Understanding Javascript Multidimensional Arrays
The world of computer programming revolves around efficient data storage and manipulation. One such potent tool for handling data in Javascript involves using multidimensional arrays. These are complex forms of Javascript's regular data storage units - the arrays.
Javascript Multidimensional Array Definition
In Javascript, a multidimensional array is essentially an array which contains one or more arrays within itself. It provides you with an easy way to store structured data in a format that is easily accessible. Each internal array has its own index, starting from 0 and increasing by 1 each time.
Key components of a Javascript multidimensional array
When you're working with a multidimensional array, there are several vital components to remember:
- Elements: The elements of a multidimensional array are actually arrays themselves. These internal arrays are what makes the parent array multidimensional.
- Indexes: Each sub-array and element inside the sub-array have their unique index position.
- Length: The length of a multidimensional array tells you the number of sub-arrays it contains.
Let's consider a 2-dimensional array:
let array = [[1, 2, 3], [4, 5, 6], [7, 8, 9]];Here, the parent array has a length of 3, as there are three sub-arrays. The first sub-array – [1, 2, 3] – is at index 0 of the parent array. The elements within this sub-array have their indexes in relation to the sub-array, not the main array.
The basis of Javascript Multidimensional Array techniques
Whether you're storing data from a spreadsheet or creating a matrix, a Javascript multidimensional array is a handy tool to use. Understanding the basic techniques for dealing with these data structures, such as accessing their elements, iterating over them, and even manipulating their structure, is essential for efficient work.
Comprehensive look at Array index concept in Javascript multidimensional arrays
One of the key components of an array in Javascript, including multidimensional arrays, is the concept of indexing. The index is a numeric value that positions an element in an array. In Javascript, the array index always starts at 0, which means the first element in an array is at the zero-ith position.
For a multidimensional array, the first level of indexing identifies the sub-array, and the second level of indexing specifies the exact element within that sub-array.
Let's revisit our 2D array example:
let array = [[1, 2, 3], [4, 5, 6], [7, 8, 9]];To access the number 5, we need to go into the sub-array at index 1 (second sub-array), and then in that sub-array, access the element at index 1 by writing
console.log(array[1][1]); // Output: 5
Remember, while Javascript has the flexibility to handle arrays of different lengths in a multidimensional array (array of arrays), it's generally a good practice to keep the lengths consistent for data manipulation and improved readability.
Exploring Practical Usage of Javascript Multidimensional Arrays
Understanding the theoretical aspect of Javascript multidimensional arrays sets the foundation. However, the true power of these data structures is unlocked when you see them in action. Practical use-cases include everything from data management to complex calculations. Let's dive deep into the application grounds of Javascript multidimensional arrays.
Javascript Multidimensional array example
Let's consider a real-world example, imagine you're developing a game and need to represent a 3x3 grid, where each cell can take on multiple properties like its current status, its colour, and the player that last interacted with it.
Here's how you might create such a grid using a multidimensional array:
let grid = [ [ {status: 'empty', colour: 'white', player: null}, {status: 'empty', colour: 'white', player: null}, {status: 'empty', colour: 'white', player: null} ], [ {status: 'empty', colour: 'white', player: null}, {status: 'empty', colour: 'white', player: null}, {status: 'empty', colour: 'white', player: null} ], [ {status: 'empty', colour: 'white', player: null}, {status: 'empty', colour: 'white', player: null}, {status: 'empty', colour: 'white', player: null} ] ];
Step-by-step explanation of a Javascript multidimensional array example
Now that we have seen the array, let's delve into understanding it:
- The variable grid is declaring an array containing three sub-arrays, each also containing three objects, mirroring the 3x3 grid of the game. This forms our 2-dimensional array.
- Each object in the sub-arrays represents a cell in the game grid, with properties like 'status', 'colour', and 'player' giving information about the cell.
- To access an individual cell of the grid, we could use double indexing like
console.log(grid[1][2]); // Output: Object {status: 'empty', color: 'white', player: null}
Javascript multidimensional array manipulation
Manipulation of Javascript multidimensional arrays is central to their usage, whether for changing values, or for carrying out operations on the entire array structure.
Let's modify our game-grid example to change the 'status' and 'player' of one of its cells:
grid[1][2].status = 'filled'; grid[1][2].player = 'Player1';
Methods and Tips for Javascript multidimensional array manipulation
There are a plethora of methods available in Javascript for multidimensional array manipulation. It's essential to utilise these for effective code processing.
The push method allows you to add one or more elements to the end of an array and return the new length of the array.
Let's extend the first sub-array in our original grid:
grid[0].push({status: 'empty', colour: 'white', player: null});Now, we have a 4 x 3 grid!
Similarly, the pop method removes the last element of an array and returns that element. It's the contrast of the push method.
While there are numerous ways to manipulate arrays in Javascript, it's important to remember to keep your data consistent. In our game-grid example, imagine the confusion if some cells were missing a 'player' field, or if some arrays had more cells than others. Mismatched data in a multidimensional array can often lead to more problems than it solves.
Unpacking The Structure of Javascript Multidimensional Arrays
Identifying the appropriate structure for storing data logically and cohesively is a crucial skill in javascript. With multidimensional arrays, the arrangement of data surpasses linear storage to provide a layered, grid-like structure, well-suited to handling complex data forms. By unpacking the structure, you'll manoeuvre the realm of Javascript multidimensional arrays with purpose and precision.
Multidimensional array structure in Javascript
In Javascript, unlike certain other programming languages, a multidimensional array is essentially an "array of arrays", where each array can separately hold its objects, be they integers, strings, or other objects. Diving into these individual arrays brings us closer to understanding the entire structure.
A 2-dimensional array, or 2D array, is an array of arrays, that gives us a 'grid' of values. It can be compared to a spreadsheet, with rows and columns. Each 'cell' in the grid holds a value. This concept extends for 3-dimensional arrays, 4-dimensional arrays, and so forth, where each additional dimension indicates an additional 'layer' to navigate into, before reaching the desired value.
While formulating the structure, it is important to know that the length of each internal array can be different, as Javascript allows for asymmetrical arrays, though this may incur additional handling difficulties.
Comparing Multidimensional array structure in Javascript and Java
Though Java and Javascript may sound similar, their handling of multidimensional arrays is quite different. In Java, multidimensional arrays are declared directly with their dimension, such as
int[][] array = new int[3][3];
In contrast, Javascript doesn't have an inbuilt syntax to declare multidimensional arrays. Instead, to create a 2-dimensional Javascript array, you have to start with an array and then make each element of that array, another array. This brings us back to the concept of Javascript multidimensional arrays as 'arrays of arrays'.
Mastering Array index concept in Javascript multidimensional arrays
An array index in a multidimensional array can be best described as coordinates that denote an element's position in the array. Understanding the indexing in multidimensional arrays is like decoding the labyrinth of values held within. The key lies in comprehending that each dimension comes with its indexing, which separates it from the other dimensions. The outermost array possesses the foremost level of indexing, and each sub-array comes with its sequence of indexing.
Consider a 2D array: each element in this array is itself an array. The outer array's index tells you which inner array you're dealing with. The index of the inner array then tells you the specific element within that array that you're referring to.
Breaking down the complexities of Array index in Javascript multidimensional arrays
Indices might not be as intuitive in multidimensional arrays as much as it is in flat arrays. Let's break it down:
For example, in a 2D array represented as:
let TwoDArray = [[1, 2, 3], [4, 5, 6], [7, 8, 9]];Here, TwoDArray[0] is [1, 2, 3], TwoDArray[1] is [4, 5, 6] and so on.
To access a specific element, two indices are required: one to specify the sub-array within the overall array, and a second to define the element's position within that sub-array.
Continuing with the example, TwoDArray[0][0] is 1, TwoDArray[0][1] is 2, and TwoDArray[1][0] is 4.
These index values aid in data manipulation, like modifying values or accessing specific data for operations. Mastering the index structure is pivotal to mastering the art of javascript multidimensional arrays.
Javascript Multidimensional Arrays - Key takeaways
- Javascript multidimensional array is an array that contains one or more arrays within itself, each with its own index. This structure provides a structured and accessible method for data storage.
- The three vital components of a Javascript multidimensional array are elements, indexes, and length. Elements refer to the arrays inside the multidimensional array. Indexes assign unique positions to each sub-array and element, while length refers to the number of sub-arrays contained in the multidimensional array.
- Array index in Javascript multidimensional arrays is a numeric value that positions an element in the array. The array index starts at 0 in Javascript. For multidimensional arrays, the first level of indexing identifies the sub-array, and the second level specifies the element within that sub-array.
- Practical applications of Javascript multidimensional arrays can be seen in data management and complex calculations. A practical example can be creating a 3x3 grid for a game, where each cell carries multiple properties and is represented in a multidimensional array.
- With Javascript multidimensional array manipulation, values can be changed or operations can be conducted on the entire array structure. Different methods like push and pop allow elements to be added or removed from these arrays, respectively.
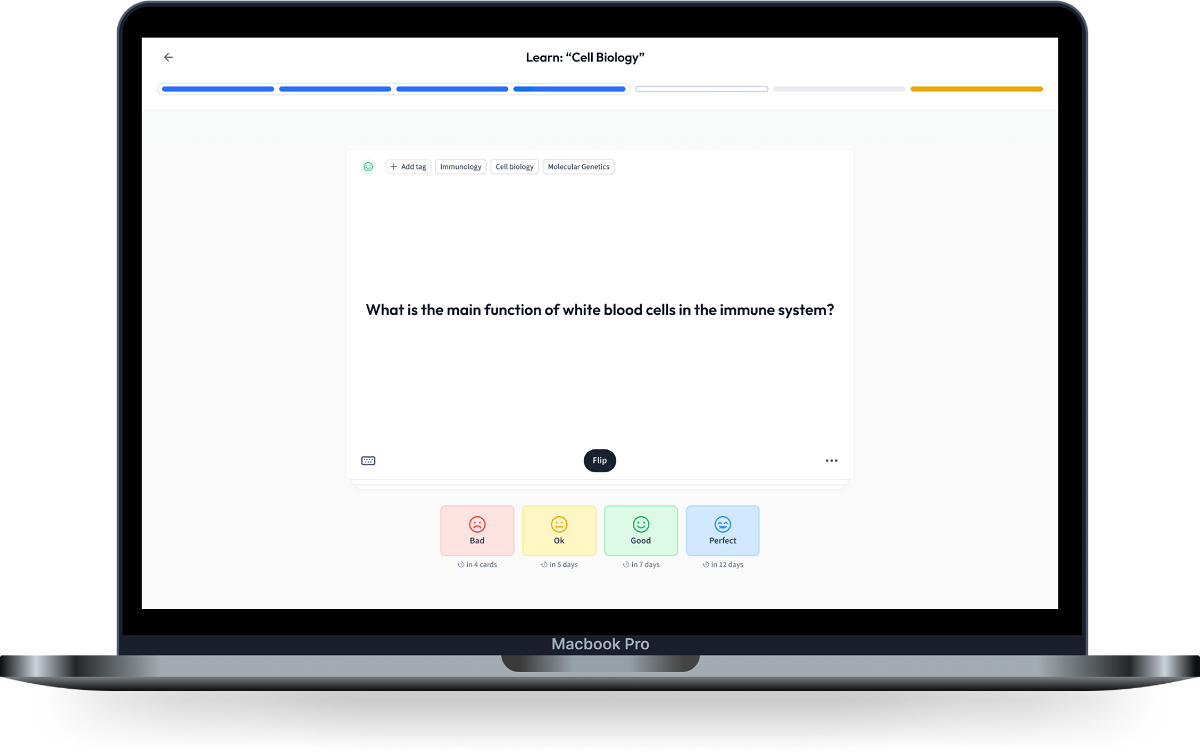
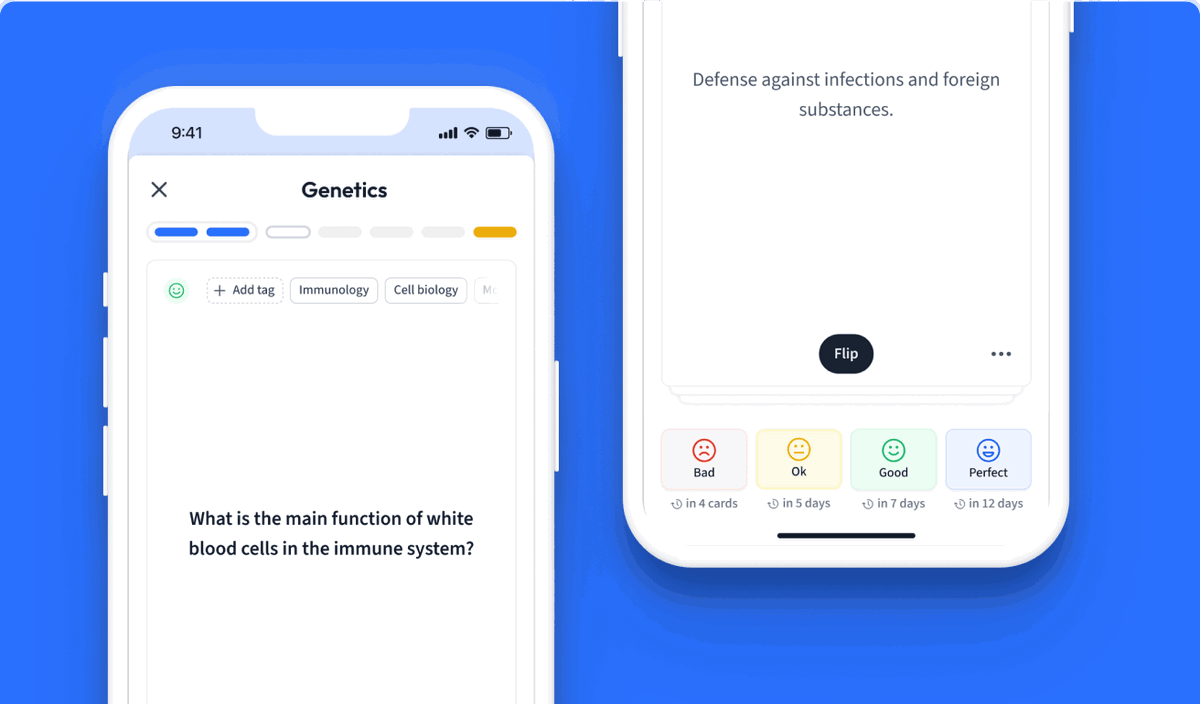
Learn with 12 Javascript Multidimensional Arrays flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Javascript Multidimensional Arrays
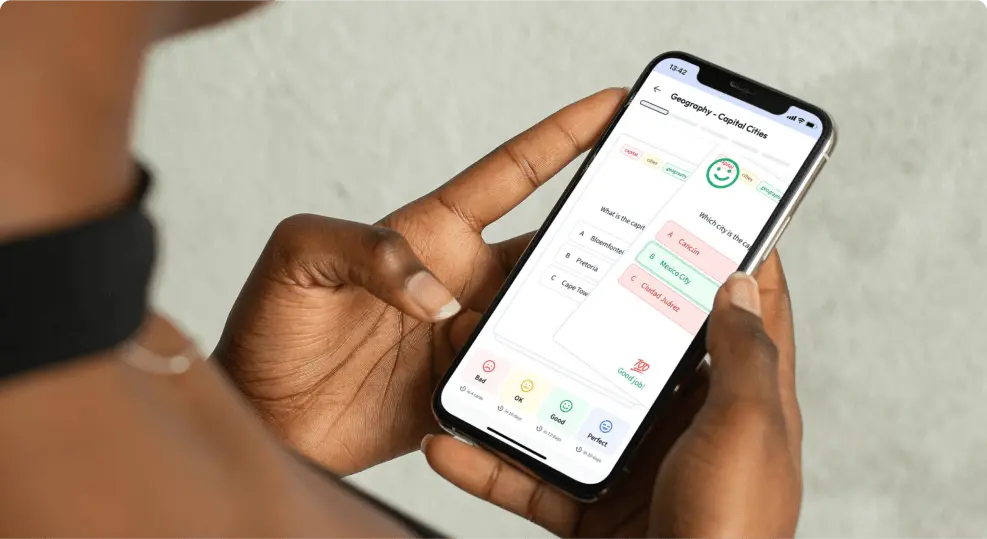
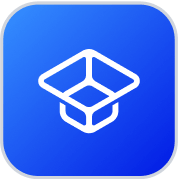
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more