Understanding Tree Data Structure
Unravelling the world of Computer Science, you might come across the concept of Tree Data Structure. This structure is significant in storing data in hierarchical form and improving the speed of operations across multiple programming languages.Definition and Importance of Tree Data Structure
A Tree Data Structure is an abstract model of a hierarchical structure. This structure is composed of nodes, where each node represents a value or condition, and the links between nodes represent the relationship between the values.In a Tree Data Structure, the topmost node is called the root, and the elements that branch from the root are known as children. Nodes with the same parent are called siblings.
A common application is in the domain of file systems. Every file storage system has a hierarchical structure with folders (directories), sub-folders, and files, easily represented as a tree data structure.
Essential Concepts related to Tree Data Structure
- Root: The top node in a tree. - Parent: The converse notion of a child. - Siblings: Nodes with the same parent. - Leaf: A node with no children. Candidates for a tree structure should satisfy these properties: - Each node has a unique identity (value). - Each node has a specific number of children, including the possibility of zero (for leaf nodes).Operating Tree Data Structure in Python
Python, like many programming languages, has no built-in data type for trees. However, it's simple to create a class representing nodes in a tree. A simple Python node class may look like this:\begin{verbatim} class Node: def __init__(self, value): self.left = None self.right = None self.value = value \end{verbatim}
To create and connect nodes, instantiate the Node class for different nodes (like root, left, and right), and link them by allocating child nodes to the parent nodes.
Practical Examples of Trees in Data Structure Python
Let’s create a simple tree with four nodes in Python:\begin{verbatim} class Node: def __init__(self, value): self.left = None self.right = None self.value = value # Allocate nodes root = Node(1) left = Node(2) right = Node(3) leaf = Node(4) # Setup children root.left = left root.right = right left.right = leaf \end{verbatim}
In this Python example, root is the parent of left and right nodes. The left node in turn is the parent of the leaf node.
Different Types of Trees in Data Structure
In the realm of the Tree Data Structure in Computer Science, you will meet different types of trees, each with its unique set of properties and use cases. Let's discuss these types to understand their structure and functionality better.Description of Various Tree Types in Data Structure
There are several types of tree data structures used in computer science to address specific problems. Each tree type possesses distinct features primarily centred on making specific operations more efficient. Here are some of the most common ones: 1. General Trees: These are ordinary trees with no specific rules about node connections, thus allowing more freedom and flexibility. They may have any number of children. 2. Binary Trees: All nodes in a Binary Tree have at most two children. This condition directs that each child is either a 'left' or a 'right' child, which makes binary trees important in search operations. 3. Binary Search Trees (BSTs): BSTs are a type of Binary Tree where each node's value is greater than or equal to its left child and less than or equal to its right child. This makes BST a powerful tool for performing search operations. 4. AVL Trees: Named after their inventors (Adel'son-Vel'skii and Landis), AVL trees are Binary Search Trees that self-balance. They ensure that the difference (the Balance Factor) between the heights of the left and right subtrees of any node in the tree is less than or equal to one. 5. Red-Black Trees: These are self-balancing Binary Search Trees where each node carries an extra bit for denoting the colour of the node, either red or black. Balancing of the tree is accomplished by painting the nodes in a way that follows certain properties, enhancing search operations within the tree.Self-balancing trees such as AVL and Red-Black Trees dynamically maintain their height to provide optimal performance for lookup, insert, and delete operations.
File systems and databases heavily use these self-balancing trees for data retrieval.
Comparing Features of Various Tree Types
Let's compare these tree types in terms of their features and uses: Source: Created using HTML.Tree Type | Special Feature | Use Case |
---|---|---|
General Tree | Unrestricted | Data that naturally forms a hierarchy (e.g., organizational structure) |
Binary Tree | Max of two children | Used in many search applications where data is constantly entering and leaving |
Binary Search Tree | Ordered Nodes | Searching for specific items in the tree |
AVL Tree | Self-Balancing | Useful in maintaining a sorted list |
Red-Black Tree | Self-Balancing, Coloured Nodes | Widely used in many open-source libraries for efficient searching, insertion, and removal |
B-Tree | Multi-Level, Wide Trees | Used in disk-based storage systems due to their efficient searching and insertion properties |
Application of Trees in Data Structure
In Computer Science, the widespread applications of tree data structures underscore their underlying utility and importance. From handling hierarchical data and facilitating easier and faster access to rapid data modifications, the trees provide versatile solutions in numerous domains.General Applications of Tree Data Structure
In data manipulations, queues and stacks might serve well, but when it surfaces to arranging and storing data in a natural, hierarchical way, tree data structures rise to the occasion. Frequently, the complications centring on database algorithms and graphic postulations can conveniently be clarified and solved using tree data structures. Here are some of the general uses of trees in data structures: - Storing naturally hierarchical data: Think of file systems on computers: Every drive is divided into folders, subfolders, and files. Thus they form a tree data structure. - Facilitating efficient searching: AVL trees and Red-Black trees are excellent for searching for elements in a tree as they maintain the tree's height to a minimum, ensuring that the time complexity of searching is logarithmic. - Manipulating sorted lists of data: Binary Search Trees (BSTs) provide moderate access/search times (quicker than Linked Lists and slower than arrays). They allow quick insertion and deletion of data. - Organising multi-stage decision-making: For instance, a Chess game AI uses a tree to consider all the possible moves and outcomes, making decision-making a breeze. - Providing a manageable way to implement Graph algorithms in a simpler way: For instance, trees can be used to serve algorithms for finding the minimum spanning tree of a graph. - Shaping networks: Trees in data structures are used to represent networks or graphs.Specific Case Studies featuring the Application of Trees in Data Structure
Let's examine two detailed practical scenarios illustrating the utilisation of tree data structures.Case Study 1: Use of BST in Databases Databases utilise Binary Search Trees (BSTs) for their operations. A database is a large amount of organised data. When a user queries the database for information, it is essential to minimise the search time. By structuring the database entries in BSTs form, the entries are sorted, allowing binary search. Given a BST where each node contains \(k\) keys and points to \(k+1\) children, the time complexity for search operations is \(O(\log n)\), making the retrieval of information swift and efficient. Case Study 2: AVL Trees in File System Hierarchy A file system on a computer is a typical example of a tree data structure. Whenever you save a file in a specific directory, you need to define both the path and the file name. The operating system then uses an AVL tree to store the folders and files in the memory. The operating system applies a unique algorithm, ensuring no two files or folders have the same path. The AVL tree properties come into the picture as the file system undergoes many read and write operations every second, and hence, balancing the tree ensures that searching a file's path remains efficient with a time complexity of \(O(\log n)\).
Advantages of Trees in Data Structure
In Computer Science, the benefits of tree data structures are plentiful. These structures serve as the pillars for organising data into a manageably structured and swiftly searchable manner. Their use is pivotal in enhancing many computational processes and algorithms, from boosting search times in databases to optimising decision-making in Artificial Intelligence.Overall Benefits of Using Trees in Data Structure
Among the data structures, trees stand unique due to many exciting advantages they offer. The inherent hierarchical nature of trees makes them naturally suitable for several applications: 1. Hierarchical Data Storage: Trees can store data in a structured hierarchical format. This becomes handy in situations where the data itself symbolises a natural hierarchy, for instance, storing file systems on a computer drive. 2. Efficient Organisation of Data: Binary Search Trees (BSTs) organise the data in such a way that the lesser values are on the left side and the larger values on the right side. Such a feature enables the performance of moderate access/search (quicker than Linked Lists and slower than arrays), quick insertion and deletion of data. 3. Optimised Search Times: AVL trees and Red-Black Trees maintain the tree's height to a minimum to ensure logarithmic time complexity for search, insert and delete operations. This attribute enables efficient searching of an ordered list of data. 4. Facilitation of Decision-making: Trees are particularly vital in the multi-stage decision-making process. A legitimate example would be a Chess game AI that uses a tree to allude possible moves and outcomes. 5. Implementation of Graph Algorithms: Tree data structures can implement many non-linear data structures and graph algorithms like Depth First Search (DFS) and Breadth First Search (BFS) in a simplified manner. 6. Network or Graph Representation: Another spot-on application of trees in Computer Science is the presentation of networks or graphs. From the above advantages, it is vivid that the tree data structures, characterised by their natural hierarchical styling, can significantly boost search and insert times, provide easier manipulations, and utilise space in an extremely effective manner.Case Scenarios Showcasing Advantages of Trees in Data Structure
To fathom these benefits, let us delve into practical scenarios that illustrate how tree data structures are embedded into our digital lives:Case Study 1: Makefile Dependency Generation using Trees In Software Engineering, it's common to have projects with multiple files. The build process often requires that certain files are compiled before others. This is because some files (parent nodes) rely on the output of other files (child nodes). Hence, trees in data structures become crucial in generating dependencies. A tree can clearly define this dependency or the sequence in which project files should be compiled, ensuring the correct and efficient build process. Case Study 2: Document Object Model (DOM) in Web Development In web development, web pages are structured as a tree of objects known as the Document Object Model (DOM). This model allows developers to manipulate web content using languages like JavaScript. The hierarchical tree structure becomes essential as it allows developers to traverse the elements on the web page and make necessary changes using specific paths. Case Study 3: Game Decision Trees In the gaming industry, game development AI often utilises trees for decision-making processes. A tree data structure represents a game's decision tree, where each node corresponds to a game state, and each edge corresponds to a game decision. This method helps derive the optimum decision leading to a win. Case Study 4: Compiler Design In Compiler design and construction, trees are useful in syntax analysis. An abstract syntax tree represents the syntactic structure of the source code according to the language's grammar rules, thereby helping the compiler understand the code structure and convert it into machine language.
Abstract Trees in Data Structure
An abstract tree in data structure signifies an extended tree model tailored to serve particular needs. Abstract trees are instrumental in simplifying many real-world problems by providing a much-needed abstract logic for creating problem-solving algorithms.Understanding Abstract Trees in Data Structure
An abstract tree is a conceptual representation of a tree data structure. Unlike concrete tree data structures like AVL trees or Red-Black trees, abstract trees primarily define the core functionality and the methods required to interact with tree structures without being tied to specific configurations or internal workings. Abstract trees usually denote conceptual models from which concrete trees inherit fundamental properties.In essence, an abstract tree is a minimalistic and high-level view of a tree data structure, focusing on the operations performed on the tree structure and the relationships between nodes, rather than the detailed implementation.
- Add a new node
- Delete a node
- Search for a node
- Traverse the tree
Importance and Role of Abstract Trees in Data Structure
Abstract trees play a crucial role in Computer Science by providing an abstract view of the tree data structures, making them easier to understand, implement, and manipulate. The benefits of using abstract trees extend to a multitude of areas: - Optimising Problem-Solving: Abstract trees help software engineers think logically about problems and derive efficient solutions. For instance, decision trees are abstract trees used in Artificial Intelligence and Machine Learning to help AI make rational decisions based on various factors. - Simplifying Complex Structures: Abstract trees can help simplify complex structures, making them easier to understand and analyse. For instance, Abstract Syntax Trees (AST) simplify the structure of a program, making it easier for compilers to read and translate the code. - Boosting Development: By providing an abstract view of tree data structures, abstract trees can speed up development time. The developer only needs to understand the abstract methods and their interactions provided by the tree without concerning the implementation details. In conclusion, abstract trees aid in perceiving tree data structures from a higher logical viewpoint, providing a blueprint for building specific tree structures. Understanding abstract trees equips you with the ability to perceive the tree data structure's underlying logic, aiding in devising algorithms more efficiently and enhancing your problem-solving skills in programming.Case Study: Abstract Syntax Trees (ASTs) in Compiler Design Let's consider the example of a compiler, a central tool in software development. Its job is to translate source code written by developers into machine code. To accomplish this task efficiently, it employs an abstract tree structure known as an Abstract Syntax Tree (AST). When the compiler reads the source code, it constructs an AST. Every sentence or expression in the source code translates into a node on the AST. The node's children represent the components of the sentence or expression. This abstract tree helps the compiler understand the syntax and structure of the source code without getting attached to the source code specifics. It enables the compiler to focus on the logical structure and the code's semantics. The AST thus provides a roadmap for the compiler to follow as it translates the source code to machine code, demonstrating how abstract trees serve as critical tools in Computer Science.
Tree data structure - Key takeaways
- A tree data structure is an abstract model of a hierarchical structure, composed of nodes which represent values or conditions and links representing the relationship between values.
- In a tree data structure, the topmost node is called the root, and nodes that branch from the root are known as children. Nodes with the same parent are siblings.
- Trees can significantly improve the speed of operations across multiple programming languages, and have a wide range of uses, including in artificial intelligence and big data.
- The different types of trees include general trees, binary trees, binary search trees, AVL trees, Red-Black trees, and B-trees, each suited to addressing specific problems.
- Tree data structures are extensively used for tasks such as storing hierarchical data, facilitating efficient searching, manipulating sorted lists of data, organising multistage decision-making, implementing graph algorithms, and more.
- Trees provide advantages such as efficient organisation of data, optimised search times, facilitation of decision-making, implementation of graph algorithms, and representation of networks or graphs.
- An abstract tree in data structure is a conceptual representation of a tree data structure, defining core functions and methods required to interact with tree structures without being tied to specific configurations or internal workings.
- Abstract trees, such as Abstract Syntax Trees used in compiler design, aid software engineers in logically solving problems, simplify complex structures, and speed up development time by providing a higher level viewpoint of tree structures.
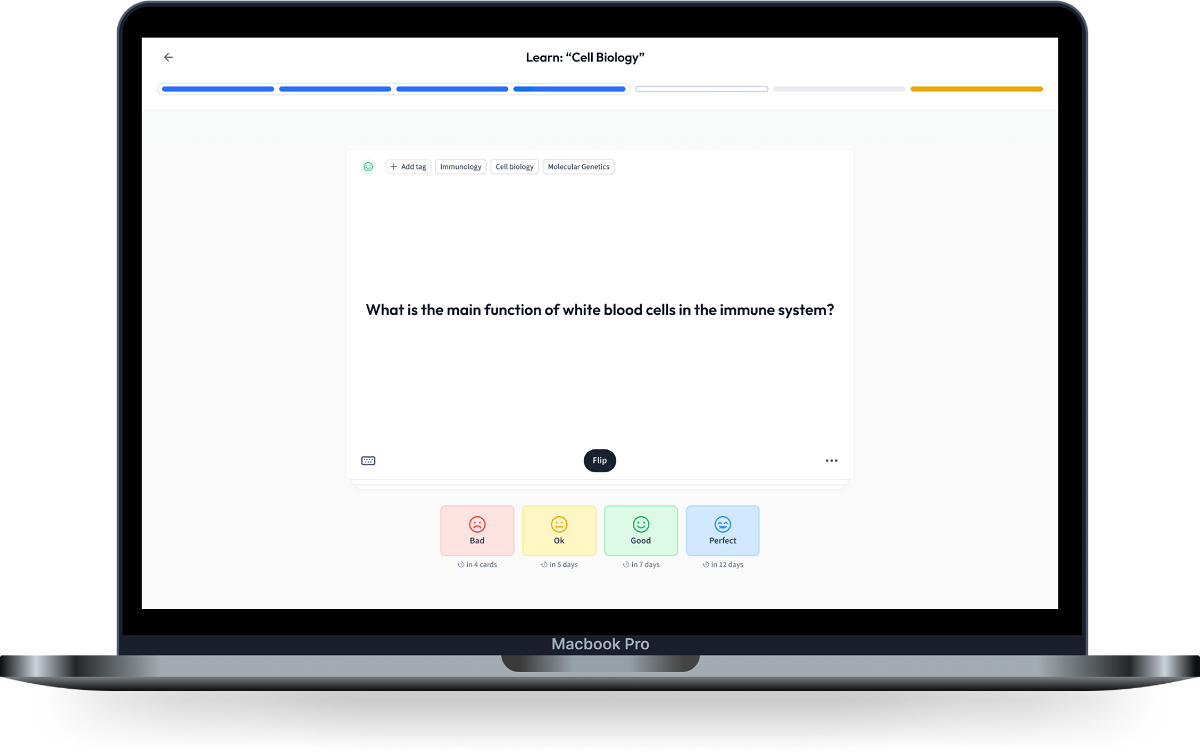
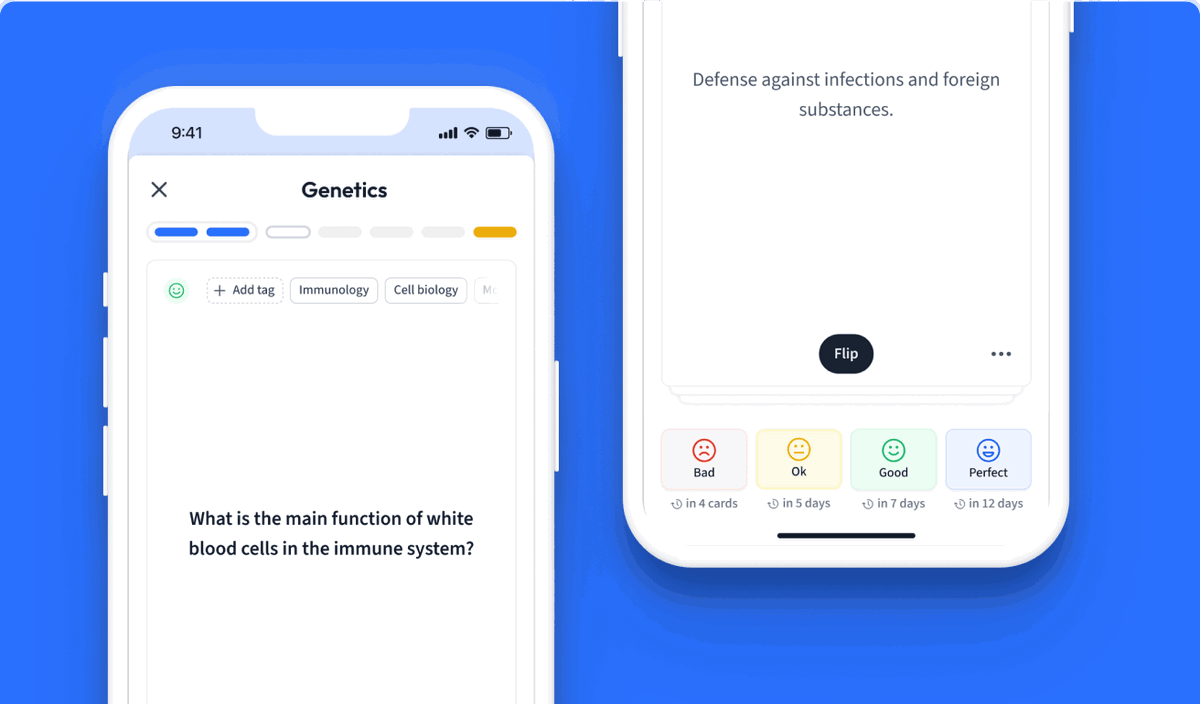
Learn with 22 Tree data structure flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Tree data structure
How many types of trees in data structures?
There are several types of trees in data structure, including Binary Tree, AVL Tree, Binary Search Tree, Red-Black Tree, 2-3 Tree, 2-3-4 Tree, N-ary Tree, B Tree, B+ Tree, Spanning Tree, Heap Tree, and Trie.
What are trees in data structure?
In data structure, a tree is a hierarchically organized set of values or 'nodes', where each node is linked to a superior node (parent) and potentially to several inferior nodes (children). The topmost node is known as the root, while the nodes at the bottom (which have no children) are called leaves. This structure lends itself well to efficiently organizing, searching, modifying, and managing sorted lists of data. Trees are used in areas such as databases, file systems and AI algorithms.
What is an example of a tree data structure?
An example of a tree data structure is the hierarchical file system in an operating system, where directories are parents and files and subdirectories are children. Another example is the Document Object Model (DOM) used in HTML, where each tag is a node and embedded tags are child nodes. These structures allow for efficient searching and modifying of elements within the system or document. Additionally, HTML parsing, document representation and the organisational structure of a corporation also utilise tree data structures.
When to use tree data structure?
Tree data structures are used when data is hierarchical in nature and needs to be organised in a manageable, searchable manner. They are particularly useful for tasks such as managing hierarchical relationships, implementing efficient search algorithms, organising data for quick insertion, deletion and searching, and creating decision trees in machine learning algorithms. For instance, the Document Object Model (DOM) of HTML pages in web development utilises a tree data structure. Tree structures are also frequently used in file and directory systems.
What is a node in tree data structure?
A node in a tree data structure is an individual element or piece of data. Each node typically holds its own value and references to other nodes in the tree. These references are known as pointers, which link parent nodes to their child nodes, thereby creating a hierarchy. This hierarchy is what defines the tree structure.
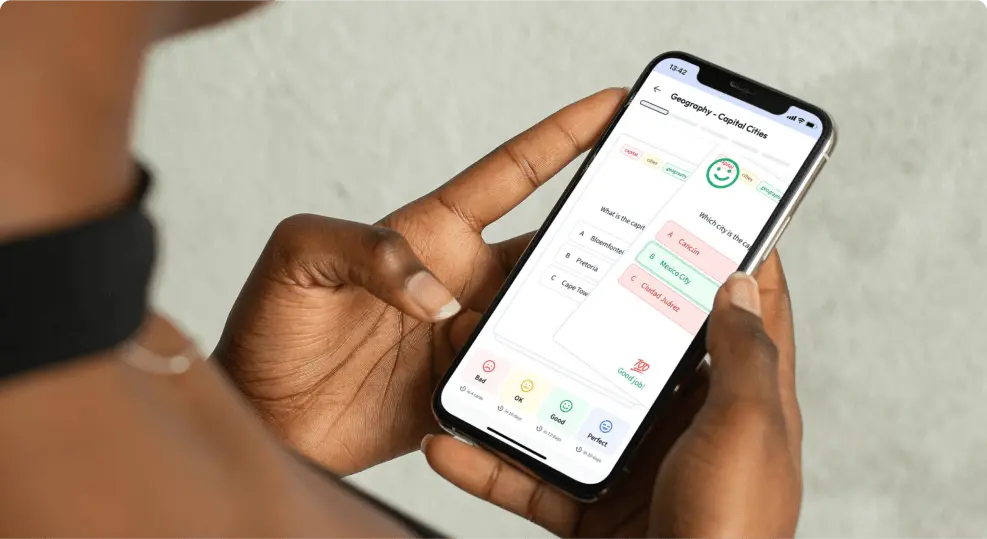
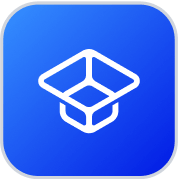
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more