Understanding the Java List Interface
The Java List Interface is an integral part of the Java programming language. It's an ordered collection of elements in Java which provides extensive methods to insert, delete, and access elements within the list.
Definition of Java list interface
Java List Interface is an interface in Java's Collection Framework, located in Java.util package. It extends the Collection interface, inheriting all the methods from its parent interface.
The Java List Interface allows you to perform the following operations:
- Insert elements
- Remove elements
- Access elements
- Iterate a list
- Sort a list, and more
Here's an example of how to utilize this interface:
List list=new ArrayList();
list.add("Example");
list.remove("Example");
The difference between Java List Interface and other interfaces
Different interfaces like Set or Queue interface also exist in Java's Collection Framework. These interfaces share common methods with List Interface but they are different based on the behavior they show.
List Interface | Set Interface | Queue Interface |
Allows duplicate elements | Does not allow duplicate elements | Allows duplicate elements |
Preserves insertion order | Does not preserve insertion order | May or may not preserve insertion order |
Allows null elements (can have multiple null values) | Allows null elements (but only one) | Allows null but depends on the implementing class |
Though List, Set, and Queue interfaces share common methods, their behaviour, application, and the set rules make them suited for various specific tasks. Understanding the differences and unique properties of these interfaces can assist you in enhancing your efficiency in Java programming.
Navigating through Java List Interface Methods
The Java List Interface comprises numerous methods that enable a multitude of operations, ranging from basic insertions and deletions to specialised functions like bulk operations. Understanding these methods and knowing how to utilise them is crucial for any Java programmer.
Key Java list interface methods you need to know
The Java List Interface includes a variety of important methods. Let's delve into some of the key ones:
.add(element): This method inserts the specified element into the list.
List list=new ArrayList();
list.add("Java");
.remove(element): This method removes the first occurrence of the specified element from the list.
list.remove("Java");
.get(index): This method retrieves the element from the list at the specified position.
String element = list.get(0);
.size(): This method provides the count of elements in the list.
int size = list.size();
.contains(element): This method checks if the list contains the specified element.
boolean exists = list.contains("HTML");
Special cases in using Java list interface methods
While the Java List Interface methods allow a great level of functionality, there are some unique cases that need special attention.
Note: All indexes in the List Interface are zero-based. This means the first element is at position 0, the second at 1, and so on.
String firstElement = list.get(0); // gets the first element
Caution: Attempting to access an element with an index that exceeds the size of the list will throw an IndexOutOfBoundsException.
String nonExistentElement = list.get(list.size()); // throws IndexOutOfBoundsException
Lastly, it's important to note that while the .add() method appends the element at the end of the list, there is also an overload of this method that accepts an index and an element. This method adds the element at the specified position, moving any subsequent elements, if any, towards the right.
list.add(0, "Python"); // adds "Python" at the start of the list
Classes Implementing List Interface in Java
In Java, several classes implement the List Interface, providing diverse options to developers for various application needs. These classes offer their own unique features and specialties catered to specific requirements while adhering to the core functionalities laid out by the List Interface.
Popular classes implementing list interface in Java
Following are the popular classes in Java that implement the List Interface:
- ArrayList
- LinkedList
- Vector
- Stack
ArrayList is a resizable array that grows automatically when new items are added and shrinks when items are removed. It provides fast access to elements using indices but may be slower in operations, such as insertion or deletion, which require shifting elements.
List arrayList = new ArrayList<>();
LinkedList is implemented as a double-linked list where each node holds the data and connections to the next and previous nodes. It offers efficient insertions or deletions but slower access to elements since it has to traverse the list.
List linkedList = new LinkedList<>();
Vector is similar to ArrayList but is thread-safe. It provides synchronised methods to ensure only one thread can access the vector at a time.
List vector = new Vector<>();
Stack is a class that implements a last-in-first-out (LIFO) data structure. It extends Vector to provide push and pop operations.
List stack = new Stack<>();
Benefits of using classes implementing list interface in Java
Understanding and employing the correct classes that implement the List Interface can greatly enhance your Java programming proficiency. Here's why:
- Flexibility: With different classes, you have many options to choose from depending on your application's requirements. Do you need quick access to elements? Try ArrayList. If you're going to make frequent insertions and deletions, LinkedList might be better.
- Standardisation: Since these classes implement the List Interface, they uphold the contract specified by the interface. This means that you can switch from one list implementation to another without radically altering your code.
- Thread Safety: Some classes like Vector and Stack come with built-in thread safety. If you're dealing with multi-threaded programs, this can be particularly advantageous.
- Advanced Features: List classes offer more than just adding or removing elements. You also get features like sorting, shuffling, copying, and more, thanks to the extensive JAVA Collections framework.
Therefore, having a comprehensive understanding of classes implementing the List Interface in Java can significantly improve your skills and efficiency as a Java developer.
Practical Approach to Java List Interface Implementation
Transitioning from theory to practice, let's take a look at how you can correctly implement the Java List Interface. It's a powerful tool in your Java arsenal with broad application.
How to correctly implement the Java list interface
The Java List Interface is part of Java's Collection Framework, meaning it adheres to the specifications set forth by the Collection Interface. By understanding not only the List Interface but also its parent interface, you're better equipped to properly implement it and leverage its full potential.
Remember, Java is an object-oriented language. The use of interfaces encourages encapsulation and abstraction, fundamental principles of object-oriented programming. Adhering to these principles will ensure you use the List interface correctly.
Here's a step-by-step guide on how to correctly implement the Java List Interface:
Step 1: First, identify whether your application requires the List Interface. Does your application need to maintain an ordered collection of objects with potential duplicates? If yes, the List Interface is suitable. Step 2: Choose a concrete implementation based on your specific needs. If random access performance is a high priority, choose ArrayList. If frequent insertions and deletions dominate your operations, consider using LinkedList. Step 3: Import the necessary classes and interfaces. Typically, you'll need to import java.util.List and java.util.ArrayList or java.util.LinkedList among others. Step 4: Create a List object and specify the type of objects it will contain. Step 5: Utilize the methods provided by the List interface for your operations. Always check the JavaDocs to understand the exact functionality and any exceptions that might be thrown.Example of Java list interface implementation
Now that you're familiar with the steps, it's time to dive into a practical example. You'll be creating an ArrayList of Strings and performing some basic operations.
Here's the code:
import java.util.List;
import java.util.ArrayList;
public class Main {
public static void main(String[] args) {
List list = new ArrayList();
list.add("C++");
list.add("Python");
System.out.println("List: " + list);
list.remove("C++");
System.out.println("After removal: " + list);
System.out.println("Does the list contain 'Java'? " + list.contains("Java"));
}
}
First, you import the necessary List and ArrayList classes. You then create a List instance, specifying String as the objects it will hold. You then use .add(String) to add a String to the list. After printing the current state of the list, you remove "C++" from the list and print it out again. Finally, you check if "Java" exists in the list, which should return false in this case.
Understanding the real-world implementation of the Java List Interface is an important stepping stone in mastering Java's Collection Framework. Through this framework, Java provides a robust and versatile set of data structures for developers to use, making it easier to solve complex problems and build efficient applications.
Expanding on the Applications of Java List Interface
Crucial to the Java Collections framework, the Java List Interface constitutes an ordered collection which permits duplicates. It incorporates methods for manipulating elements based on their position in the list. This functionality can be put to good use in various computer programming applications.
Understanding Java list interface and its applications in computer programming
In the scope of computer programming, the Java list interface carries substantial significance. A foundation of many applications, it is implemented by various classes like ArrayList, LinkedList, Vector and Stack, enabling versatile, ordered collections which handle duplicates with ease.
By learning to manipulate these structured data sets, you will be able to build dynamic, efficient applications, whether you are storing data from a user, organising internal operations or manipulating large sets of data.
- Data Processing: Dealing with data sets is a common scenario in programming, from financial transactions to processing student results. Most frequently, this data isn't just static, but requires sophisticated manipulations – retrieving specific data based on conditions, sorting the data, or eliminating duplicates. The Java List Interface provides methods like .sort() and .stream() to facilitate these operations.
- User Interfaces: In GUI applications, dynamic lists play a considerable role. Components like drop-down menus, lists and combo boxes often rely on ordered collections behind the scenes. The List Interface helps power such components, storing the selectable items and maintaining the order.
- Databases: Java's List Interface often interfaces with databases too. When retrieving records from a database using Java's JDBC, you might store the resultant data set in a List for further operations such as filtering or sorting. Notably, frameworks like Hibernate return results of queries as collections – typically, a List.
No matter the size of your application, the importance of handling collections can't be overstated. The Java List Interface, with its assortment of flexible functionalities, can immensely simplify management of collections, easing storage, retrieval, and manipulation of data.
Enhancing your programming skills with Java list interface applications
When looking to boost your programming skills, mastering the applications of the Java List Interface can prove instrumental. By effectively employing the List Interface, you can develop cleaner, more efficient code, while also saving substantial time in coding.
Here are some significant skills to master:
Implementing Different List Types: With each class implementing the List Interface offering distinct advantages, understanding when to utilise which one can be pivotal. For instance, using an ArrayList optimally offers quick, random access to list elements, while LinkedList excels when the application involves frequent additions and deletions of items. Performance Enhancement: Time complexity becomes crucial when applications involve large data sets or when performance is a concern. Knowing which operations are costly on which List implementations can help you write more efficient code. For instance, inserting an element in the middle of an ArrayList has a time complexity of \(O(n)\), whereas the same operation on a LinkedList is \(O(1)\). Bulk Operations: The List Interface offers various methods such as .addAll(Collection), .removeAll(Collection) and .retainAll(Collection) which perform operations on an entire collection at once. Harnessing these methods results in leaner, more readable code. Iterating Over Lists: While using a traditional for-loop to iterate over list elements is perfectly viable, modern Java provides more efficient methods such as Iterator and ListIterator. Advancing further, you can leverage Java's functional programming capabilities using .forEach() and .stream().Here's how to iterate over a list using the .forEach() method and a lambda:
List list = new ArrayList<>();
list.add("Java");
list.add("Python");
list.forEach(s -> System.out.println(s));
In this example, for each string 's' contained in the list, the lambda function prints it out.
Through these in-depth explorations and examples, harnessing the Java List Interface will significantly bolster your programming proficiency, accounting for the bulk of operations in a typical application.
Java List Interface - Key takeaways
- Java's Collection Framework: Java's Collection Framework includes interfaces like List Interface, Set Interface, Queue Interface, each with different behaviour and applications.
- List Interface: The List Interface allows duplicate and null elements, preserving the insertion order. Some key methods include .add(element), .remove(element), .get(index), .size(), and .contains(element).
- Classes implementing list interface in java: Popular classes include ArrayList, LinkedList, Vector, and Stack. Each class has its own applications and strengths, such as ArrayList for quick access but slow insertions/deletions, LinkedList for efficient insertions/deletions but slow access, Vector being thread-safe, and Stack representing a last-in-first-out (LIFO) data structure.
- Java list interface implementation: The List Interface should be implemented by making a careful choice of concrete implementation (ArrayList, LinkedList, etc.) based on your specific needs, importing necessary classes, creating a List object, and utilizing methods from the List interface.
- Java list interface and its applications: The List Interface is used significantly in data processing, powering user interfaces in GUI applications, and interfacing with databases, aiding in storage, retrieval, and manipulation of data.
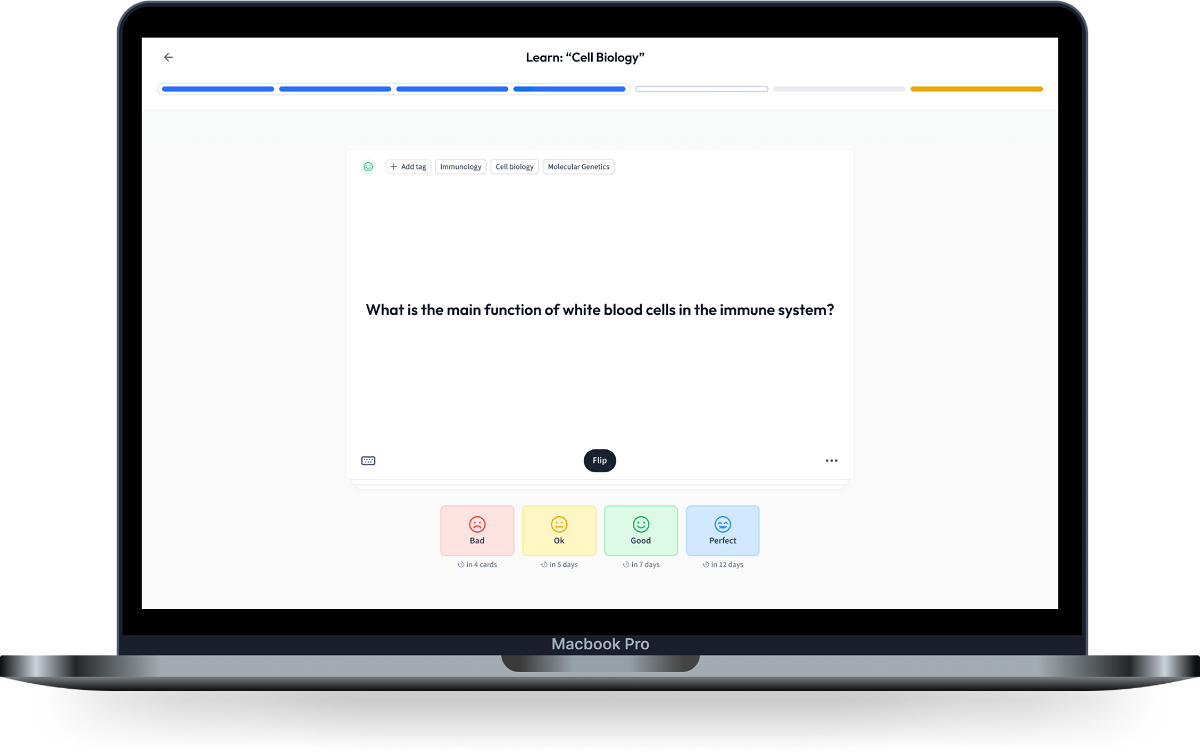
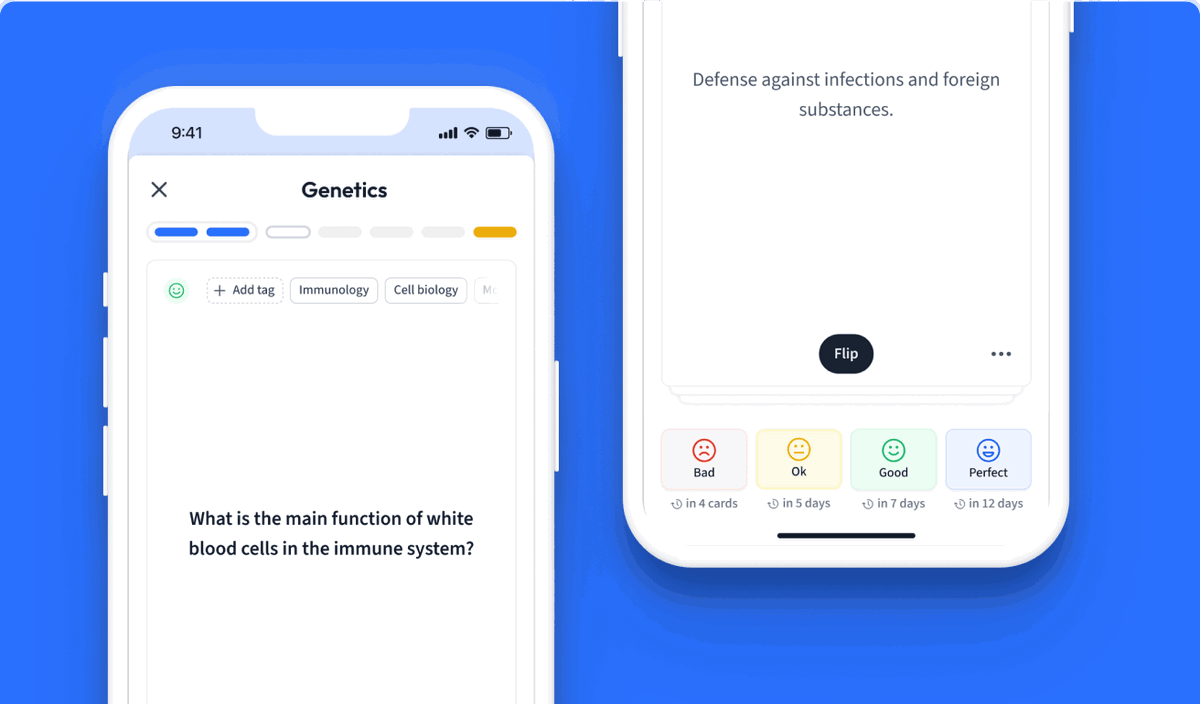
Learn with 15 Java List Interface flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Java List Interface
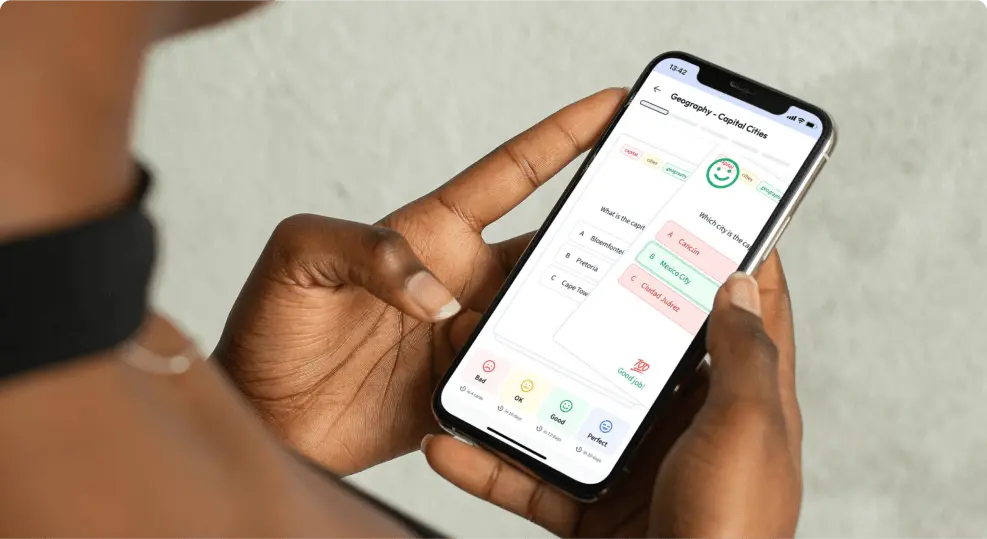
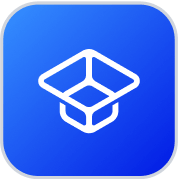
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more