Understanding Java Arithmetic Operators
Being a fundamental concept in Java programming, arithmetic operators have a significant part in performing mathematical operations such as addition, subtraction, multiplication, etc. They make computing easy, contributing immensely to the dynamics of programming.Definition and Importance of Java Arithmetic Operators
Java Arithmetic Operators refer to the special symbols in Java code that are used to perform mathematical operations like addition, subtraction, multiplication, and division. With the use of Java Arithmetic Operators, you can perform mathematical operations using variables and values in your code.
As they are fundamental elements in any programming language, they are used in an array of situations. For example, arithmetic operators can help to calculate the average of test scores, determining the speed of an object, or simply adding two numbers. Here are the main arithmetic operators in Java:- Addition (+)
- Subtraction (-)
- Multiplication (*)
- Division (/)
- Remainder (%)
Did you know? Java Arithmetic Operators not only perform calculations on integers, but they can also operate on non-integer numerical data types such as floats and doubles.
How Java Arithmetic Operators Work
Java Arithmetic Operators operate in similar ways as per the math operations in algebra. For instance, the plus (+) operator adds two values together, while the minus (-) operator subtracts one value from the other. A good way to understand how they work is by referring to this table:Operator | Description | Example |
+ | Adds two values | a + b |
- | Subtracts second value from first | a - b |
* | Multiplies two values | a * b |
/ | Divides first value by second | a / b |
% | Finds the remainder of division of two values | a % b |
Basic Principles of Java Arithmetic Operators Technique
Java Arithmetic Operators follow the order of operations as we do normally in Mathematics, that order being Parentheses, Exponents, Multiplication and Division (from left to right), Addition and Subtraction (from left to right). This rule is commonly known as PEMDAS.Text Definition: PEMDAS stands for Parentheses, Exponents, Multiplication and Division, Addition, and Subtraction. It is the order of operations in mathematics and programming.
Techniques to Use Java Arithmetic Operators Effectively
Using Java Arithmetic Operators effectively requires understanding their priorities, managing brackets' usage, and handling exceptions. For example, whenever dividing by zero, Java throws an exception. Thus, when using the division (/) operator, ensure the denominator is not zero. Let's illustrate that with this code snippet:public class Main { public static void main(String[] args) { int a = 10; int b = 5; int output; output = a + b; System.out.println("The sum is: " + output); output = a - b; System.out.println("The difference is: " + output); output = a * b; System.out.println("The product is: " + output); if( b != 0) { output = a / b; System.out.println("The quotient is: " + output); } else { System.out.println("Math error: Division by zero"); } } }In the preceding code, the arithmetic operators have been used to perform common mathematical operations. Also, notice the use of an 'if' statement to prevent division by zero. Following this technique helps prevent unexpected program interruption.
Arithmetic Operations Unraveled with Java Examples
Java, as you may well know, is filled to the brim with functions to complete arithmetic tasks effectively. In a world where numbers rule, arithmetic operations become an irreplaceable tool. Java examples aid in the deeper understanding and effectiveness of these functions.A Closer Look at Various Arithmetic Operations in Java Examples
Java's arithmetic operations can handle all sorts of numeric calculations. Each operation has a unique role in modifying, comparing, or creating new values.Text Definition: Arithmetic Operations in Java include addition, subtraction, multiplication, division and modulus (to get the remainder). These are symbolised as '+', '-', '*', '/', and '%', respectively.
public class Main { public static void main(String[] args) { int a = 6, b = 2, output; output = a + b; System.out.println("a + b = " + output); output = a - b; System.out.println("a - b = " + output); output = a * b; System.out.println("a * b = " + output); output = a / b; System.out.println("a / b = " + output); output = a % b; System.out.println("a % b = " + output); } }In the above-mentioned java code, operations are performed on the variables 'a' and 'b'.
Functions and Uses of Different Arithmetic Operations in Java
One might argue, where and how do these arithmetic operations play their roles in programming? Well, the uses are more ubiquitous than you might think. Arithmetic operations power computations across virtually every Java program. Without them, it would be impossible to calculate a running score in a game, compute an average grade for a student, or even generate simple animations. For instance, here are some practical applications of arithmetic operations: - Addition: Most commonly used operation. For instance, increasing a game's score, adding items to a cart, creating a summing algorithm, etc. - Subtraction: Used to determine differences, such as how much more one number is than another, calculating remaining days, decreasing score, etc. - Multiplication: Perfect for scaling values, doubling a score, increasing a player's speed, or simply multiplying numbers. - Division: Good for splitting things equally. For example, distributing tasks amongst threads evenly, calculating an average, converting units, etc. - Modulus: Best used to determine if one number is divisible by another, like checking for odd and even numbers, cycling through array indices, etc.Text Example: Let's consider a simple game where the player score doubles every level. Here, the multiplication arithmetic operator can be used to calculate the player's score at each level. When the level changes, you can simply multiply the current score by 2 to get the new score. For example:
int currentScore = 50; int level = 2; int newScore; newScore = currentScore * level; System.out.println("New Score is: " + newScore);
In this case, the new score will be 100.
Exploring Java Program for Arithmetic Operations
In every programming language, arithmetic operators are the building blocks to developing any functional code. They facilitate the core mathematical functions such as addition, subtraction, multiplication, and division. Particularly in Java, these arithmetic operators allow you to create dynamic and complex calculations within your code.Step-by-step Approach to Building a Java Program for Arithmetic Operations
Creating a Java Program for arithmetic operations involves a series of systematic steps. Here's a step-by-step guide: Step 1 - Setting Up the Environment: First, ensure that you have the Java Development Kit (JDK) installed on your computer. This kit includes the runtime environment and the tools you need to compile and run your Java programs. Step 2 - Creating a New Java Class: Once you have JDK installed, create a new Java class where your code will reside. To do this, open your Java Integrated Development Environment (IDE), and create a new Java file. You may name it as you deem fit, bearing in mind that the name should reflect the purpose of the class. Step 3 - Defining Variables: Ideally, the next step should be to define the variables which will be used in the program.int a = 15; int b = 5;Step 4 - Performing Arithmetic Operations: You can now proceed to perform arithmetic operations on these variables in the main method. The main method is where your program begins running.
public static void main(String[] args) { int a = 15; int b = 5; int sum = a + b; int diff = a - b; int prod = a * b; int quot = a / b; int rem = a % b; }Step 5 - Display Results: Lastly, display your results using the System.out.println() command.
Understanding the Structure of Java Program for Arithmetic Operations
Understanding the structure of a Java program for arithmetic operations is crucial in comprehending your code. Here, we examine each part of the program. Main Class: At the top of the program is the main class. In Java, every application must contain a main class that wraps up all the other classes. Always remember to name your main class in a way that reflects the goal of your program for easy reference.public class Main { }Main Method: Within the main class, you embed the main method. The main method serves as the application's entry point. The Java Virtual Machine (JVM) calls the main method when the program starts. The main method must always be declared public, static, and void.
public static void main(String[] args) { }Variable Declaration: Next, the program defines two integer variables, a and b, inside the main method. These variables are the operands upon which operations are performed.
int a = 15; int b = 5;Arithmetic Operations: Following this, the Java program code performs arithmetic operations using the variables a and b. Each operation is stored in a separate variable (sum, diff, prod, quot, and rem).
int sum = a + b; int diff = a - b; int prod = a * b; int quot = a / b; int rem = a % b;Print Output: Lastly, the program prints the results of the arithmetic operations to the console using System.out.println(). This method prints the arguments passed to it and then terminates the line.
System.out.println("The sum is " + sum); System.out.println("The difference is " + diff); System.out.println("The product is " + prod); System.out.println("The quotient is " + quot); System.out.println("The remainder is " + rem);To run the program, simply press the run button in your IDE. It will compile and execute your program, displaying the results in the output console. This way, you have successfully built and understood a simple Java program that effectively performs arithmetic operations.
Application of Arithmetic Operations using Switch Case in Java
Java offers an array of control flow statements that can be utilised to improve the logic of the program. Building a program utilising arithmetic operations and the switch case control flow statement portrays a common, yet incredibly beneficial application in Java. It's an excellent way to build a calculator or perform different operations depending on user input.Getting Started with Arithmetic Operations using Switch Case in Java
The switch case statement is essentially a multi-way branch - it’s an efficient alternative to a lengthy series of if...else statements. The switch keyword is followed by an expression that returns an integer or character value. Switch cases in Java function as follows: 1. The switch expression is evaluated once. 2. The value of the expression is compared with the values of each case. 3. If there is a match, the associated block of code is executed. 4. If there is no match, the default code block is executed. In the context of arithmetic operations, the concept is to create a switch case statement that prompts the user to input two numbers and to choose an operator. Depending on the operator chosen, the output will be the result of the applied arithmetic operation on the two number inputs. To start, you would need to import the Scanner class, which is used for capturing the user input in Java. Here's how to do it:import java.util.Scanner;Next, declare integer variables to hold the numbers and a character variable to hold the operator:
int num1, num2; char operator;For instance, let's create a simple calculator. Use a Scanner to get user input like this:
Scanner scanner = new Scanner(System.in); num1 = scanner.nextInt(); operator = scanner.next().charAt(0); num2 = scanner.nextInt();Finally, you can utilise the switch case statement to perform different arithmetic operations depending on the operator chosen:
switch(operator) { case '+': System.out.println(num1 + num2); break; case '-': System.out.println(num1 - num2); break; case '*': System.out.println(num1 * num2); break; case '/': System.out.println(num1 / num2); break; default: System.out.println("Invalid operator!"); break; }
Common Cases and Purposes of Arithmetic Operations using Switch Case in Java
Applying the switch case statement to arithmetic operations is especially useful in scenarios where you have multiple possible outcomes. Here are a few common instances and purposes: - Calculator applications: As shown above, a beginner's calculator program can be one of the best demonstrations for implementing switch case arithmetic operations. - Menu-driven programs: For applications with multiple options available to a user, switch case with arithmetic operations can assist in navigating and selecting the different functions. - Game Programming: In games where on-screen entities have different behaviours based on certain conditions, you can use a switch statement to perform different calculations representing the behaviour patterns. - Data Analysis: When analysing data, switch case statements can quickly assess and manipulate data based on certain conditions, performing relevant calculations. There's no end to the possibilities where arithmetic operations embedded within switch case statements can be utilised. Below is a table that provides some examples of where these can be put into use:Calculator Apps | Add, subtract, multiply, or divide user-entered numbers |
Menu-Driven Programs | Choose to perform calculations from a set of options |
Data Analysis | Performing relevant calculations based on requirements |
Game Programming | Trigger certain events or behaviours based on game conditions |
Delving into Precedence of Arithmetic Operators in Java
Operator precedence determines the grouping of terms in an expression, which in turn affects how an expression is evaluated in Java. Arithmetic operators in Java have a pre-determined order of precedence that helps avoid ambiguity in expressions.What is Precedence and Why is it Important in Java Arithmetic Operations?
In mathematics and most programming languages, precedence (also known as operator precedence) is a collection of rules that define the sequence in which operations are carried out in an expression, particularly when different operations occur sequentially. For instance, in the expression 7 + 3 * 2, it's not immediately clear if the operation to be performed first is the addition or multiplication. Here, precedence steps in. According to the precedence rules of arithmetic operators, multiplication is performed before addition. Hence, the value of the expression would be 13, not 20. So, why is precedence important in Java arithmetic operations? The answer is simple: 1. Eliminate Ambiguity: Operator precedence helps in avoiding ambiguity by providing clear rules for performing operations. 2. Implicit Parentheses: It can be thought of as surrounding parts of the expressions with implicit parentheses. 3. Ensure Comparability: It ensures that expressions and equations remain comparable across different contexts, aiding consistency and readability. The exact order of precedence can differ slightly between different languages. That said, within a particular language like Java, it remains constant and is defined by the language’s own specifications. In Java, arithmetic operators follow the precedence order as shown below: 1. Parentheses 2. Unary operators 3. Multiplication, division, and modulo 4. Addition and subtractionApplying the Rule of Precedence in Java Arithmetic Operators
Applying the rule of precedence in Java arithmetic operations involves following the sequence: parentheses always have the highest priority, followed by unary operators, multiplicative operators, and finally, additive operators. Here is a short example:int result = 10 + 2 * 3;In the above code, you might expect that operations are carried out from left to right, and 'result' would be 36 (12 multiplied by 3). However, considering the rule of precedence, the multiplication happens first, so 'result' would be 16 (2 multiplied by 3, plus 10). You can alter the default precedence by using parentheses. When parentheses are used, operations enclosed in parentheses are executed first. For instance, in the expression:
int result = (10 + 2) * 3;Here, due to the parentheses, the addition operation is carried out first, and 'result' would indeed be 36. Understanding and applying the rule of precedence can avoid confusion and make your code more readable and consistent. It's one aspect that can seem trivial, but getting it wrong can lead to a whole range of unexpected behaviours in your programs. Always take note of operator precedence when performing operations in your code. It may save you from mathematical inaccuracies, and it's a good habit that can benefit your coding skills in the longer term.
Java Arithmetic Operators - Key takeaways
- Java Arithmetic Operators are used to perform arithmetic operations such as addition (+), subtraction (-), multiplication (*), division (/), and modulus (%), which returns the remainder after division.
- To use Java Arithmetic Operators effectively, understand their priorities, manage brackets usage, and handle exceptions. For example, when dividing by zero, Java throws an exception, thus, always ensure the denominator is not zero.
- In programming, arithmetic operations are ubiquitous, powering computations across virtually every Java program. They are used for tasks like calculating a running score in a game, computing an average grade for a student or generating simple animations.
- Creating a Java Program for arithmetic operations involves several steps: setting up the environment, creating a new Java Class, defining variables, performing arithmetic operations, and displaying results. Always ensure the main method is declared as public, static, and void.
- Using the switch case statement in conjunction with arithmetic operations in Java can be beneficial for applications like calculator programs, menu-driven programs, game programming, and data analysis. The switch case statement is a multi-way branch and an efficient alternative to a lengthy series of if...else statements.
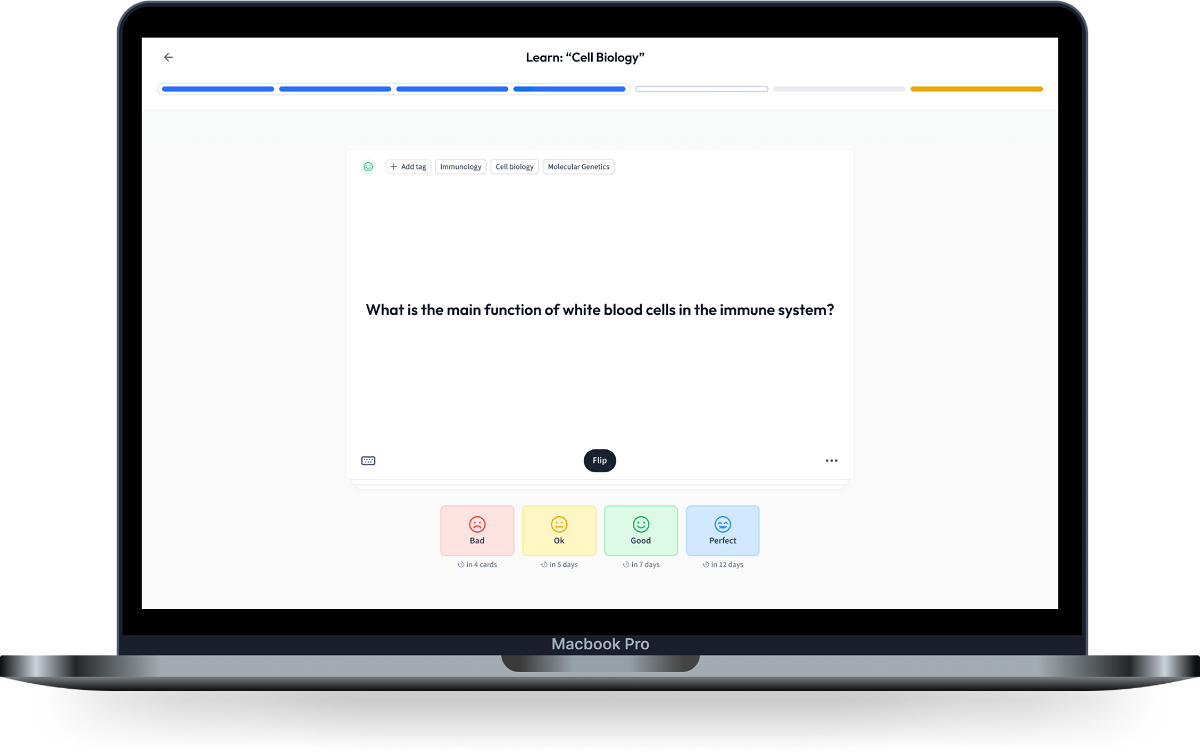
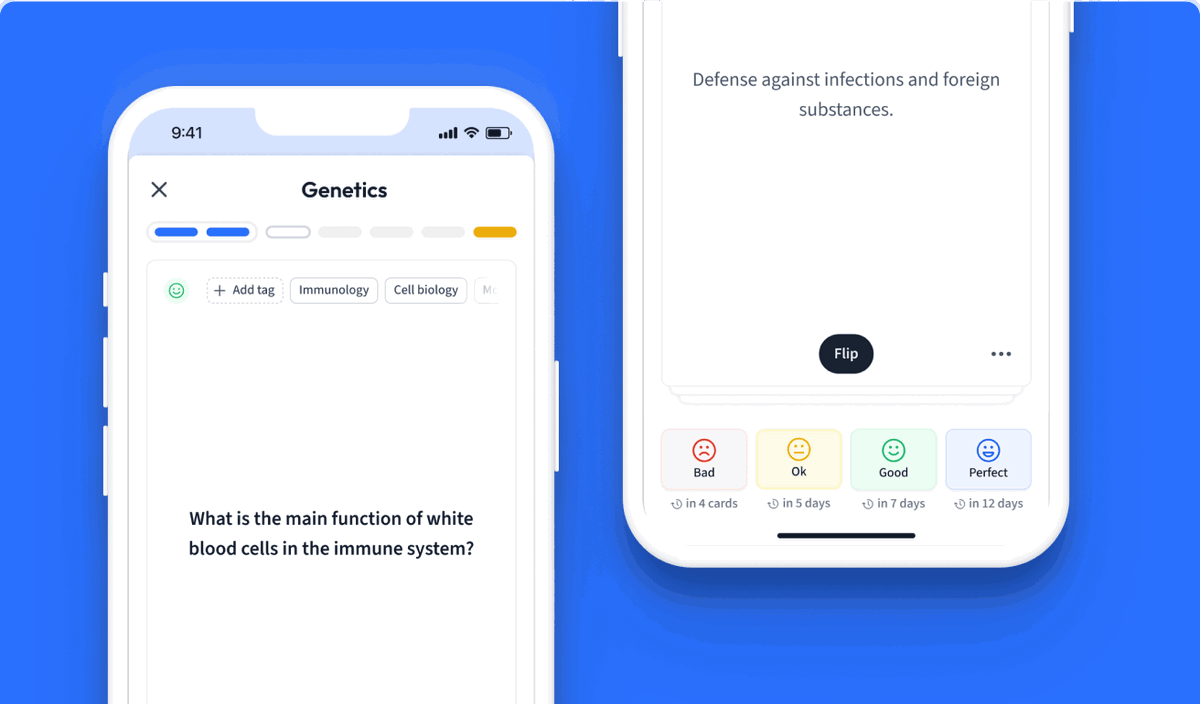
Learn with 15 Java Arithmetic Operators flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Java Arithmetic Operators
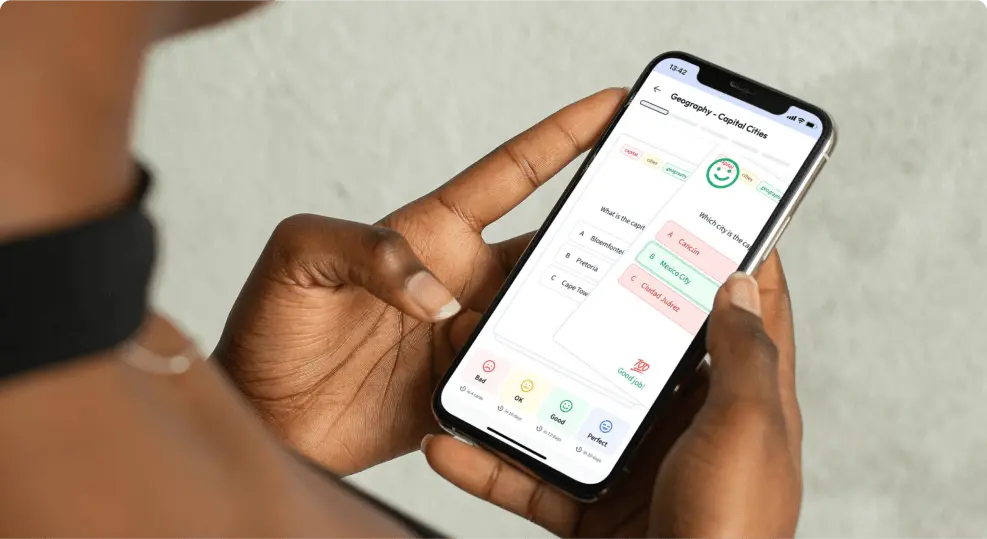
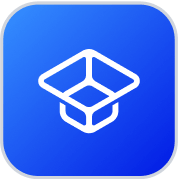
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more