Jump to a key chapter
Understanding the Concept of Framework in Computer Science
The concept of a framework in computer science might at first seem complex, but it is, in fact, a simple and powerful tool that aids in creating applications, be they web, mobile, or desktop. Simply put, a framework is a platform for developing software applications. It provides a foundation on which software developers can build programs for a specific platform.
A framework, in general, is an environment that offers functionality and structure, making the process of software development more controlled and efficient. It's a sort of platform which facilitates the coding process, reducing the time and effort required.
Defining Framework in the Context of Computer Science
In computer science, a framework is a concrete or conceptual platform where common code with generic functionality can be selectively specialized or overridden by developers or users. Much like a physical framework, it offers structure and guidance on where to place elements, but it also offers a great deal of flexibility, allowing the developer to fill in the details as they see fit.
A software framework is an abstraction in which software, providing generic functionality, can be selectively modified by additional user-written code, thereby providing application-specific software.
An instance worth sharing is Django, a python web framework. It includes several packages that can help in developing a web application. These packages include authentication, forms, an administrative interface, and a templating engine. The developer does not need to spend time reinventing these, they can use Django's, and spend more time on application logic.
Importance and Role of Frameworks in Programming
Frameworks play a crucial role in programming by enabling developers to avoid the hassle of starting from scratch every time they write a new program. They provide a structure and a set of protocols for building applications, thereby promoting code reusability and modular programming.
- Frameworks support the development of faster, more reliable and more efficient software.
- They offer libraries of coding modules and helpers, which help in preventing repetitive coding tasks.
- They facilitate best programming practices by abstracting away some of the complexities of non-functional aspects, such as row security and transaction handling.
The .NET framework by Microsoft is an example of this. It offers tools and libraries to build applications and manage execution environment, thereby simplifying development and making it much more efficient.
It's intriguing to note that certain frameworks, like AngularJS, have gained immense popularity because of their principles of declarative programming for building user interfaces. This style of setting up programming can be contrasted with that of jQuery: a direct manipulation style involving traditional imperative programming with a list of operations to perform, rather than declaring the final state.
Deep Dive into Different Types of Framework
There are numerous types of frameworks in the realm of computer science. Primarily created to aid developers in the process of development, they fall into several categories depending on the nature of the task they are designed to assist with. In the following sections, let's delve deeper into some notable types: the .NET Framework, the Spring Framework, and the Entity Framework.
Exploring the .NET Framework
Often referred to as just .NET, the .NET Framework is a software development platform that was developed by Microsoft. It runs primarily on Microsoft Windows and supports a plethora of programming languages, including C#, Visual Basic, and F#.
.NET Framework is a versatile platform with a broad collection of class libraries, which include pre-packaged sets of classes that aid in accomplishing a variety of tasks. It is important to note that .NET Framework is language-agnostic, meaning you can use any .NET language to develop .NET applications.
The .NET Framework includes a large class library known as FCL (Framework Class Library) and provides language interoperability across several programming languages.
A simple .NET program is typically coded using Visual Studio and written in C#. Here is an example of C# code for a "Hello World" program:
using System; namespace HelloWorldApp { class Geeks { static void Main(string[] args) { Console.WriteLine("Hello World!"); Console.ReadKey(); } } }
Learning about the Spring Framework
The Spring Framework is a comprehensive open-source application framework and inversion of control container for the Java platform. It is often used for creating sophisticated, reliable, and efficient enterprise-level applications. Being a comprehensive solution, it brings together several well-integrated modules like Spring JDBC, Spring ORM, Spring Web MVC, and others which provide everything you need to develop an application.
The Spring Framework provides a comprehensive programming and configuration model for modern Java-based enterprise applications - on any kind of deployment platform.
A central focus of the Spring Framework is Inversion of Control (IoC) which is a design principle used to invert different kinds of controls in object-oriented design to achieve loose coupling.
The Spring Framework implementation uses a special variant of IoC called Dependency Injection (DI). This allows for dividing an application into different components, which are interconnected without being dependent on each other.
Here is a basic piece of code in a Spring-powered application, showing the creation and usage of a Java bean:
// Create a Hello World Bean public class HelloWorld { private String message; public void setMessage(String message){ this.message = message; } public void getMessage(){ System.out.println("Your Message : " + message); } } // Create the Spring Container and Use the Bean ApplicationContext context = new ClassPathXmlApplicationContext("Beans.xml"); HelloWorld obj = (HelloWorld) context.getBean("helloWorld"); obj.getMessage();
Understanding the Entity Framework
The Entity Framework is an open-source object-relational mapper (ORM) that enables .NET developers to work with a database using .NET objects. Simplifying database access by eliminating the need for most data access code, it enables developers to focus more on the business logic of the application.
The framework produces data-centric applications that are efficient and robust, supporting a wide range of database systems including Microsoft SQL Server, Oracle, MySQL, and PostgreSQL to name just a few.
The Entity Framework is a set of technologies in ADO.NET that support the development of data-oriented software applications.
The Entity Framework can automatically create (or update) database-schema from your model-classes and vice-versa, and it supports a technique called Code First that provide full control over the code rather than database activity.
Below you can see a simplified example of two classes in C# that define a User and a Role:
public class User { public Guid UserId { get; set; } public string Username { get; set; } public virtual Role Role { get; set; } // Navigation Property } public class Role { public int RoleId { get; set; } public string RoleName { get; set; } public virtual ICollectionUsers { get; set; } // Navigation Property }
These User and Role classes can be used in a DbContext:
public class MyContext : DbContext { public DbSetUsers { get; set; } public DbSet Roles { get; set; } }
Practical Application of Frameworks in Code
Frameworks are essential instruments in any software development project. They simplify the coding process by providing a pre-established architecture and set of libraries developers can incorporate into their applications. Proper understanding and application of frameworks can greatly improve the quality of your code and the efficiency of your software development process.
Overview of How To Use Frameworks in Code
To integrate a framework into your code, you need to have a general comprehension of the framework's architecture and functionality. Each framework has its specific set of instructions, modules, and libraries that you will need to utilise to implement your program successfully. Here are some general steps to follow when incorporating a framework:
- Understanding the Framework: Learn about the structure of the framework, its components, and libraries.
- Install the Framework: Follow the specific instructions provided by the framework's developer. This could be via a package manager or downloaded directly from a website.
- Integrate the Framework: Update your files to include the necessary code snippets or import statements for the framework. This could be adding a script tag in HTML for JavaScript frameworks or an import statement in Python.
- Utilise the Framework: Use the functions and features provided by the framework in your code.
- Test Your Application: Ensure your application works as expected after implementing the framework.
A common use case is the integration of Bootstrap, a popular CSS framework, into a website design. Firstly, you need to understand what Bootstrap offers, such as grid layout and responsive design. Secondly, you would install Bootstrap by downloading it or linking via a Content Delivery Network (CDN) in your HTML file. Afterwards, you would adjust your HTML to use Bootstrap classes for layout and styling, and finally, test the visual appeal and responsiveness of the website.
Deciphering Examples of Software Frameworks
There are myriads of software frameworks available in the market, each with its unique features, advantages, and uses. Some of the most common ones include AngularJS for web applications, Spring for enterprise Java applications, and .NET for Windows applications. Let's take a deep dive into these three:
AngularJS is a JavaScript-based open-source front-end web application framework developed by Google. AngularJS's main aim is to simplify both the development and the testing of web applications by offering a framework for Model–View–Controller (MVC) and Model–View–ViewModel (MVVM) architectures, along with components commonplace in rich internet applications.
An example of using AngularJS would be creating a single-page application that dynamically updates as users interact with it. This could include a feature that filters products on an e-commerce site without requiring the page to reload. Here is an example of how data binding in AngularJS works:
Name:
You wrote: {{ name }}
Spring is an application framework and inversion-of-control (IoC) container for the Java platform. The container is responsible for the life cycle of the beans or objects that make up an application. This could range from creating a bean to finally disposing of it. The Spring container uses DI to manage the components that make up an application.
An application using Spring might, for example, include a user login feature. The data entered by the user would be collected by a controller and passed into a service. The service utilises a repository bean to interact with the database and validate the user's credentials. Additionally, Spring Security could be utilised to ensure user data is handled securely:
@RestController public class LoginController { @Autowired private LoginService loginService; @GetMapping("/login") public String loginUser(@RequestParam String username, @RequestParam String password) { return loginService.login(username, password); } }
.NET is a free, cross-platform, open-source developer platform for building many different types of applications. With .NET, you can use multiple languages, editors, and libraries to build for web, mobile, desktop, gaming, and IoT.
In a .NET application, you might create a form where customers could enter their details to join a mailing list. The application could be connected to a SQL database to store the customer's details, and all of this could be done utilising .NET's library:
public class CustomerController : Controller { private CustomerContext _context; public CustomerController(CustomerContext context) { _context = context; } [HttpPost] public IActionResult Create(Customer customer) { _context.Add(customer); _context.SaveChanges(); return RedirectToAction("Index"); } }
The Impact of Framework on Computer Networks
When discussing the impact of frameworks on computer networks, it's important to remember that a framework provides a standardised set of practices and tools that help achieve a particular goal efficiently and effectively. In a computer network, a framework can offer an essential structure for operations such as protocol definition, network management, and traffic control, which are key to the seamless functioning of a computer network.
Relationship between Framework and Computer Networks
Firstly, a framework establishes some ground rules and mechanisms for how different components in a computer network should interact with each other. This aspect is particularly crucial in ensuring reliable and seamless data transfer across the network, where different devices may have varying capabilities. A well-made framework can also contribute to a more efficient use of network resources by offering tactics like load balancing or task allocation.
Moreover, a comprehensive network framework also defines protocols to recover from abnormal scenarios, theoretically ensuring uninterrupted operation of the network.
Let's break down further to understand the intrinsic relationship between frameworks and the various components of computer networks:
- Protocol Definition: In a computer network, protocols define how data is transmitted, received, and interpreted. A good networking framework typically specifies these protocols to ensure that all network elements are on the same page. Open Systems Interconnection (OSI) is one of the prominent examples of such a framework.
- Transmission Control: This concept relates to effective data transfer in a computer network. By utilising a precise framework, it's possible to optimise the rate of data transmission to increase speed and reduce congestion.
- Error and Flow Control: When transmitting data over networks, errors are inescapable. A well-formulated framework can anticipate and quickly respond to these issues, reducing delays and ensuring data integrity.
In essence, the framework to computer networks is similar to the role of the constitution to a country. It clearly lays out the dos and don’ts, ensuring smooth operation, data exchange, and efficient management of the network.
Influence of Frameworks in Enhancing Computer Networks
The influence of frameworks on enhancing computer networks is quite significant. From seamless data transmission to robust security, the impact of frameworks is visible in all aspects of a computer network. Let's talk about three main ways in which frameworks contribute to computer networks:
- Data Flow Management: Frameworks play a crucial role in effectively managing the flow of data in a computer network. This involves optimising the network for efficient data transmission and regulating network traffic. Given these functionalities, a framework can make a network faster and more productive.
- Resource Management: They also manage network resources effectively by determining the best path for data transmission, ensuring effective bandwidth usage, and implementing fail-safe mechanisms in the face of potential network failures. The more structured and orderly the framework, the better resource utilisation it offers.
- Security Enhancements: Security is a critical aspect of computer networks. A good framework provides security guidelines and mechanisms for data encryption, access control, and network surveillance. This ensures that data is protected against unauthorised access or malicious attempts, making the network more secure.
A more case-specific representation is the role of frameworks in wireless networks. Here, frameworks like 802.11 provide protocols that improve data transmission speed by using different frequencies and channels. Frameworks also enable features like Quality of Service (QoS) to be implemented, allowing prioritisation of different types of data packets, which ensures the seamless operation of time-sensitive applications such as video conferencing or streaming services.
Similarly, frameworks like OpenFlow influence the field of Software-Defined Networking (SDN), enabling more flexible and efficient network management by separating control and data planes, and thereby further enhancing network performance.
To summarise, computer network efficiency relies heavily upon the effectiveness of the frameworks in use, highlighting the significant role they play. From the moment you do a Google search to watching a Netflix series, it's frameworks at the back-end ensuring that data packets reach your device efficiently and reliably.
Framework - Key takeaways
- A Framework in computer science is a predefined set of tools, libraries and best practices that help in application development.
- The .NET framework by Microsoft simplifies development and makes it more efficient by offering tools, libraries and a managed execution environment.
- The Spring Framework is an open-source application framework and inversion of control container for the Java platform, often used in enterprise-level application development.
- The Entity Framework is an open-source object-relational mapper (ORM) enabling .NET developers to work with a database using .NET objects.
- Frameworks improve the quality of code and the efficiency of the software development process by providing a pre-established architecture and set of libraries.
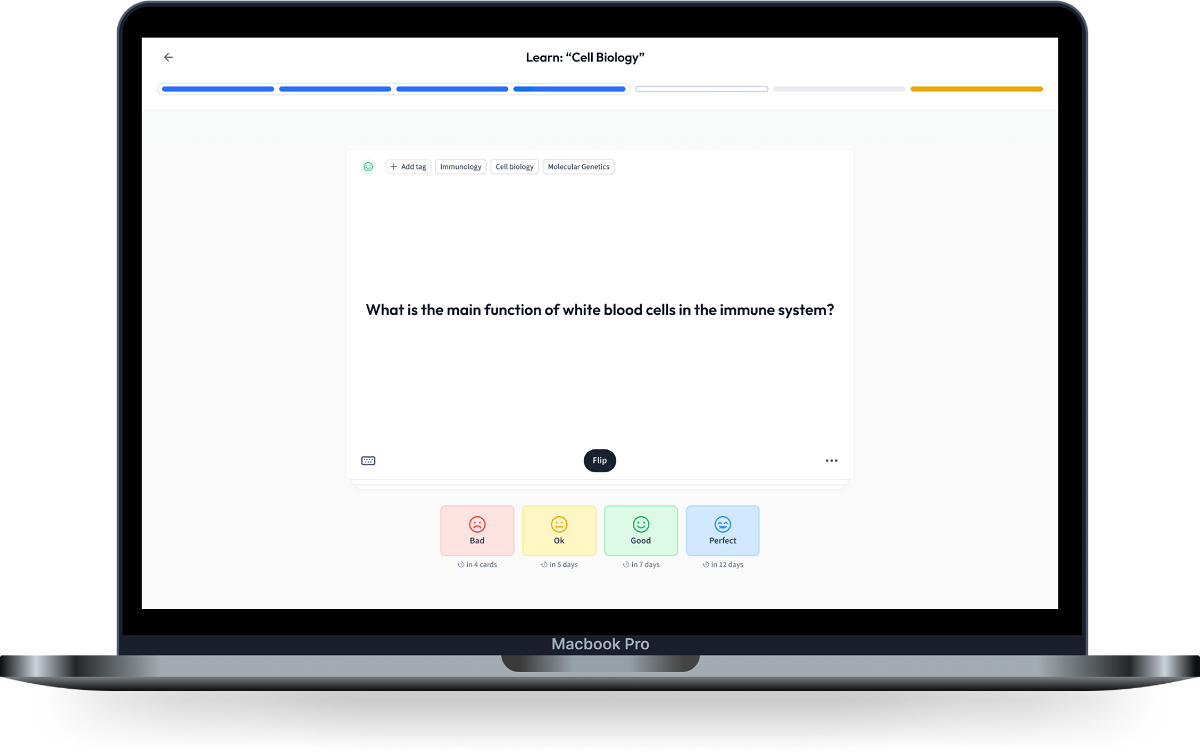
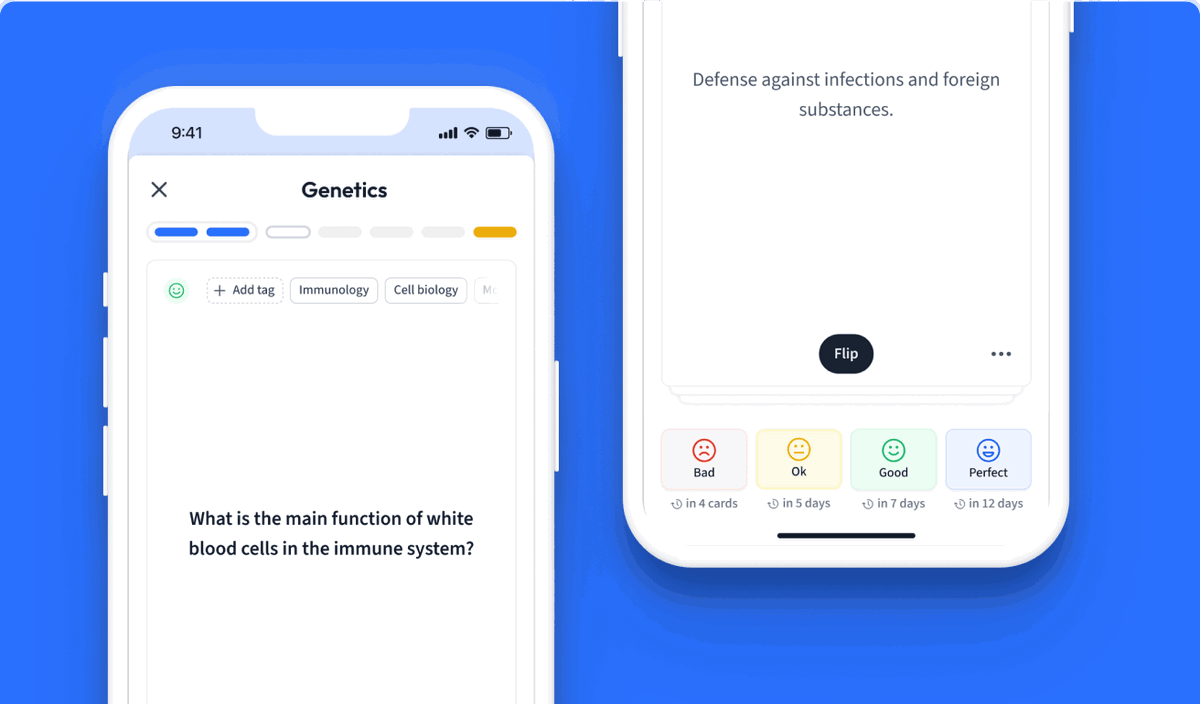
Learn with 12 Framework flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Framework
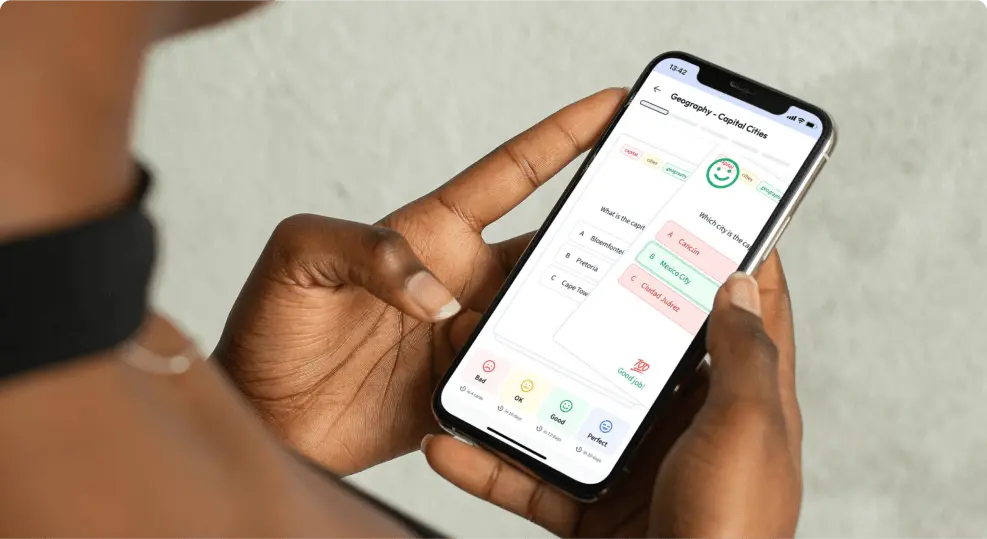
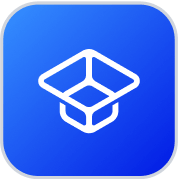
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more