Introduction to Python Loops
Python loops are an essential part of programming in this versatile language. They allow you to execute a block of code multiple times, making it easier and more efficient to work through repetitive tasks or iterate over sequences like lists, strings, and more. In this article, you'll explore the different types of loops in Python, their syntax, and how to use them effectively in your code.
Types of loops in Python
Python offers two main types of loops: the 'for' loop and the 'while' loop. Each type of loop has its unique use cases and serves different purposes in your code. Understanding the key differences between these loops will ensure that you make the best decision when deciding which one to use in your programs.
Loop: A programming construct that allows you to repeatedly execute a block of code until a specific condition is met.
The for loop in Python
A 'for' loop in Python is used to iterate over a sequence, such as a list, tuple, string, or other iterable objects. In each iteration, the loop variable takes the value of the next item in the sequence. The 'for' loop is extremely helpful when you know the exact number of times you want to execute a code block.
Here's an example of a simple 'for' loop that iterates through each item in a list and prints it:
fruits = ['apple', 'banana', 'cherry']
for fruit in fruits:
print(fruit)
Besides iterating over sequences directly, you can also use the built-in 'range()' function to create a range of numbers to iterate over. The 'range()' function takes three arguments: start, end, and step, with default values 0, required value, and 1, respectively.
Remember, when using the 'range()' function, the start is inclusive, while the end is exclusive. This means that the generated range will include the start number but exclude the end number.
The while loop in Python
The 'while' loop in Python is used to repeatedly execute a block of code as long as a specific condition is 'True'. This type of loop is more flexible than the 'for' loop since it does not rely on a predefined iterable sequence. Instead, it relies upon a dynamic condition that can change during each iteration of the loop.
While loop: A programming construct that repeats a block of code as long as a given condition remains 'True'.
Here's an example of a simple 'while' loop that prints the numbers 1 to 5:
number = 1
while number <= 5:
print(number)
number += 1
It is essential to remember that the 'while' loop's condition must eventually change to 'False', or the loop will continue to run indefinitely. This can cause an infinite loop, leading to a crash or unresponsive program. Always ensure that your 'while' loop has an appropriate termination condition.
Both types of Python loops, 'for' and 'while', provide powerful and flexible ways to perform repetitive tasks and work through sequences. By understanding their use cases and differences, you can make more informed decisions when implementing loops in your Python programs.
Looping Through a List in Python
When working with lists in Python, it's common to iterate through each element in the list and perform operations or manipulate the data. Python provides several options for looping through a list, making it convenient and efficient to work with this popular data structure.
Iterating over elements in a list with a for loop
One of the most common ways to iterate through a list in Python is by using a 'for' loop. As mentioned earlier, the 'for' loop is designed to work with sequences like lists. It allows you to access and manipulate each element in the list in a clean and efficient manner. You can perform various tasks, such as filtering, transforming, or simply printing the list elements, using a 'for' loop.
Here's an example of a 'for' loop that multiplies each element of a list by 2:
numbers = [1, 2, 3, 4, 5]
doubled_numbers = []
for num in numbers:
doubled_numbers.append(num * 2)
print(doubled_numbers)
When using a 'for' loop, you can access each element of the list directly, without needing to refer to their index. However, if you want to loop through a list using indices, you can combine the 'for' loop with the 'range()' function and the 'len()' function, which returns the length of a list.
Here's an example of iterating over elements in a list using their indices:
fruits = ['apple', 'banana', 'cherry']
for i in range(len(fruits)):
print(f"Element {i} is {fruits[i]}")
When you need to iterate through multiple lists concurrently, you can use the built-in 'zip()' function with a 'for' loop. The 'zip()' function combines multiple iterables, such as lists, into tuples that contain a single element from each iterable.
Here's an example of using 'zip()' to iterate through multiple lists simultaneously:
first_names = ['John', 'Jane', 'Mark']
last_names = ['Doe', 'Smith', 'Collins']
for first_name, last_name in zip(first_names, last_names):
print(f"{first_name} {last_name}")
In summary, the 'for' loop provides a flexible and efficient way to iterate through elements in a list. It is suitable for a wide range of tasks, from simple manipulation to more complex operations involving multiple lists. With the right combination of built-in functions and techniques, you'll be able to create powerful and efficient Python code for working with lists.
Break and Continue in Python Loops
When working with Python loops, it's essential to have control over the execution flow. Two important keywords in this context are 'break' and 'continue', which allow you to control the loop's behaviour during runtime. Both keywords can be used in 'for' and 'while' loops. Understanding how to use 'break' and 'continue' will enable you to write more efficient and cleaner code.
How to use break in a Python loop
The 'break' keyword in a Python loop is used to exit a loop prematurely before its natural termination. When the execution reaches a 'break' statement, the loop is terminated immediately, and the program continues executing the next statement after the loop.
Here are some common use cases for the 'break' keyword:
- Finding a specific element in a list or other iterable
- Stopping a loop when a certain condition is met
- Breaking out of a loop in a nested loop scenario
Here's an example illustrating the use of 'break' to find a specific element in a list:
fruits = ['apple', 'banana', 'cherry', 'orange']
target = 'cherry'
for fruit in fruits:
if fruit == target:
print(f"{target} found")
break
If the 'break' statement was not used within the loop, the loop would continue iterating through the entire list even after finding the target element, which would be an unnecessary operation. The 'break' statement improves efficiency by stopping the loop as soon as the target element is found.
How to use continue in a Python loop
The 'continue' keyword in a Python loop is used to skip the remaining code inside a loop iteration and jump to the next iteration. When the execution reaches a 'continue' statement, the loop moves to the next iteration immediately, without executing any subsequent statements within the current iteration.
Here are some common use cases for the 'continue' keyword:
- Skipping specific elements in a list or other iterable
- Continuing with the next iteration when a certain condition is met
- Filtering or processing elements within a loop
Here's an example illustrating the use of 'continue' to skip even numbers in a loop:
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9]
for num in numbers:
if num % 2 == 0:
continue
print(num)
In this example, the 'continue' statement causes the loop to skip even numbers. As soon as an even number is encountered, the 'continue' statement is executed, and the loop starts with the next iteration without executing the 'print()' statement, effectively only displaying odd numbers.
In conclusion, both 'break' and 'continue' keywords provide additional control over the execution flow of loops in Python. By using these keywords wisely, you'll be able to write more efficient, cleaner, and easier-to-debug code for your Python applications.
Practical Examples of Python Loops
In this section, we dive deeper into practical examples of using Python loops for various tasks such as calculations, data manipulation, and user input validation.
Using for loop in Python for calculations and data manipulation
Python 'for' loops are incredibly versatile when it comes to performing calculations and manipulating data. We will explore practical examples of using 'for' loops in these scenarios, including:
- Calculating the factorial of a number
- Reversing a string
- Calculating the sum of a list of numbers
Calculating the factorial of a number: The factorial of a non-negative integer n, denoted as n!, is the product of all positive integers less than or equal to n. In this example, we calculate the factorial using a 'for' loop.
Here's an example of using a 'for' loop to calculate the factorial of a number:
def factorial(n):
result = 1
for i in range(1, n + 1):
result *= i
return result
number = 5
print(f"The factorial of {number} is {factorial(number)}")
Reversing a string: Another practical use of 'for' loops is to reverse a string. In this example, we use a 'for' loop to iterate over the characters in the original string and build a new string with characters in reverse order.
Here's an example of using a 'for' loop to reverse a string:
def reverse_string(s):
result = ''
for char in s:
result = char + result
return result
input_string = 'hello'
print(f"The reverse of '{input_string}' is '{reverse_string(input_string)}'")
Calculating the sum of a list of numbers: When dealing with lists of numbers, it's often necessary to calculate the sum of all elements. In this example, we use a 'for' loop to traverse the list and add up the numbers.
Here's an example of using a 'for' loop to calculate the sum of a list of numbers:
def sum_of_list(numbers):
total = 0
for num in numbers:
total += num
return total
number_list = [1, 2, 3, 4, 5]
print(f"The sum of the elements in {number_list} is {sum_of_list(number_list)}")
These practical examples demonstrate the power and versatility of Python 'for' loops in performing a variety of calculations and data manipulation tasks.
Implementing while loop in Python for user input validation
A common use case for 'while' loops in Python is validating user input. We will explore practical examples of using 'while' loops for this purpose, including:
- Checking for an integer input
- Validating input within a specified range
Checking for an integer input: When user input should be an integer, a 'while' loop can be utilized to ensure the user provides a valid integer before proceeding.
Here's an example of using a 'while' loop to check for an integer input:
def get_integer_input(message):
while True:
user_input = input(message)
if user_input.isdigit():
return int(user_input)
else:
print("Please enter a valid integer.")
number = get_integer_input("Enter a number: ")
print(f"The entered number is {number}")
Validating input within a specified range: Sometimes, user input must fall within a specific range. In this example, we use a 'while' loop to request user input until a number within the specified range is provided.
Here's an example of using a 'while' loop to validate input within a specified range:
def get_number_in_range(min_value, max_value):
message = f"Enter a number between {min_value} and {max_value}: "
while True:
user_input = input(message)
if user_input.isdigit() and min_value <= int(user_input) <= max_value:
return int(user_input)
else:
print(f"Invalid input. Please enter a number between {min_value} and {max_value}.")
number = get_number_in_range(1, 5)
print(f"The selected number is {number}")
In these examples, 'while' loops are used to effectively ensure that user input is valid before proceeding with further processing or calculations. This demonstrates the versatility and practicality of 'while' loops in Python for user input validation.
Python Loops - Key takeaways
Python loops: 'for' loop and 'while' loop; used to execute repetitive tasks efficiently
Looping through a list in Python: using 'for' loop to iterate over elements in a list
Break in Python loop: exit loop prematurely when a specific condition is met
Continue in Python loop: skip the remaining code in a loop iteration and move on to the next iteration
Practical examples of Python loops: using loops for calculations, data manipulation, and user input validation
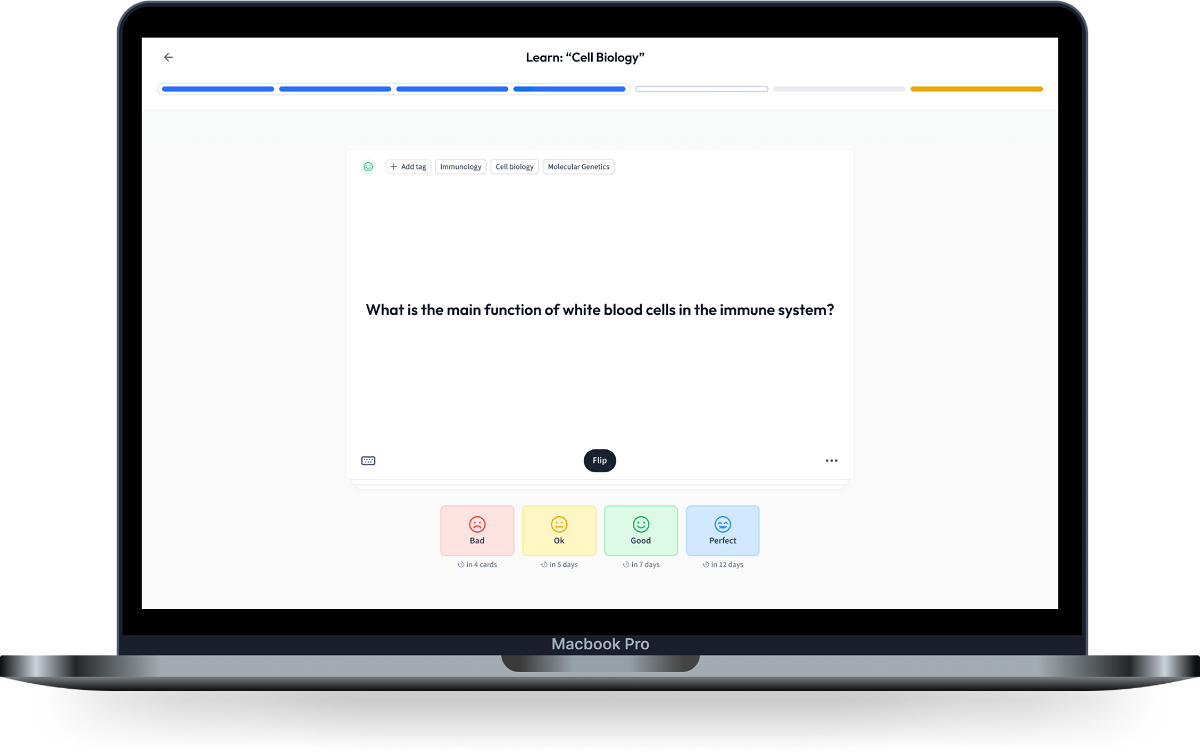
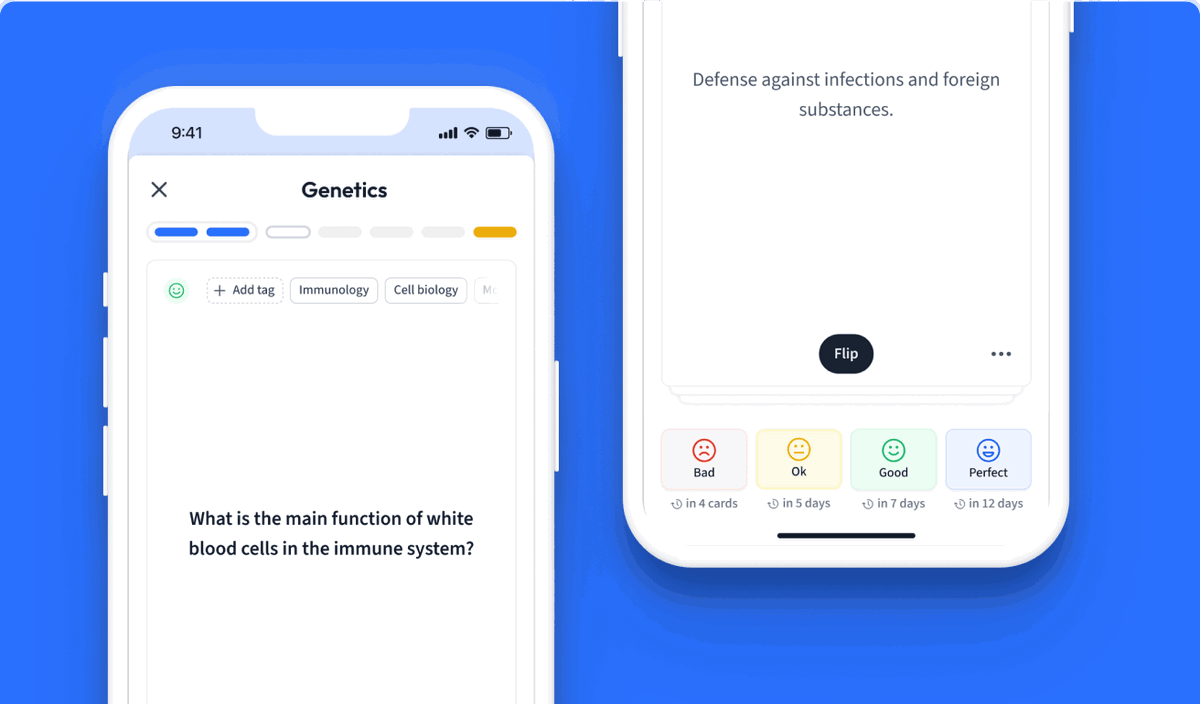
Learn with 169 Python Loops flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Python Loops
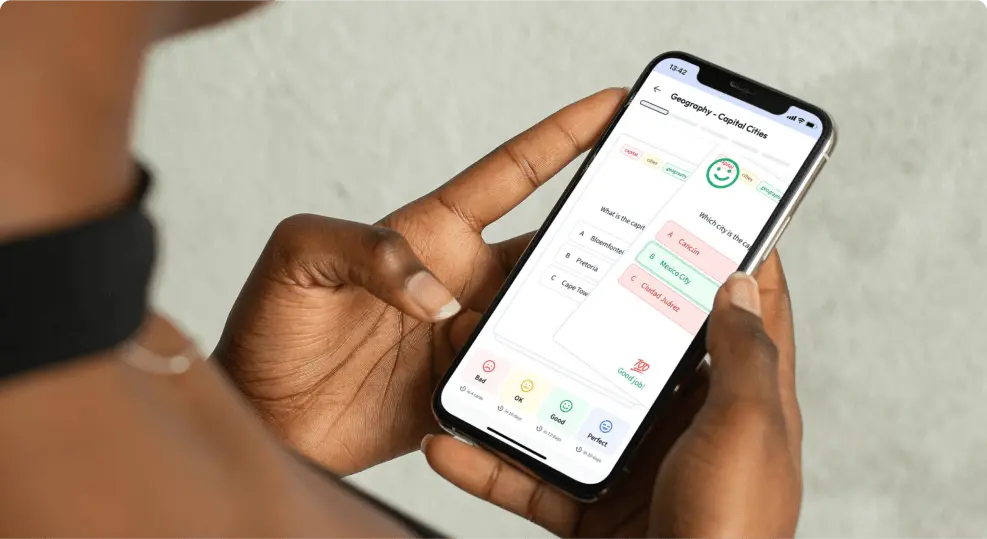
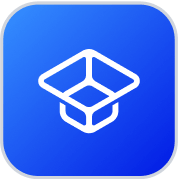
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more