Basics of Functions in Python
A function is a block of reusable code that performs a specific task in Python. Functions help break your code into modular and smaller parts, making it easier to understand and maintain. Functions also help reduce code repetition and enhance code reusability. In Python, there are two types of functions:
- Built-in functions
- User-defined functions
A built-in function is a pre-defined function provided by Python as part of its standard library. Some examples of built-in functions in Python are print(), len(), and type().
A user-defined function is a function that is created by the user to perform a specific task according to the need of the program.
Defining Functions in Python
In Python, you can create a user-defined function using the def
keyword followed by the function name and a pair of parentheses ()
that contains the function's arguments. Lastly, you should use a colon :
to indicate the start of a function block. Make sure to use proper indentation for the function body code. The syntax for defining a function in Python is as follows:
def function_name(arguments): # Function body code # ...
Here is an example of defining a simple function that calculates the square of a number:
def square(x): result = x * x return result
Calling Functions in Python
To call or invoke a function in Python, simply use the function name followed by a pair of parentheses ()
containing the required arguments. Here is the syntax for calling a function in Python:
function_name(arguments)
Here is an example of calling the square
function defined earlier:
number = 5 squared_number = square(number) print("The square of {} is {}.".format(number, squared_number))
This code will output:
The square of 5 is 25.
Types of Functions in Python
In Python, functions can be broadly classified into the following categories:
- Functions with no arguments and no return value
- Functions with arguments and no return value
- Functions with no arguments and a return value
- Functions with arguments and a return value
Type of Function | Function Definition | Function Call |
Functions with no arguments and no return value | def greeting(): print("Hello, World!") | greeting() |
Functions with arguments and no return value | def display_square(x): print("The square of {} is {}.".format(x, x * x)) | display_square(4) |
Functions with no arguments and a return value | def generate_number(): return 42 | magic_number = generate_number() print(magic_number) |
Functions with arguments and a return value | def add_numbers(a, b): return a + b | sum_value = add_numbers(10, 20) print(sum_value) |
Exploring Log Log Plots in Python
Log Log plots, also known as log-log or double logarithmic plots, are a powerful tool for visualising data with exponential relationships or power-law distributions. In these plots, both the x-axis and y-axis are transformed to logarithmic scales, which allow you to easily compare data across a wide range of values and observe trends that may not be apparent in linear plots. In Python, the Matplotlib library provides an efficient and flexible way to create and customise log-log plots.
Creating Log Log Plots with Python Matplotlib
Matplotlib is a versatile and widely used plotting library in Python that enables you to create high-quality graphs, charts, and figures. To create a log-log plot using Matplotlib, you'll first need to install the library by running the following command:
pip install matplotlib
Next, you can import the library and create a log-log plot using the plt.loglog()
function. The syntax for creating log-log plots using Matplotlib is as follows:
import matplotlib.pyplot as plt # Data for x and y axis x_data = [] y_data = [] # Creating the log-log plot plt.loglog(x_data, y_data) # Displaying the plot plt.show()
Here are some essential points to remember while creating log-log plots in Python with Matplotlib:
- Always import the
matplotlib.pyplot
module before creating the plot. - Unlike linear plots, you should use
plt.loglog()
function for creating log-log plots. - Insert the data for the x-axis and y-axis that you want to display on the log-log plot.
- Use the
plt.show()
function to display the generated log-log plot.
Log Log Plot Python Example
Here is an example of creating a log-log plot in Python using Matplotlib. This example demonstrates plotting a power-law function, \(y = 10 * x^2\), where x ranges from 0.1 to 100:
import matplotlib.pyplot as plt import numpy as np x_data = np.logspace(-1, 2, num=100) y_data = 10 * x_data**2 plt.loglog(x_data, y_data) plt.xlabel('X-axis') plt.ylabel('Y-axis') plt.title('Log-Log Plot of a Power-Law Function') plt.grid(True) plt.show()
Customising Log Log Plots using Matplotlib
Matplotlib allows you to customise several aspects of log-log plots, such as axis labels, plot titles, gridlines, and markers. Here are some customisation options you can apply to your log-log plots:
- Axis labels: Use the
plt.xlabel()
andplt.ylabel()
functions to set custom labels for the x-axis and y-axis, respectively. - Plot title: Add a custom title to the plot using the
plt.title()
function. - Gridlines: You can add gridlines to the plot by calling the
plt.grid()
function with theTrue
argument. - Markers: To change the marker style, you can pass the
marker
argument to theplt.loglog()
function. Common markers include'o'
(circle),'s'
(square), and'-'
(line). - Line style: Change the line style using the
linestyle
argument in theplt.loglog()
function. Popular line styles include':'
(dotted),'--'
(dashed), and'-.'
(dash-dot).
Here's an example of a customised log-log plot using the various options mentioned above:
import matplotlib.pyplot as plt import numpy as np x_data = np.logspace(-1, 2, num=100) y_data = 10 * x_data**2 plt.loglog(x_data, y_data, marker='o', linestyle=':', linewidth=1.5) plt.xlabel('X-axis') plt.ylabel('Y-axis') plt.title('Customised Log-Log Plot of a Power-Law Function') plt.grid(True) plt.show()
By understanding and utilising these customisation options, you can create more visually appealing and informative log-log plots for your data analysis and presentations.
Analysing Log Log Scatter Plot and Graphs in Python
Log Log Scatter plots are widely used in Python to visualise and analyse data that have underlying exponential or power-law relationships. Generating these plots allows for the identification of trends and patterns not easily observed in linear graphs. Python offers various libraries for creating and analysing Log Log Scatter Plots, such as Matplotlib and Seaborn.
To create a Log Log Scatter Plot using Matplotlib, start by installing and importing the library with the following command:
pip install matplotlib
Once the library is installed, use the plt.scatter()
function along with the plt.xscale()
and plt.yscale()
functions to create the Log Log Scatter Plot. Here are the essential steps for creating a Log Log Scatter Plot using Matplotlib:
- Import the Matplotlib.pyplot module.
- Set logarithmic scales for both x and y axes using
plt.xscale('log')
andplt.yscale('log')
functions. - Generate the scatter plot using the
plt.scatter()
function by providing the x-axis and y-axis data. - Customise the axes labels, plot titles, and other visual aspects as needed.
- Display the plot using the
plt.show()
function.
Log Log Scatter Plot Python Example
Here is an example of creating a Log Log Scatter Plot in Python using Matplotlib:
import matplotlib.pyplot as plt x_data = [1, 10, 100, 500, 1000, 5000, 10000] y_data = [0.1, 1, 10, 20, 40, 90, 180] plt.xscale('log') plt.yscale('log') plt.scatter(x_data, y_data, marker='o', color='b') plt.xlabel('X-axis') plt.ylabel('Y-axis') plt.title('Log Log Scatter Plot') plt.grid(True) plt.show()
Advantages of Using Log Log Scatter Plots
Log Log Scatter Plots offer numerous benefits when it comes to data analysis and presentation. Some of the main advantages of using Log Log Scatter Plots in Python are:
- Compress Wide Ranges: Log Log Scatter Plots can significantly compress a wide range of values on both axes, making it easier to visualise and analyse large data sets.
- Reveal Trends and Patterns: These plots are particularly useful for identifying trends and patterns in data that follow power-law distributions or exhibit exponential relationships.
- Linearise Exponential Relationships: Log Log Scatter Plots can convert exponential relationships into linear ones, simplifying the analysis and allowing for the use of linear regression and other linear techniques.
- Visual Appeal: They are visually appealing and can effectively communicate complex information, which is crucial in presentations and reports.
- Customisation: As with other Python plotting libraries, Log Log Scatter Plots can be easily customised in terms of markers, colours, and labels to suit the user's preferences.
- Adaptability: These plots are applicable to various fields, such as finance, physics, biology, and social sciences, where such non-linear relationships are common.
Understanding the advantages and use cases of Log Log Scatter Plots can help you effectively apply this powerful tool in your data analysis and visualisation tasks.
Enhancing Data Visualisation with Log Log Graphs in Python
Log Log Graphs are powerful tools for data visualisation that can help reveal hidden patterns, trends, and relationships in your data, especially when dealing with exponential or power-law functions. Python, with its extensive range of plotting libraries such as Matplotlib and Seaborn, offers an excellent platform for creating and analysing Log Log Graphs.
Log Log Graph Python for Better Data Analysis
Log Log Graphs, also known as log-log or double logarithmic plots, display data in a visually appealing way and help identify trends obscured in linear graphs. By transforming both the x and y axes to logarithmic scales, Log Log Graphs effectively communicate complex information about power-law distributions, exponential relationships, and non-linear phenomena. With powerful plotting libraries like Matplotlib and Seaborn in Python, it is possible to create and customise Log Log Graphs to suit your data analysis and presentation needs.
Analysing Trends and Patterns in Log Log Graphs
The analysis of trends and patterns in Log Log Graphs offers insight into the underlying behaviour of the data and the relationships between variables. Some steps to follow while analysing Log Log Graphs in Python are:
- Plot the data: Use a suitable Python library, like Matplotlib or Seaborn, to plot the data on a Log Log Graph. Ensure both axes are in logarithmic scales to reveal trends that might not be visible in linear graphs.
- Identify patterns: Look for trends, such as linear or curved patterns, in the Log Log Graph that might indicate power-law or exponential relationships. Use visual cues like markers and gridlines to help identify these patterns.
- Fit models: To better understand the data, fit appropriate models, such as power-law or exponential functions, to the data points in the Log Log Graph. Python libraries like NumPy and SciPy provide robust tools for fitting such models to your data.
- Evaluate the goodness of fit: Evaluate the goodness of fit for the chosen models using relevant statistical measures like R-squared, Mean Squared Error (MSE), or the Akaike Information Criterion (AIC). The better the fit, the more accurately the model represents the data.
- Interpret results: Based on the model fits and patterns identified, draw conclusions about the relationships between variables and the underlying behaviour of the data.
Following these steps will allow you to cover a broad range of analyses, from identifying trends to fitting and evaluating models, enabling deep insights into your data's behaviour.
Applications of Log Log Graphs in Computer Science
Log Log Graphs find applications in various fields of computer science, including performance analysis, parallel computing, and network analysis. Some notable applications are:
- Performance Analysis: Log Log Graphs can visualise and analyse large-scale systems' execution times, memory usage, and power consumption, where the performance metrics often follow power-law distributions or exponential functions.
- Parallel Computing: In parallel computing, Log Log Graphs can help evaluate computational scaling, communication overheads, and load balancing across multiple processors, which usually follow non-linear patterns.
- Network Analysis: In network analysis, Log Log Graphs can reveal critical insights into the complex and non-linear relationships between network elements like nodes, edges, and clustering coefficients, as well as the impact of network size on these relationships.
- Algorithm Analysis: Log Log Graphs can help analyse the time complexity and space efficiency of algorithms, uncovering non-linear relationships between input size and computational resources such as CPU time and memory usage.
- Data Mining and Machine Learning: In data mining and machine learning, Log Log Graphs can aid in visualising and analysing large-scale, high-dimensional data sets and model performance, where non-linear patterns are common.
Embracing Log Log Graphs, coupled with the powerful data analysis tools available in Python, can lead to better comprehension and improved decision-making in various computer science domains.
Functions in Python - Key takeaways
Functions in Python: Reusable code blocks for specific tasks, divided into built-in and user-defined functions.
Defining functions: Use the
def
keyword, function name, arguments, and a colon to indicate the start of the function block.Log Log Plots: Powerful data visualisation tool for exponential relationships or power-law distributions, created using Python's Matplotlib library.
Log Log Scatter Plots: Reveal hidden patterns, trends, and relationships in data that exhibit exponential characteristics, easily created in Python with Matplotlib and Seaborn.
Log Log Graphs: Enhanced data visualisation tool in Python, with applications in computer science fields like performance analysis, parallel computing, and network analysis.
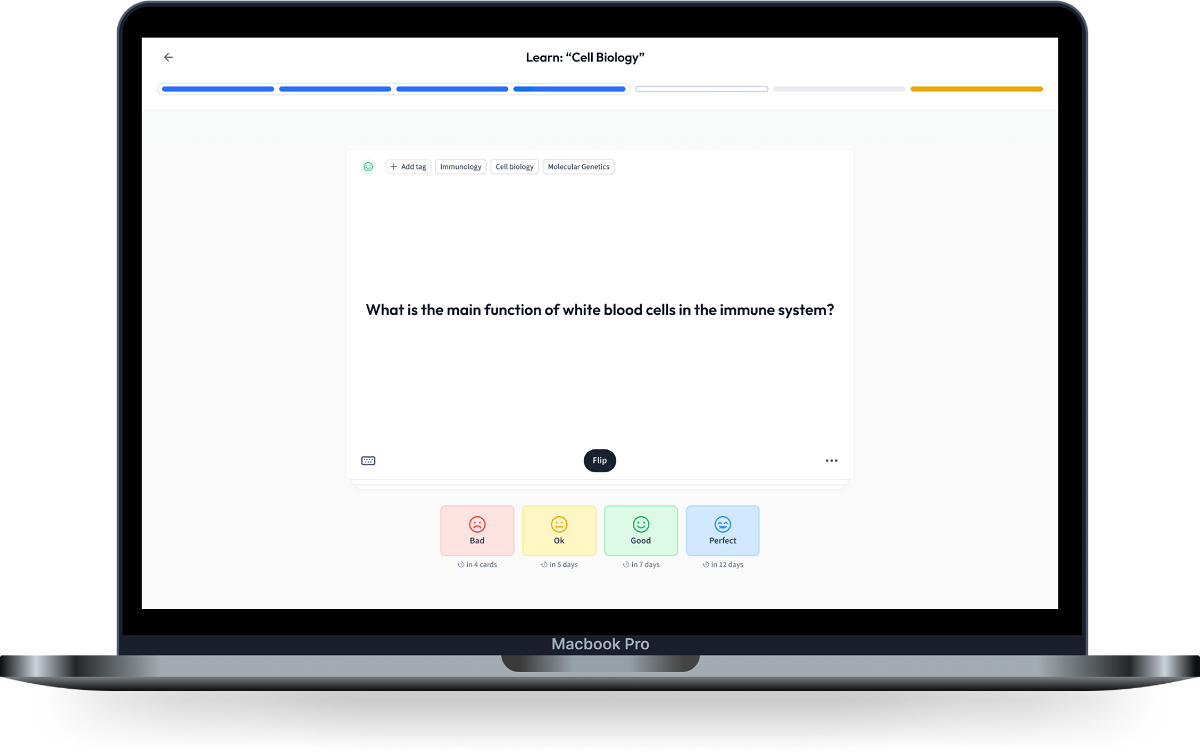
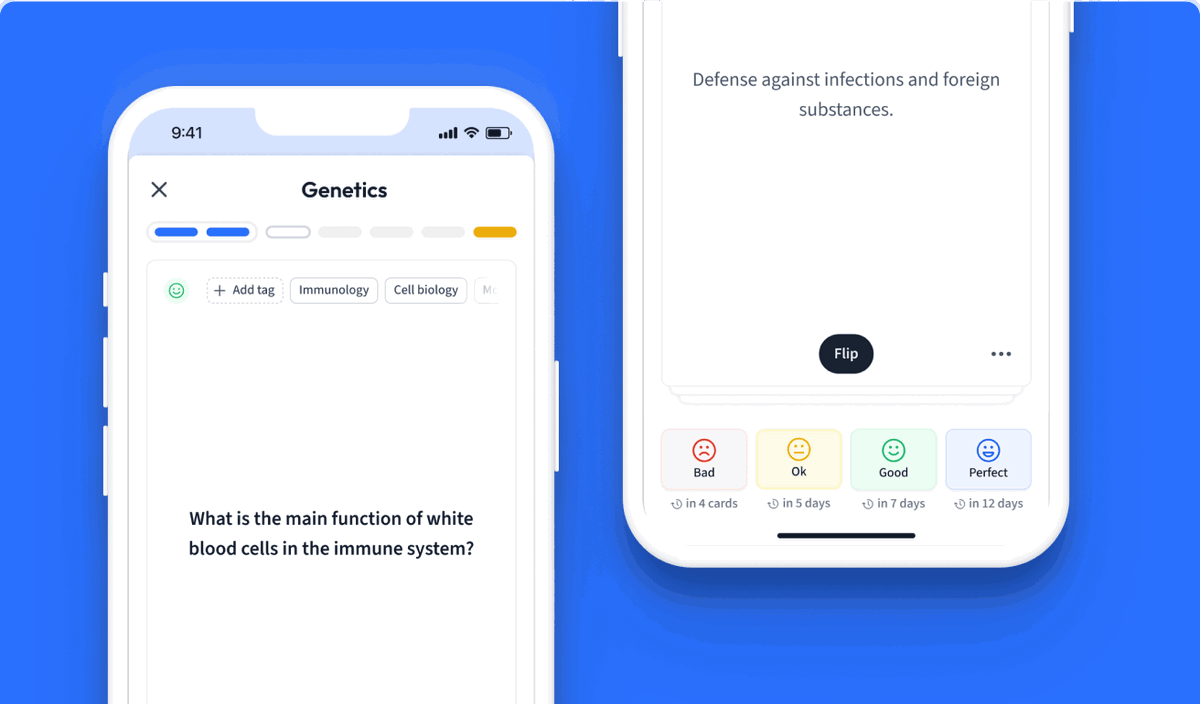
Learn with 99 Functions in Python flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Functions in Python
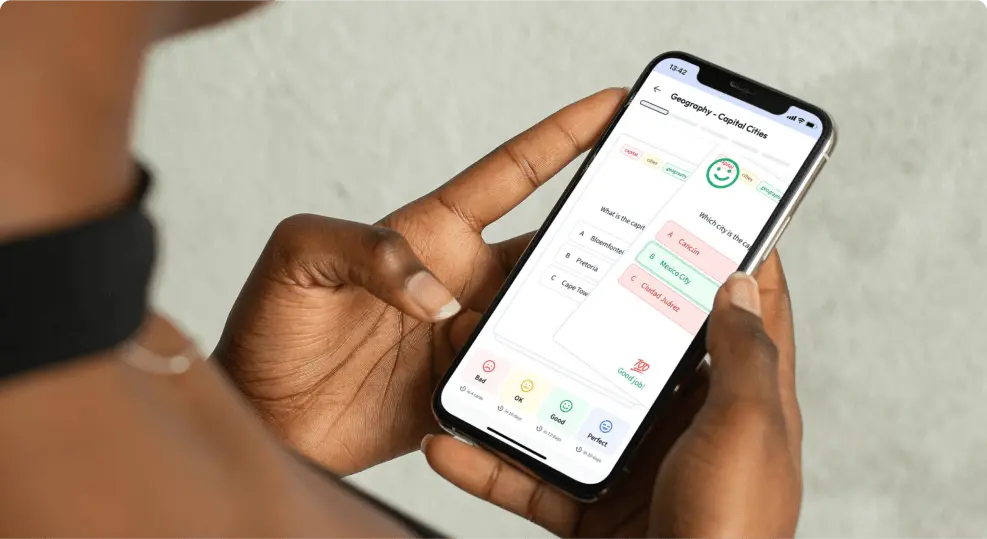
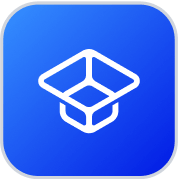
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more