Understanding the Android Operating System
In your computing journey, understanding the Android operating system may often emerge as a significant topic. The Android operating system is a mobile operating system based on a modified version of the Linux kernel and other open source software.An operating system, or OS, is a software that communicates with the hardware and allows other programs to run.
Core Characteristics of the Android Operating System
The Android operating system is known for its distinctive features that set it apart from other mobile operating systems.- Open Source: The source code of Android is freely available. This allows for increased compatibility and adaptability.
- Compatible: Android can operate on various devices, from smartphones to televisions and cars.
- Customizable: Users can modify and customize the user interface of Android to their preference.
For instance, Android users can use launchers to change their device's look and feel, from changing the icons to the entire layout.
Exploring Android OS Architecture
Delving into the architecture of Android, you will come across a layered setup. A multi-layered architecture allows for better compartmentalization and easier troubleshooting when an issue arises.Components of Android OS Architecture
The Android OS Architecture consists of five layers:1. Linux Kernel | This is the foundation of the Android platform. It provides a level of abstraction between the device hardware and the upper layers of the Android software stack. |
2. Libraries | These are a set of codes that allow tasks of the operating system to execute successfully. |
3. Android Runtime | This includes core libraries and the Dalvik Virtual Machine. |
4. Application Framework | These are services like Activity Manager, Resource Manager etc. which can be used by all applications. |
5. Applications | This involves all the apps that you interact with on your Android device like Phone, Contact, Browser, etc. |
Understanding the Android Software Stack
The Android Software Stack refers to the collection of software components that comprise the Android architecture. These include Linux Kernel, Libraries, Android Runtime, Application Framework, and Applications. Understanding this stack can provide insightful knowledge of how requests are handled, processes are managed, and tasks are executed in an Android system.Linux Kernel | Libraries | Android Runtime | Application Framework | Applications
Did you know? Android makes up more than 80% of all smartphone operating systems worldwide.
Deep Dive into Android Programming and Development
Unveiling the layers of Android programming and development could be rather intriguing. Android development might seem complex, but understanding the underlying concepts can significantly simplify the process.Essential Android Programming Concepts
Android programming is vast, encompassing multiple concepts and principles. Here are a few essential ones that you should learn about:- Activity LifeCycle: An activity in Android is a single screen with a user interface. The lifecycle of an activity, right from its creation to its destruction, involves various callback methods such as onCreate(), onStart(), onResume(), onPause(), onStop(), onDestroy(). Understanding the activity lifecycle can help manage resources optimally.
- Intent: In Android programming, an Intent facilitates communication between components. It allows you to either transition from one screen to another or to carry out an action, such as opening a URL or dialling a phone number.
- Services: A Service in Android is a long-running operation running in the background and does not provide a user interface.
- Broadcast Receivers: Broadcast Receivers in Android respond to system-wide broadcast messages. It could be system-generated, such as device booting up or battery low, or application-generated.
Practical Android Code Examples to Understand Better
Seeing the concepts in action can better enhance understanding. Here are some code examples for better clarity. To start an activity using an Intent:Intent intent = new Intent(getApplicationContext(), SecondActivity.class); startActivity(intent);To start a service:
Intent serviceIntent = new Intent(getApplicationContext(), MyService.class); startService(serviceIntent);Registering a Broadcast Receiver in an Activity:
BroadcastReceiver receiver = new MyReceiver(); IntentFilter filter = new IntentFilter("android.intent.action.BATTERY_LOW"); registerReceiver(receiver, filter);
The Basics of Android App Development
Android App Development involves a series of steps and processes. From setting up the development environment in Android Studio to publishing your app on Google Play Store, every stage has its importance.- Environment Setup: The first step is to set up Android Studio, the official IDE for Android app development. You need to install the Java Development Kit (JDK) and Android SDK (Software Development Kit).
- Project Creation: After setting up the environment, you create a new project, selecting the appropriate Android SDK version and template.
- Application Development: On creating the project, you write code and develop your application, typically using Java or Kotlin. This involves creating multiple activities, adding user interface elements, and defining their behaviours.
- Testing: Once the application is ready, it must be thoroughly tested. You can test the app on an Android Emulator or a real device.
- Deployment: After successful testing, you publish your application on the Google Play Store.
Utilising an Android Emulator in App Development
An Android Emulator is a tool that allows you to run Android applications on your computer. It mimics the functions of an Android device. It is essential in the development process for testing applications. To start an emulator, follow these steps:// List all available emulators emulator -list-avds // Start an emulator emulator -avd [YourEmulatorName]Yes, Android Emulator is a great tool, but don't forget to test your application on a real Android device. Different devices have different characteristics like screen sizes, pixel densities and hardware capabilities, and running your app on an actual device provides a more authentic estimate of your application's performance.
Mastering the Android Device Manager and SDK
The Android Device Manager and SDK are two crucial tools that you might want to master if you're interested in Android development. While the Android Device Manager helps manage your Android devices with ease, the Android SDK or Software Development Kit provides you with all the necessary tools for creating Android applications.The Functionality of the Android Device Manager
The Android Device Manager, now known as "Find My Device" by Google, plays a vital role in managing your Android devices. It is a security feature that helps locate, ring, or wipe your device if you happen to lose it or it gets stolen. To use these features, you must set up the Find My Device app on your Android device and sign in to your Google Account. Google uses the location data from your device to show its location on the map. You can make your device play a sound even if it's on silent. If you're certain that your device has been stolen, you can also remotely erase all data on your device. But be cautious with this step as it's irreversible, and you won't be able to locate your device after it's erased.Ensure to always keep your device's location turned on and be signed in to Google Play Store for the Find My Device to function properly.
Managing Your Devices with Android Device Manager
Harnessing the power of the Android Device Manager, or the new "Find My Device" functionality, you can take several actions to either recover your lost phone or protect precious data. Before you can utilise the features, ensure the following are enabled on your device:- Location: ON
- Find My Device: Turned ON
- Google Play visibility: ON
- Play Sound: Makes your device ring at full volume for five minutes, even if it's set to silent.
- Secure Device: Locks your screen and displays a message with an option to call back your number. This is helpful if you hope a good Samaritan may find and return your device.
- Erase Device: Permanently deletes all data on your phone but does not delete content on your SD cards. After you erase, Find My Device won't work on the phone.
The Android SDK Explained
The Android Software Development Kit (SDK) is a collection of software development tools in one installable package. It essentially provides everything you require to start creating applications for the Android platform. The kit contains code samples, software libraries, handy developer tools, and even an emulator for you to test your budding Android applications. Android SDK allows you to create Android apps using APIs provided by Google. This way, your apps can interact with Google services like Google Maps, Firebase, Google Drive and more.APIs, or Application Programming Interfaces, are a set of rules and protocols that let one software communicate with another.
Utilising SDK in Android App Development
Android SDK is the cornerstone in developing Android apps. Here's how you can utilise it: First, you must install a suitable IDE (Integrated Development Environment) like Android Studio or Eclipse, which comes with the Android SDK. Android Studio is the official IDE for Android, and it greatly simplifies the process of developing Android apps. A typical workflow involving Android SDK might include the following stages:- Setting Up: Install the Android SDK, set up a development environment (IDE), and create a new project.
- Developing: Write code using Java or Kotlin, design your app, and integrate various features using Android APIs.
- Testing: Test your app on the Android Emulator or a real Android device. The Android Emulator is part of the Android SDK.
- Debugging: Use the Android Debug Bridge (ADB - a versatile command-line tool part of Android SDK) to debug your apps.
- Deploying: Finally, compile your app into an APK file, sign it using the Android system’s Signing Your Applications tool (part of the SDK), and then you can distribute it on Google Play Store or other platforms.
Securing Your Android Device
Shifting focus to an aspect that's as crucial as learning about the Android programming or ecosystem - its Security. With millions of users worldwide and a plethora of sensitive data, securing Android devices becomes a vital task. Android provides several security features like sandboxing, secure interprocess communication, secure boot, encryption, and more. Leveraging these features, users can shield both their devices and data against potential threats.Understanding the Android Security Architecture
Diving deep into the Android security architecture, it's pivotal to note that it operates in a multi-layered fashion. Its design aims to safeguard both the device and the data from threats at several levels.At its core, the Android Security Architecture is built around a Sandbox model. Sandboxing keeps applications from interfering with each other. Each Android app runs in its own sandbox and cannot access other apps' data or system resources unless they have suitable permissions. This process keeps the app's components isolated, offering a robust shield against malicious activities.
Importance of Android Security Architecture
The Android Security Architecture is of paramount importance when it comes to safeguarding the extensive user base Android caters to. Its multi-layered approach of defence allows for enhanced protection against various types of security threats. Here's why the Android Security Architecture holds such significance: - App Isolation: Each app runs within its own sandbox, providing a crucial layer of data security. This condition restricts any malicious app from accessing data from other apps. - Protection against Malware: With Google Play Protect consistently scanning the device, exposure to harmful applications is significantly reduced. - Data Encryption: Encrypting user data adds another layer of security, making it challenging for unauthorised users to access the data. - Secure Boot: Android devices employ a secure boot process that checks and verifies each stage's integrity during startup. - Regular Updates: Android provides regular security updates, including patches for any newly discovered vulnerabilities, ensuring continued protection for the devices. - Manage App Permissions: User control over app permissions is a critical aspect of privacy protection in Android. Users decide what kind of access to grant and can revoke these permissions at any point.For instance, if a gaming application tries to access your contacts, Android prompts you to allow or deny the permission. If you believe the app has no valid reason to access your contacts, you can deny this permission, safeguarding your privacy.
Overview of Technical Aspects of Android
As you delve deeper into the world of Android, you'll encounter a variety of technical aspects that are at the heart of this expansive mobile operating system. From its unique software stack that consists of an array of elements working in harmony, to the programming concepts that bring Android applications to life, there's an extensive range to explore and comprehend.In-depth Look at Android Software Stack
Taking an in-depth look at the Android Software Stack, we find it to be a vital part of the Android ecosystem, that caters to the successful functioning of Android-powered devices globally. The Android Software Stack is compromised of five layers:- Linux Kernel: This forms the base of the stack and handles input/output requests from software, memory management, as well as processes. It is also responsible for hardware drivers like display and camera drivers.
- Libraries: These are sets of instructions that help software interface with the system hardware. Examples include SQLite, webkit, OpenGL etc.
- Android Runtime: This layer homes the Dalvik Virtual Machine and Core Libraries that allow Android applications to run efficiently.
- Application Framework: These are services that applications can utilise. Examples include Activity Manager, Content Providers, View System etc.
- Applications: This is the topmost layer of the software stack, encompassing both pre-installed system applications and downloaded applications from the Play Store.
Role of Android Software Stack in the Android Ecosystem
The Android Software Stack plays a crucial role in the Android ecosystem by ensuring smooth functioning of Android devices and applications. Its role can be appreciated when you understand the stacked structure: each layer in the stack corresponds to a different component of the Android operating system and has its distinct role. With the Linux kernel handling core system functionality and hardware interoperability, libraries and Android Runtime ensuring smooth app functionality, and Application Framework providing core services, the stack collectively works together to establish a coherently functioning Android system. The Software Stack's importance also stems from its role in securing Android devices. The Linux kernel ensures that applications run within their designated sandboxes, preventing nefarious apps from accessing data they shouldn't. Application permissions within the Application Framework layer control access to phone features and data.Getting Hands-on with Android Programming Concepts
Getting hands-on with Android programming concepts can prove to be a turning point in your understanding of Android. These concepts include understanding the lifecycle of an Android application, learning about Intents and Services, and comprehending various layout designs, amongst others. For instance, the Activity Lifecycle is a fundamental concept in Android programming. An Activity represents a single screen with a user interface, and Android manages the lifecycle of this Activity using a set of callback methods that the system calls when the Activity transitions between different states. The Android framework provides several default methods to manage the lifecycle of an application, from when an application is first created with itsonCreate()method, through when it is stopped with its
onStop()method, and eventually destroyed with its
onDestroy()method.
Understanding through Android Code Examples
Comprehending through coding examples is one of the most efficient ways to understand Android programming concepts. Consider this simple example, which illustrates the lifecycle of an Android activity:public class MainActivity extends Activity { @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); } @Override protected void onStart() { super.onStart(); } @Override protected void onResume() { super.onResume(); } @Override protected void onPause() { super.onPause(); } @Override protected void onStop() { super.onStop(); } @Override protected void onDestroy() { super.onDestroy(); } }In this code, you can see how the Android system calls these lifecycle methods. When an activity first starts, the system calls the
onCreate()and
onStart()methods. Then, whenever the activity comes to the forefront, the system calls the
onResume()method. This hands-on approach not only makes the theoretical idea concrete but also allows you to experiment and learn more effectively.
Android - Key takeaways
- Android Programming Concepts: This involves the use of Intents (a facility for communication between components), Services (long-run operations happening in the background) and Broadcast Receivers (components that respond to system-wide broadcast messages).
- Android App Development: This revolves around several steps, which include Environment setup, Project creation, Application development, Testing on an emulator or a real device, and Deployment.
- Android Emulator: This is a tool that lets programmers test Android apps on computers, mimicking the Android device functions.
- Android Device Manager: Now rebranded as "Find My Device" by Google, it is a handy tool that helps in managing Android devices and provides security features such as location tracking and remote wiping of data.
- Android SDK: The Android Software Development Kit is a package of development tools that streamline the creation of Android applications, and allows developers to integrate their apps with Google services through APIs (Application Programming Interfaces).
- Android Security Architecture: This implicates a multi-layered approach that builds upon a Sandbox model to secure Android devices and user data against potential threats.
- Android Software Stack: This represents the running environment of an Android device, consisting of layers like the Application layer, Android Runtime, Native Libraries, and Linux Kernel.
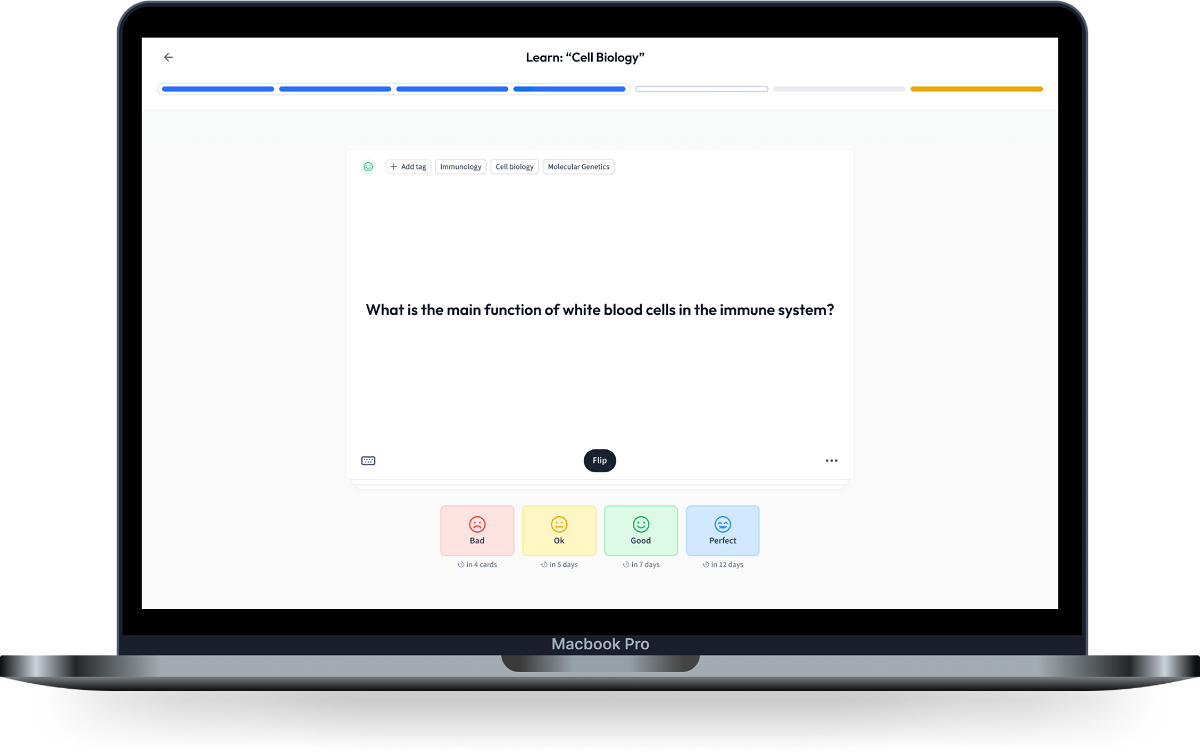
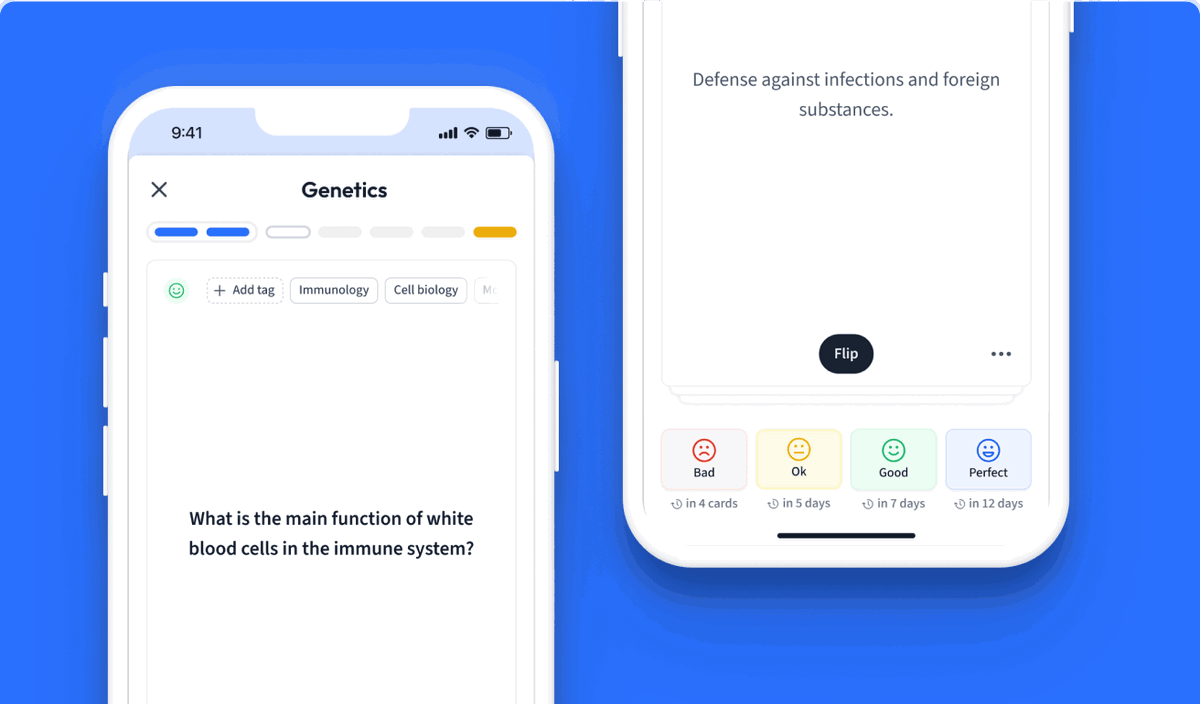
Learn with 15 Android flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Android
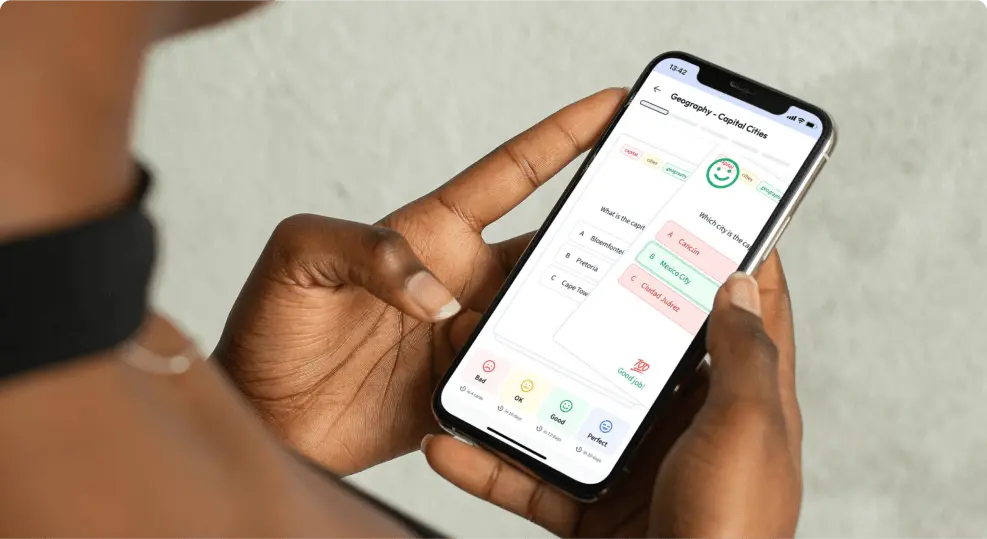
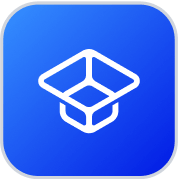
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more