Understanding the Heap Data Structure
A heap data structure in computer science is a type of binary tree. It holds a unique property where each parent node is either less than or equal to its child node (Min Heap) or greater than or equal to its child node (Max Heap).Introduction to Heap Data Structure in Computer Science
In the world of computer science, a heap is a unique data structure primarily used when managing data sets.
Heap can be visualised as a nearly complete binary tree, and operates under strict rules making it one of the optimal choices when it comes to tasks such as sorting methods, priority queues or scheduling programs.
A binary tree is a structure wherein a parent node can, at most, have two child nodes.
The heap data structure is complete if, for a given height, all levels are entirely filled, save for possibly the last level which is filled from left to right.
Heap data structure falls under the category of trees in computer science, with queue and stack being other categories.
Heap Definition in Data Structure: Making Sense of the Basics
When exploring the terrain of data structures in computer science, a fundamental concept you'll come across is the heap data structure. For beginners or even seasoned programmers, understanding the intricacies of heap data structure is crucial as it plays a paramount role in numerous algorithms and applications.Breaking Down the Heap Definition in Data Structures
In data structures, a heap is essentially a complete or near-complete binary tree that satisfies the heap property. Now, let's break down these terms for a deeper understanding. In computer science, a binary tree is a tree-type non-linear data structure with a maximum of two children for each parent. So, each node has at most two children, generally identified as the left child and the right child.Binary tree is one where every node in the tree has at most two children nodes, commonly referred to as the left child and the right child. This 'binary' nature of each node makes it valuable in applications which involve binary decisions or two-way branching.
What makes a binary tree a complete binary tree is if all the levels of the tree are entirely filled, except the last level, which must be filled from left to right. This completeness axiom ensures the optimality of the tree, leading to efficient usage of memory.
Now, the heap property brings another layer to this binary tree. This property states that each parent node's value must be less than or equal to its child nodes in the case of Min Heap.
Conversely, in a Max Heap, the parent node's value is greater than or equal to its child nodes. By maintaining these properties, a heap helps facilitate the extraction of an element with maximum or minimum value, making it popular in applications calling for algorithms like heapsort or priority queue implementation.
- Enforces an order amongst elements, enabling efficient implementation of heap algorithms like heapsort.
- Provides efficient query operations for the min/max element.
Heaps form the underlying data structure in the priority queue abstraction, a key component in graph algorithms like Dijkstra and Prim, and event-driven simulations.
Understanding the Core Aspects of Heap Definition
The definition of heap in data structures may appear straightforward, but it is rich in terms of functionality and use cases, due to its unique properties and efficiency of operations. To illustrate, imagine a binary tree having a data element at each node. If the tree follows a specific order where each parent node is less than or equal to its child node (Min Heap), it is a heap data structure. This particular order, commonly known as the "heap property", leads to the root being the minimum element in the tree. Thus, this provides an efficient solution to extract minimum from a set of elements, resulting in its usage in algorithms which need to repeatedly remove the smallest or largest element. What's more, heaps are often visually represented as trees for conceptual understanding. Yet, the most common practical way to represent them is by using arrays. This helps make the implementation of heaps more space-efficient and simplifies the manipulation of heap elements. Consider the following visualisation of a binary heap as a tree and the corresponding representation as an array:Binary Tree Representation | Array Representation |
---|---|
[10]/ \[20] [40] | [10, 20, 40] |
This makes heap data structures extremely useful in a multitude of applications. From sorting algorithms like heap sort and efficient priority queues to scheduling jobs on computers, heaps have you covered.
In conclusion, the heap as a data structure adds a layer of order and efficiency to a binary tree, making many tasks more efficient, particularly those that require frequent access to the minimum or maximum element. The heap's logical simplicity paired with its computational effectiveness is a testament to its widespread usage in various algorithms and makes it an indispensable part of the study of data structures.
Concepts of Heap Data Structure: Explained
There are two types of heap data structures: Max Heap and Min Heap.In a Max Heap, the parent node is always greater than or equal to its child nodes.
In a Min Heap, the parent node is less than or equal to its child nodes. These rules apply regardless of number of child nodes.
Term | Definition |
---|---|
Root | The top node in a tree. |
Parent | A node, other than the root, that forms a connection to subsequent nodes, or children. |
Child | Nodes that are directly connected to a given node parent. |
Role and Functions of Heap Data Structure
The heap data structure has a wide range of applications where efficiency is paramount in computer science. Using heap data structure can significantly improve computational time in sorting, searching or building functions.For instance, the Heap sort algorithm, one of the renowned sorting methods, uses either the structure of Max Heap or Min Heap to sort numbers in either ascending or descending order.
A priority queue is commonly used in CPU scheduling. It organises items according to individual priority levels and this strategy is efficiently implemented using a heap.
Binary Heap Data Structure: A Closer Look
In heap data structures, binary heap is the most commonly used variant. This data structure is a complete binary tree and can be divided further into two categories: Min and Max heap.Binary Heap Type: Its Design and Functionality
Functioning as a complete binary tree, a binary heap maintains a strict structure. This means that all levels of the tree must be entirely filled except for possibly the last level, which should be filled from left to right.
A complete binary tree demonstrates an excellent balance between tree-like structure and array-like access, thus contributing to the high versatility and usability of the binary heap data structure.
- The root is always available at index 0 (Except in some cases where array starts with index 1)
- For each element at index i, its children are found at index 2i+1 (for left child) and 2i+2 (for right child)
- Similarly, for each child element at index i, its parent can be found at index floor((i-1)/2)
- Insertion (with time complexity of \(O(\log n)\))
- Deletion (also with time complexity of \(O(\log n)\))
- Extraction of minimum/maximum (in constant time \(O(1)\) for an ideal binary heap)
For instance, consider a Min binary heap, with root at index 0. To insert a new value into this heap, you'd start by adding it to the next available space in the array. After insertion, the heap property must be restored.
This is done by comparing the inserted value with its parent. If the parent's value is larger, you'd swap parent and child. Continue this process until the heap regimen is maintained.
Binary Heap: A Key Component of Heap Data Structure
A binary heap, with its intuitive arrangement and efficient operations, serves as the backbone of heap data structures. The binary heap is a space-efficient method to execute priority queue operations due to its \(O(\log n)\) complexity. This efficiency has led the binary heap to be the chosen data structure for algorithms like Dijkstra’s and Prim’s, both of which require priority queue operations. Binary heap is tuned for efficiency but not for searching. Searching a binary heap for an arbitrary value requires \(O(n)\) time in the worst case.Remember, although they share the name "heap," the heap data structure is not related to the heap memory in your computer!
Time Complexity in Heap Data Structure
In any data structure, efficiency is a critical aspect. In this context, efficiency is commonly understood as the time complexity of various operations. Heap data structure is no exception. The time complexity of different operations performed on heaps is a key consideration when using this data structure.How Time Complexity Affects Heap Data Structure
When dealing with any algorithm or data structure, you need to understand the efficiency of operations. This is encapsulated by the concept of time complexity. In layman’s terms, time complexity signifies the amount of time an algorithm takes to run as a function of the size of the input. It is expressed with the big O notation.Time complexity in computer science is a computational measure that describes the change in the amount of computational time taken by an algorithm as the input size changes. It’s referred to using Big O notation such as \(O(n)\), \(O(\log n)\), or \(O(1)\).
- The insertion operation in a binary heap has a time complexity of \(O(\log n)\). This is because insertion might require a traversal up the height of the binary heap, and since it’s a binary tree, it will have a height of log(n).
- Deletion is another common operation in a heap. In the worst scenario, deletion of an element can also take up to \(O(\log n)\) time. This is due to the possible need to maintain the heap property by sifting downwards, a process that could touch each level of the heap.
- One significant advantage of the heap data structure is the ability to extract the minimum or maximum element in constant time, \(O(1)\), for an ideal binary heap. However, it’s worth noting that after removing the root item (minimum or maximum), the binary heap will need to perform a re-heapify operation to maintain the heap property, which takes \(O(\log n)\) time.
Heap Data Structure: Time Complexity Aspects
The timeless principle of time complexity continues to govern the functionality and efficiency of all data structures, and heaps are not exempt from that. As mentioned, heaps particularly excel in their capacity for insertion, deletion, and extraction operations, and their time complexities are worth a deep dive.Let’s say you have a Max Heap, and you need to insert a new element. You add the element to the end (keeping the structure complete), and then you ‘bubble up’ this element to restore the heap property. In other words, as long as the element’s value is greater than its parent's value, you swap it with its parent.
This process continues until the heap property is satisfied. In the worst-case scenario, you might have to traverse the entire height of the heap. As a heap is a binary tree by nature, it results in a height, and by extension a time complexity, of \(O(\log n)\).
Heap Data Structure vs Heap Memory: A Comparative Analysis
In computer science, the term 'heap' refers to two different concepts, each critical in its own domain. Heap serves as a fundamental data structure in one instance and as a critical region of memory management in the other. While they share the same name, they are distinct in their operation and function.Heap in Data Structure: A Delineation
In the context of data structures, a heap primarily refers to a binary heap, which is a complete binary tree modelled as a heap data structure. It is a dynamic data structure that allows you to manipulate hierarchical data swiftly and efficiently. The heap data structure finds wide application in implementing priority queues, in sorting algorithms like heapsort and in graph algorithms. Key characteristics of a heap data structure are:- Each node in the heap has a value. The value of the parent node is either greater than or equal to its children (Max Heap), or less than or equal to its children (Min Heap).
- A heap is usually implemented as an array, providing the heap with impressive space efficiency.
- It exhibits logarithmic time complexity \(O(\log n)\) for insertion and deletion whilst extraction operations can be conducted in \(O(1)\), making the heap data structure highly efficient for manipulating large data sets.
Think of a priority queue, a data structure where elements are served based on their priority rather than based on their sequence in the queue.
For instance, in a printer queue, priority could be defined by the number of pages to print; fewer pages mean higher priority. In such a scenario, using a heap data structure would allow the device to efficiently serve the highest-priority tasks first.
Heap Memory: Conceptual Understanding and Differences
In contrast, the term 'heap' in memory management denotes a region in the computer's memory space used for dynamic memory allocation. It isn't a data structure but a part of a system's memory that is utilised at runtime to dynamically allocate and deallocate memory blocks as per the program’s requirements. Heap memory holds the following characteristics:- Memory blocks within the heap are dynamically allocated and deallocated as required during runtime and not compile time.
- All global variables are stored within the heap memory space.
- The size of heap memory isn't fixed and can shrink or grow as per the requirements of the runtime environment.
- Heap memory is slower in comparison to stack memory, another region of a computer's memory space, because it needs to keep track of all the allocated memory blocks. Thus, it requires extra overhead for memory management.
Heap data structure - Key takeaways
Heap data structure is a type of binary tree that holds a unique property: each parent node is less than or equal to its child node (Min Heap) or greater than or equal to its child node (Max Heap).
Heap data structure is primarily used in managing datasets and can be visualised as a nearly complete binary tree.
Different types of heap data structures include Max Heap, where the parent node is always greater than or equal to its child nodes, and Min Heap, where the parent node is less than or equal to its child nodes.
Key terms for understanding heap data structure include Root (the top node in a tree), Parent (a node that forms a connection to subsequent nodes or children), and Child (nodes directly connected to a given parent node).
The heap data structure's wide range of applications includes sorting, searching, or building functions, thanks to its efficiency of operations ensured by its complete binary tree structure and either Max Heap or Min Heap properties.
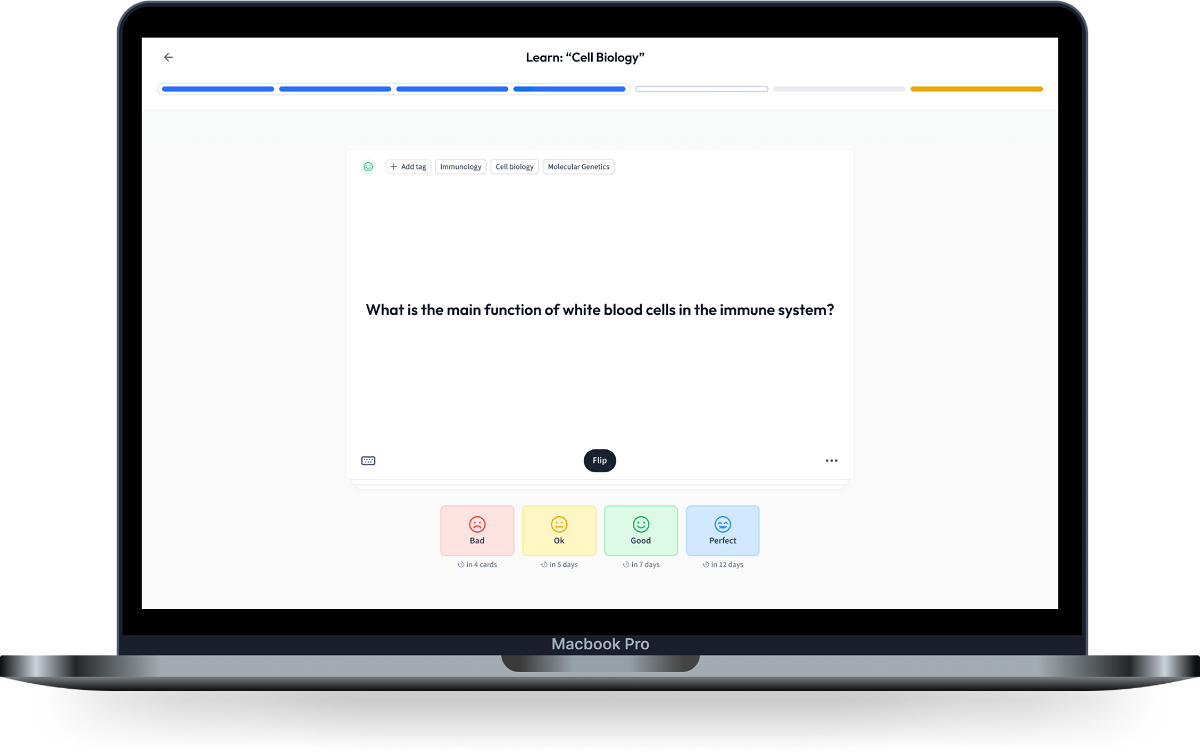
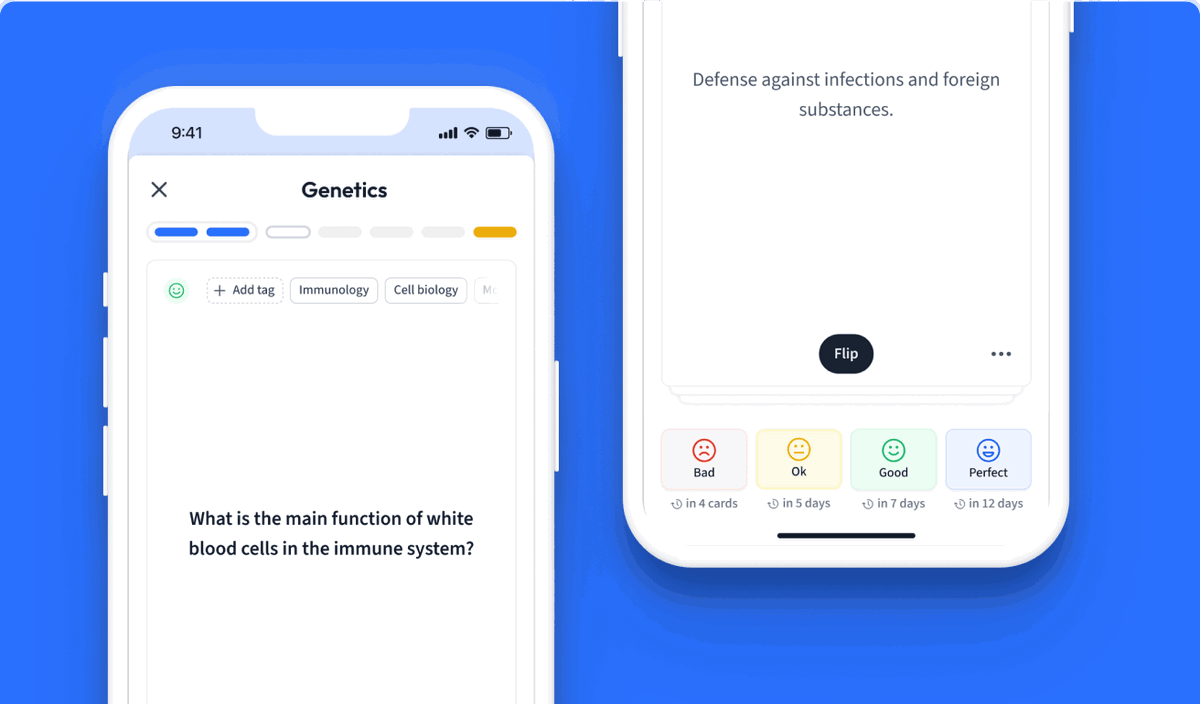
Learn with 15 Heap data structure flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Heap data structure
What is a heap data structure?
A heap data structure is a complete binary tree that satisfies the heap property. It's primarily used in sorting algorithms and priority queue implementations. The heap property dictates that the key of each node is either greater than or equal to (in a max heap) or less than or equal to (in a min heap) the keys of its children. This characteristic makes it ideal for quick access to the maximum or minimum element.
How to create a heap data structure?
To create a heap data structure, start by initializing an empty array or list. Then, to accommodate the heap property, build the heap by continuously adding elements and re-arranging them. If it's a max heap, the parent node should always be larger than its child nodes. If it’s a min heap, the parent node should always be smaller than its child nodes.
What is a heap used for dynamic data structure?
A heap is a specialised tree-based data structure which is used for organising and manipulating dynamic data efficiently. It is mainly used for implementing priority queues, supporting operations like insertions, deletions, and retrieval of the maximum/minimum element in optimal time. Heaps are also utilised in sorting algorithms (like heapsort) and in graph algorithms where the lowest or highest priority element needs to be continuously determined. Use of heap data structure results in significant reduction of running time complexity in such operations.
What is heap sort in data structure?
Heap sort in data structure is a comparison-based sorting algorithm that relies on binary heap data structures. It divides its input into a sorted and an unsorted region, consistently shrinks the unsorted region by extracting the largest element and moving that to the sorted region. The improvement from an "insertion" sort is that it only requires a single pass through the data to build a heap, consequently improving efficiency. Heap sort maintains the property of a heap structure during the sorting process.
What is heap stack data structure?
A heap stack data structure is a combination of two types of data structures - heap and stack. A heap is a type of data structure that satisfies the heap property (in a max heap, for each parent node i, the value should be greater than or equal to its child nodes, and vice versa for a min heap), while a stack is a linear data structure that follows a specific order for its operations, typically LIFO (Last In First Out). These two are used for different types of operations, with heap primarily used for memory allocation and deallocation, and stack used for execution order and backtracking. A heap stack data structure, therefore, combines these attributes to manage memory in an efficient way in computer programs.
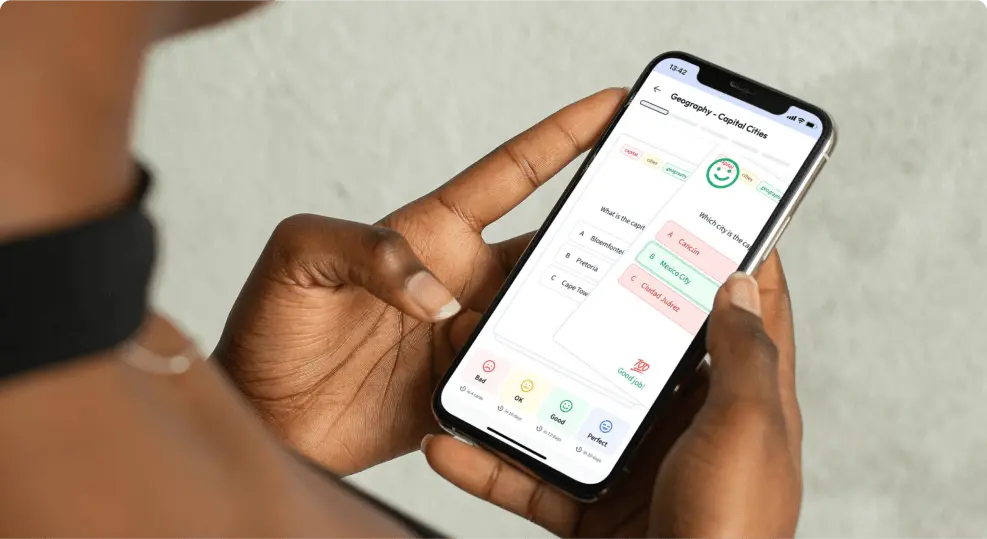
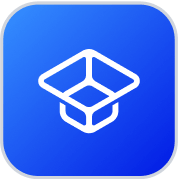
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more