Understanding Java Function
When diving into Java programming, mastering the concept of functions is paramount to crafting efficient and modular code. Functions in Java allow you to encapsulate operations for reuse and organisation, thus enhancing the readability and maintainability of your code. Let’s explore the essence of Java functions, their syntax, types, and see them in action through examples.
What are Java Function
Java Function: A block of code designed to perform a specific task, which can be called by other parts of the program. A function typically has a name, can accept parameters, perform actions, and often returns a value.
Understanding functions in Java is crucial for developing programs that are easy to read and maintain. By dividing the code into smaller, reusable pieces, you make troubleshooting and code management simpler.
Java Function Syntax
The syntax of a Java function involves several components including the return type, function name, parameters, and the body of the function where the actual operations are performed. Here’s a look at the essential elements:
returnType functionName(parameters) { // body }
Every function in Java starts with a return type, which can be any data type, including void
if the function does not return any value. The function name comes next, followed by parentheses containing parameters (if any), and finally, the function body enclosed in curly braces.
Java Function Types
Java supports various types of functions, each serving different needs in the program. Two primary categories are:
- Standard Library Functions: Predefined functions available in Java’s standard libraries, providing common functionalities.
- User-Defined Functions: Functions created by programmers to perform specific tasks not covered by the standard libraries.
Additionally, functions can return values or perform actions without returning anything (void
functions). The latter is especially useful for modifying data or producing side effects.
Java Function Example
public int addNumbers(int a, int b) { return a + b; }
This simple example defines a function named addNumbers
that takes two integers as parameters and returns their sum. It showcases the basic structure and functionality of a Java function.
Exploring Java functions opens up a world of possibilities for modular programming. By understanding function syntax and types, you can begin to build complex programs from simple, reusable pieces. Consider functions as the building blocks of Java programs, each with a specific task but together creating a comprehensive and efficient application.
Diving into Java Functional Interface
Java functional interfaces are a pivotal concept in modern Java programming, especially with the advent of lambda expressions in Java 8. They enable you to achieve cleaner, more readable code and leverage the power of functional programming within an object-oriented context. Let's explore the definition and practical usage of Java functional interfaces.
Defining Java Functional Interface
Java Functional Interface: An interface in Java that contains exactly one abstract method. Despite this limitation, it can contain multiple default and static methods. Functional interfaces are intended for lambda expression implementation.
The @FunctionalInterface
annotation, while not mandatory, is a good practice to ensure an interface meets the functional interface contract.
How to Use Java Functional Interface
Java functional interfaces pave the way for using lambda expressions, making code more concise and readable. Lambda expressions are a means to implement the single abstract method of a functional interface without the boilerplate code of anonymous classes. Here’s how you can use Java functional interfaces:
@FunctionalInterface public interface SimpleFuncInterface { void execute(); } public class Test { public static void main(String[] args) { SimpleFuncInterface sfi = () -> System.out.println("Hello, World!"); sfi.execute(); } }
In this example, SimpleFuncInterface
is a functional interface with a single abstract method execute
. In the main
method, a lambda expression is used to implement execute
, allowing sfi
to run the implemented action with sfi.execute()
.
Functional interfaces in Java aren’t just limited to simplistic scenarios. They are the backbone of Java’s streams API and many other APIs where immutability, statelessness, or concurrency are important. The functional interface pattern encourages you to think in terms of behaviour parameters or callbacks, making it easier to work with code constructs like collections, asynchronous processes, and event listeners in a more expressive and less error-prone way. By understanding and using functional interfaces, you’re embracing a more functional style of programming that can coexist harmoniously with Java’s object-oriented principles.
Exploring Lambda Function in Java
Within the Java programming language, lambda functions represent a powerful way to implement instance of functional interfaces succinctly. They have been introduced in Java 8, significantly improving the brevity and clarity of the code, particularly when dealing with collections or APIs that require interfaces. Let’s embark on a journey to unwrap the mysteries surrounding lambda functions in Java, understand their basics, and how to implement them with practical examples.
Basics of Lambda Function Java
Lambda expressions, or lambda functions as they are often called, provide a clear and concise way to represent one method interface using an expression. They are especially useful in scenarios where a short block of code is to be executed or passed as an argument. Understanding the anatomy of a lambda expression is crucial for anyone looking to harness the power of functional programming in Java.
Lambda expressions are characterised by the following syntax:
(parameter list) -> body
This syntax allows for parameters to be passed to the lambda expression, and the "->" symbol leads to the body of the expression, which contains the actual code to be executed.
Lambda expressions can have zero, one or more parameters, and these parameters do not require specifying the type explicitly; the compiler can infer the types.
Implementing Lambda Function Java in Practical Examples
Implementing lambda functions in Java can greatly simplify your code, making it easier to read and maintain. They are particularly useful when working with Java's Collection framework, where they can be used to filter, map, or reduce elements with minimal effort. Below are practical examples showcasing the use of lambda expressions.
Listnames = Arrays.asList("John", "Jane", "Doe", "Sarah"); names.stream() .filter(name -> name.startsWith("J")) .forEach(System.out::println);
This example demonstrates the use of a lambda expression to filter a list of names starting with the letter 'J'. The filter
method accepts a predicate, which is effectively implemented using a lambda. This makes looping through collections and applying conditions both easy and intuitive.
Aside from collections, Java lambda functions are extensively used in the development of event listeners for GUI applications. They simplify the code needed to implement functionalities on events like clicking a button or selecting an item. For instance, setting an action on a button click in a GUI can be done concisely using lambda expressions. This approach enhances code readability and efficiency, illustrating how lambda functions can be employed beyond collections to improve overall programming paradigms in Java.
Lambda expressions significantly aid in writing instances of single-method interfaces (functional interfaces) more concisely. This feature, combined with Java's Stream API, opens up a wealth of possibilities for processing data in a functional style, an approach that favours immutability and thread-safety, essential characteristics for modern application development.
Mastering String Functions in Java
In Java, string functions are essential tools for manipulating text, allowing developers to perform operations such as searching, comparing, converting, and splitting strings. Understanding these functions is crucial for handling textual data efficiently in any Java application. Let's embark on a comprehensive exploration of Java string functions, covering their overview and the most commonly used ones in practice.
Overview of String Functions in Java
String functions in Java are methods that are available in the String
class, designed to operate on strings. These functions can perform a multitude of tasks that involve string manipulation, making string handling in Java both versatile and powerful. Whether you're formatting user input, parsing data from a file, or constructing dynamic SQL queries, understanding how to use string functions will greatly enhance your programming efficiency and capability.
Commonly Used String Functions in Java
Java provides a considerable number of string functions within the String
class. These functions cater to a wide range of needs, from basic string manipulation to more complex text processing tasks. Below is an overview of some of the most commonly used string functions in Java, including their purpose and how to use them.
- length(): Returns the length of a string.
- charAt(int index): Returns the character at the specified index.
- substring(int beginIndex, int endIndex): Returns a new string that is a substring of the original string, starting from
beginIndex
and extending to the character at indexendIndex - 1
. - toLowerCase(): Converts all the characters in the string to lowercase.
- toUpperCase(): Converts all the characters in the string to uppercase.
- trim(): Removes whitespace from both ends of the string.
- contains(CharSequence s): Returns
true
if and only if the string contains the specified sequence of char values. - replace(char oldChar, char newChar): Returns a new string resulting from replacing all occurrences of
oldChar
in the string withnewChar
.
String greeting = "Hello, World! "; // Example of using trim() to remove whitespace String trimmedGreeting = greeting.trim(); // Example of using toUpperCase() String upperCaseGreeting = trimmedGreeting.toUpperCase(); System.out.println(trimmedGreeting); // Output: "Hello, World!" System.out.println(upperCaseGreeting); // Output: "HELLO, WORLD!"
The examples above illustrate the use of trim()
to eliminate leading and trailing spaces and toUpperCase()
to convert all characters in the string to uppercase. These practical examples highlight the simplicity and power of string functions in Java.
Going beyond basic manipulation, Java string functions also support regular expression-based operations, which are invaluable for pattern matching and text processing tasks. Functions such as matches(String regex)
, replaceAll(String regex, String replacement)
, and split(String regex)
unlock a whole new level of string manipulation by allowing developers to apply powerful regular expression patterns to search, modify, and split strings according to specific rules. Mastering these functions, along with the basics, equips developers to address even the most complex string manipulation challenges with ease.
Java Function - Key takeaways
- Java Function: A code block designed to perform a specific task, which can be called by other program parts, accepts parameters, and often returns a value.
- Java Functional Interface: An interface with exactly one abstract method, used as the basis for lambda expressions in functional programming within Java.
- Lambda Function Java: A concise way to represent one method interface using an expression, enabling simpler and less verbose code, especially beneficial in collections processing.
- String Functions in Java: Methods in the
String
class that allow various text manipulations, such as searching, comparing, and splitting strings. - Examples: Demonstrates Java concepts such as a function to add numbers, usage of functional interfaces and lambda expressions, and manipulation of strings with string functions.
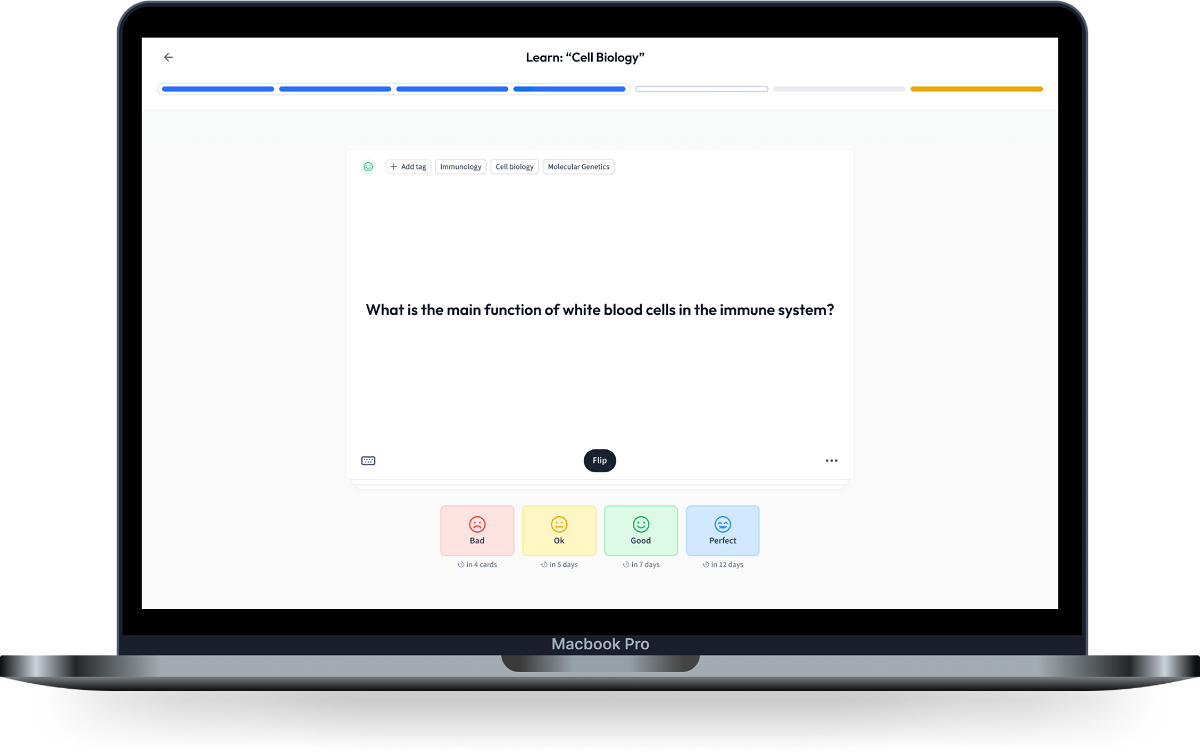
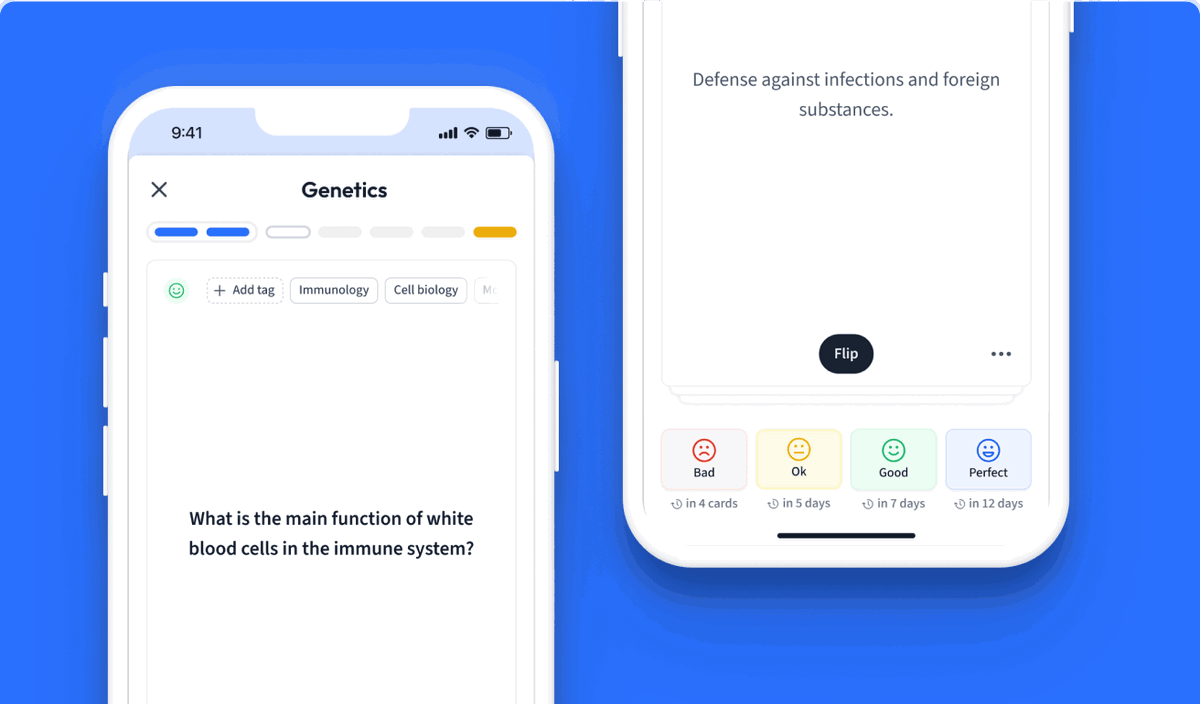
Learn with 63 Java Function flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Java Function
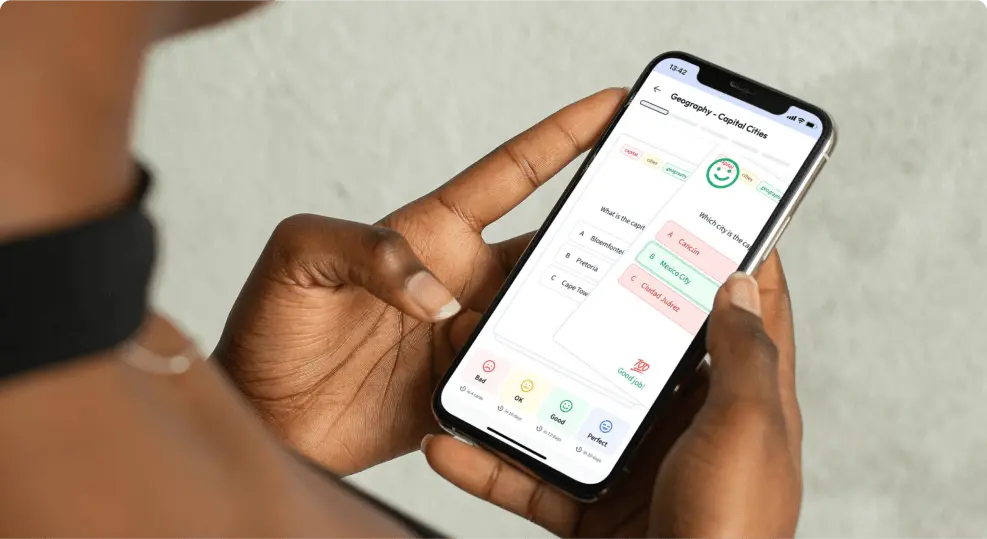
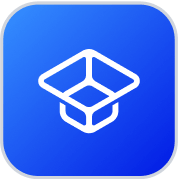
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more