Introduction to Tabulation in Computer Science
Tabulation is a method that plays a vital role in the field of Computer Science, specifically in the creation and execution of various algorithm techniques. It is a form of dynamic programming, where computations are stored in tables to avoid redundancy and improve efficiency.Tabulation in the context of Computer Science, refers to a technique where the intermediate results of computations are stored in a table for future reference, reducing redundant calculations in any process.
Defining Tabulation: An Essential Algorithm Technique
Tabulation can be attributed to an important data structure concept - the table or 2D array. Each cell in the table represents a solution to a subproblem, and these cells are filled in order, which allows the solving of complex problems by breaking it down into simpler manageable sub-problems.A classic example of a tabulation problem would be the Fibonacci sequence calculation.
function tabulationFib(n) { let fibTable = Array(n + 1).fill(0); fibTable[1] = 1; for(let i = 0; i <= n; i++) { if(i + 1 <= n) fibTable[i + 1] += fibTable[i]; if(i + 2 <= n) fibTable[i + 2] += fibTable[i]; } return fibTable[n]; }
Often the expedited speed at which tabulation solves problems is overlooked due to the trade-off of higher space complexity, because storing the results of each sub-problem can be space-intensive.
The Origin and Evolution of Tabulation in Computer Science
Tabulation originated in the early days of computing as a method to handle recursion and dynamic programming problems. The primary idea was to store results of sub-problems so that each sub-problem was only solved once, thereby reducing the computational time drastically.The concept of Tabulation came into the picture when dynamic programming was being explored. Dynamic programming involves breaking a problem into smaller sub-problems in a recursive manner. The issue with this was that it often resulted in recalculating solved sub-problems, wasting computational resources. Tabulation presented a solution where each sub-problem would be solved just once, its result stored in a table and directly referenced as required.
Importance of Tabular Data in Tabulation
In tabulation, each subproblem's solution is stored in a cell in a table. Each cell represents a small portion of the bigger problem. These cells are filled up in an order that ensures the whole problem is solved once the final cell is filled.- Helps achieve better time complexity
- Frequently used in dynamic programming problems
- Reduces redundancy and repetition
Tabulation technique can be visualized with the problem of calculating the length of the longest common subsequence (LCS) of two strings. In this, the strings 'ABCDEFG' and 'BDGK' are broken down into smaller subproblems in the form of 2D array. The idea is to calculate the LCS of every prefix of both strings and store the result in the 2D array.
function LCS(X, Y, m, n) { let L = Array.from(Array(m+1), () => new Array(n+1)); for (let i=0; i<=m; i++) { for (let j=0; j<=n; j++) { if (i === 0 || j === 0) L[i][j] = 0; else if (X[i-1] === Y[j-1]) L[i][j] = L[i-1][j-1] + 1; else L[i][j] = Math.max(L[i-1][j], L[i][j-1]); } } return L[m][n]; }This tabular approach helps to keep track of all the sub-problems and thus aid in efficient problem-solving.
Exploring the Tabulation Process in Depth
In the domain of computer science, tabulation is often associated with its application in dynamic programming. When embarking on the understanding of the tabulation process, it is vital to dissect it into its primary stages - defining the problem, initializing the table, populating the table, and finally, solving the problem.Understanding the Main Phases of Tabulation Process
The defining the problem phase is paramount. A clear identification and understanding of the problem to be solved is essential for the successful implementation of tabulation. After defining the problem, the process steps into the phase of initialising the table. This involves creating an appropriate data structure, mainly a table or a multidimensional array to store the solutions or results of subproblems.Once the table has been initialised, we progress onto the stage of populating the table. This step relies heavily on the nature of the problem. The table is filled using a bottom-up approach, which essentially means starting with the smallest subproblems and gradually proceeding to larger ones. Each table cell or element represents an instance of the original problem with lesser complexity; and hence, the values of each cell often depend on previously calculated cells.
// Example of populating the table in Fibonacci calculation. for(let i = 0; i <= n; i++) { if(i + 1 <= n) fibTable[i + 1] += fibTable[i]; if(i + 2 <= n) fibTable[i + 2] += fibTable[i]; }Finally, we reach the climax of the process - solving the problem. In this step, the final problem, often lying in the last cell of your table, is solved using the preceding solutions. This end-game strategy makes tabulation a powerful and efficient tool in dynamic programming.
How Tabulation Augments Performance in Computer Science
Tabulation, with its systematic and efficient methodology, is often seen as a solution to dealing with problems that display overlapping subproblems and optimal substructure properties. It's particularly beneficial when you are working with problems that involve a large number of overlapping subproblems, which would otherwise cost heavily in terms of execution time if solved using simple recursion. The underpinning of tabulation that drives its performance augmentation lies in the fact that it abandons the redundancy of computations. By storing solutions of subproblems and reusing them when necessary, it eliminates the need to solve the same problem again and again. This drastically cuts down on the number of operations, thereby increasing efficiency and decreasing the time complexity of the problem at hand. Using tabulation also often leads to a more write-intensive code as it pushes you to think about the problems in a more procedural way, as in contrast to the recursive nature of top-down dynamic programming approaches. This means your code performs more write operations to memory, enhancing the performance.Key Benefits and Challenges of Tabulation Process
Tabulation as a dynamic programming approach provides numerous benefits in problem-solving, but it also carries its own set of challenges. Benefits include:- Reduces time complexity: By storing and reusing answers to subproblems, tabulation techniques avoid unnecessary calculations, thus reducing time complexity.
- User-friendly visualization: Tabulation presents a systematic, tabular representation of how a problem is broken into subproblems and solved, making it easy to understand.
- Works out of the box: With tabulation, no need to check whether optimal substructure or overlapping subproblems properties exist, unlike with other dynamic programming methods.
- Space complexity: While tabulation speeds up computations by storing results, it can quickly take up a considerable amount of memory, thus leading to increased space complexity.
- Unnecessary computations: Tabulation often requires solutions to all subproblems while the solution for the main problem could have been determined without solving some of them. This can potentially result in unnecessary computation.
A Deep Dive into the Tabulation Technique
The tabulation technique is a fundamental algorithmic principle in computer science, primarily used for optimising computational problems. Created for handling large data sets and problems with overlapping sub-problems, it is significant in saving computational time and offering dynamic programming solutions.The Theory Behind Tabulation Technique
At its core, the tabulation technique is a bottom-up approach to solving complex problems in computer programming. Focusing on the distribution of sub-problems, this technique stores solutions to overlapping sub-problems in a structure similar to a table or 2D array. The strategy of tabulation is simple yet effective – solve sub-problems first, store their solutions, and then use these solutions to construct solutions for larger problems. For instance, consider a problem with an input size of \(n\), you first solve all the sub-problems for smaller inputs up to \(n\), and save their solutions. Once you have all the necessary solutions, you can solve the actual problem effectively. But here lies a key point of the tabulation that you should take note of – tabulation works best when there are not too many distinct sub-problems to be solved. If there are \(\Theta(n^k)\) distinct sub-problems for some \(k > 1\), tabulation might not be efficient because of the considerably high number of computations.// Populating a table for a Fibonacci function for(let i = 0; i <= n; i++) { if(i + 1 <= n) fibTable[i + 1] += fibTable[i]; if(i + 2 <= n) fibTable[i + 2] += fibTable[i]; }
Tabulation Example: Learning from Practical Scenarios
Looking at the practical application of tabulation, we can examine how it's used to solve a classic problem in computer science - calculating the Fibonacci series.In a Fibonacci series, the next number in the series is the sum of its two predecessors. Given a number 'n', the problem is to print the 'n'th number in the Fibonacci series.
function fibonacci(n) { let fib = Array(n + 1).fill(0); fib[1] = 1; for(let i = 2; i <= n; i++) { fib[i] = fib[i-1] + fib[i-2]; } return fib[n]; }In this case, the function first initialises a 1D array that will contain the Fibonacci numbers. The array is filled in a loop from index 1 onwards. Each Fibonacci number is computed as the sum of the two preceding numbers, so the function adds `fib[i-1]` to `fib[i-2]` to get `fib[i]`.
Smart Tips to Master the Tabulation Technique
Although understanding the tabulation technique may seem straightforward, having an educated and nuanced approach can aid you considerably.- Understand the problem: Begin by thoroughly understanding the problem you need to solve, including its various sub-problems.
- Select a structure: Once you understand the problem to its core, choose the right data structure to store intermediate results.
- Fill up the structure progressively: Study the pattern and dependencies in the initial subproblems and use them to fill up the data structure in a bottom-up manner.
- Take care of the space complexity: Since tabulation uses extra space to store the results of sub-problems, it is always diligent to know if the problem can be solved without any extra space.
- Optimize where possible: Often, the solution for a given problem doesn’t require the solutions to all its sub-problems. Identify such scenarios during problem-solving, and exclude those unnecessary computations.
Strategies and Techniques for Effective Tabulation
Creating an efficient tabulation strategy encompasses many areas of programming skills, ranging from understanding your approach to designing a proper data structure, and writing a code that manifests the heart of tabulation technique.Crafting a Successful Tabulation Strategy: Basic Tips
When you are engaged in developing a tabulation strategy, the underlying objective should always be to enhance efficiency and readability, along with making sure your code can handle a variety of input scenarios. Tip 1: Identifying Auxillary Space Requirements: This is the supplementary space required by your program other than the input and output size. By understanding the auxillary space requirements, you can manage your limit of space complexity. Tip 2: Optimising Data structure: An important aspect of mastering the tabulation technique is picking the right data structure. The choice of data structure is often pivotal in determining both the time and space complexity of your tabulation solution. Tip 3: Modelling an Iterative Approach : A distinctive feature of the tabulation technique is that it calls for an iterative model of problem-solving, unlike other coding techniques which primarily favour a recursive approach. This enables us to start from the smallest sub-problem and tackle larger problems in the order of complexity. Tip 4: Remembering Last Calculated Values: Tabulation involves the storage and usage of previously computed values. This not just reduces redundant computations but also adds to the efficiency of the code, saving both time and memory.// Create an array 'table' with size 'n' and initialize all elements as 0. int[] table = new int[n]; // Base case table[0] = 1; // Iterate over different elements and update the table accordingly. for (int i=1; iAdapting Tabulation Techniques for Different Data Sets
A proficient coder or programmer is one who can adapt tabulation techniques for different data sets. The area of tabulation lends itself to versatility, wherein slight tweaks and variations can suffice for different input types or problem statements. Variable Length Subproblems: While dealing with problems which have variable length subproblems, it often becomes necessary to maintain a dynamic table which can be updated as per the changes in length of subproblems. Multidimensional Input: Often, the input is not unidimensional but involves multiple parameters. In such cases, tabulation tables can be expanded into multidimensional arrays. This requires a more careful and methodical way of populating the table. Decoding Dependencies: Deciphering how the current problem or value is dependent on the preceding ones can aid in solving large problem instances. The thrust should be to establish a hierarchical dependency and then solve in a manner that follows this order.Hierarchical Dependency: it refers to the pattern of reliance that bigger problems have on smaller ones and how clear the transition from one level to the next is.
Tabulation in Programming: Language-Specific Strategies
As different programming languages come with their own set of features and rules, the way tabulation is applied can vary across these languages. Python - Python's ability to support multi-dimensional arrays directly, serves as an asset in tabulation technique. This, combined with Python's in-built function, proves to be extremely efficient. Java - Java employs traditional HashSet and HashMaps for storing the data. It also provides greater flexibility in the implementation of tabulation approach. Ruby - Ruby holds an edge in dealing with string related problems, as strings in Ruby are mutable. C++ - C++ with its STL feature, allows terse and compact code, facilitating easy readability and faster development. In conclusion, understanding the idiosyncrasies of your programming language and using them to your advantage employs the tabulation method most effectively. You should adapt and modify techniques according to the unique attributes and constraints of your chosen programming language. Be it Python, Java, Ruby, or C++, a robust grip on the language will enable you to unleash the full power of the tabulation method.The Impact of Tabulation on Contemporary Computer Science
The advent of the tabulation technique has indeed been a true game-changer in computer science. As a core strategy of dynamic programming, tabulation has proven to be indispensable in designing efficient algorithms that solve complex computational problems and manage large data sets.Role of Tabulation Algorithm in Modern Computation
The tabulation method, owing to its bottom-up approach, has been a vital tool in modern computation. It's a fundamental concept behind many algorithms used in various domains of computing, from databases and computer graphics to artificial intelligence and machine learning. Tabulation is extremely useful when dealing with an array of overlapping sub-problems. This method involves answering the smaller instances of the problems first, storing the results in a table, and then using these results to solve the larger instances. Sub-problems are solved only once, and their results are stored to avoid re-computation, which eliminates redundancy and massively saves computational resources. For instance, the calculation of Fibonacci numbers can be optimised using tabulation. Standard recursive procedures can lead to repeated computation and a runtime in the order of \(2^n\), where \(n\) is the input size. By applying tabulation, you can bring down the runtime complexity to linear, which is an immense saving, especially for large values of \(n\).// Fibonacci Series using Tabulation void fibonacci(int n) { int f[n+2]; // array to store Fibonacci numbers f[0] = 0; f[1] = 1; for (int i = 2; i <= n; i++) { f[i] = f[i-1] + f[i-2]; } printf("%d", f[n]); }The Future of Tabulation in Computer Science
As computer science continues to advance, the role of tabulation is only poised to grow. Already, it's a fundamental part of modern algorithms used in diverse computational fields. In the future, the tabulation technique could develop new dimensions with the burgeoning role of quantum computing, parallel processing, and vast data analysis. To keep up with advanced computational challenges posed by Big Data, Artificial Intelligence and Machine Learning, the tabulation technique has been applied more ingeniously and optimised to suit these complex issues. The development of sophisticated algorithms for better space-time complexity trade-offs showcases a forward trend in the future of tabulation. One field where further exploration could be done is in the realm of graph algorithms and network analysis. The combination of efficient graph data structures with dynamic programming solutions using tabulation can lead to breakthrough improvements in speed and complexity.Tabulation and Big Data: A Path to Enhanced Analysis
With the exponential explosion of data in today's digital age, extracting meaningful insights from such vast information has become a significant challenge, commonly termed as the 'Big Data problem'. Tabulation serves as a crucial strategy to efficiently manage, analyse, and derive valuable insights from massive data sets. The critical principle behind the usefulness of tabulation in Big Data analysis is the mitigation of redundant computations by storing solutions to overlapping sub-problems. When dealing with vast quantities of data, avoiding repetitive calculations translates into substantial savings in computation time and resources. Moreover, tabulation can be effectively employed to solve data-intensive tasks such as dynamic Data Aggregation and Data Mining. Through tabulation, complex analyses, such as finding correlations or patterns among millions or billions of datasets, is achievable with optimum efficiency. Another area where tabulation offers significant benefits is in the formulation of machine learning algorithms. Training these models involves solving many smaller, repetitive tasks. The use of tabulation decreases the computational complexity and significantly accelerates the training process, making it more feasible to use larger datasets for more accurate predictive modelling. Indeed, as data continues to grow in its volume, velocity, and variety, the demand for tabulation technique– owing to its inherent advantages of tackling overlapping issues and efficient problem-solving– is set to surge in the realm of Big Data.Tabulation - Key takeaways
- Tabulation is an approach in computer science often associated with dynamic programming, aiming to enhance efficient problem-solving.
- Key phases of the tabulation process include defining the problem, initializing the table (data structure), populating the table using a bottom-up approach, and solving the final problem using the preceding solutions.
- Benefits of tabulation include reducing time complexity, user-friendly visualization, and applicability with overlapping subproblems and optimal substructure properties.
- Challenges of tabulation include increased space complexity from storing results and potential for unnecessary computations.
- The tabulation technique is extensively used for optimizing computational problems, favoring a bottom-up approach to storing solutions to overlapping sub-problems.
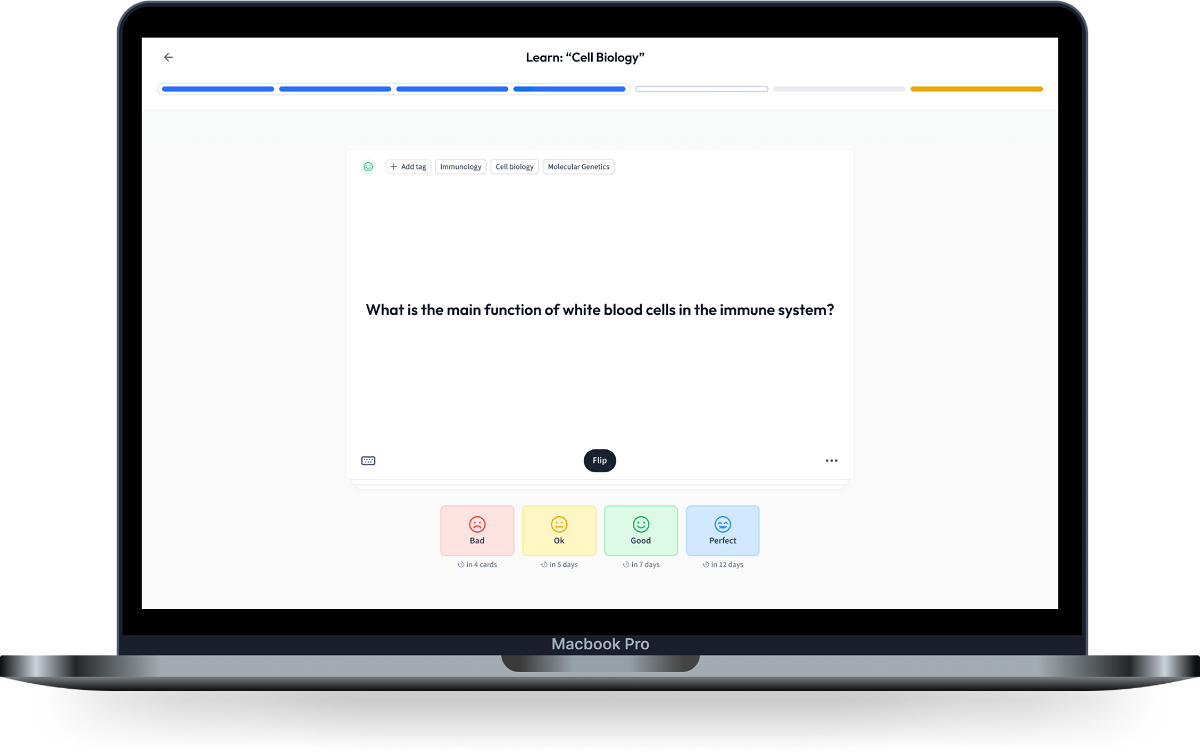
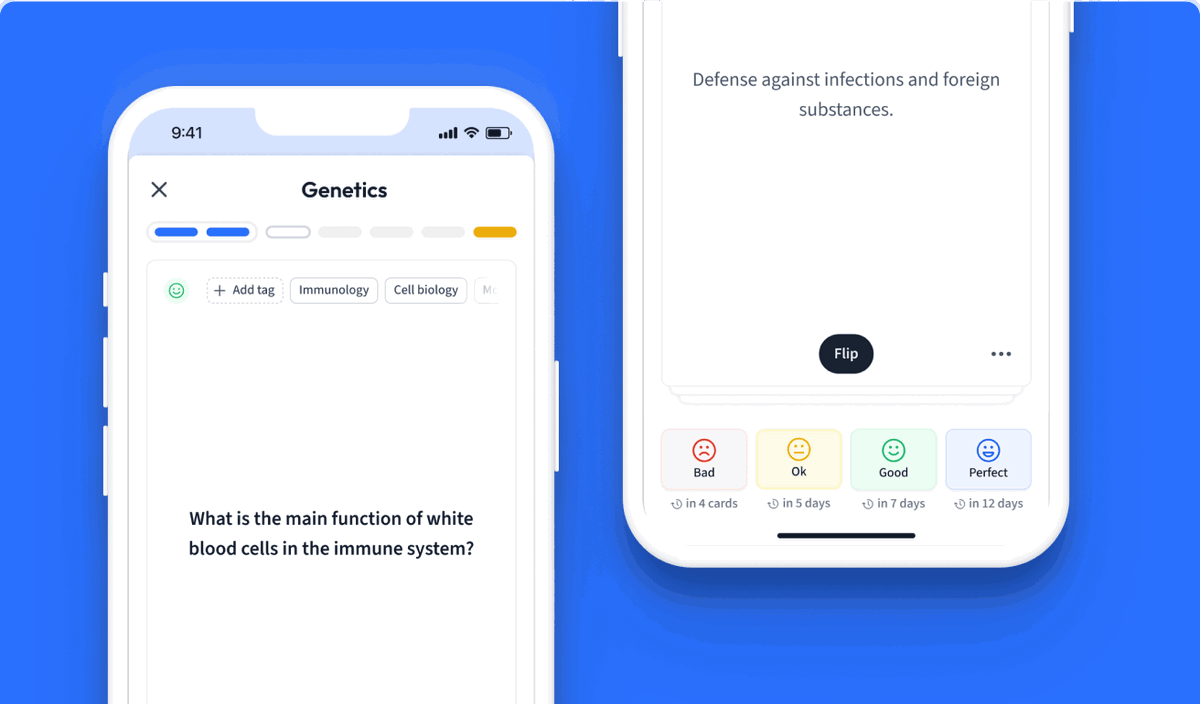
Learn with 15 Tabulation flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Tabulation
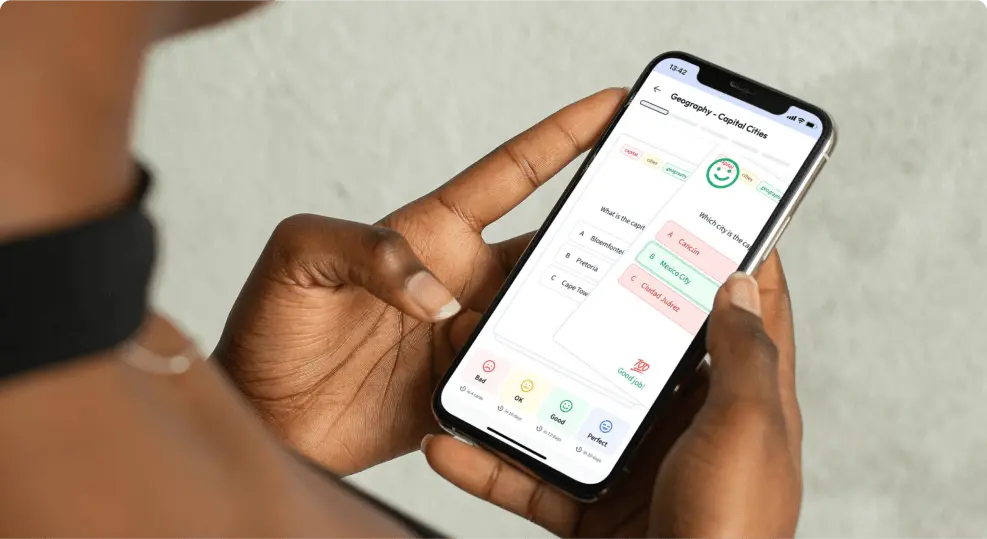
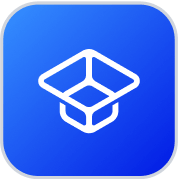
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more