Understanding the Vertex Cover Problem in Computer Science
Let's bring you closer to an interesting and stimulating topic of study within the field of Computer Science known as the Vertex Cover Problem. This subject area is rich with computational theories and algorithms that are used to solve relevant and often complex real-world problems.What is the Vertex Cover Problem?
The Vertex Cover Problem is a classic question from the world of mathematical graph theory and computer science.In simple terms, it's a problem of identifying a set of vertices (the smallest possible) in a graph where each edge of the graph is incident to at least one vertex from the set.
// An example of Vertex Cover Problem in code Graph *G; VerticeCoverProblem(G) { for each edge e in G: if neither endpoint of e is covered: include one endpoint of e in cover; }
Historical View of Vertex Cover Problem
The Vertex Cover problem has a rich history and is one of the foundational issues in computational theory and algorithmic research.Its roots trace all the way back to the 1970s when it was first defined and used in computational analysis. It is an NP-complete problem, making it part of the famous Karp's 21 NP-Complete problems which were stated in 1972 as a challenge to the field of algorithm and complexity theory.
The Evolution of the Vertex Cover Problem
Over the years, the Vertex Cover Problem has evolved and found its place in various applications. Here's a quick look at its development chronology:- 1970s: Initial definition and recognition as an NP-complete problem.
- 1980s: Introduction of approximation algorithms to provide near-optimal solutions for NP-Complete problems, including the Vertex Cover problem.
- 1990s - 2000s: Integration into genetic algorithms, artificial intelligence and machine learning approaches for problem-solving.
- 2010s - Present: Current research focusing on parallel and distributed computing solutions for the Vertex Cover problem, among other large-scale problem-solving techniques.
Importance of the Vertex Cover Problem in Computer Algorithms
The Vertex Cover Problem is an important concept in the world of computer algorithms. Here are a few reasons why:- It aids in understanding the fundamental characteristics of NP-complete problems.
- Playing a pivotal role in complexity theory, it allows for the study of difficult-to-solve problems.
- It serves as a basis for creating algorithms that deal with real-life problem solving.
Delving Into the Minimum Vertex Cover Problem
The adventure through the fascinating realm of Computer Science continues as we dive deep into the concept of the Minimum Vertex Cover Problem. It is a notable topic that carries a lot of importance in the field of graph theory and computer science.)Defining the Minimum Vertex Cover Problem
The term Minimum Vertex Cover Problem refers to a variant of the Vertex Cover Problem. The objective here, as you might have guessed, is to find the smallest possible vertex cover in a given graph.A Vertex Cover is a set of vertices that includes at least one endpoint of each edge in the graph. In the "Minimum" version of this problem, we're trying to find the vertex cover that involves the fewest vertices.
// Pseudo code of minimum vertex cover problem Graph *G; MinimumVerticeCoverProblem(G) { while there are uncovered edges e in G: select a vertex v from e having maximum degree: include this vertex v in cover; }This problem, like most other NP-complete problems, was standardised and established in the 1970s as a part of Karp's 21 NP-complete problems. It has held a dominant position in the fields of algorithmic research and computational procedures ever since. Let's explore its practical applications in the real world in the next section.
Practical Applications of Minimum Vertex Cover Problem
Make no mistake about it, the Minimum Vertex Cover Problem is not just limited to theoretical discussions and academic debates. It holds extensive real-life applications and implications in a multitude of fields.- In network security, it represents an efficient way to place the minimum number of security forces on nodes to prevent breaches.
- In the telecommunication industry, it guides where to place the least number of antennas for maximum coverage.
- It finds use in operational research for optimal resource utilisation.
- In computational biology, it helps map complex interactions within biological networks.
Field | Applications of Minimum Vertex Cover Problem |
Network Security | Optimal Placement of Security Forces |
Telecommunication | Efficient Antenna Placement |
Operational Research | Optimal Resource Utilisation |
Computational Biology | Mapping Complex Biological Networks |
Illustrating the Minimum Vertex Cover Problem through Real-World Examples
Let's make it real! It's always easier to grasp complex concepts when we can link them to real-life scenarios. For instance, consider a local internet service provider trying to establish a Wi-Fi network in a new housing estate. The houses are scattered and the provider wants to use the least number of towers to cover all houses. In this scenario, houses are the edges and towers are vertices. The provider's dilemma essentially translates to the Minimum Vertex Cover Problem.In some cities, CCTV cameras are installed at various locations to monitor street traffic efficiently. Municipalities want to use the smallest number of cameras while ensuring that every junction is covered. This task resembles the Minimum Vertex Cover problem, where junctions are edges and cameras are vertices.
Exploring the NP Complete Vertex Cover Problem
Broadening your understanding of computer science, let's explore another intriguing domain known as the NP Complete Vertex Cover Problem. As a part of the infamous trio of P, NP, and NP-Complete problems, delving into the nuances of this problem paves the path to deeper comprehension of computational complexity.Deciphering the NP Complete Vertex Cover Problem
The NP Complete Vertex Cover Problem isn't just a mouthful to say, it's a thrilling brain tease in the realm of theoretical computer science and optimisation. It's an excellent playing field to understand the challenges of real-world computational problems and the limitations of current algorithms.In basic terms, NP-Complete problems are those whose solutions can be verified quickly (i.e., in polynomial time), but no efficient solution algorithm is known. The Vertex Cover Problem is classified as NP-Complete because it ticks these criteria.
// Pseudo code illustrating NP Complete Vertex Cover Problem Graph *G; NpCompleteVerticeCoverProblem(G) { for each subset of vertices V' in G: if V' is a vertex cover: return V'; } // Note: Running time is exponential in the number of vertices of G
Link Between Graph Theory and NP Complete Vertex Cover Problem
There's a deep-rooted connection between graph theory and the NP Complete Vertex Cover Problem. This link underpins many practical applications in domains ranging from operations research to bioinformatics. In graph theory, a vertex cover of a graph is a set of vertices that includes at least one endpoint of each edge in the graph. This concept is directly applicable to understanding the Vertex Cover Problem. As the problem is considered NP-Complete, devising an algorithm that can solve the vertex cover problem efficiently for all types of graphs is one of the holy grails of theoretical computer science.How Graph Theory Influences the NP Complete Vertex Cover Problem
Graph theory plays a significant role in shaping and understanding the NP Complete Vertex Cover Problem. For starters, the definition of the Vertex Cover Problem itself is a notion extracted straight from graph theory. Indeed, the vertex cover problem exists s on any graph \( G=(V,E) \), where you're trying to find a subset \( v' \) of vertices \( V \) that touches each edge in \( E \) at least once. The vertices in \( v' \) essentially "cover" all the edges.// Pseudo code illustrating the influence of graph theory Graph *G; GraphTheoryInfluence(G) { for each edge e in G: if neither endpoint of e is covered in v': include it in the vertex cover v'; }At the heart of the problem lies the concept of 'cover', a fundamental notion in graph theory. Therefore, understanding graph theory is akin to holding a key to solving the NP Complete Vertex Cover Problem. Additionally, various algorithms from graph theory, such as the well-known Depth-First Search (DFS) or greedy algorithms, can provide approximation solutions to the NP Complete Vertex Cover Problem. This union of concepts helps shed light on some of the most complex and computationally challenging problems known in computer science.
Analysing the Time Complexity of the Vertex Cover Problem
Grasping the fundamentals of the Vertex Cover Problem is just one step of the journey. It's as crucial to understand its inherent complexity, especially concerning time. When you're running computation-intensive tasks, the time an algorithm takes can make a vast difference. That's where the concept of time complexity in the Vertex Cover Problem comes into the frame.The Role of Time Complexity in the Vertex Cover Problem
The time complexity aspect of the Vertex Cover Problem is fundamental to comprehend the efficiency of algorithms that solve this problem. In computer science, time complexity signifies the computational complexity that describes the amount of computational time taken by an algorithm to run, as a function of the size of the input to the program.The Vertex Cover Problem is a quintessential example of an NP-hard problem. In layman terms, it signifies that the problem belongs to a class of problems which, for large inputs, cannot be solved within an acceptable timeframe using any known algorithm.
Regarding the Vertex Cover Problem, time complexity is outlined by a function corresponding to the number of vertices and edges in the Graph \( G=(V,E) \). Formally, if \( |V| \) is the number of vertices and \( |E| \) is the number of edges, the time complexity for finding a vertex cover is \( O(2^{|V|}) \), making it an exponential function.
This effectively implies that a small increase in the problem size could drastically inflate the time taken to solve the problem.Time Complexity vs Space Complexity in the Vertex Cover Problem
While time complexity is a crucial aspect in understanding the efficiency of an algorithm, space complexity is equally vital. Space Complexity pertains to the total amount of memory space that an algorithm needs to run to completion. For the Vertex Cover Problem, the space complexity is a function of the number of vertices. Formally this can be expressed as \( O(|V|) \), implying it is linear with respect to the graph's vertices.// Pseudo code representing space requirements in Vertex Cover Problem Graph *G; VertexCoverProblemSpaceRequirements(G) { VertexCover[]; for each vertex v in G: include v in VertexCover; } // At its peak, the space need could equal the total vertices in the graph.The complexity conundrum in the domain of computer science is always a trade-off between time and space. Minute improvements in time complexity can sometimes lead to significant increases in space complexity and vice versa. This is a critical principle to master not just for the Vertex Cover Problem, but for all computer science problem-solving.
Ways to Improve Time Complexity of Vertex Cover Problem Algorithm
Yet all is not lost when it comes to the astronomical time complexity in the Vertex Cover Problem. While it's true that the problem is intrinsically complex, there are stratagems and tactics to manage and even improve the time complexity of related algorithms. While the intricacies of these strategies are beyond the immediate scope of this discussion, some of the widely employed methods include heuristics, approximation algorithms, and using memoisation in dynamic programming.One of the most widely used heuristics is choosing a vertex with the maximum degree (number of edges) at each step. This approach alone, however, doesn't guarantee the minimum vertex cover and can sometimes even lead to inferior results.
Deep Dive Into the Vertex Cover Decision Problem and Its Algorithm
If you're interested in the Vertex Cover Problem, you should also familiarise yourself with the Vertex Cover Decision Problem. While it's a variant of the Vertex Cover Problem, it rests on a fully different aspect – it focuses on decision rather than optimisation.Understanding Vertex Cover Decision Problem Within Algorithms
The Vertex Cover Decision Problem is an important concept to grasp when it comes to understanding the foundations of the Vertex Cover Problem. More specifically, it's a formal question within computer science asking whether a graph has a vertex cover of a certain size.Unlike its counterpart, the Vertex Cover Decision Problem simply asks whether a vertex cover of a specified size exists. It doesn't necessarily require the smallest vertex cover; it just needs to confirm if a vertex cover of a particular size is feasible or not.
The Mechanics of Vertex Cover Decision Problem Algorithm
The algorithm developed to handle the Vertex Cover Decision Problem is actually quite intuitive. It efficiently uses recursion coupled with conditional statements to precisely discern whether a particular size of vertex cover is achievable within a given graph. The key is to understand that if a graph has a vertex cover of size \( k \), then it must also have vertex covers of sizes \( k+1, k+2, \ldots, |V| \). Thus, the algorithm essentially needs to create all possible subsets of vertices, and for each subset, check whether the size is less than or equal to \( k \) and whether it covers all the edges.// Pseudo code for Vertex Cover Decision Problem VertexCoverDecisionProblem(Graph G, int k) { for each subset V' of vertices in G: if |V'| <= k and V' covers all edges in G: return 'Yes'; return 'No'; }This process is repeated until all subsets have been examined or a subset matching the criteria is found. While this approach is conceptually straightforward, its execution can be highly time-consuming due to the exponential rise in combinations, especially in larger and denser graphs.
Exploring Vertex Cover Problem Approximation Algorithm
While exact algorithms to solve the Vertex Cover Problem are burdensomely slow for large graphs, approximation algorithms offer a gleam of hope. These cleverly designed algorithms aim to construct an almost optimal solution in significantly less time. Let's begin with a basic 2-Approximation algorithm for the Vertex Cover Problem. This type of algorithm finds a solution in polynomial time whose size is, at most, twice the size of an optimal solution. The idea behind the 2-Approximation algorithm is relatively simple: * Start with an empty cover * Pick an uncovered edge * Add the vertices of the selected edge to the cover * Repeat this process until all edges are covered. The time complexity here is clearly polynomial in the size of the graph. Moreover, the size of the vertex cover selected by this algorithm is as most twice the size of the optimum vertex cover, thereby justifying the tag '2-Approximation'.Practical Implementations of Vertex Cover Problem Approximation Algorithm
You might wonder where these Vertex Cover Problem Approximation Algorithms are used in practice. They pop up in several fields, including network design, bioinformatics, and operations research, amid other areas. In the field of networks, these algorithms help formulate efficient plans for network coverage, focusing predominantly on reducing costs. Bang in the middle of the bioinformatics world, the Vertex Cover Problem ties in with protein-protein interaction networks, aiding the analysis and prediction of protein functions.// Pseudo code for 2-Approximate Vertex Cover Problem 2ApproxVertexCover(Graph G) { VertexCover = empty set; while (G has edges) { Pick any edge (u,v); Add u and v to VertexCover; Remove all edges connected to either u or v; } return VertexCover; } // This approach ensures that the final vertex cover size is at most twice the optimal sizeImplementing a Vertex Cover Problem Approximation Algorithm can save precious computation time. But always bear in mind: these solutions aren't perfect and may result in larger vertex covers than needed.
Vertex Cover Problem Solution Example: A Step-by-Step Guide
Let's walk through an example to illuminate how you'd go about solving the Vertex Cover Problem. Imagine you've been given a simple undirected graph \( G = (V, E) \), with four vertices \( V = \{1, 2, 3, 4\} \) and five edges \( E = \{(1,2), (1,3), (2,4), (2,3), (3,4)\} \).You start by selecting an edge arbitrarily, say \( (1,2) \). Add vertices 1 and 2 to the vertex cover and you remove all edges that connect to either vertex 1 or vertex 2. This leaves you with just one edge - \( (3,4) \). Now, you select this remaining edge, and add vertices 3 and 4 to your vertex cover. With this, all edges are covered, so your vertex cover consists of vertices \( \{1, 2, 3, 4\} \). Although this isn't the smallest vertex cover (which would be vertices \( \{2, 3\} \) or \( \{1, 4\} \)), the approximation algorithm led us to a valid, albeit slightly inflated, solution.
Vertex Cover Problem - Key takeaways
- The Minimum Vertex Cover Problem refers to a variant of the Vertex Cover Problem. The objective is to find the smallest possible vertex cover in a given graph. A Vertex Cover is a set of vertices that includes at least one endpoint of each edge in the graph.
- In practical scenarios, the Minimum Vertex Cover Problem has extensive real-life applications in fields such as network security, the telecommunications industry, operational research, and computational biology.
- The NP Complete Vertex Cover Problem belongs to P, NP, and NP-Complete problems in theoretical computer science and optimisation. These problems' solutions can be verified quickly, but no efficient solution algorithm is known.
- Regarding the Vertex Cover Problem, the time complexity is outlined by a function corresponding to the number of vertices and edges in the graph. If \( |V| \) is the number of vertices and \( |E| \) is the number of edges, the time complexity for finding a vertex cover is \( O(2^{|V|}) \), making it an exponential function.
- The Vertex Cover Decision Problem is a formal question within computer science asking whether a graph has a vertex cover of a certain size. The problem is a decision problem, so the output is always binary – it can either be 'Yes' or 'No'.
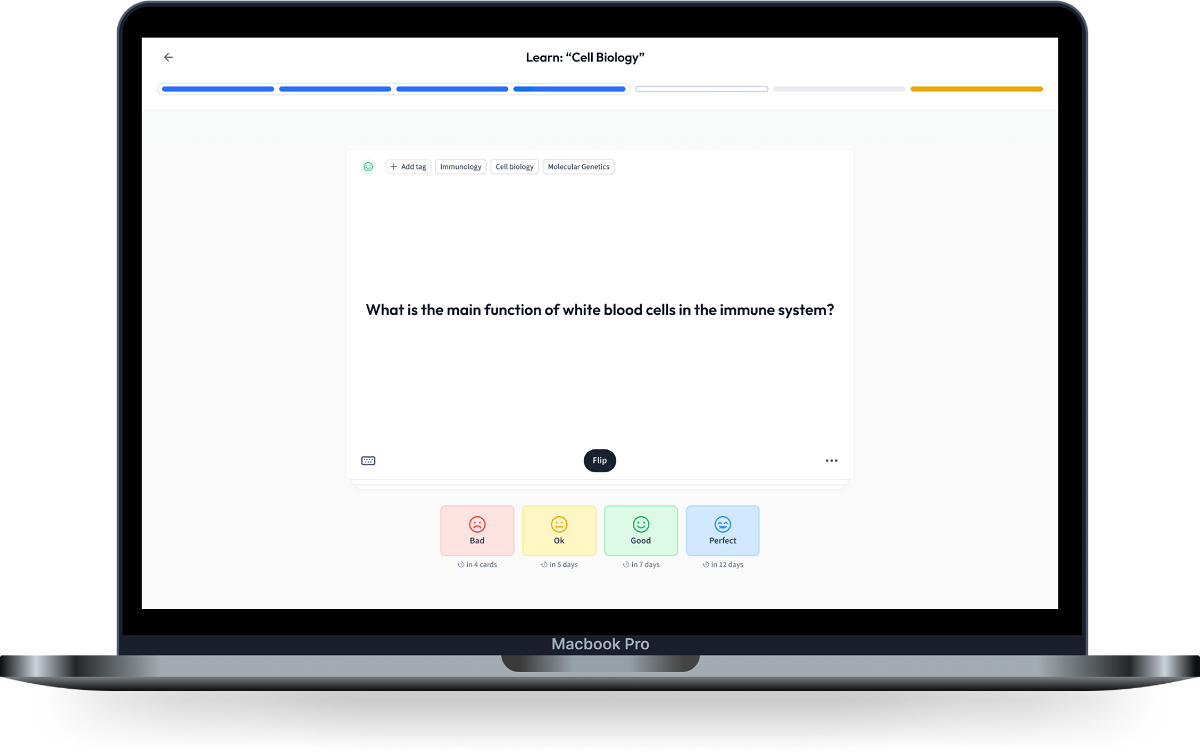
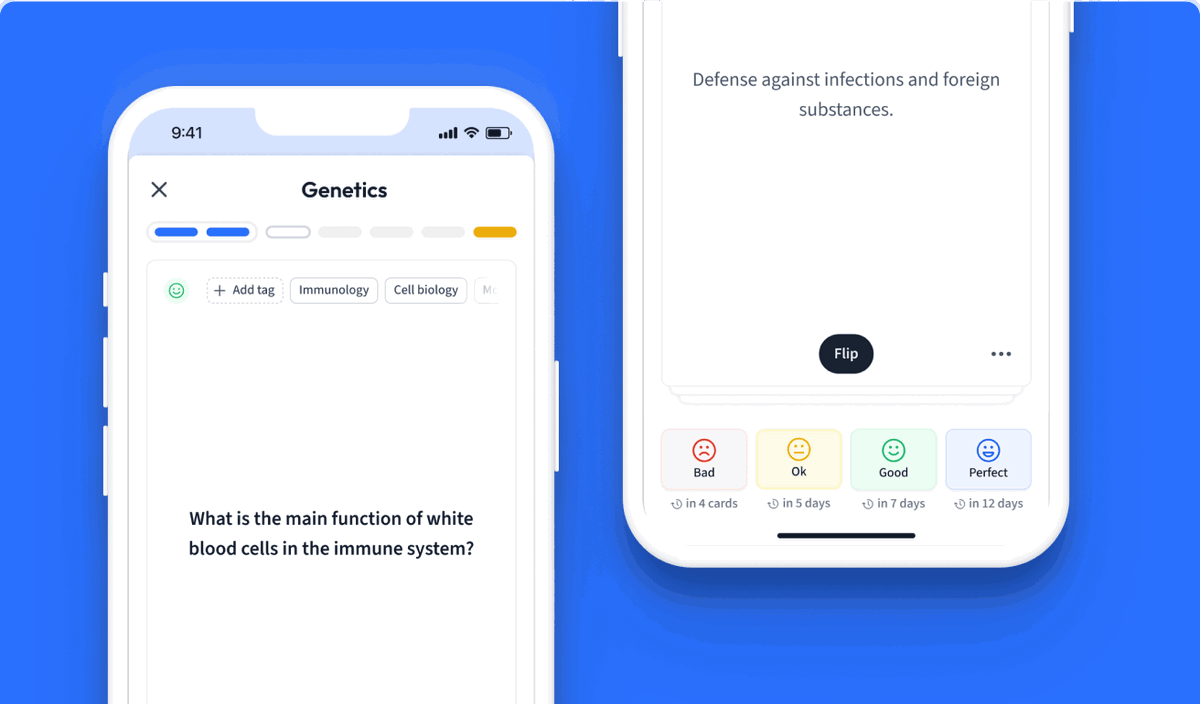
Learn with 15 Vertex Cover Problem flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Vertex Cover Problem
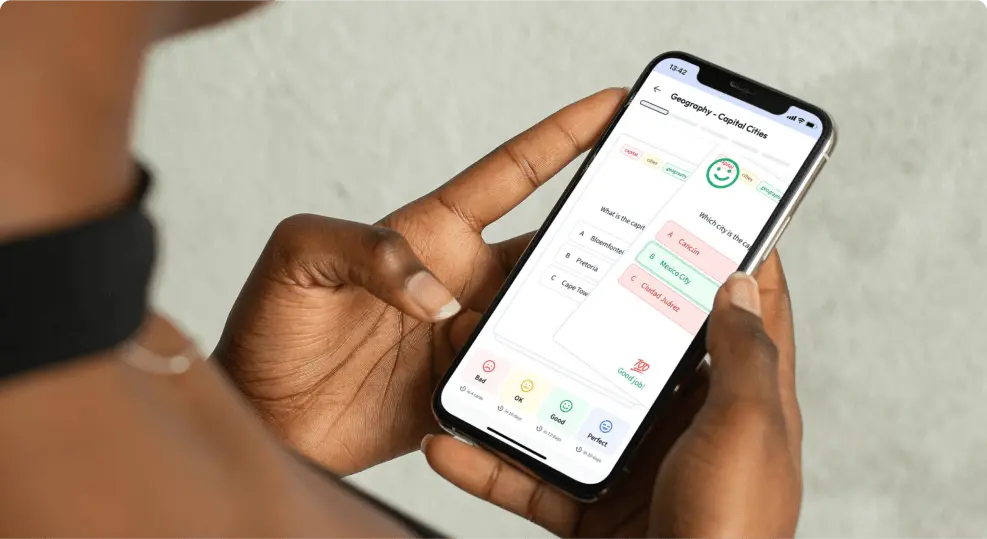
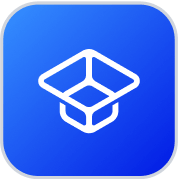
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more