Understanding Javascript Function Definition
In the landscape of modern web development, Javascript functions stand as pivotal elements, enabling developers to write concise, reusable code. This section aims to demystify the concept and characteristics of Javascript functions, offering insights that will help you grasp the fundamentals of this essential programming construct.
What is a Javascript Function?
A Javascript function is a block of code designed to perform a particular task. It's essentially a "subprogram" that can be called by other code. Functions are a fundamental aspect of Javascript, providing a way to encapsulate code for reuse, making your script more modular and maintainable.Function definition in Javascript involves specifying the function keyword, followed by a unique function name, a list of parameters (also known as arguments) enclosed in parentheses, and the function body enclosed in curly braces.
A Javascript function is defined as: A reusable set of statements designed to perform a specific task.
function greet(name) { console.log('Hello, ' + name); } greet('Alice');This example showcases a simple function named greet which takes one parameter, name, and prints a greeting message to the console.
Characteristics of Javascript Function
Javascript functions come with a set of distinctive characteristics that enhance their utility in web development. Understanding these traits will help you leverage functions to their full potential.
- First-Class Objects: In Javascript, functions are treated as first-class objects. This means they can be assigned to variables, passed as arguments to other functions, and even returned from other functions.
- Hoisting: Function declarations are hoisted to the top of their scope. This implies that functions can be invoked before they are defined in the code.
- Closures: Functions in Javascript form closures. A closure is the combination of a function and the lexical environment within which that function was declared. This feature enables function to remember and access variables from its outer scope, even after the outer function has executed.
- Arguments Object: Inside every function, an arguments object is available, providing an array-like list of all the arguments passed to the function.
The concept of first-class objects truly sets Javascript apart from many other scripting languages. Being able to assign functions to variables allows for a level of abstraction and reuse of code that is instrumental in creating sophisticated web applications. This characteristic underlines the importance of understanding and effectively using functions in Javascript.Furthermore, the arguments object provides a rich array of possibilities for functions. It allows functions to accept any number of arguments, offering flexibility in how functions are called and interacted with. This characteristic enhances the dynamism of function usage in complex scenarios.
Types of Javascript Function
Javascript is renowned for its flexibility, offering various types of functions to suit different programming scenarios. Each type of function has its unique features and benefits, making it essential to understand the differences to write efficient and readable code.
Named vs Anonymous Functions in Javascript
In Javascript, functions can be categorised into named and anonymous functions. Understanding the distinctions between these two types is crucial for effective function use and management in your code.
Named functions are declared with a specific name. This name is used to refer to the function in different parts of the code, making it easier to debug and understand the function's purpose. On the other hand, anonymous functions are not given a name. Instead, these functions are often used as arguments to other functions or assigned to variables.
function namedFunction() { console.log('This is a named function.'); } var anonymousFunction = function() { console.log('This is an anonymous function.'); };This example illustrates both a named function and an anonymous function in Javascript.
One of the primary advantages of named functions is their stack traceability. In debugging, named functions provide clearer stack traces, making it easier to identify the source of errors. Anonymous functions, while offering the benefits of flexibility, especially in functional programming patterns, may lead to more challenging debug scenarios.An important consideration is the execution context of these functions. Named functions are hoisted, meaning they are processed before any code is executed. Meanwhile, anonymous functions, assigned to variables, follow the variable hoisting rules, which may affect when and how they are used.
Arrow Functions: A Modern Twist
Introduced in ES6, arrow functions provide a more concise syntax for writing functions in Javascript. They are particularly useful for short, single-operation functions, often seen in functional programming or callbacks.
Arrow functions are defined using a new syntax that includes the arrow symbol (=>). They can be both anonymous or stored in variables, but they do not have their own this, arguments, super, or new.target bindings.
const greet = name => console.log(`Hello, ${name}!`); greet('Alice');This example showcases a simple arrow function that takes one parameter and uses template literals to print a greeting message.
Arrow functions are not suited for all situations. For instance, they cannot be used as constructors and their 'this' context is lexically scoped, meaning it uses 'this' from the enclosing execution context.
Immediately Invoked Function Expressions (IIFE)
An Immediately Invoked Function Expression (IIFE) is a function that runs as soon as it is defined. This pattern is beneficial for creating a private scope and avoiding pollution of the global namespace.
(function() { var privateVar = 'secret'; console.log('Inside IIFE: ' + privateVar); })();This IIFE contains a variable that is not accessible outside of its scope, illustrating how IIFE can be used to encapsulate and protect variables.
Using IIFE in Javascript programming provides an excellent way to execute functions immediately and to maintain clean and uncluttered global namespace. This is especially useful in larger applications, where variable and function name clashes can become problematic. Additionally, IIFEs can be used to manage asynchronous code execution, making them a versatile tool in a Javascript developer's arsenal.Another interesting use-case of IIFEs is in the modular design pattern, where they can encapsulate module logic and expose only public methods and properties, mimicking the behaviour of traditional classes in object-oriented programming.
Javascript Function Examples and Applications
Delving into the practical side of programming, this section covers essential examples and applications of Javascript functions. By exploring these practical scenarios, you'll gain a deeper understanding of how functions operate within the context of real-world projects. Whether it's manipulating arrays or integrating functions into larger applications, the versatility of Javascript functions becomes evident.
Basic Javascript Function Example
Understanding the basic structure and execution of a Javascript function is fundamental in learning to code. Let's look at a straightforward example to illustrate how a Javascript function is defined and called.
A basic Javascript function consists of the function declaration, a set of parameters, and a function body that encapsulates the code to be executed.
function addNumbers(a, b) { return a + b; } var result = addNumbers(5, 10); console.log(result); // Outputs: 15This function addNumbers takes two parameters a and b, adds them together, and returns the result. It showcases the fundamental structure of a function in Javascript.
Array in a Function Javascript
Arrays in Javascript are powerful data structures, and when handled within functions, they unlock a myriad of possibilities. Let’s explore how to manipulate arrays using functions.
One common use of functions with arrays is to iterate through each element of the array, applying a specific operation, such as transformation or evaluation.
function sumArrayElements(arr) { var sum = 0; for (var i = 0; i < arr.length; i++) { sum += arr[i]; } return sum; } var array = [1, 2, 3, 4, 5]; var total = sumArrayElements(array); console.log(total); // Outputs: 15This example demonstrates a function that takes an array as an argument, iterates over each element, and calculates the sum of the elements.
Javascript Function Application in Real World Projects
Javascript functions find their application in numerous real-world scenarios, from web development to server-side programming. Their versatility allows developers to create interactive websites, manage server requests, and much more. Here, we'll delve into how these functions can be incorporated into larger projects to solve complex problems.
In web development, for instance, functions are often used in event handling. When a user interacts with a webpage (clicking a button, submitting a form), functions can respond to these actions, providing an interactive experience for the user.
window.onload = function() { document.getElementById('button').addEventListener('click', function() { alert('Button was clicked!'); }); };This example ties a click event listener to a button on a webpage. When the button is clicked, a function is executed, displaying an alert message. It demonstrates how functions enable interactivity within web applications.
Beyond client-side applications, Javascript functions are also pivotal in server-side development using environments like Node.js. Here, they manage everything from handling HTTP requests to connecting with databases. In such contexts, functions not only contribute to the business logic but also to the application's scalability and maintenance.Consider a Node.js application where functions handle API requests, parsing input data, and interacting with the database to retrieve or store information. This modular approach, facilitated by functions, simplifies the development process and enhances the application's ability to manage complex operations efficiently.
Advanced Javascript Function Techniques
Advancing your understanding of Javascript functions opens up a world of possibilities. It's not just about defining and calling functions; it's about leveraging sophisticated concepts like callback functions, closures, and high-order functions to write cleaner, more efficient code. These techniques are fundamental in navigating the intricacies of asynchronous operations, encapsulating functionality, and abstracting code logic.
Callback Function Javascript: Enhancing Asynchronicity
Callback functions play a pivotal role in Javascript, particularly in handling asynchronous operations such as events, server requests, and timeouts. By understanding how to effectively use callback functions, you can ensure that certain parts of your code do not execute until others have completed, thus enhancing the functional asynchronicity of your application.
A callback function is a function passed into another function as an argument and is intended to be called or executed inside the outer function.
function greeting(name) { alert('Hello, ' + name); } function processUserInput(callback) { var name = prompt('Please enter your name.'); callback(name); } processUserInput(greeting);This example demonstrates how a callback function (greeting) is passed as an argument to another function (processUserInput) and executed within it.
Callback functions are the backbone of Javascript's asynchronous nature, allowing for operations such as API calls to fetch data without blocking the main thread.
Implementing Closure in Javascript Functions
Closures in Javascript are a fundamental concept that allows a function to access variables from an enclosing scope, even after the outer function has completed execution. This feature is particularly useful for data encapsulation and creating factory and module patterns.
A closure gives you access to an outer function's scope from an inner function.
function makeAdder(x) { return function(y) { return x + y; }; } var addFive = makeAdder(5); console.log(addFive(2)); // Outputs: 7This example showcases a closure where the inner function retains access to the x variable of the outer function, even after the outer function has finished executing.
The power of closures lies in their ability to maintain state between function invocations, allowing for sophisticated data structuring and manipulation.
Utilising High-Order Functions for Cleaner Code.
High-order functions are functions that can take other functions as arguments or return them as results. They're a cornerstone of functional programming in Javascript and offer a more expressive, concise way to handle lists, arrays, and more without writing boilerplate code.
A high-order function is a function that takes one or more functions as inputs and/or returns a function as its output.
const numbers = [1, 2, 3, 4, 5]; const doubled = numbers.map(number => number * 2); console.log(doubled); // [2, 4, 6, 8, 10]This example utilises the .map() method, a high-order function, to double the values of an array of numbers.
The utilisation of high-order functions goes beyond mere array manipulation. These functions facilitate the creation of highly reusable, more abstract components that can significantly enhance code readability and reduce redundancy. For instance, in the context of web development, high-order functions can elegantly handle event listeners, time-outs, and API calls by abstracting repetitive patterns into reusable function calls. This not only makes the codebase cleaner but also more maintainable and easier to understand for other developers.
Javascript Function - Key takeaways
- Javascript function definition: A block of code designed to perform a particular task, acting as a 'subprogram' that can be reused throughout the code.
- Array in a function javascript: Functions can take arrays as arguments to perform operations like iteration and summation over array elements.
- Javascript function example: Demonstrates function declaration, parameters, and how to call a function, such as the
addNumbers
function which adds two numbers and returns the result. - Javascript function application: Functions are key in event handling within web development, enabling interactive user experiences, and are also critical in server-side environments like Node.js.
- Types of Javascript Function: Functions are treated as first-class objects and include varieties such as named, anonymous, arrow functions, and Immediately Invoked Function Expressions (IIFE), each with distinct characteristics and uses.
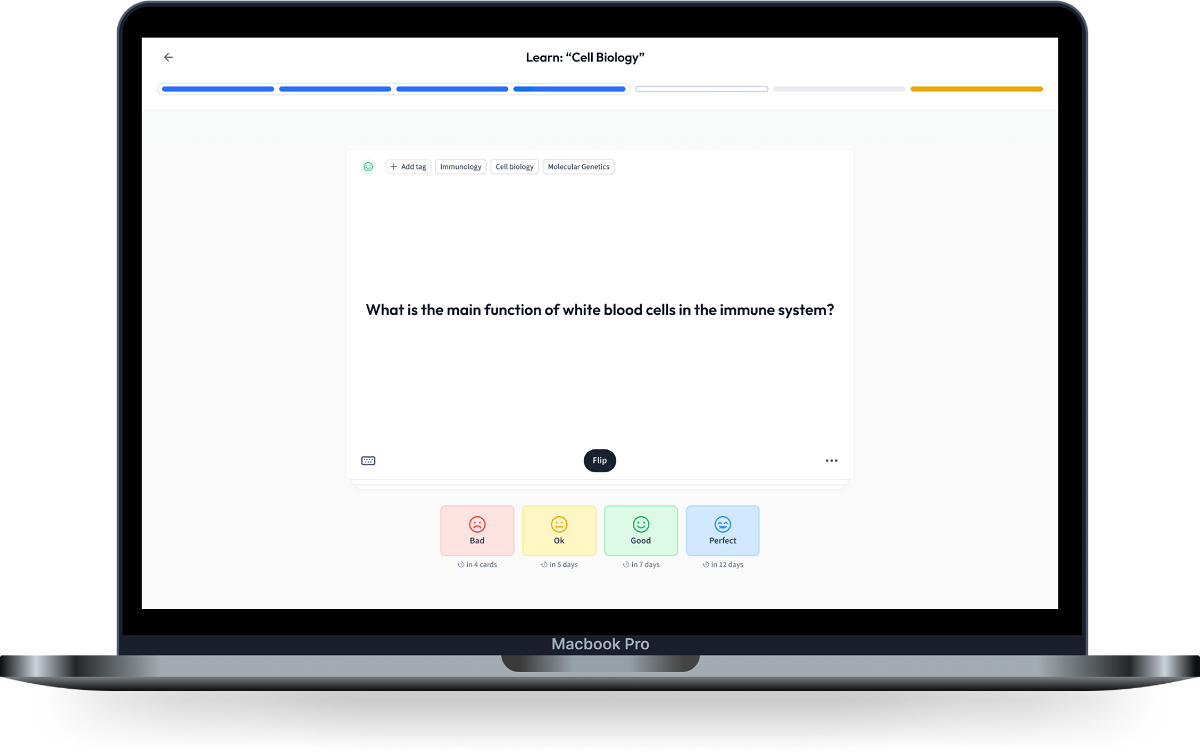
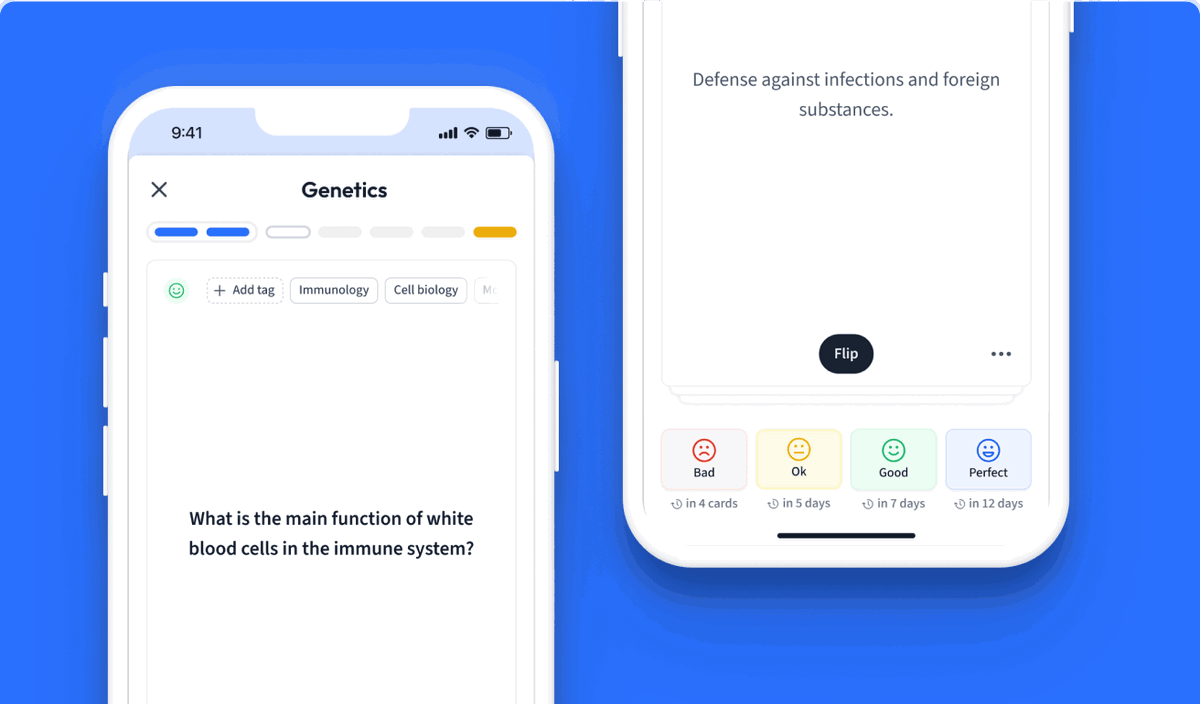
Learn with 92 Javascript Function flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Javascript Function
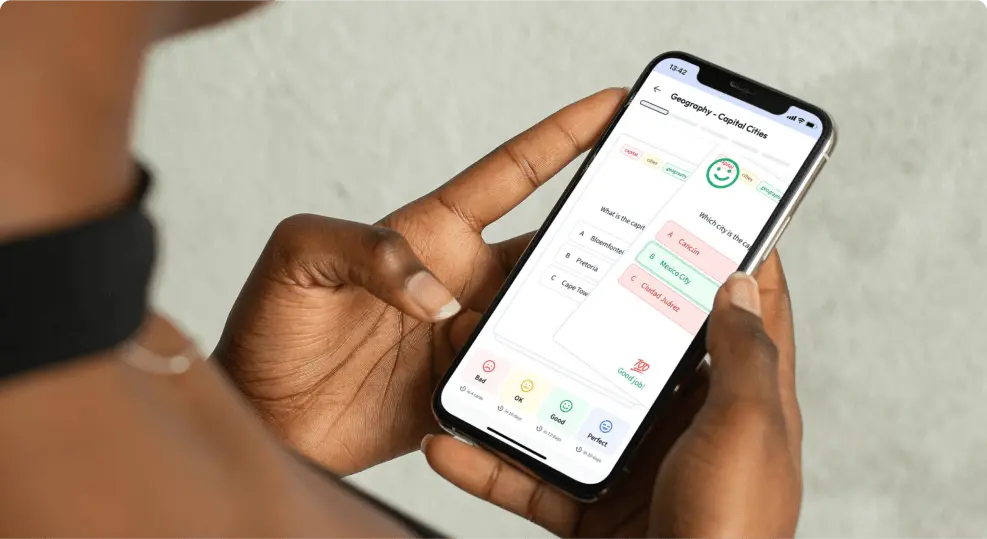
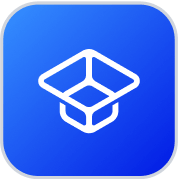
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more