What is Selection Sort in Computer Science?
Selection sort is an uncomplicated and intuitive sorting algorithm. It is often taught in beginner Computer Science courses as it illustrates the fundamentals of sorting. Despite this, it is not the most efficient algorithm for handling large data sets, but it certainly has its uses.Defining Selection Sort
Selection sort is a simple comparison-based sorting algorithm. Given an array, the algorithm searches for the smallest element, swaps it with the element in the first position, and repeats this process for the remainder of the list.
procedure selectionSort(A : list of sortable items) n = length(A) for i = 1 to n do { • // Find the least element in A[i … n] min = i for j = i+1 to n do { if (A[j] < A[min]) then min = j } swap(A[i] and A[min]) } end procedure
Key Principles of Selection Sort
The selection sort algorithm operates based on a very clear set of principles. Recognition of these principles can assist in understanding and implementing this algorithm effectively. Here's how it works: • The algorithm starts by finding the smallest (or largest, if sorting in descending order) element in the array and swaps it with the first element. • It then finds the second smallest element and swaps it with the second element of the array, and so on. • This continues until the entire array is sorted.Let’s assume you have an array [29,10,14,37,13]. The first pass of the loop finds 10 as the smallest element and swaps it with 29. The array becomes [10,29,14,37,13]. The second pass finds 14, the smallest in the rest of the array, so it swaps 14 and 29 to give [10,14,29,37,13]. This process repeats until the array is sorted.
Did you know that despite its simplicity, selection sort has various practical applications, such as in applications where memory space is a limiting factor? Additionally, selection sort tends to perform well when you need to minimise the number of swaps, as it always makes \(N\) swaps in the worst-case scenario.
Delving into Selection Sort Algorithm
Indulging further into the nitty-gritty of the Selection Sort Algorithm reveals a harmonious blend of simplicity and precision that optimises resources and ensures a successful sorting process. Developed on a few but important steps, each stage of the Selection Sort algorithm plays a significant role in manipulating the given dataset to produce the desired orderly arrangement.Role of Algorithm in Selection Sort
The fundamental role of the algorithm in Selection Sort is to organise an array of data either in ascending or descending order. This is achieved through a systematic and repetitive process of analysis and decision making. Below are the key roles the algorithm plays:- Scans the entire array: It starts from the first element, treating it temporarily as the smallest, and scans through the entire array to search for any smaller element.
- Identifies the smallest element: After scanning the complete array, it identifies the smallest element.
- Swaps elements: Upon identifying the smallest element, the algorithm swaps it with the first element of unsorted part of array.
- Iterates: Next, it moves to the second element and repeats the process until the whole array is sorted.
Step by Step Breakdown of Selection Sort Algorithm
Breaking down the Selection Sort's entire process, we uncover a series of methodical steps that lead to the algorithm's successful execution:- The algorithm begins with the initialisation stage, in which it recognises the first element of the array as the smallest.
- It then continues to compare this item to the rest of the unsorted section of the array in search of an even smaller item.
- Upon succeeding in its quest, the algorithm swaps the positions of the two elements, with the smaller one moving to the sorted section of the array.
- The procedure is repeated with the rest of the array until no unsorted elements remain.
Initialisation in Selection Sort Algorithm
The initialisation process sets the groundwork for the entire sorting operation. The algorithm starts by identifying the array's first unsorted element as the smallest. This element serves as the reference point for the remaining unsorted array elements and is compared with each of them to verify if indeed it is the smallest element. The selection sort algorithm can be written as follows:// The outer loop iterates from first element to n for (min = 0; min < n-1; min++) { // The inner loop finds the smallest value for (j = min+1; j < n; j++) { // Comparing each element with the first element if (arr[j] < arr[min]) { min = j; } }
Swapping Process in Selection Sort Algorithm
Swapping constitutes the highlight of the Selection Sort Algorithm. After identifying the smallest element in the unsorted part of the array during the initialisation stage, the algorithm proceeds to swap this element with the first element of the unsorted part of array. This is how the swap operation is implemented:// Swapping the minimum value with the first unsorted value temp = num[min]; num[min] = num[i]; num[i] = temp;Each swap shifts the smallest remaining number from the unsorted section to the end of the sorted section until all numbers are sorted. This constant swapping repeats until the algorithm is left with a single unsorted element—the largest. Since it's the only one left, it logically takes the last place, marking the completion of the entire sorting process. The outcome is a neatly arranged array making the Selection Sort Algorithm both a fascinating and efficient approach to array sorting in Computer Science.
Selection Sort Across Programming Languages
Numerous programming languages offer support for the implementation of the Selection Sort algorithm. With slight variations to cater to each language's unique syntax and conventions, the algorithm is comparably implemented in all. Now, let's explore specific examples: Java and C++.Implementing Selection Sort in Java
Java, an object-oriented programming language, offers robust support for Selection Sort. As powerful as it is versatile, Java allows precise execution of this algorithm. Here is the Java code for the Selection Sort algorithm:public class SelectionSort { void sort(int arr[]) { int n = arr.length; for (int i = 0; i < n - 1; i++) { int minIndex = i; for (int j = i + 1; j < n; j++) { if (arr[j] < arr[minIndex]) { minIndex = j; } } int temp = arr[minIndex]; arr[minIndex] = arr[i]; arr[i] = temp; } } }After running this code, get a sorted array as output.
Selection Sort Java Code Breakdown
Let's delve into the details of each line to better understand how this algorithm works in Java:void sort(int arr[]) {This code initiates the method "sort" where \( arr[] \) refers to the input array.
int n = arr.length;The length of the input array is stored in \( n \).
for (int i = 0; i < n - 1; i++) { int minIndex = i;The loop starts sorting from the first number, and the variable "minIndex" is used to store the index of the smallest element found.
for (int j = i + 1; j < n; j++) { if (arr[j] < arr[minIndex]) { minIndex = j; } }A nested for-loop is used to search through the unsorted part of the list to identify potential minimum elements.
int temp = arr[minIndex]; arr[minIndex] = arr[i]; arr[i] = temp;Swapping the smallest unsorted element (found at index minIndex) with the first unsorted element.
Utilising Selection Sort in C++
C++, another multifaceted programming language, supports Selection Sort, demonstrating its deterministic approach in a succinct manner. Be it for large or small arrays, C++ handles the algorithm with an unequivocal efficiency. Here's the code for implementing Selection Sort in C++:void selectionSort(int array[], int size) { for (int i = 0; i < size - 1; i++) { int minIndex = i; for (int j = i + 1; j < size; j++) { if (array[j] < array[minIndex]) minIndex = j; } // Swap the smallest unsorted element with the first unsorted one int temp = array[minIndex]; array[minIndex] = array[i]; array[i] = temp; } }
Exploring Selection Sort C++ Code
Let’s take a closer look at the C++ version of the Selection Sort algorithm:void selectionSort(int array[], int size) {This function declaration denotes that the 'selectionSort' function receives an array and its size as input.
for (int i = 0; i < size - 1; i++) { int minIndex = i;The outer loop starts sorting from the first element, and "minIndex" is used to store the index of the currently smallest unsorted element.
for (int j = i + 1; j < size; j++) { if (array[j] < array[minIndex]) minIndex = j; }The nested loop searches the rest of the unsorted list to find potentially smaller elements, comparing and updating the minIndex accordingly.
int temp = array[minIndex]; array[minIndex] = array[i]; array[i] = temp;Finally, this code swaps the positions of the first unsorted element and the smallest remaining unsorted element, thus growing the sorted section of the list by one element. While the above-mentioned code may appear daunting at first, upon closer inspection, it becomes apparent that both Java and C++ follow a systematic repetition of similar operations brought to life by their own unique syntax rules.
Analysing Selection Sort Time Complexity
The time complexity of an algorithm significantly impacts its speed and performance. In selection sort, time complexity plays a prominent role as it helps in determining the total time taken for sorting. By examining the time complexity of selection sort, one can gain a better understanding of the effectiveness and efficiency of the algorithm, considering various numbers of inputs.Understanding Time Complexity in Algorithms
Time complexity can be thought of as the computational complexity that describes the amount of time an algorithm takes to run as a function of the length of the input. In simpler terms, it's a measure of the time taken by an algorithm to execute its instructions and produce the output, in relation to the size of the input data. The time complexity of an algorithm is commonly expressed using Big O notation. It is of three types:- Best Case: The minimum time taken for program execution. For a sorted input, it is \(O(n^2)\).
- Average Case: The average time taken for program execution. For random input, it is \(O(n^2)\).
- Worst Case: The maximum time taken for program execution. For a descending order input, it is \(O(n^2)\).
Big O notation is a mathematical notation that describes the limiting behaviour of a function when the argument tends towards a particular value or infinity.
How Time Complexity Impacts Selection Sort
In terms of time complexity, the selection sort algorithm could be considered inefficient for large datasets. The primary reason for this is that it always takes a quadratic amount of time to sort an array, regardless of the original array's order or content. This is due to the nested for-loops in the algorithm: the outer loop runs \(n\) times and the inner loop runs \(n-i\) times, where \(i\) is the current iteration of the outer loop. This constant time complexity doesn't fluctuate between best case, average case, and worst case scenarios. They all feature an algorithmic time complexity of \(O(n^2)\). As a result, the performance of the Selection Sort algorithm deteriorates swiftly as the size of the input grows. Therefore, it's not typically used for large scale or real-world applications where efficiency matters. The selection sort algorithm works as follows:for (int i = 0; i < n; i++) { int minIndex = i; for (int j = i+1; j < n; j++) { if (arr[j] < arr[minIndex]) //Searching minimum element { minIndex = j; //Updating minimum index } } swap(arr[i], arr[minIndex]); //Swapping minimum element at its proper place }The outer loop scales with every single addition to the size of the data set, and the second loop needs to compare with every other element to identify the smallest and conclude the swap. Hence it always performs \(O(n^2)\) comparisons and \(O(n)\) swaps, making a worst-case and average time complexity of \(O(n^2)\). Understanding the time complexity of algorithms, such as selection sort, is imperative for any computer science student or professional. By recognising the implications it brings to programming efficiency, one can make more informed decisions about the proper tools and algorithms to use in different programming situations.
Practical Application of Selection Sort
Through a combination of efficiency in smaller data sets and simplicity in understanding, the selection sort algorithm finds real-world usage in multiple sectors. While not optimum for larger data arrays due to its \(O(n^2)\) time complexity, its practice in teaching computational logic and algorithmic thinking cannot be discounted.Selection Sort Examples in Daily Life
Selection sort, though a simple algorithm, influences many aspects of your daily life. Think about the various times in a day when you arrange or organise things. These instances mirror the logic behind the selection sort algorithm. Imagine you have a stack of books to organise. You start by picking the smallest or the largest book (depending upon whether you want to sort in ascending or descending order). This book is then placed in the correct location, and the process is repeated until all books are sorted. The act of sorting files on a computer by name, size, type, or date modified can all be likened to the selection sort algorithm. Similarly, organising your music playlist alphabetically or by genre also mimics the selection sort methodology.Selection Sort in Real-time Computing
Due to its deterministic nature, selection sort finds application in real-time processing systems. There are specific instances in real-time computing where the predicted time of a task has to be shorter than the deadline, regardless of the size of the input data. In such cases, algorithms with predictable time behaviour, such as selection sort, are preferred over more efficient algorithms with uncertain worst-case behaviour. Even in systems where saving memory space is paramount, selection sort becomes advantageous due to the fact that it is an in-place sort. It only requires a constant amount \(O(1)\) of additional memory space to sort an array, which could be crucial when working with memory-constrained devices or systems.Importance and Limitations of Selection Sort
Understanding both the strengths and weaknesses of the selection sort algorithm is vital to effectively choosing the optimal sorting procedure for a given task. The same qualities that make selection sort valuable also set its limitations. On the one hand, its simple and intuitive nature makes it an ideal teaching tool for programming and algorithm design. The relatively consistent performance of selection sort, regardless of the initial arrangement of the data, can be beneficial in circumstances where the input data has an unpredictable order. It is also considered an efficient algorithm when the main goal is to minimise the number of swaps. On the other hand, selection sort falls short in efficiency when dealing with larger datasets because of its \(O(n^2)\) time complexity. For applications where performance is the main concern, other more complex but faster sorting algorithms, like merge sort or quick sort, may be more fitting.When to Use Selection Sort
Despite its limitations, there are circumstances where selection sort serves as the preferred choice:- Teaching Purposes: As selection sort is simple to understand and implement, it is usually the first sorting algorithm taught in computer science classes.
- Memory Efficiency: Since selection sort is an in-place sorting algorithm, it is helpful when memory is a constraint.
- Minimising Swaps: If the cost of swapping items greatly exceeds the cost of comparing them, then selection sort's swap-efficient nature proves advantageous.
Selection Sort - Key takeaways
- Selection Sort is a simple sorting algorithm with various practical applications, including instances where memory space is a limiting factor or when the number of swaps needs to be minimized.
- The selection sort algorithm organizes an array of data in ascending or descending order through multiple steps including scanning the array, identifying the smallest element, swapping elements, and iterating through the process.
- Selection Sort Algorithm can be implemented in various programming languages with some nuances to cater to each language's unique syntax and conventions, such as Selection sort Java and Selection sort C++.
- Selection Sort has a time complexity of \(O(n^2)\) in all cases (best, average, worst). This makes the algorithm inefficient for large datasets as its performance deteriorates with the increase in the size of the input.
- Despite its time complexity, Selection Sort has practical applications and is frequently used in teaching computational logic and algorithmic thinking. It is also a common method for sorting files on a computer by name, size, type, or date modified.
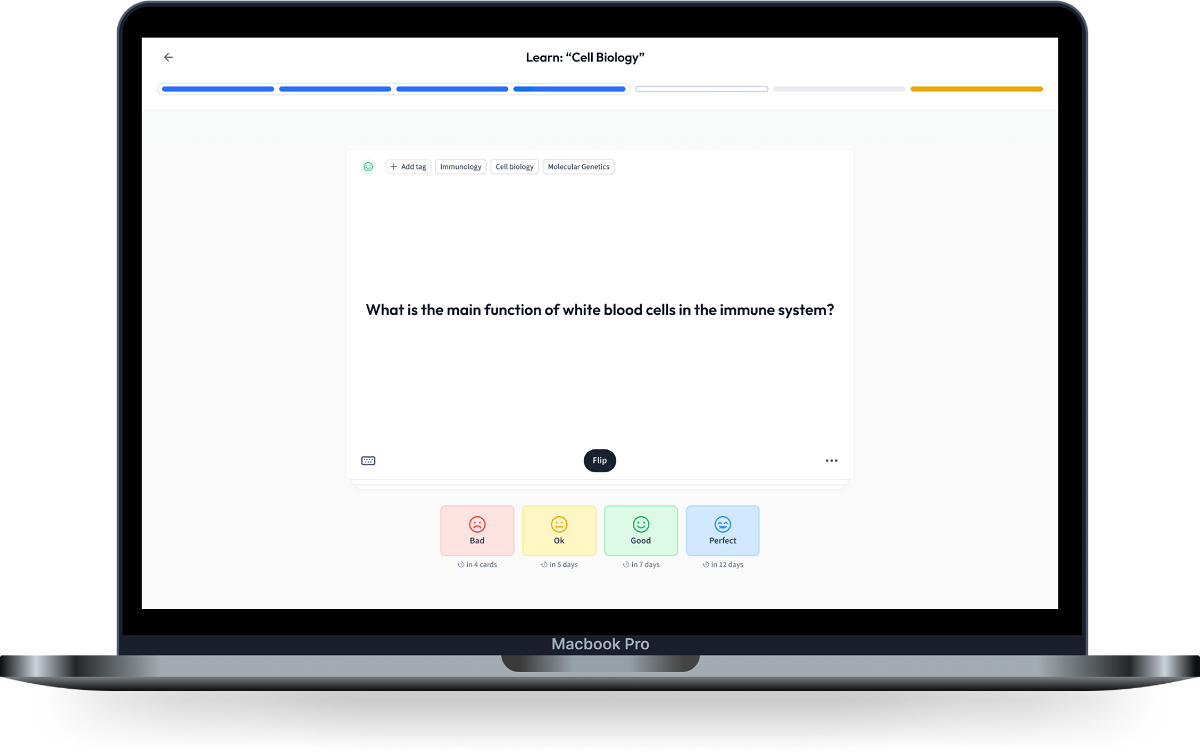
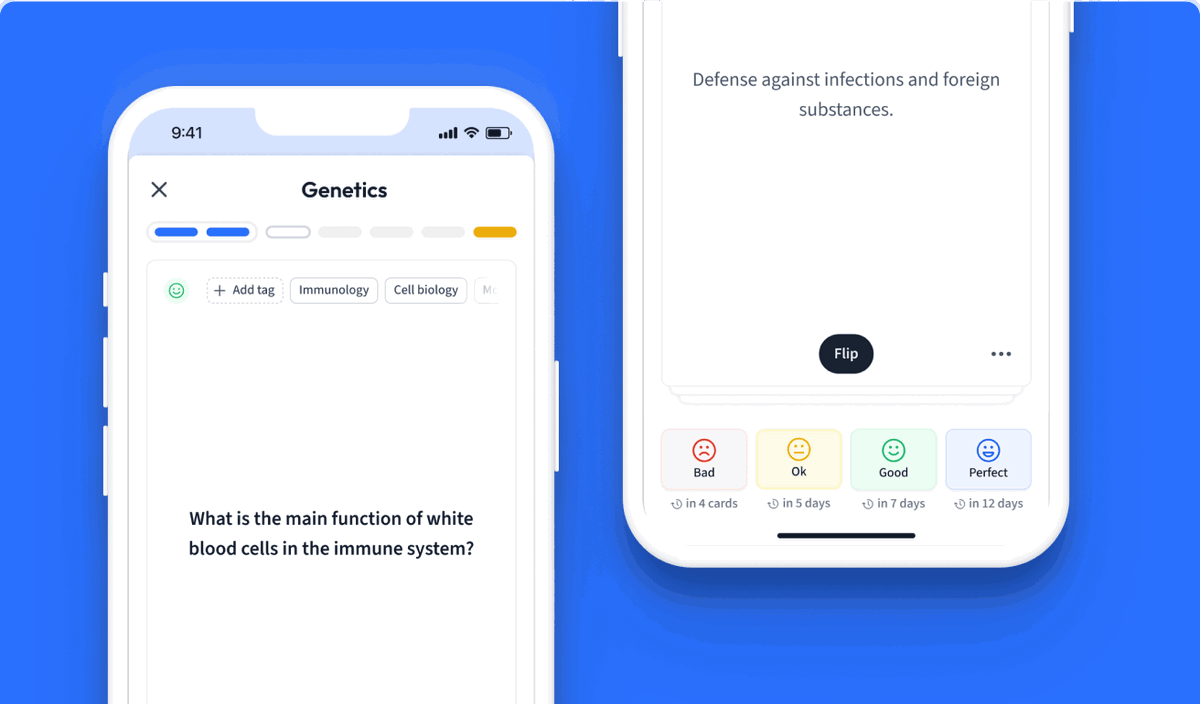
Learn with 15 Selection Sort flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Selection Sort
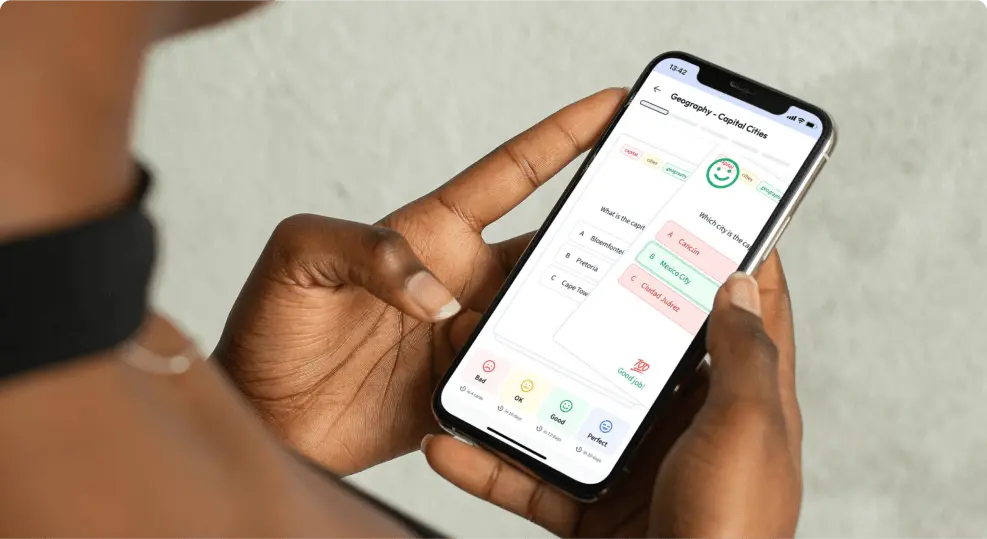
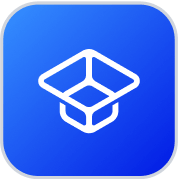
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more