What is Breadth First Search in Computer Science?
Breadth-first search (BFS) is a key technique in computer science, particularly within the areas of graph theory and data structure manipulation. It's essentially an algorithm for traversing or searching tree or graph data structures. The unique factor of this search algorithm is that it explores all the vertices of a graph at the present depth level before moving to nodes at the next depth level.
Depth level, in this context, refers to the distance (the minimum number of edges) from the root or designated start node. So, depth level 0 means the start node; depth level 1 includes all nodes directly connected to the start node, and so on.
Understanding the Breadth First Search Algorithm
The Breadth First Search algorithm systematically visits all the nodes at the current depth level before moving to the next. This 'level by level' process distinguishes BFS from other search algorithms like Depth First Search, which goes through a path as deep as possible before backtracking. To implement the BFS algorithm, you primarily use a data structure called a Queue, following the FIFO (First in, First out) strategy.
The simple pseudocode for BFS is as follows:BFS (graph G, start vertex s): // all nodes initially unexplored mark s as explored let Q = queue data structure, initialized with s while Q is not empty: remove the first node of Q, call it v for each edge(v, w): // for v's neighbors if w unexplored: mark w as explored add w to Q (at the end)
Key Components of the Breadth First Search Algorithm
The core components of the Breadth First Search algorithm are:- The graph: a non-linear data structure consisting of nodes and edges.
- The queue: a particular type of abstract data structure where items are kept in order, and the primary operations are enqueue (addition of entities) and dequeue (removal of entities).
- The visited array: to keep track of already visited nodes, preventing infinite loops.
Practical Breadth First Search Examples
When using the BFS algorithm on a graph structure, you implicitly create a Breadth-First tree. This tree roots at the source node, and all nodes at the same distance from the source are at the same depth-level of the tree.Suppose there's an unweighted and undirected graph like the following:
1 | --- | 2 |
| | | | |
3 | --- | 4 |
Real-life Breadth First Search Use Cases
BFS holds a lot of practical significance in real-life scenarios. Here are a few:- In network routing algorithms, BFS helps in finding all neighbour nodes.
- For path finding in real geographical, pixel-based, or virtual maps.
- In web crawling, where spiders use BFS to limit their depth of crawling.
- For social networking websites, where BFS can help find all people within a given 'friendship' distance, for example.
Moreover, the BFS algorithm is critical for machine learning. It plays a crucial role in the decision tree-based learning model in extracting useful patterns or decision trees from datasets.
Breadth First Search vs Depth First Search
When examining the contrast between Breadth First Search (BFS) and Depth First Search (DFS), we observe that they are indeed two different cornerstones of the graph theory in computer science, each with distinctive features and use-cases. Their primary commonality is that they are both algorithms for traversing and searching within graph data structures, yet how they carry out this process is significantly different.
Similarities and Differences: Breadth First Search and Depth First Search
Despite their obvious differences, BFS and DFS share a handful of characteristics. For instance, they both start at a root node or any arbitrary node for traversing the graph. Moreover, their goal is to visit every node in the graph exactly once, hence they maintain a 'visited' list or hash to track the nodes already encountered.
The primary difference between these search algorithms lies in their approach to traversing the structure. BFS traverses the graph in a level by level manner. It visits nodes level by level, meaning first it visits nodes at depth level 0 (the root), then at depth level 1, and so on. On the contrary, DFS follows a different strategy. It goes as deep as possible down one path before backtracking.
Another considerable difference is the data structure that they use. BFS utilises a Queue, which follows the First In First Out (FIFO) rule. In contrast, DFS uses a Stack, obeying the Last In First Out (LIFO) principle.
Let's articulate the BFS and DFS more clearly:
Algorithm | Traversal Pattern | Data Structure |
Breadth First Search | Level by level | Queue (FIFO) |
Depth First Search | As deep as possible | Stack (LIFO) |
Another noteworthy aspect of BFS and DFS is their time and space complexity. Both BFS and DFS have linear time complexity, marked as \( O(V + E) \), where \( V \) is the number of vertices, and \( E \) is the number of edges. While their time complexity is similar, their space complexity differs due to their contrasting nature. BFS's space complexity could be higher, especially when dealing with a graph that grows exponentially, while DFS has a smaller footprint as it only needs to store the nodes along the active branch of the tree or graph.
Choosing Between Breadth First Search and Depth First Search: When and Why
To decide between using BFS or DFS, you must consider the nature of the problem you are dealing with and what you hope to achieve. Below is a listed guide to help you make a mindful selection:
- If you know a solution exists close to the root of the tree, BFS would be a better option since it minimises the path cost.
- If the tree is extremely wide (i.e., contains many nodes), you might want to use DFS as it takes less memory.
- If the graph is acyclic or tree-structured, DFS can be more beneficial.
- If you need to find the shortest path or need a level by level traversal, BFS should be your choice.
- DFS can serve you significantly better if it's okay to get any valid answer, not necessarily the shortest route.
It's worth mentioning that when dealing with issues of connectivity or finding bridges/articulation points in a network, DFS usually outperforms BFS and is preferred. But remember that BFS is generally the go-to for unweighted graphs or for finding the shortest path in weighted graphs that have non-negative weights.
In the end, the choice between BFS and DFS isn't always clear-cut. Often, the most effective algorithm will largely depend on the specifics of the task at hand.
Exploring the Breadth First Search Tree
When it comes to the implementation of the Breadth First Search (BFS) algorithm, we can visualise the process and outcome through a particular kind of tree called the BFS tree. This data structure essentially maps the sequence of exploration used during the BFS traversal.
The Structure and Function of the Breadth First Search Tree
The BFS tree, otherwise known as the Breadth First Search Tree, is a rooted tree that is used to exhibit the order of node exploration during a BFS traversal algorithm. It's a reliable diagrammatic representation that clearly outlines how BFS explores the nodes of a graph, proceeding level by level.
The BFS tree is so designed that it roots at either a specific node called the 'source' or 'start' node or any arbitrary node in the graph. The tree then expands breadth-wise, incorporating all nodes reachable from the source. Each node in the tree represents a vertex in the graph, and each edge in the tree corresponds to a direct edge in the graph. The tree grows level by level, each level comprising nodes that are at the same distance (number of edges) from the root node. Each level is explored entirely before progressing onto the next level.
A level, in this case, refers to the set of nodes that are the same distance from the root node. Level 0 is the start node; level 1 is all nodes directly connected to the start node, and so forth.
Node in BFS Tree | Corresponds to |
Root Node | Source/Starting Vertex in the Graph |
Edge in the BFS Tree | Direct Edge in the Graph |
Level in the BFS Tree | Nodes at the equal distance from the Root Node |
For a connected graph, the BFS tree is typically unique, given that the starting vertex is specified. However, for a disconnected graph or a graph where the starting vertex isn't unique, there can be multiple possible BFS trees.
The primary function of this tree is to emphasise how nodes are encountered during the BFS traversal. Moreover, it also helps in illustrating how BFS can find the shortest path between two nodes in terms of the number of hops (or edges).
The Process of Developing a Breadth First Search Tree
The construction of a BFS tree aligns with the systematic operation of the BFS algorithm. If you remember, BFS progresses level by level, visiting each unvisited node at the current level before moving onto the next. Each time a fresh node is discovered during this traversal, an edge is created from the current node (already part of the BFS tree) to the newly discovered node. This systematic approach results in the formation of a BFS tree.
The process for developing a Breadth First Search tree can be illustrated with the following steps:
Start from a root node in graph G. Create a queue, Q, and append the root to it. Mark the root as visited. While Q isn't empty, perform the following steps: Dequeue the first node in Q, reference it as 'n'. For every adjacent yet unvisited node, 'm', of 'n': Append 'm' to Q and mark it as visited. Draw an edge from 'n' to 'm' in the BFS tree.
By following this process, you'll obtain a BFS tree that mirrors the steps taken by the BFS algorithm during the traversal of the original graph. Remember, the BFS tree won't necessarily represent the structure of the original graph fully, but it will depict the shortest path (least number of edges) from the root node to any other node in the tree.
To summarise, creating a Breadth First Search tree involves using a systematic algorithm to traverse a graph level by level. By developing such a tree, we can visualise the path that the BFS algorithm takes and obtain the shortest path from the root node to any other node within the tree.
The Time Complexity of Breadth First Search
In the realm of computer science, understanding time complexity is fundamentally crucial. It allows you to evaluate the efficiency of an algorithm in terms of the time it takes to complete given its input size. While implementing the Breadth First Search (BFS) algorithm, you need to comprehend its time complexity to judge its performance and efficiency, particularly for larger data sets.
Understanding Breadth First Search Time Complexity
Time complexity essentially refers to the computational complexity that describes the rate of growth of the time taken by an algorithm with respect to its input size. It provides an upper bound on the time required for executing a program. In terms of BFS, the time complexity is decisive in assessing the algorithm's performance and scalability.
The BFS algorithm starts at a chosen root node and explores neighbouring nodes at the present depth level before moving on to nodes at the next depth level. As BFS explores each edge in the graph once, the time complexity can be associated with the number of edges and vertices the algorithm processes.
In BFS, every vertex is processed exactly once and every edge is also processed once when we get to either of its endpoints. Therefore, the total time to process all the vertices and edges is proportional to the number of vertices plus the number of edges. This can be expressed as:
\[ \text{{Time Complexity of BFS}} = O (V + E) \]where:
- \(O\) represents the Big O notation – used to describe the upper bound of the time complexity.
- \(V\) represents the number of vertices in the graph.
- \(E\) represents the number of edges in the graph.
Take note that the time complexity applies to both worst-case and average-case scenarios as each vertex and edge will always be explored once in a connected graph. It's also noteworthy to mention that the time complexity does not account for the time needed to initialise or declare the auxiliary data structure (the queue), which may increase the time requirement depending on the implementation.
Improving the Time Complexity of Breadth First Search Algorithms
The time complexity of a BFS algorithm can be considered efficient in most practical scenarios as it scales linearly with the size of the input graph—number of vertices and edges. However, certain adjustments and approaches can further optimise its run-time, especially in specific use-cases or data conditions.
One crucial factor that influences the time complexity of BFS is the auxiliary data structure used. BFS typically uses a queue implemented as a linked list or a dynamic array, contributing to constant time complexity for enqueue and dequeue operations. However, in some scenarios, using a deque (double-ended queue) can enhance the time efficiency of BFS, particularly if the algorithm requires searching in reverse or bidirectional search.
Another aspect to consider is the graph's representation. The BFS time complexity assumes that the graph is represented using an adjacency list. If an adjacency matrix is used, the time complexity would escalate, making BFS significantly slower. Hence, choosing an adjacency list representation over an adjacency matrix can lead to major time savings, especially for sparse graphs.
Writing efficient, well-structured code can also contribute to reducing run-time. This includes avoiding unnecessary computations, utilising appropriate operations on data structures, and ensuring proper memory management. For example, marking visited nodes immediately when they're added to the queue can help avoid revisiting nodes, thereby improving efficiency.
Additionally, BFS can be parallelised to leverage concurrency and multi-core systems. The frontier concept in BFS, which refers to all the nodes being explored at the current depth level, can be exploited to create a parallel BFS where multiple nodes of the same frontier can be processed simultaneously.
While there may be ways to optimise a BFS algorithm, it's essential to do so cautiously. Each optimisation should be justified by the nature of the problem and input graph, and care should be taken to ensure that such optimisations do not inadvertently introduce complexity or errors.
Practical Implementation of Breadth First Search
Putting Breadth First Search (BFS) into practice involves a series of steps that you should carefully follow to solve a problem at hand efficiently. It's not just about understanding the core concepts – applying them strategically and logically is essential too. Below, we'll delve right into the steps you need to consider to make BFS work effectively for you, followed by some real-world case studies highlighting its implementation in coding problems.
Steps to Implement Breadth First Search in Problem Solving
The practical implementation of BFS involves a logical and well-planned approach. This approach can be broken down systematically, guiding you to morph from the theoretical to implementation effortlessly.
- Identify the problem that requires a BFS implementation.
- Determine the graph's representation. For BFS, an adjacency list is typically a better choice as it optimises the time complexity.
- Define the root or the starting node in the graph.
- Create a queue and visited list (or hash). Enqueue the root into the queue and mark it as visited.
- Start a loop that continues until the queue is empty. During each iteration:
- Dequeue a node from the queue.
- Process the node (an operation depending on the specific problem to be solved).
- For each vertex adjacent to the current node that hasn't been visited yet, mark it as visited and enqueue it.
Take note that the BFS algorithm visits each vertex once and traverses each edge once, which leads to an overall time complexity of \( O(V+E) \) where \( V \) is the number of vertices and \( E \) is the number of edges. This supports its efficient and scalable nature.
Case Studies: Breadth First Search in Real-World Coding Problems
BFS finds applications in multiple real-world scenarios, especially when optimisation problems exist in network routing, AI, web crawling, path-finding in geographic maps, and social networks. Here, we'd look at a couple of more specific instances where implementing BFS in problem-solving has proven indispensable.
Case Study 1: Social Network Friend SuggestionIn social networking services like Facebook or LinkedIn, 'People you may know' or 'Friend suggestion' is a popular feature. Using BFS, we can easily implement such feature. Initially, one can run a BFS from a user's node for 2 levels deep (considering that people with up to 2 degrees in separation are more likely to know each other). The discovered nodes on the second level (disregarding those on the first level as those are user's friends already and those he doesn't know), serves as the list of friend suggestions.
Case Study 2: Google Maps Shortest PathBFS is a core component of the shortest path algorithm used in Google Maps. When a user wants to find the most efficient route from location A to location B, BFS comes to the rescue. In this scenario, each location on the map is a node, and the roads connecting these locations are edges. The aim is to find the shortest path - the one with the least weight or cost (the cost can represent distance, time, or road conditions). If the distance or cost is uniform for all edges (implying an unweighted graph), a simple BFS from the source to the destination would find the shortest path. But if the graph has weights (each edge has a differing cost), BFS can be tweaked into a Dijkstra's algorithm, which finds the shortest path in a graph with non-negative weights.
These case studies underscore the practicality and power of BFS. However, given BFS's linear time complexity and breadth-based traversal style, it isn't always the optimal approach. That's why knowing when to use BFS is just as important as understanding how to use it.
Breadth First Search - Key takeaways
- Breadth First Search (BFS) is a graph traversal algorithm that explores nodes level by level, starting from a root node. It's widely used in network routing algorithms, path finding in maps, web crawling, and social networking sites.
- BFS and Depth First Search (DFS) are both graph traversal algorithms but with different strategies. BFS explores nodes level by level using a Queue data structure, while DFS explores as deep as possible down one path before backtracking using a Stack data structure.
- The time complexity of BFS is \(O(V + E)\), where \(V\) is the number of vertices and \(E\) is the number of edges. This applies to both worst-case and average-case scenarios as each vertex and edge will always be explored once in a connected graph.
- A Breadth First Search Tree is a rooted tree that exhibits the order of node exploration during a BFS traversal algorithm. It's a reliable representation of how BFS traverses the nodes of a graph level by level.
- Deciding between using BFS or DFS depends on the nature of the problem and the desired results. BFS is usually chosen when a solution exists close to the root or a level by level traversal is required, while DFS is preferred when the tree is very wide or does not necessarily require the shortest route.
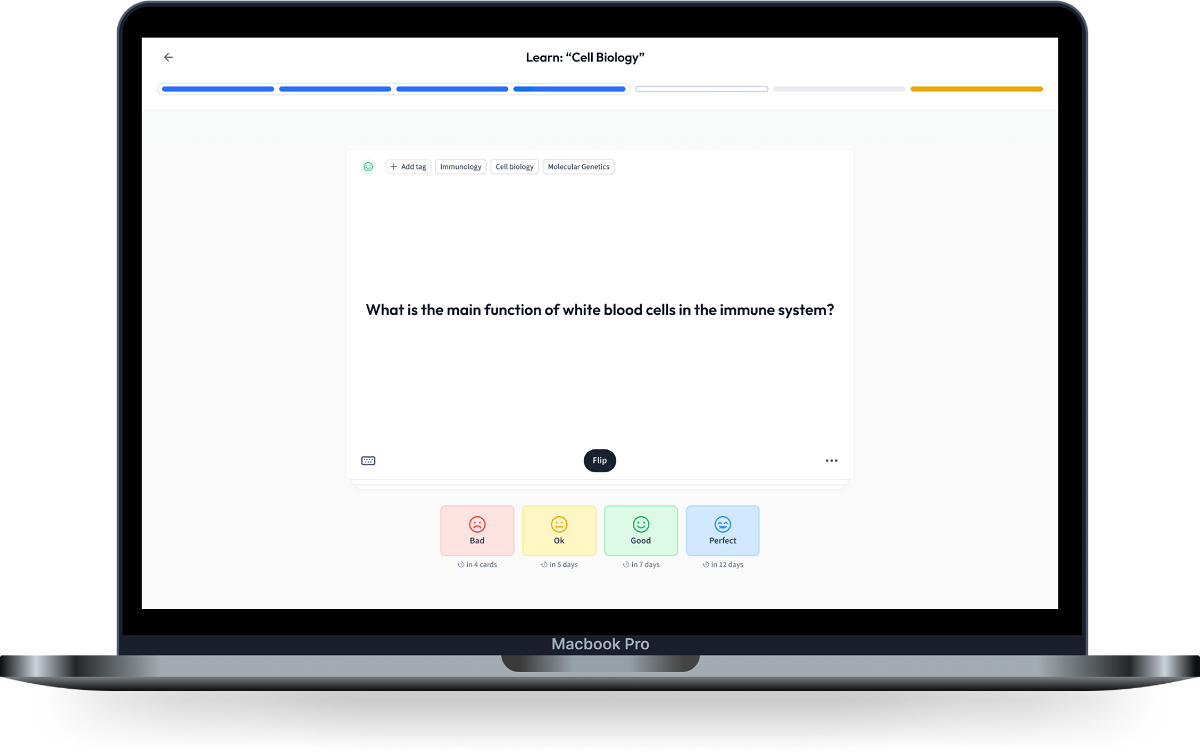
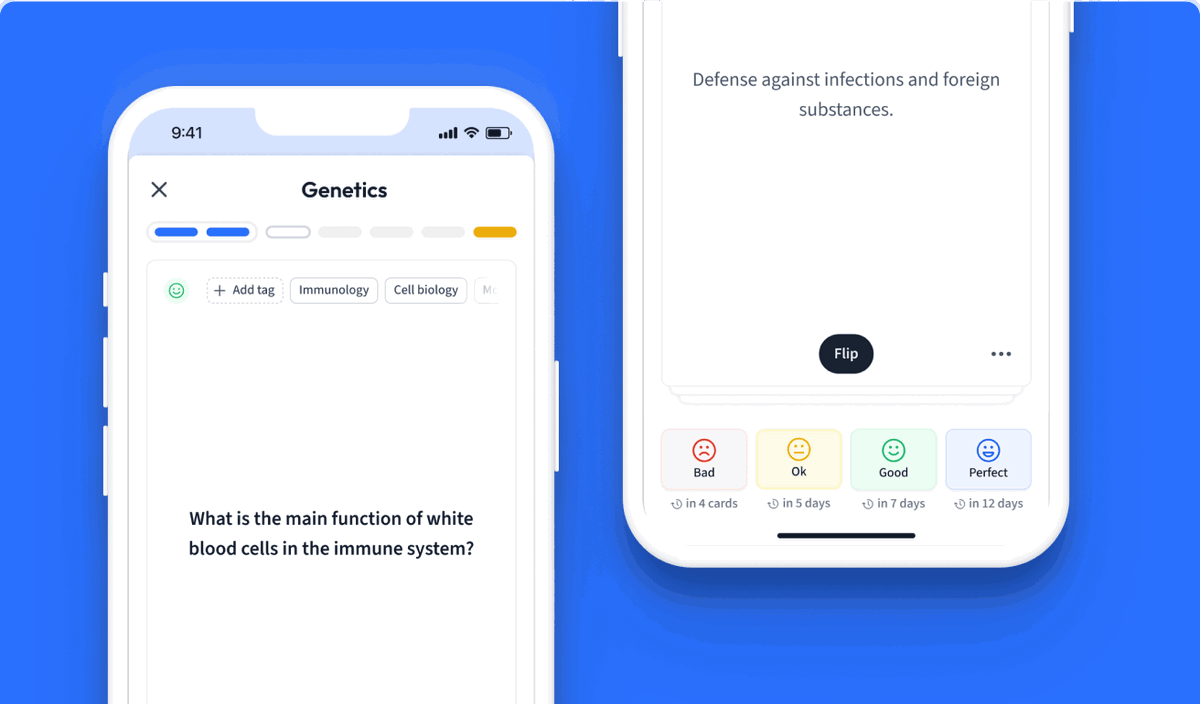
Learn with 15 Breadth First Search flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Breadth First Search
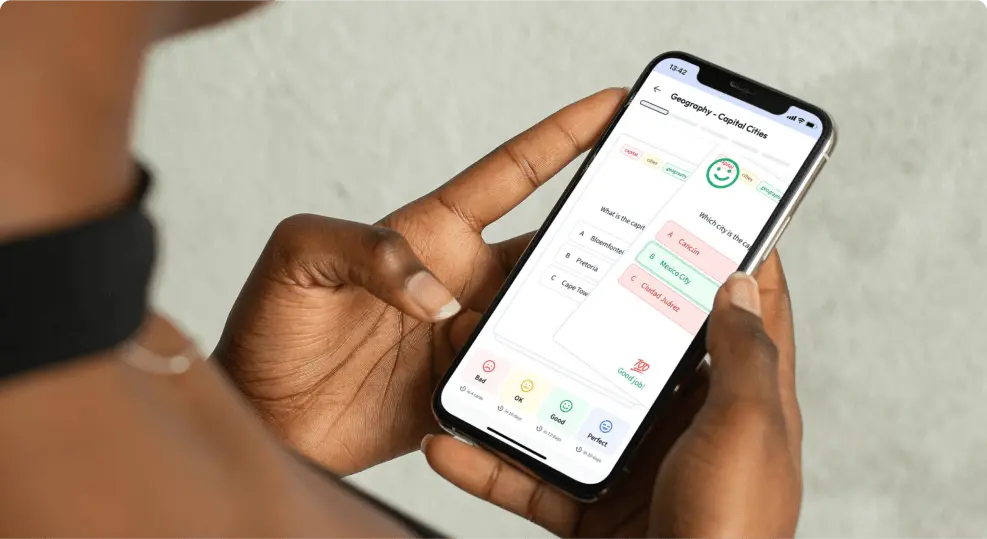
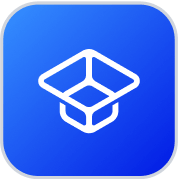
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more