Introduction to Java Bitwise Operators
Java bitwise operators play a pivotal role in programming since they allow you to manipulate individual bits of data. If you're diving into coding in Java, it'll be highly advantageous to familiarise yourself with these operators, as they can provide shortcuts for performing operations on binary numbers.
A Dive into the Java Bitwise Operators Definition
Bitwise operators, as used in Java, are operators that can manipulate individual bits of an Integer.
The bitwise operators in Java include:
- & (bitwise and)
- | (bitwise or)
- ^ (bitwise XOR)
- ~ (bitwise compliment)
- << (left shift)
- > (right shift)
- >> (zero fill right shift)
Let's dive a little deeper into what each of these operators does.
& | The & operator compares each binary digit of two integers and gives back a new integer, with "1" wherever both compared bits were "1". |
| | The | operator compares each binary digit across two integers and returns a new integer, with "1" wherever at least one compared bit was "1". |
^ | The ^ operator compares each binary digit across two integers and returns a new integer, with "1" wherever the two compared bits were different. |
~ | The ~ operator takes one integer and flips all of its bits. |
Now we will look into shift operators. Remember, these shift the binary representation of their first operand, to the left or the right. The number of places to shift is given by the second operand.
Core Principles of Java Bitwise Operators
public class Main { public static void main(String[] args) { int a = 60; /* 60 = 0011 1100 */ int b = 13; /* 13 = 0000 1101 */ System.out.println("a & b = " + (a & b)); /* 12 = 0000 1100 */ System.out.println("a | b = " + (a | b)); /* 61 = 0011 1101 */ System.out.println("a ^ b = " + (a ^ b)); /* 49 = 0011 0001 */ System.out.println("~a = " + ~a); /* -61 = 1100 0011 */ System.out.println("a << 2 = " + (a << 2)); /* 240 = 1111 0000 */ System.out.println("a >> 2 = " + (a >> 2)); /* 15 = 0000 1111 */ System.out.println("a >>> 2 = " + (a >>> 2)); /* 15 = 0000 1111 */ } }
In the code above, you can see bitwise operators (&, |, ^, ~) and shift operators (<<, >>, >>>) in action. The operands are a and b, and the results are printed out with an accompanying binary comment.
A deep dive into Java's bitwise operations turns up some interesting insights. Did you know they can be used for a variety of tasks, like finding the opposite sign of an integer, swapping two numbers without a temp, or checking if a number is a power of two? They're amazing tools to have in your programming toolbox, offering efficient solutions to many problems.
Syntax of Java Bitwise Operators
Similar to many other programming languages, the syntax for Java bitwise operators is straightforward. The operators themselves are generally punctuation marks and are placed between the operands they're meant to act upon. They work on integral data types and only work with integers.
Deciphering the Java Bitwise Operators Syntax
Deciphering the syntax of Java bitwise operators essentially revolves around understanding that these operators function on the binary representations of integers. The operator is positioned between the operands. Let's see what this looks like.
In the context of the bitwise AND operator (&), the syntax would look like this: int c = a & b; Break it down, 'a' and 'b' are the operands and '&' is the bitwise AND operator.But don't forget, the same principle applies for other operators too.
int a = 42; /* 42 = 101010 */ int b = 24; /* 24 = 011000 */ int c; c = a & b; /* c gets the result of 42 AND 24, which is 8: 001000 */ System.out.println("a & b = " + c);
The above code showcases an operation where bitwise AND is used between the two decimal numbers 42 and 24. It gives us an output of 8 because it does the task of computing the binary AND-operation between the two conversions. Take the binary version of 42, which is 101010, and 24, which is 011000. Now, perform the AND operation. Whenever both bits are '1', the result is '1'. Where one (or both) of the bits are '0', the result is '0'. Hence, you get the resulting binary 001000, and converting it back to decimal provides you with 8.
The Role of Punctuation in Java Bitwise Operators Syntax
In Java bitwise operators, punctuation plays the crucial role of representing a particular operation that we want to perform. As you have seen, the unique symbolism of each operator (&, |, ^, ~, <<, >>, >>>) stands for different bitwise operations.
For instance, the left shift operator (<<) takes two operands: a binary digit and an integer. This operator shifts the first operand’s binary representation to the left by a number of bits specified by the second operand.
The syntax is as follows: int c = a << b; where 'a' and 'b' are the operands and '<<' is the left shift operator. If you have int a = 15 (binary 1111) and you want to shift it two places to the left (a << 2), you end up with 60 (binary 111100).
As an added note, the right shift operator (>>) works in a similar way, except the bits are shifted to the right. And the zero fill right shift operator (>>>) also shifts to the right, but fills leftmost bits with zeros, which is different from the regular right shift operator, that propogates the leftmost (sign) bit.
Assuming int a = -15 (binary 1111 1111 1111 1111 1111 1111 1111 0001) and you want to shift it two places to the right using the regular right shift (a >> 2), you end up with -4 (binary 1111 1111 1111 1111 1111 1111 1111 1110). If you were to use the zero fill right shift (a >>> 2), you would end up with 1073741820 (binary 0011 1111 1111 1111 1111 1111 1111 1100).
In essence, punctuations in Java bitwise operators serve as symbols representing a specific operation on binary digits.
Practical Examples of Java Bitwise Operators
To provide a proper understanding of Java bitwise operators, let's examine some practical examples. They will illustrate how you can use these operators to solve real-world programming challenges efficiently. Remember, thorough comprehension of these examples requires you to apply your understanding of the operators' syntax and function.
Full Explanation of Java Bitwise Operators Examples
Here, we have chosen two practical examples: one for computing the absolute value of an integer, and the other to check if an integer is even or odd.
1. Computing Absolute Values: Java bitwise operators can be put to use in order to find the absolute value of an integer. This can reduce the use of if-else conditions. The method applied involves using the bitwise NOT (~) and bitwise right shift (>>) operators.
// Function to calculate absolute value static int absolute(int n) { int mask = n >> 31; // Bitwise AND of n and mask gives n if // n is positive else gives ~n return ((n + mask) ^ mask); } // Driver code public static void main(String[] args) { int n = -10; System.out.println(absolute(n)); }
The Java method 'absolute' takes an integer as its argument, applies the right shift operator to create a mask that is utilised thereafter. The result of right shift operation is negative if the entered number is negative, and zero otherwise. When this mask is added to the number 'n' and then XORed with the mask, a positive number is returned in all cases. Thus, bitwise operators are instrumental in determining the absolute value of a number.
2. Determining Even or Odd: The bitwise AND operator can be used to check whether a given number is even or odd. The binary representation of all even numbers ends with 0, while that of odd numbers ends with 1. Using bitwise AND with 1 will yield 0 for even numbers and 1 for odd.
static String checkEvenOrOdd(int n) { return ( (n & 1) == 0 ) ? "Even" : "Odd"; } public static void main(String[] args) { int n = 7; System.out.println(n + " is " + checkEvenOrOdd(n)); }
In the 'checkEvenOrOdd' method, a number 'n' is taken as input. It is then ANDed with 1. If 'n' is an even number, its binary representation will end with 0, and ANDing with 1 yields 0. For an odd number, the result will be 1. Therefore, this method successfully checks for even or odd numbers using bitwise operators.
Step-by-Step Creation of Java Bitwise Operators Example
Now, let's create an example using the bitwise XOR operator to swap two numbers.
Swapping Two Numbers: With the help of bitwise XOR, you can swap the values of two variables without using a third variable.
Step 1: Declare and initialise two variables, let's say 'a' and 'b'.
int a = 5; int b = 4;
Step 2: Use the bitwise XOR operator to perform the swap.
// XORing 'a' and 'b' a = a ^ b; b = a ^ b; a = a ^ b;
First, 'a' is XORed with 'b' and the result is assigned to 'a'. At this point, 'a' has the XOR of 'a' and 'b', while 'b' still holds its original value.
Next, 'b' is XORed with the new 'a' (which is the original 'a' XOR 'b'), yielding the original 'a', which is assigned to 'b'. Now 'a' is still 'a' XOR 'b', while 'b' has become 'a'.
Finally, 'a' is XORed again with 'b' (which is now the original 'a'), yielding the original 'b', which is assigned to 'a'. This leaves 'a' with the original 'b' value and 'b' with the original 'a' value. Hence, the swap is achieved.
public static void main(String[] args) { int a = 5; int b = 4; // Swapping a = a ^ b; b = a ^ b; a = a ^ b; System.out.println("After swapping: a = " + a + ", b = " + b); }
This practical application demonstrates how Java bitwise operators can be used to efficiently solve problems, making your code cleaner and more optimal.
Deeper Understanding of Java Bitwise Operators
A deeper understanding of Java Bitwise Operators necessitates an expanded view of how they function, both on the mathematical and logical level. Let's dig into the details to unlock your full potential in utilising these powerful tools in your Java programming practices.
Expanding Knowledge on the Use of Java Bitwise Operators
Java Bitwise Operators allow manipulations of individual bits in an integer number. They are primarily used for testing, setting, or shifting bits. Given that everything in digital computation ultimately gets traced back to binary data at the most basic level, mastering bitwise operators can grant you a high degree of flexibility and optimality.
Bitwise AND operator (&): This operator performs a Boolean AND operation on each bit of the integer. It returns 1 if both bits are 1, else it returns 0.
Bitwise OR operator (|): This operator performs a Boolean OR operation on each bit of the integer. It returns 1 if at least one bit is 1, else it returns 0.
Bitwise XOR operator (^): This operator performs a Boolean exclusive OR (XOR) operation on each bit of the integer. It returns 1 if the two compared bits are different, else it returns 0.
The XOR operator can particularly be of great help in multiple practical scenarios. Since XOR of any number with itself returns 0, and XOR of any number with 0 gives the number itself, this property can be used in numerous sorts of applications. For instance, it can be used to find a missing number in an array, or as previously mentioned, for swapping two numbers.
The Dos and Don'ts When Using Java Bitwise Operators
While Java Bitwise operators are extremely insightful, they do come with certain dos and don'ts that are necessary to understand for writing precise and error-free code:
- Do use bitwise operators when performing operations on bits, especially when working directly with binary numbers or when optimisation of speed and memory is necessary.
- Don't use bitwise operators on float or double data types. These operators are designed to work only with integers.
- Do use a bitmask when you need to set, test, or shift a particular bit in a number.
- Don't forget that the bitwise shift operators effectively multiply and divide by powers of two. So, use them instead of algebraic operations for optimising code.
- Do remember that the unsigned right shift operator (>>>) fills the leftmost bits with 0 while the right shift operator (>>) propagates the leftmost bit. You should take this into account when dealing with negative numbers.
Just like all other tools, bitwise operators also have their strengths and weaknesses. It's crucial to understand their behaviours, especially when working with negative numbers and large integers. Remember that on applying the shift operators on large integers, you need to take into account that Java does not have unsigned integers. Therefore, when right shifting negative numbers with (>>), you need to be extra careful because the sign will be carried to the right.
In conclusion, mastering Java bitwise operators entails both understanding their usage on a deeper mathematical and logical level, as well as knowing what to do and what to avoid when using them. By adhering to these dos and don'ts, you'll definitely sharpen your skill set in Java programming and elevate your code writing practices to a new level of proficiency!
Problem Solving with Java Bitwise Operators
In your programming journey with Java, you will often encounter scenarios that require optimisation and efficient problem-solving strategies. One of the tools at your disposal is the set of Java Bitwise Operators. The bitwise operators work directly with the binary form of data, allowing the manipulation of individual bits in an integer, which can help craft optimal solutions to many problems, especially those related to the digital world.
Mastering Practical Use of Java Bitwise Operators
Making effective use of Java Bitwise Operators involves understanding their functionality and knowing when to deploy them. Bitwise operators have specific operations like shifting the bits left or right, flipping the bits, or performing logical operations like AND, OR and XOR on the bits.
For instance, one often used operator is the Bitwise AND operator (&). It performs a Boolean AND operation on each bit of the integer. In mathematical terms, it returns 1 only if both compared bits are 1. For example, let's take two numbers 12 and 25. Their binary representations are 1100 and 11001, respectively. Bitwise AND operation of 12 and 25 can be calculated as:
1100 11001 ----- 11000 (This is the binary equivalent of 24)So, 12 & 25 would return 24.
Another highly useful operator is the Bitwise OR operator (|), which performs a Boolean OR operation on each bit of the integer. It returns 1 if at least one bit is 1. In terms of numbers, 12 | 25 would return 29 (11101 in binary).
Yet, arguably one of the most potent tools you've got is the Bitwise XOR operator (^). Its beauty lies in the fact that it returns 1 only if the two compared bits are different, breaking new grounds when it comes to detecting changes in bit patterns. A practical example could be in error detection and correction codes in digital communications, where XOR is used to identify the bits that have been changed due to noise or other data distortions.
For instance, if we were to XOR 12 and 25, we get: 1100 (12) 11001 (25) ------ 11101 (29)
Thus, 12 ^ 25 would return 29.
Reflecting on these examples, you can clearly see that mastering Java Bitwise Operators can expose new ways to optimise and enhance code. Importantly, these operators enable direct access and manipulation of individual bits, bringing about solutions that might not be possible or as efficient with standard mathematical operators.
Tips and Tricks for Applying Java Bitwise Operators
Finally, let's move onto some nifty tips and tricks to help you navigate the application of Java Bitwise Operators. These techniques not only assist assured coding, but will enhance your understanding as well, making Java Bitwise Operators a robust tool in your programming arsenal.
- Optimise Arithmetic Operations: Bitwise left shift (<<) and right shift (>>) operators can be used as a quick way to multiply or divide a number by two, respectively. Note that for negative numbers, using the right shift operator (>>) can lead to unexpected results as it carries the sign bit. For such cases, use the unsigned right shift operator (>>>).
- Manipulate specific bits: A common use of bitwise operators is to set, clear, or toggle specific bits in a number. This is achieved by creating a mask with the bit pattern you want and then using bitwise AND, OR, or XOR to change the desired bits.
- Test bits quickly: Applying bitwise AND with a mask can quickly test whether certain bits in a number are set. For example, if you have an integer and you want to check if the 3rd bit is set, you can AND the number with the binary number 1000 (8 in decimal), and if the result is non-zero, then the 3rd bit is set.
int num = 14; // 1110 in binary int mask = 8; // 1000 in binary if ((num & mask) != 0) { // The 3rd bit is set }
These are just a few examples of the many ways in which you can apply Java Bitwise Operators creatively to solve problems. The more you work with these operators, the more familiar you will become with their quirks and characteristics. Remember to keep your bitwise operator skills sharp by implementing them regularly in your programming tasks and challenges. Happy coding!
Java Bitwise Operators - Key takeaways
- Java Bitwise Operators: These operators allow manipulation of individual bits in an integer number and can be used to efficiently solve programming challenges. These include bitwise AND (&), OR (|), XOR (^), NOT (~), and shift operators (<<, >>, >>>).
- Java Bitwise Operators definition: Bitwise operators in Java perform actions at the bit level and are primarily used for testing, setting or shifting bits. They only work with integers.
- Java Bitwise Operators Syntax: In Java, bitwise operators are typically punctuation marks such as &, |, ^, ~, <<, >>, >>>, and are placed between the operands they're meant to act upon. For example, int c = a & b; in the context of the bitwise AND operation. The operands are a and b, and & is the bitwise AND operator.
- Example of Java Bitwise Operators: Bitwise operators can be used to solve programming challenges such as computing the absolute value of an integer, determining whether a number is even or odd, or swapping two numbers without using a third variable.
- Use of Java Bitwise Operators: Bitwise operators are valuable tools in programming as they offer efficient solutions to many problems. Mastering these operators can provide a high degree of flexibility and optimality in coding. However, care should be taken not to use them on float or double data types, and understanding their behaviour with negative numbers and large integers is crucial.
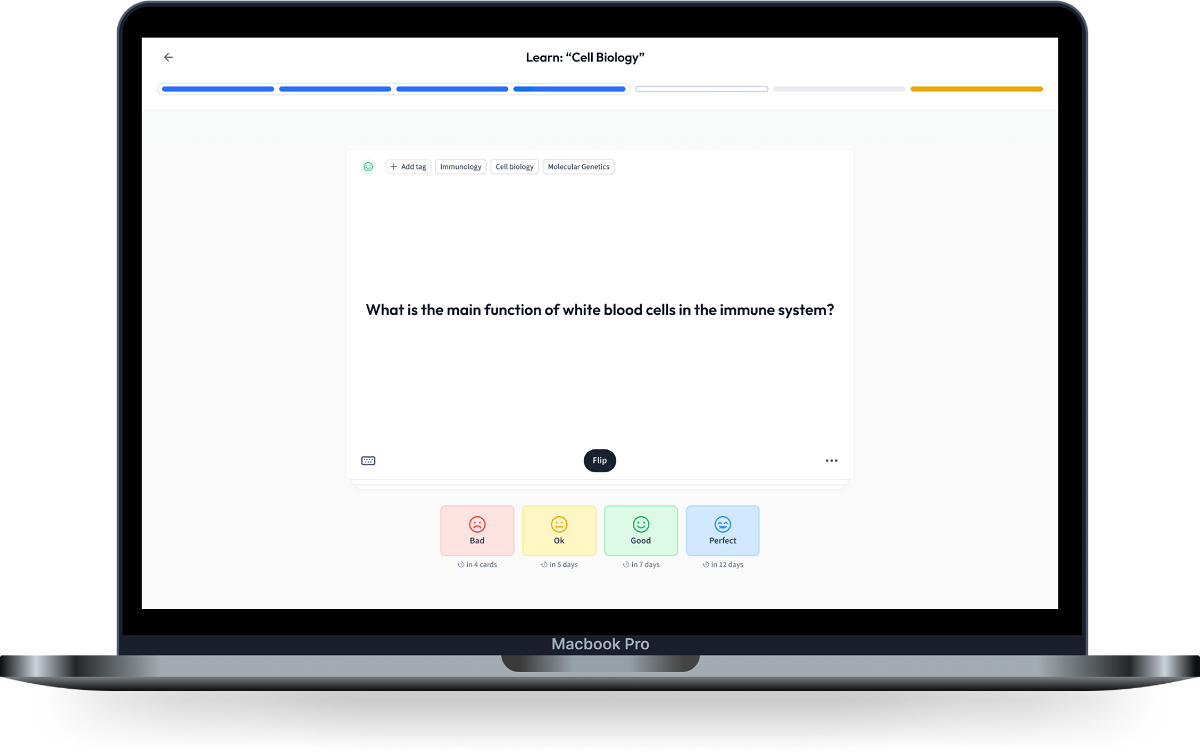
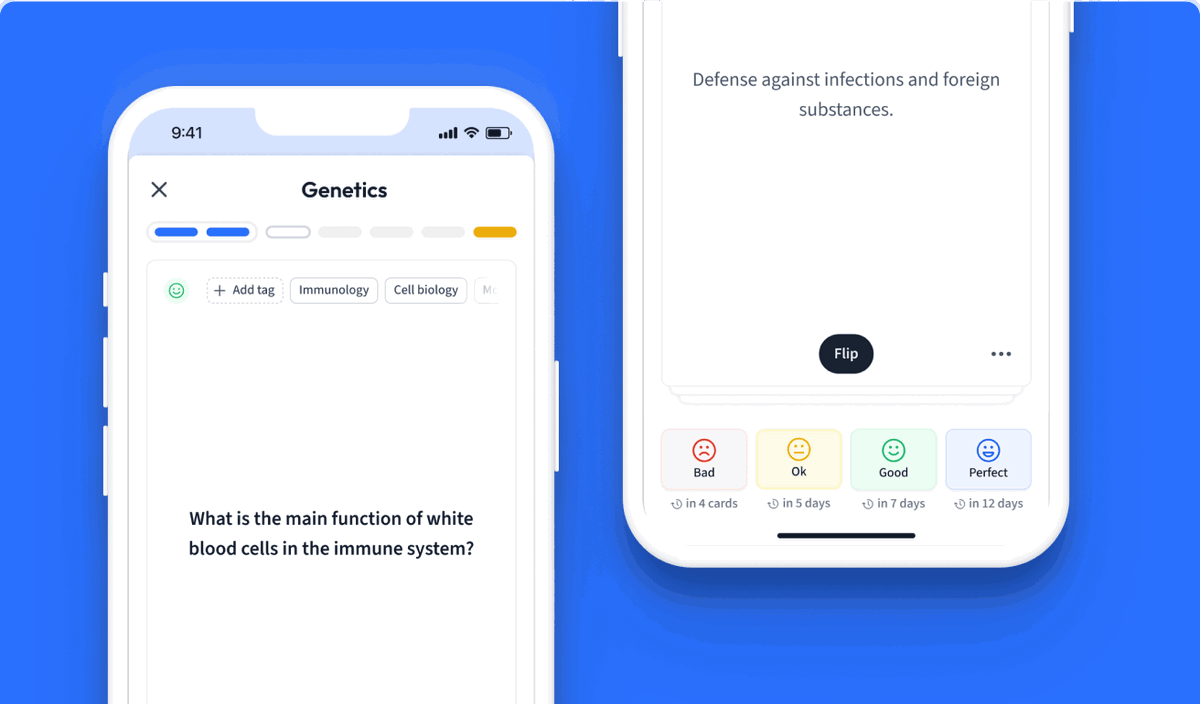
Learn with 15 Java Bitwise Operators flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Java Bitwise Operators
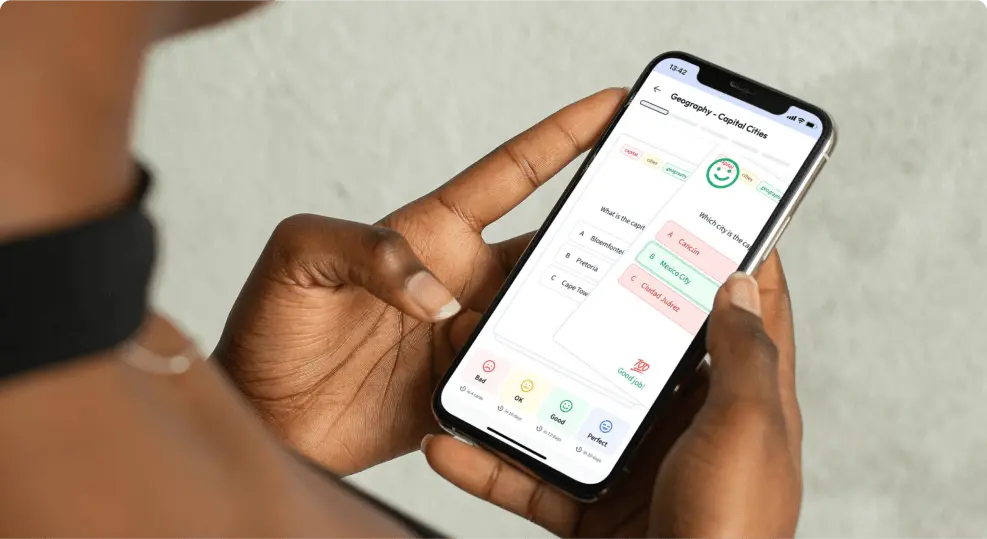
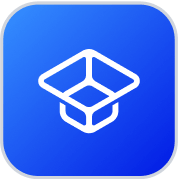
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more