Understanding Javascript Data Types
JavaScript is a programming language that alongside HTML and CSS, forms the foundation of creating web pages. In this journey towards understanding JavaScript, you'll find 'JavaScript Data Types' an essential topic to cover. These data types illustrate the various kinds of information you can store and manipulate within a JavaScript program.The Basics of Javascript Data Types
Underneath this JavaScript umbrella, you'll discover seven fundamental data types. With these data types, you can construct any data structure as per your requirement. Here are the seven types:- Number
- String
- Boolean
- Null
- Undefined
- Symbol
- Object
A Data Type in Javascript represents the type of value, instructing the JavaScript engine how the programmer wants to use the value.
Number Data Type in Javascript
The number data type in JavaScript represents both integers and floating-point values. There isn't any different type for integers in JavaScript hence all numbers can be either integer or decimal values.Let's consider some examples of numbers in JavaScript. For instance, 10 is an integer, and 10.25 is a floating-point value.
let integer = 10; let float = 10.25;Furthermore, use +, -, *, / for arithmetic operations. There are also certain special numeric values which are considered as belonging to this data type, some of which include Infinity, -Infinity, and NaN ("Not a Number").
String Data Type in Javascript
Strings in JavaScript are represented by the string data type. They serve as a way to store text. You may define strings using either single or double quotes.In the example, you can see how to define a string which reads, "Hello, world!":
let greeting = "Hello, world!";Interestingly, you can perform certain operations on strings. An operation like string concatenation done using the '+' operator allows you to combine strings.
Boolean Data Type Javascript
Then, we have the Boolean data type. It's pretty straightforward, really. A boolean value can either be true or false. Booleans are fundamental to control structures like loops and conditionals.As an example, let's consider a variable representing if it's raining or not:
let isRaining = true;
The world of JavaScript data types is vast and complex, filled with intricate detail and design decisions that affect how you work with data on the web. These three categories we've delved into – Numbers, Strings, and Booleans – represent just the beginning of the journey.
How to Change Data Types in Javascript
Manipulating and changing data types is a key aspect of JavaScript, and understanding how to do so efficiently can enhance the flexibility and functionality of your code. Luckily, JavaScript provides in-built methods to convert between its various data types as needed.Steps to Change Data Type in Javascript
In JavaScript, conversions can be either implicit, automatically done by the language, or explicit, where you manually convert through methods. Here are the broad steps to change data types in Javascript:- Understand the data type of the original variable.
- Determine the data type you want to convert to.
- Use proper JavaScript method or operation for conversion.
Converting to Number Data Type in Javascript
One of the most common conversions you might find useful is from a String or a Boolean to a Number. To convert to a number data type, you can use the Number() function or simpler methods like the unary plus (+) or minus (-) operators. For example, given a string "1234", you can convert this string to a number as follows:let str = "1234"; let num = Number(str); // explicit conversionYou can also use the unary plus (+) operator to implicitly convert a string to a number:
let str = "1234"; let num = +str; // implicit conversionIt's noteworthy that if your string contains non-numerical characters, both methods will return NaN.
Changing to String Data Type in Javascript
Sometimes, you'll find a need to convert other data types to a String. It's as easy as calling the String() function or indirectly forcing a conversion by performing a string operation like concatenation. For instance, to convert a number to a string, you can simply do:let num = 42; let str = String(num); // explicit conversionAlternatively, use concatenation with an empty string to implicitly make the conversion:
let num = 42; let str = num + ""; // implicit conversionFinally, the toString() method is another way tou can convert a number to a string, with the only caveat being that it fails when the value is null or undefined. In Javascript, the possibilities with data types and conversions are many and varied. As you get the most effective methods to these conversions, your ability to create flexible and dynamic codes in JavaScript is greatly enhanced.
Checking Data Types in Javascript
As you navigate through JavaScript, the need to check the type of a data value is inevitable, whether it's detecting user input, validating JSON structure, or debugging code. Luckily, JavaScript accommodates this through several techniques or methods, which are notably easy to grasp.Methods to Perform Javascript Check Data Type
Empowered by JavaScript, you can determine the type of a variable or a value by employing a handful of methods. Among these methods, the most fundamental ones include typeof and instanceof operators. Additionally, functions like Array.isArray() and Object.prototype.toString.call() play a crucial role when dealing with specific value checks.- typeof: Gives the data type of the operand
- instanceof: Checks whether an object is an instance of a specific class
- Array.isArray(): Confirms whether the passed value is an array
- Object.prototype.toString.call(): Works for more complex data type checks
Understanding the typeof Operator
The typeof operator is the fundamental method for checking data types in JavaScript. When you apply it to an operand, it returns a string that represents the data type of the operand. The general syntax is as follows:typeof operandHere's how you apply the typeof operator to check a variable's type.
let message = "Hello, World!"; let messageType = typeof message; // return "string"Apart from identifying bigints, numbers, strings, and booleans, the typeof operator is even capable of discerning functions and undefined values. However, the operator is not flawless. Especially when working with null values or arrays, typeof can prove misleading since it identifies both as an object. In these cases, you'd have to employ other methods like Array.isArray() or null check.
Checking for Boolean, Number, and String Data Types
To ascertain if a value is Boolean, Number, or String, The typeof operator can play a central part. For instance, to check if a value is of Boolean type, you'd compare the typeof result with the string "boolean". This is shown in the example below:let isStudent = true; if (typeof isStudent === "boolean") { console.log('This is a boolean'); }A similar principle applies to strings and numbers. Here's an example of how you can confirm if a value is a number:
let age = 24; if (typeof age === "number") { console.log('This is a number'); }The string check works on a parallel fashion as shown here:
let greeting = "Hello, there!"; if (typeof greeting === "string") { console.log('This is a string'); }Keep in mind, while confirming whether a value is of a Boolean, Number, or String type, using the triple equals (===) is vital. This is because the triple equals checks for both the value and the type, hence ensuring an accurate check. Remember, the process of checking the types of values can be exceedingly instrumental in debugging, handling exceptions, and most importantly, understanding how your code manipulates and processes data.
Understanding the Syntax for Javascript Data Types
Understanding the syntax for basic JavaScript data types such as number, string, and boolean is integral to script creation. Crafting the syntax correctly is pivotal to setting up variables and working with values effectively.Syntax for Defining Number, String, and Boolean Types
When defining number, string, or boolean types in JavaScript, you have to follow specific rules or syntax. The process generally revolves around the let keyword, the assigned variable, and the value coupled by its datatype. Here's the basic syntax for type definition:let variableName = value;In this setup, let is the keyword introducing the variable declaration statement. The variableName represents the name of your variable, and the value signifies the assigned value. The data type is inferred through this value automatically by JavaScript. Now, let's scrutinize the syntax for each fundamental JavaScript data type in more detail.
Syntax for Number Data Type in Javascript
In JavaScript, numbers can either be integers or floating-point values. The syntax for defining a number in JavaScript does not require any special characters—just declare your variable and assign a numerical value to it. Here's the syntax for defining a Number:let variableName = number;In this syntax, number represents any numerical value. Here is an example:
let studentCount = 30; let piValue = 3.14;In the first line, studentCount is declared as a number with an integer value of 30. In the second line, piValue is another number, but this time it's a floating-point value.
Syntax for String Data Type in Javascript
The string data type in JavaScript serves to represent textual data. String values must be enclosed within either single quotes (' '), double quotes (" "), or backticks (` `). Here's the syntax for defining a String:let variableName = "text";In this syntax, "text" stands for any sequence of characters. For example:
let greeting = 'Hello'; let name = "John"; let phrase = `Good morning, ${name}`; // Template literalIn the example, greeting and name are both string variables. The phrase is a unique form of string known as a template literal. Here, it dynamically includes the value of the name variable within the string.
Syntax for Boolean Data Type Javascript
As for the Boolean data type in JavaScript, you'll navigate between two possible values: true or false. Booleans are mighty useful in creating conditional statements and loops. Here's the syntax for defining a Boolean:let variableName = true/false;In this syntax, true/false represents either of the boolean values. Take a look at this example:
let isRaining = false; let hasCoffee = true;In this example, isRaining and hasCoffee are boolean variables. The first is set to false, indicating it's not raining, and the latter is set to true, implying the presence of coffee. As you see, understanding the syntax for defining different data types in JavaScript is a matter of knowing where and how to use these values. With practise, your ability to read and write JavaScript will enhance exponentially.
Looking at Javascript Data Types Examples
Unleashing the full power of JavaScript comes with a deep understanding of its data types, their behaviour and how to use them correctly in various scenarios. So, let's roll up our sleeves, and dive into real examples of number, string, and boolean data types in JavaScript code.Examples of Number, String, and Boolean Data Types
One of the brilliant facets of JavaScript is its dynamic and loosely typed nature, which allows variables to hold values of any data type. In this section, we'll look at examples of how to use and manipulate the number, string, and boolean data types, covering their initialisation, assignment, and manipulation in JavaScript code.Numerical Javascript Data Types Examples
In JavaScript, numbers are used to hold numerical values. Both integers and floating-point numbers fall into this data type. Let's take a look at some examples of numerical JavaScript data types:let wholeNumber = 7; let decimalNumber = 3.14;In these examples, wholeNumber is an integer while decimalNumber is a floating-point number. You can perform various mathematical operations with numbers. For instance:
let a = 5; let b = 2; let sum = a + b; let difference = a - b; let product = a * b; let quotient = a / b; let remainder = a % b;In the above snippet, the sum, difference, product, quotient, and remainder are computed using JavaScript's arithmetic operators, showcasing the versatile usage of number data types.
String Javascript Data Types Examples
On the other hand, strings represent sequences of characters. They can be defined in JavaScript using single quotes, double quotes, or backticks, which are used for template literals. Check out these examples:let singleQuotes = 'single quotes string'; let doubleQuotes = "double quotes string"; let templateLiteral = `A template literal with ${singleQuotes}`;In these examples, singleQuotes and doubleQuotes are simple string values. However, templateLiteral is a template literal, providing an elegant way of embedding expressions within strings. You can perform several operations on strings too, such as concatenation or searching for substrings:
let hello = 'Hello'; let world = 'world'; // Concatenation let helloWorld = hello + ' ' + world; // 'Hello world' // Searching for substrings let position = helloWorld.indexOf('world'); // 6These operations illustrate how strings in JavaScript can be concatenated, and how you can search for substrings within a string.
Boolean Javascript Data Types Examples
Booleans, on the other hand, represent logical values and can have only one of two values: true or false. Here's how you might define boolean variables:let isDay = true; let isNight = false;In this example, isDay is set to true, signifying it is day time. Conversely, isNight is set to false, indicating it's not night time. Booleans prominently feature in conditional statements, where they can help execute different blocks of code based on certain conditions:
let age = 16; if (age >= 18) { console.log('You can vote!'); } else { console.log('You are too young to vote.'); }In the above example, a condition checks whether the value of the age variable is greater than, or equal to, 18. Depending on this condition, different pieces of code are executed, efficiently using the boolean data type to control program flow. By mastering JavaScript data types through practical examples, you garner the knowledge necessary to write versatile, efficient, and cleaner code, making your problem-solving process both effective and enjoyable.
Javascript Data Types - Key takeaways
- Javascript Data Types include numbers, strings, and booleans.
- In Javascript, numbers can be integers or floating-point values, and can be used for arithmetic operations.
- String data type in Javascript represents text, and strings can be defined using single or double quotes.
- Boolean data type in Javascript represents a value that can either be true or false and is used in control structures like loops and conditionals.
- The syntax for Javascript data types involves the let keyword, a variable name, and a value.
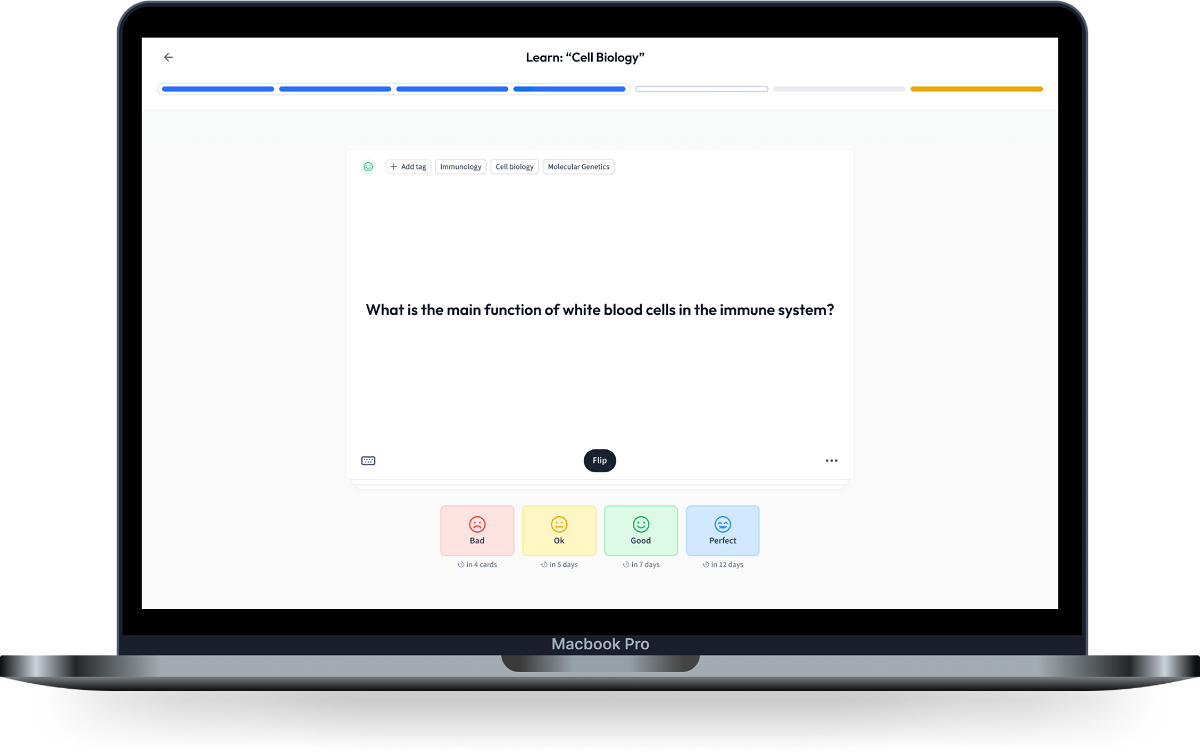
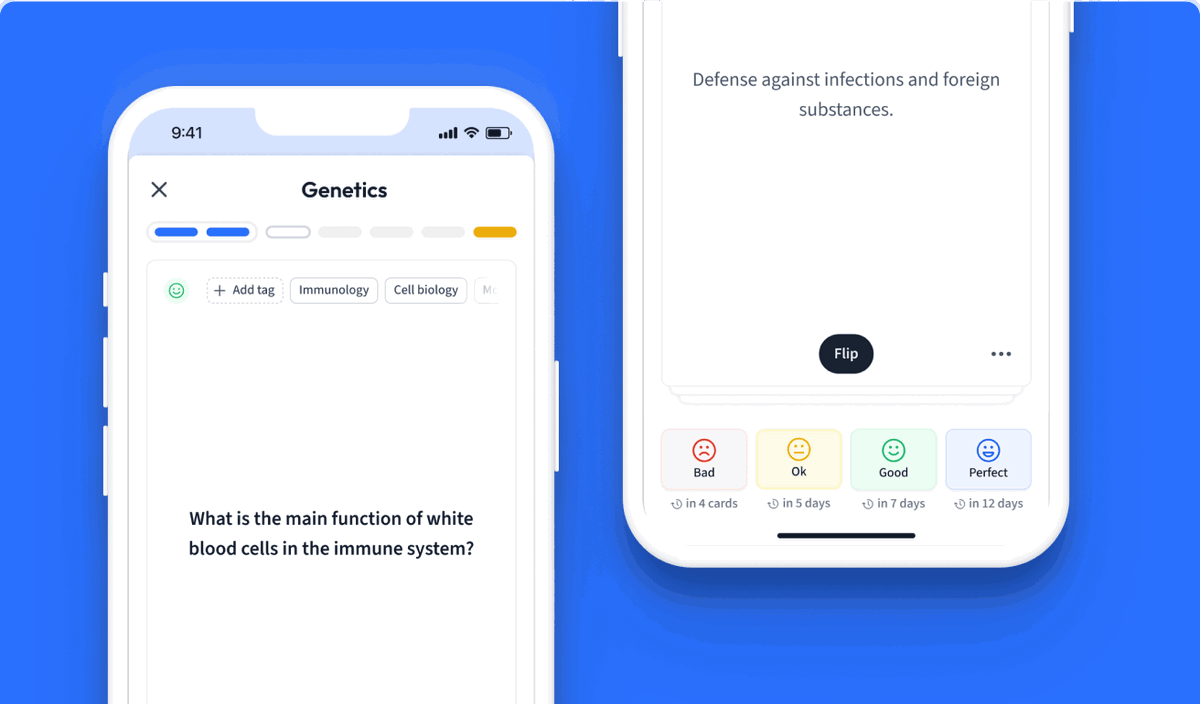
Learn with 57 Javascript Data Types flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Javascript Data Types
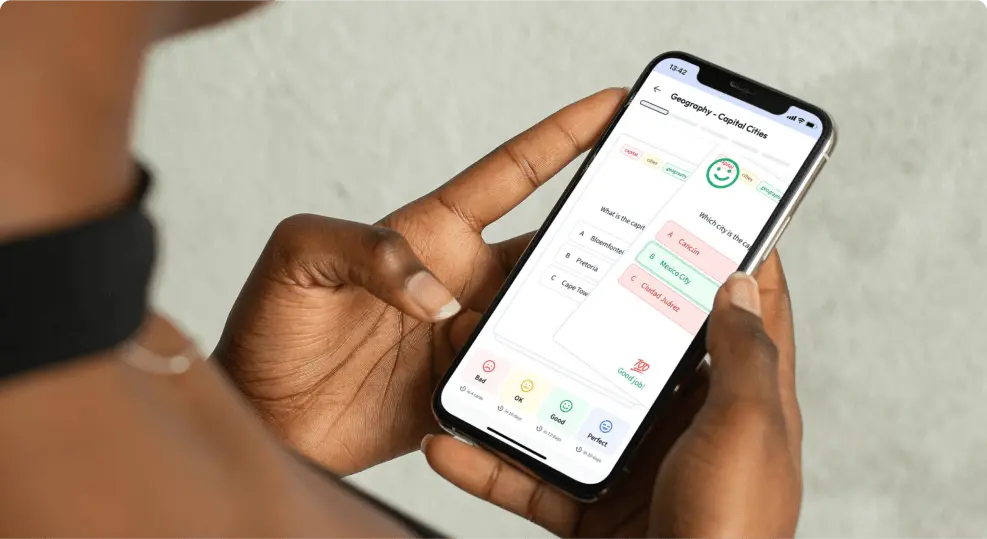
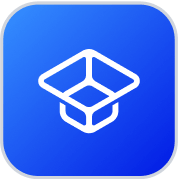
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more