Understanding Javascript Spread and Rest Syntax
Javascript features certain operators that greatly simplify array and object manipulations. Among these are the Spread and Rest operators, which, despite using the same syntax (...), serve different purposes. They make code more concise and readable, thus improving the efficiency of development.
What is Rest and Spread in Javascript?
Spread Syntax (...) allows an iterable such as an array or string to be expanded in places where zero or more arguments (for function calls) or elements (for array literals) are expected. It can also be used to spread object properties in object literals.
Rest Parameter (...) provides an improved way to handle function parameter lists. In functions, the rest syntax appears in the function definition to Readily consolidate a variable number of arguments into an array.
Despite sharing the same three-dot syntax, the context in which they are used determines whether they're applying the spread or rest functionality.
Javascript Spread and Rest Operator Examples
// Spread operator in arrays const fruits = ['apples', 'bananas']; const moreFruits = [...fruits, 'oranges', 'grapes']; console.log(moreFruits); // Output: ['apples', 'bananas', 'oranges', 'grapes']
// Spread operator with objects const person = { name: 'John', age: 30 }; const updatedPerson = { ...person, occupation: 'Engineer' }; console.log(updatedPerson); // Output: { name: 'John', age: 30, occupation: 'Engineer' }
// Rest parameters in function function sum(...numbers) { return numbers.reduce((accumulator, currentValue) => accumulator + currentValue); } console.log(sum(1, 2, 3, 4)); // Output: 10
The Spread operator efficiently combines arrays, adds items to arrays, and spreads elements out into function arguments. On the other hand, the Rest operator is adept at gathering a list of a function's arguments into an array, providing a flexible way to handle various inputs.
The introduction of Spread and Rest operators in ES6 marked a significant improvement in making JavaScript more readable and expressive. For instance, copying arrays or merging multiple objects can now be achieved with a simple syntax instead of relying on functions like concat()
or Object.assign()
. These operators reflect JavaScript's evolving nature, aimed at making the language more powerful and user-friendly for developers.
Difference Between Rest and Spread Operator in Javascript
In the dynamic world of JavaScript, understanding the distinction between the Rest and Spread operators is crucial for writing clean and efficient code. Both operators use the same syntax (...), but their use cases and functionality differ significantly.Exploring these differences not only amplifies the ability to manipulate arrays and objects but also enhances the readability and maintainability of the code.
Syntax and Use Cases
The syntax for both Spread and Rest operators in JavaScript is elegantly simple, utilising three dots (...). However, the context in which they are used is what sets them apart.A Spread operator is used to expand or spread iterable elements such as arrays, objects, and strings into something else. It could be another array, function arguments, or object properties. Conversely, the Rest operator is utilised to condense multiple elements into a single array element.
// Using Spread to combine arrays const firstArray = [1, 2, 3]; const secondArray = [4, 5, 6]; const combinedArray = [...firstArray, ...secondArray]; console.log(combinedArray); // Output: [1, 2, 3, 4, 5, 6]
// Using Rest to gather function arguments into an array function sum(...args) { return args.reduce((prev, curr) => prev + curr, 0); } console.log(sum(1, 2, 3, 4)); // Output: 10
The contextual deployment of the three-dot syntax determines its role as either a Spread or Rest operator.
Functional Differences in Detail
Although they share the same symbolic representation, the functional disparities between the Spread and Rest operators are substantial and worth noting. To begin with, the Spread operator is essentially about 'expanding' or distributing elements, making it ideal for copying arrays, merging arrays or objects, and applying arguments to functions. The strength of the Spread operator lies in its ability to decompose an entity into individual components, enhancing flexibility in data manipulation.In contrast, the Rest operator 'condenses' or aggregates multiple arguments passed to a function into a single array. Its primary purpose is to capture an indefinite number of arguments, allowing functions to accept varied amounts of data seamlessly.
The applications of Spread and Rest operators go beyond the obvious, intersecting with advanced JavaScript concepts like destructuring. For example, Spread can be used in destructuring to 'pick' what properties to take from an object, while the Rest operator can gather the remaining properties. This synergy between operations crafts a robust toolkit for developers, streamlining complex operations into more manageable, readable, and concise code.
// Spread in object destructuring const person = { name: 'Jane', age: 32, job: 'Designer' }; const { name, ...otherProps } = person; console.log(name); // Output: 'Jane' console.log(otherProps); // Output: { age: 32, job: 'Designer' }
// Rest in function arguments function logNames(...names) { names.forEach(name => console.log(name)); } logNames('John', 'Jane', 'Mary'); // Outputs: John\nJane\nMary
Practical Applications of Javascript Spread and Rest
Javascript's Spread and Rest operators provide powerful yet subtlety different ways to work with arrays, objects, and functions. By mastering these operators, you can simplify complex operations, making your code more readable and maintainable.
Simplifying Array and Object Operations
The Spread operator (...) shines in operations involving arrays and objects. Whether you're merging arrays, combining objects, or needing elements of an array as arguments to a function, the Spread syntax offers a neat solution.On the flip side, when encountering objects with many properties, or when you need to clone an object to avoid mutations, Spread becomes indispensable.
// Cloning an array const original = [1, 2, 3]; const cloned = [...original]; console.log(cloned); // Output: [1, 2, 3] // Merging objects const defaultSettings = { sound: 'off', brightness: 'low' }; const userSettings = { sound: 'on' }; const settings = { ...defaultSettings, ...userSettings }; console.log(settings); // Output: { sound: 'on', brightness: 'low' }
Rest operators, although seemingly similar because they use the same syntax, play a different role. They're most useful in functions when you don't know how many arguments will be passed. This becomes exceptionally handy in mathematical operations or when handling varying input lengths.
Enhanced Function Parameter Handling
In functions, the versatility of the Rest operator comes to light. It helps in neatly collecting arguments into an array, making variable arguments processing straightforward.From simplifying the handling of multiple parameters to supporting the creation of variadic functions (functions that accept any number of arguments), Rest significantly bolsters function parameter management.
// A function using Rest to collect parameters function concatenateStrings(...strings) { return strings.join(''); } console.log(concatenateStrings('JavaScript ', 'Spread ', 'and ', 'Rest')); // Output: 'JavaScript Spread and Rest'
These operators facilitate dealing with sequences of elements in a more expressive way, thus broadening the horizon for arrays and function parameters handling.
Using Spread for function arguments takes the guesswork out of applying an array of arguments to a function, especially when the function doesn't inherently support an array as input. Here is where Spread truly excels, gracefully translating an array into individual arguments.
// Using Spread as function arguments function sum(x, y, z) { return x + y + z; } const numbers = [1, 2, 3]; console.log(sum(...numbers)); // Output: 6The elegance of Spread in distributing array items as function arguments contrasts with the traditional approach of manually indexing the array, showcasing its prowess in both simplicity and maintainability.
Coding with Javascript Spread and Rest Operators
Utilising the Javascript Spread and Rest operators can significantly enhance coding practices by simplifying array and object manipulations. These operators, though sharing the same syntax, serve distinct purposes which, when properly leveraged, can make code not only more readable and shorter but also more flexible.
Tips for Efficient Coding
Adopting certain strategies and best practices when using the Javascript Spread and Rest operators can drive the efficiency of your code. Here are some tips for capitalising on these powerful features:
- Use the Spread operator to combine arrays or objects without mutating the original structures.
- Leverage the Rest parameter for functions that take an indefinite number of arguments, thereby making your function signature more descriptive.
- Consider employing the Spread operator for cloning arrays and objects to avoid unintended side-effects from mutability.
- Utilise Rest parameters to destructure arrays and objects neatly, enhancing the readability of your code.
Remember, usage of Spread for function calls and array construction tends to improve code readability and reduces the likelihood of errors.
Moreover, an understanding of when not to use these operators is just as important as knowing how to use them. Applying them indiscriminately can lead to decreased performance, especially in scenarios involving large datasets or deep copying of complex objects.Always measure the performance implications if you're applying Spread or Rest in critical paths of your application.
Common Pitfalls and How to Avoid Them
While the Spread and Rest operators are invaluable tools within Javascript, there are common pitfalls to be wary of:
- Overusing Spread for deep cloning: Spread provides shallow copying, which can lead to bugs when working with nested objects. Utilise libraries or write functions for deep cloning when necessary.
- Ignoring performance impact: Especially in case of the Spread operator, spreading large arrays or objects can impact performance. Be mindful of the datasets you're working with.
- Misusing Rest parameters: The Rest parameter synthesises arguments into an array. Ensure that functions leveraging Rest are designed to handle array inputs effectively.
- Forgetting Spread in function calls: It's easy to forget that Spread can be used to unpack an array into individual arguments for a function call, leading to verbose and unnecessary code.
// Incorrect use of Spread for deep cloning const original = { a: { b: 2 } }; const clone = { ...original }; clone.a.b = 3; console.log(original.a.b); // Incorrectly outputs 3, not 2 // Correct approach using a deep clone function function deepClone(obj) { return JSON.parse(JSON.stringify(obj)); } const deepCloned = deepClone(original); deepCloned.a.b = 4; console.log(original.a.b); // Correctly outputs 2
Incorporating Javascript Spread and Rest operators into your coding practice offers a plethora of benefits but demands a clear understanding of their limitations to avoid pitfalls. When used judiciously, these operators can not only drive code efficiency but can also influence the structural and logical clarity of your programming efforts.As you become more proficient with these tools, you'll find a balance between their powerful capabilities and the pragmatic aspects of application performance and maintainability. Encountering and overcoming these challenges is part of the journey towards becoming a more effective Javascript developer.
Javascript Spread And Rest - Key takeaways
- Spread Syntax: The spread operator (
...
) expands an iterable such as an array, or string in situations where arguments or elements are expected. - Rest Parameter: The rest operator (
...
) consolidates a variable number of arguments into an array in the context of function parameters. - Difference between Rest and Spread: The spread operator is used to expand or spread elements, while the rest operator is used to condense or gather elements into an array.
- Javascript Spread and Rest applications: Spread is commonly used for combining arrays or objects, while Rest is beneficial for handling an indefinite number of function arguments.
- Functional Utility: Spread allows for easier copying and merging of arrays or applying arguments to functions, and Rest helps in collecting arguments as an array, simplifying variadic function creation.
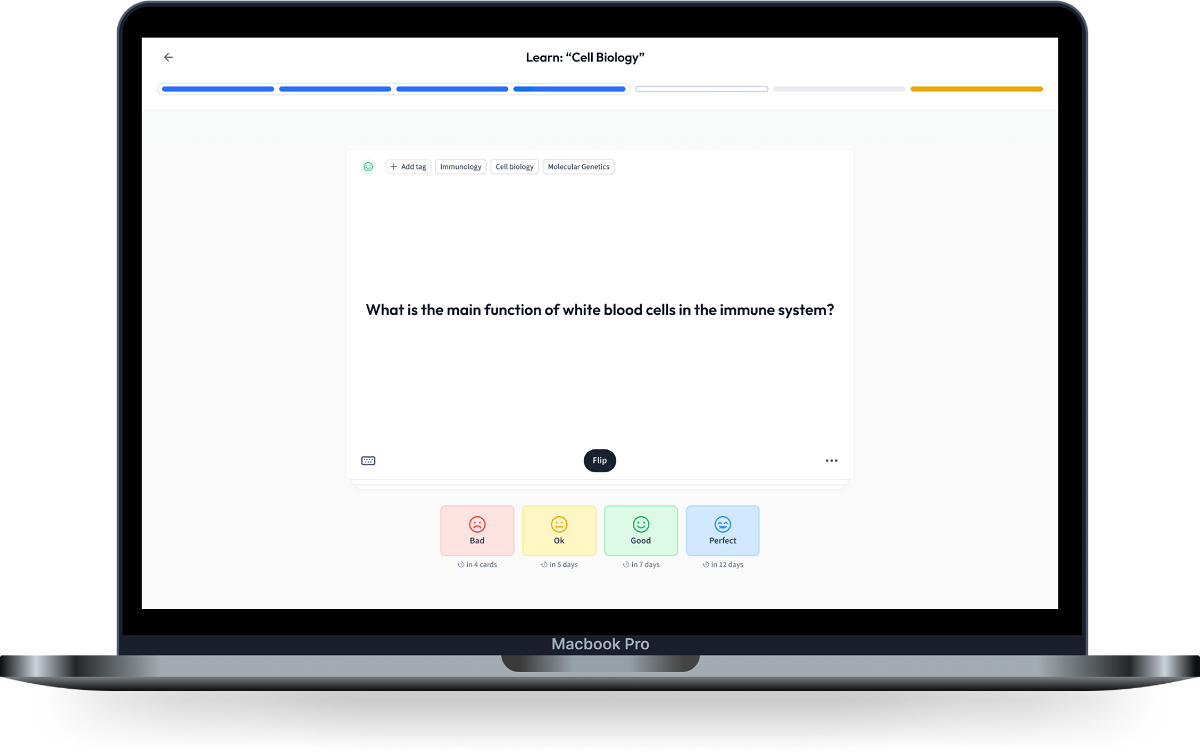
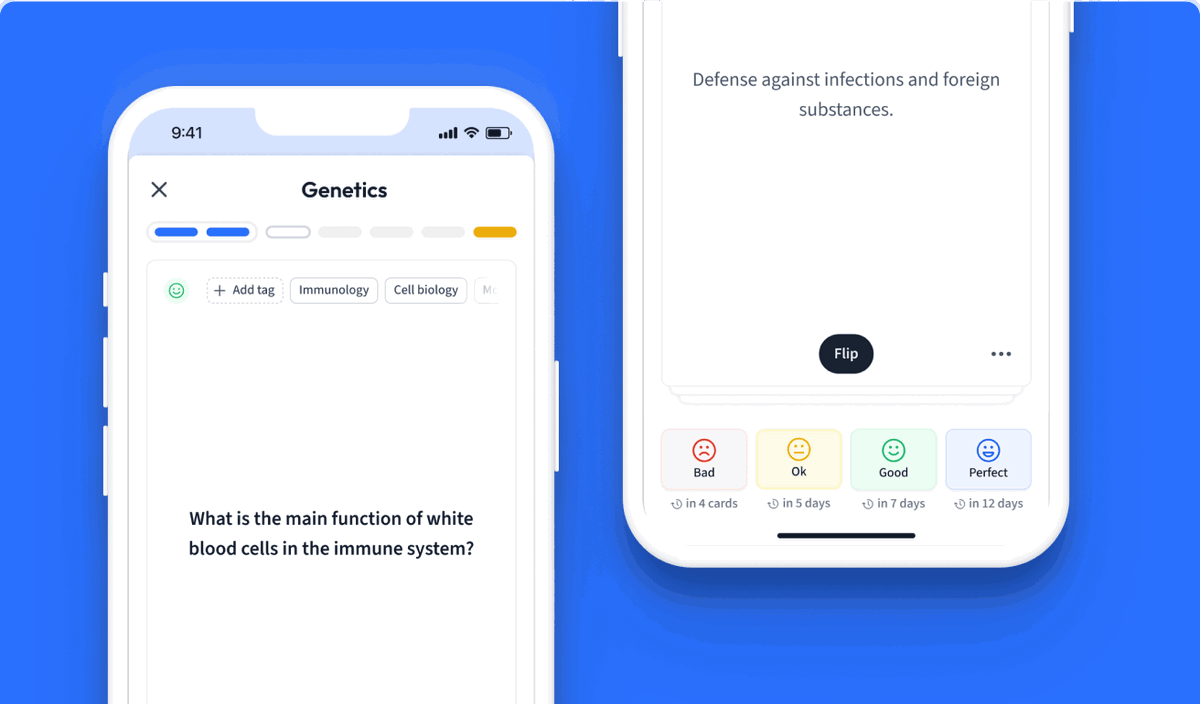
Learn with 24 Javascript Spread And Rest flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Javascript Spread And Rest
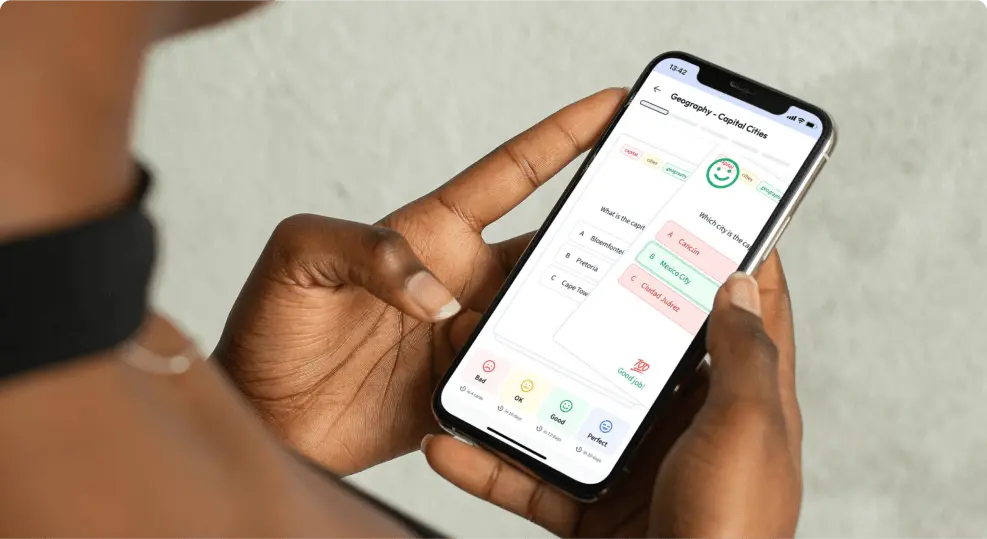
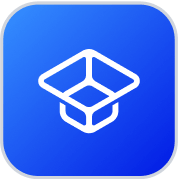
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more