Understanding Hash Tables in Computer Science
Unveiling the realm of computer science, you'll be delving into various key data structures. One such is the Hash Table, an underlying concept in most efficient computer algorithms.Basic Principles of Hash Tables
Hash Tables are data structures that store items in an associative manner. In a hash table, data is organised to allow for extremely quick insertion and lookup. This standout feature sets Hash Tables apart from other types of data structures.A Hash Table is a data structure that implements an associative array abstract data type, a structure which can map keys to values.
Understanding the role of hash tables
Hash Tables play a significant role in developing efficient algorithms. As you continue to engage with computer science, consider the two primary use-cases for hash tables: data retrieval and data insertion.- Data Retrieval: Hash Tables are known for their phenomenal speed in data retrieval. Given the key, the value can be retrieved in O(1) time complexity.
- Data Insertion: Similar to data retrieval, data insertion in a Hash Table is also O(1). This is because it directly places the data at the address calculated by the hashing function.
Key components of a hash table
A hash table consists of several major components:- Key: The unique identifier with which data is associated.
- Value: The data which is stored and retrieved.
- Hash Function: A function that computes an index into an array of slots, from which the desired value can be fetched.
- Collisions: When two keys hash to the same index.
- Load factor: A measure that decides when to resize the hashtable.
Exploring Array Hash Tables
Diving further, you'll find the most traditional form of hash tables - array hash tables. These can be understood as a simplistic yet efficient version of hash tables.Array Hash Tables are a type of hash table where the underlying data structure is an array. It utilises the concept of direct-address tables which is ideal when the amount of data is small.
Array hash tables explained
Array Hash tables are primarily used when the maximum size of the table is already known. Here, the key is used directly as an index to access its associated value. Underneath is a simple representation of how an array hash table looks:Index | Value |
---|---|
0 | Value1 |
1 | Value2 |
2 | Value3 |
How to utilise array hash tables effectively
Utilising Array Hash Tables effectively requires careful consideration of the hash function and the handling of collisions.Consider a situation where you are tasked to store employee records in a Hash Table. The table 'key' can be an employee's unique ID number, and the associated 'value' could be the employee's details. The hash function can be as straightforward as 'key modulo size of table', which ensures that the hash always falls within the array bound. To handle collisions, you may implement chaining, which means that instead of storing a single value at every location of the Hash Table, you can maintain a list of records that hash to the same location.
Understanding the nuances of hash tables, their role, components, types, and effective usage can greatly boost your ability to write efficient code. Hash tables, with their unique ability to access data in constant time, help in designing fast algorithms, especially in the fields of databases, caching, and set operations.
The Execution of Hash Table Implementation in Python
While hash tables are a fundamental concept in computer science, their application in Python is particularly streamlined. Let's explore how this construction unfolds.Steps for Hash Table Implementation
Python provides a very efficient way of implementing hash tables where it uses the built-in dictionary data structure. The dictionary in Python uses a hashing algorithm to provide key, value pairs stored for fast access.Setting up the environment
To start implementing hash tables in Python, you will require a Python environment. This is done by installing Python and an Integrated Development Environment (IDE) of your choice. One recommended IDE is PyCharm, as it features numerous tools for code analysis, graphic debugging and unit testing that assist in holistic Python development. Once acquired with the basics, begin with the implementation of hash tables. Here are the steps:- Install Python from official Python website
- Download and Install an IDE like PyCharm
- Create a new Python project/file
Implementation process details
To implement a hash table, instantiate a Python dictionary using the built-in data type dict. Simply declare a variable as a dictionary and you can start using this as a hash table: employee_record = dict()Next, you can add key-value pairs to the dictionary which acts as inserting into the hash table: employee_record['emp_id'] = 'E101' employee_record['name'] = 'John Doe' For data retrieval from a hash table, you can use the keys: print(employee_record['name']) This would print the employee name associated with the key 'name' from the hash table. Because it's a dictionary, the lookup time is in constant time, O(1), which highlights the advantage of a hash table. Deletion of a key-value pair is also straightforward: `del employee_record['emp_id'] Lastly, checking for a key in the hash table can be done using the 'in' keyword: 'emp_id' in employee_recordRemember, Python's dictionary takes care of collisions and hashing function implementation so you don't have to worry about it when implementing a simple hash table in a Python environment.The ease of hash tables implementation in Python, using dictionaries, is a testament to Python's status as a powerful and user-friendly language. Behind the scenes, the built-in hash table functionality involves complex algorithms optimised for efficiency, but as a developer, you're able to enjoy the benefits without the nitty-gritty.
Debugging in Python: Hash Table related issues
Even though Python simplifies the use of hash tables, you may still encounter issues. Most of these problems stem from a misunderstanding of how Python dictionaries work. Here are some common issues and their respective workarounds.Handling non-existent keys
A typical error you may encounter occurs when accessing a key that doesn't exist in the dictionary. This raises a KeyError:print(employee_record['phone'])If 'phone' isn't a key in the dictionary, Python raises a KeyError. To prevent this, use the `get()` method, which returns None if the key doesn't exist, or a default value that you can set:
print(employee_record.get('phone', 'Default'))
Immutable Keys and Hashability
It's essential to remember that dictionary keys must be immutable and hashable. You can still use mutable and non-hashable data types as values, but not as keys. For instance, lists can't be used as keys since they are mutable. You'll encounter a TypeError if you try to use a list as a key in a dictionary.employee_record[['first_name','last_name']] = 'John Doe'To circumvent this issue, convert the list to a tuple, which is immutable, then use it as a key:
employee_record[('first_name','last_name')] = 'John Doe'
Consider an example where you might want to store phone numbers for each employee. Since an employee can have multiple phone numbers, you'd use a list as a value in the dictionary. This is perfectly acceptable and demonstrates how you can store mutable data types as values in a hash table.
Through careful understanding and appropriate debugging, you can avoid such pitfalls and make full use of Python's hash table implementation.
Mastering Hash Tables in C#
Venturing into the sphere of C#, hash tables emerge as an indomitable utility for efficient data storage. Let's discover the intricacies of employing hash tables in a C# environment.C# and Hash Table Basics
C#, a general-purpose, modern and object-oriented programming language, indeed offers native support for hash tables. The .NET Framework's System. Collections namespace includes a class named Hashtable that you can use to create and manage hash table based data structures.Hashtable in C# is a non-generic collection of key/value pairs. It is widely used because of its functionality and flexibility. Hashtable class provides a way to access objects based on keys, and keys are hashed to generate a unique code for storing items into the Hashtable.
The link between C# and hash tables
In C#, hash tables are a thread-friendly, efficient collection for storing key-value pairs. Hash tables set themselves apart from arrays or lists because they can, under optimal conditions, access any given entry in constant time, denoted as \(O(1)\). Here's how a hash table works in C#- The hash table uses a 'hash function' to compute an index into an array where the value will be stored.
- Given a key, you can find its value by feeding it to the hash function and visiting the location it returns.
- Modulo operation is commonly used as a hash function because it distributes entries evenly among an array.
Setting up hash tables in C#
A hash table in C# can be set-up using the Hashtable class. Firstly, you need to include the System.Collections namespace using the 'using' directive at the top of the codes:csharp using System.Collections;To create a new hashtable object:
csharp Hashtable hashtable = new Hashtable();To add an item into the hash table, use the Add() method: csharp hashtable.Add(1, "One"); hashtable.Add(2, "Two"); Suppose you want to retrieve the element of a specific key, use an indexer for this:
csharp string item = (string) hashtable[1];To remove an item from the hash table, use the Remove() method:
csharp hashtable.Remove(1);This way, you can easily perform CRUD operations with the hash table in C#.
Common challenges when using hash tables in C#
While HashTables in C# are incredibly versatile and efficient, certain challenges may come alongside. It's a part of your journey to master these aspects and navigate smoothly with hash tables.Handle Collisions
In an ideal scenario, the hash function will assign each key to a unique slot. However, there is a chance that the hash function will produce the same hash code for different keys, which leads to a collision.Collision in hashing is a scenario that occurs when the hashing function maps two distinct keys to the same hash value.
Dealing with null key or value
It’s important to note that hash tables in C#, as implemented by the Hashtable class, don’t allow null values or keys. Attempting to add or retrieve a null key or value will result in a ArgumentNullException. To avoid this exception, before performing any add or retrieval operation, always use a if clause to check whether the key or value is null. For example:csharp if (key != null) { hashtable.Add(key, "Value"); }
Imagine you're creating a hashtable to store student grades. The 'key' can be a student's unique ID and the 'value' can be the student's grade. By checking the non-nullness of both the student's ID and grade, one can ensure that ArgumentNullException is not thrown and invalid data is not added to the Hashtable.
Delving into Distributed Hash Tables
Venturing beyond traditional hash tables, now you will find yourself exploring the realm of distributed hash tables (DHTs), a pillar of distributed systems. Unlike regular hash tables, DHTs store key-value pairs in a decentralised manner across numerous nodes in a network, maximising robustness and efficiency even as the system scales.Principles of Distributed Hash Tables
DHTs are essentially key-value stores that operate over a cluster of machines, maintaining high performance and reliability even in the face of node arrivals and departures. Through clever use of hashing, DHTs distribute data uniformly across the nodes, thus mitigating data hotspots, providing load balancing and ensuring efficient data lookup.A distributed hash table (DHT) is a decentralised distributed system that partitions ownership of a set of keys among participating nodes, and allows any node to efficiently retrieve the value associated with any given key.
Definitions and practical applications
Key elements within a DHT include:- Nodes: Each participant in the DHT network.
- Key-Value Pairs: Data is stored in the form of key-value pairs, with the key acting as the unique identifier.
- Hash Function: A function that maps keys to nodes in the network.
Advantages and challenges of DHT
DHTs come with numerous advantages, including:- Scalability: DHTs handle large volumes of data and heavy traffic by distributing the load across several nodes in the network.
- Fault Tolerance: Even if nodes fail or depart from the network, the DHT can continue functioning without data loss.
- Efficient Lookup: DHTs provide an efficient mechanism for data lookup, with a time complexity of \(O(\log N)\).
Real-world Use Cases of Distributed Hash Tables
DHTs have found wide use in various fields, owing to their inherent efficiency, scalability and decentralisation.DHTs in Peer-to-Peer Networks
DHTs form the backbone of many large-scale peer-to-peer (P2P) systems, such as BitTorrent. Here, the peers form nodes in a DHT, which aids in resource location in the network. A file, divided into several fragments, is stored across various nodes. When a peer requests a file, the DHT provides the address of the nodes that contain the different fragments, enabling high-speed, parallel downloads.DHTs in Distributed File Systems
DHTs play a crucial role in large-scale distributed file systems like Google’s GFS and Apache's Hadoop DFS. These are key-value systems where keys are file names and values are file data. DHTs help in maintaining metadata about the file chunks across multiple servers, aiding in efficient file location and retrieval.Imagine a distributed file system manages petabytes of digital media. When a user needs a specific media file, the system's DHT locates the file across thousands of servers in an instant, fetching the file ready for the user to download. This streamlined process showcases the DHT's power in practical applications.
DHTs in Blockchain
In blockchain technology, nodes collaboratively maintain a continually growing list of records, or blocks, linked via cryptography. DHTs serve as efficient node-to-node communication systems in decentralized networks such as blockchain, contributing to the quick retrieval of transaction records. As you navigate the diverse domain of computer science, understanding DHTs equips you to comprehend and implement a variety of cutting-edge distributed systems. Their innate qualities of being decentralized, scalable, and fault-tolerant make them applicable and desirable in designing robust systems for handling substantial data.The Role and Applications of Hash Tables
Hash tables are a cornerstone of computer science, acting as a foundation for various data structures and algorithms used across numerous applications. A comprehensible understanding of the roles and applications of hash tables can deepen your insights and enhance your command over computer science.Why Hash Tables are Indispensable to Computer Science
Hash tables, the unsung heroes of computer science, contribute extensively to the discipline's logic and functionality. They work behind the scenes, powering up diverse functionalities and enhancing the ability to write efficient, optimised code.
Importance and Benefits of Hash Tables
The essence of hash tables lies in their decisive structure that strengthens computer science operations. They are especially significant for the below reasons:- Efficient Lookups: Hash tables allow for rapid data lookups, proving advantageous when dealing with large datasets. This efficiency, denoted by the time complexity \(O(1)\), outpaces other data structures that might seem efficient but don't match the swift retrieval of hash tables.
- Value-Intensive Operations: When operations revolve around values more than the sequence of data, hash tables come into the picture. It facilitates quick value-based retrieval, adding a significant asset to your computational efficiency.
- Flexible Keys: In hash tables, keys are not restricted to integer values. They can encompass strings or other data types, providing much-needed flexibility in variable-rich applications.
- Effective Duplicates Handling: Hash tables not just negate duplicates but optimise such operations to ensure clean, unique datasets for accurate processing.
Prominent Uses of Hash Tables
Capitalising on their hashing function and O(1)-efficiency, hash tables have cemented their spot in a plenitude of applications:- Databases:Databases employ hash tables for indexing, allowing data to be retrieved quickly.
- Compilers: Hash tables store identifiers in compilers, aiding in quicker access and processing of instructions.
- Cache Memory: Hardware implementations use hash tables to organise cache memory and expedite lookup time.
- Networking: Hash tables enable efficient routing information storage in the implementation of routing protocols.
- File Systems: In operating systems, hash tables assist in file retrieval by storing file paths and references.
Advanced Applications of Hash Tables
Pushing boundaries, hash tables have been instrumentalising novel, forward-facing applications within the computer science realm. They are underpinning future technologies, offering optimal solutions for complex problems that arise with advancements in data and computing capabilities.Cryptographic Algorithms
One breakthrough realm of hash tables is cryptography, where they play a vital role in ensuring data security and integrity. Empowered with their hash functions, cryptographic algorithms utilise hash tables to transform data into cryptic versions, enabling secure transactions across networks. For instance, blockchain technology thrives on hash functions. Here, hash tables facilitate 'chaining' of blocks, where each block contains a hash of the previous block, thereby delivering robust security.Distributed Systems
Another groundbreaking area where hash tables make their mark is in distributed systems. Using variations known as Distributed Hash Tables (DHTs), systems efficiently tackle issues like load balancing and data retrieval across a network of systems. A classic example is BitTorrent, a peer-to-peer protocol for sharing files. BitTorrent utilises DHTs to track multiple files across numerous systems in the network. In tandem with DHTs, BitTorrent expertly manages data division, storage, and retrieval, making large file sharing a breeze.Machine Learning Algorithms
Hash tables are pivoting a significant shift in machine learning too. In the form of hashing algorithms, they compress high dimensional data into lower dimensions, streamlining complex computations. Known as the hashing trick, this technique aids in memory-efficient storage and speedy processing of high-volume datasets, propelling advancements in machine learning and data science. By comprehending the vast repertoire of hash table applications, you map your step deeper into the intricacies of computer science, gaining well-rounded insights into efficient data handling and its impact across modern technologies.Hash Tables - Key takeaways
Hash tables are fundamental data structures in computer science that store data in an associative manner and allow for quick data insertion and retrieval.
An array hash table, a basic form of hash table, uses the key directly as an index to access its associated value, optimising performance when the maximum size of the table is known.
Distributed hash tables (DHTs) are decentralised distributed systems that partition ownership of a set of keys across multiple nodes, allowing any node to efficiently retrieve the value associated with a key.
Hash tables also play a key role in databases, compilers, cache memory, networking, and file systems, contributing to efficient data storage and retrieval.
Advanced applications of hash tables include cryptographic algorithms such as those used in blockchain, distributed systems like BitTorrent, and machine learning algorithms that require efficient data handling.
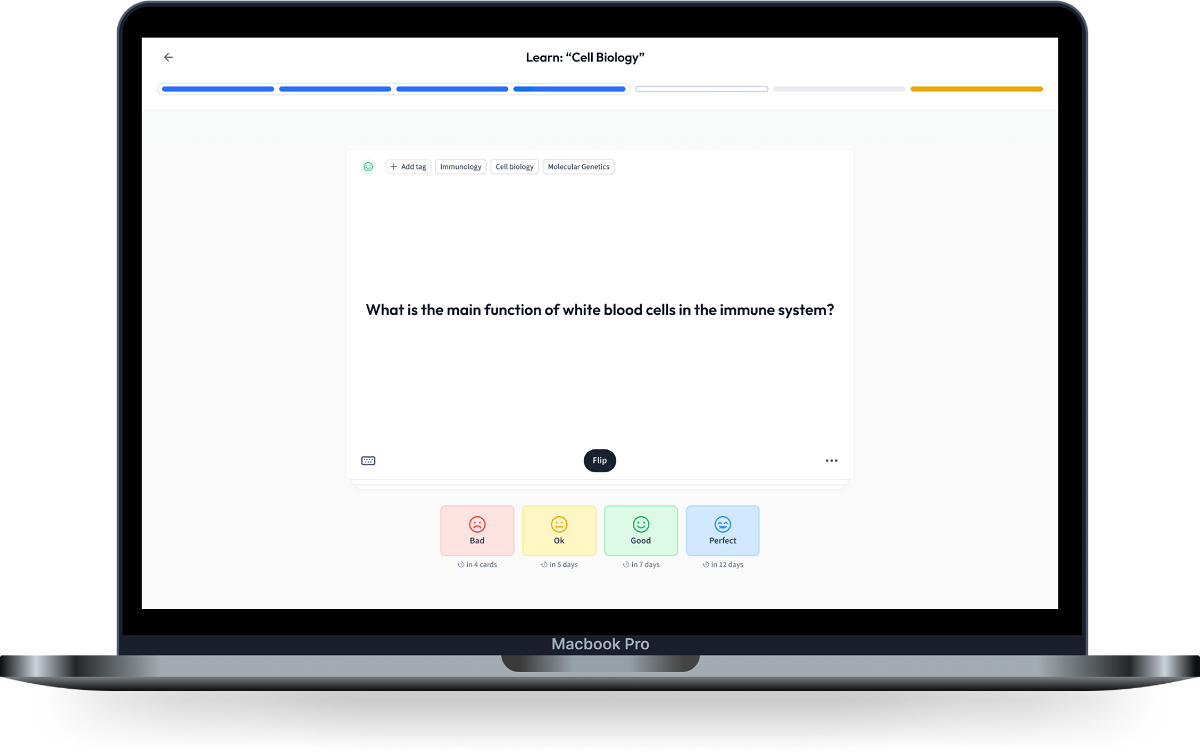
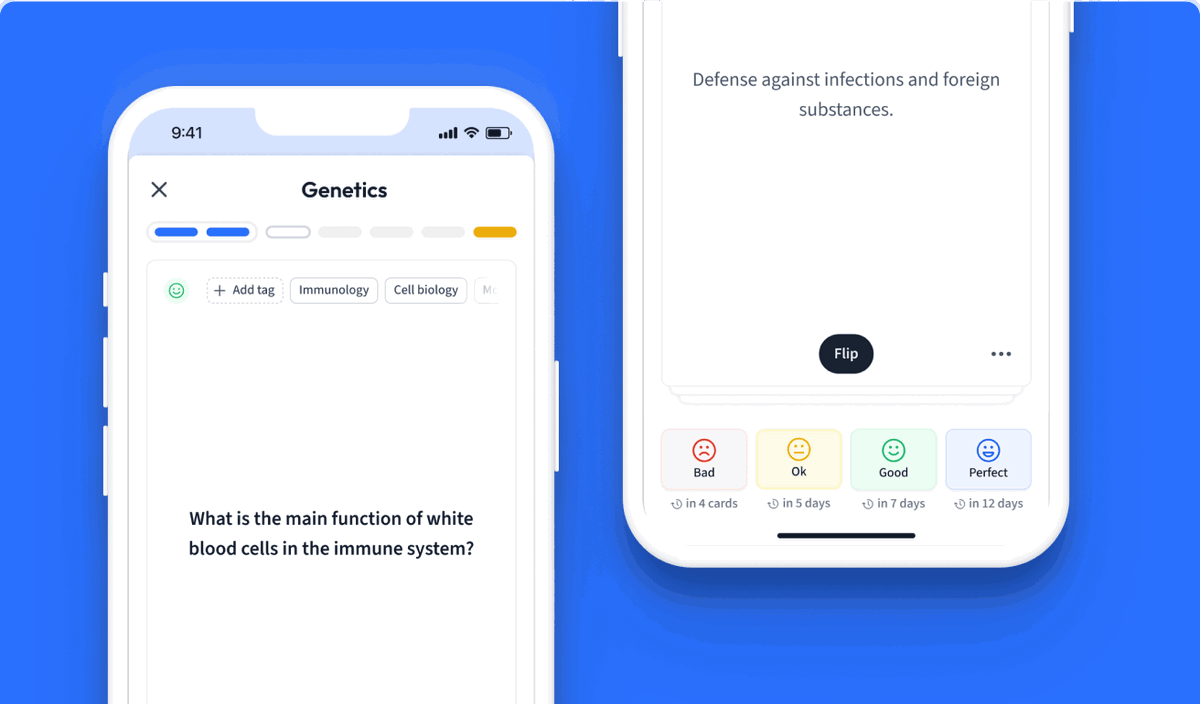
Learn with 15 Hash Tables flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Hash Tables
What are hash tables?
Hash tables are a type of data structure used in computer science to store data in the form of key-value pairs. They use a hash function to compute an index into an array of 'buckets' or 'slots', from which the desired value can be found. This makes them very efficient for data retrieval. Hash tables are widely used in databases and caching systems due to their fast data access capabilities.
What is a hash table java?
A hash table in Java, also known as HashMap, is a part of the Java Collections Framework. It stores data in a key-value pair format which allows for efficient retrieval when the key is known. The key objects must implement the hashCode() and equals() methods. The HashMap class uses a hashing function to map keys to specific indexes in an array, allowing for fast data access.
What is hash table in python?
A hash table in Python is a data structure that implements an associative array abstract data type, essentially a structure that can map keys to values. It uses a hash function to compute an index into an array of buckets or slots, from which the desired value can be found. In Python, the built-in dictionary data type uses a hash table internally. The keys of a dictionary in Python are hashed for immediate access to the values.
How are has tables implemented?
Hash tables are implemented using an array and a hash function. The hash function takes the key associated with the data you want to store and computes an index in the array to store the it. If two keys hash to the same index, a collision is handled usually by linked list or open addressing. This mixture of array indexing and linked list allows efficient searching, insertion, and deletion operations.
How do hash tables work in computer science?
Hash tables in computer science work by utilising a hash function to map data to a specific point in an array or table. This function takes an input, or 'key', and returns a 'hash code', which is then stored in the hash table. When a query is made using a particular key, the hash table uses the hash function to find the location of the data quickly. This technique provides fast access to large amounts of data.
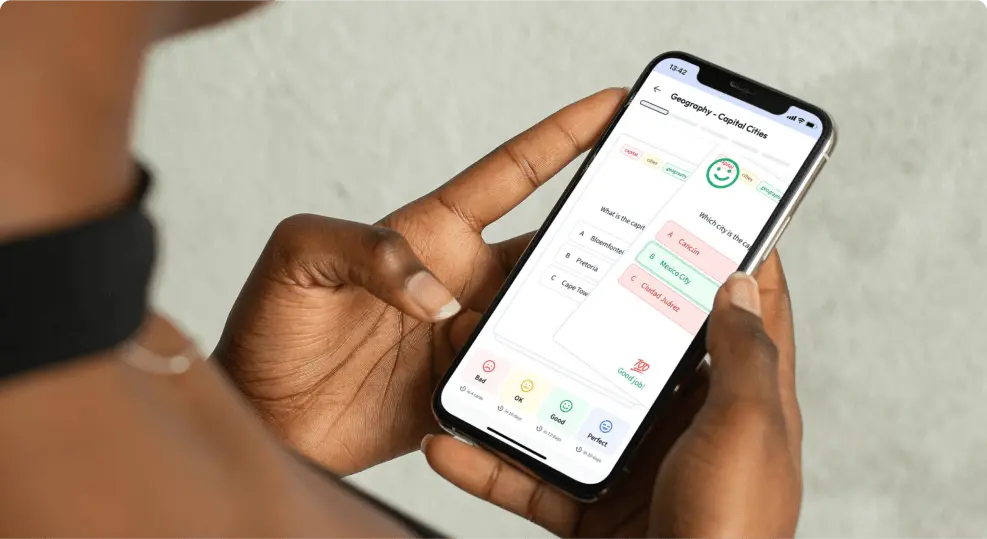
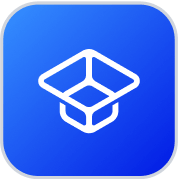
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more