Understanding the Concept of Java Abstraction
The concept of Java Abstraction is a primary and fundamental principle in object-oriented programming languages such as Java. In simple terms, abstraction is a process of hiding the complex implementation details from the user and only providing the functionality to them. This establishment of a simple interface to the complex systems not only enhances user's interaction with the system but it also provides a mechanism to protect the underlying code and data.
Defining Java Abstraction in Computer Science
In the sphere of computer science and programming languages, Java Abstraction has a specific and important definition. Java abstraction is a process that lets you focus on the essential features of an object by hiding non-essential details. Abstraction describes every object in terms of its characteristics and behaviours.
Java Abstraction is a technique in object-oriented programming that hides implementation details, allowing programmers to view objects in terms of their types and interfaces instead of their underlying code.
The process of abstraction in Java works as follows:
- Abstraction is achieved through either abstract classes or interfaces
- An abstract class can have methods, variables, and constructors that are open to inheritance.
- You cannot create an instance of an abstract class. You can only create objects of its subclasses.
- If a class includes abstract methods, then the class must be declared abstract as well.
public abstract class AbstractDemo { public abstract void display(); } public class Demo extends AbstractDemo{ public void display(){ System.out.println("Hi! This is an example."); } }
In an abstract class, you can provide complete, default code and, or you can just define the method signatures without a body, also known as abstract methods.
The Fundamental Role of Java Abstraction in Computer Programming
The role of Java Abstraction in computer programming is of prime importance. It plays a pivotal role in managing code complexity, as abstraction allows you to represent complex systems in a simplified manner. Moreover, it allows programmers to reduce redundancy and increase code reusability.
Let's consider a real-world example. When you use a laptop, you are not exposed to the complex operations happening behind the screen. Rather you use its functionalities - typing, browsing, playing, etc. The internal system complexities are abstracted away from you. Similarly, when you write Java programs, you abstract out the complex details and provide the users with the functions they need.
Here's a brief overview of benefits of Java Abstraction:
Code readability | Implementation details are hidden, making the code easier to read and understand. |
Data hiding | The user does not have access to the internal working of the method, enhancing security. |
Code reusability | Abstract classes and interfaces encourage code reuse. |
Java Abstraction is undeniably an essential principle in object-oriented programming and the foundation for many advanced topics. Understanding it is crucial to becoming an efficient and effective Java programmer.
Delving into Java Abstract Class
A Java Abstract Class is one that is declared using the keyword 'abstract'. This type of class is primarily used to encapsulate a common structure and behaviour of subclasses and cannot be instantiated on its own. But what does it mean, and why is it important? Let's dig deeper into these classes.
What is an Abstract in Java: An Explanation
In Java, an Abstract is a non-access modifier for methods and classes. If something is declared abstract, it cannot be instantiated; instead, it's meant to be subclassed. Specifically, an "Abstract class" is used as a blueprint for other classes, and an "Abstract method" in Java is declared without an implementation—its functionality is provided by methods in subclasses.
abstract class Animal { abstract void sound(); }
In the example given, 'Animal' is an abstract class, and 'sound()' is an abstract method.
Abstract classes and methods deliver certain advantages:
- The usage of abstract class enables reusability of code.
- Abstract methods force the child classes to provide the method implementation, ensuring a certain level of design rigidity.
Differentiating Between Interface and Abstract Class in Java
Note that, while both abstract classes and interfaces are used for abstraction in Java, they're not the same. They serve somewhat different purposes and impose different rules, even though they can seem similar at first.
Key differences include:
Interface in Java | Abstract Class in Java |
Interface can't contain any concrete (implemented) methods | Abstract class can contain both abstract and concrete methods |
All methods in an interface are implicitly public and abstract | Methods in abstract classes can have any visibility: public, private, protected |
Interface can't have instance variables | Abstract class can have instance variables |
A class can implement multiple interfaces | A class can extend only one abstract class |
Implementing an Abstract Method in Java: A Step-by-Step Guide
After covering what an abstract method in Java is, you might be wondering, "How do we actually use it?" Here is a step-by-step explanation on how to implement an abstract method in Java.
In order to implement an abstract method in Java:
- You need to create an abstract class first, with the abstract method(s).
- Create another class extending the above abstract class.
- Inside this class, provide the implementation of the abstract method.
abstract class Animal { abstract void sound(); }
class Dog extends Animal
void sound() { System.out.println("The dog barks"); }
With these steps, you have successfully implemented an abstract method in Java.
Grasping Java Abstraction through Practical Examples
Java Abstraction is a core principle in Object-Oriented Programming (OOP), acting as a mechanism to hide complex implementation details and present only the necessary functionalities to the user. Seeing this principle play out in some practical examples will facilitate a better understanding of its functionality and significance.
Deconstructing a Java Abstraction Example
Consider an example where you define an abstract class, 'Vehicle', with an abstract method 'travel()'. This method should be implemented in the subclasses that extend 'Vehicle'.
abstract class Vehicle { abstract void travel(); }
In the above code, 'Vehicle' is an abstract class, and 'travel()' is an abstract method. Let's create 'Bicycle' and 'Car' as subclasses that extend 'Vehicle' and provide implementation for the abstract method.
class Bicycle extends Vehicle { void travel() { System.out.println("The bicycle travels with two wheels."); } } class Car extends Vehicle { void travel() { System.out.println("The car travels with four wheels."); } }
In this particular example:
- Vehicle: It is an abstract class containing the abstract method 'travel()'.
- Bicycle, Car: These are concrete classes or subclasses providing the implementation of the abstract method 'travel()', defined in the abstract superclass 'Vehicle'.
In essence:
The 'Vehicle' class has abstracted away the complex implementation details of the method 'travel()' and left it to the individual subclasses to handle. This allows for different ways to travel, depending on the type of vehicle, without having to modify the Vehicle class or its interface to the user.
Step-by-Step Application of Abstract Method in Java
To understand Java Abstraction thoroughly, let's delve into a complete example and explain how the abstraction process occurs step by step in Java. Suppose we are having a class hierarchy for geometric shapes where we have an abstract class 'Shape' and other subclasses 'Circle', 'Rectangle', etc.
- Define the Abstract Class
- Extend the Abstract Class 'Shape' by creating subclasses 'Circle' and 'Rectangle' and provide the implementation for the abstract method 'area()'.
abstract class Shape { abstract double area(); }
class Rectangle extends Shape { double length; double width; Rectangle(double length, double width) { this.length = length; this.width = width; } @Override double area() { return length * width; } } class Circle extends Shape { double radius; Circle(double radius) { this.radius = radius; } @Override double area() { return Math.PI * Math.pow(radius, 2); } }
In this code example:
- The 'Shape' class is an abstract class that includes an abstract method 'area()'.
- The 'Rectangle' and 'Circle' classes are subclasses of 'Shape' that provide their own implementations of the 'area()' method.
- The use of the '@Override' annotation informs the compiler that the following method overrides a method of its superclass.
This way, every 'Shape' object can have a different implementation of the 'area()' method appropriate to the specific type of shape, maintaining the abstraction principle. Plus, adding any new shape to the system would only require extending the 'Shape' class and providing its specific implementation.
The Advantages of Java Abstraction
The principle of abstraction in Java brings valuable benefits to the table, from fostering code reusability to ensuring a robust design structure. It's no brainer that Java abstraction is regarded as a pillar of Object-Oriented Programming.
Enumerating the Benefits of Utilising Java Abstraction
Proficient utilisation of abstraction in Java is paramount while writing professional code. It provides a multitude of advantages that would simply be unattainable without it.
- Code Reusability: Abstract classes in Java become the blueprint for other classes, allowing for a higher level of code reusability. The common features among classes can be encapsulated within an abstract class, eliminating redundant code.
- Secure Code: Java abstraction allows showing only the necessary details to the user and hiding the complex implementation. This aids in retaining the confidentiality of the code, offering a security advantage.
- Robust System Design: Abstract methods are declared in the abstract class and are implemented by its subclasses. This essentially enforces a rigid design structure, enhancing the robustness of a programming system.
- Flexibility: Changes in the abstract class can be easily propagated to all the inheriting classes, thereby increasing flexibility. A single amendment in the parent class brings about change in all subclasses, streamlining the update process.
- Enhanced modularity: As the complex details are hidden, and only relevant data is provided to the user, it makes the code more manageable, increasing its modularity.
Real-world Applications and Advantages of Java Abstraction
Java Abstraction has real-time applications across various domains, providing robust solutions for intricate problems.
For instance, in the area of Database Connectivity (JDBC), Java uses an abstraction layer to enable communication with any database (Oracle, MySQL, DB2, etc.) using the same set of APIs. This means you don't have to know the under-the-hood implementation of each database to work with it. You only engage with a set of standard APIs, and Java manages the rest.
public interface Connection extends Wrapper, AutoCloseable { Statement createStatement() throws SQLException; // ... other methods }
In this example, the method 'createStatement()' is part of the 'Connection' interface, one of the building blocks of JDBC. The client thinks it's just using simple 'Statement' objects to execute queries, but behind the scenes, it uses a vendor-specific driver and complex network code.
Similarly, in Graphical User Interface (GUI) applications, Java AWT and Swing provide an abstract layer on top of the underlying, OS-specific code. This allows developers to focus on designing the interface, while Java handles the communication with the underlying system.
public abstract class Component extends Object implements ImageObserver, MenuContainer { protected abstract void paint(Graphics a0); // ... other methods }
The 'Component' class in AWT includes the 'paint()' method, which works with the OS-specific graphics code to display GUI elements. Abstraction makes it possible for you to create GUI applications without knowing the intricate details of graphic rendering on each operating system.
Moreover, JavaScript Object Notation (JSON) parsing libraries, such as JSON-P, empowers you to parse and generate JSON using a standard set of APIs, regardless of the underlying implementation details.
public interface JsonParser extends Iterator, AutoCloseable { // ... API methods }
Overall, Java Abstraction is a paramount concept that simplifies the handling of complex systems, facilitates secure and manageable coding practices, and promotes reusability, resulting in elegant and efficient design structures.
Java Abstraction - Key takeaways
- Java Abstraction in computer science refers to the process that focuses on essential features of an object by hiding non-essential details. It encapsulates complexity within an object in the form of characteristics and behaviours.
- Abstraction in Java can be achieved through abstract classes or interfaces. The former can have methods, variables, and constructors open to inheritance, but cannot be instantiated. If a class contains abstract methods, it must also be declared abstract.
- A Java Abstract Class is declared using the keyword 'abstract' and is primarily used to encapsulate a common structure and behaviour of subclasses. It cannot be instantiated on its own.
- Abstract in Java is a non-access modifier for methods and classes. An abstract class is used as a blueprint for other classes, whereas an abstract method is declared without an implementation — its functionality is provided by methods in subclasses.
- While both abstract classes and interfaces are used for Java abstraction, they serve different purposes. Notably, abstract classes can contain both abstract and concrete methods and instance variables, can have any visibility, and a class can extend only one abstract class. In contrast, interfaces can't contain any concrete methods or instance variables; all their methods are implicitly public and abstract, and a class can implement multiple interfaces.
- Java Abstraction offers numerous advantages such as code reusability, secure code, robust system design, flexibility, and enhanced modularity. It is used in real-world applications across various domains, including Database Connectivity (JDBC) and Graphical User Interface (GUI) applications.
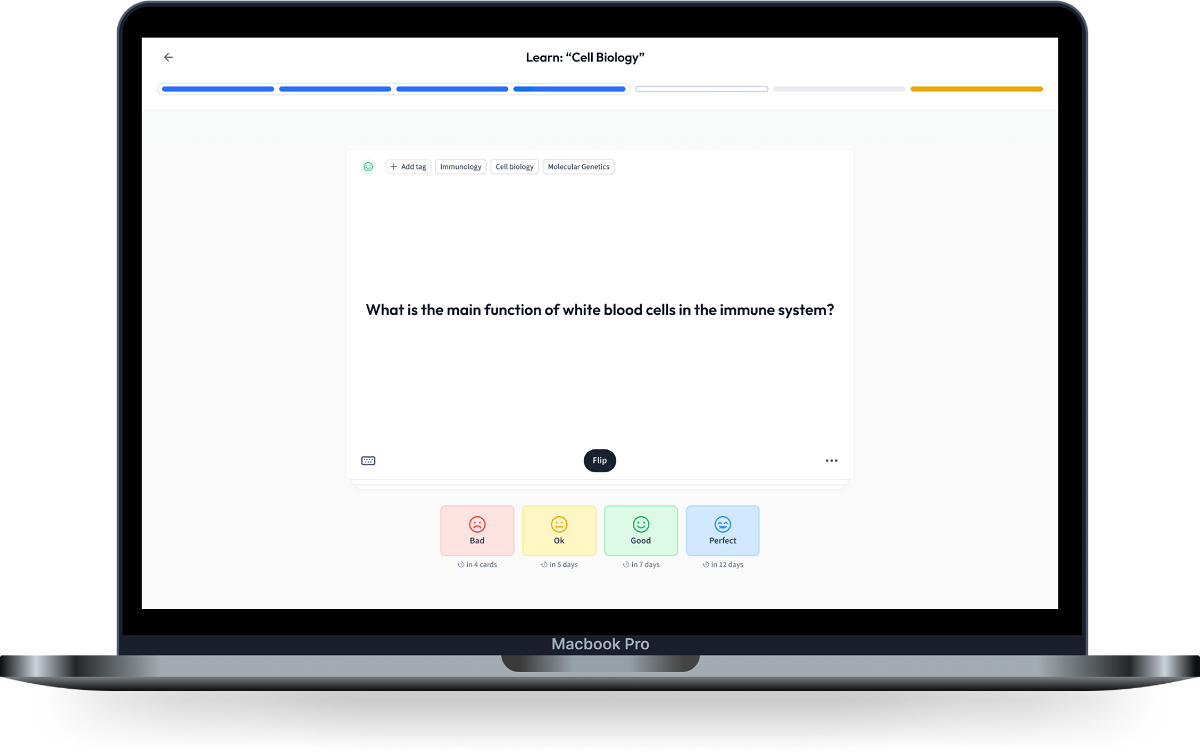
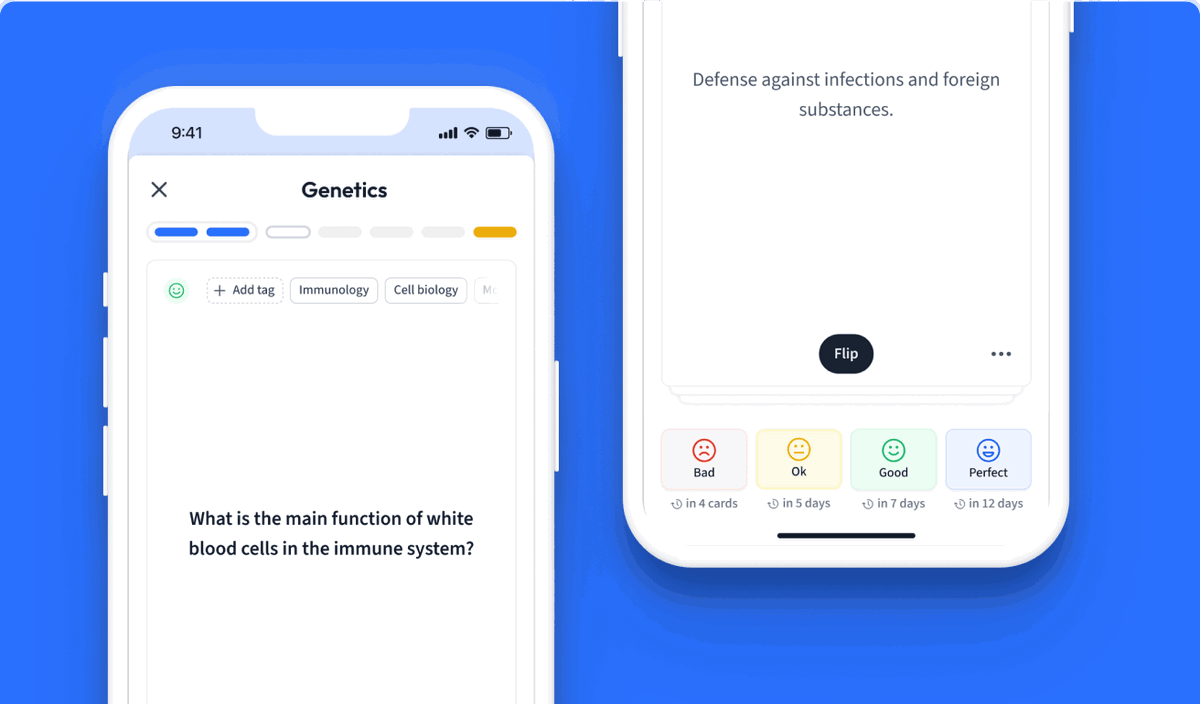
Learn with 12 Java Abstraction flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Java Abstraction
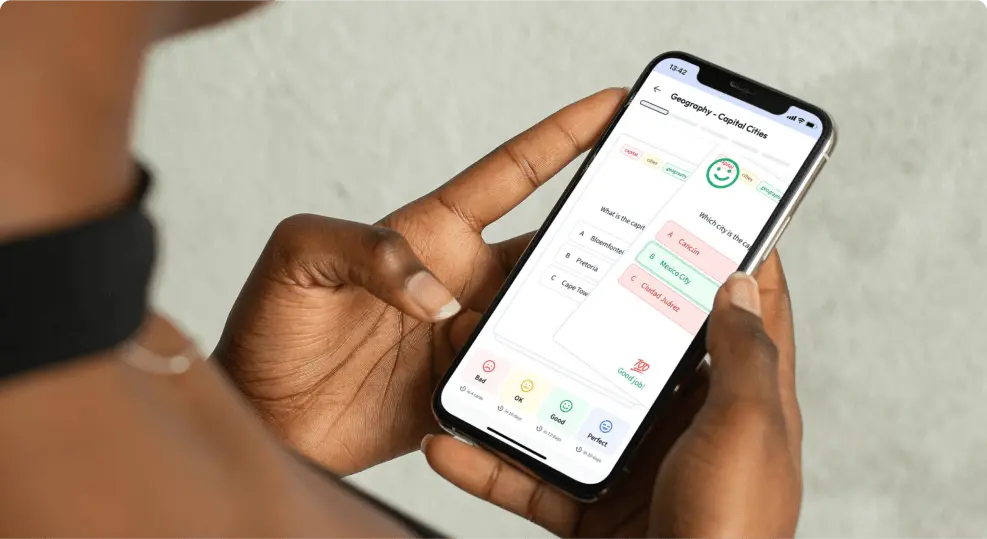
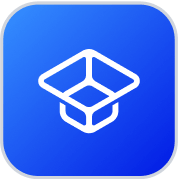
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more