Understanding Pseudocode in Computer Science
Decoding the mysteries of computer science begins with understanding essential components like pseudocode. Characterised as a user-friendly method of expressing algorithms, pseudocode plays a pivotal role in programming and coding realms.
What is Pseudocode: An Overview
Pseudocode, as the prefix 'pseudo-' suggests, isn't actual code. It's a plain language representation of the steps involved in an algorithm or a computer program. By facilitating easy communication of programmatic concepts and reducing the barriers of specific programming languages, it offers an agile way to describe and share algorithms.
Pseudocode: A verbose description of an algorithm or process that uses the structural conventions of programming languages but omits detailed sublanguage syntax.
The Importance of Pseudocode in Algorithm Development
In algorithm development, pseudocode serves as the blueprint that organises complex tasks into manageable, understandable steps. Its importance cannot be overstated, due to several key reasons:
- Appreciation of Logical Structures: Pseudocode helps you understand the logical sequence of execution, and promotes clear thinking and efficient problem-solving.
- Platform Independence: Pseudocode isn't tied to a specific programming language, making it universally understood by programmers regardless of their primary code languages.
- Smooth Transitions to Code: Once a pseudocode is devised, it's easier to translate it into actual code, irrespective of the programming language. It acts as the stepping stone towards creating effective programs.
As per the latest StackOverflow Developer survey, a substantial proportion of participants highlighted the usefulness of pseudocode for debugging and algorithm development purposes.
Pseudocode Syntax: Decoding the Language of Algorithms
While pseudocode does not adhere to the strict rules of programming, it does follow a structure similar to most programming languages. Common pseudocode syntax elements include:
IF-THEN-ELSE | Expressions for conditional instructions. |
FOR | Indication of repetitive loops. |
WHILE-ENDWHILE | Symbols denoting a block of commands to be executed until a certain condition is met. |
READ | Conducting an input operation. |
Performing an output operation. |
Example: Sorting an array using the bubble sorting algorithm.
FOR i = 1 to array length - 1 FOR j = 1 to array length - i IF array[j] > array[j+1] THEN swap array[j] with array[j+1] ENDIF ENDFOR ENDFOR
Pseudocode Techniques and Best Practices
As with code, making pseudocode readable and consistent is crucial. Here are some tips and best practices:
- Create Structured Flow: Pseudocode should represent a logical flow between various stages of the algorithm.
- Use Indentation: Correct indentation aids in visually demarcating blocks of related lines, improving readability.
- Use Expressive Variable Names: Variable names should be descriptive enough to infer their role in the algorithm.
- Keep Consistent Conventions: While there are no strict rules, keeping your syntax and naming conventions consistent throughout your pseudocode will enhance clarity.
Writing Pseudocode: A Comprehensive Guide
Developing a working algorithm is a significant aspect of computer science, yet writing pseudocode comes before this stage. A comprehensive guide to writing pseudocode will facilitate easy cross-over to various programming languages and empower you to tackle more complex problems.
How to Write Pseudocode: Step-by-Step
Devising pseudocode doesn't require extensive coding expertise but it does necessitate logical reasoning and a step-by-step approach. Here is a detailed guide to creating pseudocode, with a focus on clarity and structured thinking.
Step 1: Understand the Problem The first step is to comprehend the problem you are trying to solve. Identify what inputs are required and what outputs are expected. Step 2: Identify the Main Components Identify the algorithm's main components, which can include various operations like input, output, calculations, and conditional decisions. Step 3: Define the Program Flow Next, you need to define how these components interact. This includes determining the sequence of steps, any decision points, and possible iterative operations. Step 4: Write the Pseudocode Now it's time to pen the pseudocode. Remember to focus on logic rather than syntax.Example: Writing a pseudocode for an algorithm to add two numbers.
Read number1 Read number2 Sum = number1 + number2 Print Sum
Pseudocode Logic: Thinking Like a Developer
Creating effective pseudocode requires thinking like a developer, which is synonymous with logical reasoning and problem-solving-oriented thought.
Developer Mindset: A problem-solving approach that involves logical reasoning for breaking down complex tasks into smaller, manageable steps, and identifying efficient paths to solutions.
Example of a logical expression: If it is NOT raining AND you have an umbrella, then walk to the park.
Using Loops in Pseudocode for Iterations
Loops are an indispensable aspect of programming logic, and naturally, they play a significant role in pseudocode as well. Loops come into play when a particular operation needs to be repeated - whether for a specified number of times or until a specific condition is met.
FOR loop format: FOR variable = start TO end Code to execute ENDFOR
WHILE loop format: WHILE condition Code to execute ENDWHILE
Example: Writing pseudocode for printing the numbers 1 to 10.
FOR i=1 To 10 PRINT i ENDFOR
Pseudocode and Key Algorithms
Equipped with a solid grasp of pseudocode writing and its core concepts, now it's time to delve into some key algorithms commonly used in computer science. Knowledge of how these algorithms are depicted in pseudocode will further bolster your computational thinking skills, enabling you to tackle more complex computational problems.
Merge Sort Pseudocode: A Closer Look at Sorted Lists
The Merge Sort algorithm uses a divide-and-conquer approach to sort lists of elements, consequently demonstrating efficient performance even with large datasets.
Merge Sort: A sorting algorithm that divides a dataset into smaller chunks, sorts these chunks individually, and merges them to produce the final sorted list.
Procedure MergeSort(A, start, end) IF start < end mid = (start + end) / 2 MergeSort(A, start, mid) MergeSort(A, mid+1, end) Merge(A, start, mid, end) ENDIF EndProcedure Procedure Merge(A, start, mid, end) create array left from A[start..mid] create array right from A[mid+1..end] index = start WHILE left and right arrays both have elements IF first element of left <= first element of right A[index] = first element of left remove first element from left ELSE A[index] = first element of right remove first element from right ENDIF increment index ENDWHILE copy any remaining elements of left or right back into A EndProcedure
Binary Search Pseudocode: Navigating Large Data Sets
With large data sets, an efficient search technique becomes critically important. Binary Search is one such algorithm that reduces ‘search space’ by half with each iteration, making it exceptionally efficient for searching for an item in a large, sorted dataset.
Binary Search: A search algorithm that finds the position of a target value within a sorted array by halving the search interval with each step.
Procedure BinarySearch(A, n, x) Set lowerBound = 1 Set upperBound = n WHILE x is not found IF upperBound < lowerBound EXIT: x does not exist. compute midPoint roughly, not exceeding (upperBound + lowerBound) / 2 IF A[midPoint] < x Set lowerBound = midPoint + 1 ELSE IF A[midPoint] > x Set upperBound = midPoint - 1 ELSE EXIT: x is found at location midPoint ENDIF ENDWHILE EndProcedure
Dijkstra Algorithm Pseudocode: Optimising Path Finding
Dijkstra's algorithm, named after the Dutch computer scientist Edsger Dijkstra, is used to find the shortest path between nodes in a graph, which may represent, for example, road networks.
Dijkstra's Algorithm: An algorithm for finding the shortest paths between nodes in a graph, which may represent, for example, road networks.
Procedure Dijkstra(Graph G, Node s) Let Q be a priority queue FOR each vertex v in Graph G: distance[v] = ∞ previous[v] = undefined Q.add(v) ENDFOR distance[s] = 0 WHILE Q is not empty: u = node in Q with smallest distance remove u from Q FOR each neighbor v of u: alt = distance[u] + length(u, v) IF alt < distance[v]: distance[v] = alt previous[v] = u ENDIF ENDFOR ENDWHILE EndProcedure
Recursion in Pseudocode: An Intro to Recursive Calls
Recursion in programming is a powerful concept, where a function calls itself as part of its execution. Recursion aids in writing cleaner code, particularly when faced with problems that require multiple nested iterations.
Recursion: In computer science, recursion is a method of solving problems wherein a function, being executed, will call itself for execution.
Procedure Fibonacci(n) IF n<=0 RETURN 0 ELSE IF n==1 RETURN 1 ELSE RETURN Fibonacci(n−1)+Fibonacci(n−2) ENDIF EndProcedureThis pseudocode depicts how each recursive call chips away at a large problem (computing the nth Fibonacci number), turning it into a mix of smaller problems (computing the (n-1)th and (n-2)th Fibonacci numbers), and purely mechanical transformations. That is the essence of recursion: a big problem is divided into smaller easily solvable parts.
Applying Pseudocode in Real World Scenarios
Pseudocode's versatility and simplicity make it an invaluable tool in various domains, be it in the classroom of a computer science course, professional coding workshops, large scale software development, or even in explaining complex algorithms in research papers. Going beyond simple coding exercises, pseudocode has real-world application and serves as an integral bridge between problem formulation and getting your hands dirty with code.
Code vs Pseudocode: Understanding the Differences
In order to understand the practical role pseudocode plays in real-world scenarios, it's important to first distinguish it from actual code. While both serve to instruct a computer on what steps to take, there are some notable differences that can impact how, when, and why they transpire.
- Programming Language Specific: Code is written in a specific programming language, adhering to its strict syntax rules. Pseudocode, on the other hand, is essentially language-agnostic, focusing more on logic and less on syntax.
- Detail Level: When writing code, the smallest of details matter. Everything from the semicolon at an instruction's end to the type of brackets used can affect the overall result. Conversely, pseudocode is more akin to a high-level human language, and it focuses on the general idea or flow of the task, rather than minute programming details.
- Interpretability: Code, since it adheres to a specific syntax, needs compilers or interpreters to execute on a computer. Pseudocode, lacking a strict syntax, cannot be executed on any machine but is easier for humans to comprehend and understand.
- Platform Dependency: Many coding languages exhibit platform dependency - they behave differently on different operating systems. Pseudocode, being non-executable and illustrative, has no such dependencies.
Aspect | Code (Python) | Pseudocode |
Syntax | def add(a, b): return a+b |
PROCEDURE add(a, b): sum = a + b OUTPUT sum ENDPROCEDURE |
Execution | Yes | No |
Platform Dependency | Yes | No |
Detail Level | High | Low |
Pseudocode in the Classroom: Enhancing Learning and Understanding
The usage of pseudocode in the classroom setting, especially in computer science education, is incredibly beneficial. It serves as a stepping stone, easing the transition for students into the world of programming. Pseudocode provides a means for beginners to learn the logic of coding, without being overwhelmed by complex syntax.
Also, pseudocode allows students to concentrate on the art of problem-solving. By focusing more on procedural steps and less on language syntax, pseudocode fosters a more fundamental understanding of algorithms. It aids in breaking down complex coding problems into manageable parts, enhancing analytical thinking, and helps in grasping the art of efficient algorithm design. Moreover, since pseudocode is language-independent, it encourages versatile thinking. This agility proves valuable, as students progress to learn multiple programming languages through their courses.Pseudocode in Professional Settings: Streamlining the Development Process
In professional settings, pseudocode usage is not only encouraged but often considered a best practice. Writing pseudocode is a preliminary step in the software development process aimed at improving the efficiency and clarity of the final product.
Pseudocode serves as a blue print or skeleton for the final code. Clearly defined pseudocode allows developers to understand and agree upon how the program should work. Regardless of the language used to write the final code, the core logic remains the same, making pseudocode a useful tool for discussion and collaboration on a team project. What’s more, it facilitates effective debugging by enabling the programmer to reason out the logic before being engulfed by syntax or language dependent complexities. In conclusion, understanding and writing pseudocode is an essential skill for fledgling programmers and seasoned software developers alike. Whether or not you're a student on a learning journey or a professional aiming to streamline their software development process, there's no denying that pseudocode is an indispensable tool in the world of computing.Pseudocode - Key takeaways
- Pseudocode is a high-level, language-agnostic representation of the logical flow of an algorithm, serving as a bridge between problem formulation and actual code.
- Some common pseudocode syntax elements include: IF-THEN-ELSE for conditional instructions, FOR for repetitive loops, WHILE-ENDWHILE for blocks of commands executing till a condition is met, READ for input operation and PRINT for output operation.
- Writing pseudocode involves understanding the problem, identifying main components, defining the program flow, writing the code focusing on logic rather than syntax, and reviewing, and refining as necessary.
- Central to pseudocode logic is the sequence (step-by-step progression of instructions), selection (decisions made in the program) and Iteration (repetitive execution of loops).
- Pseudocode also plays a significant role in representing key algorithms in computer science like Merge Sort, Binary Search and Dijkstra's Algorithm.
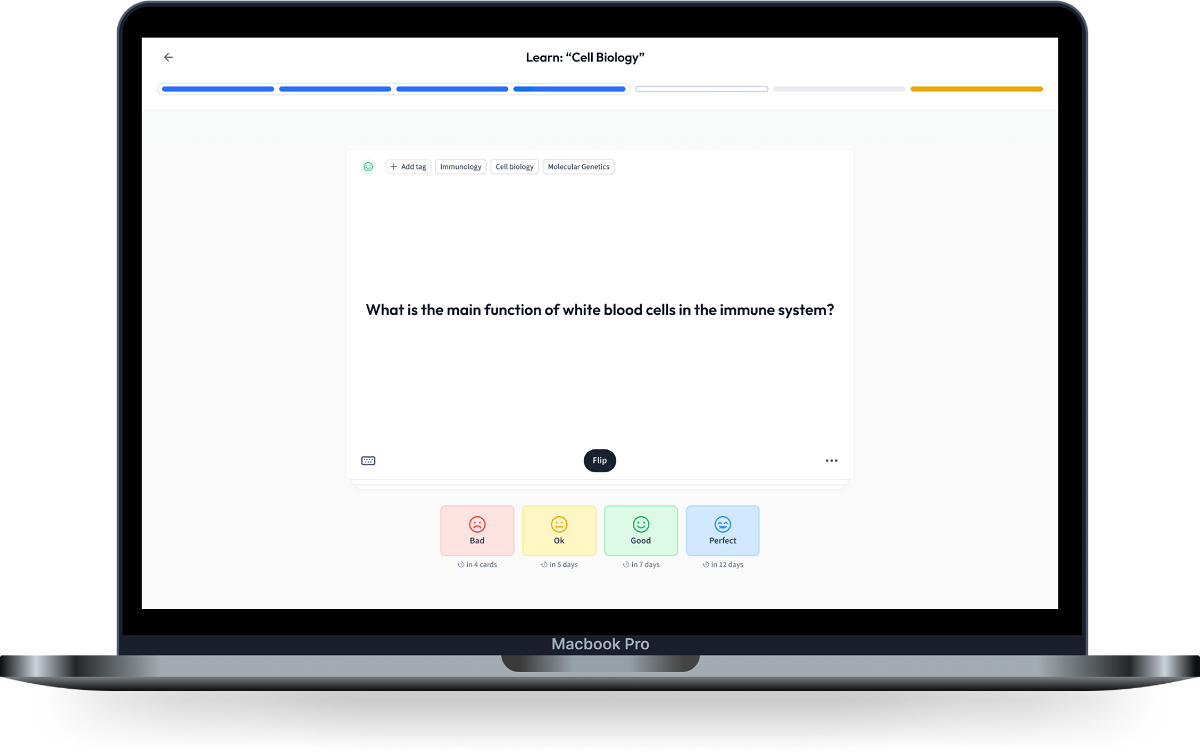
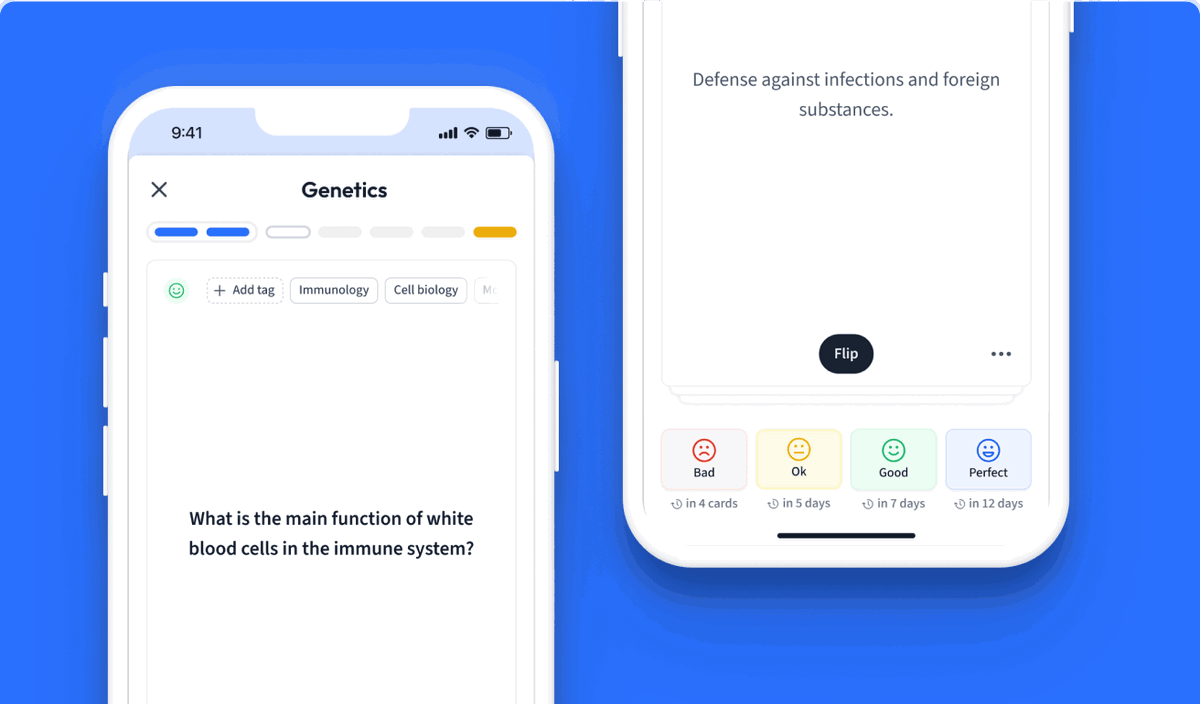
Learn with 12 Pseudocode flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Pseudocode
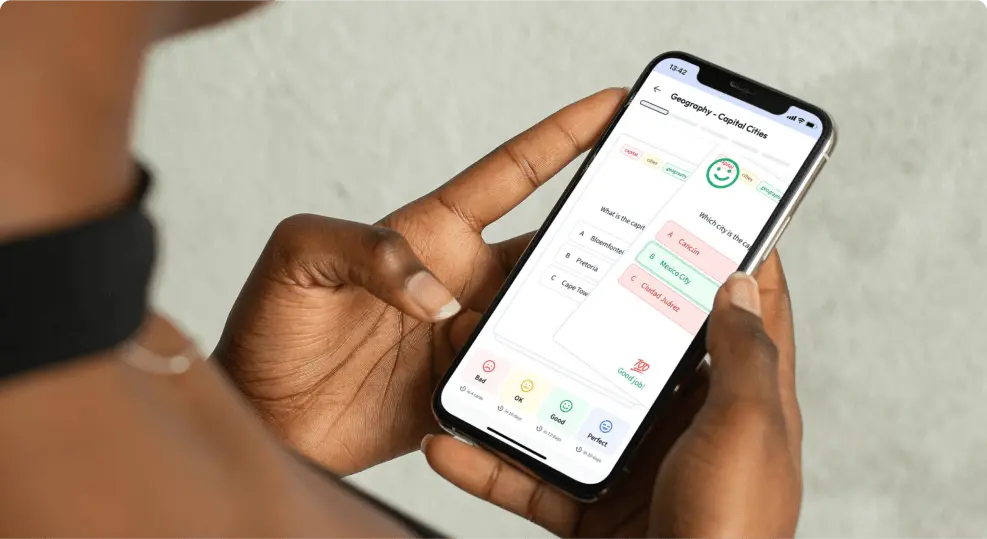
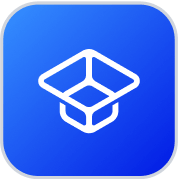
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more