Understanding Red Black Tree in Computer Science
Embarking on the journey to understand computer science, you will encounter various powerful tools and structures. Among these, one of the imperative data structures you're likely to come across is the Red Black Tree.
Red Black Tree: A Basic Definition
A Red Black Tree, belonging to the family of self-balancing binary search trees, aims to maintain balanced data as it's inserted and removed, offering reliable and swift access to stored items. Each node in this kind of tree is denoted by either red or black colours, justifying the name 'Red Black Tree'.
Performance efficiency in binary trees is often hampered when they become unbalanced. But with red black trees, this issue is cleverly taken care of - making them significant in many computer applications.
The language of colour in Red Black Trees is simply metaphorical. The underlying principle is that the 'colour' carries a value that helps balance the data structure by constraining how nodes can be attached to them.
The Structure of a Red Black Tree
Like other binary trees, a Red Black Tree consists of nodes. Each node has the primary components: a key holding the data, pointers to left and right child nodes, and parent node pointer. What sets apart these trees is the addition of a colour property. Here's a simple description of the node structure:
struct Node { int data; //holds the data struct Node *parent; //pointer to the parent struct Node *left; // pointer to left child struct Node *right; //pointer to right child int color; // 1 denotes Red, 0 denotes Black }
An important point to be noted: In Red Black Trees, a NULL reference is treated as a black node. This is an artificial black node, often referred to as an 'external' node.
Primary Rules Governing a Red Black Tree
The functionality and efficiency of the Red Black Trees are maintained by abiding by five primary rules which are:
- Each node is either red or black
- The root node is always black
- All leaves (NULL) are black
- If a node is red, then both its child nodes are black
- Every path from a node (including root) to any of its descendant NULL node has the same number of black nodes
Why Red Black Trees Matter in Computer Science
In the computer science realm, you'll find Red Black Trees playing a pivotal role in various scenarios for their impressive capability to maintain data balance, ensuring optimal worst-case performance for crucial operations such as insertion, deletion, and search. Key usages include:
- Data storage in Maps and Dictionaries
- Scheduling disciplines for network and disk accesses
- Memory allocation
- Used in Completely Fair Scheduler (CFS) which is a process scheduler
For example, the Java Collections Framework has a TreeMap class which is a Red Black Tree based NavigableMap implementation.
Exploring Red Black Tree Insertion
Delving deeper into the core of a Red Black Tree, let's unravel the fascinating process of data insertion. This operation is quite fundamental to harnessing the diverse potential of Red Black Trees, but it's pivotal to adhere to colouring and rotation rules to maintain the tree's balance.
Step-by-step Guide to Red Black Tree Insertion
Insertion in a Red Black Tree involves a systematic process of finding an adequate spot by traversing the tree and fixing any violations to the Red Black Tree properties as a consequence of the insertion. Here's a detailed guide:
- Begin by considering the new node as a Red Node. This is because, by inserting a red node, you limit disturbing the black height property. However, if this red node becomes a child of another red node, then you'd violate the "no two consecutive red nodes" property.
- Then, proceed as you would with a regular Binary Search Tree insertion - traverse from root to leaf, compare the new node and the existing node, move left if lesser, or move right if more.
- Once the appropriate spot for the new node is found, insert it there.
Now, the tree may not be balanced as it should be and might violate the Red Black properties. This imbalance is rectified by a process known as "Fixing the Tree" which is a sequence of colour changes and rotations. Depending on the colour of the parent, uncle, and grandparent nodes of the newly inserted node, the tree can be balanced in one of the below three ways:
- Recoloring: When the parent and uncle are red, the solution is to change the colours: color parent and uncle Black, the grandparent Red.
- Right Rotation: If the parent is red but the uncle is black, a right rotation around the grandparent is performed. Nodes are subsequently recolored.
- Left Rotation: Same conditions as the right rotation, but this time a left rotation is performed. This is usually the case when the new node is a right child.
Practical Examples of Red Black Tree Insertion
It can be particularly beneficial to comprehend the process of Red Black Tree insertion through practical examples. With such understanding, you can solve complex problems with a clear conception of how the data is handled inside these unique trees.
Let's take an example of inserting value 15 into a Red Black Tree. First, the appropriate location for 15 is identified. It's inserted there as a red node. If the parent of 15 is black, then no more action needed.
But in a scenario where 15's parent (say 10) is red, this causes a violation as there will be two consecutive red nodes. Pertaining to the rules of fixing a red black tree, you will then need to check if the uncle node is red or black. If the uncle is red, then recoloring is performed. If the uncle is black, rotation is required upon the grandparent node, and nodes are accordingly recolored. The final step is to recolor the root as black.
Common Challenges Faced in Red Black Tree Insertion
While Red Black Tree insertion might seem pretty straightforward when you grasp the rules, there can be a few challenges or misconceptions that can possibly curtail getting the ideal results:
- Disregarding the Balancing Rules: One of the biggest mistakes can be neglecting the balancing rules after insertion. Without rectifying violations, the tree would lose its optimal efficiency.
- Incorrectly Identifying the Uncle Node: Correctly identifying the uncle node is crucial in determining whether a rotation or recoloring is required. A wrong identification can lead to an incorrect balance operation.
- Forgetting to Recolor the Root: One common blunder is not recoloring the root as black after each insertion or rotation. This is a fundamental part of retaining Red Black Tree properties.
It's important to remember that mastering Red Black Tree operations requires time, patience and hands-on practice. Don't get disheartened by initial complexities. With a clear understanding of the rules and ample practice, it can become an indispensable tool in your computer science pursuits.
Examining Red Black Tree Balancing
As your journey into the realms of computer science unfolds, we find ourselves delving into the intriguing mechanisms governing the Red Black Tree, a unique data structure known for its innate capacity to maintain balance with every insertion or deletion. In this section, you will discover why balancing is integral to the optimal functioning of a Red Black Tree and learn some of the most common techniques employed to achieve this balance.
The Importance of Balancing a Red Black Tree
Before you plunge into the 'how' of Red Black Tree balancing, let's pause a moment to grasp the 'why'. Why is balancing so significant in a Red Black Tree? At its core, balancing a Red Black Tree is integral to exploiting the true superpower of this data structure - swift and reliable access to stored data.
When you insert or delete a node in a Red Black Tree, this action may lead to a violation of the essential colouring and chain rules that govern these trees, resulting in the tree becoming 'unbalanced'. An unbalanced tree can compromise the worst-case performance time of \(O(\log n)\) for fundamental operations, thereby causing inefficiencies in the applications utilising this tree.
Through balancing, we can rectify these violations, restore the Red Black Tree's properties, and retain the efficient logarithmic time complexity. Ultimately, balanced Red Black Trees fortify our programs and applications to deliver optimal performance, driving the overall efficiency in our data manipulations.
Consider, for instance, databases that rely on Red Black Trees for searching index records; or language libraries that use these trees for ordered data manipulation. Without efficient, balanced Red Black Trees, these applications could experience performance lag which can have cascading implications in real-time usage.
Case Study: Balancing a Red Black Tree
Immerse yourself in a practical understanding of Red Black Tree Balancing in action. Assume you're inserting a new node in the Red Black Tree. You begin the procedure as per standard Binary Search Tree insertion. Once the node is inserted, now is the crucial time to address the colouring and chaining rules, rectify violations and restore the tree's balance.
Let's take a case where the new node to be inserted is 'A'. Post insertion, 'A' becomes the right child of its parent 'B', causing a violation as 'B' is also red. Here, 'B' is the left child of 'A's' grandparent 'D'. Thus, according to the rules, we'll rotate right at 'D'. This swapping places 'A' as a child of 'B', and 'D' becomes a child of 'A'. Now, we switch colours: 'A' turns black and 'D' turns red.
Common Techniques in Balancing a Red Black Tree
Accomplishing balance in a Red Black Tree revolves around mastering two essential techniques: rotation and recolouring. These operations, governed by precise rules, hold the key to rectifying any imbalances triggered by node insertions or deletions.
- Rotation: The operation of rotation is performed when the uncle node of the newly inserted node is black, which could be either a 'Right Rotation' or a 'Left Rotation', depending on the position of the new node. If the new node is a 'right child', perform a 'Left Rotation'. Similarly, if the new node is a 'left child', perform a 'Right Rotation'. Whilst rotation rearranges the nodes, it is also essential to recolour them correctly.
- Recolouring: This operation becomes crucial when the uncle node of the newly inserted node is red. In such cases, a colour reversal is performed. The parent and uncle nodes are painted black and the grandparent node is coloured red. It's noteworthy that if the grandparent node was the root, its colour would remain black to adhere to the rule stating every Red Black Tree must have a black root.
Undertaking a balancing operation in a Red Black Tree underscores the fine balance maintained between the structural rules and algorithmic operations within this unique data structure. Remember, precision and accuracy in executing these operations pave the way for a successfully balanced Red Black Tree.
Practical Examples of Working with a Red Black Tree
As fascinating as theories might be, let's move into a more practical dimension. This will give you an insightful perspective on how to work with a Red Black Tree, applying the knowledge you've gained so far.
Comprehensive Example of a Red Black Tree
Applying the colouring and rotation rules in actual manipulation and creation of a Red Black Tree can offer a solid understanding of how these trees function. Let's explore a comprehensive example to demonstrate how you'd build a Red Black Tree using Red Black Tree properties.
Consider you have to construct a Red Black Tree using numbers 7, 3, 18, 10, 22, 8, 11, 26, 2, 6, and 13.
We begin by inserting 7 as a black root node. Following the rules of a Binary Search Tree, we insert 3 as a left child of 7 and colour it red. Next is 18, which is more than 7, so 18 becomes the red right child of 7. 3’s left child becomes 2 and is coloured black (to avoid two consecutive reds).
Next when 10 is inserted, it turns out red (avoiding consecutive reds with 18). When we insert 22 (red), it becomes 18’s right child causing no violations. The insertion of 8 represents a complex case with multiple transformations. Post insertion, we get two consecutive reds (between 10 and 8). To solve this, first, a left rotation around 10 is performed. Then, recolouring switches colours of 10 and 18, and we perform a right rotation around 7. As per the rules, the root is set as black.
The remaining nodes are inserted orderly, performing the necessary transformations to avoid violations of Red Black Tree properties. The resulting Red Black Tree demonstrates the fascinating synergy of the colouring, rotation, and chaining rules that underpin these unique data structures.
Applying Red Black Tree Rules in Real-Life Examples
Taking a leap from abstract numbers to tangible, real-world scenarios can help you visualise the application and benefits of Red Black Trees. Work through these examples to appreciate how these self-balancing trees make a tangible impact in daily applications.
- Database Systems: Imagine you're creating a database system for a bustling library. The library has an extensive range of books, and for efficient searching, this immense data needs to be stored in a manner that enables speedy retrieval. A Red Black Tree can be used to store book records indexed based on unique identifiers. The balance maintained in this tree ensures that any addition (new book), deletion (book removed), or search (book location) happens in logarithmic time, paving the way for an efficient database management system.
- Operating Systems: Let's assume you're designing a scheduler in an operating system. It has to handle a multitude of processes effectively and quickly. Packaging these processes into a Red Black Tree facilitates efficient and equitable distribution of CPU time. The tree's balancing properties ensure that high-priority processes are served swiftly.
- Wireless Networking: Envisage you're engineering a wireless network system where countless packets jostle to be transmitted through network routers. To implement a fair queuing algorithm efficiently, these packets can be sorted in a Red Black Tree structure. This tree aids in swiftly identifying the next packet to be transmitted and quickly updating the queue after each transmission.
That said, the mastery of Red Black Tree operations dictates how effectively you can handle these real-life scenarios. So, remember those rules, tweak those nodes, recolour as necessary, rotate them right and left, and balance your way to build idealist Red Black Trees ready to marshal your data efficiently.
Delving Deeper into Advanced Red Black Tree Concepts
As you deepen your understanding of Red Black Trees, it's pivotal to explore some of the more complex, yet crucially important aspects of this distinctive data structure. Sailing through some of these advanced facets enables you to navigate the vast sea of Red Black Trees more confidently and facilitates a comprehensive grasp on implementing them in varied computational scenarios.
Understanding Red Black Tree Rotation
Rotations are one of the most instrumental operations employed in maintaining the balance of a Red Black Tree. Essential while inserting or deleting nodes from the tree, rotations come in two avatars - right rotation and left rotation. The choice between right or left rotation isn't arbitrary; it's driven by the position of the node causing the imbalance.
Imagine rotation as a pivot operation where nodes are rearranged around a pivot node, with the pivot node and its subtrees changing places. This rearrangement is performed respecting the order property of the Binary Search Tree.
During a Right Rotation, the pivot is the node's left child. In this operation, the parent node becomes the pivot node's right child. All nodes in the subtree to the pivot's right get linked to the parent's left.
Conversely, in a Left Rotation, the pivot is the node's right child. The parent node becomes the left child of the pivot, and all nodes in the subtree to the pivot's left are linked to the parent's right.
Despite sounding complex, rotations in Red Black Trees adhere strictly to the ordering rules of Binary Search Trees. Remember, the aim is to restore balance, ensuring the tree remains an efficient pathfinding structure.
Exploration: Deletion in Red Black Tree
The operation of deletion in Red Black Tree invokes a series of well-defined steps. Removing a node in a Red Black Tree isn't ordinary, as the process often impacts the balance of the tree. Therefore, every deletion operation would likely trigger one or more transformations to restore the tree's properties. As you follow these sets of steps and rules, often resulting in rotation and recolouring, you can maintain a true Red Black Tree.
When deleting a node:
- The node to be deleted is first identified. If the located node has less than two non-NULL child nodes, it gets deleted and replaced by its child (which could be NULL).
- If the node to be deleted has two non-NULL children, it's replaced by its inorder successor, maintaining the Binary Search Tree property.
Post deletion, attention is directed towards maintaining the Red Black Tree properties. If the deleted node is red, there's no issue. But things escalate when the deleted node is black. Black nodes are crucial to maintaining the black-height property of a Red Black Tree. Therefore, losing one can cause an imbalance.
To correct this, Red Black Tree follows a detailed recolouring and rotation process. This process depends on the sibling of the current node, and it has different cases handling such as if the sibling is black (and its children), when the sibling and its children are red, when the sibling is black and its left child is red (but right child is black), or vice versa.
This intricate dance of deletion and rebalancing is a spectacular exhibition of the Red Black Tree properties coming into play, exerting themselves to ensure the tree stays efficient, balanced and poised for rapid data access and manipulation.
Advanced Topics: Red Black Tree Variations and Uses
As we ascend up the ladder of complexity, it's fitting to introduce some variations and uses of the Red Black Tree. These variations demonstrate how the core Red Black Tree structure can be extended and adapted for specific applications, contributing to their versatility.
AA Trees are a variation of Red Black Trees. They constrain red nodes to have only left children, enforcing a strict left-leaning structure. This simplifies operations, although at the cost of slightly increased tree height.
Another variation is the Left-leaning Red Black Tree (LLRB), similar to AA Trees but permitting red right children under temporary conditions. This type also simplifies implementation by ensuring no two red nodes are adjacent, reducing instances needing recolouring or rotation.
Not just variations, Red Black Trees find myriad applications across different domains:
- Resource Allocation: Red Black Trees can perform resource allocation in operating systems, scheduling disks, and CPU in real-time systems.
- Ranges and Dimensions: In computational geometry, they help efficiently solve problems involving ranges two and multi-dimensions.
- Databases: Red Black Trees structure database indexes to ensure balanced, quick data retrieval.
From subtle variations to diverse uses, the versatile Red Black Tree dominates a range of computing landscapes, proving to be an essential asset in the realm of data structures.
Red Black Tree - Key takeaways
- Red Black Tree: It is a unique data structure known for its innate capacity to maintain balance with every insertion or deletion and provides swift and reliable access to stored data. The system of color-coding and rotations are responsible for its balance.
- Insertion in Red Black Tree: It involves finding an adequate spot by traversing the tree and fixing any violations to the Red Black Tree properties as a consequence of the insertion. Newly inserted nodes are generally considered red to limit disturbing the black height property.
- Balancing a Red Black Tree: It involves maintaining the Red Black Tree's properties (coloring and chain rules) and retaining the efficient logarithmic time complexity. This could be achieved by two techniques - rotation and recoloring.
- Rotation in Red Black Tree: It comes into play when the uncle node of the newly inserted node is black. We perform right rotation if the new node is left child, and left rotation if the new node is right child.
- Recoloring in Red Black Tree: When the uncle node of newly inserted node is red, we perform recoloring changing the colors of parent, uncle and grandparent nodes.
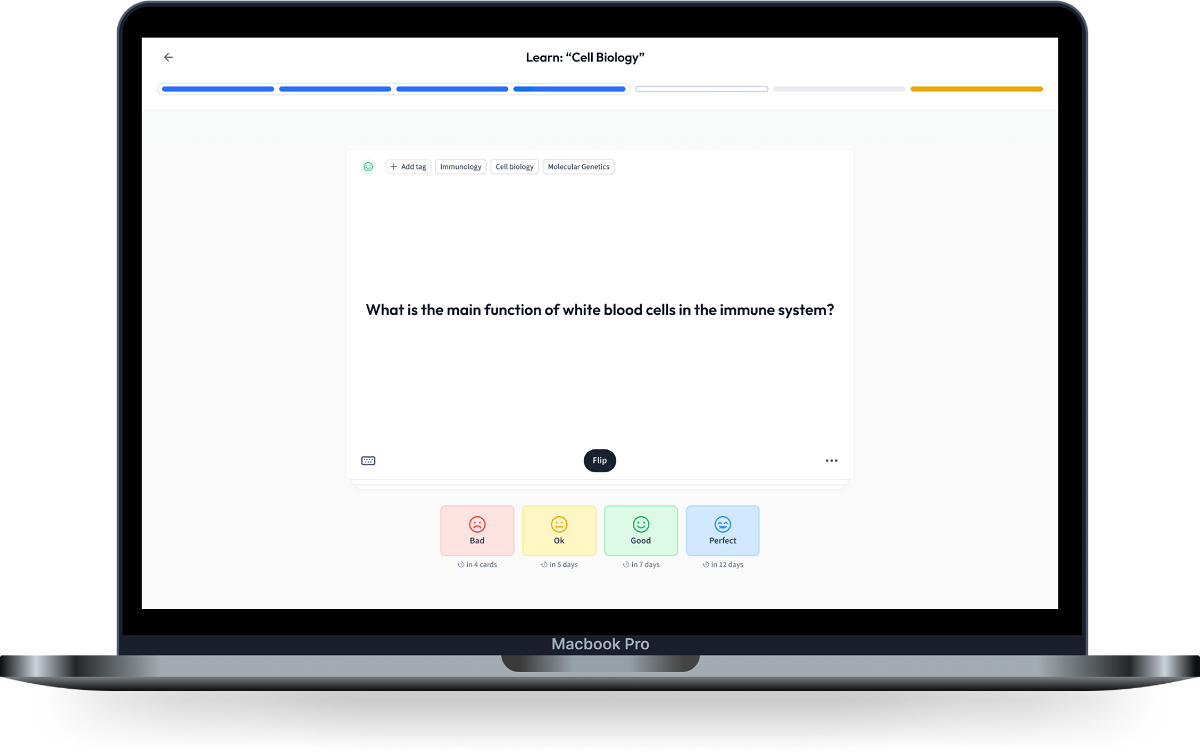
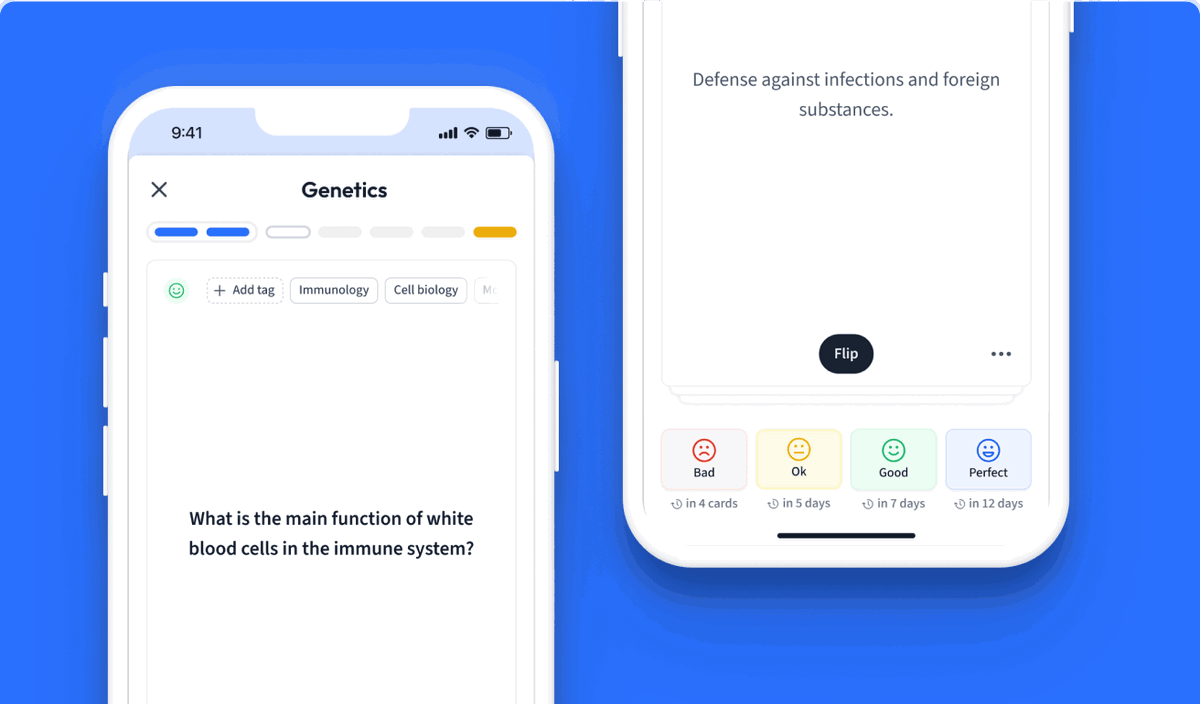
Learn with 15 Red Black Tree flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Red Black Tree
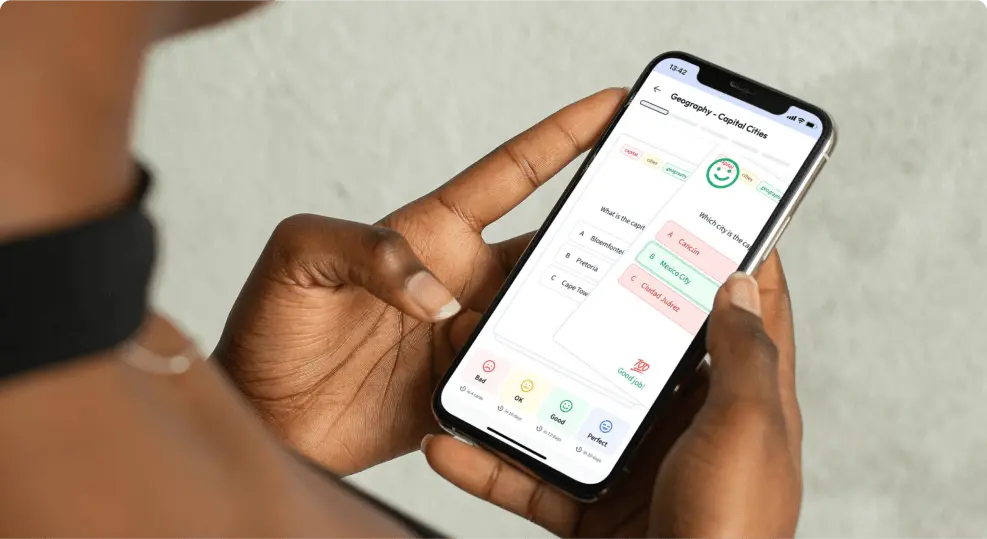
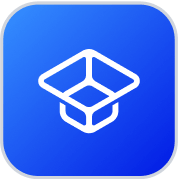
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more