Understanding Javascript Assignment Operators
Javascript assignment operators, at their core, are used to assign values to variables. This is a fundamental aspect of almost any programming language, including Javascript. These operators play a vital role in creating, manipulating and storing the data that makes up your applications.What is an assignment operator in Javascript?
The assignment operator is denoted by the equals sign “=” in Javascript. Essentially, it assigns the value of the right-hand side expression to the variable on the left-hand side.let x = 5;In this example, the assignment operator is assigning the value 5 to the variable x. This operation makes it possible for the variable to hold and store an assigned value.
An assignment operator assigns a value to its left operand based on the value of its right operand.
The role of Assignment Operators in Javascript
Assignment operators are critical in coding as they enable the creation of dynamic applications. They are the foundation for all data translations, manipulations, and operations in Javascript. Without assignment operators, it would be impossible to change or adjust variables, which would, in turn, make applications very rigid and incapable of handling dynamic data input.The various types of Javascript Assignment Operators
While the basic assignment operator is the most commonly used, Javascript also offers a variety of compound assignment operators. These operators provide more efficient, shorter syntax to perform an operation and an assignment in one step.= | Assignment |
+= | Addition assignment |
-= | Subtraction assignment |
*= | Multiplication assignment |
/= | Division assignment |
The use of Compound assignment operators in Javascript
Compound assignment operators both perform an operation and assign a value. They are essentially a shorthand for performing an operation on a variable and then reassigning the result back to the original variable.Consider this sequence:
let x = 5; x = x + 5;Here, you're performing an addition operation on x (x + 5) and then assigning the result back to x. This can be more concisely written using the addition assignment (+=) operator:
let x = 5; x += 5;Both sequences set x to 10, but using the compound assignment operator is more concise.
Javascript logical assignment operators
Logical assignment operators are relatively new addition to Javascript, introduced in ES2021. These operators combine the logical operations of AND (&&), OR (||), and nullish coalescing (??) with assignment.The logical AND (&&) assignment only assigns the value if the original value is truthy, the OR (||) assignment only assigns if the original value is falsy, and the nullish coalescing (??) assignment only assigns if the original value is null or undefined. This can reduce redundant code when conditionally assigning a value.
Practical Application of Javascript Assignment Operators
In programming, theory and practice both are important. It’s not just enough to know about Javascript assignment operators; understanding their practical application is equally vital. Let's see how these operators can be put to use in real-life coding scenarios.Javascript assignment operators example in real-life coding
Let's say you're building a web-based calculator. This calculator needs to perform various mathematical operations. This is where Javascript assignment operators come into play. Consider an operation like addition. Without a compound assignment operator, adding a number to a variable would require first performing the operation and then reassigning the result to the variable. Imagine a situation where a user wants to add 5 to a pre-existing number (10) to get a new number. The typical way to go about could be:let number = 10; number = number + 5; // The variable number now holds the value 15There's nothing wrong with this approach, but it involves writing the variable "number" twice. In scenarios where you're dealing with complicated expressions or long variable names, redundancy can lead to errors and reduce the readability of your code. Compound assignment operators can reduce this redundancy. The example above can be re-written as:
let number = 10; number += 5; // Use of '+=' compound assignment operatorThis code does exactly the same thing as the previous example, but it's simpler and easier to read.
How to use Javascript Assignment Operators effectively
While the basic use of assignment operators is straightforward, some techniques can help you use these operators more effectively in your Javascript code:- Utilise compound assignment operator to reduce redundancy. If you're doing an operation (like addition, subtraction, etc.) and want to assign the result back to original variable, compound assignment operator is an optimal choice.
- Remember that assignments evaluate right-to-left. So, in expressions like `a = b = c = 5;`, all variables will have the value 5.
- Use parentheses to clarify order of operations in complex expressions. This may not involve assignment operators directly, but can help ensure that values are correct before assignment.
- Use logical assignment operators introduced in ES2021 where applicable. They can make your code cleaner and more readable.
What symbol is used as the assignment operator in Javascript
In Javascript, the equal sign (`=`) is used as the assignment operator. This operator assigns the value on the right to the variable on the left. Imagine it as a "store manager" directing "stock" into a "storage bin", with the storage bin representing the variable, the stock representing the value, and the store manager being the assignment operator. The manager’s job is to ensure the right stock goes into the right bin. For example:let storageBin = "Stock";In this line of code, the equals sign (`=`) is the assignment operator, with "storageBin" as the variable and "Stock" as the value to be stored. It's now saved in the "storageBin". This basic assignment operator forms the baseline for other more complex operators in Javascript, like the compound assignment operators (`+=`, `-=`, `*=`, and `/=`) and logical assignment operators (`&&=`, `||=`, and `??=`).
Mastering Javascript Assignment Operators
Just like mastering any skill, mastery in Javascript assignment operators involves more than just understanding their definitions. It requires the knowledge around how to use them effectively, the ability to identify common pitfalls and implementing best practices at all times.Tips and Tricks for Using Javascript Assignment Operators
One of your primary goals when coding should be to keep your code as concise and readable as possible. It aids in debugging, future enhancements and understanding by others. One way to achieve that is through the effective use of Javascript assignment operators. Compound Assignment Operators: Javascript provides a variety of compound assignment operators beyond the basic equals `=`. This includes operators for addition (`+=`), subtraction (`-=`), multiplication (`*=`) and division (`/=`).let x = 10; // Instead of x = x + 5; x += 5;Use these operators for concise expressions. Chaining Assignments: Javascript allows you to chain assignments together, which could be utilised for assigning a single value to multiple variables.
let a, b, c; // Assigns 5 to a, b, and c a = b = c = 5;For debugging purposes, bear in mind that assignment expressions always have the value of the right most expression. Decrement and Increment: When you want to decrease or increase a numeric variable by one, `--` or `++` can be used respectively. Rather than `x += 1` or `x -= 1` you can simply use `x++` or `x--`.
let x = 5; x++; // x is now 6 x--; // x is back to 5This shorthand results in more streamlined code.
Common mistakes to avoid with Javascript Assignment Operators
As powerful as Javascript assignment operators are, it's important to be aware of potential mistakes that could lead to bugs or logic errors: Mistaking `==` for `=`: `==` is a comparison operator, while `=` is an assignment. This is a common mistake that even experienced developers make.let x = 5; if (x = 10) { // This will always evaluate as true // This code will always run because x = 10 is an assignment, not a comparison }Unintended assignments: One pitfall to avoid is unintended assignment in your if statements. Always check that you’re using the comparison operator (`==` or `===`) inside if statements. Assignment versus Equality: `==` performs type coercion, meaning that it converts the variable values to a common type before performing comparison, whereas `===` does not perform type coercion.
let x = 5; console.log(x == '5'); // true console.log(x === '5'); // falseThis is important to keep in mind when evaluating certain conditions in your Javascript code.
Best practices for using Javascript Assignment Operators in Programming
Best practices in any coding language, including Javascript, is vital for maintaining clean, readable code. Consistency: Try to be consistent in your use of assignment operators. If you decide to use compound assignment operators, make sure you use them throughout your code. Code Readability: Opt for clarity and readability over cleverness. It's more important that your code is easy to read and understand than it is to use every shorthand possible. Understanding of concepts: Have a deep understanding of different assignment operators and exactly what they do. This might seem like a no-brainer, but understanding the core concepts can prevent a lot of mistakes, especially in complex programming environments. Evaluate Conditions Properly: Always be careful with conditions. It's easy to write an assignment (`=`) when you actually meant to write a comparison (`==` or `===`). Use linters in your code editor. They can catch many of the potential mistakes that occur with assignment operators. Incorporating these best practices into your programming habits not only helps you write clean and efficient code, but also reduces the likelihood of runtime errors, making your Javascript code more reliable and robust.Javascript Assignment Operators - Key takeaways
- Javascript assignment operators are used to assign values to variables, which is a key aspect of most programming languages.
- The basic assignment operator in Javascript is denoted by the equals sign “=”, which assigns the value of the right-hand side expression to the variable on the left-hand side.
- Compound assignment operators in Javascript, such as +=, -=, *=, and /=, provide a more efficient, shorter syntax to perform an operation and an assignment in one step.
- Javascript logical assignment operators, introduced in ES2021, combine the logical operations of AND (&&), OR (||), and nullish coalescing (??) with assignment.
- Implementing effective use of assignment operators, covering the various types, helps to make code more concise and maintainable and is a key part of mastering Javascript.
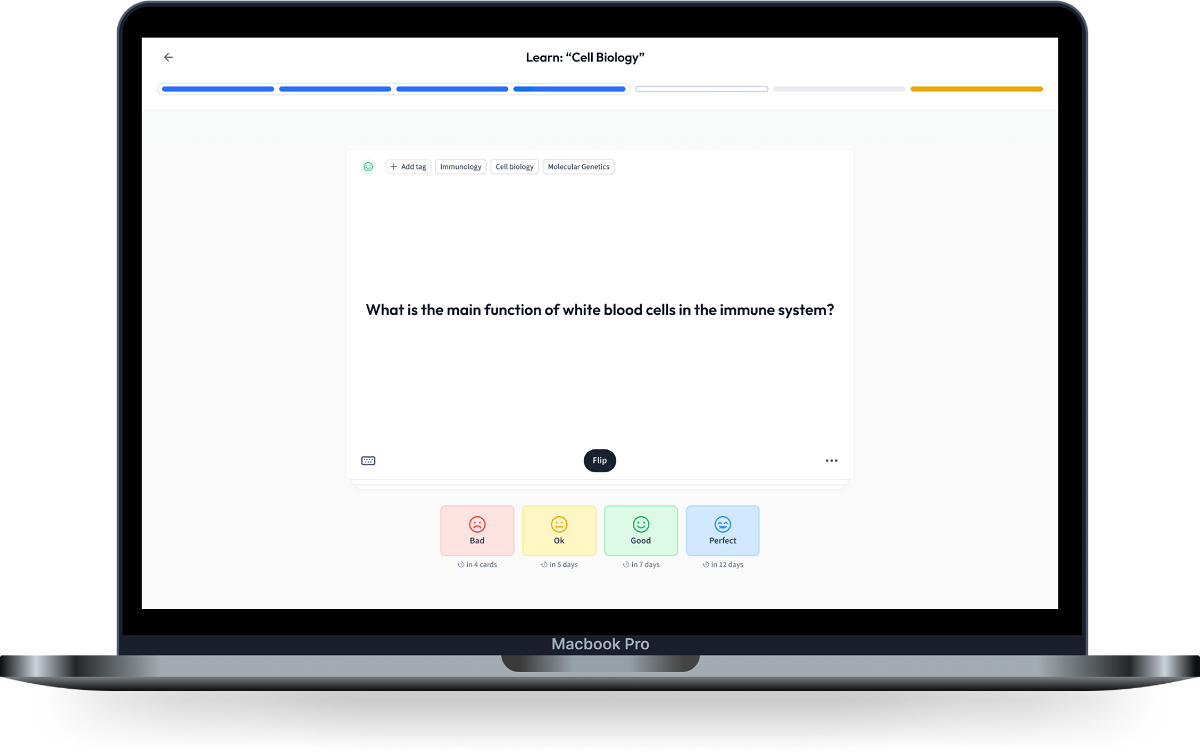
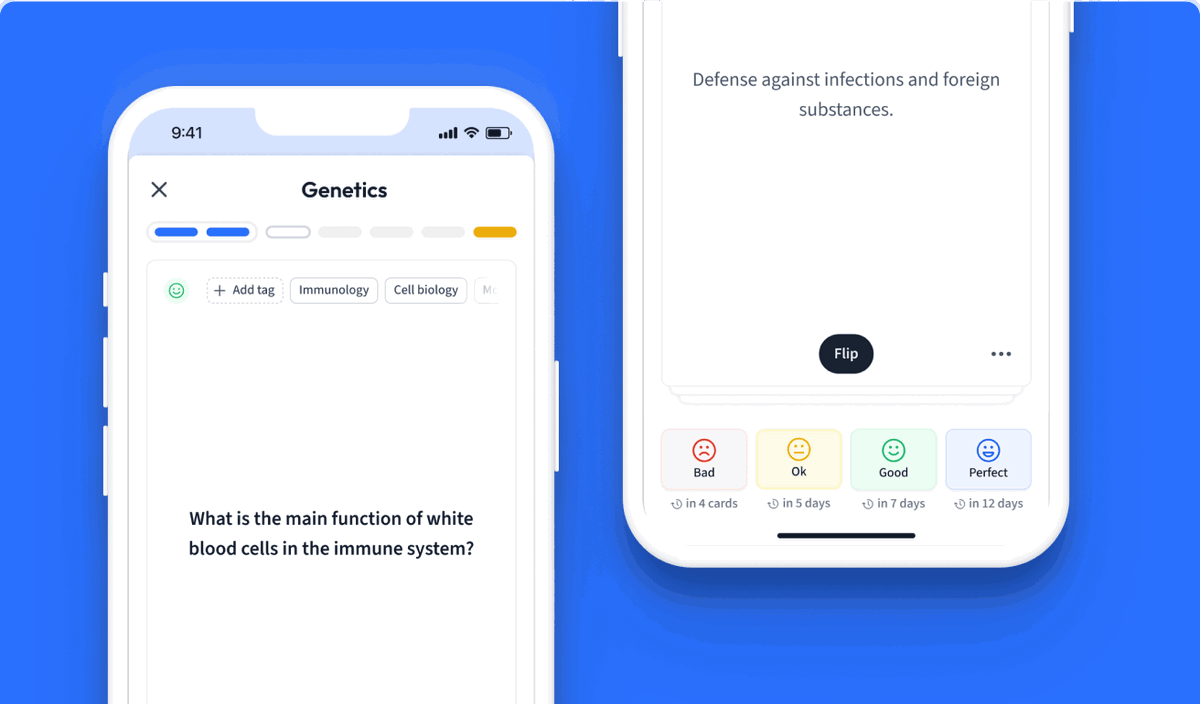
Learn with 12 Javascript Assignment Operators flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Javascript Assignment Operators
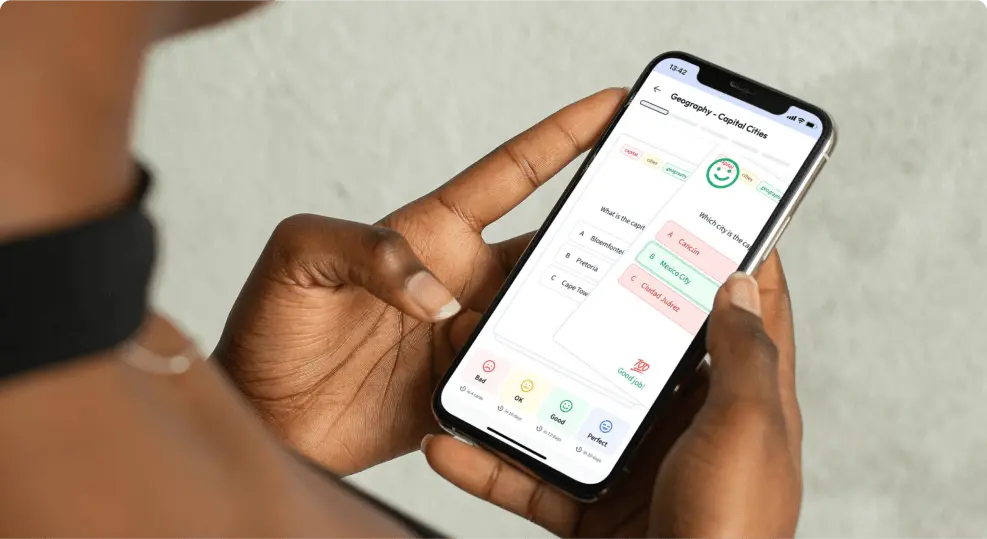
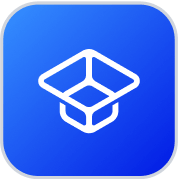
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more