Document Object in JavaScript: An Overview
In computer science, especially in the realm of web development, the significance of JavaScript cannot be under-emphasized. Particularly, the JavaScript Document Object is a vital part of this extensive language, making it quintessential for you to grasp its functionality. But what is this game-changer concept of JavaScript Document Object? How did it originate, and how has it developed over time? What are its basics? Dive into these exciting topics to expand your Computer Science knowledge.
Understanding What is Document Object in JavaScript
The Document Object Model (DOM) in JS is a programming interface for web documents. In essence, it represents the structure of the webpage, allowing scripts to change the document's structure, style, and content. Your web page becomes a document accessible in script.
When a web page is loaded, the browser creates the DOM of that page, which is essentially an object-oriented representation of the webpage. The HTML nodes are translated into the DOM and objects are created according to the Document Object Model. This translates to a more dynamic and interactive user experience since JavaScript can manipulate the Document Object to make realtime changes to the web page.
Here are some crucial aspects:
- Objects inherit methods and properties from
Object.prototype
, although they may be overridden. - Objects can be altered, extended, and pruned arbitrarily.
- Variables are not directly associated with objects; however, variables reference objects and are manipulated with JavaScript's command statements.
Origin and Development of JavaScript Document Object
The JavaScript Document Object Model was initially implemented as a way to deal with the Shortcomings of the static HTML and to enable a more dynamic and interactive experience for the user. Initially, each browser had its own proprietary model, but this led to compatibility issues. To resolve this, the World Wide Web Consortium (W3C) standardized the DOM.
Additional improvements and extensions to the Document Object Model have been implemented over the years. This has led to the introduction of methods and properties which allow for complex manipulation of HTML documents.
Defining the JavaScript Document Object: The Basics
On a fundamental level, the JavaScript Document Object Model defines the logical structure of documents and the way a document is accessed and manipulated.
To be more precise, the JS Document Object represents your webpage. If you want to access any element in an HTML document, you always start with accessing the Document Object.
Some basics include:
- Document Object is part of the hierarchy of objects in JavaScript, at the global level.
- It is used in the concept of DOM (Document Object Model).
- It provides numerous properties and methods that can be used to perform operations like change content, stylize, and more.
Understanding the Concept Explanation of JavaScript Document Object
Delving deeper into JavaScript, the JavaScript Document Object merits a detailed discussion. If you were to picture your webpage as a document, then the JavaScript Document Object is akin to the blueprint of that document, depicting a vivid structural representation.
The Structure of JavaScript Document Object
The structure of a JavaScript Document Object is a fascinating study. It's based on the Document Object Model (DOM), which presents a hierarchical tree-like structure. This 'DOM tree' consists of a multitude of nodes corresponding to HTML elements, wherein each node could be an HTML tag, attribute, or text.
Imagine you have an HTML document with the following structure:
My Title My Heading
Each of these HTML elements corresponds to a node in the DOM tree. The root of this tree is the Document Object. This model is perfect since it allows you to interact with and control the web document in a programmatic and structured manner.Let's illustrate this with a table:
Root Element | |
Child of | |
Child of and grandchild of | |
Child of |
Nodes and Elements in JavaScript Document Object
In the JavaScript Document Object landscape, the terms 'Node' and 'Element' are of utmost importance. Here, a Node represents any type of object in the DOM hierarchy, while an Element is a type of Node that corresponds specifically to an HTML element, which contains both a start and end tag.
For instance, an element node can represent the HTML element, while a text node would be the text content within this element. Notice that all nodes (including Element Nodes) are objects. Therefore, they have properties and methods. But Element Nodes have extra methods making it possible to manipulate the content.
Let's consider an example:
Hello, world!Here, "Hello, world!" is a text node, a child of the body element node.
Properties and Methods of JavaScript Document Object
Just like any other object in JavaScript, the Document Object comes with a set of properties and methods. These allow you to do useful things like alter the document structure, modify the document's content, or change the document's look and feel with CSS. Properties are values that you can get or set (like changing the content of an HTML element). Methods are actions you can do (like add or delete an HTML element).
Below are some examples of the properties:
document.URL
returns the URL of the documentdocument.body
returns the body elementdocument.forms
returns form elements
The Document Object methods include:
document.getElementById(id)
finds an element by element iddocument.getElementsByTagName(name)
finds elements by tag namedocument.createElement(name)
creates a new element
With a deeper understanding of JavaScript Document Object, you have access to all nodes on your webpage - no small feat! Remember, it's all about being able to access and manipulate these nodes to render a webpage that's interactive and dynamic.
Practical Approach: Examples of JavaScript Document Object
The key to mastering the JavaScript Document Object lies in practical application and active coding. Let's dive into some examples, helping you understand how to use the Document Object in JavaScript, interact with users, and manipulate webpage elements effectively.
Simple Examples of Using Document Object in JavaScript
Delving into examples is an excellent strategy to solidify your understanding of JavaScript Document Object. From accessing to manipulating webpage elements, these examples encompass a range of operations you can perform using the Document Object.
Accessing Elements with JavaScript Document Object
One of the most frequent operations in JavaScript involves accessing various elements. For instance, let's say you want to access a specific element using its ID.
Suppose you have an HTML element like this:
Hello, world!Here's how you can access it in JavaScript using Document Object:
var element = document.getElementById("myElement");
Now, 'element' contains a reference to the corresponding element in the DOM. You can now manipulate or simply read information from this element. For instance, to retrieve the text inside the element, you could use the innerHTML property like so:
var text = element.innerHTML;
Changing Content with JavaScript Document Object
JavaScript Document Object is not just for fetching information. You can use it dynamically to alter the content on your webpage. For that, you use the innerHTML
property as a setter, as demonstrated below.
If you want to change the content of the above element, here's how you could do it:
element.innerHTML = "New content!";After executing this JavaScript code, the content within will change to 'New content!'.
User Interaction with JavaScript Document Object
The JavaScript Document Object plays a central role in enabling vibrant, interactive user experiences on the web. By setting up event listeners on your webpage elements, you can execute JavaScript code in response to user actions.
Here is an example of how you can create an event listener for a button click:
var button = document.getElementById("myButton"); button.addEventListener("click", function() { alert("Button was clicked!"); });In this example, whenever the user clicks the button with the id of 'myButton', an alert box with the message 'Button was clicked!' will pop up.
Manipulating Webpage Elements using JavaScript Document Object
Beyond just changing the content of an existing element on your webpage, you can use JavaScript Document Object to create entirely new elements or remove existing ones. This is how you can create dynamic websites that change in response to user actions or other events.
Here's an example of how you could create a new paragraph element and add it to the body of your webpage:
var paragraph = document.createElement("p"); paragraph.innerHTML = "A new paragraph!"; document.body.appendChild(paragraph);In this code, a new paragraph element is created, assigned some text, and then appended to the body of the webpage. This will add a new paragraph to the bottom of the page.
The JavaScript Document Object offers a limitless set of possibilities for you to interact and manipulate webpages. From getting elements, changing content, user interactions, to manipulating elements, the JavaScript Document Object forms the heart of dynamic web programming.
Guide to the Technique of Using Document Object in JavaScript
Gaining a firm grasp on the application of JavaScript Document Object in real programming situations can take your JavaScript knowledge to a whole new level. This practical guide will walk you through the important techniques on using the Document Object to handle events and manipulate elements programmatically, thereby making your web page dynamic and interactive.
Applying JavaScript Document Object in Programming Tasks
JavaScript Document Object offers an expansive array of techniques and uses within web development. It allows you to interact with HTML elements within a webpage, make changes programmatically and help the webpage respond to user events. Let's delve into how you can apply the JavaScript Document Object in your programming tasks.
Event Handling Using JavaScript Document Object
A significant aspect of interactive web development is event handling. In JavaScript, events are actions taken by users such as mouse clicks, key presses, or page load events. With the JavaScript Document Object, you can set up handlers for these events and trigger specific actions when the events take place.
For instance, if you want a certain operation to be triggered when a button on your webpage is clicked, this can be accomplished using the event handling function within the JavaScript Document Object Model. Here's how you do it:
var button = document.getElementById("btn"); button.onclick = function(){ alert('Button clicked!'); }
In the above code snippet, a simple HTML button is associated with a JavaScript function using the onclick event handler. This function triggers an alert with the message 'Button clicked!' whenever the button is clicked. This is a simple demonstration of the huge potential that handling user events via JavaScript Document Object carries.
Event handlers can be set for a wide array of user actions such as:
- Mouse click: onclick
- Keyboard key press: onkeypress
- Page Load: onload
The real challenge and the artistry in web development lies not just in setting up event handlers, but in using them to craft seamless user interactions, creating an immersive experience.
Selecting and Changing Elements with Document Object in JavaScript
A vital utility of JavaScript Document Object is to manipulate the HTML elements of a webpage. To carry out such a manipulation, you first need to select the element. The Document Object provides methods like getElementById
, getElementsByClassName
, getElementsByTagName
, etc. Here's a primer on selecting elements:
To select an element with a particular ID:
var element = document.getElementById('elementId');
If you want to select elements with a specific class name:
var elements = document.getElementsByClassName('className');
Once you've selected your HTML element or a set of elements, next comes the phase of manipulating these. Thanks to the JavaScript Document Object, you're empowered to make changes to the content of HTML elements, style them differently, hide/display them based on certain conditions, and so much more.
For instance, you could change the textual content of an HTML element like this:
element.innerHTML = 'Some new text';
Taken together, the selection and manipulation of HTML elements allow you to create a dynamic webpage that transforms and responds to the user's actions and preferences.
Causes of Errors in JavaScript Document Object and Overcoming Them
As with any other programming languages, JavaScript too is susceptible to errors. Largely, issues occur due to overlooking JavaScript Document Object's intricacies. Broadening your insights about common errors in JavaScript Document Object and refining your debugging techniques can significantly ease your programming journey. Here, you will also learn about best practices that many experienced developers follow to avoid running into common pitfalls.
Common Errors in Using JavaScript Document Object
JavaScript Document Object offers plenty of functionality which, if not used accurately, can result in unexpected errors. Below, you'll find a detailed discussion of common errors and how to address them.
One of the most common issues is the Uncaught TypeError: Cannot read property '...' of null. This occurs when you try to access a property or call a method on an object that doesn't exist or isn't yet available in the DOM. It most commonly happens when your JavaScript code runs before the webpage finishes loading and the DOM isn't fully ready. To fix this, you can place your script tag right before the closing
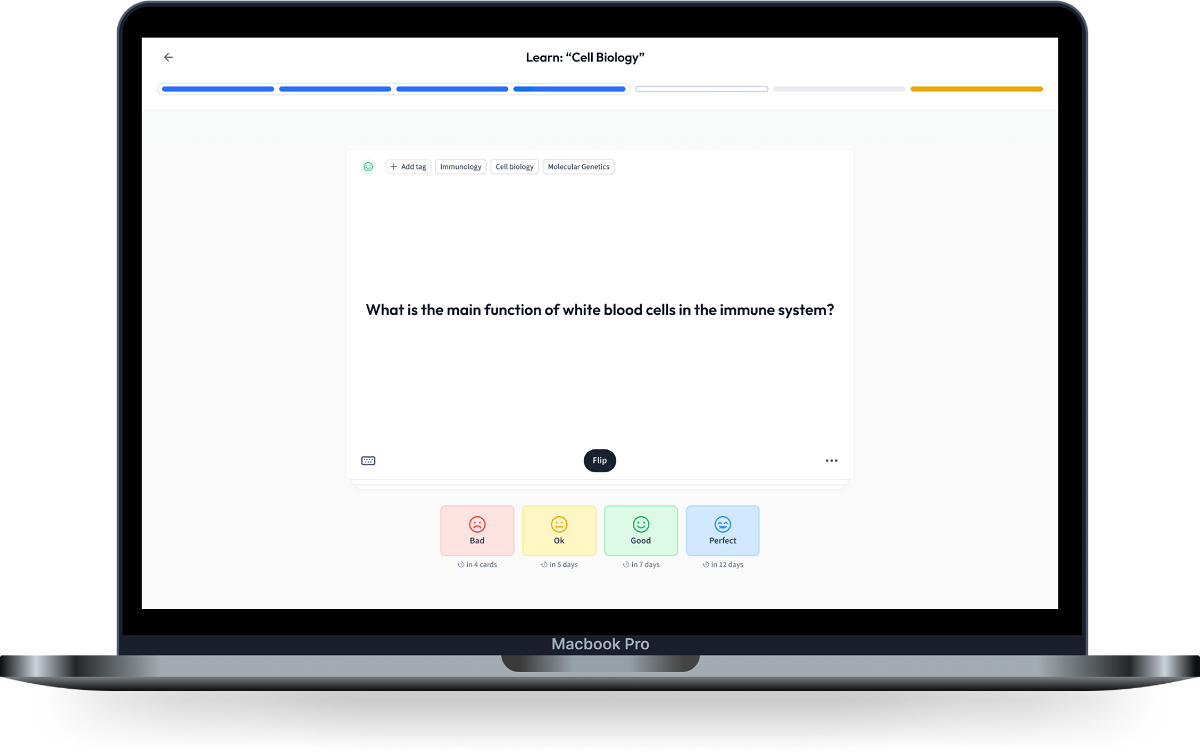
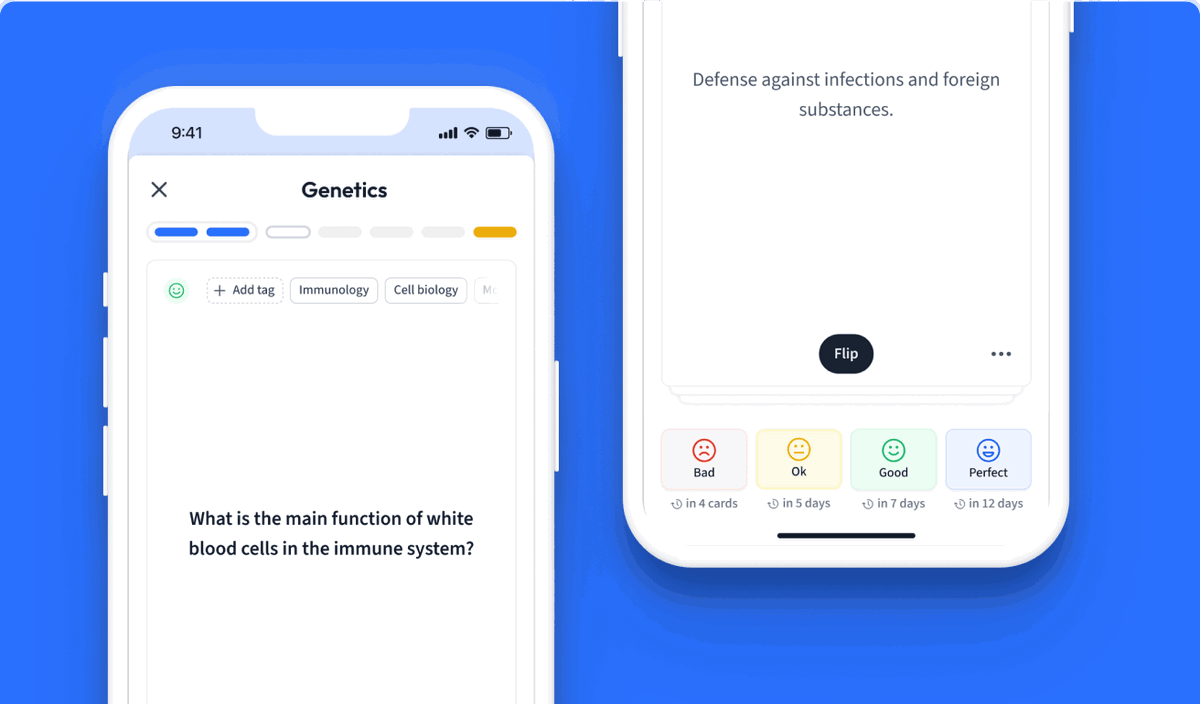
Learn with 15 Javascript Document Object flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Javascript Document Object
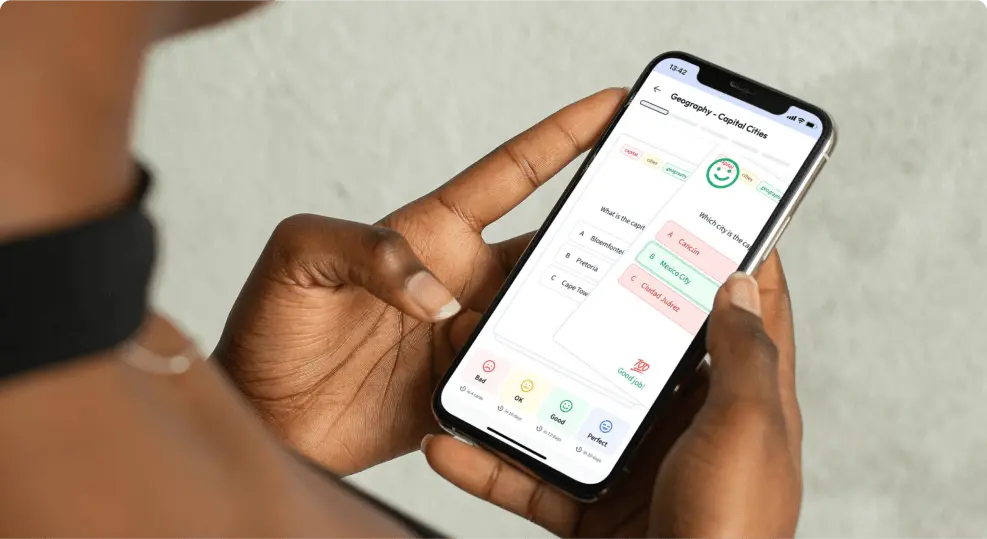
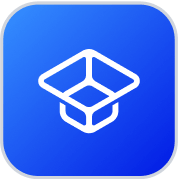
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more