What is C Sharp?
C Sharp, commonly known as C#, is a modern, object-oriented, and type-safe programming language developed by Microsoft. It combines the power and flexibility of C++ with the simplicity of Visual Basic. C# is designed to be a high-level language for developing a wide range of applications, including desktop, web, mobile, and game development. It runs on the .NET Framework, enabling developers to build applications that work seamlessly across various platforms.
Understanding C Sharp Programming Basics
The foundation of C# lies in its object-oriented principles, which facilitate structured and organized code that is both reusable and extensible. At its core, understanding C# involves getting to grips with its syntax, data types, control structures, classes, and methods.
- Syntax: The set of rules that defines the combinations of symbols considered to be correctly structured programs in C#.
- Data Types: A classification specifying the type of value that a variable can hold in C#, such as int, double, char, and string.
- Control Structures: Allow the flow of the program to change, executing certain sections of code depending on conditions using if-else statements, loops, and switch statements.
- Classes and Methods: C# is built on the concept of encapsulating data and behaviour into classes, with methods being the actions that can be performed on or by objects of these classes.
public class HelloWorld { public static void Main(string[] args) { // Print "Hello World" to the console System.Console.WriteLine("Hello World"); } }
This simple example demonstrates a basic C# program that prints 'Hello World' to the console. It illustrates the use of a class, public access modifier, static method, and the Main method, which is the entry point of a C# program.
The Evolution of C Sharp
Since its introduction in 2000, C# has undergone numerous updates and enhancements. Each version of C# has brought new features and improvements, significantly advancing its capability and making it more versatile.
- Version 1.0: Introduced in 2002, focusing on the basics of object-oriented programming.
- Version 2.0: Added generics, partial classes, and anonymous methods.
- Version 3.0: Introduced LINQ, lambda expressions, and extension methods.
- Version 4.0: Added support for dynamic binding and named/optional arguments.
- Version 5.0 and Beyond: Continued improvements including async/await patterns for asynchronous programming, pattern matching, and more.
The development of C# has been closely linked with the evolution of the .NET Framework and now .NET Core, ensuring that the language keeps pace with modern software development needs.
C# is part of the C-family of languages, sharing similarities with C, C++, and Java, making it easier to learn for those familiar with these languages.
Why Choose C Sharp for Software Development?
There are numerous reasons why developers choose C# for their software development projects. Its robustness, efficiency, and versatility make it a preferred choice for a wide range of applications.
- Cross-Platform Development: With the .NET Core framework, C# enables development for multiple platforms including Windows, macOS, and Linux.
- Rich Library Support: The .NET Framework provides an extensive class library that simplifies many programming tasks, from file handling to networking.
- Easy to Learn: Despite its powerful capabilities, C# has a gentle learning curve, making it accessible for beginners.
- Support for Modern Programming Practices: C# supports modern programming paradigms, including asynchronous programming, generics, and functional programming concepts.
Additionally, the vibrant community around C# and its integration with Visual Studio offers developers extensive resources and tools to develop, debug, and deploy applications effectively.
C Sharp Control Structures
Control structures in C# play a pivotal role in dictating the flow of execution within a program. These structures enable developers to orchestrate conditional operations, repetitions, and decision-making processes effectively. By understanding and applying these control structures, you can design programs that are efficient, scalable, and easy to debug.
Introduction to C Sharp Control Flow Mechanisms
In C#, control flow mechanisms refer to the constructs that manage the sequence in which instructions are executed. These include loops, conditional statements, and switch cases, each serving a unique purpose in guiding the execution path of a program.
Understanding these mechanisms is the first step towards mastering C# programming, as they form the backbone of creating dynamic and responsive applications.
A solid understanding of control flow mechanisms is essential for writing complex and efficient C# programs that can handle various runtime scenarios effectively.
Utilising Loops in C Sharp
Loops in C# are used to execute a block of code repeatedly until a specified condition is met. The language supports several types of loops, including for, while, and foreach loops, each tailored for different scenarios.
By leveraging loops, you can simplify code that requires repetitive tasks, such as processing collections of data, without manually repeating the code block.
For Loop: A control structure used to iterate a section of code a specific number of times. It consists of an initializer, condition, and iterator.
for(int i = 0; i < 10; i++) { System.Console.WriteLine(i); }
This example demonstrates a basic for loop in C# that prints numbers 0 through 9 to the console.
While Loop: Executes a block of code as long as a specified condition remains true.
int i = 5; while(i > 0) { System.Console.WriteLine(i); i--; }
This example shows a while loop that prints the numbers 5 to 1 in descending order.
Decision Making in C Sharp
Decision making in C# involves using conditional statements to execute different blocks of code based on specified conditions. The primary conditional statements are if, if-else, and switch statements.
These control structures are essential for creating branches in your code, allowing for more complex logic and code behaviours depending on runtime conditions.
If Statement: A basic conditional control structure that executes a block of code if a specified condition is true.
int number = 10; if(number > 5) { System.Console.WriteLine("The number is greater than 5."); }
This example demonstrates a simple if statement that checks if a number is greater than 5 and prints a message if the condition is true.
Switch Statement: Allows executing different blocks of code based on the value of a variable. It's especially useful when checking for multiple conditions.
int day = 3; switch(day) { case 1: Console.WriteLine("Monday"); break; case 2: Console.WriteLine("Tuesday"); break; case 3: Console.WriteLine("Wednesday"); break; // Other cases }
This example shows how a switch statement is used to print the day of the week based on the value of the day variable.
C Sharp Inheritance and Polymorphism
C Sharp (C#) is a powerful programming language that supports object-oriented programming (OOP) principles, among which inheritance and polymorphism are fundamental.
Inheritance allows classes to inherit properties, methods, and events from another class, promoting code reuse and the creation of a hierarchical class structure. Polymorphism, on the other hand, enables objects to be treated as instances of their parent class rather than their actual class, allowing for more flexible code.
Fundamentals of C Sharp Inheritance
Inheritance in C# lets you create a new class that reuses, extends, and modifies the behaviour that is defined in another class. The class whose properties are inherited is known as the base class, and the class that inherits those properties is called the derived class.
One of the key benefits of inheritance is the ability to use existing code, which helps in reducing redundancy and errors.
Base Class: The class from which features are inherited.
Derived Class: The class that inherits features from another class.
class Vehicle // Base class { public int Wheels = 4; public int MaxSpeed; public Vehicle(int maxSpeed) { this.MaxSpeed = maxSpeed; } } class Car : Vehicle // Derived class { public Car(int maxSpeed) : base(maxSpeed) { } }
In this example, Car inherits from Vehicle. Car can access the Wheels field and MaxSpeed property defined in Vehicle.
Implementing Polymorphism in C Sharp
Polymorphism in C# allows methods to do different things based on the object that is calling them. There are two types of polymorphism in C#: compile-time (or static) polymorphism and run-time (or dynamic) polymorphism.
Compile-time polymorphism is achieved through method overloading while run-time polymorphism is achieved through method overriding.
Method Overloading: Having multiple methods in the same class with the same name but different parameters.
Method Overriding: Redefining the implementation of a method in a derived class that has been inherited from a base class.
public class Shape { public virtual void Draw() { Console.WriteLine("Drawing a shape."); } } public class Circle : Shape { public override void Draw() { Console.WriteLine("Drawing a circle."); } }
This is an example of method overriding, where the Draw method in the Circle class overrides the Draw method in the base Shape class.
C Sharp Examples of Inheritance and Polymorphism
Let's look at a more concrete example to demonstrate both inheritance and polymorphism in action within a C# application.
public class Animal { public virtual void Speak() { Console.WriteLine("The animal speaks."); } } public class Dog : Animal { public override void Speak() { Console.WriteLine("The dog barks."); } } public class Program { public static void Main(string[] args) { Animal myAnimal = new Animal(); Animal myDog = new Dog(); myAnimal.Speak(); // Outputs: The animal speaks. myDog.Speak(); // Outputs: The dog barks. } }
In this example, the Dog class inherits from the Animal class and overrides the Speak method. This demonstrates polymorphism where myDog is treated as an Animal but calls the Speak method of Dog.
C Sharp Exception Handling
Exception handling in C# is a fundamental aspect of writing clean, reliable, and robust software. It allows developers to gracefully handle errors and exceptions that occur at runtime, ensuring that the application can recover or at least terminate gracefully, providing valuable feedback to the user and preserving data integrity.
Basics of Exception Handling in C Sharp
In C#, exceptions are unexpected or erroneous conditions that arise during the execution of a program. These could be due to invalid input, file not found, network issues, etc. Exception handling provides a way to transfer control from one part of a program to another, when an error occurs.
At the heart of exception handling in C# are several key concepts:
- Try: A block where exceptions can occur and is followed by one or more catch blocks.
- Catch: A block used to catch and handle the exception thrown from the try block.
- Finally: A block that executes after the try and catch blocks regardless of whether an exception was thrown or not.
- Throw: Used to explicitly throw an exception, either an existing one caught in a catch block or a new one.
Try, Catch, and Finally in C Sharp
The try, catch, and finally blocks form the backbone of exception handling in C#. They enable clear demarcation of code sections for potential problem areas, catching exceptions, and cleaning up resources respectively.
Try Block: The code that may cause an exception is enclosed in a try block. It is followed by one or more catch blocks.
try { // Code that may throw an exception }
This showcases a simple try block awaiting potential exceptions.
Catch Block: Specifies the type of exception you are trying to catch. It contains code that reacts to the exception.
catch(Exception e) { // Handle the caught exception }
This demonstrates how a catch block is used to handle an exception.
Finally Block: This block executes after the try and catch blocks regardless of whether an exception was caught or not. It is typically used to clean up resources.
finally { // Code to execute after try-catch, used for cleaning up }
An example showing the use of a finally block to perform clean-up.
Best Practices for C Sharp Exception Handling
Applying best practices in exception handling can significantly improve the reliability and maintainability of your C# applications. Here are some recommended strategies:
- Catch what you can handle: Avoid catching exceptions you cannot handle. It is generally a good practice to catch specific exceptions rather than all exceptions.
- Use finally for resource cleanup: Always use a finally block or, where available, a using statement to ensure resources are cleaned up properly.
- Throw meaningful exceptions: When throwing exceptions, include a clear and concise message that helps in understanding the root cause of the problem.
- Avoid exception handling for logic control: Use exception handling for exceptional cases and not for controlling the flow of your application logic.
Utilising the using statement can also provide a syntactically clearer way to ensure the disposal of resources, tightly coupling the lifespan of a resource with its scope.
Beyond these general tips, custom exception classes can be created in C# to represent specific error conditions more precisely. Inheriting from the System.Exception class enables you to add additional data and functionality to your exceptions, making them more meaningful to other developers and users.
Creating a Simple C Sharp Application
Developing a simple C# application is an excellent way to begin your journey in computer programming. C#, with its clear syntax and powerful framework support, offers an inviting path into the world of software development.
Setting Up Your Environment for C Sharp Programming
To start coding in C#, you need to prepare your development environment. This involves selecting an Integrated Development Environment (IDE) and installing the necessary tools.
- Visual Studio: One of the most popular IDEs for C# development, available for Windows and macOS.
- .NET Core SDK: A free, open-source development platform for building many kinds of applications.
After installing these, you're ready to create your first C# application.
Visual Studio Code is a lightweight, powerful source code editor that runs on your desktop. It’s available for Linux, macOS, and Windows.
Step-by-Step Guide to Your First C Sharp Application
Creating your first C# application involves several key steps, guiding you through writing, compiling, and running your code. Here’s a simple guide to get you started:
- Create a new C# Console App project in your IDE.
- Add the following code snippet into the Program.cs file:
using System; class Program { static void Main(string[] args) { Console.WriteLine("Hello, World!"); } }
This code represents a basic C# program that prints "Hello, World!" to the console.
- Click on the build option to compile your program.
- Run the compiled application to see the "Hello, World!" message in the console.
using System; class Program { static void Main(string[] args) { Console.WriteLine("Hello, World!"); } }
This example demonstrates the basic structure of a C# program, including the use of namespaces, a class definition, and the Main method, which is the entry point for execution.
Debugging C Sharp Applications
Debugging is a crucial part of the development process, allowing you to find and fix errors in your code. In C#, you can use IDE features to debug applications effectively.
- Breakpoints: Set breakpoints to pause your program and inspect its state at specific points.
- Step Over/Into: Use these features to execute your code line by line and investigate how it operates.
- Watch Window: Observe variable values and how they change over time during debugging.
These tools help you to understand the flow of your program and diagnose issues quickly.
Always ensure you understand the error messages displayed by your IDE, as they can provide valuable clues to the nature of the problem.
Understanding the underlying principles of C#, such as object-oriented programming, and mastering the subtle art of debugging are steps towards becoming proficient in C# development. As you grow more comfortable, experiment with different project types and explore C#'s extensive libraries and frameworks to build more complex and powerful applications.
C Sharp - Key takeaways
- C Sharp (C#): An object-oriented and type-safe programming language developed by Microsoft for various application development, running on .NET Framework.
- C# Programming Basics: Involves syntax, data types, control structures, classes, and methods that dictate the structure and behaviour of C# programs.
- C Sharp Control Structures: Key mechanisms like loops and conditional statements that manage the flow and decision-making within C# applications.
- C Sharp Inheritance and Polymorphism: OOP concepts in C# where inheritance allows class hierarchies and polymorphism lets methods perform differently based on the calling object.
- C Sharp Exception Handling: Techniques in C# for managing runtime errors gracefully using try, catch, and finally blocks, ensuring robust applications.
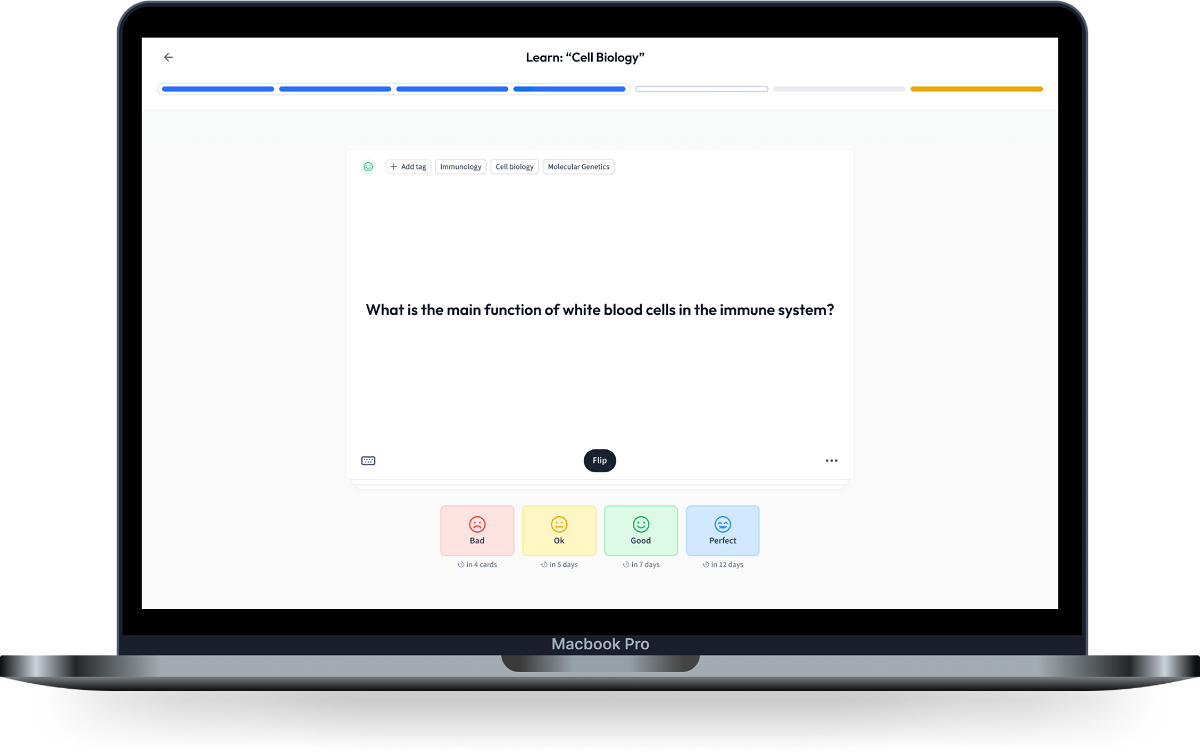
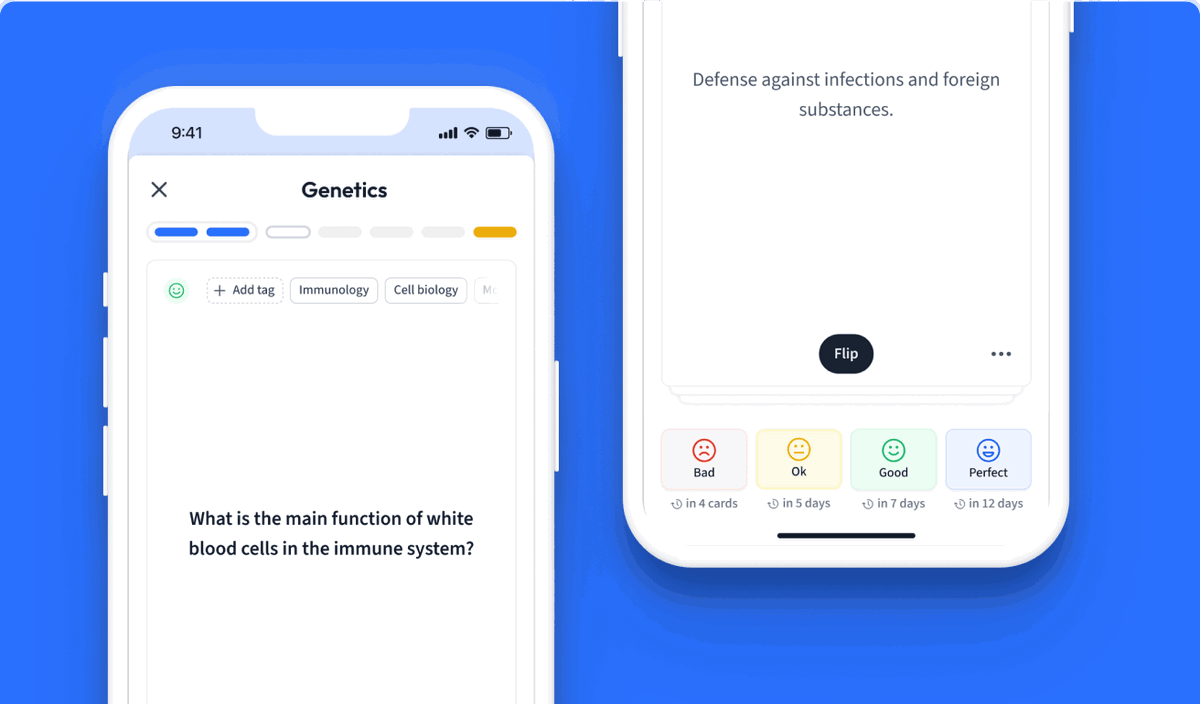
Learn with 33 C Sharp flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about C Sharp
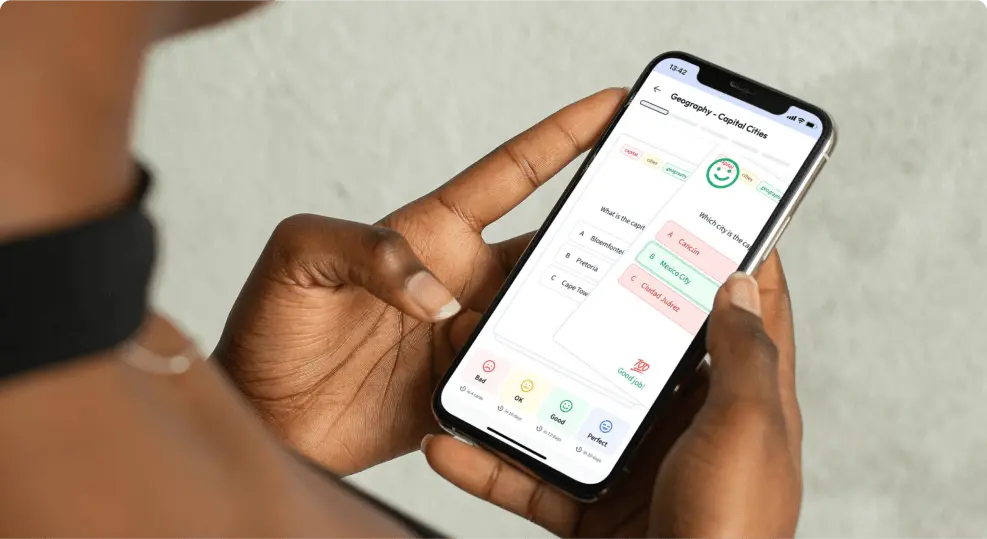
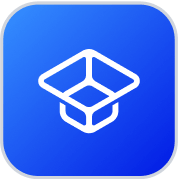
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more