Understanding Java Method Overloading
Let's dive into the realm of Java programming and unravel the concept of Java Method Overloading. This topic holds immense significance not only for budding Java developers but also for seasoned software professionals.What is Method Overloading in Java?
Method Overloading in Java is an essential feature of Object-Oriented Programming. It is a mechanism which enables a class to have two or more methods having the same name but different parameters. This distinctiveness of parameters could refer either to a difference in the type of parameters or their number.Method Overloading: A technique in Java where a class can have more than one method with the same name, but different parameters.
The Basics of Method Overloading in Java
How does one overload a method in Java? It's straightforward! Just declare multiple methods using the same name but ensure each has different parameters, either in type or number. Here's the basic structure:void methodName (int parameter1) { // Code } void methodName (double parameter1, double parameter2) { // Code }Note that method overloading is not determined by the return type of the method. If two methods have the same name, same parameters, but a different return type, Java does not consider it as method overloading.
Key Characteristics of an Overloaded Method in Java
Here are some noteworthy characteristics of overloaded methods in Java:- Method overloading enhances code readability and re-usability since you can use the same method name for similar actions.
- Method overloading is determined at compile-time, hence also known as compile-time polymorphism.
- Methods can be overloaded in the same class or in subclasses.
class Geometry { void calculateArea(int side){ int area = side * side; System.out.println("Area of square is: " + area); } void calculateArea(int length, int breadth){ int area = length * breadth; System.out.println("Area of rectangle is: " + area); } }
Methods of Overloading in Java
Overloading methods in Java comes with a set of rules and practices that dictate the process. There are few ways developers generally accomplish method overloading. This primarily involves manipulating the type and number of arguments, and as well as the order of arguments in method declarations. Most importantly, method overloading can't be achieved by a change in return types alone.Constructs Used for Method Overloading
Method overloading in Java is achieved by using different constructs and variations; predominantly these variations are related to the method's parameters.Parameters: The variables passed to a method which influence the method's functionality. In the context of method overloading, parameters can be distinguished based on their type, their number, and their sequence.
- Varying Number of Parameters: Overloaded methods can have a different number of parameters.
- Different Data Type of Parameters: The methods can have parameters of different data types.
- Different Order of Parameters: If two methods have the same number and type of parameters, the position (or sequence) of the parameters can be changed to overload the methods.
Guidelines for Overloading Methods in Java
When overloading methods in Java, the developer must be cognisant of a set of guidelines and principles that direct this process.Here is a table with some critical guidelines for overloading methods in Java:
Guideline | Description |
Same Method Name | The name of the methods should be identical for overloading to occur successfully. |
Different Parameter List | Parameters must differ either in their type, their number, or their sequence. |
Return Type Doesn't Matter | Contrary to common assumption, the return type is not part of the method signature and does not participate in method overloading. |
Compile-Time Polymorphism | Method overloading is determined at compile-time, hence classified as compile-time polymorphism or static polymorphism. |
Practical Method Overloading Examples in Java
To further illuminate the concept of method overloading in Java, let's take a practical example.public class Vehicle { void run(int speed){ System.out.println("The vehicle is running at " + speed + "km/hr."); } void run(int speed, int gears){ System.out.println("The vehicle is running at " + speed + "km/hr with " + gears + " gears."); } }In the above example, the method 'run()' is overloaded to accommodate different states of the 'Vehicle' class. This provides flexibility and a more detailed description of the vehicle's state depending on the input parameters. It's always crucial to remember that method overloading is about making your code cleaner, more flexible, and more intuitive. Extensive utilization of method overloading can facilitate writing highly efficient and readable code.
Method Overloading vs Method Overriding in Java
As you venture deeper into Java, you come across several techniques that let you write flexible, versatile, and efficient code. Among them, Method Overloading and Method Overriding stand out. On the surface, they seem quite similar, bearing names that bear resemblance. However, they are fundamentally different, each with their unique purposes and applications in Java programming.Differences between Method Overloading and Method Overriding
Before you dive deep into the technicalities, draw a concrete distinction between Method Overloading and Method Overriding.
Method Overriding: This is when a subclass provides a specific implementation of a method that is already provided by its parent class. It is used for runtime polymorphism and to provide the specific implementation of the method.
- Method Overloading is about having the same method name but with different parameters within the same class, while Method Overriding is all about providing a specific implementation to a method already provided by its superclass. Essentially, in Method Overriding, the method in a subclass has the same name, type, and parameters as the one in its superclass.
- Method Overloading is the example of compile-time (or static) polymorphism, while Method Overriding is an example of runtime (or dynamic) polymorphism.
- Method Overriding is done across classes that have inheritance relationships, while Method Overloading can be accomplished within a single class.
- The return type can be different in Method Overloading, but it must be the same or covariant in Method Overriding.
Understanding when to use Method Overloading vs Method Overriding
Now that you have a good grasp on what differentiates Method Overloading and Method Overriding, it’s time to take a closer look at when to use each ability. Method Overloading is generally used when you want a class to have more than one method that performs similar tasks yet with different parameters. It is a way of increasing readability and reusability of the code. For example, you might need to carry out an operation with two integers or three integers, with Method Overloading you can create two separate methods with the same name but different parameters:void addNumbers(int num1, int num2) { // addition code here } void addNumbers(int num1, int num2, int num3) { // addition code here }On the other hand, Method Overriding is primarily used to define a method in a subclass that the superclass already provides. By overriding a method, you can inherit the methods of a superclass and still can change the behaviour of a method based on your requirements in the subclass. An example of this would be to override the 'draw()' function in subclasses 'Rectangle' and 'Circle' while inheriting from the superclass 'Shape':
class Shape { void draw() { // draw a generic shape } } class Rectangle extends Shape { @Override void draw() { // draw a rectangle } } class Circle extends Shape { @Override void draw() { // draw a circle } }Having a clear understanding of these two operative concepts in Java, will enable you, as a developer, to exploit Java's flexibility to the fullest. It opens the door to writing more flexible, efficient and up to quality code, fulfilling the core principles of Object-Oriented Programming.
Analysing a Java Method Overloading Example
One of the most effective ways to truly understand Java Method Overloading is by diving into practical examples, ranging from a simple one to a more complex one. Doing so will solidify your grasp of this essential Java concept, enabling you to write code that is clearer, more adaptable, and efficient. So, let's get you on the track to becoming a more competent Java programmer.Breakdown of a Simple Method Overloading Example in Java
To start off, you'll take a look at a straightforward example of Java Method Overloading. Here's a simple code of an overloaded method 'Sum()':class Mathematics { public static int add(int a, int b) { return a + b; } public static double add(double a, double b) { return a + b; } }The example above illustrates a simple case of method overloading happening within the same class. Essentially, the same method name 'add()' was used twice, but with different parameters every time. The first method 'add()' takes two integers as parameters, and adds them. The second 'add()' method does almost the same thing, but this time it's dealing with two double values. Notice that the type of parameters used in both methods are different hence differentiating these two methods apart. This way, if you need to add two integers, the integer method will be invoked, and if two doubles are to be added, the double 'add()' method will be executed.
Interpreting a Complex Method Overloading Example in Java
Now that you are clear about how a simple method overloading works, let's move to a slightly complex example. This will further reinforce the concept of method overloading.class Geometry { // method to calculate area of square static double area(int side) { return Math.pow(side, 2); } // method to calculate area of rectangle static double area(int length, int breadth) { return length * breadth; } // method to calculate area of circle static double area(double radius) { return Math.PI * Math.pow(radius, 2); } }In the example you see here, the class 'Geometry' has three variations of the method named 'area'. Each of these methods calculates the area of a different geometric shape. 1. The first version of 'area', accepts one integer as an argument, indicating that it's intended to calculate the area of a square (since a square has all sides equal). 2. The second version of 'area' takes two integer parameters, which signifies that it's designed to compute the area of a rectangle (where length and breadth are different). 3. The third version of 'area' has one double parameter, indicating that it's meant for finding the area of a circle. All these methods have the same name (i.e., 'area') but their parameter lists differ, effectively making them unique. This is a perfect example of method overloading, which elegantly combines three different functionalities under the same method name, thereby boosting readability and brevity of the code. Remember that method overloading is a powerful tool available in Java that assists you in creating clean and efficient codes. By being aware of this concept, you will be able to employ more effective programming practices and take your Java mastery to the next level. And the more you practice, the more you'll understand and be able to apply these principles. Happy learning!
Delving into the Purpose of Java Method Overloading
It's crucial to comprehend the purpose of Method Overloading as an advantageous feature of Java, which was largely introduced to enhance the readability and reusability of code. As a feature of polymorphism in Java, method overloading allows you to define multiple methods with the same name but with different parameters. It eases the ability to perform a single task in several different ways with different inputs, enhancing the flexibility and readability of your code.Why is Method Overloading Important in Java Programming?
Diving into the significance of Method Overloading in Java programming, you need to realise that every design decision in a programming language serves an intended purpose, solving a wide-range of issues that arise during software development. Method Overloading in Java bears no exception to this. It stands as an integral feature in the Java programming language and here's why: 1. Code Organization and Readability: Method overloading promotes clean and manageable code where similar actions for different input parameters are encapsulated within a single class. This code structure improves readability, making it easier for you and other developers to navigate and understand the logic revolving around a specific functionality.int multiply(int a, int b) { return a * b; } double multiply(double a, double b, double c) { return a * b * c; }In the code snippet above, you can use the 'multiply' method for different purposes in the same class. This eliminates the need to memorise myriad method names performing similar operations, thereby making the code cleaner and readable. 2. Extensibility: Method overloading offers higher extensibility allowing you to add more to a method's functionality without altering existing method's signature. This means that you can add more operands to a method as per your needs, while still keeping the relevance intact. 3. Type Casting: Another key reason for employing method overloading in Java is to avoid the erroneous results that might arise from implicit type casting. For example, consider a situation where you want to multiply integer and double. In such cases, if you don’t have an overloaded multiply method taking one integer and one double as parameters, implicit casting will occur, leading to unexpected results.
Real-world applications of Java Method Overloading
No matter how useful a programming concept may seem within a theoretical context, its true utility is measured by its practical application. Here are some real-life examples showcasing the usage of Java Method Overloading: 1. GUI Development: While developing Graphical User Interface (GUI) in Java using classes like 'JButton', 'JLabel', 'JTextField', etc., method overloading is commonly applied. These classes often have a set of overloaded constructors that enable you to create instances in multiple ways, based on the data available. 2. Data Printing: The 'println()' method in the 'System.out' class is another prominent example of method overloading in Java. This method is overloaded to print several types of data, such as integer, double, float, long, enum, and String. 3. JDBC Statement Interfaces: In JDBC, Statement interfaces like CallableStatement, PreparedStatement, and ResultSet have methods that are overloaded for setting input parameters of different data types. 4. Image Processing (Java 2D API): The Graphics2D class used for image processing and graphics programming in Java provides a handful of overloaded methods. In conclusion, Java Method Overloading brings method flexibility and code readability to the table by allowing the same method to handle differing parameter counts or types. It works remarkably well in a variety of scenarios in real-world software development, from GUI creation, database querying, to even data printing and image processing. As you can see, it further solidifies Java's strengths as an efficient, resourceful, and streamlined language for a myriad of programming tasks.Java Method Overloading - Key takeaways
- Java Method Overloading enhances code readability and re-usability. It enables the use of the same method name for similar actions.
- Method overloading is determined at compile-time and hence is also known as compile-time polymorphism.
- Methods can be overloaded in the same class or in subclasses.
- Method overloading rules include manipulating the type, number, and order of arguments in method declarations. It can't be achieved by a change in return types alone.
- Method Overloading in Java and Method Overriding are fundamentally different - Method Overloading involves using the same method name but with different parameters, while Method Overriding involves providing a specific implementation to a method already provided by its superclass.
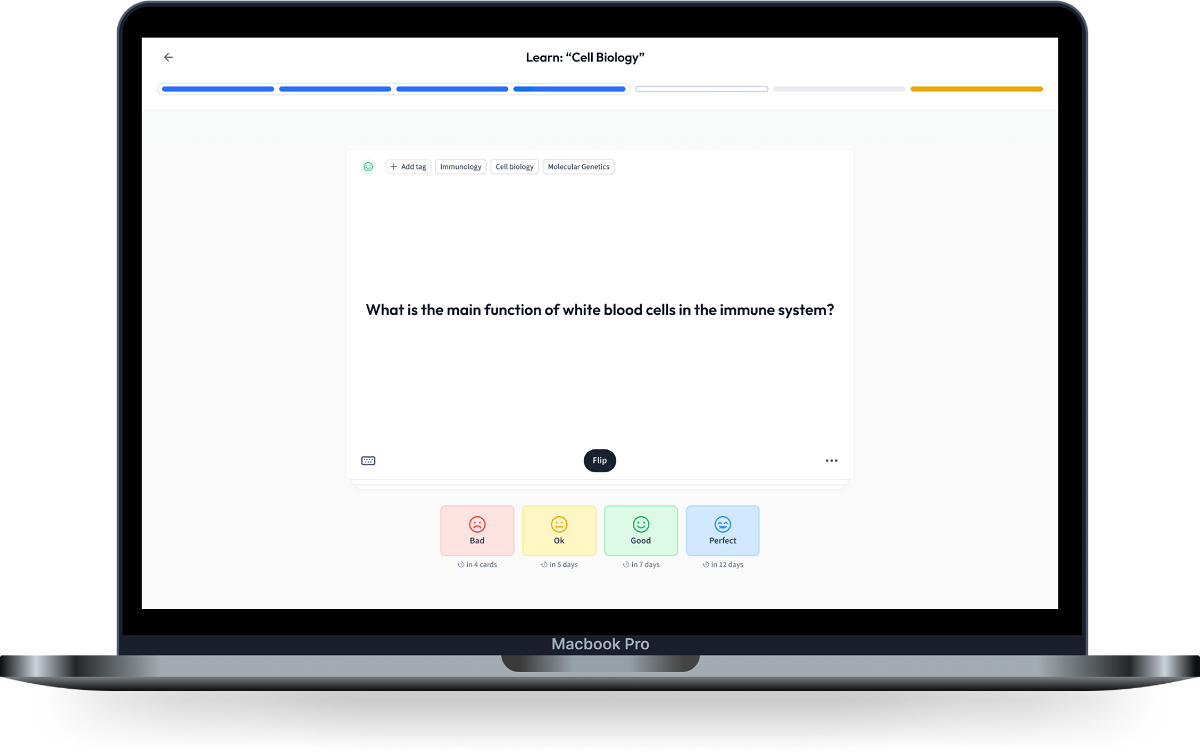
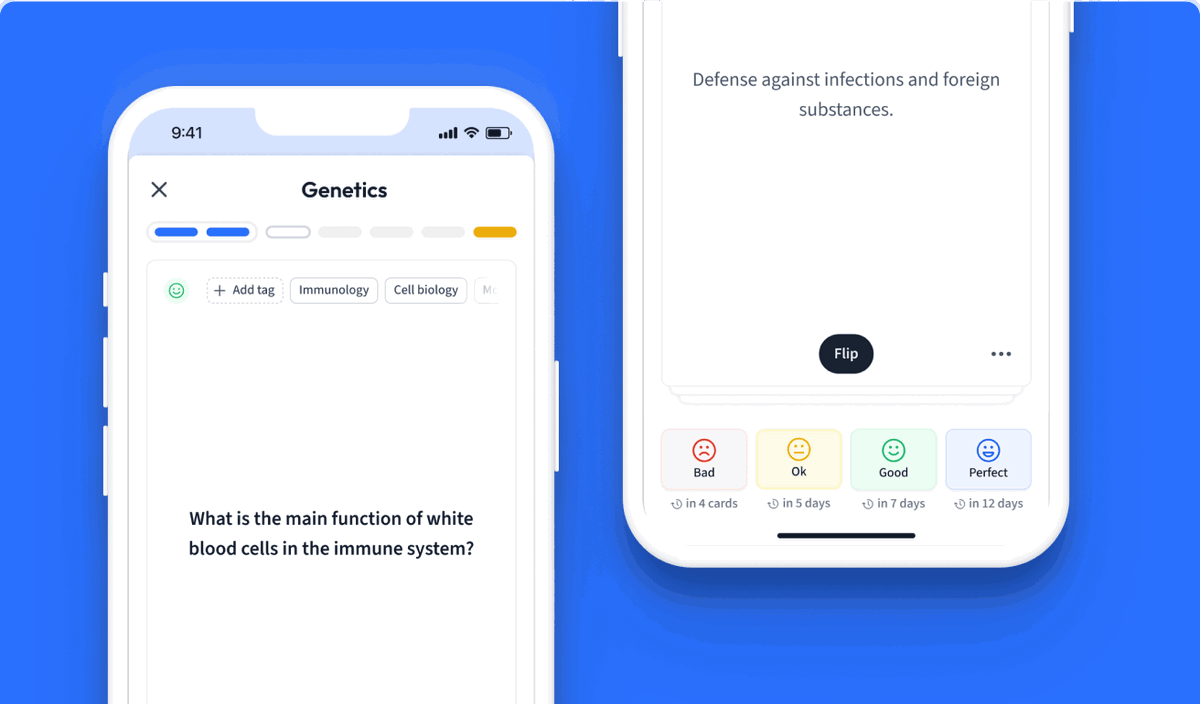
Learn with 15 Java Method Overloading flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Java Method Overloading
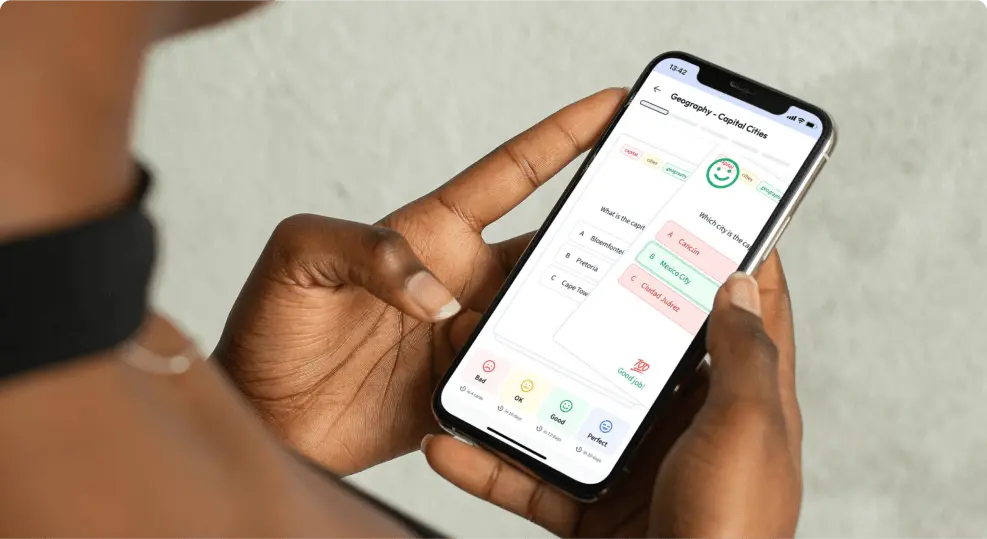
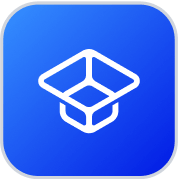
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more