Understanding Java Non Primitive Data Types
In the realm of computer science, particularly the Java programming language, different types of data are crucial in determining how operations are performed on the variables holding this data. Java fundamentally categorises these into two: primitive and non-primitive data types. As a student venturing into the world of Java, your understanding of non-primitive data types is paramount to assist with the successful development of your applications.
The Basics of Non Primitive Data Types in Java
Non-primitive data types are as essential to Java as integers are to basic arithmetic. They’re also referred to as reference or object data types since they reference memory locations where data is stored, unlike primitive types that store the actual values. The beauty of non-primitive types resides in their flexibility as they can hold multiple values and perform various operations on those values.
A non-primitive data type is a type of data that references a memory location through which data can be accessed, stored, and manipulated. They are created by the programmer and not defined by Java (except for the String).
Key features of non-primitive types include:
- They contain methods to manipulate data.
- Their size is based on the amount of data they need to hold.
- They can be used to store multiple values of their element type.
Categories of All Non Primitive Data Types in Java
Non-primitive data types in Java are broadly categorized into three, namely:
- Classes
- Arrays
- Interfaces
Each category has its unique properties and uses. For example:
Classes are templates from which objects are created. In non-primitive data types, all values are instances of a particular class.
Category | Description |
Classes | Classes are templates for creating user-defined data types and objects. They can contain fields, methods, constructors, and blocks. |
Arrays | Arrays are homogeneous data structures used to store elements of the same type. They hold a fixed number of values of the same type. |
Interfaces | Interfaces are reference types similar to classes but only contain static constants and abstract methods. These truly cement the concept of abstraction in Java. |
Examples and Usage of Non Primitive Data Types in Java
Let's start with a simple string example. A string is a common non-primitive data type in Java:
String greeting = "Hello, World!"; System.out.println(greeting);
Another example is using arrays, where multiple values can be stored in a single array object:
int[] myNum = {10, 20, 30, 40}; System.out.println(myNum[2]);
Non-primitive data types bring versatility and power to Java programming. Harnessing their capabilities is an essential stride towards becoming a proficient Java developer.
Difference between Primitive and Non Primitive Data Types in Java
In Java programming language, programmers work with two types of data: primitive and non-primitive. Each of these data types has unique characteristics, and understanding them is vital in writing effective code. In particular, the divide between primitive and non-primitive data types is a crucial concept to comprehend.
What are Primitive Data Types in Java: A Brief Overview
Java has eight basic or primitive data types. These are predefined by the language and defined to be the building blocks for data manipulation. They include byte, short, int, long, float, double, boolean, and char. Each of these data types has a pre-determined size and can hold a specific range of values.
For instance, the int data type consumes 4 bytes of memory and can store values from -2,147,483,648 to 2,147,483,647. Furthermore, primitive data types are stored directly in the memory and are faster to access because of their fixed size and values.
The following table provides an overview of the Java primitive data types:
Type | Size | Example |
byte | 1 byte | byte myByte = 100; |
short | 2 bytes | short myShort = 5000; |
int | 4 bytes | int myInt = 100000; |
long | 8 bytes | long myLong = 15000000000L; |
float | 4 bytes | float myFloat = 5.75f; |
double | 8 bytes | double myDouble = 19.99d; |
boolean | 1 bit | boolean myBool = true; |
char | 2 bytes | char myChar = 'A'; |
What are Non Primitive Data Types in Java: A Brief Overview
Unlike the primitive data types, non-primitive data types include Classes, Arrays, and Interfaces. They are also called reference or object data types because they reference a memory location where values are stored, not the actual values themselves, as with primitive data types.
Non-primitive types are mutable, meaning their values can be changed. They don't have a preset size, as their size becomes defined when they get constructed. For instance, an array's size gets determined when it's created, and it can hold different types of data.
//array of integers int[] myArray = new int[10]; //array of strings String[] myStringArray = new String[5];
Another vital feature of non-primitive data types is that they can store null as a value, unlike primitive data types. A null value represents no value or no object. It's not equivalent to an initial value of zero, as in primitive types.
The Key Differences Between Primitive and Non Primitive Data Types in Java
The differences between primitive and non-primitive data types in Java are multifaceted. The fundamental difference lies in the way Java treats these data types. Here are the key differences:
- Memory: Primitive types are stored directly in memory, while non-primitive types store a reference or an address to the location in memory where the data is found.
- Size: Primitive types have a fixed size, while non-primitive types do not. The size of non-primitive types depends on the programmer's implementation.
- Null value: Primitive types cannot be null, while non-primitive types can be null.
- Methods: Non-primitive types (because they're objects) can perform various operations using methods. Primitive types can't do this.
- Default value: Each primitive data type has a default value that gets assigned if no value is specified. Non-primitives (except for Strings) yield a default value of null.
Understanding these differences helps programmers write more efficient, reliable, and bug-free Java applications. Remember, the right choice of data type can optimize your program's performance and make it easier to build and maintain.
Practical Guide to Using Non Primitive Data Types in Java
Java's non-primitive data types differ from primitive types in multiple ways that affect their interaction with one another within a programme. Non-primitive data types broaden the scope of what can be accomplished in Java, providing more methods and techniques for manipulating data and creating complex programming structures. An understanding of these distinctions is essential for accomplishing more intricate tasks, which build upon basic Java knowledge.
Techniques for Using Non Primitive Data Types in Java
Non-primitive data types open the door to more advanced programming techniques. They can hold multiple values, allowing for complex data structures and facilitating operations that would be inconvenient or impossible with primitive data types alone. Mastering these techniques is vital for writing efficient and optimised code.
Methods: Non-primitive types, being objects, can call upon various methods to manipulate their data. This is a gigantic plus you wouldn't find in primitive types. For instance, Strings, a non-primitive data type, have a host of useful methods:
String name = "Java"; int length = name.length(); String lowerCaseName = name.toLowerCase();
The aforementioned code utilises methods to determine the length of the string and convert it to lower case.
Null Value: Unlike primitive types, non-primitive data types can store null as a value. A null value represents no value or no object. It's not equivalent to an initial value of zero, as in primitive types. That said, one must handle null values correctly since they can lead to NullPointerException issues.
String str = null; // stores a null reference str.length(); // would throw a NullPointerException
Here, the length method is called on a null reference, leading to an error. So, it's always good practice to check for null before invoking methods.
In non-primitive types, Constructors play a key role in creating new objects. These special blocks of code initialise the object when called using the new keyword.
ArrayListlist = new ArrayList ();
In the preceding code, ArrayList's constructor is used to create an object, which can store multiple Integer values.
Common Applications of Non Primitive Data Types in Java Example
There are countless applications of non-primitive types since they are the cornerstone for building complex applications. They're used to create data structures, read and write data, handle exceptions, and so much more. To illustrate further, here are specific examples.
Data Structures: Non-primitive types are integral in defining and managing data structures in Java, such as arrays and collections (ArrayLists, LinkedLists, Stacks, and more). These structures handle multiple, possibly vast, data effectively.
//array of strings String[] names = {"John", "Alice", "Bob"}; //List collection example Listcities = new ArrayList (); cities.add("London"); cities.add("New York");
The above snippets show the declaration of an array and a collection (ArrayList), both vital tools to manage and manipulate large data sets.
Handling Files: Java's File class, one such non-primitive data type, provides myriad useful methods for handling files and directories. This class paves the way for file operations, like creating, reading, and writing data to files.
File file = new File("demo.txt"); boolean createNewFile = file.createNewFile();
The code above creates a new file named "demo.txt".
Advance Implementation of Non Primitive Data Types in Java
Advanced implementations of non-primitive data types are frequently found in designing custom classes, abstract data types, and working with APIs. One ubiquitous case is the creation of user-defined classes—a testament to the advantages of non-primitive types. These classes enable better encapsulation and more complex object behaviour.
class Student { String name; int age; // constructor Student(String name, int age){ this.name = name; this.age = age; } } ... Student stu = new Student("Tom", 22);
In the above code, a new class, Student, is defined with its properties and constructor. And then, an object of the Student class is created, which is an instance of the Student class.
The non-primitive data types' broad applicability and scale make them indispensable in Java development. They're used in more complex programming constructs, allowing Java developers to handle complex problems more efficiently, be it for designing UI, building back-end systems or contributing to software architecture. In essence, non-primitive data types are the cornerstone of complex data manipulation and software development practices.
Java Non Primitive Data Types - Key takeaways
- Java Non Primitive Data Types are essential in Java as they can hold multiple values and perform various operations on these values.
- A non-primitive data type is a data type that references a memory location where data can be accessed, stored and manipulated, they are created by the programmer.
- There are three main categories of non-primitive data types i.e. Classes, Arrays, Interfaces. They have their unique properties and uses. For example, Classes are templates from which objects are created.
- Non-primitive data types bring versatility and power to Java programming, examples of these are strings and arrays where multiple values can be stored in a single object.
- The main differences between primitive and non-primitive data types in Java are in the way they are stored memory-wise, their size, NaN value representation, methods application, and default values.
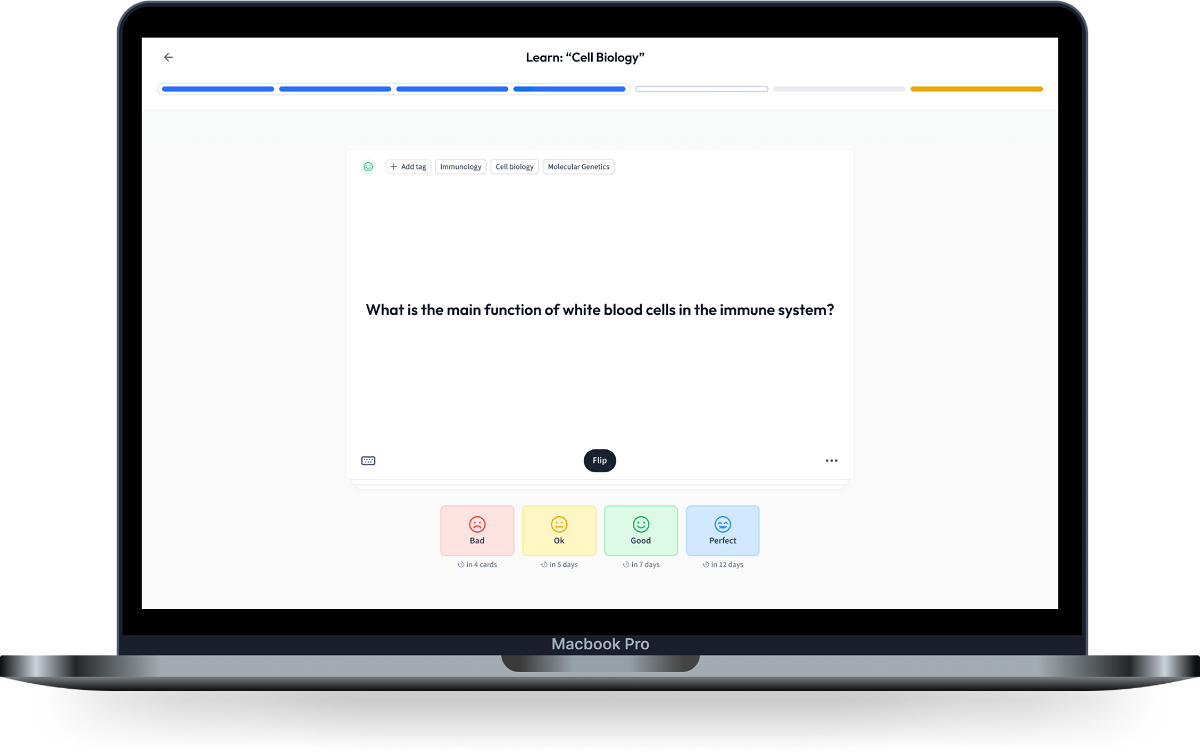
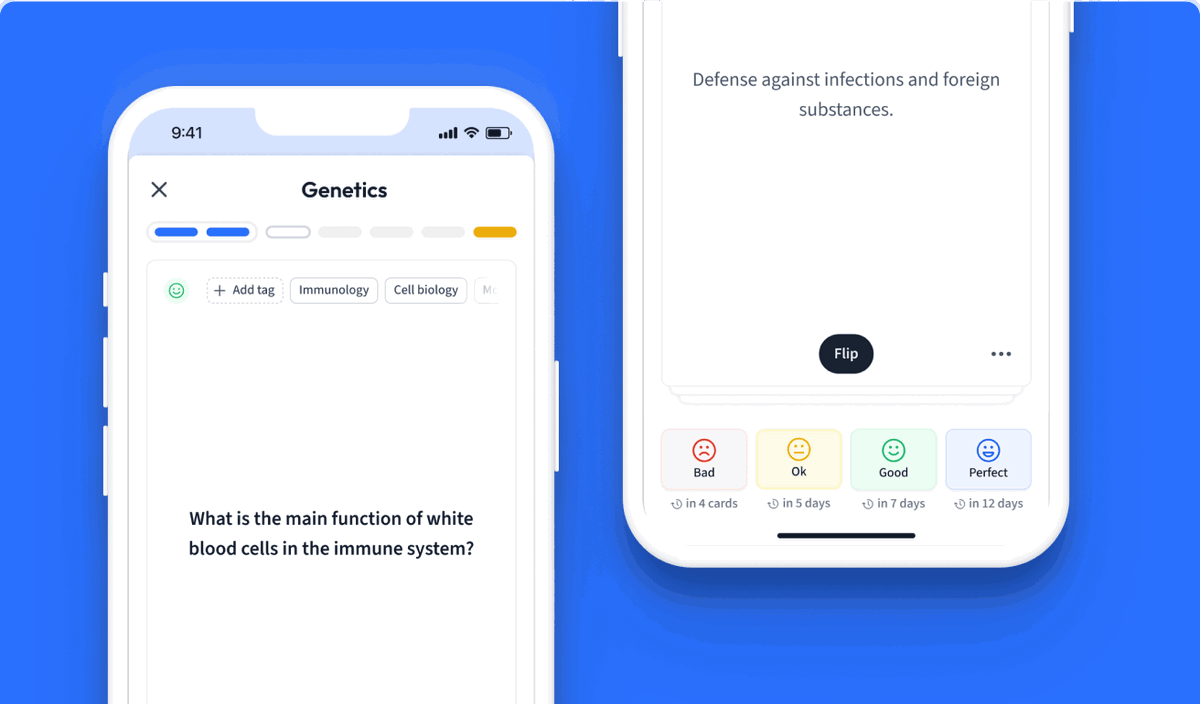
Learn with 12 Java Non Primitive Data Types flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Java Non Primitive Data Types
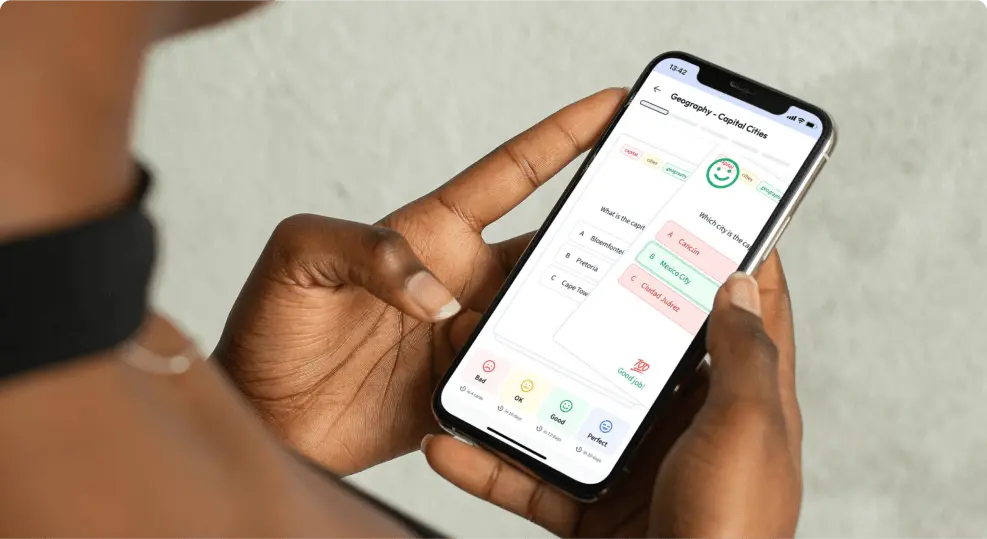
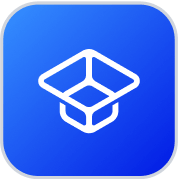
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more