definition: What is the Shell Sort Algorithm?
The Shell Sort algorithm, named after its creator Donald L. Shell, is a sorting algorithm that starts by sorting pairs of elements spaced apart in an array or list — these spacings are known as gaps or intervals. To understand Shell Sort better, it is first essential to comprehend its foundational concept - the idea of "gaps".
Illustrating the Shell Sort with Examples
To facilitate a better understanding of how Shell Sort works, let's dive into some illustrative examples.Shell Sort Example: Simple Case Study
Let's consider a simple array of numbers - [9, 8, 3, 7, 5, 6, 4, 1]. If we were to sort this array using Shell Sort, we would first define a gap. Let's say, we choose a gap of 4. Consequently, Shell Sort would sort elements at every fourth position.
[9, 5] [8, 6] [3, 4] [7, 1]After sorting these, the array would look as follows:
[5, 6, 3, 1, 9, 8, 4, 7]We would then reduce the gap by half to 2 and sort the elements at every second position. This process repeats until the gap is reduced to 1, at which point, the array should be sorted.
The Practical Application: Shell Sort in Java
Shell Sort finds widespread use in practice, thanks to the straightforward nature of its implementation across programming languages. Here is an illustrative implementation of Shell Sort in Java:public class ShellSort { public static void sort(int array[]) { int n = array.length; for (int gap = n / 2; gap > 0; gap /= 2) { for (int i = gap; i < n; i++) { int key = array[i]; int j = i; while (j >= gap && array[j - gap] > key) { array[j] = array[j - gap]; j -= gap; } array[j] = key; } } } }
Analysing the Shell Sort Algorithm's Efficiency
In the world of computer science, the efficiency of a sorting algorithm is measured primarily by its time complexity. Shell Sort's time complexity depends on the gap sequence used — the complexity ranges from \(O(n^{1.5})\) to \(O(n \log n)\).An Insight into the Shell Sort Time Complexity
Understanding the time complexity of the Shell Sort algorithm involves considering the gaps used in the sorting process. The worst-case time complexity of Shell Sort is typically \(O(n^2)\), based on the nature of the gaps utilised. However, by using an optimised gap sequence, the time complexity can notably be improved. Some common sequences include:- Original Gap Sequence by Shell: \( n/2, n/4, ..., 1 \)
- Knuth's Sequence: \( (3^n-1)/2 \)
- Ciura's Sequence: 1, 4, 10, 23, 57, 132, 301, 701, 1750, and so on.
On a fascinating note, despite in-depth research, the question on which gap sequence provides the best time complexity remains open for discussion in computer science.
Ways to Optimise the Shell Sort Algorithm
The primary way to optimise the Shell Sort algorithm is through careful selection of the gap sequence. Choosing an optimal gap sequence can significantly enhance the algorithm's running time. However, optimising the Shell Sort algorithm can take a more profound nuance. An additional factor, often undermined, lies in the nature of the data being sorted. For nearly sorted data, Shell Sort operates close to \(O(n)\), showing its adaptive nature.Practical Steps for Optimizing Shell Sort
Optimising the Shell Sort algorithm can be a process of trial and error, but there are several practical steps you can apply:- Using an empirically determined sequence: The Ciura gap sequence has been observed to provide good results in both theoretical and practical evaluations.
- Modifying the sequence based on the nature of the data: For arrays with specific characteristics, certain custom gap sequences may bring about better performance.
- The adaptive nature of Shell Sort makes it highly suitable for nearly sorted data. Keeping this scenario in mind, Shell Sort can be the chosen algorithm for practical applications where the data is nearly sorted.
Debating the Characteristics of Shell Sort
When diving into the distinct world of the Shell Sort algorithm, there are several distinctive characteristics which set it apart from other sorting algorithms. Key points of debate often revolve around its stability, the optimisation of its time-efficient nature, and the unique pros and cons it introduces to the field of data sorting.Is Shell Sort Stable? An Exploration
Looking closely at the Shell Sort algorithm, it becomes apparent that the algorithm is, notably, not stable. Stability in a sorting algorithm refers to the ability to maintain the original relative order of equal elements in the sorted output. Unstable sorts, such as Shell Sort, do not guarantee this, as equal elements can swap positions during the sorting process.Consider an array of pairs (a, b), where 'a' is the major key and 'b' is the minor key - for example, [(2,1), (1,2), (1,1), (2,2)]. Now, if we wish to sort this array using a stable sort, the minor keys maintain their relative order for equal major keys. Hence, after sorting based on the major key 'a', a stable sort yields [(1,2), (1,1), (2,1), (2,2)].
However, with an unstable sort like Shell Sort, the relative order may not be preserved. Using Shell Sort could result in the output [(1,1), (1,2), (2,2), (2,1)], where the order of pairs with equal major key '1' has changed.
Breaking Down the Pros and Cons of Shell Sort Algorithm
Like any algorithm in computer science, the Shell Sort algorithm brings along both advantages and disadvantages. Understanding these pros and cons can guide you in making a well-informed selection of the sorting algorithm best fit for your specific needs.Advantages of Using Shell Sort
The Shell Sort algorithm introduces several notable benefits:- Efficiency: Shell Sort offers relatively efficient performance with time complexity that can reach \(O(n \log n)\) with an optimal gap sequence.
- Adaptability: It is an adaptive sorting algorithm that shows superior efficiency when the input list is partially sorted.
- Simplicity: The algorithm is simple to understand and implement, making it a popular choice among programmers.
Disadvantages of Using Shell Sort
However, there are also certain limitations of using Shell Sort:- Unstability: As discussed in-depth above, the Shell Sort algorithm is not stable, and it may not preserve the original order of equal elements in the sorted output.
- Dependence on Gap Sequence: The efficiency of Shell Sort depends critically on the choice of gap sequence, which can lead to inconsistent performance.
Shell Sort - Key takeaways
- The Shell Sort is an efficient algorithm for data sorting that achieves better time complexity than many traditional comparison-based sorting algorithms.
- The Shell Sort algorithm sorts pairs of elements spaced apart in an array or list (gaps). The gap size reduces at each pass until it reaches one.
- Shell Sort can be implemented efficiently across different programming languages, such as Java, where it sorts an array by iterating over it in decreasing gaps until the array is fully sorted.
- The time complexity of the Shell Sort algorithm depends on the gap sequence used, ranging from \(O(n^{1.5})\) to \(O(n \log n)\). By using an optimised gap sequence, the time complexity can notably be improved.
- The Shell Sort algorithm is not stable as it does not guarantee to maintain the original relative order of equal elements in the sorted output. Additionally, its performance highly depends on the choice of gap sequence.
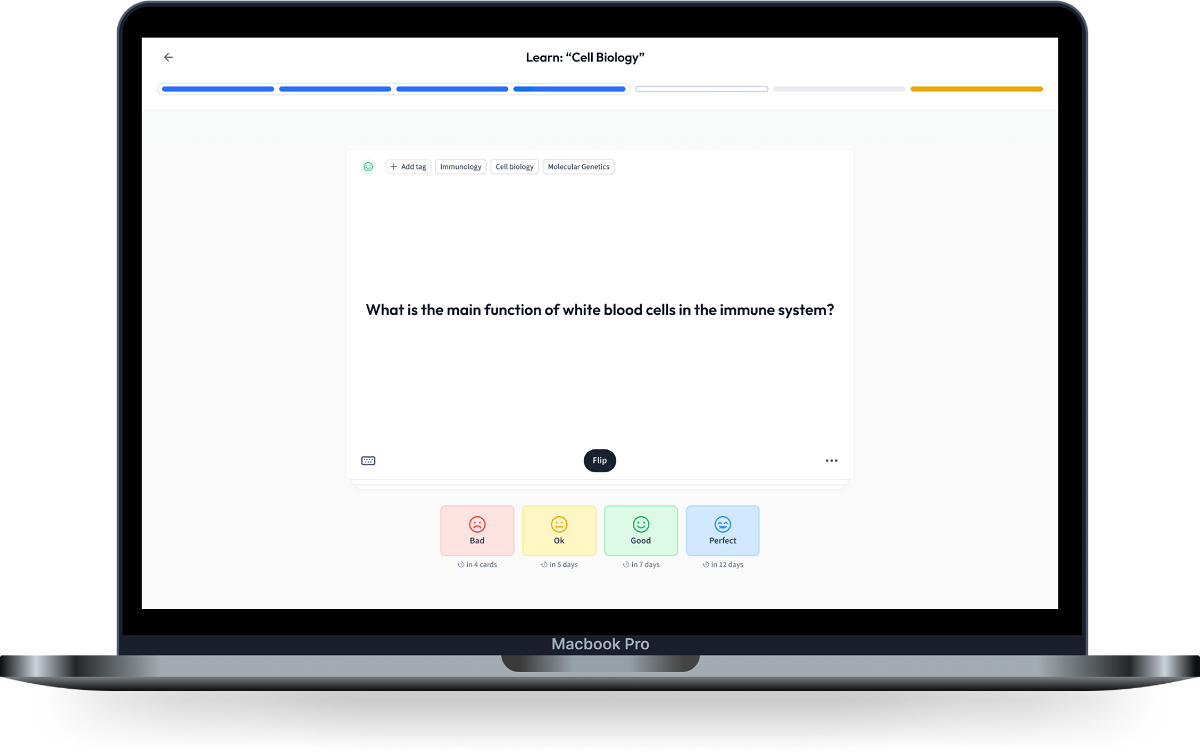
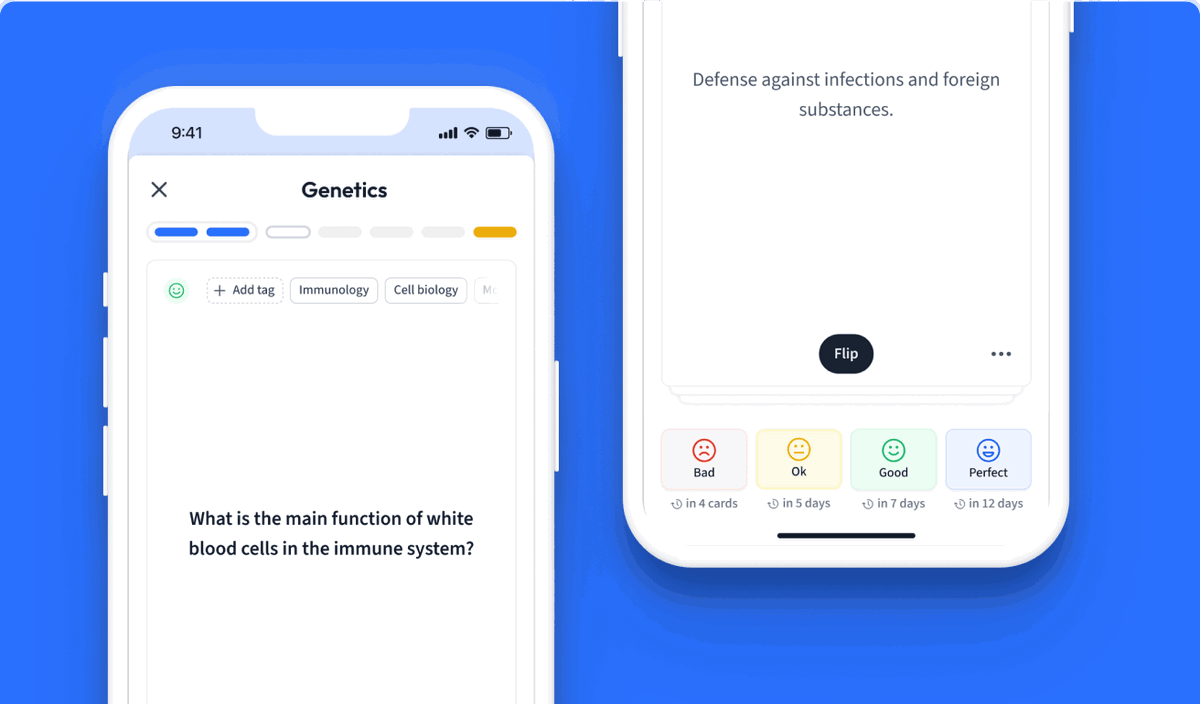
Learn with 12 Shell Sort flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Shell Sort
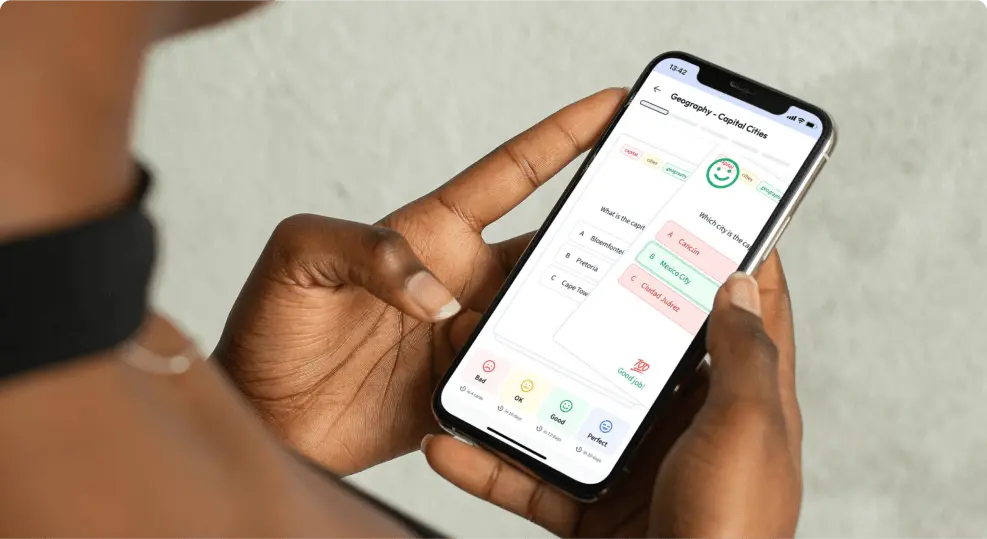
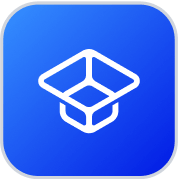
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more