Understanding Variables in C Programming
Variables play a significant role in C programming, as they store and represent data. They enable you to manipulate and store values throughout your program. In this section, we will explore the different types of variables available in C and how to declare and use them effectively.
Types of Variables in C
In C programming, variables are divided into several types, each of which can store specific data. These variable types include integer, character, float, double, and byte. It is crucial to understand their characteristics and how to use them appropriately to ensure your program works correctly.
Integer and Character Variables
Integer variables store whole numbers without any decimal part. The size of an integer variable can be either 2 or 4 bytes, depending on the compiler and system architecture. You can declare an integer variable using the int
keyword. For instance:
int counter;
Character variables are used to store a single character, such as a letter or a number. They take up one byte of memory and are declared using the char
keyword. Here's an example:
char letter;
A variable's data type determines the kind of data it can store and the amount of memory it occupies.
Float and Double Variables
Float variables store floating-point numbers, which are numbers with a decimal point. They use 4 bytes of memory and are declared using the float
keyword. For example:
float temperature;
Double variables, like float variables, store real numbers with a decimal point. However, double variables occupy 8 bytes of memory, giving them a larger range and more precision than float variables. They are declared using the double
keyword. For instance:
double salary;
Byte Variable in C
In C programming, the term "byte" refers to a group of 8 bits. To create a byte variable, you would use an unsigned char
data type, which can store values between 0 and 255. Here's an example:
unsigned char myByte;
Variable Declaration in C
Variable declaration is an essential step in any programming language, including C. It is important to understand how to declare variables correctly, using proper syntax and naming conventions.
Naming Conventions
To ensure your code is readable and maintainable, follow these naming conventions when declaring variables in C:
- Variable names should be meaningful and descriptive.
- Use lowercase letters for variable names.
- Use underscores to separate words in a variable name, e.g.,
item_count
. - Do not use reserved words or C keywords, such as
int
orif
. - Avoid starting variable names with an underscore or a digit.
Syntax and Examples
To declare a variable in C, you need to specify the data type followed by the variable name and an optional initial value. Here are some examples:
int age = 25;
float height_cm = 180.5;
char initial = 'M';
Global and Local Variables in C
Understanding the difference between global and local variables, their scope, and lifespan is essential when working with variables in C programming.
Global Variable in C: Definition and Usage
Global variables are defined outside any function and can be accessed throughout the entire program. They are stored in the data segment of the program's memory.
A global variable has a program-wide scope, meaning it can be accessed by any function within the program.
To declare a global variable, place the variable declaration outside any function, at the top of your C source file. For example:
int globalVar = 100;
void main() {
// Access and use the global variable here
}
Here's an example of using a global variable in a C program:
int counter = 0;
void incrementCounter() {
counter++;
}
void main() {
printf("Counter: %d\n", counter);
incrementCounter();
printf("Counter: %d\n", counter);
}
Local Variable in C: Scope and Lifespan
Local variables are declared within functions or blocks of code and can only be accessed within the specific function or block where they are defined. They are stored in the program's stack segment, and their lifespan only exists as long as the function or block is executing.
A local variable's scope is limited to the function or block of code where it is declared.
To declare a local variable, place the variable declaration inside a function or block of code. For instance:
void main() {
int localVar = 50;
// localVar can only be accessed within the main function
}
Another important concept in C programming is the use of "static" local variables, which retain their value between function calls. This can be useful when you need to track a value across various invocations of a particular function.
Importance of Static Variables in C
Static variables are essential in C programming as they provide unique values that persist across multiple function calls. They are beneficial in cases where tracking a value across various invocations of a function is necessary. In this section, we will further delve into their definition, properties, characteristics, and best practices to better understand their importance in C programs.
Static Variable: Definition and Properties
In C programming, a static variable is a special kind of local variable that retains its value even after the function or block of code in which it was declared has completed execution. Contrary to regular local variables, which are destroyed after the function or block terminates, static variables preserve their value throughout the entire runtime of the program.
Characteristics of a Static Variable
Static variables in C possess several unique attributes setting them apart from regular local and global variables. These features include:
- Memory Allocation: Static variables are allocated in the static memory area, not on the stack.
- Lifespan: They exist for the duration of the program execution, unlike local variables which have limited lifespans confined to their respective functions or blocks.
- Storage: The variable is stored in a single memory location and retains its value across different invocations of the function.
- Default Initialization: Static variables, if not initialized explicitly, are automatically initialized to zero.
- Visibility: The scope of a static variable is restricted to the function or block it is declared in, preventing unauthorized access to its value from other functions.
Static Variable in C: Examples and Best Practices
To declare a static variable in C, use the static
keyword before the data type and variable name. Here's an example:
void incrementCounter() {
static int counter = 0;
counter++;
printf("Counter: %d\n", counter);
}
In this example, the counter
variable is incremented each time the incrementCounter()
function is called. Since the variable is static, its value is preserved between function calls, resulting in its incremented value being displayed.
Here are some best practices to follow when working with static variables in C:
- Use static variables judiciously to avoid cluttering the static memory area.
- Remember that static variables are initialized only once, so be cautious when resetting their values if required.
- Limit the usage of static variables to cases where the value needs to be preserved across multiple function calls.
- Never use a static variable to share data between functions. Instead, use function arguments or global variables for that purpose.
- Keep the static variables restricted to the functions where they are genuinely needed to avoid issues related to scope and visibility.
By understanding their distinctive characteristics and adhering to the best practices mentioned above, you can precisely employ static variables in C programming to achieve efficient and organized code.
Implementing Variables in C: Practical Applications
Variables in C programming language have a wide range of practical applications, especially in decision-making and controlling the flow of execution using loops. To utilize variables effectively, you must have a thorough understanding of different control structures, such as conditional statements and loops, and how variables are employed within them.
Use of Variables in Decision Making
Decision-making in C programming relies heavily on variables and their values, allowing programs to run different sets of instructions based on specific conditions. Applying variables in decision-making control structures such as if-else and switch-case statements enables efficient and precise execution of desired actions.
Conditional Statements: If-else
Conditional statements, specifically if-else, are used to control the flow of your program based on specific conditions or the values of variables. Here, we discuss the syntax and usage of if-else statements, incorporating variables in the decision-making process:
- Syntax: The if statement is used to test a specific condition, while the optional else statement provides an alternative block of code to execute if the condition is false.
if (condition) {
// Statements to execute if the condition is true
} else {
// Statements to execute if the condition is false
}
In this example, you can use variables in the condition to make decisions. For instance:
int num = 10;
if (num > 0) {
printf("The number is positive.\n");
} else {
printf("The number is non-positive.\n");
}
Switch-case
Switch-case statements allow you to execute different blocks of code based on the value of a variable. They are an efficient alternative to using multiple if-else statements when the decisions are based on a single variable. The syntax for a switch-case statement is as follows:
switch (expression) {
case constant1:
// Statements to execute if expression equals constant1
break;
case constant2:
// Statements to execute if expression equals constant2
break;
...
default:
// Statements to execute if none of the constants match the expression
}
Here, the expression
is typically a variable whose value determines which case block is executed. The default
block runs when no cases match the expression. An example using a switch-case statement is given below:
int grade = 85;
switch (grade / 10) {
case 10:
case 9:
printf("A grade\n");
break;
case 8:
printf("B grade\n");
break;
case 7:
printf("C grade\n");
break;
...
default:
printf("Failing grade\n");
}
Implementing Variables in Loops
Loops in C programming enable repetitive execution of a block of code while a specific condition is true. Incorporating variables in loops as counters or sentinel values provides precise control over how many times the block of code is executed. Let's delve into the details of using variables in for, while, and do-while loops.
For Loop
For loops are used when you need to execute a block of code a fixed number of times. Variables play an essential role as loop control variables, initial values, and conditions in a for loop. The syntax for a for loop is as follows:
for (initialization; condition; loop control) {
// Statements to execute in each iteration
}
An example of a for loop using an integer variable as a counter is given below:
int i;
for (i = 1; i <= 10; i++) {
printf("%d\n", i);
}
While Loop
While loops are executed as long as the specified condition holds true. Variables are used in the condition, controlling the number of iterations. The syntax for a while loop is as follows:
while (condition) {
// Statements to execute in each iteration
}
An example of a while loop using an integer variable as a counter is provided below:
int counter = 1;
while (counter <= 10) {
printf("%d\n", counter);
counter++;
}
Do-while Loop
Do-while loops function similarly to while loops, but with one significant difference: the do-while loop checks the condition at the end of the iteration, ensuring that the block of code is executed at least once. Variables are used in the condition and loop control, just like in while and for loops. The syntax for a do-while loop is:
do {
// Statements to execute in each iteration
} while (condition);
An example of a do-while loop using an integer variable as a counter is presented below:
int counter = 1;
do {
printf("%d\n", counter);
counter++;
} while (counter <= 10);
In conclusion, understanding the practical applications of variables in decision-making and looping structures is essential for implementing efficient and flexible C programs. By mastering these concepts, you will be well-equipped to develop functional, dynamic, and complex code in C programming.
Variables in C - Key takeaways
Variables in C: Essential components for storing data, performing calculations, and controlling program flow; types - integer, character, float, double, and byte.
Variable declaration in C: Specify data type followed by variable name and optional initial value, e.g.,
int age = 25;
.Global variable in C: Defined outside any function, accessible throughout the entire program, stored in the data segment of program's memory.
Static variable in C: Local variable that retains its value across multiple function calls, stored in static memory area, automatically initialized to zero if not explicitly set.
Practical applications of variables in C: Decision-making using conditional statements (if-else, switch-case) and controlling flow of execution with loops (for, while, do-while).
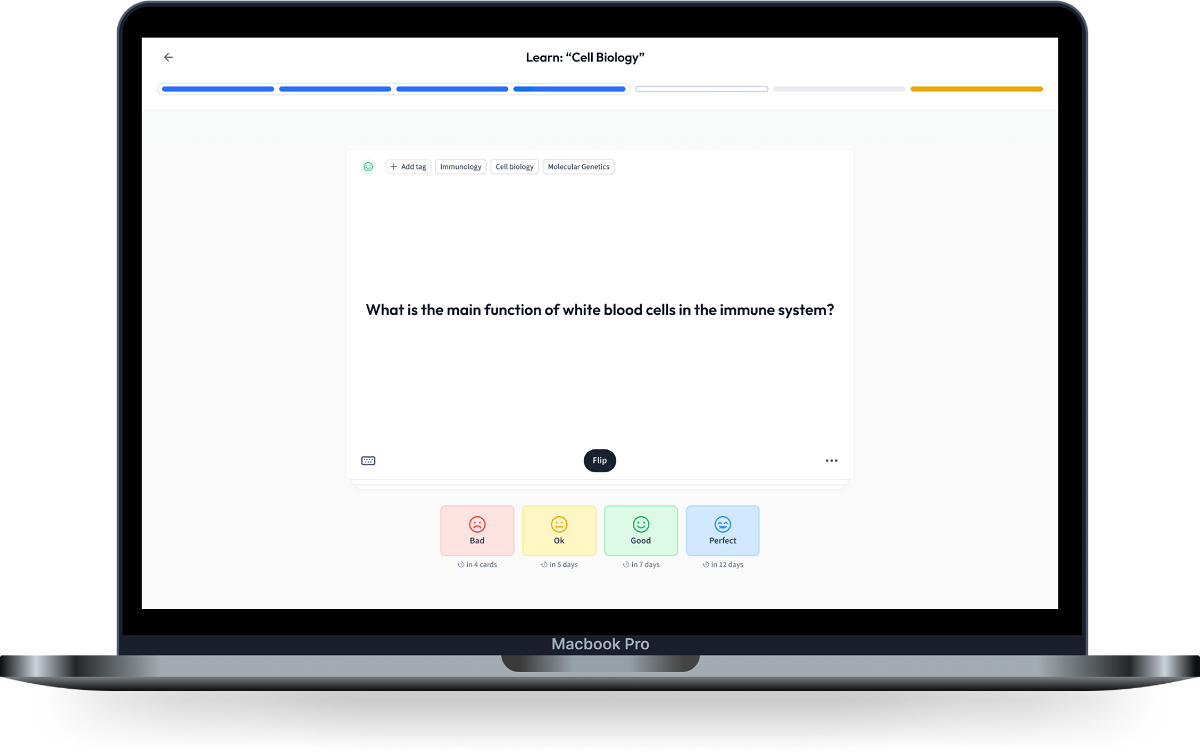
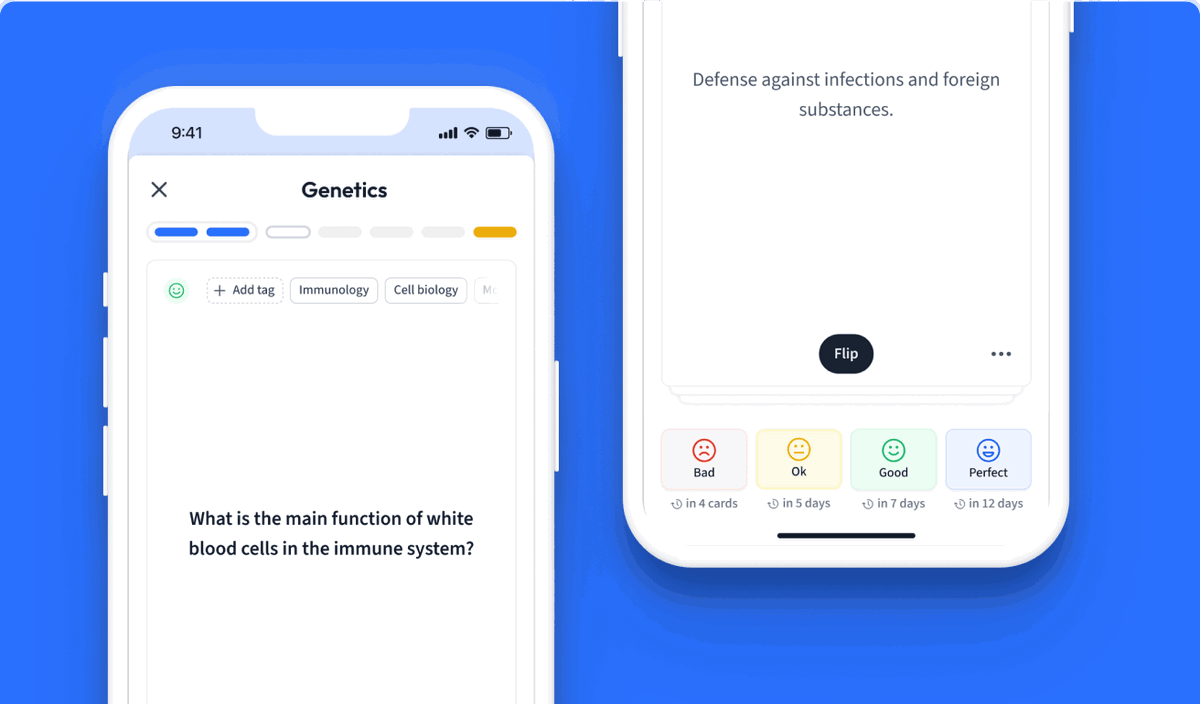
Learn with 15 Variables in C flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Variables in C
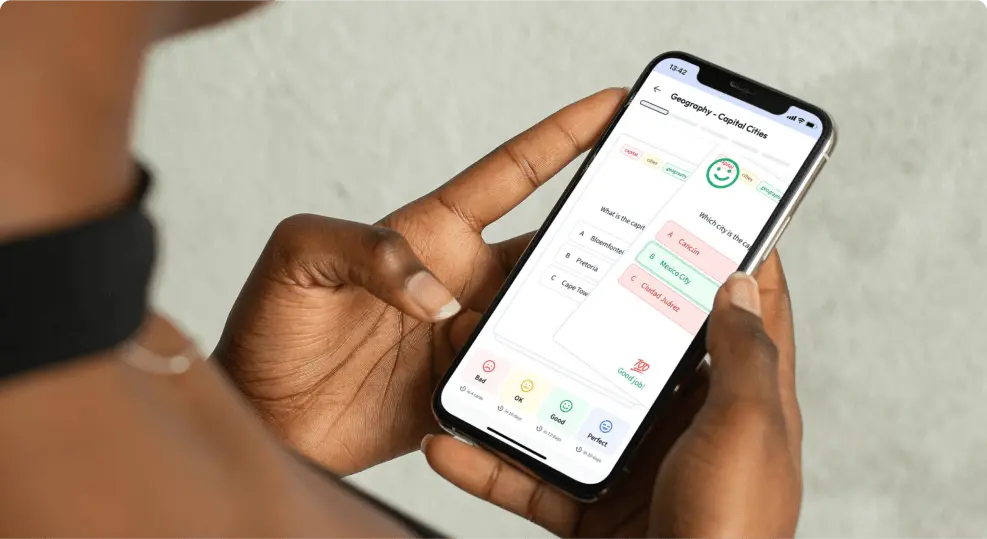
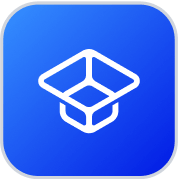
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more