Understanding JavaScript Anonymous Functions
As you delve into your journey of learning programming with JavaScript, you're bound to come across the concept of anonymous functions. For those beginning their coding adventure or even seasoned pros looking to brush up their skills, understanding anonymous functions is key.
Definition of JavaScript Anonymous Functions
JavaScript anonymous functions, as the name implies, are functions that are declared without any named identifier. They are often called function literals or lambda functions due to their unnamed nature.
While their application may seem complex, with good understanding, you'll learn to appreciate their utility in making your code more efficient and maintainable.
In JavaScript, functions are first-class objects, meaning functions are treated just like any other object. This characteristic is what makes anonymous functions, closures, callbacks, and high order functions possible.
Implementing Anonymous Functions in JavaScript
Implementing anonymous functions in JavaScript can be as simple as:
var anonymousFunction = function () { return 'Hello World!'; };
This declaration creates a function that produces the output 'Hello World!'. The function doesn't have a name, so it's anonymous. It's stored in the variable anonymousFunction, which can be invoked by calling the variable followed by parentheses, like this:
anonymousFunction();
JavaScript also provides other ways to define anonymous functions, which includes Arrow Functions introduced in ES6.
Examples of JavaScript Anonymous Functions
Let's take a look at a few examples to see the power of anonymous functions.
Here's an example where we pass an anonymous function as a parameter:
setTimeout(function(){ alert('This is the anonymous function at work!'); }, 2000);
In this example, an anonymous function is passed to the setTimeout function. The anonymous function pops up an alert box that says "This is the anonymous function at work!" after a delay of 2000ms (equal to 2 seconds).
Ponder over these examples, practice them in your JavaScript console, and very soon, you'll be a pro at using anonymous functions!
A crucial aspect of mastering JavaScript or any programming language is understanding core concepts like Anonymous Functions and their application, which can make your code more proficient and potent. So, keep exploring, and happy coding!
Distinguishing Anonymous Functions in JavaScript
JavaScript, like many other programming languages, offers various ways to define functions. As you progress with JavaScript, you'll frequently encounter not just named functions but also anonymous functions and arrow functions. In essence, these are all functions, with some distinct features and uses that separate them. Understanding these differences is imperative to choose the right function type for your coding solutions.
Anonymous vs Named Function JavaScript: A Comparison
In JavaScript, a function can be defined in two ways: named functions and anonymous functions. Understanding the differences between them would provide a comprehensive grasp of when to utilise each type.
A Named Function in JavaScript is a function that, as the name implies, has a definite name when defined. It is declared using the function keyword followed by the name of the function. Here is an example of a named function:
function greet() { console.log('Hello World!'); }
Here, 'greet' is the name of the function. You can call this function using the name followed by parentheses 'greet()'.
On the other hand, an Anonymous Function in JavaScript is a function without a name. These function expressions are often declared with the function keyword, followed by a set of parentheses containing zero or more parameters, followed by a pair of curly brackets that enclose the body of the function. Here is an example:
var greetAnonymously = function() { console.log('Hello, world!'); }
The distinguishing factor here is that this function does not have a name. It gets assigned to a variable, and that variable is then used to call the function.
Features and Differences
Let's shed some light on the characteristics that differentiate these two types of functions.
- Naming: Anonymous functions don't have a name, unlike named functions.
- Hoisting: Named functions are hoisted, which means you can call them before they are defined. This is not the case with anonymous functions.
- Use Case: Anonymous functions are primarily used as arguments for other functions or as immediately invoked function expressions (IIFEs).
Difference between Arrow Function and Anonymous Function in JavaScript
Arrow functions, introduced in ECMAScript 6 (ES6), are another way to declare functions in JavaScript. They can be seen as a more concise syntax for writing function expressions. They omit the need to use the 'function' keyword, use fat arrow \( => \) to denote a function, and offer more nuanced differences when compared to anonymous functions.
An Arrow Function has a syntax shorter than function expressions and lexically bind the this value. Arrow functions are anonymous and change the way this binds in functions.
let arrowGreet = () => { console.log('Hello, world!'); };
This function is identical to the one defined using the function keyword. There are a few key differences:
Features and Differences
- Syntax: Arrow functions have a shorter syntax compared to anonymous functions.
- this Keyword: In arrow functions, the this keyword behaves differently. It's bound lexically i.e., "this" retains the value of the enclosing lexical context's this. In global scope, it will be set to the global object: window.
Let's summarize the differences in the table below:
Criteria | Arrow Functions | Anonymous Functions |
Syntax | Shorter | Longer |
Use of this keyword | Lexical binding | Dynamic |
Arrow functions can result in shorter and cleaner code but understanding when to use which function type will make you a proficient JavaScript programmer. Each has its place, and understanding their nuances is foundational to mastering JavaScript.
Practical Uses of JavaScript Anonymous Functions
JavaScript Anonymous Functions, despite their obscure name, are a powerful tool in every programmer's tool kit. These functions come in handy in a variety of scenarios, whether you're dealing with higher-order functions, callbacks, or want to encapsulate logic not needed elsewhere.
How to Use Anonymous Function in JavaScript
To understand the utility of anonymous functions, you need to dive deep into the different ways you can use anonymous functions in JavaScript. Let's start with the basic syntax of JavaScript anonymous functions: they are declared using the keyword 'function', followed by a set of parentheses that can contain parameters, followed by a pair of curly brackets that enclose the function's body.
var myFunction = function() { console.log('Hello, World!'); }
The function in the example above does not have a name, and it's stored in the variable 'myFunction'. However, the most interesting aspect of anonymous functions lies in their ad-hoc nature – they can be declared just in time to be used, and discarded right after.
One common use of anonymous functions is as arguments in higher-order functions. In JavaScript, functions are objects, and just like other objects (strings, numbers, arrays, etc.), they can be passed as arguments to other functions. This can enable some powerful programming paradigms, including functional programming and callback-driven programming.
['John', 'Jane', 'Jim'].forEach(function(name) { console.log('Hello, ' + name + '!'); });
In the above example, an anonymous function is passed to the 'forEach' function, which is a higher-order function. The anonymous function is invoked for each element in the array, printing a special greeting for each name.
Employing Anonymous Callback Function in JavaScript
Another crucial use of anonymous functions is as callbacks in JavaScript. In simple terms, a callback function is a function that is passed as an argument into another function, to be called (or executed) later. The callback function can be a named or an anonymous function. However, it's a popular practice in JavaScript to employ anonymous functions for callbacks since callbacks are often one-off functions that have a specific purpose for the host function.
In asynchronous JavaScript programming, anonymous callback functions are common, especially when dealing with events or asynchronous data retrieval. For instance, whenever you fetch data from a server using AJAX, you usually provide a callback function to execute when the response from the server is ready.
$.get('server/data', function(response) { console.log('Data received: ' + response); });
In the code snippet above, 'function(response)' is an anonymous callback function. This function will be called when data responses return from the server.
Common Scenarios for Implementing Javascript Anonymous Functions
The beauty of anonymous functions lies in their flexibility, which allows for quick, ad-hoc function creation. This aspect is exceptionally beneficial in scenarios that do not require reuse of function logic, hence eliminating the need for named functions.
- Event Handling: JavaScript anonymous functions shine in event handling. Events such as button clicks or mouse movements can be managed using anonymous functions without naming the function.
- Callback Functions: Anonymous functions are the preferred choice when developing callback functions owing to their versatile nature.
- Self-invoking Functions: These are anonymous functions that run automatically/immediately after they’re defined. Also known as Immediately Invoked Function Expression (IIFE), they’re created by adding a parenthesis at the end of the function.
- Closures: By combining anonymous functions with outer function scope, closures allow private state to be bundled and controlled.
Consider the impact of anonymous functions and how they have changed programming norms. While they're potent tools, remember that named functions have their benefits, including better readability and debugging. Finding the balance based on your coding context is paramount to developing effective, efficient code.
Javascript Anonymous Functions - Key takeaways
- JavaScript Anonymous Functions: These are functions declared without any named identifier, also known as function literals or lambda functions. They often act as arguments passed to higher-order functions or are used for constructing the results of a higher-order function that needs to return a function.
- Named vs Anonymous Functions in JavaScript: Named functions in JavaScript have a defined name at the time of declaration, whereas anonymous functions do not have a name. Named functions can be called before they are defined, a characteristic known as hoisting. Meanwhile, anonymous functions are often used as arguments for other functions or as immediately invoked function expressions (IIFEs).
- Arrow Functions vs Anonymous Functions in JavaScript: Arrow functions, introduced in ECMAScript 6 (ES6), offer a more concise syntax for writing function expressions compared to anonymous functions. In particular, the 'this' keyword in arrow functions binds lexically and retains the value of the enclosing lexical context's 'this'.
- Usage of JavaScript Anonymous Functions: Anonymous functions are primarily used as arguments in higher-order functions or as callbacks, often providing ad-hoc solutions to problems. They are also used widely in event handling and server data retrieval since they can be declared just at the moment of their usage and discarded afterward.
- Common Scenarios for Implementing JavaScript Anonymous Functions: Some common applications of JavaScript anonymous functions include event handling, callback functions, immediate invocation (self-invoking functions), and closures.
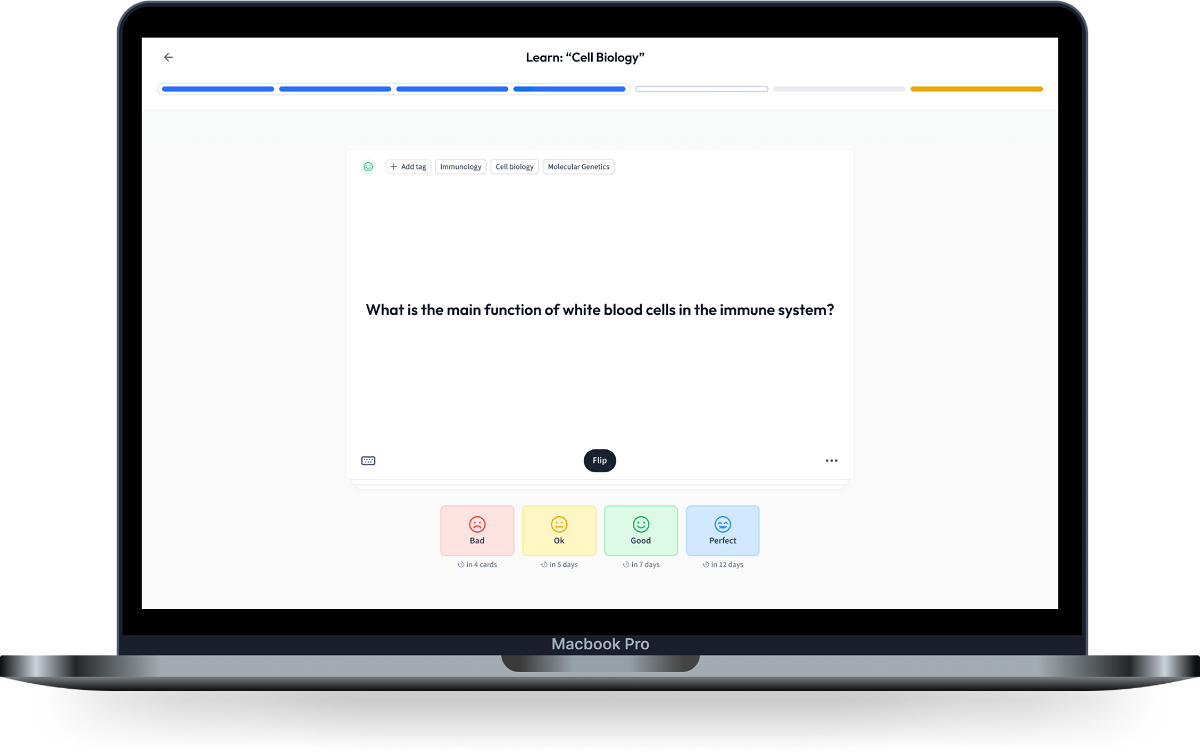
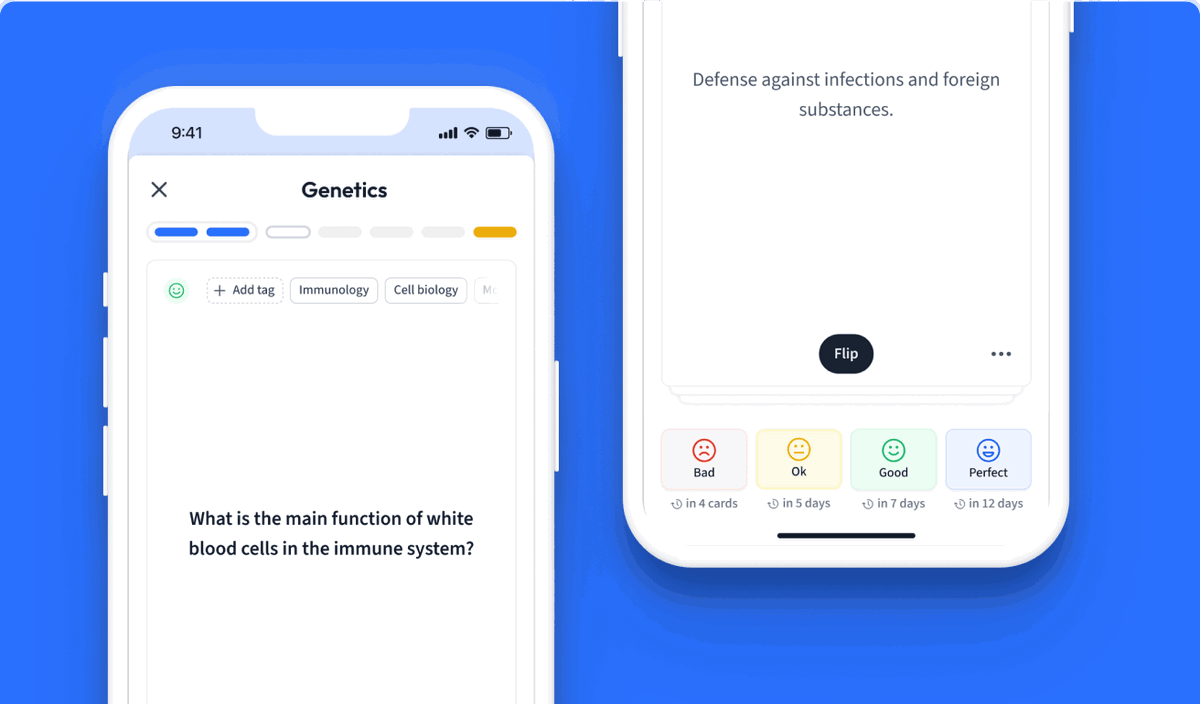
Learn with 12 Javascript Anonymous Functions flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Javascript Anonymous Functions
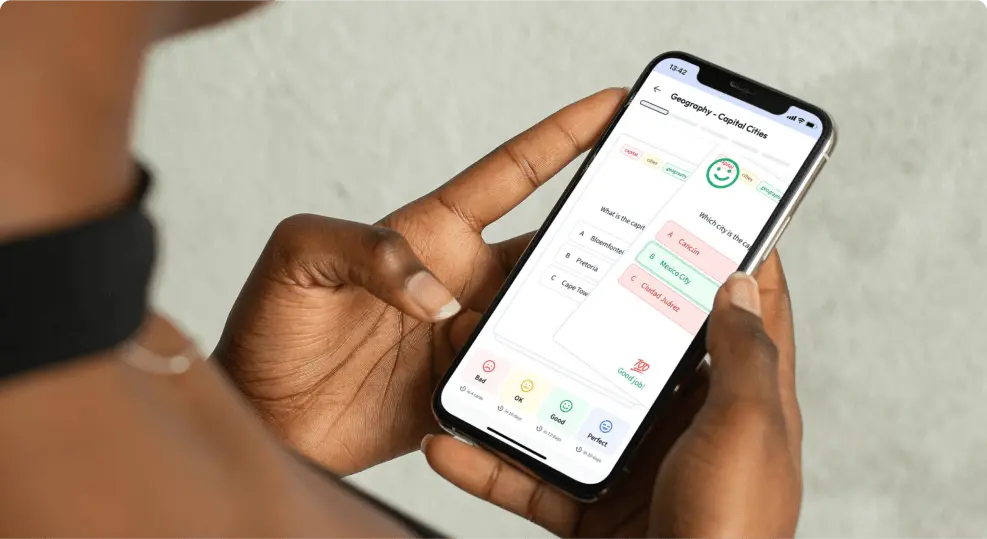
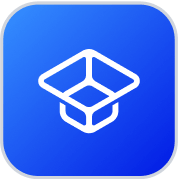
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more