What is a while loop in Python?
A while loop in Python is an important programming control structure which enables you to execute a block of code repeatedly, as long as a specified condition remains true. While loops are particularly useful when you need to perform an action multiple times, but the exact number of iterations is either unknown or depends on certain factors determined during the execution of your code.
While loop: A control structure in programming that executes a block of code repeatedly, as long as a specified condition remains true.
In comparison to other loop structures like the for loop, the while loop might have more flexible iteration patterns. This is because its execution does not rely on a predefined sequence or range, but dynamically on the evaluation of a condition.
How to create a while loop in Python
To create a while loop in Python, you need to follow these steps:
- Define a condition for the loop.
- Write the keyword 'while' followed by the condition you defined.
- Place a colon after the condition to indicate the beginning of a new block of code.
- Indent the block of code that you want to execute repeatedly.
- Ensure that the loop condition eventually changes to false, in order to prevent an infinite loop.
Here is a basic example of a while loop in Python:
counter = 0
while counter < 5:
print(f"Counter: {counter}")
counter += 1
In this example, the code block inside the while loop is repeatedly executed as long as the 'counter' variable is less than 5. With each iteration, the value of the counter is incremented by 1. Once the counter reaches 5, the condition evaluates to false and the loop terminates.
Example of a while loop used for validation:
user_input = ""
while user_input != "exit":
user_input = input("Enter a command or type 'exit' to exit: ")
print(f"You entered the command '{user_input}'")
Common use cases of while loops
While loops can be used for various purposes in Python programming. Some of the most common use cases include:
- User input validation: You can use a while loop to ensure that a user enters valid input by checking their input against certain validation criteria and prompting them to enter new input until it passes the validation check.
- Repeating actions until a specific condition is met: A while loop can be used to perform a certain action until a desired outcome is achieved, such as finding the first number in a sequence that meets specific criteria.
- Menu-driven applications: While loops are often used to create simple user interfaces that repeatedly display a menu of options and perform the corresponding actions until the user decides to exit the program.
- Calculations using iterative methods: Some calculations, such as finding the square root of a number or approximating the value of a mathematical function, can be performed using iterative methods which involve executing a loop until a certain level of accuracy has been achieved.
- Game loops: Many games use while loops to continuously update the game state and receive user input until the game ends or the player decides to quit.
In conclusion, the while loop is an essential and versatile control structure in Python programming. By mastering its usage and understanding common use cases, you can effectively enhance your coding abilities and create more efficient and flexible programs.
Working with Range in While Loop Python
In Python, the range function plays a significant role in generating a sequence of numbers, rather than a list, tuple, or other data structure. It is often utilised together with 'for' loops for iteration purposes. However, it can also be applied in conjunction with while loops to achieve similar outcomes.
Range function: A built-in Python function that generates a sequence of numbers, usually used in loops for iteration.
The basic syntax of the range function is as follows:
range(start, end, step)
The range function has three parameters:
- start: The starting value of the sequence (default: 0)
- end: The end value of the sequence, exclusive
- step: The amount to increment between values in the sequence (default: 1)
It is important to note that the 'end' parameter is required, while the 'start' and 'step' parameters are optional.
Implementing range within a while loop
While the 'range' function is commonly used with for loops, it can also be implemented in while loops to achieve similar iteration patterns. This can be done by converting the range function into an iterator using Python's 'iter()' built-in function and explicitly extracting the next value using the 'next()' function.
Here is an example of implementing range within a while loop:
start = 0
end = 5
iterator = iter(range(start, end))
while True:
try:
value = next(iterator)
print(value)
except StopIteration:
break
In this example, the range creates a sequence of numbers from 0 to 4 (5 values). The iterator is set to the sequence created by the range function and the 'next()' function is used within the while loop to extract each value. When the iteration reaches the end of range, it raises a 'StopIteration' exception, which is caught to break out of the while loop.
Practical examples of using range in while loops
There are various scenarios in which implementing range within while loops can be useful. The following examples provide an insight into these situations:
Example 1: Multiplication table using the range function in a while loop
table_number = 2
multiplier_range = iter(range(1, 11))
while True:
try:
multiplier = next(multiplier_range)
result = table_number * multiplier
print(f"{table_number} * {multiplier} = {result}")
except StopIteration:
break
In this example, the while loop is used to print the multiplication table of 2 using the range of multipliers from 1 to 10.
Example 2: Square numbers within a specific range
square_range = iter(range(1, 11))
while True:
try:
number = next(square_range)
square = number ** 2
print(f"{number} squared is {square}")
except StopIteration:
break
This example demonstrates the use of a while loop with range to calculate and print the squares of numbers in the range 1 to 10.
In conclusion, using the range function in conjunction with while loops allows for more flexible and creative iteration patterns. By understanding the implementation details and practical examples provided, you can successfully incorporate range within while loops for various problem-solving tasks.
Break and Continue in While Loop Python
The break statement is used to terminate the execution of the innermost loop (while loop or for loop) it is part of and resume the execution after that loop. In the context of a while loop, it is particularly useful for exiting the loop when a certain condition is met, even if the main loop condition remains true. This allows for more control over when to stop the loop, which is helpful in situations like searching for a particular element in a sequence or stopping the loop based on user input.
The use of the break statement in a while loop can be described by the following steps:
- Construct a while loop with a specific condition.
- Include an 'if' statement within the loop to check for an additional condition.
- Use the break keyword inside the 'if' statement body to exit the loop if the additional condition is met.
Here's an example that demonstrates the use of the break statement in a while loop:
count = 0
while count < 10:
print(count)
if count == 5:
break
count += 1
In this example, the while loop iterates until the count variable reaches 10. However, an inner 'if' statement checks whether the count is equal to 5. If this condition is met, the loop is terminated immediately using the break statement, even though the main loop condition (count < 10) remains true.
Employing continue in while loop Python
The continue statement, like the break statement, is used to control the loop execution. However, instead of terminating the loop, the continue statement skips the remaining part of the loop body for the current iteration and jumps to the next iteration, effectively continuing with the loop. This can be helpful in situations where you want to skip specific iterations, such as when filtering out certain values or processing data conditionally.
The use of the continue statement in a while loop can be described by the following steps:
- Construct a while loop with a specific condition.
- Include an 'if' statement within the loop to check for an additional condition.
- Use the continue keyword inside the 'if' statement body to skip the rest of the loop body for the current iteration if the additional condition is met.
Here's an example that demonstrates the use of the continue statement in a while loop:
count = 0
while count < 10:
count += 1
if count % 2 == 0:
continue
print(count)
In this example, the while loop iterates until the count variable reaches 10. The inner 'if' statement checks whether the count is an even number (using the modulo operator). If the condition is met, the loop body goes to the next iteration using the continue statement, thus skipping the print statement for even numbers. As a result, only the odd numbers between 1 and 10 are printed.
Real-world scenarios for break and continue statements
Both the break and continue statements are crucial tools for controlling loop execution in Python. Their practical uses can be found in various real-world programming scenarios, some of which are outlined below:
- Searching in a data structure: You may use the break statement to stop the loop when the intended element is found in a list, tuple, or another data structure without iterating through the entire data structure.
- Input validation: You can employ the break statement to exit a loop once the user provides valid input, allowing the program to proceed to the next task.
- Filtering data: Using the continue statement, you can filter specific elements from a data structure and process only the desired elements. For example, you might wish to process only positive numbers or strings that meet specific criteria.
- Error handling: The continue statement can be helpful when processing data that may contain errors or missing values, allowing you to skip the problematic entries and continue with the remaining data.
Understanding and implementing break and continue statements in while loop Python allows you to achieve more control over your loop execution, enabling you to write efficient, robust, and flexible code to solve complex programming challenges.
Advanced Techniques for While Loop in Python
Nested while loops refer to the practice of placing one while loop inside another. This technique is widely used for addressing multi-dimensional problems, where control structures must be executed within a loop to form a matrix or grid pattern. Some common applications of nested while loops include traversing matrices, performing calculations in multi-dimensional arrays, or creating complex iterative patterns.
Implementing a nested while loop involves the following steps:
- Construct an outer while loop with a specific condition.
- Inside the outer loop, create another while loop (inner loop) with a different condition.
- Include the code that needs to be executed during each iteration of the inner loop.
- Make sure you modify the inner loop condition to avoid infinite loops and update the outer loop condition accordingly.
Here's an example illustrating the use of nested while loops in Python:
outer_count = 1
while outer_count <= 3:
inner_count = 1
print("Outer loop iteration:", outer_count)
while inner_count <= 3:
print("\tInner loop iteration:", inner_count)
inner_count += 1
outer_count += 1
In this example, the outer loop iterates three times, and during each iteration of the outer loop, the inner loop will also iterate three times. The output represents the different combinations of the two loops, in the form of a 3x3 grid pattern.
Combining while loops with if-else statements
Combining while loops with if-else statements enables you to add conditional control structures within the loop, allowing for complex decision-making scenarios during iteration. This technique can be advantageous for numerous tasks, such as validating user input, filtering data, or controlling the flow of execution based on certain conditions.
To combine while loops with if-else statements, follow these steps:
- Construct a while loop with a specific condition.
- Inside the while loop, insert an if-else or if-elif-else statement based on another condition.
- Add the respective code blocks to be executed when the individual conditions are met, under their respective if, elif, or else clauses.
- Ensure the loop condition is updated properly to avoid infinite loops.
Here is an example demonstrating the combination of a while loop and an if-else statement:
number = 1
while number <= 10:
if number % 2 == 0:
print(f"{number} is even")
else:
print(f"{number} is odd")
number += 1
In this example, the while loop iterates over the numbers from 1 to 10, and for each number, the if-else statement checks if it is even or odd and prints the corresponding output. This demonstrates the conditional decision-making ability within a while loop using if-else statements.
Tips and tricks to enhance while loop efficiency
While using while loops in Python, there are various tips and tricks to enhance their efficiency, readability, and maintainability. Some of these tips include:
- Avoid Infinite Loops: Make sure that the while loop condition will eventually become false to prevent the creation of infinite loops. Always update the loop variable or condition accordingly within the loop body.
- Optimise Loop Body: Minimise the amount of computation performed within the loop by precomputing values or extracting common calculations out of the loop, if possible.
- Utilise Built-In Functions: Use Python's built-in functions, such as enumerate or zip, to simplify iteration over complex data structures or coordinate multiple iterators within a loop.
- Use Comprehensions: Consider using list, dictionary, or set comprehensions whenever possible to create a more readable and concise code, especially when generating new collections based on existing ones.
- Optimise Exit Conditions: If possible, exit the loop early using the break statement when an exit condition is met, to avoid unnecessary iterations.
By following these tips and tricks, you can improve the efficiency, readability, and maintainability of your while loops, helping you write higher-quality Python code.
while Loop in Python - Key takeaways
While loop in Python: A control structure that executes a block of code repeatedly as long as a specified condition remains true.
Range in while loop Python: The range function generates a sequence of numbers for iteration and can be implemented in while loops using the iter() built-in function and next() function.
Break in while loop Python: The break statement terminates the execution of the loop and resumes the execution after that loop, useful for exiting when a certain condition is met within the loop.
Continue in while loop Python: The continue statement skips the remaining part of the loop body for the current iteration and jumps to the next iteration when a specified condition is met.
Advanced techniques for while loop in Python: Nested while loops, combining while loops with if-else statements, and optimizing loop efficiency with various tips and tricks.
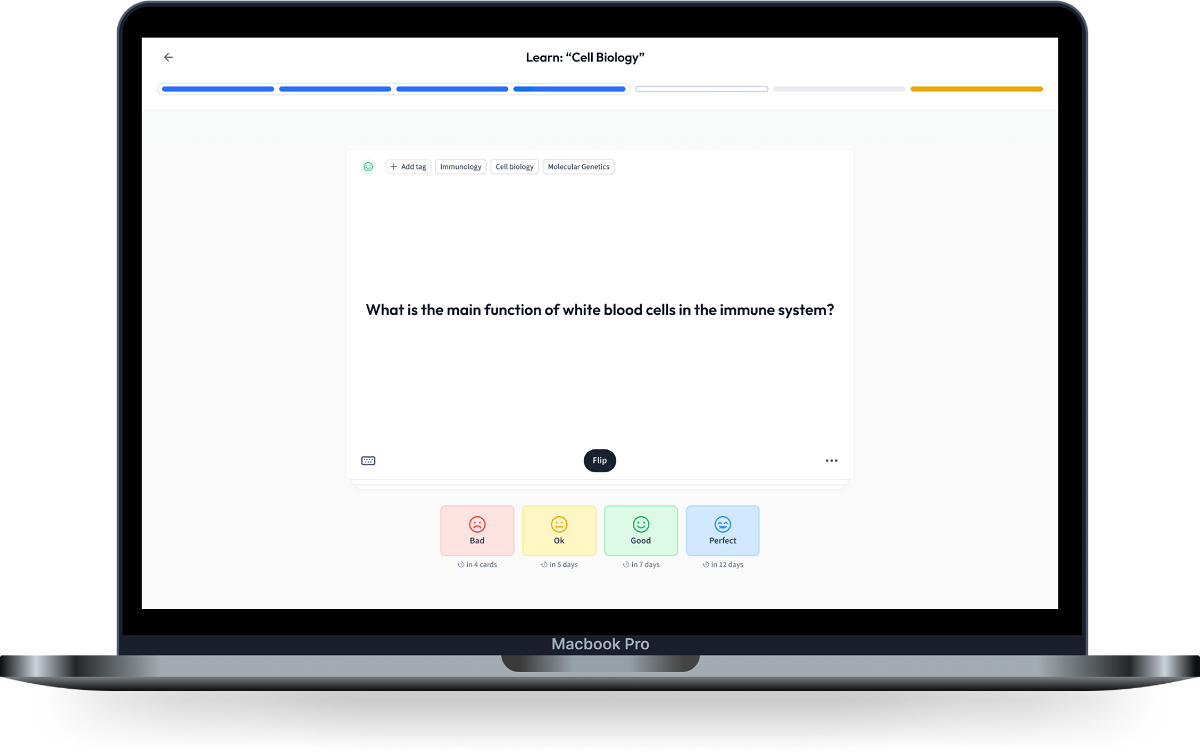
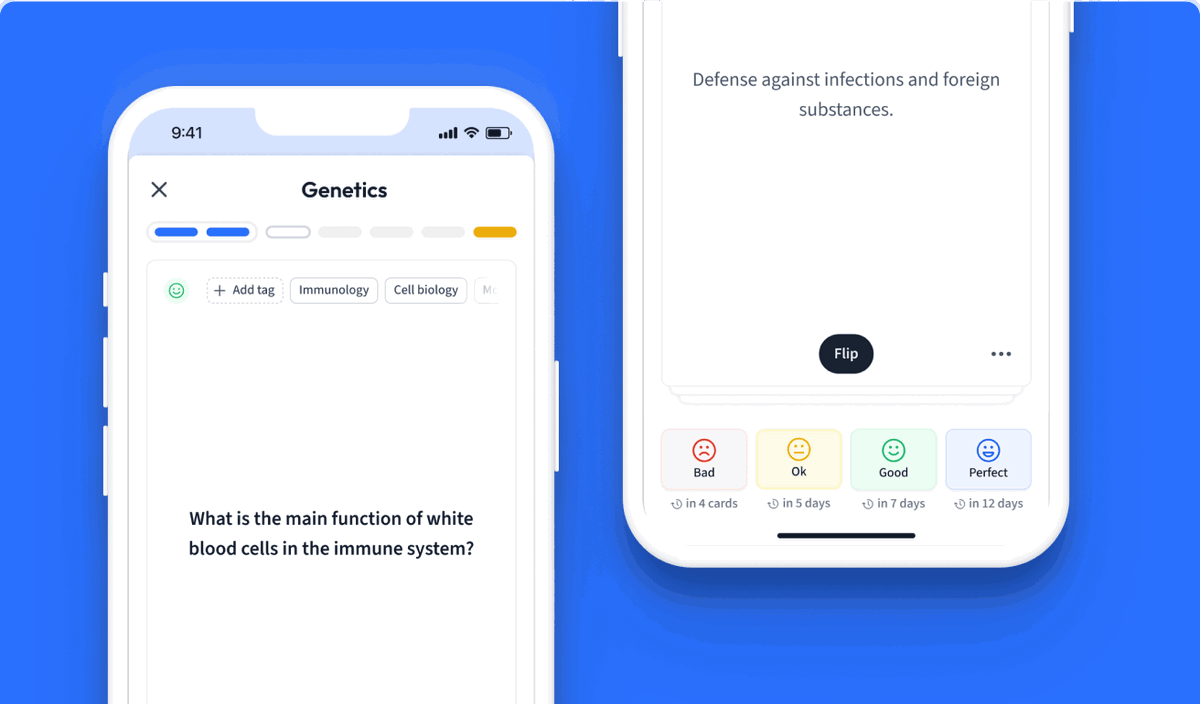
Learn with 30 while Loop in Python flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about while Loop in Python
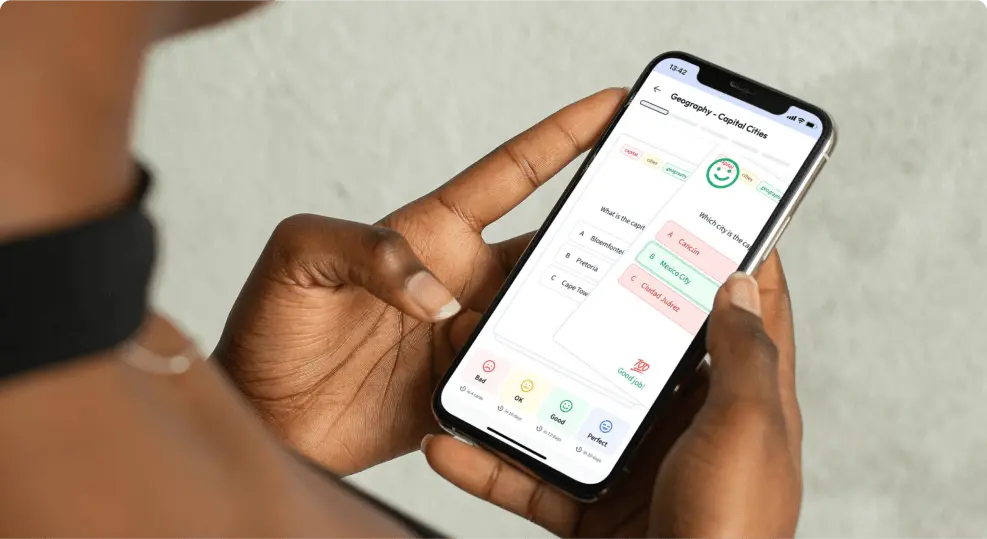
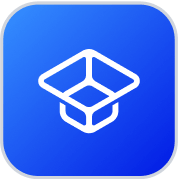
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more