Understanding Depth First Search in Computer Science
In order to truly grasp the concept of Depth First Search in Computer Science, you will need to equip yourself with the knowledge of what it is, the components involved, as well as an understanding of its specifics through a detailed example.The Basics of Depth First Search Algorithm
Depth First Search (DFS) is a fundamental algorithm in computer science for traversing or searching through a graph or tree data structure. The primary concept behind DFS is to go as deep as possible into the structure, and backtrack once you have visited all possible avenues from a given vertex.DFS begins at a chosen node (also called a 'vertex'), explores as far as possible along each branch before retreating. Hence, it goes deep into a branch before exploring neighbours.
- Start at a selected node (often the root in case of a tree, or an arbitrary node in case of a graph)
- Explore the neighbour nodes at the current depth prior to moving on to nodes at the next depth level
For instance, imagine a maze where the DFS would go down one path until it hits a dead end, then it will backtrack to find the next available path and continue.
Detailed Explanation of a Depth First Search Example
Consider an undirected graph with five vertices and the following edges: (1, 2), (1, 3), (2, 4), (2, 5), (3, 5).1 ----- 2 | | \ | | 4 | | 3 5
- Starting at vertex 1, we select an arbitrary neighbour, say 2, and continue
- From 2, we see unexplored neighbours 4 and 5. We select one (say 4) and continue
- 4 has no unexplored neighbours, so we backtrack to 2
- From 2, we now choose 5 and continue
- 5 also has no other unexplored neighbours, so we backtrack all the way to 1
- Finally, we proceed from 1 to its unexplored neighbour 3 and continue
- 3 has no unexplored neighbours, so the search ends here.
Components Involved in Depth First Search Algorithm
At its core, DFS is all about navigating graphs or trees. Thus, it's essential to understand the key elements involved - vertices, edges, and the stack data structure that facilitates the search.Vertices (also called 'nodes') are the fundamental units of any graph or tree. In DFS, each vertex can be in one of two states: visited or unvisited.
Nodes and Edges in Depth First Graph Search
When applying a DFS algorithm, you start at a chosen node (often known as the 'root'), and explore along each branch to its fullest, before backtracking to explore the next branch. Edges are crucial in this search. When an edge leads to an unvisited node, it's marked as a 'discovery edge'. If it leads to a previously visited node, it's marked as a 'back edge'.An interesting property of DFS is that the discovery edges form a tree, known as a 'DFS tree', while the back edges can only connect a node to its ancestor. This property is often used in algorithms to solve graph-related problems.
Implementing Depth First Search: Practical Approaches
Now that you understand the theory behind the Depth First Search algorithm, this section will explain how to implement DFS using popular programming languages like Python and Java. Remember, practice is key to mastering these concepts and developing efficient problem-solving skills.Depth First Search Python: Getting Started
Python is an excellent language for implementing DFS due to its readability and powerful data structures. To implement DFS, you'll need to get comfortable with Python's list data structure, which you can use to represent both the graph and the stack needed to keep track of your traversal. Firstly, understand how to represent a graph in Python. You can use a Python dictionary, with vertices as keys and their neighbours as values:graph = { 'A' : ['B', 'C'], 'B' : ['A', 'D', 'E'], 'C' : ['A', 'F'], 'D' : ['B'], 'E' : ['B', 'F'], 'F' : ['C', 'E'] }Remember that you will navigate this graph using the DFS principles: going as deep as possible on a branch before backtracking. To aid in your understanding, here are some key points:
- The Python **list append()** function is used to add elements to the stack
- The Python **list pop()** function is used to remove an element from the stack
- The Python **set** data structure is used to keep track of visited nodes, since lookup times are constant, and you won't visit the same node twice.
Step-by-Step Depth First Search Implementation Python
Here's how you can implement the DFS algorithm in Python:def dfs(graph, start): visited, stack = set(), [start] while stack: vertex = stack.pop() if vertex not in visited: visited.add(vertex) stack.extend(graph[vertex] - visited) return visitedLet's walk through this step by step:
1. You start by defining a function called dfs, which takes two parameters: the graph and a starting vertex. 2. Next, you initialize two empty sets, `visited` and `stack`. You add the starting vertex to the stack. 3. Then you enter a while loop which continues as long as the `stack` is not empty. In each iteration of the loop: 3.1. You `pop()` a vertex from the stack. 3.2. If that vertex has not been visited before, you add it to the visited set. 3.3. Then, you add all of its adjacent vertices to the `stack`. However, you avoid including vertices that have been visited before by subtracting the `visited` set from the set of adjacent nodes. 4. When the stack is finally empty, the function returns the `visited` set which contains all the nodes traversed by the DFS.
Java and Depth First Search: A Complete Guide
In Java, implementing the Depth First Search algorithm requires usage of its rich collection framework. For instance, you may use a HashMap to represent the graph. HashMaps can store vertices and their corresponding adjacency lists. Let's define a Graph class in Java:class Graph { private int V; // No. of vertices // Array of lists for Adjacency List Representation private LinkedListadj[]; Graph(int v) { V = v; adj = new LinkedList[v]; for (int i=0; i In this class, the adj[] array of LinkedList objects represents the adjacency lists. The `addEdge` function adds an edge to the adjacency list of a vertex. Here are some additional points to consider:
- In Java, you do not need to implement a stack specifically, because the built-in call stack takes care of it
- Java's recursive method calling mechanism is effectively a stack
- You can use a boolean array to keep track of the visited nodes
Mastering Depth First Search Java with Examples
Now, here's how to implement a `DFS` method within the `Graph` class:void DFSUtil(int v,boolean visited[]) { // Mark the current node as visited and print it visited[v] = true; System.out.print(v + " "); // Recur for all the vertices adjacent to this vertex IteratorMuch like the Python example, this DFS implementation uses a boolean array, `visited[]`, to track the visited vertices. The `DFSUtil()` function is used to traverse the graph. Initially all vertices are not visited, so the `visited[]` array is set to false by default. The `DFS()` function is used to call `DFSUtil()` and begin the DFS traversal. Do note that this is a typical implementation of DFS in Java, and there may be variations to this approach based on specific problem requirements.i = adj[v].listIterator(); while (i.hasNext()) { int n = i.next(); if ( !visited[n]) DFSUtil(n, visited); } } // The function to do DFS traversal. It uses recursive DFSUtil() void DFS(int v) { // Mark all the vertices as not visited(set as // false by default in java) boolean visited[] = new boolean[V]; // Call the recursive helper function to print DFS traversal DFSUtil(v, visited); } Comparing Depth First Search Techniques
While the core concept behind the Depth First Search (DFS) algorithm remains constant, the way it is implemented can vary significantly between different programming languages. This section will extensively compare the techniques used in Python and Java, which will help you understand the nuances involved in employing DFS in different programming scenarios.Differences Between Depth First Search Python and Depth First Search Java
On a high level, the key differences between Python and Java in the context of implementing DFS are due to the inherent differences in their languages. Despite DFS following the same logic in both languages, the data structures and language syntax used for representing and traversing the graph leads to a contrast in the DFS implementations. One of the fundamental differences lies in the type of data structures used to represent the underlying graph. Python, being a dynamically typed language, utilises a dictionary for its built-in convenience of representing a mapping from keys to values, perfect for denoting relationships between vertices. On the other hand, Java, being a statically typed language, employs arrays of LinkedLists to simulate adjacency lists. Let's dig even further into specifics:
- Stack implementation: In Python, you explicitly create a stack using a list and use list methods like append() and pop() to add and remove elements. In contrast, in Java, the call stack built into the JVM is used implicitly, with recursion serving to push and pop frames from the stack.
- Keeping track of visited nodes: Python uses a set to store visited vertices due to an average time complexity of O(1) for set operations, making it highly efficient. Java utilises a boolean array, using the index positions as the vertices and marking the corresponding indices as true for visited vertices.
- Way of implementing the DFS traversal: Python’s DFS implementation is iterative while Java’s DFS utilises recursion. This doesn't affect the logic of DFS but it's relevant when discussing space complexity.
Depth First Graph Search: A Comparative Analysis
Performing a more detailed analysis of both implementations, let's consider the algorithm's time complexity, space complexity, readability, and adaptability.In conclusion, while the underlying Depth First Search algorithm remains constant across languages, how it's leveraged via language features can differ quite significantly. By understanding these differences, you can select the optimal language for your specific needs when employing DFS in your projects.
- Time Complexity: Regardless of whether we're discussing Python’s or Java’s implementation, the DFS algorithm's time complexity is \(O(V+E)\), where \(V\) and \(E\) are the number of vertices and edges in the graph respectively. This is because each vertex and each edge will be visited in the DFS traversal.
- Space Complexity: In Java, the inherent call stack associated with recursion contributes to the space complexity. Thus, the worst-case space complexity for Java’s implementation is \(O(V)\) if the graph becomes skewed, taking the form of a linked list. Python's space complexity, however, relies heavily on the built list-based stack, and can also switch between \(O(V)\) and \(O(log(V))\) based on the depth and breadth of the graph.
- Readability: Python's implementation tends to be more readable due to the simplicity of the Python language, availability of powerful data structures like sets and dictionaries, and the lower number of lines of code. However, Java with its strong type system can provide more compile-time checks and can prevent potential runtime errors.
- Adaptability: With Python’s DFS implementation, there's flexibility to manually manage the stack and control over what gets pushed or popped, making it adaptable for variations in DFS applications. However, Java’s recursive approach can be significantly more challenging to manage and adapt for non-standard use-cases of DFS.
Depth First Search - Key takeaways
- Depth First Search (DFS) is a fundamental algorithm in computer science used for traversing or searching graph or tree data structures. Its core principle is to go as deep as possible into the structure, then backtrack once all possible avenues from a given vertex have been explored.
- DFS algorithm works by starting at a selected node and exploring the neighbour nodes at the current depth prior to moving on to nodes at the next depth level.
- In DFS, vertices (nodes), edges and the stack data structure, which tracks the traversal, are the key components. Nodes can be visited or unvisited, edges can be directed or undirected, and the stack is used to store the vertices being explored.
- DFS can be implemented in various programming languages, such as Python and Java. In Python, a DFS algorithm can be represented using lists and dictionaries, while in Java, a HashMap can be used to store vertices and their corresponding adjacency lists.
- The implementation of DFS can differ between languages due to their inherent characteristics. For instance, Python uses an iterative approach while Java is based on recursion. However, the underlying logic of DFS remains the same in all implementations.
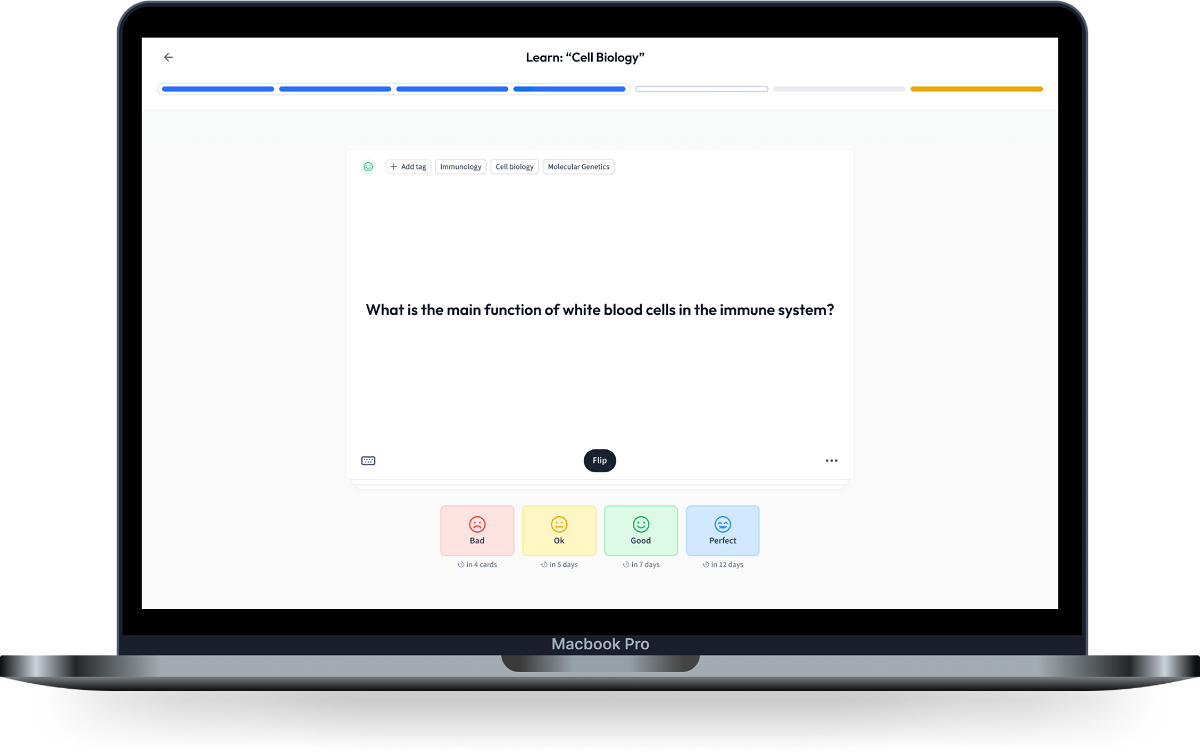
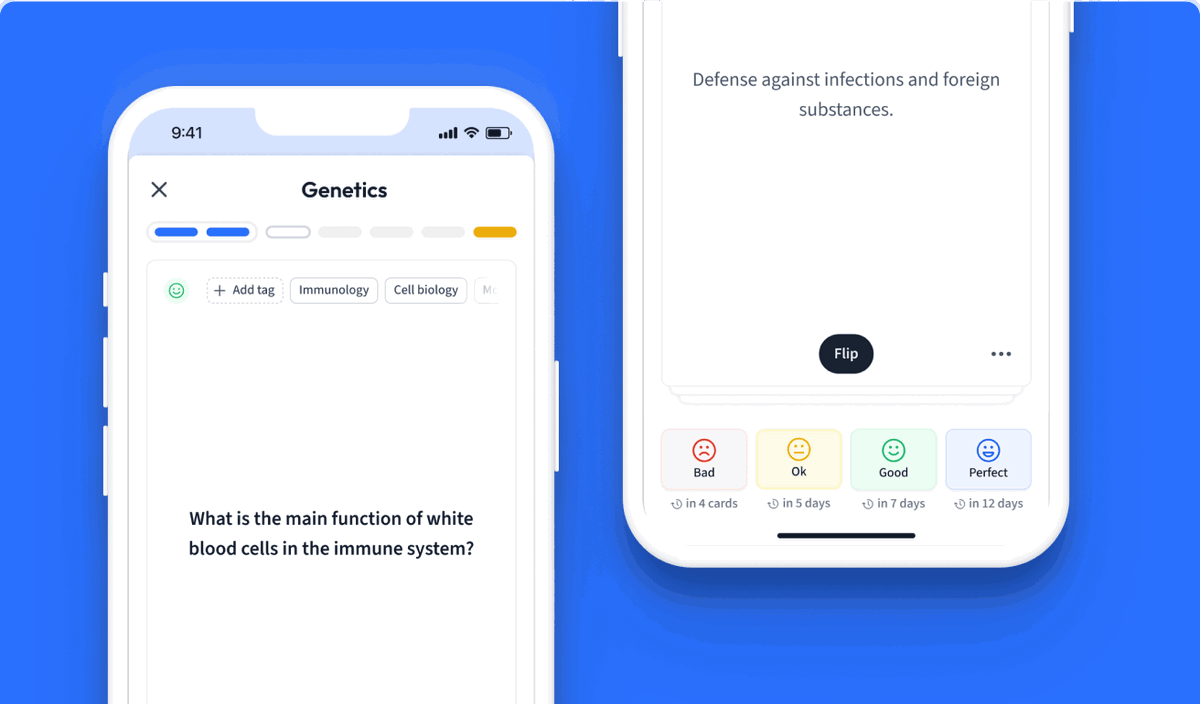
Learn with 12 Depth First Search flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Depth First Search
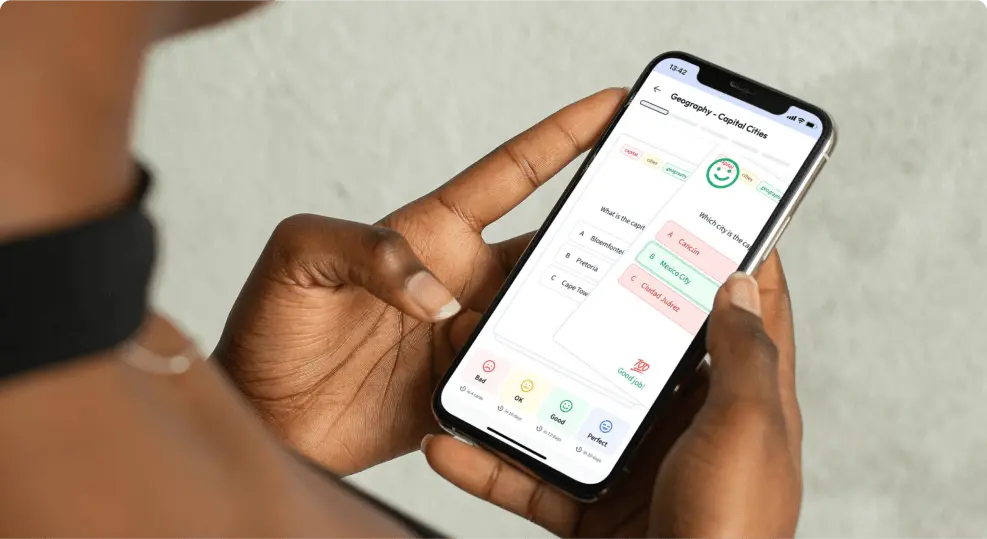
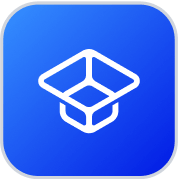
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more