Introduction to Threading in Computer Science
Threading in computer science is a complex yet incredibly fascinating concept. To truly appreciate the power of threading, it is important first to understand the basics of computer processes and how they work. A thread, in essence, constitutes a separate sequence of instructions within a computer program's process. Threading ushers in the possibility of executing multiple processes simultaneously, which is known as multithreading. This innovative capability is instrumental in implementing concurrent operations, making applications faster and more efficient.
Defining Threading in Computer Science
In computer science, threading refers to the smallest sequence of programmed instructions that can be managed independently by a scheduler. In a broader context, threads are entities within a process that can run concurrently in shared memory spaces.
For instance, consider you have a program that is designed to do two things: download a file from the internet and write a text file on your computer. Without threading, your computer would first have to finish downloading before it could start writing. But with threading, your computer can perform both these actions concurrently.
How Threading Works: An Engaging Illustration
Imagine a classroom where you are the teacher. Every student is working on a different activity, akin to a thread. Some students may be reading, others may be writing or drawing. Despite performing various tasks, each student shares the same classroom resources, and their activities can happen concurrently. This is a simplified illustration of how threading works. Take a look at the table below explaining how threads interact with different processes:Process | Thread 1 | Thread 2 | Thread 3 |
Process 1 | Reading a file | Writing to a file | Calculating data |
Process 2 | Downloading a file | Uploading a file | Rendering a video |
Coding Example: // C++ code for thread creation and joining #includeIn the above C++ code sample, a new thread is created which executes the function 'threadFunction'. After completion, the thread is joined back to the main thread denoting the end of concurrent execution. Meanwhile, the main thread continues to execute in parallel with the newly spawned thread.#include void threadFunction() { std::cout << "Welcome to concurrent world\n"; } int main() { std::thread t(threadFunction); t.join(); return 0; }
It's fascinating to know that threading is the backbone for modern high-performance computing. Solutions to computationally intensive problems in fields such as real-time graphics, artificial intelligence, and scientific computation would be inconceivable without the power of threading.
Real-World Examples of Threading in Computer Science
Threading plays a key role in the functioning of multiple sectors and domains in our digital world. From enhancing the user interface responsiveness to playing a pivotal role in high-performance computing, threading’s applicability is vast and indispensable.
Examining an Example of Threading in Computer Science
To truly grasp the power of threading, let's delve into the intricacies of a real-world example related to online banking. Online banking systems handle millions of concurrent users who are performing numerous operations such as fund transfers, balance checks, bill payments, and more. How is this managed smoothly? The answer lies in threading.
In the context of an online banking system, each user session can be considered a separate thread. All these threads are handled independently within the broader process, making it possible for millions of transactions to take place concurrently without any interference.
Let's have a closer look at how this unfolds:
- An online banking system has to be constantly live and responsive. Any delay can be catastrophic to operate. Imagine a scenario where a user starts a transaction but on the server-side, the software waits for another user's transaction to finish before catering to the new one. This could lead to significant delays, something a banking system cannot bear. Here, threading allows multiple user transactions to be processed concurrently, making banking operations rapid and efficient.
- In such scenarios, each transaction initiated by a user is treated as a separate thread. This ensures real-time processing, reducing delays and enhancing user experience.
In essence, threading is the silent engine that powers the seamless operations one observes in an online banking system.
How Threading is Applied in Everyday Computer Operations
Threading's role isn't confined to high-scale applications like banking systems. It is integral to everyday computer operations too. From the seamless functionality of operating systems to the smooth performance of web browsers and word processors, threading is everywhere.
Operating Systems, for example, make extensive use of threading. Microsoft Windows, Linux, and MacOS all use threading to manage multiple applications concurrently. This allows you to surf the web, listen to music, download a file, and have a word processor open, all at the same time.
Let's consider another everyday example: web browsers. When you open multiple tabs in a browser, each tab is typically handled by a separate thread. This means you can load multiple web pages concurrently, enjoy an uninterrupted Youtube video on one tab while a heavy web application loads on another.
Another real-life application is seen in word processors. A spell check feature in a word processor, for example, runs on a separate thread. You can continue typing your document while the spell check function concurrently highlights any misspelled words, without causing any disturbance to your typing.
These examples serve to highlight how threading, while not directly visible to the end user, remains an inherent part of modern computing, making it more efficient and dynamic.
Different Types of Threading in Computer Science
Threading in computer science opens up a world of parallel execution and concurrent processing, but not all threads are the same. Different types of threads exist, each lending itself to distinct use cases. Broadly speaking, the three primary types of threads are: User Threads, Kernel Threads, and Hybrid Threading. Understanding these is fundamental to the comprehensive knowledge of threading in computer science.
Exploring the 3 Kinds of Threads in Computer Science
Let's delve into these three types of threads in order to gain a deeper understanding of threading in computer science.
User Threads
User threads, as the name implies, are threads managed entirely by userspace libraries. They have no direct interaction with the kernel and are managed outside the operating system. They are faster to create and manage as they don’t need to interact with the system kernel. Some common user level thread libraries include POSIX Pthreads and Microsoft Windows Fibers.
A user thread is one that the operating system kernel isn't aware of, and thus, couldn't manage or schedule directly.
Kernel Threads
Kernel threads, on the other hand, are managed directly by the operating system, providing benefits such as support for multi-processor systems and system-wide scheduling. However, these benefits come at the cost of slower performance due to the overhead of context-switching between kernel and user mode.
A kernel thread is one that is directly managed and scheduled by the kernel itself, giving the operating system more control over their execution and scheduling.
Hybrid Threading
Recognising the different trade-offs between user and kernel threads, some systems employ a hybrid model, where multiple user threads are mapped onto a smaller or equal number of kernel threads. This allows programmers to create as many user threads as needed without the overhead of creating the same number of kernel threads, while still gaining the advantages of kernel level scheduling.
Hybrid threading mixes features from both user level threads and kernel level threads, providing a balanced solution to leverage the advantages of both types.
Comparing and Contrasting Different Threads
Although the three types of threads share some similarities, their features, benefits and drawbacks differ greatly. An understanding of these distinctions is critical for efficient and effective application of threads in computer science.
Comparisons of different thread types are best demonstrated via a tabular representation:
Type | Speed | Scheduling | Control | Overhead |
User Threads | High | User-level | User | Low |
Kernel Threads | Lower | Kernel-level | Kernel | High |
Hybrid Threads | Moderate | Both | Both | Moderate |
User threads are the fastest, but their scheduling isn't controlled by the kernel, which makes it difficult for the system to take global decisions about process scheduling. Conversely, kernel threads have kernel-level scheduling, so they can be managed more efficiently by the operating system, but they also take longer to create and destroy due to kernel overhead.
Lastly, Hybrid Threading models seek to strike a balance by mapping many user-level threads onto an equal or smaller number of kernel threads. This offers more flexibility than pure User or Kernel threading, resulting in efficient management and lower overheads.
Understanding the Role of Starvation in Computer Science Threads
Starvation in computer science is a real challenge that can hamper the efficacy of computer programs and systems. While it is an innate part of the world of threading, starvation is often perceived negatively because it results in unfair allocation of processing time among different threads, thus affecting the performance and execution speed of programs.
Defining Starvation in Context of Computer Science Threads
Starvation is a scenario in multi-threading environments where a thread is constantly denied the necessary resources to process its workload. Specifically, if a thread doesn't get enough CPU time to proceed with its tasks while other threads continue their execution unhindered, this thread is said to be experiencing starvation.
Starvation happens when a thread in a computer program or system goes indefinitely without receiving necessary resources, leading to delays in execution or a complete halt.
Management of resources among multiple threads weaving in and out of execution is a complex process. Scheduling algorithms determine the sequence of thread execution, and these can sometimes lead to a scenario where a thread becomes a low priority and is denied necessary resources. This usually happens when some threads take up more resources or CPU time than others, leaving less space for the remaining threads.
Algorithmically speaking (though it is simplified), starvation is akin to the below condition, where a thread \( t \) is not allocated CPU time over a given period \( p \): \[ \int_{0}^{p} CPU(t) \, dt = 0 \]
In essence, the role of starvation in threading is a balancing act that maintains the ebb and flow of thread execution in multi-threading environments. However, it is usually a situation to be mitigated or avoided, as it can lead to inefficiencies and delays in task completion.
Starvation in Threads: Causes and Consequences
Identifying causes and understanding consequences is critical in addressing and resolving any issue, and thread starvation is no exception. Since starvation pertains to the unfair or inadequate allocation of resources to threads, its causes are usually rooted in flaws or biases in the process scheduling algorithm.
Scheduling algorithms are designed to prioritise certain threads, based on various properties such as process size, priority level or time of arrival in the queue. Sometimes, high-priority threads can dominate resources, leaving low-priority threads languishing without receiving the necessary CPU time—a typical cause of starvation.
Another common cause of thread starvation is related to thread priority. Certain streaming or gaming applications, for example, may be coded to take priority, leaving other applications with fewer resources.
Often, mutual exclusion could lead to thread starvation. If two threads require the same resource and one gets access to it for an extended period, the other will starve indefinitely until the resource becomes available again.
Now, what are the consequences of thread starvation? This doesn't merely slow down individual threads; it often leads to significant performance degradation of the entire process or system. A thread undergoing starvation can delay dependent threads and processes, leading to a ripple effect of reduced performance. For example, a web server could start performing poorly if critical threads handling client requests undergo starvation.
Moreover, starvation could lead to complete process termination in severe cases. This could occur when a thread doesn't get the necessary resources to reach a certain system requirement or fails to meet a timing constraint—an extreme case being program failure.
Conclusively, starvation could wreak havoc on thread execution and program performance if not identified and handled promptly. Therefore, it is crucial to anticipate the possibility of starvation during thread handling and include preventive or mitigating measures in the programming or system design phase.
Practical Aspects of Threading in Computer Science
In computer science, threads are not just theoretical concepts. They're vital components that underpin many aspects of practical software development. The optimal usage of threads can significantly improve the efficiency of programs, while improper use can lead to performance degradation or even failure. The practical aspects of threading include managing starvation and implementing effective threading techniques. These are critical for writing efficient and robust software applications.
Mitigating Starvation in Computer Science Threads
In threading, starvation is a critical issue that can lead to impaired performance or even failure of applications. However, it is also a preventable one, and with the right techniques, its negative effects can be largely mitigated.
One effective solution to counteract starvation is careful design and implementation of scheduling algorithms. Going beyond simple priority-based scheduling, algorithms such as Round Robin or the Shortest Job First algorithm can prevent starvation by ensuring fair distribution of CPU time amongst threads. In Round Robin scheduling, each thread is given an equal slice or 'quantum' of CPU time. The Shortest Job First algorithm, on the other hand, gives preference to threads with smaller processing demands.
Consider using priority aging, a technique that progressively increases the priority of waiting threads, ensuring that no thread waits indefinitely. Another way is to implement feedback mechanisms in scheduling algorithms where starving threads are gradually elevated in priority.
Let’s look at a piece of sample code that can help illustrate the concept of starvation:
Thread highPriority = new Thread(() -> { while (true) { count++; } }); highPriority.setPriority(Thread.MAX_PRIORITY); Thread lowPriority = new Thread(() -> { while (true) { count++; } }); lowPriority.setPriority(Thread.MIN_PRIORITY); lowPriority.start(); highPriority.start();
In the code snippet above, two threads are started: one with low priority and the other with high priority. Since these two threads are competing for the same resource (CPU), the thread with higher priority will consume most of the CPU time, while the low priority thread will eventually starve, and the system will suffer from performance degradation. Mitigation strategies like re-adjusting the priority levels or re-configuring the scheduler can help handle such situations in a better way.
Effective Threading Techniques for Better Performance
The effective use of threads can significantly enhance the performance of your programs. The following advanced techniques and methodologies can help you optimise your use of threads.
First, always consider the problem of Thread Overhead. Modern operating systems and programming environments improve thread performance, but there is still a cost associated with thread creation, context-switching, and termination. It's more prudent to have a fixed set of worker threads to handle tasks, rather than continuously ending and creating new threads, as in a Thread Pool model.
To illustrate, let's consider two different threading solutions to handling multiple incoming network requests:
// Initial Approach for (int i = 0; i < requests.size(); i++) { new Thread(new NetworkRequestHandler(requests.get(i))).start(); } // Thread Pool Approach ExecutorService executor = Executors.newFixedThreadPool(10); for (Request request : requests) { executor.execute(new NetworkRequestHandler(request)); }
In the initial approach, a new thread is created for each request, leading to substantial overhead due to continuous thread creation and termination. The Thread Pool approach, however, reuses a set of threads to process incoming requests, thereby reducing overhead and improving overall system performance.
Furthermore, use synchronization judiciously. Overusing synchronization constructs (like locks or mutexes) can lead to thread contention, where multiple threads are waiting for a shared resource, potentially leading to Deadlocks or Starvation.
Finally, try to take advantage of thread-local storage, a method which provides separate storage for variables for each thread. While this might slightly increase memory usage, it can drastically reduce the need for synchronization and mitigate problems like contention or race conditions.
Consider the below code saving the user session in a web server context:
// Before Using Thread Local public class UserContext { private static Session session; public static void setSession(Session s) { session = s; } public static Session getSession() { return session; } } // After Using Thread Local public class UserContext { private static ThreadLocaluserSession = new ThreadLocal<>(); public static void setSession(Session s) { userSession.set(s); } public static Session getSession() { return userSession.get(); } }
In the initial approach, there's only one session for all threads, leading to possible overwriting when multiple threads try to access the session. In contrast, the ThreadLocal-based approach provides each thread with its own separate version of the session, effectively removing the need for synchronization.
Conclusively, threading can greatly enhance or impair your programs' performance, depending on how effectively you use it. It's therefore crucial to understand and use threading techniques to write efficient, robust, and scalable software applications.
Threading In Computer Science - Key takeaways
- Threading in Computer Science enables simultaneous concurrent operations within a single process, enhancing performance and efficiency across various applications, including online banking systems, Operating Systems, web browsers, and word processors. When applied correctly, it increases application responsiveness and speed.
- An example of Threading in Computer Science is in online banking systems where each user session is treated as a separate thread, enabling the handling of millions of transactions concurrently without any interference.
- There are 3 main types of threads in Computer Science:
- User Threads: Managed entirely by userspace libraries with no direct interaction with the kernel. They are faster to create and manage but lack system-level control.
- Kernel Threads: Directly managed by the operating system, these threads offer efficient system-wide scheduling but have slower performance due to the overhead of interaction between user and kernel modes.
- Hybrid Threads: As the name suggests, this type of threads combines the features of User and Kernel threads, providing a balanced solution that minimises overheads while offering the benefits of both types.
- Starvation in Computer Science threads refers to a condition where a thread is perpetually denied of necessary resources to process its work resulting in processing delays or even halt. This occurs often when scheduling algorithms prioritise certain threads over others.
- To mitigate Starvation in threads, methods such as effective design of scheduling algorithms including Round Robin or Shortest Job First can be employed. Alternately, the use of priority ageing and the introduction of feedback mechanisms in scheduling algorithms can also help address this prevalent issue.
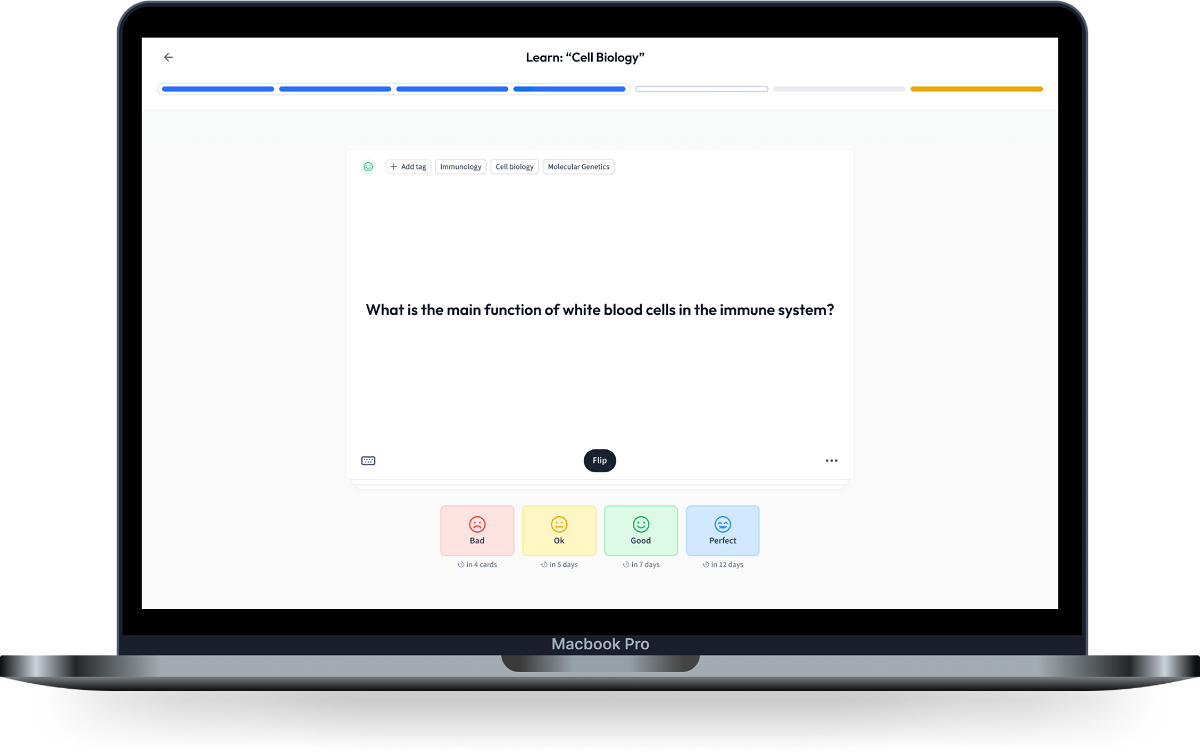
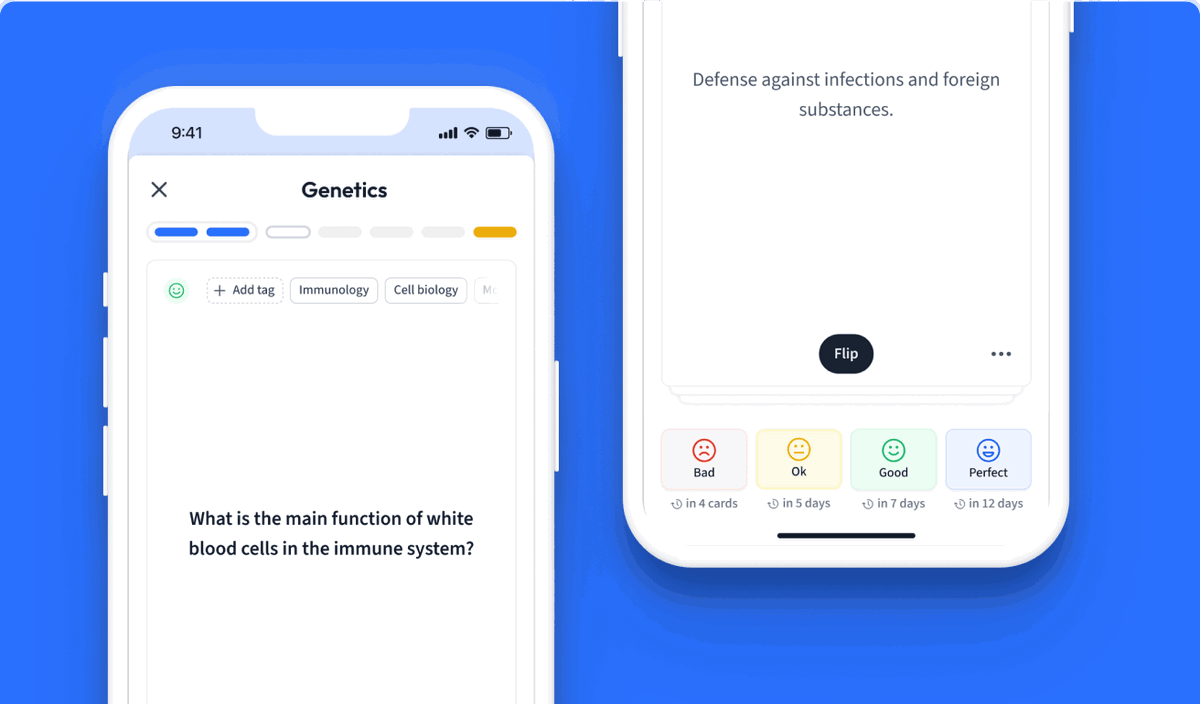
Learn with 15 Threading In Computer Science flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Threading In Computer Science
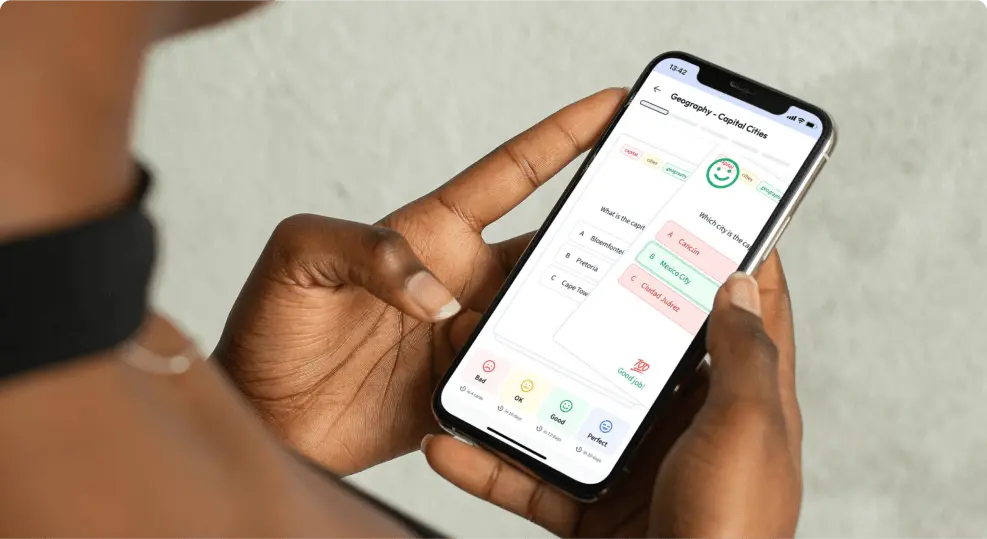
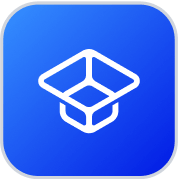
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more