Understanding Javascript Do While Loop
Diving deep into the Javascript world, one important element to understand is the Javascript Do While Loop. Below, you will find detailed information about this crucial concept in Javascript programming.Defining the Javascript Do While Loop concept
A Javascript Do While Loop is an important control structure in Javascript, which is used to repeat a block of code until a certain condition becomes false. Unlike other loop forms, it does not evaluate the condition before running the loop. Instead, it will execute the code block at least once before checking the condition.
What is Do While Loop in Javascript?
In Javascript, the Do While Loop is unique due to its 'post-test' loop nature. This means that the test condition, which controls the loop, is evaluated after the loop has been executed. The loop will always run at least once, regardless of the test condition. This is a contrast to a standard 'while loop' or 'for loop', which are 'pre-test' loops where the condition is tested before the loop is run.
Here's an example that illustrates the functioning of a do while loop.
do { statement(s); } while (condition);
Implying, that the 'statement(s)' is/are executed first, and then the 'condition' is evaluated. If the 'condition' is true, then the loop is executed again, and this continues until the 'condition' is false.
Analysing Do While Loop Syntax in Javascript
Javascript Do While Loop's syntax is quite similar to other looping structures. Below are its primary components:- ‘do’ keyword
- A code block (which is executed)
- ‘while’ keyword
- A condition to evaluate
Detailed Dissection of Javascript Do While Loop Syntax
Keyword 'do' | This keyword begins the do while loop structure. It is followed by the block of code within curly brackets { } |
Block of code | This is the segment of code that is to be repeatedly executed by the loop. It is enclosed within curly brackets { } |
Keyword 'while' | This keyword signifies the start of the condition expression. It is followed by a specific condition enclosed within parentheses ( ) |
Condition | This is the test condition which is evaluated after each iteration of the loop. If it's true the loop continues, else it halts. |
For Instance,
let i = 0; do{ console.log(i); i++; }while(i < 5);
In this example, Javascript Do While loop will print numbers 0 to 4. Firstly, 'i' is initialized to 0. The loop will run and print the value of 'i'. Then, 'i' is incremented by 1. This continues till 'i' is less than 5, post which the loop will terminate.
A Comprehensive Look at Do While Loop Example in Javascript
In this section, you will be taken through a comprehensive dissection and analysis of examples related to the Javascript Do While Loop. This will enable you to grasp not just the theoretical facets, but also the practical applicability of this distinct loop structure.Illustrative Samples of Do While Loop in Javascript
Exposure to a variety of examples is one of the most effective ways to comprehend a new concept. In this regard, the forthcoming segment shall illustrate a few select examples of the Javascript Do While Loop. The first case under consideration is a simple counter that utilises a do while loop to print numbers 1 to 5.let counter = 1; do { console.log(counter); counter++; } while (counter <= 5);In the given example, the variable 'counter' begins with the value of 1. During each passing of the loop, the value is printed and then incremented by 1. This cycle continues until the counter exceeds 5. Secondly, consider a more complicated scenario involving an array and a do while loop.
let arrayExample = [ 'apple', 'banana', 'cherry' ]; let i = 0; do { console.log(arrayExample[i]); i++; } while (i < arrayExample.length);In this case, the variable 'i' acts as the index for the array. Starting from 0, the script prints the value at the current index, before incrementing the index by 1. This cycle repeats until 'i' no longer falls within the array's length.
Practical Understanding: Javascript Do While Loop Uses
Linking theory with practice is essential to firm your grasp on any concept. Therefore, this section will comprehensively discuss the possible applications of a Javascript Do While loop. From fundamental to complex, each use-case will enhance your understanding. To begin with, Javascript Do While loops commonly feature in counter-controlled iterations. For instance, when a given task must be carried out a fixed number of times, such as printing the numbers from 1 to 100.let countUpToHundred = 1; do { console.log(countUpToHundred); countUpToHundred++; } while (countUpToHundred <= 100);In another example, suppose a programme requiring user input until it receives a valid value. The do while loop would be an apt choice, as it guarantees execution of the statement at least once, thereby seeking input before proceeding.
let userInput; do { userInput = prompt("Enter a valid number!"); } while (isNaN(userInput));Lastly, consider a complex scenario that emulates a guessing game. In this game, the aim is to guess the correct number. A Do While loop can ensure the game continues until the correct guess is made.
let correctNumber = 7, guessedNumber; do { guessedNumber = prompt("Guess the number!"); } while (guessedNumber != correctNumber);In these use-cases, the utility of Javascript Do While Loop becomes clear. While its usage can vary in complexity, the core remains intact - execute the code block once, and continue until the while condition evaluates to false.
Digging Deeper: The Technique of Do While Loop in Javascript
After having established the fundamental understanding of the Javascript Do While Loop, you should feel comfortable to delve deeper into the mechanics of this unique control structure. Let's further illuminate the advanced techniques and tips associated with this loop style.Javascript Do While Loop: Advanced Techniques and Tips
As you refine your Javascript skills, several powerful techniques become accessible that can significantly improve both the efficiency and quality of your coding work. Bear in mind that the Do While Loop, similarly to other control structures, is not merely a syntax rule to be memorised. Utilising it effectively requires logical thinking, practice, and a deeper understanding of the underlying concept. Firstly, consider the execution flow of the Do While Loop. Remembering the unique 'post-test' nature of the loop can help you employ it more strategically in your scripts. Given that the test condition is verified following the execution of the loop block, you should use a Do While Loop when the code block must be run at least once. Remember, also, that the loop will continue to iterate as long as the test condition remains true. A common pitfall among beginners is causing an 'infinite loop' by forgetting to update the variables affecting the test condition within the loop.let i = 0; do{ console.log(i); // A mistake: i is not being incremented, causing an infinite loop. }while(i < 5);
let outerLoop = 0; do{ let innerLoop = 0; do{ console.log(outerLoop, innerLoop); innerLoop ++; }while(innerLoop < 5); outerLoop++; }while(outerLoop < 5);
Making the Most of the Do While Loop Technique in Javascript
One of the key advantages of the Do While Loop technique is that it guarantees the execution of the loop's code block at least once. Therefore, it's beneficial in circumstances where user input is required before any further processing. For example, prompting users to fill out a form or input a suitable value. In some cases, you might want the loop to prematurely exit based on certain conditions. For this, use the 'break' keyword. This strategy can prove handy in cases such as looking for a specific value inside an array or database.let arrayExample = [ 'apple', 'banana', 'cherry', 'date', 'elderberry' ]; let i = 0; do { if(arrayExample[i] == 'cherry') { alert('Found the cherry!'); break; // Loop exits here } i++; } while (i < arrayExample.length);
Exploring the Difference Between While and Do While Loop in Javascript
Identifying and understanding the differences between the While and Do While loops in Javascript can greatly improve your ability to choose the correct structure for any given scenario. Through a comprehensive comparison, you'll gain clarity about which loop form best fits your coding needs.Contrasting While and Do While Loop in Javascript
For the sake of comprehensive understanding, it's crucial to compare the While and Do While loops side by side, highlighting similarities and, more importantly, differences. The primary disparity between these two loop structures lies in their checking condition mechanism. In a **While loop**, the condition is checked before the execution of the loop's code block. If the condition returns false on the very first check, the code block will not be executed at all.while (condition){ // Code to be executed }Conversely, the **Do While loop** follows a 'post-test' loop scheme. This means that the loop's code block will be manoeuvred once before the condition is evaluated. Hence, regardless of whether the condition is true or false, the code block inside a Do While loop will always run at least once.
do { // Code to execute } while (condition);To illustrate this difference:
- In the case of a While loop, if the condition is false, no iteration occurs.
- In contrast, for a Do While loop, despite the condition being false initially, the code block executes once.
Comparative Analysis: While vs Do While Loop in Javascript
Consider two examples demonstrating the distinct use of While and Do While loops with identical conditions and code blocks. Firstly, a While loop:let i = 5; while (i < 5) { console.log(i); i++; }The While loop will not run the code block because the condition i < 5 is false at the outset. Nothing will be printed to the console in this scenario. Now we will use a similar code block and condition, but within a Do While loop:
let i = 5; do { console.log(i); i++; } while (i < 5);Although the condition i < 5 is false when the loop starts, the console will print the number 5 once. This is because the Do While loop will always run its code block once, before the condition is checked. In terms of syntax, both structures are similar but have a slight difference as seen in their respective code structures shared above. Syntax isn't usually a decider when choosing between them. The significant deciding factor is often the requirement of whether the code block should run at least once, or ought to check the condition prior to the first execution. To summarise these differences:
Aspect | While Loop | Do While Loop |
Initial Check | The condition is check before first execution. | The loop executes once before checking the condition. |
Execution | Execution occurs only if the condition is true. | Execution happens at least once, irrespective of the condition. |
Syntax | Condition appears before the code block. | Condition appears after the code block. |
Further Insights into Javascript Do While Loop
The Do While Loop in Javascript is a potent control structure, but its usefulness does not stop at simple looping operations. Further applications can utilise the unique properties of the Do While Loop to deliver powerful and efficient solutions to a range of programming challenges. Let's unravel some of these advanced use cases.Advanced Use Cases of Do While Loop in Javascript
From manipulating data structures to managing user input, the Do While Loop can support a myriad of programming tasks. The key to unlocking these advanced use cases lies within a strong grasp of the principle distinguishing the Do While Loop from other looping structures: that the code block will always execute once, even if the condition is false at the beginning.A Data structure is a specialised format for organising and storing data.
var myArray = [1, 2, 3, 4, 5]; var i = 0; do { myArray[i] *= 2; i++; } while (i < myArray.length);In this example, the loop iterates over the elements of 'myArray', doubling the value of each element. It's also possible to use a Do While Loop for iterating through linked lists. Linked lists are unique data structures in which elements point to the next element in the list, often used in more complex data manipulation tasks.
var currentNode = headNode; do { console.log(currentNode.value); currentNode = currentNode.next; } while (currentNode !== null);In this script, each node in the linked list is accessed and its value logged to the console. Another robust use case of Do While Loop is in **interactive user processes**. The loop can be used to repeat a prompt until the user provides satisfactory input.
do { var userAge = prompt('Please enter your age'); } while (isNaN(userAge));In the interactive user process script, the prompt repeats until the user inputs a number, demonstrating a common use case in web development or any other user-oriented applications. From manipulating complex data structures such as arrays and linked lists, to designing user-friendly interactive processes, the Do While Loop plays a major role in Javascript programming.
Exploring Real-World Applications of Do While Loop in Javascript
Venturing deeper into real-world applications, we find the Do While Loop is a stalwart structure in many essential functions of Javascript programming. It can be regularly spotted in scripts managing user inputs and system prompts, complex multi-layered data or linked structures, and repetitive tasks with variable iterations. Consider **image processing applications**. They make heavy use of Do While Loops in combination with other control structures. Each pixel in an image is processed in a loop, and often these loops are nested within each other. One loop will process rows, and another, nested loop will process each pixel in a row.let x = 0; do { let y = 0; do { loadImage.pixel(x, y); y++; } while (y < loadImage.height); x++; } while (x < loadImage.width);In this fragment of code, the outer loop navigates through the rows, while the inner loop scans individual pixels in each row, applying the 'loadImage.pixel()' method. Consider as well the role of Do While Loops in **file system tasks**. When managing file inputs or outputs, the Do While Loop becomes a formidable tool for reading input streams or writing to output streams.
let dataChunk; do { dataChunk = fileInputStream.read(); if (dataChunk !== null) { console.log(`Read data: ${dataChunk}`); } } while (dataChunk !== null);The above script utilises the Do While Loop to read data from a file input stream and print it to the console. The loop will continue as long as there is data to read. Whether manipulating complex data in mathematical and computational applications, implementing highly interactive user interfaces, or supporting advanced file I/O operations, the Do While Loop is a versatile instrument in JavaScript, fit for real-world programming applications. Its flexibility makes it a bedrock construct in the coder's toolkit.
Javascript Do While Loop - Key takeaways
- Javascript Do While Loop: This looping structure begins with the keyword 'do' and is followed by a block of code to be repeatedly executed, enclosed by curly brackets. The keyword 'while' followed by the loop condition expression comes next. The loop repeats the block of code as long as the condition is true.
- Example of do while loop: The loop initializes a variable then executes the block of code to display the variable value and increments it, repeating until the condition no longer holds true, as shown in extracting and printing elements from an array or counting from 1 to 100.
- Difference between while loop and do while loop: The main difference is in the checking of the loop condition. In the while loop, the condition is checked before executing the block of code. Therefore, if the condition is false right from the start, the block of code will not execute at all. In contrast, for the do while loop, the block of code executes once before the condition is checked, guaranteeing at least one execution of the code block regardless of the condition.
- Technique of Do While Loop in JavaScript: This loop is mostly used in situations where the code block must be run at least once. Also, the do while loop can work with other control structures like nested loops for complex, multi-dimensional operations.
- Advanced use of the do while loop: Besides basic looping functions, the do while loop can be used in more complex tasks such as parsing arrays and performing operations on each element or iterating through data structures like linked lists.
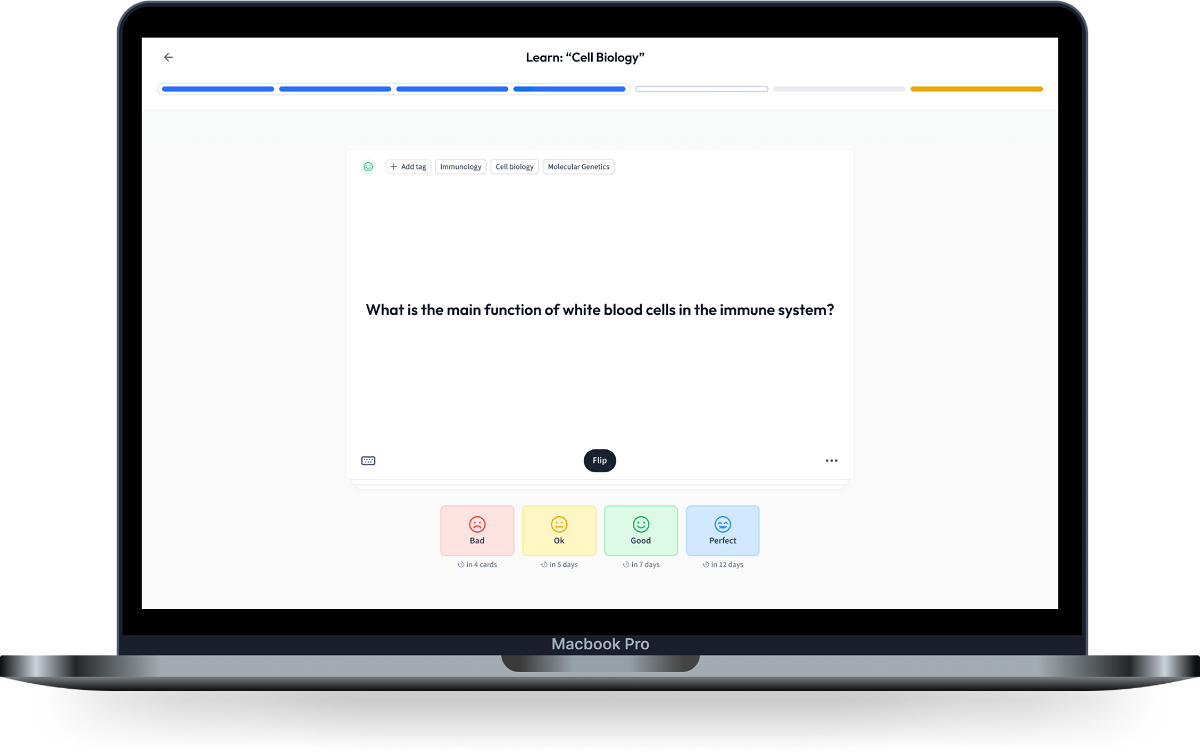
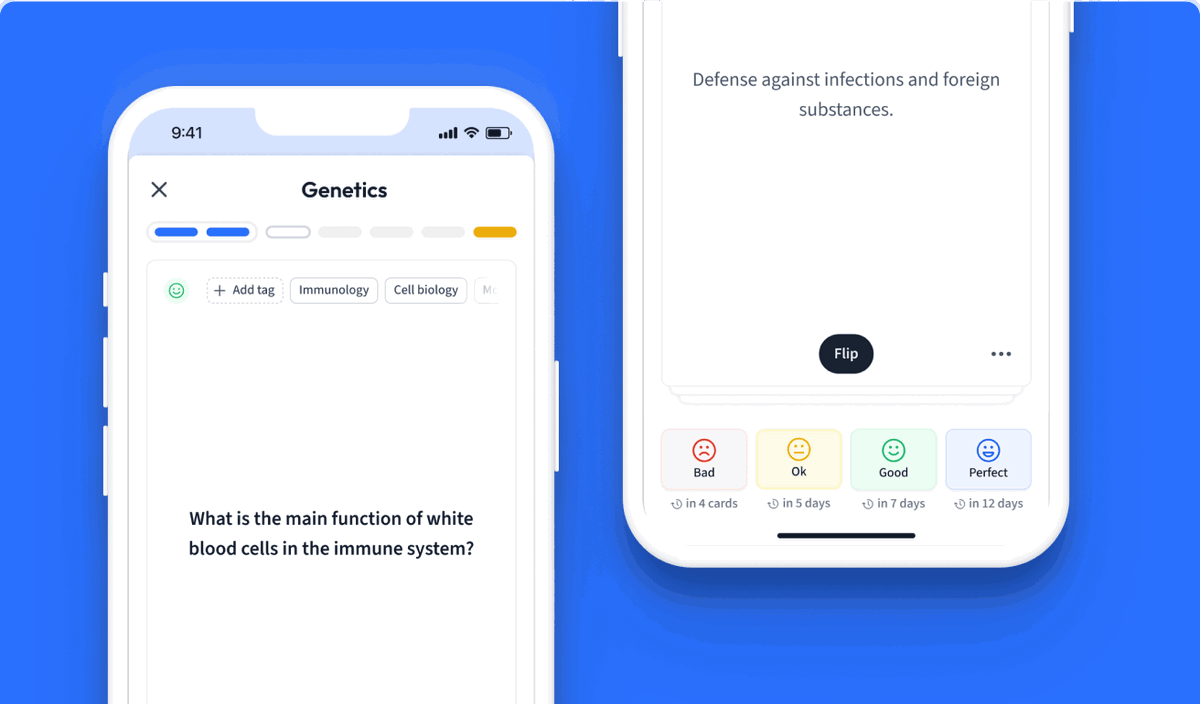
Learn with 15 Javascript Do While Loop flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Javascript Do While Loop
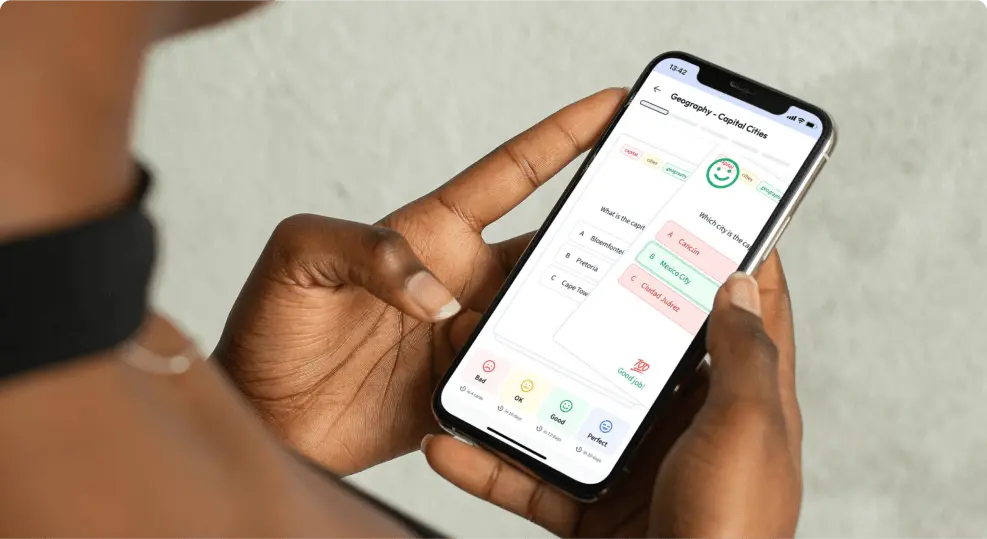
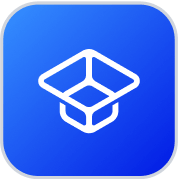
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more