An Inclusive Understanding of Java Loops
While running applications in Java, you might come across situations where you need to repeatedly execute a certain block of code. That's where Java Loops come into play. Understanding how these function is a powerful tool in your computer science toolkit. Let's delve into the world of Java loops and see how they can make coding much easier.The Core Definition of Java Loops
Java Loops are programming structures that help a certain block of code to be executed repeatedly until a certain defined condition is met. Essentially, these loops are the pillars of automation in software development.
while (condition) { // code to be executed }The code block inside the loop will continue to be executed as long as the condition returns true.
Loop Name | Syntax |
While loop | 'While(condition){code}' |
For loop | 'For(initialization; condition; inc/dec){code}' |
Do-While loop | 'Do{code}while(condition);' |
An Exploration of Different Java Loop Types
Java supports several different types of loops, which can be used depending on the specific requirements of your code.- While Loop: This executes a block of code as long as the given condition is true.
- For Loop: It is used when you know how many times you want to execute a block of code.
- Do-While Loop: Executes a block of code at least once and then repeats the loop as long as the given condition is true.
For example, a 'for' loop might be useful if you're writing a method to iterate over an array. On the other hand, a 'while' loop could be more appropriate if you're waiting for a certain condition to occur, such as a specific button press in a graphical user interface.
Java Loop Syntax: The Formula for Success
The structure of a Java loop is vital to understanding how they function. Here are the essential components of a Java loop:- Initialization: This is where you set up the loop variable for the loop.
- Condition: It is checked each time through the loop. If it holds, the loop continues; if not, the loop ends.
- Increment/Decrement: Here, you can increase or decrease the value.
For instance, while 'for' loops and 'while' loops look different in syntax, they can actually achieve the exact same results. The decision to use one over the other usually just comes down to personal code readability preferences or the specific requirements of the task at hand.
Exploring Various Java Loop Constructs in Detail
To master Java programming, grasping the workings of various Java loop constructs is pivotal. Over the years, Java has introduced different looping structures to cater to diverse coding needs in the software development sphere.Introduction to For Each Loop in Java
The 'for' loop, while a versatile tool, can sometimes be overkill for tasks such as traversing collections or arrays. That is where the For-Each loop, also known as the Enhanced For loop, comes in handy.The For-Each loop is designed for iteration over arrays and collections. It functions without requiring the length or size specification, making the code more compact and readable.
for (type var : array) { // commands using var }The 'type' should match the element type of your array or collection. Then 'var' is a variable that represents the current element in the loop. Let's say you have an array of integers and you want to print them all.
int[] numArray = {1, 2, 3, 4, 5}; for (int num : numArray) { System.out.println(num); }
Infinite Loops in Java: A Double-Edged Sword
Infinite loops, as the name suggests, are loops that go on indefinitely. These loops don't have a valid boolean condition that ends the loop or an update statement that eventually fulfils the loop condition. While these might seem like an error, there are situations where you might require an infinite loop intentionally. For example, you might create an infinite loop that runs until the user performs a certain action, such as clicking a button. However, be cautious as incorrect usage of infinite loops can lead to unresponsive or resource-hungry programs. Thus, it is paramount to control the execution of an infinite loop properly to ensure it breaks at a desired event or condition. An example of an infinite loop would be:while (true) { // code to be executed indefinitely }
The Essential Loop Structures in Java
Java primarily offers three types of loop structures.- For loop: It's often used when the number of iterations is known.
- While loop: It's used when the number of iterations is not known and the loop needs to be executed until a specific condition is met.
- Do-While loop: This loop also works like the 'while' loop but differs in how it tests the condition. The test condition appears at the end of the loop, so the code inside the loop is executed at least once.
Loop Type | Syntax |
For loop | 'For(initialization; condition; inc/dec){code}' |
While loop | 'While(condition){code}' |
Do-While loop | 'Do{code}while(condition);' |
Diving Deep into Java Loops Examples
In essence, the beauty of Java lies in its sophisticated yet adaptable framework, precisely seen through various examples of Java loops applications. Diving into practice, let's explore how these loops practically offers solutions to complex problems in coding.Practical Examples of Java Loops
A theory without practice is like a car without fuel. It might look good, but it doesn't quite take you anywhere. Now that you've learnt the ins and outs of the theory of Java Loops, let's put your knowledge into practise. Firstly, let's look at a **For Loop** example. Supposing you want to print the first ten natural numbers, the following code accomplishes this: ```htmlfor(int i=1; i<=10; i++){ System.out.println(i); }``` As per this code, it starts with 'i' initialised to 1, and the loop runs until 'i' is less than or equal to 10. The value of 'i' is incrementally increased at each iteration. Secondly, a **While Loop** can also print the first ten natural numbers. Although quite similar to the above 'for loop' structure, it offers a more flexible approach. ```html
int i = 1; while(i <=10){ System.out.println(i); i++; }``` As per the code above, you first initialise 'i' to 1. The loop then runs as long as 'i' is less than or equal to 10. With each iteration, 'i' increases once, until it finally becomes 11 to finish the loop. Finally, let's consider a **Do-While Loop**. If we choose to print the first ten natural numbers using this loop, even though the loop condition is at the end, it can still perform the task. ```html
int i = 1; do { System.out.println(i); i++; } while(i <=10);``` This structure ensures that the loop body executes at least once, irrespective of the condition defined. It's because the loop's test condition is checked after executing the loop's body.
Case Studies of Loop Control in Java
Java offers two keywords: 'break' and 'continue' to serve as loop control mechanisms in special circumstances. In a nutshell, the **'break'** statement is used to completely break the loop prematurely based on a condition. Conversely, the **'continue'** statement halts the current iteration and forces the loop to start the next one. Look at an illustrative example of the 'break' keyword below: ```htmlfor(int i=1; i<=10; i++){ if(i == 5){ break; } System.out.println(i); }``` In the presented 'for loop', the condition checks to see if 'i' equals 5. If true, the loop instantly breaks, ignoring the rest of its body. As a result, the output skips the rest, stopping at 4. Similarly, here's an example using the 'continue' keyword: ```html
for(int i=1; i<=10; i++){ if(i == 5){ continue; } System.out.println(i); }``` In this code, when 'i' equals 5, the 'continue' statement is executed, causing the loop to instantly jump to the next iteration, skipping the rest of the code following it within the loop. Consequently, all values except 5 are printed as output.
The Most Common Issues with Java Loops Examples
Despite its utility, Java loops do come with their fair share of common pitfalls if used inaccurately. Here's a more thorough look into these issues, spreading over different scenarios. A common issue you might face with loops is the **infinite loop**. As you know, this loop runs indefinitely because its condition either doesn't exist, or never becomes false. One small mistake can cause your system resources to drain out rapidly. ```htmlfor(int i=1; i>0; i++){ System.out.println(i); }``` Heed that in the aforementioned piece of code, the termination condition 'i > 0' will never become false. Hence, the code gets trapped in an endless loop. Make sure to double-check conditions and maintain appropriate initialization and updation of loop variables. Another issue is the **off-by-one error**. This occurs when your loop iterates one time too many or too few. This is often caused due to <= or >= in the loop condition where simple < or > should have been used.
Avoid these common issues by writing clear, well-commented code, and always testing your loops carefully. Loops can be incredibly powerful, but like any tool, they must be used with precision to truly be effective.
How to Master Loop Control in Java
Mastering loop control in Java allows you to dictate the flow of your program precisely. When you understand the mechanisms behind controlling loops, you can write more efficient and flexible code. Major components of this mastery revolve around two principle keywords: 'break' and 'continue'.Essential Steps to Control Java Loops Efficiently
An efficient Java program often involves optimising the performance of loops. Let's explore how you can control Java loops more efficiently.- Identify loop conditions wisely: Loops always run based on certain conditions. Therefore, you must carefully determine these conditions. Establish a precise plan for when your loop should start, continue, and terminate in response to specific conditions.
- Use 'break' and 'continue' proficiently: 'Break' and 'continue' are two control statements that allow you to have more control over your loops. 'break' terminates the loop prematurely, while 'continue' halts the current iteration and jumps to the next one. Effectively using these control statements can make your loops more dynamic.
int[] numArray = {10, 20, 30, 40, 50}; int searchElem = 30; for (int num : numArray) { if (num == searchElem) { System.out.println("Element found!"); break; } }In the above example, when the element 30 is found, the 'break' statement executes and the loop stops. No other iterations take place, saving time and computational resources. Similarly, the 'continue' statement can be used to skip unwanted iterations without breaking the loop.
for (int num=1; num<=10; num++) { if (num % 2 == 0) { continue; } System.out.println(num); }The example skips printing even numbers by using the 'continue' statement. The moment an even number is encountered, the ‘continue’ statement brings control to the start of the loop for the next iteration.
Overcoming Challenges in Loop Control in Java
Despite the benefits that loops bring to the table, they often present developers with challenges. Here are common problems relating to Java loops and how to overcome them:- Infinite Loops: An Infinite loop is a loop that never terminates due to an inaccurate or non-existent condition. Debug your code and scrutinise your loop condition, ensuring it can be false at some point to exit the loop.
- Off-by-One Errors: Off-by-one errors happen when your loop runs one iteration too many or too few. They derive from an error in the boundary conditions of the loop. Try to replace <= or >= comparisons with < or > where possible, as it's often the cause of these kinds of errors.
int i = 1; while (i > 0) // No provision for i to be ever less than 0 { System.out.println(i); i++; }In this code, the 'while' loop will continue indefinitely because 'i' is always greater than 0 and keeps incrementing. Be vigil to not fall into this pitfall. Loop control in Java, while appearing simple, often requires thoughtful consideration of conditions and scenarios. As you handle complex Java applications, a proper understanding of loops, their conditions, and controls becomes ever more paramount for efficient programming.
The Effective Usage of Different Java Loop Types
Java offers a diverse portfolio of loop types to choose from, each tailored to different scenarios. Despite this diversity, these various loops all share the primary goal of executing repetitive tasks with ease and control. But how does one decide which loop best fits a particular situation? That depends on several factors, each explored in-depth in the next sections.When to Deploy Different Loop Types in Java
Knowing when to use each type of loop in Java is pivotal in writing effective code. As a general rule, the type of loop to be used often hinges on the complexity of iteration, the knowledge of the number of iterations, and whether the loop condition is checked before or after the loop code block among other factors.- For Loop: Ideal for situations when the exact number of iterations is known beforehand. The 'For Loop' combines declaration, condition, and increment/decrement in one line, making code neat and digestible. You can also loop through data structures like arrays and lists using 'For Loop'.
- While Loop: Used when the number of iterations is unknown and the loop must continue until a specified condition becomes false. For example, reading user input until they enter a certain value.
- Do-While Loop: Much like the 'While Loop', but with a fundamental difference - the code block in a 'Do-While loop' executes at least once, even if the condition is false. This loop is very useful when the loop body needs to run at least one time, like menu-driven programs.
// While Loop int a = 0; while(a > 0) { System.out.println("This line will not print."); a--; } //Do-While Loop int b = 0; do { System.out.println("This line will print once."); b--; } while(b > 0);In the above example, the 'while' loop won't run as the condition "a > 0" is false. However, the 'do-while' loop prints once, regardless of the condition "b > 0" being false initially.
The Role of Loop Types in Functional Java Programming
Different types of loops in Java are not only used to control the iterations in a program but also play a major role in functional Java programming. The choice between 'For', 'While', and 'Do-While' affects the execution flow and efficiency of your solution. An apt selection of loop can lead to faster execution of the program, better resource management, and more readable code. One of the frequently used paradigms in functional programming is recursion—a function that calls itself until a termination condition is met. In Java, this can be efficiently achieved using 'For' loops or 'While' loops. As a rule of thumb, if your iteration demands a significant degree of complexity, involves operations like recursion or traversal, or simply needs a definitive start and end point, a 'For Loop' will serve the purpose effectively. On the other hand, situations where the loop should continue until a certain condition is met, 'While Loop' or 'Do-While Loop' comes in handy. It's the flexibility of these loops that allow you to manage more dynamic scenarios where the conditions for iteration do not solely depend on numeric counters. One fantastic aspect of Java programming is the @bold{enhanced 'For Loop'}, also known as the for-each loop—an exceptionally useful feature in functional Java programming. This loop is primarily used when you need to traverse arrays or collections, providing significantly simplified syntax and more robust code. For example:int[] myArray = {1, 2, 3, 4, 5}; for(int num : myArray) { System.out.println(num); }In the above code, 'num' automatically collects subsequent array elements with every iteration, no manual update is required. This helps in avoiding common errors that bedevil manual loop control, making your code cleaner and more reliable. By using different loop types properly, you can utilise resources better, reduce redundancy, and write much more efficient, functional codes in Java. Understanding their uses and implementations will definitely step up your Java programming game.
Java Loops - Key takeaways
- Java Loops Definition: Java loops are control structures that repeat a block of code as long as the test condition is true. They help by reducing the effort of writing the same code multiple times.
- For Each Loop Java: This is designed for iteration over arrays and collections without needing the length or size specification, making the code more compact and readable.
- Infinite Loops in Java: Infinite loops are loops that continue indefinitely without a valid boolean condition that ends the loop. They are useful when a loop needs to run until a user performs a specific action, but can lead to unresponsive or resource-hungry programs if not controlled properly.
- Java Loop Constructs: Java provides three types of loops - For loop, While loop and Do-while loop. Choosing the right type depends on the conditions or requirements of the iteration.
- Loop Control in Java: Java offers two keywords for loop control - 'break' and 'continue'. 'Break' is used to terminate the loop prematurely based on a condition, and 'continue' stops the current iteration and starts the next one.
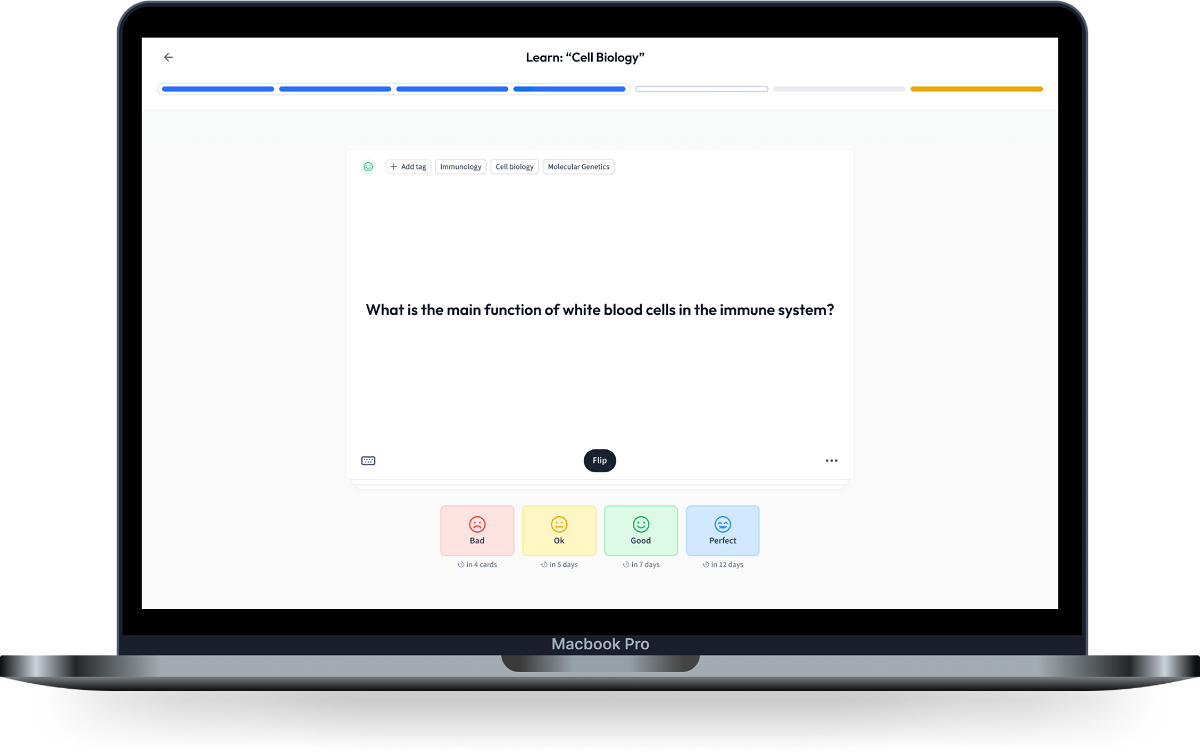
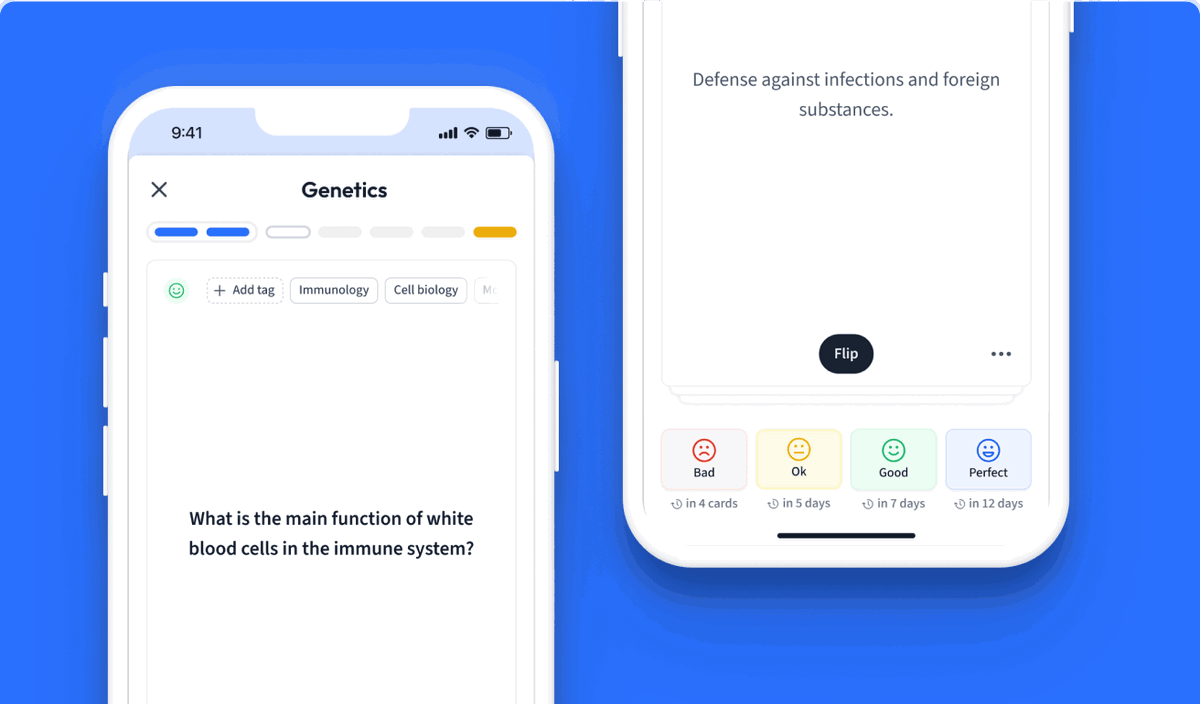
Learn with 84 Java Loops flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Java Loops
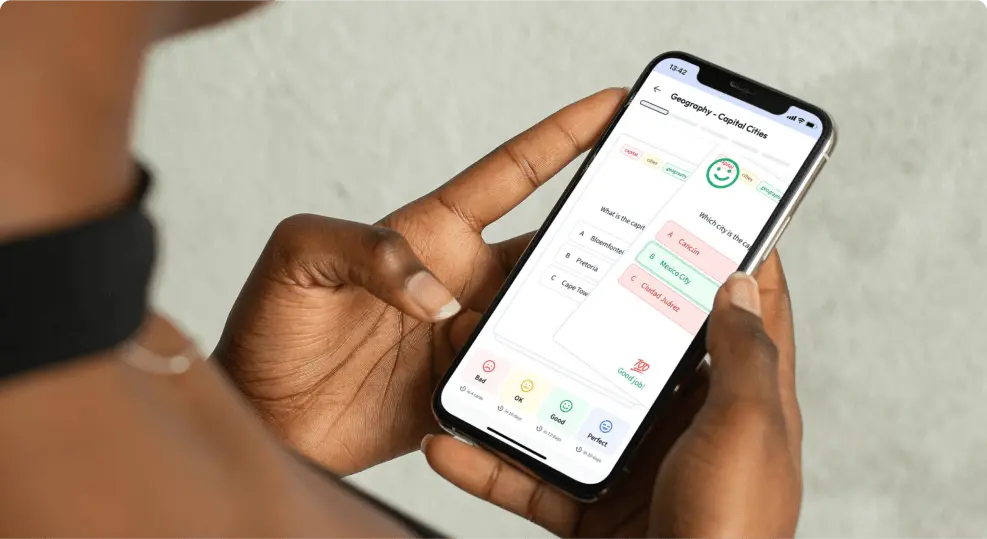
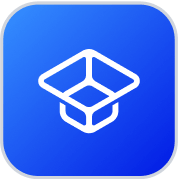
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more