What are Arrays in Javascript?
When delving into the world of Javascript, understanding the concept of arrays is fundamental. Arrays are versatile tools that allow you to store multiple values in a single, organised structure, making data manipulation and retrieval both efficient and straightforward.
Defining Javascript Arrays
In Javascript, an array is a single, complex data type that allows for the storage of multiple values. Think of it as a container that holds items, where each item can be of any Javascript data type, such as numbers, strings, or even other arrays.The unique aspect of arrays is their ability to store different types of values simultaneously, providing a flexible means to group related data together.
Javascript Array: An ordered collection of values, which may include various data types like numbers, strings, or other arrays, and accessible by an index.
const fruits = ['Apple', 'Banana', 'Cherry'];This example demonstrates a simple Javascript array storing the names of three fruits. Each fruit is an element in the array and can be accessed using an index.
Key features of Javascript Arrays
Javascript arrays come packed with several key features that make them an indispensable tool for developers. Here are some of the most notable features:
- Dynamic Size: Unlike in some other programming languages, the size of a Javascript array can change dynamically. This means elements can be added or removed at runtime, providing incredible flexibility in managing data.
- Index-Based Access: Every element in an array has an index, starting from zero. This makes it easy to access any element by using its index.
- Mixed Data Types: Arrays in Javascript can hold elements of different data types simultaneously, allowing for the storage of complex data structures.
- Built-in Methods: Javascript provides a plethora of built-in methods for arrays that facilitate common tasks such as sorting, slicing, reversing, and more, simplifying the manipulation of array data.
Considerations for Dynamic Arrays:While the dynamic nature of Javascript arrays offers flexibility, it's important to manage these arrays carefully. Adding or removing elements frequently can lead to performance issues in certain scenarios. Understanding and utilising array methods efficiently is crucial for maintaining optimal performance and clean code.
The push()
and pop()
methods are useful for adding and removing elements at the end of an array, respectively, making array manipulation more intuitive.
Javascript Array Methods
Javascript provides a plethora of methods to work with arrays, offering powerful functionalities that make data manipulation more efficient and straightforward. Understanding and utilising these array methods can significantly improve your coding practices.
Utilising the Javascript array length property
The length property of Javascript arrays is incredibly useful for various purposes, one of the simplest yet most powerful tools at your disposal. It returns the number of elements in an array, which is particularly helpful for iterating over array items or determining if an array is empty.Moreover, the length property is not just read-only; you can also set it to truncate or extend an array, offering a straightforward way to manipulate the array's size.
const colours = ['Red', 'Green', 'Blue']; console.log(colours.length); // Output: 3 colours.length = 2; console.log(colours); // Output: ['Red', 'Green']This example demonstrates how the length property is used to obtain and manipulate the number of elements within an array.
Setting length
to a value less than the actual number of elements truncates the array, removing elements from the end.
The power of Array filter in Javascript
The filter method in Javascript is a powerful tool for creating a new array populated with elements that pass a test implemented by a provided function. This method is ideal for tasks like removing unwanted values from an array or collecting a subset of data that meets certain criteria.A key benefit of filter is that it does not modify the original array, making it a preferred choice for many developers who advocate immutability in coding practices.
const numbers = [1, 2, 3, 4, 5]; const evenNumbers = numbers.filter(function(number) { return number % 2 === 0; }); console.log(evenNumbers); // Output: [2, 4]This example shows how the filter method is used to create a new array,
evenNumbers
, that contains only the even numbers from the original numbers
array. Other essential Javascript Array Methods
Beyond length and filter, there are several essential Javascript array methods that every developer should be familiar with:
- map(): Creates a new array populated with the results of calling a provided function on every element in the calling array.
- forEach(): Executes a provided function once for each array element.
- reduce(): Applies a function against an accumulator and each element in the array (from left to right) to reduce it to a single value.
- push() and pop(): Add elements to the end of an array and remove the last element of an array respectively.
- shift() and unshift(): Remove the first element from an array and add one or more elements to the start of an array, respectively.
- sort(): Sorts the elements of an array in place and returns the sorted array.
Enhancing efficiency with map() and reduce():Combining map() with reduce() can transform complex data manipulation tasks into simple, clean code. map() can first adjust each item in the array as needed, then reduce() can compile those changes into a single outcome. This pattern is especially powerful for data analysis and processing tasks.
Javascript Array Examples
Javascript Arrays are fundamental structures in programming that enable you to store, manipulate, and access a list of data items efficiently. Through practical examples, you will learn how to utilise these powerful tools in both simple and advanced scenarios to solve common problems and streamline your code.
Simple examples of Javascript Arrays
If you're new to Javascript or programming in general, starting with basic operations on arrays can lay a strong foundation for your coding skills. Let's explore some simple yet essential examples of how to create, access, and manipulate Javascript arrays.
// Creating an array of numbers const numbers = [1, 2, 3, 4, 5]; // Accessing elements from the array console.log(numbers[0]); // Output: 1 console.log(numbers[3]); // Output: 4 // Adding an element to the end of the array numbers.push(6); console.log(numbers); // Output: [1, 2, 3, 4, 5, 6] // Removing the last element from the array numbers.pop(); console.log(numbers); // Output: [1, 2, 3, 4, 5]This sequence of operations demonstrate the basics of creating, accessing, adding to, and removing from a Javascript array.
Remember, arrays in Javascript are zero-indexed, meaning the first element is at position 0.
Advanced usage examples of Javascript Arrays
Once you're comfortable with the basics, you can delve into more complex operations that showcase the true power of Javascript arrays. These advanced examples highlight methods and techniques for manipulating arrays in ways that are both elegant and highly functional.
// Merging two arrays into one using the spread operator const fruits = ['Apple', 'Banana']; const vegetables = ['Carrot', 'Potato']; const combined = [...fruits, ...vegetables]; console.log(combined); // Output: ['Apple', 'Banana', 'Carrot', 'Potato'] // Filtering an array based on a condition const numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9]; const even = numbers.filter(x => x % 2 === 0); console.log(even); // Output: [2, 4, 6, 8] // Mapping an array to create a new array of squared numbers const squares = numbers.map(x => x * x); console.log(squares); // Output: [1, 4, 9, 16, 25, 36, 49, 64, 81]These examples illustrate more advanced techniques such as merging arrays with the spread operator, filtering based on conditions, and transforming arrays with the map function.
The filter and map methods are especially powerful when combined. For instance, you can first filter an array to include only certain elements, then map those elements to a new form or value. Such compositions of array methods can lead to elegant and concise code solutions for complex problems. Here’s how you might use both to find the square of all even numbers in an array:
const numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9]; const evenSquares = numbers.filter(x => x % 2 === 0).map(x => x * x); console.log(evenSquares); // Output: [4, 16, 36, 64]By understanding and applying these advanced techniques, you enhance your ability to manipulate and interpret data efficiently.
Javascript Arrays Applications
In the dynamic world of web development, Javascript Arrays play a pivotal role. These data structures are not just tools for handling a collection of elements; they are the backbone for numerous real-world applications. From managing user inputs in forms to manipulating data retrieved from APIs, arrays make the job significantly easier and more efficient.Understanding how to leverage arrays in Javascript opens up vast possibilities for creating interactive and user-friendly web applications.
Real-world applications of Javascript Arrays
Arrays in Javascript find their application across various domains, making them indispensable in modern web development. Here are some real-world applications where Javascript arrays shine:
- Data Handling: Arrays are extensively used to handle lists of data entries, such as user details, product information, or posts in a blog.
- Interactive Features: Features like auto-complete search bars, sliders, and galleries use arrays to store and manipulate relevant data dynamically.
- Game Development: In browser-based gaming, arrays track player scores, manage game states, or even map out game grids.
- Analytics and Reporting: Arrays facilitate the analysis of data sets for generating reports, graphs, and charts, aiding in data-driven decision making.
Utilising arrays for data manipulation and storage is a critical skill for web developers. When handling arrays, methods such as map()
, filter()
, and reduce()
can drastically simplify your code and improve performance.
How Javascript Arrays enhance web development
The contribution of Javascript arrays to web development cannot be overstated. They offer a multitude of benefits that enhance both the development process and the end-user experience.
- Efficiency and Performance: With built-in methods for sorting, filtering, and manipulation, arrays allow for efficient processing of data with minimal code, leading to faster execution times.
- Flexibility: The dynamic nature of Javascript arrays, where their size and the type of elements they contain can be modified at runtime, allows for greater flexibility in developing applications that require data to be dynamically updated.
- Improved Readability: Organising data into arrays and employing array methods can greatly improve the readability of code, making it easier for other developers to understand and collaborate on a project.
- Enhanced User Experience: Arrays play a crucial role in creating interactive and dynamic web pages that respond to user inputs instantaneously, thus enhancing the overall user experience.
One intriguing aspect of arrays in web development is their use in Asynchronous Javascript and XML (AJAX) for making asynchronous web applications. When fetching data from a server without reloading the web page, arrays can store and process the received data, making it available for immediate manipulation and display. This technique has revolutionised web development, allowing for smoother interactions on the web without the traditional loading times.Moreover, frameworks and libraries like React and Angular make extensive use of arrays to manage the state within applications, further illustrating the array's foundational role in modern web solutions.
Javascript Arrays - Key takeaways
- Javascript Arrays: An ordered collection of values that may include various data types like numbers, strings, or other arrays, accessible by an index.
- Javascript array length: A property that returns the number of elements in an array, also allowing size manipulation by setting its value.
- Array filter in javascript: A method that creates a new array with elements that pass a defined test, without modifying the original array.
- Javascript array methods: Essential functions such as map(), forEach(), reduce(), push(), pop(), shift(), unshift(), and sort() for efficient data manipulation.
- Javascript Arrays applications: Utilised for data handling, interactive features, game development, and analytics in web development for dynamic and user-friendly experiences.
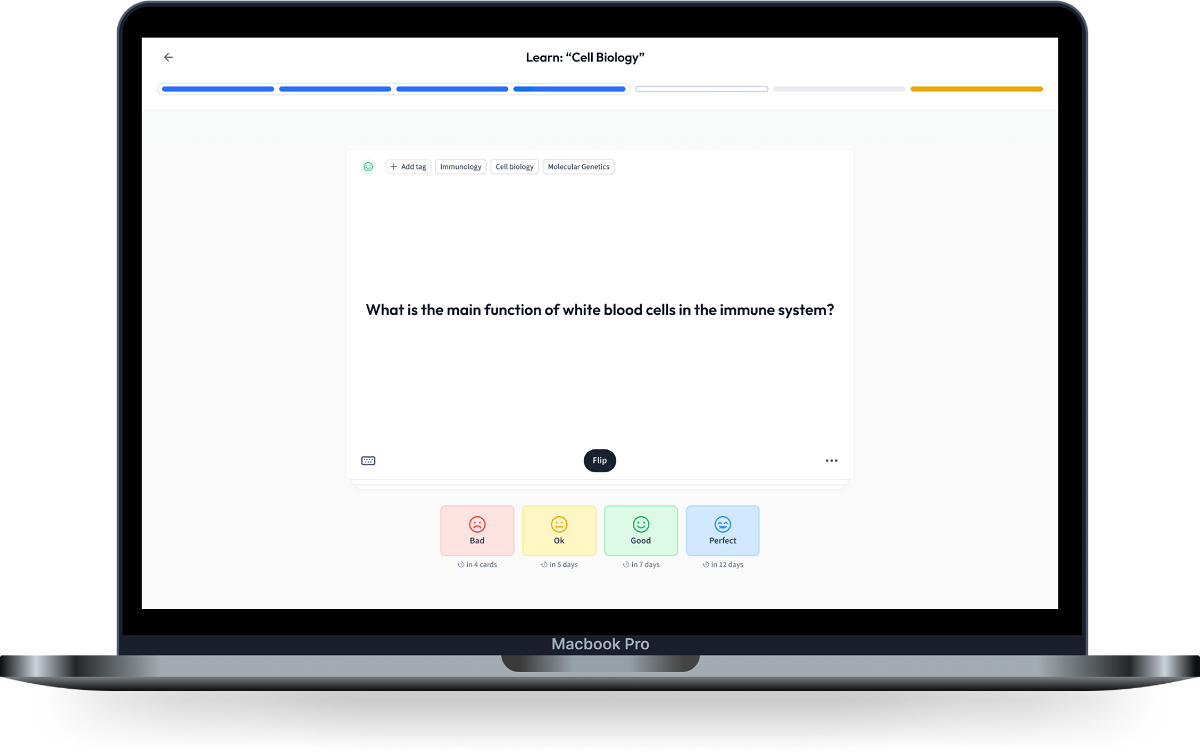
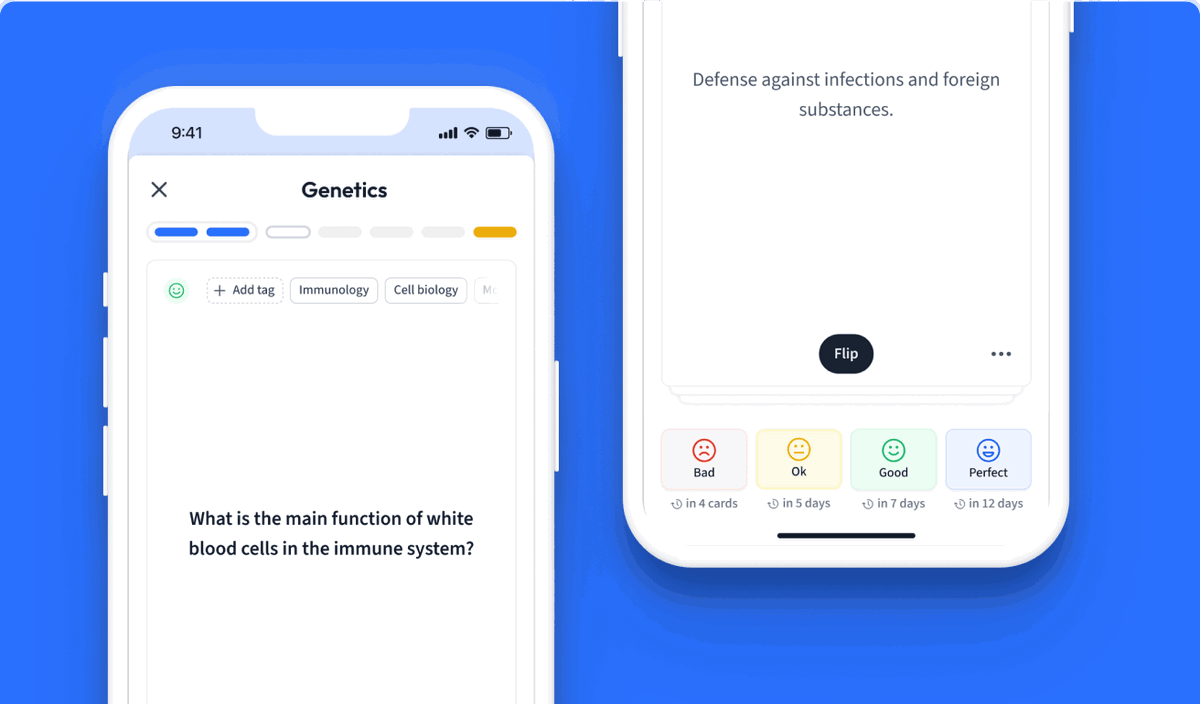
Learn with 111 Javascript Arrays flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Javascript Arrays
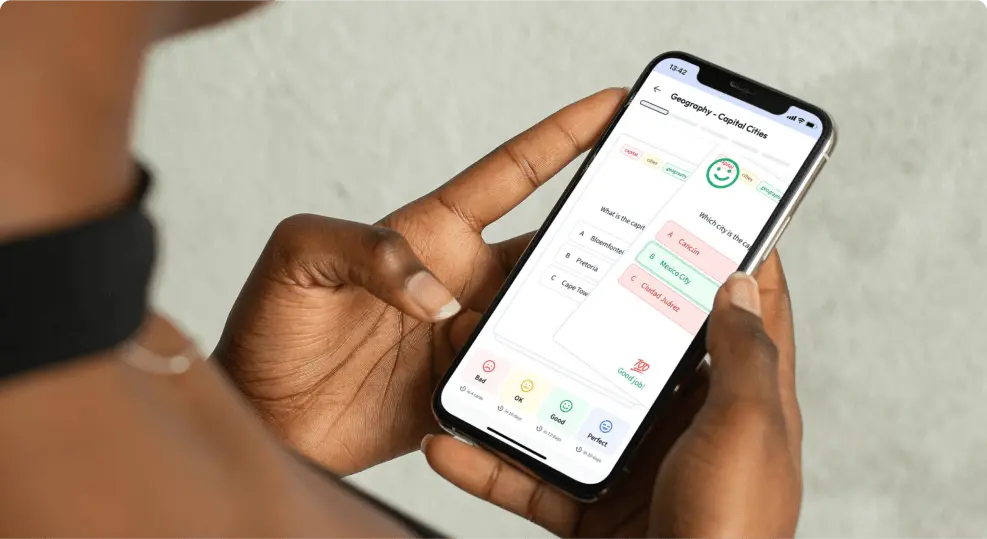
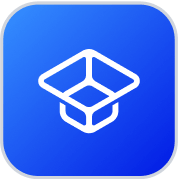
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more