Understanding Java Set Interface
Java, an essential programming language in today's digital landscape, offers various classes and interfaces to help you structure your code. Among the useful offerings is the Java Set Interface - a crucial part of the Java Collections Framework. It brings together many related but unique elements, enabling you to enhance your programming skills effectively.What is Set Interface in Java: The Basics
In the Java Collections Framework, the Set Interface plays a crucial role. Functioning much like a collection, the Set Interface ensures that it contains no duplicate elements. It models a mathematical set abstraction and its collection classes include HashSet, LinkedHashSet, and TreeSet.The Set interface contains methods inherited from the Collection interface and extends the collection framework's functionality.
- add()
- remove()
- contains()
SetIn this example, we create a set of String objects, add a few colours to it, and then clear it of all elements.set = new HashSet<>(); set.add("Red"); set.add("Blue"); set.add("Green"); set.clear();
Core Features of Java Set Interface
Establishing a firm grip on any programming concept involves delving into its core strengths. Here are the key features of the Set Interface in Java, which makes it a tool worthy of mastering:The Java Set Interface has an in-built mechanism to prevent duplicate entries, which is its defining characteristic. This feature sets it apart from its sibling interfaces in the Java Collections Framework, like List and Queue, which allow redundancies.
No duplicates | Ensures that there are no duplicate elements |
Unordered | Doesn't provide guarantees on the order of elements |
Null elements | Allows one null element in the collection |
Thread-safe | Isn't thread-safe in nature except for few implementations like CopyOnWriteArraySet or Collections.synchronizedSet() |
SetIn this example, you try adding '1' twice into the set. Yet, when printing the set content, it will only include each unique number once.numbers = new HashSet<>(); numbers.add(1); numbers.add(2); numbers.add(3); numbers.add(1); System.out.println(numbers);
Exploring Set Interface Methods in Java
Diving deeper into the Java Set Interface requires an understanding of its methods and their uses. The Interface extends the Collection Interface and inherits its methods while adding no new functionalities. However, how these methods perform in the context of the Set Interface's uniqueness feature offers a unique perspective.Key Functions and Uses of Set Interface Methods in Java
An understanding of its commonly used methods is crucial for effective use of the Set Interface in Java. These include- add(E e)
- remove(Object o)
- contains(Object o)
- size()
- isEmpty()
- iterator()
- clear()
SetThis code snippet successfully adds "London" to the set. Attempting to add "London" again will make the add function return false, prompting the conditional clause to display a message.cities = new HashSet<>(); boolean isAdded = cities.add("London"); if(!isAdded) { System.out.println("Element already exists in the set."); }
Java Set Interface Example: Practical Insight
To offer practical insights on using the Java Set Interface, let's create a program that prompts a user to enter names until they wish to stop. Making sure no name is entered more than once:import java.util.*; public class SetExample { public static void main(String[] args) { SetIn the above example, we've employed the Set Interface to keep track of unique names only. The program continuously accepts input until "STOP" is entered. During this, it warns the user if a duplicate name is entered. Once finished, it prints all the unique names entered. The Java Set Interface, with its distinct intrinsic features and inherited methods, shows how the Java Collections Framework provides tools and designs to solve unique programming problems. Applying these tools in your programming practice plays a crucial role in developing concise and efficient code.namesSet = new HashSet<>(); Scanner sc = new Scanner(System.in); String name = ""; while(!name.equals("STOP")) { System.out.println("Enter a name or type 'STOP' to finish:"); name = sc.nextLine(); boolean isAdded = namesSet.add(name); if(!isAdded) { System.out.println("The name already exists in the list."); } } sc.close(); System.out.println("List of unique names:"); for(String s : namesSet) { System.out.println(s); } } }
Distinguishing List and Set Interface in Java
Much of the Java Collections Framework's power derives from its vast and diverse array of tools. Two of these tools, namely the Set Interface and List Interface, might appear similar at first glance. They both belong to the Collection Framework and contain a group of objects. However, they differ in fundamental ways that dictate when to use each effectively.Comparing Characteristics: List vs Java Set Interface
When considering whether to engage the Set or List Interface in your programming, the decision rests upon the data structure's requirements. Despite both storing collections of elements, the interfaces serve unique roles due to their individual characteristics. Key distinguishing features to consider are: Duplicates: A fundamental difference between Java's List and Set Interfaces is their approach to duplicates. Remember, a List can contain duplicate elements, while a Set inherently prevents duplicate entries, guaranteeing the uniqueness of elements. Ordering: The List Interface has a significant feature in that it is an ordered collection. In other words, it maintains the insertion order, which means you can retrieve elements from a List in the order you added them. Conversely, the basic Set Interface is an unordered collection and does not preserve the sequence of element addition. Null Elements: Both the List and Set Interfaces allow one null element. However, be cautious while using sorted sets like TreeSet since they throw a NullPointerException. Here, a comparison is summarised in the form of a table:Aspect | List Interface | Set Interface |
Duplicates | Allowed | Not Allowed |
Ordering | Maintains insertion order | Does not maintain insertion order |
Null Elements | Allowed | Allowed (with exceptions) |
ListIn this code, we try to add "Java" twice to both a List and a Set. However, the size of the List is 2 (counting the duplicate), while the size of Set remains 1 despite the attempt to add a duplicate.list = new ArrayList<>(); list.add("Java"); list.add("Java"); Set set = new HashSet<>(); set.add("Java"); set.add("Java"); System.out.println(list.size()); // Prints 2 System.out.println(set.size()); // Prints 1
Making the Most of List and Set Interface in Java: Practical Tips
Knowing when to use a List or a Set in your projects can drastically impact the efficiency of your programs. Here are some practical tips: 1. Value Uniqueness: When uniqueness of elements is required, a Set becomes your best option due to its inherent duplicate rejection. Conversely, when multiplicities matter, rely on a List. 2. Data Access: If you are frequently accessing elements from the collections, consider Lists. They provide positional access and a wider range of methods to manipulate elements. 3. Maintaining Order: If it's critical to keep the order of input, Lists come into play. But remember, if you need a sorted Set, TreeSet offers a sorted version despite being a type of Set. 4. Computational Efficiency: HashSet operations have constant time complexity for the basic operations (add, remove, contains and size). Use Set when you need to perform these operations frequently and the collection size is large. In conclusion, effective Java programming involves a comprehensive understanding of the various tools at your disposal, including knowing when it's best to use a Set or a List.Implementing Set Interface in Java Collections
To manipulate groups of objects as a single unit, Java offers a powerful tool known as the Collection Framework. Within this framework, the Set Interface hosts a distinctive role. By design, the Set Interface maintains no specific order of elements and rejects duplicate entries. This makes it an ideal toolset when handling collections requiring element uniqueness.Mastering the Implementation of Set Interface in Java Collections
The most common implementations of the Set Interface in the Java Collection Framework are the HashSet, TreeSet, and LinkedHashSet.- HashSet: It's the most frequently used Set Interface implementation. However, it doesn't guarantee any order of elements. Especially suited for quick search operations, its computational complexity for fundamental operations such as add, remove, and contains is approximately \(O(1)\).
- TreeSet: To keep elements in a sorted order, TreeSet is used. It implements the NavigableSet Interface, which extends the SortedSet Interface. It's less preferred for operations that require time efficiency due to \(O(\log(n))\) time complexity. Nonetheless, it ensures the elements stay sorted, which might be necessary in certain circumstances.
- LinkedHashSet: This strikes a balance between HashSet's performance benefits and the ordered nature of the TreeSet. It maintains a LinkedList of the entries providing predictable iteration order, which is typically the insertion order. While it's slightly slower than HashSet due to linkage maintenance, it still offers considerably good performance.
import java.util.HashSet; HashSetHere, you have created a HashSet, added elements to it using the add() method, and checked for an element's presence using contains(). To create a TreeSet or LinkedHashSet, replace the HashSet keyword accordingly. Observe the ordering of elements in the different Set Interface implementations by printing them out.set = new HashSet<>(); set.add("Apple"); set.add("Banana"); set.add("Cherry"); System.out.println(set.contains("Banana")); // Prints true
Examples of Java Collections Using Set Interface
The power of the Set Interface becomes apparent only when used to solve real-world problems. Consider an application required to catalogue various unique books in a library. Each book has unique ISBN, making the Set Interface an ideal candidate for this job.import java.util.HashSet; class Book { String isbn; String title; // constructors, getters and setters omitted for brevity } HashSetHere, despite an attempt to add the book "Design Patterns" twice, the size of the Set remains 2, demonstrating the Set Interface's uniqueness aspect. For a scenario needing to maintain the order of addition (for example, registration queues), LinkedHashSet fits perfectly:library = new HashSet<>(); library.add(new Book("978-0201633610", "Design Patterns")); library.add(new Book("978-0590353403", "Harry Potter and the Sorcerer's Stone")); library.add(new Book("978-0201633610", "Design Patterns")); System.out.println(library.size()); // Prints 2
import java.util.LinkedHashSet; LinkedHashSetUsing a LinkedHashSet, the order of addition remains, and "Alice" is present only once. Indeed, the Set Interface in Java Collections Framework provides a robust toolkit to handle group elements effectively. Its implementations - HashSet, TreeSet, and LinkedHashSet, with their unique traits, pave the way for clean and efficient solutions to diverse programming challenges.registrationQueue = new LinkedHashSet<>(); registrationQueue.add("Alice"); registrationQueue.add("Bob"); registrationQueue.add("Charlie"); registrationQueue.add("Alice"); System.out.println(registrationQueue.toString()); // Prints [Alice, Bob, Charlie]
How Does Java Set Interface Work: Unravelling the Process
To appreciate the inner workings of the Set Interface in Java, it's crucial to consider its place within the Java Collections Framework. More so, the interface inherits the Collection interface. The Set Interface, by design, is a mathematical model of a Set: a collection of unique elements, no repetitions allowed.Developing Understanding: How does Java Set Interface Function
- Uniqueness of Elements: Being a set, this interface inherently rejects duplicate values. Think of it as a bundle of unique items, much like unique ISBNs of books or individual ID numbers. The interface employs a method named .equals() to verify if an object is a duplicate.
- Regular Methods: The Set Interface features the same methods as the Collection interface, including add, remove, clear, contains, and size. Each is used for manipulation and retrieval of items.
- Static Methods: Since Java 8, four new static methods have been added: copyOf, of, sorted, and unordered. Notably, the of() and copyOf() methods were added in Java 9 and are used for creating an unmodifiable set in an efficient way.
- Null Element: The Set Interface generally allows a null element. However, in the case of class implementations like TreeSet, null values are not permitted.
HashSet | TreeSet | LinkedHashSet | |
Ordering | Unordered | Sorted | Preserves insertion |
Null Elements | Allowed | Not Allowed | Allowed |
Practical Demonstration: Operational Mechanism of Java Set Interface
To better understand the functioning of the Set Interface, let's examine code snippets featuring its use:import java.util.*; public class Main { public static void main(String[] args) { SetIn this example, you are adding four pieces of fruit to three types of Set: HashSet, TreeSet, and LinkedHashSet. Despite attempting to insert "Apple" twice, the duplicates are eliminated in each case as a characteristic of the Set Interface. However, observe how the three Sets display the elements in different orders when printed..HashSet shows unordered entities while TreeSet has sorted them, even though you added them in the same order. LinkedHashSet preserves this insertion order. The operational mechanism of the Java Set Interface and its implementation classes is quite efficient due to sophisticated algorithms and data structures, such as HashTable in HashSet and TreeMap in TreeSet. With these tools in a programmer's toolkit, tackling more complex data tasks becomes a streamlined process. However, to capitalise successfully on these tools, a thorough understanding of their functionality and applications is indispensable.set1 = new HashSet<>(); Set set2 = new TreeSet<>(); Set set3 = new LinkedHashSet<>(); for (String fruit : new String[]{"Apple", "Banana", "Cherry", "Apple"}) { set1.add(fruit); set2.add(fruit); set3.add(fruit); } System.out.println(set1); // [Banana, Apple, Cherry] System.out.println(set2); // [Apple, Banana, Cherry] System.out.println(set3); // [Apple, Banana, Cherry] } }
Java Set Interface - Key takeaways
- The Java Set Interface doesn't allow duplicate elements. This ensures the uniqueness of each element in a collection.
- The Set Interface doesn't provide an ordered collection, due to it being an unordered collection. It does not maintain any index-based collection order.
- The Java Set Interface allows one null element in the collection.
- The Set Interface isn't thread-safe by default, but it can be made thread-safe with certain implementations like CopyOnWriteArraySet or Collections.synchronizedSet().
- Common Set Interface methods in Java include add(E e), remove(Object o), contains(Object o), size(), isEmpty(), iterator(), and clear(). These methods are inherited from the Collection Interface, allowing for manipulation of the Set Interface.
- Key differences between the Java List and Set Interfaces include: handling duplicates (List allows, Set rejects), ordering of elements (List maintains insertion order, Set does not), and null elements handling (both allow one null element, but sorted sets like TreeSet may throw NullPointerException).
- Common implementations of the Set Interface in Java Collections Framework include HashSet, TreeSet, and LinkedHashSet. Each has unique features; HashSet for quick search operations, TreeSet for sorted order of elements, and LinkedHashSet as a balance of performance benefits and order maintenance.
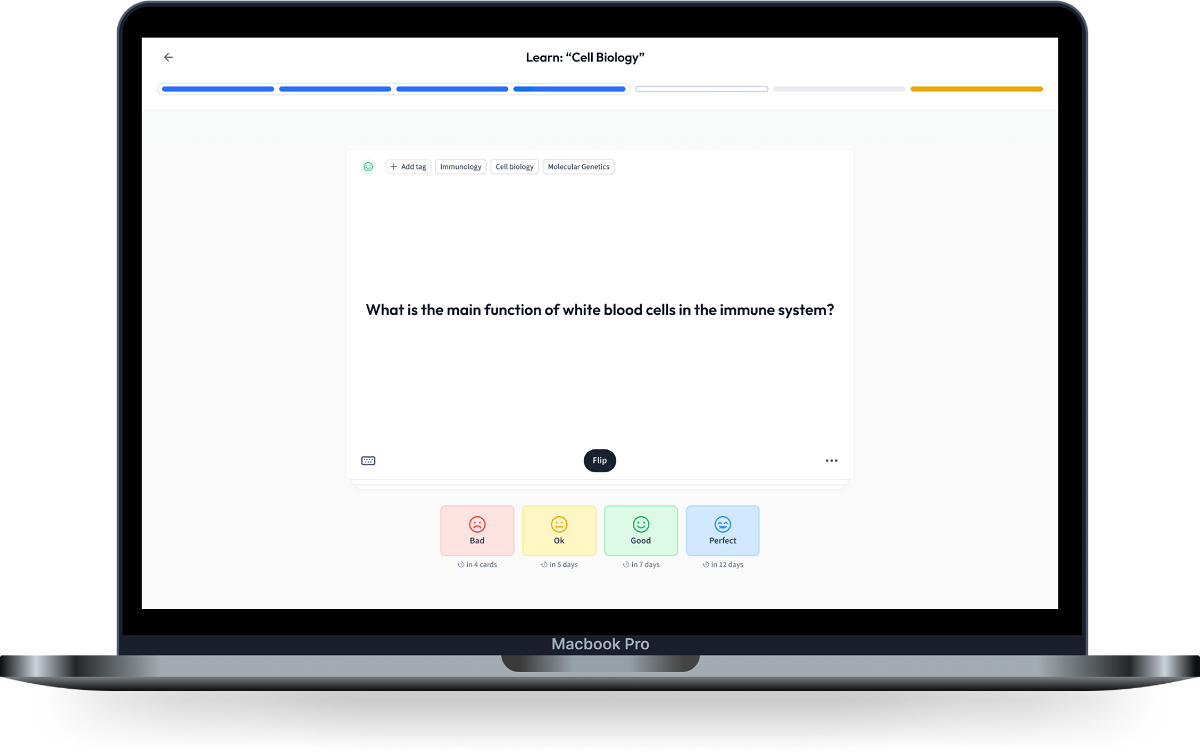
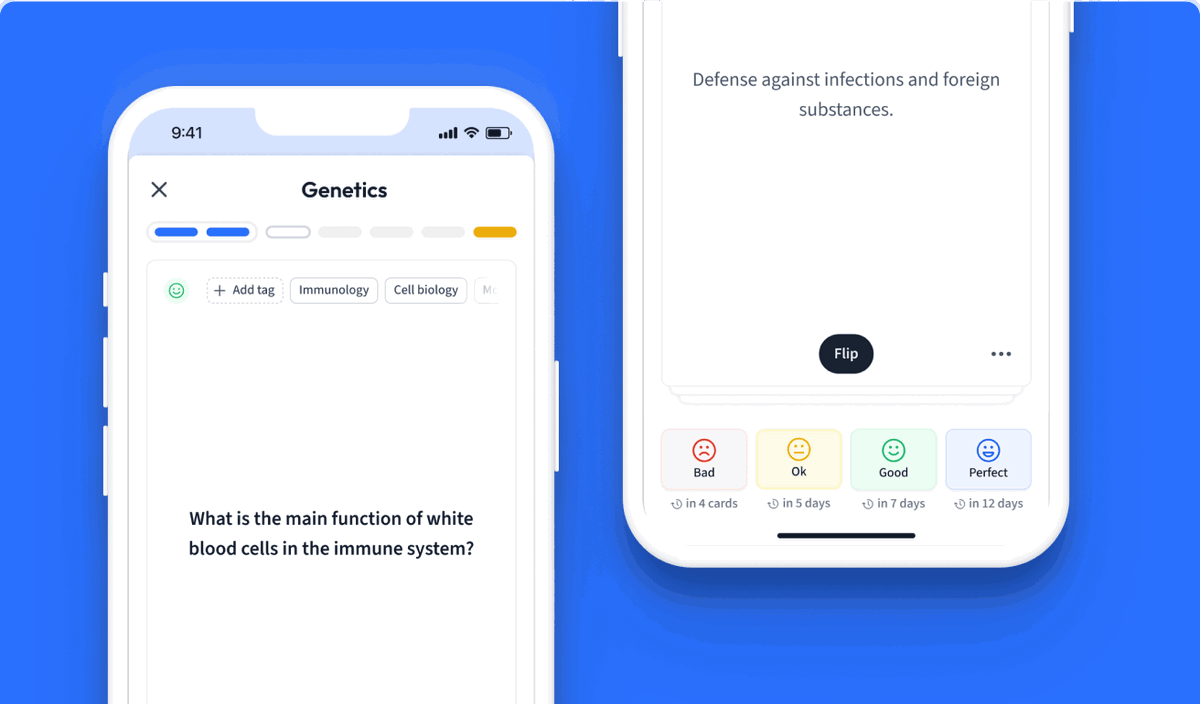
Learn with 15 Java Set Interface flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Java Set Interface
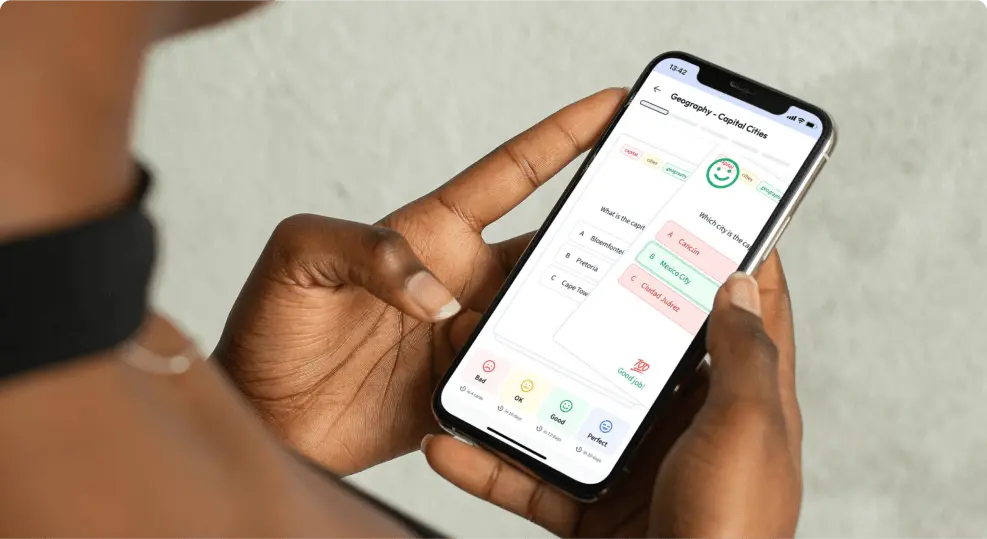
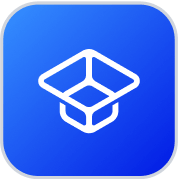
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more