Understanding Javascript Hoisting
Javascript Hoisting is a default behaviour of JavaScript where all the declarations (whether variables or functions) are moved or 'hoisted' to the top of their local scope or the top of their global scope during the compilation phase, before the code has been executed. It allows you to call functions before they appear in the code.
In simpler terms, when a variable is declared and not initialised, the JavaScript compiler understands this as undefined. Similarly, any function or variable can be used before it is declared, as they are hoisted to the top of their scope. This phenomenon is referred to as hoisting.
Primary Concepts of Hoisting in Javascript
A critical point you should remember is that only the declarations are hoisted, not the initialisations. This means if a variable or function is declared and initialised after using it, the value will be undefined.
A variable can be declared in three ways in JavaScript. These are:
- var
- let
- const
Each declaration has its hoisting behaviour, which can be represented by the table below:
Declaration | Hoisting |
var | Hoisted |
let | Not hoisted |
const | Not hoisted |
By using var, the variable will be hoisted to the top of its scope and initialised with a value of undefined. But with let and const, the variable is in a temporal dead zone. This means it is uninitialised and any reference to it will result in a ReferenceError, due to it not being hoisted. To show this, you can use the following codes:
console.log(myVar); //undefined var myVar; console.log(myLet); //ReferenceError let myLet;
When it comes to function hoisting, function declarations are hoisted completely to the top of their scope, unlike variable declarations.
Consider the following example:
console.log(myFunction()); // 'Hello, world!' function myFunction() { return 'Hello, world!'; }
Even though the function is called before the function declaration, it still returns 'Hello, world!'. That's because the function declaration is hoisted to the top of the scope.
Comprehensive Account of Hoisting in JavaScript
Despite hoisting being a complex concept, it is integral for JavaScript's execution context and plays a significant role in producing cleaner and more readable code. By understanding hoisting, you equip yourself with the ability to avoid common pitfalls and errors in coding.
One popular myth to dispel is that hoisting physically moves your code to the top of JavaScript file. This isn't true. As discussed previously, hoisting occurs at the compilation phase, so the compiler merely assigns memory space for variable and function declarations.
Therefore, the physical order of your code doesn't change, just how the JavaScript interpreter views it.
The behaviour of the JavaScript interpreter concerning hoisting can be briefly summarised as:
- Variable and function declarations are put into memory during the compile phase.
- Variable declarations default to undefined, but keep their place and wait for execution.
- Complete functions with their bodies get hoisted.
- Using a variable before it's declared results in a ReferenceError.
So, when you're writing JavaScript code, always keep in mind hoisting and its impact on your declarations and initialisations. It is recommended to always declare your variables at the top of your scope.
Exploring Functions and Hoisting in Javascript
When working with Javascript, it is important to understand how the language handles both functions and variables. One of the fascinating features of Javascript is its phenomenon of 'hoisting'. Unlike other languages, Javascript tends to behave differently when dealing with function declarations and expressions, notably due to this concept of hoisting.
Are Functions Hoisted in Javascript Explained
In Javascript, the theory of hoisting is just as applicable to functions as it is to variables. The interpreter moves the declarations to the top of the current scope, meaning that you can call functions before they are defined in the code block. However, this can be somewhat confusing if you don't understand how it works. Hence, it's crucial to distinguish between two types of functions within Javascript - function declarations and function expressions.
A function declaration defines a function without requiring variable assignment. It is of the form:
function myFunc () { //do something }
A function expression defines a function as part of a larger expression syntax (typically variable assignment). For instance:
var myFunc = function() { //do something };
So, what is the difference in hoisting between these two? Well, it is significant. Function declarations are completely hoisted, meaning that the entire function block is moved to the top of the scope. Thus, if you have a function declaration in your code, you can call it whenever you want, irrespective of its location in your code.
An Insight into Function Hoisting in Javascript
Now, you may think that since function declarations move to the top of the scope, function expressions should do the same. That is, however, not the case. When using a function expression, hoisting does occur, but it's restricted to only the variable declaration, not the assignment. Therefore, if a function expression is invoked before declaration, you'll receive an error stating it's not a function.
Consider this example:
console.log(myFunction()); // myFunction is not a function var myFunction = function() { return 'Hello, world!'; };
But why does Javascript have this odd behaviour? Well, Javascript uses a two-pass interpreter. The first pass scans for all the declarations and hoists them to the top of their respective scopes. During this phase, the initialisations are not hoisted. In function expressions, the function is assigned to a variable. The declaration (variable) is hoisted, but the assignment (function) is not.
Here are some key aspects to remember about hoisting and functions:
- Function declarations and variable declarations are always hoisted.
- Variable assignments and initialisations are not hoisted.
- Function expressions result in undefined when called before they are evaluated due to hoisting.
This dichotomy assures proper execution context and maintains the consistency of the Javascript language. To avoid unexpected results, it's advisable to declare and initialise variables at the start of your scripts and to call functions after their declaration or simple assign them to variables if you wish to utilise them earlier in your scripts, assuring predictability in your code's outcome.
Unravelling Javascript Class Hoisting
As you may know, Javascript introduces hoisting behaviour in relation to functions or variable declarations. Here, you'll dive into an intriguing concept known as 'Class Hoisting'.
In-depth Analysis of Class Hoisting in Javascript
In some languagues, classes act similarly to functions or variables in the context of hoisting. But in Javascript, the scenario is somewhat different. The interesting aspect of understand 'class hoisting' in JavaScript is that unlike variables and function declarations, class declarations are not hoisted. If you try to access your class before declaring it, JavaScript will throw an error.
A class is a type of function in JavaScript, but defined with the keyword 'class'. An important distinction to remember is that classes in Javascript are block-scoped like 'let' and 'const' variables.
console.log(myClass); //ReferenceError class myClass{};
You'll notice this deviates from function behaviour where you can use functions before the declaration because of automatic hoisting behaviour. This deviation is due to the nature of the 'class' keyword. Much like 'let' and 'const', 'class' declarations are block-scoped and thus can't hoist to the top of their enclosing block.
However, classes, like functions, have a slightly more detailed story due to the existence of 'class expressions', which mirror the functionality of 'function expressions' and 'function declarations'. But unlike function expressions, class expressions are not hoisted.
A class expression is yet another way to define a class in JavaScript. Class expressions can be named or unnamed. The value of the name property of a function is used within the class to call static methods.
var MyClass = class Klass { // methods go here }; console.log(Klass); //ReferenceError: Klass is not defined
The class expression here is named 'Klass'. However, the scope of 'Klass' is limited only within the class body, which respects the principle of data encapsulation where objects hide their properties and methods.
Understanding Class Hoisting in the Context of Javascript
When talking about JavaScript hoisting, 'var', 'let', 'const', function declarations, function expressions, and now classes come into the discussion. It's vital to realise how all elements behave within the larger scope to avoid bugs and maintain clean, efficient code.
Just like 'let' and 'const', class definitions are block-scoped. They're not hoisted to the top of your code. Attempting to access the class before its declaration will result in a ReferenceError. This error is rendered because JavaScript classes, unlike functions, are not initialised with a space in memory. They, therefore, do not have a value until evaluation during the execution of the script.
Below is a demonstration of class hoisting v/s function hoisting:
console.log(myFunction()); // 'Hello, world!' console.log(myClass); // ReferenceError - cannot access 'myClass' before initialization function myFunction() { return 'Hello, world!'; } class myClass {};
Given their hoisting characteristics, classes should be declared at the start of the block where they're expected to be referenced. While this seems restrictive, remember that good coding practices encourage this kind of structure. By putting all of your declarations at the top of their respective scopes, you allow your code to be clear, maintainable, and less prone to pesky bugs.
The Role of Variable Hoisting in Javascript
In Javascript, the term 'hoisting' is often mentioned in respect to the behaviour of variables. This is a key concept to understand when writing code, as it can easily be a source of confusion for new and even experienced programmers. To get to grips with Javascript's nuances, you need to see variable hoisting in action.
A Close-up on Hoisted Variables in Javascript
In a standard programming language, the idea is simple: you declare a variable, then you use it. But Javascript throws a little curveball called hoisting. Essentially, Javascript "hoists" or lifts all the variable declarations (not initialisations) to the top of the current scope, be it a function or a global scope.
A variable declaration is that moment when Javascript is told that a variable exists, by using 'var', 'let' or 'const', often paired with the assignment of a value.
The surprising aspect of variable hoisting is that it allows the use of a variable before it is technically declared in the code. This can cause unexpected behaviours in your program if you don't anticipate it.
For instance, observe the code below:
console.log(x); // undefined var x = 5; console.log(x); // 5
Even though x is declared after the first console.log statement, it doesn't result in an error because the declaration (not initialisation) is hoisted to the top of the scope.
However, it's important to note that the hoisting mechanism works differently for 'var', 'let', and 'const':
- 'var' declarations are hoisted and initialised with a value of 'undefined'.
- 'let' and 'const' declarations are hoisted but remain uninitialised. Hence, referencing them before declaration results in a ReferenceError.
For example:
console.log(y); // ReferenceError: y is not defined let y = 5;
While variable hoisting in Javascript can be slightly complex, it forms the basis of understanding function and class hoisting and is a critical feature of the language.
The Mechanism of Variable Hoisting in Javascript
A more in-depth understanding of how Javascript processes variable hoisting requires diving into the compilation and execution phases. It's easy to think that Javascript is a purely interpreted language, but it undergoes a two-step process: Compilation and Execution.
In the Compilation phase:
- Javascript scans the entire code looking for variable and function declarations.
- It allocates memory for the declared variables and functions, creating a reference to them in Javascript's execution context.
- 'var' type variables are assigned a default value of 'undefined'. In contrast, 'let' and 'const' remain uninitialised.
- This is only for the variable declarations, not initialisations.
In the Execution phase:
- It executes the code line by line from top to bottom.
- It assigns values to the variables and executes the functions as it encounters them during this phase.
- 'let' and 'const' variables are only now available for use after their line of code has executed, unlike 'var' type variables that were available from the start.
Thus, the two-stage process of Compilation and Execution in Javascript is what makes variable hoisting possible.
Consider the example below:
console.log(z); // undefined var z = 5; // variable initialisation console.log(z); // 5
Even though we attempted to log 'z' before it was declared, this didn't result in an error, just 'undefined'. This 'undefined' is the default initialisation that 'var' gets due to its hoisting. Only during the execution phase does 'z' receive the value of '5'.
The hoisting mechanism is a fascinating feature of Javascript, albeit one that can lead to puzzling outcomes if not fully understood. Through variable hoisting, you can obtain a clearer comprehension of the compilation and execution phases in Javascript.
Practical Illustrations of Javascript Hoisting
In Javascript, the hoisting mechanism can create some seemingly unusual behaviours if not properly understood. It's a key difference that sets apart JavaScript from the majority of other computing languages. But fear not, grasping this concept is a definite aid in debugging and writing clean code.
Hoisting Examples: How Does Javascript Behave?
Let's dive into understanding the way Javascript behaves when it comes to variable and function declarations. Once you see it in action, the anomalistic design of hoisting becomes a lot more comprehensible.
Firstly, here's how hoisting treats 'var' declared variables:
console.log(aVar); // undefined var aVar = 5;
In this example, JavaScript hoists the 'var' variable declaration to the top of the scope, even though it's declared after the first console.log reference. This results in 'undefined' rather than an error.
Now, observe how hoisting differs with 'let' and 'const' declarations:
console.log(aLet); // ReferenceError let aLet = 5;
Unlike 'var', 'let' and 'const' are hoisted but not initialised, so trying to access them before their line of declaration throws a ReferenceError (not undefined).
Beyond varying variables, function expressions and declarations too have noteworthy behaviours:
console.log(namedFunction()); // 'Hello, world!' function namedFunction() { return 'Hello, world!'; }
Function declarations, like 'var' variables, are hoisted and initialised. Consequently, you can call the function before it's declared, and it won't result in an error.
Conversely, function expressions present a different scenario:
console.log(namedFunctionExpression()); // TypeError var namedFunctionExpression = function() { return 'Hello, world!'; };
Function expressions fall under the same hoisting rules as 'var'. The function expression is hoisted, but the function's value isn't initialised until Javascript reaches the line where the function is assigned. So an attempt to invoke them prior to their assignment leads to a TypeError.
These illustrations underline how hoisting behaves in various contexts within Javascript. Being aware of these behaviours is vital to avoiding potential errors or unexpected outcomes in your scripts.
Decoding Hoisting Through Javascript Examples
Several practical examples help clarify the specific hoisting behaviours in Javascript. Given these examples, you'll understand how Javascript handles variable and function declarations during the compilation phase.
Consider the hoisting of 'var' inside a function scope:
function hoistTest() { console.log(hoistVar); // undefined - Not a ReferenceError var hoistVar = "I'm hoisted"; console.log(hoistVar); // "I'm hoisted" } hoistTest();
A 'var' variable, when declared inside a function, obeys function-scoping. Its declaration is hoisted to the top of the function scope. Hence, the first console.log results in 'undefined', not an error. But obviously, the variable's initialisation occurs only at its line of code, making the variable's value accessible afterwards.
In contrast, 'let' and 'const' have block-scoping:
if(true) { console.log(hoistLet); // ReferenceError let hoistLet = "I'm hoisted"; }
'let' and 'const' declarations remain unaccessable unitl Javascript hits the line where they're declared. Attempting to use them before their line of declaration causes a ReferenceError, even if they're inside an 'if' block or any other block.
Be vigilant of hoisting nuances when you have function declarations and function expressions:
console.log(hoistFunction()); // "I'm hoisted" function hoistFunction() { return "I'm hoisted"; }
Function declarations are fully hoisted. Hence, you can call a function above its declaration without running into an error.
But beware of function expressions with 'var':
console.log(hoistFunctionExpression()); // TypeError var hoistFunctionExpression = function() { return "I'm hoisted"; };
A function expression has the same hoisting principle as 'var': the declaration is hoisted, not the initialisation. Thus, invoking the function before it's assigned results in a TypeError, not a return value.
These examples should provide you with a transparent understanding of how hoisting works in Javascript. The comprehension of the concept makes it easier to predict the outcomes, debug issues, and write more efficient coding practices.
Javascript Hoisting - Key takeaways
- Javascript Hoisting occurs at the compilation phase where memory space is assigned for variable and function declarations.
- Hoisting in Javascript affects the interpretive view of the physical order of the code without changing the actual order.
- Hoisting applies to both functions and variables in Javascript, moving the declarations to the top of the current scope. This allows calling of functions before they are defined in the code block.
- In Javascript, function declarations get completely hoisted, thus can be called irrespective of their location in the code. Function expressions however are only hoisted to the point of variable declaration, not assignment.
- Variable declarations in Javascript are hoisted and initialised with 'undefined' if 'var' is used, while 'let' and 'const' declarations are hoisted but remain uninitialised resulting in a ReferenceError if accessed before declaration.
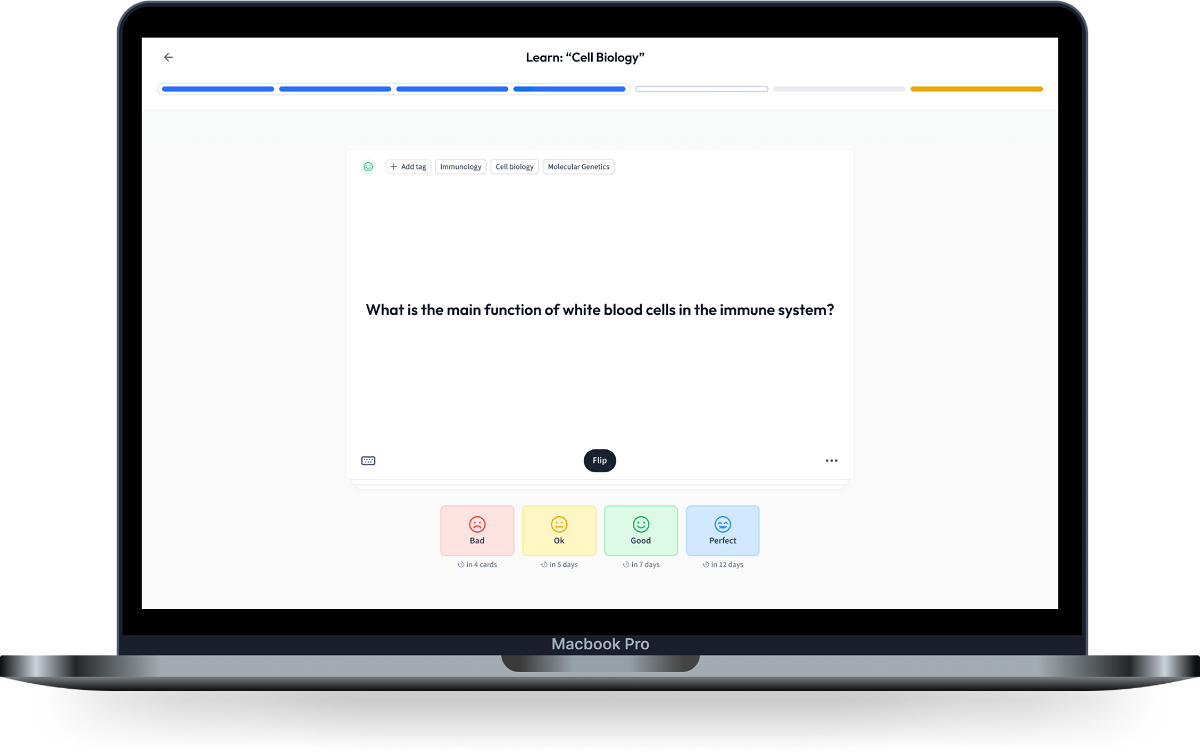
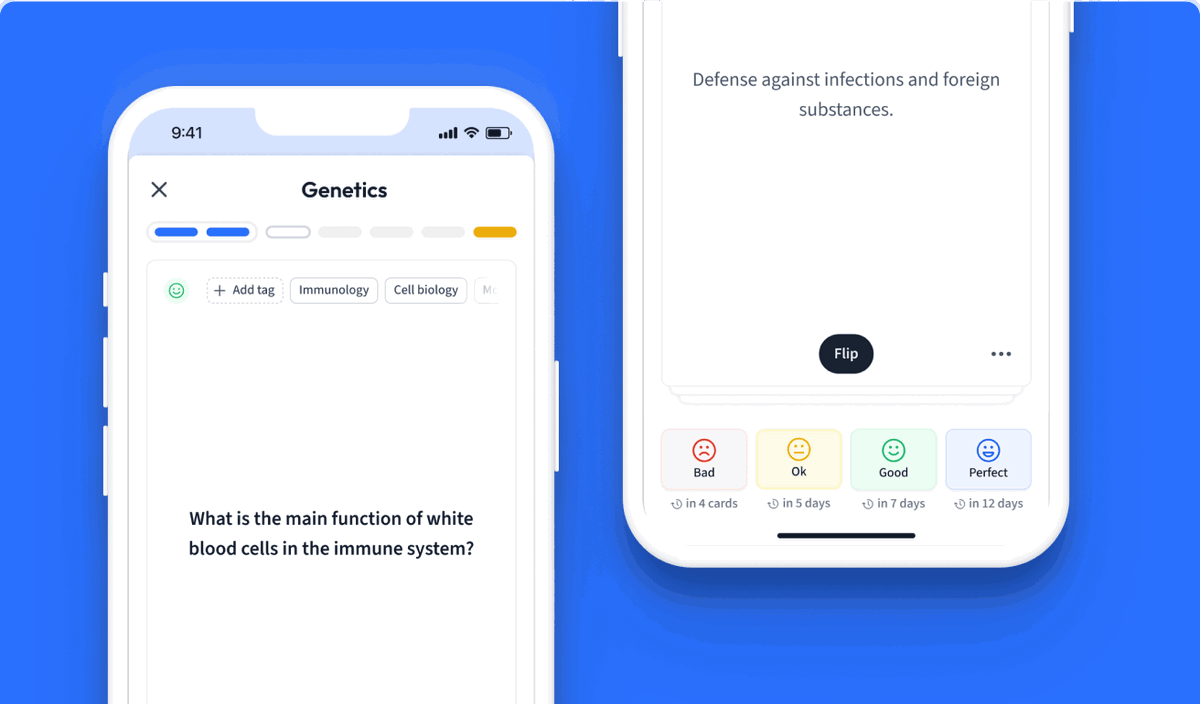
Learn with 15 Javascript Hoisting flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Javascript Hoisting
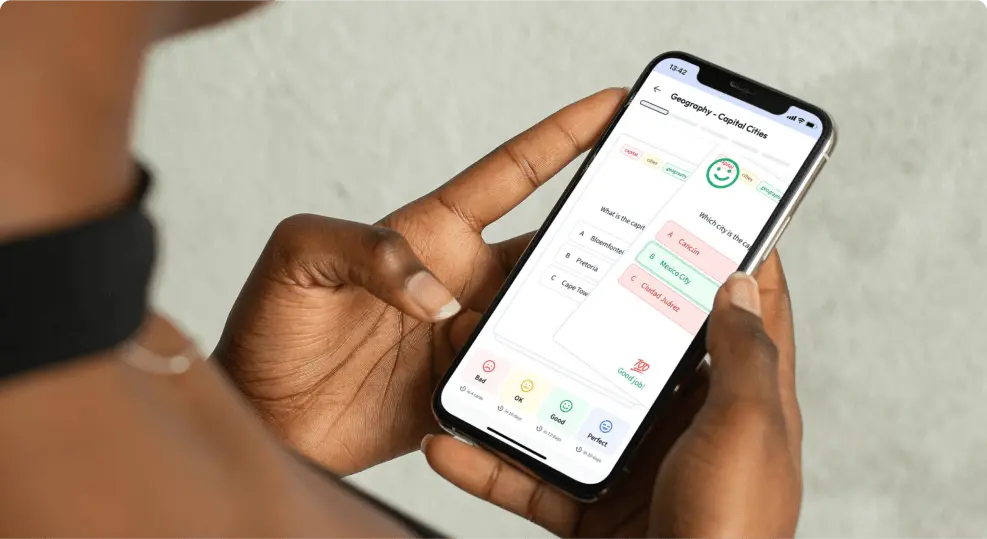
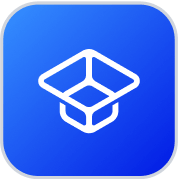
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more