Understanding the Javascript If Statement
Taking a journey into the world of computer science, you'll often find yourself entangled with some essential control structures. One such indispensable component is the Javascript If Statement. Knowing how to use an If Statement can take your coding skills to a higher level, making this subject a valuable tool in your programming arsenal.
Definition of Javascript If Statement
A Javascript If Statement is a fundamental control structure used to create conditions in your code. This statement tests a specified condition and then performs different actions based on whether the condition is true (passes the test) or false (fails the test).
Did you know that the name Javascript was inspired by Java due to its marketing potential? However, despite their names, these two programming languages are vastly different and shouldn't be confused.
Basic structure and usage of Javascript If Statement
- Structure: The general syntax of a Javascript If Statement is: if (condition) {do this} else {do something else}
- Condition: The condition is written inside parentheses and can be any expression that returns a boolean value — true or false.
- Actions: The actions to be performed are written inside curly braces and can be any valid Javascript statements.
Here is an example:
if (temperature > 30) { alert("It's hot outside."); } else { alert("It's not hot outside."); }
In this example, the If Statement checks if the variable temperature is greater than 30. If it is (the condition is true), it executes the action inside the first curly braces and shows an alert saying "It's hot outside". If it's not (the condition is false), it executes the action inside the else curly braces and shows an alert saying "It's not hot outside".
Using the Javascript If Statement with operators
You can make your If Statement conditions even more powerful by using comparison and logical operators. Examples of comparison operators include less than (<), greater than (>), equals (==), and not equals (!=). Examples of logical operators include and (&&), or (||), and not (!).
Here's an example of using an If Statement with operators:
if (age > 18 && citizen == true) { alert("You are eligible to vote."); } else { alert("You are not eligible to vote."); }
In this example, the If Statement checks if two conditions are true: if the variable age is greater than 18 and if the variable citizen is true. If both conditions are true, it shows an alert saying "You are eligible to vote". If either of the conditions is false, it shows an alert saying "You are not eligible to vote".
Common mistakes when using the Javascript If Statement
As you learn to work with If Statements in Javascript, there are a few common mistakes you may encounter.
A common mistake is forgetting to enclose the condition within parentheses. Another mistake is forgetting to use curly braces {} around the actions to be performed. Yet another mistake is confusing the assignment operator (=) with the comparison operator (==).
Here's an example of a common mistake and how to correct it:
// Common mistake: if temperature > 30 alert("It's hot outside.") // Correct code: if (temperature > 30) { alert("It's hot outside."); }
Incorporating these insights, feel prepared to tackle Javascript If Statements more confidently and accurately, optimising your coding efficiency.
How to Write an If Statement in Javascript
Writing an If Statement in Javascript is a vital skill to acquire when diving into the depths of this programming language. Being a crucial part of control structures, the If Statement is used to manage and dictate the flow of your program based on certain conditions. Grasping a good understanding of its syntax and functionality can allow you to craft intricate and intelligent scripts. Let's delve deeper into how to write an If Statement correctly in Javascript.
Step by Step Guide to Write an If Statement in Javascript
The basic syntax for writing an If Statement in Javascript follows this structure:
if (condition) { statement(s) to be executed if condition is true } else { statement(s) to be executed if condition is false }
As depicted, the If Statement in Javascript begins with the keyword 'if' followed by a condition encapsulated by parentheses. These conditions are usually comparisons, but any expression that evaluates to true or false can be used. If the condition is found to be truthful, the code block within the first curly braces is executed. If the condition doesn't hold, the code within the 'else' part is carried out, provided that an 'else' clause has been defined. Let's outline this process step by step, in an understandable and practical manner.
- Define the condition within brackets: The condition within the if clause should resolve to a boolean value, i.e., either true or false. A common use case is comparing two values using comparative operators like ==, <, >, !=, etc.
- Write the code to be executed if the condition is true within the {} braces. This could be one statement or multiple ones. Make sure every statement inside the braces ends with a semicolon (;).
- If you want to perform some action when the condition is not met (i.e., it is false), you can use the optional 'else' clause. After the 'if' clause, write 'else' and then write the statement(s) to be executed if the condition is false, again within {} braces.
An example for better understanding:
let x = 5; let y = 10; if (x > y) { console.log("x is greater"); } else { console.log("y is greater"); }
In this example, the If Statement checks if the variable x is greater than y. Since x (5) is not greater than y (10), the console logs "y is greater".
Expert Tips When Writing an If Statement in Javascript
As you further familiarise yourself with writing If Statements in Javascript, here are some expert tips which are bound to be useful.
- It's best to always use the triple equal sign (===) for comparison and not the double equal sign (==) in your condition. This is because === not only checks the values but also the type of the variables being compared.
- If comparing more than two conditions, use else if to chain multiple conditions, instead of using multiple if else statements to improve readability and performance. This also prevents unnecessary checks from being executed.
- Remember to use parentheses () for your condition and always use curly braces {} for your if and else blocks, even if there’s only one statement. This helps keep your code clean and easy to understand.
An example might look like this:
let fruit = "apple"; if (fruit === "banana") { console.log("Banana is good for digestion."); } else if (fruit === "apple"){ console.log("Apple keeps the doctor away."); } else { console.log("There are lots of fruits to choose from."); }
Common Pitfalls to Avoid While Writing an If Statement
When writing If Statements in Javascript, there are a few common pitfalls to keep in mind.
- Avoid forgetting to use round brackets for conditions, curly brackets for statements inside the block, and semicolon after every statement. These lead to syntax errors.
- Make sure you're not using the assignment operator = when you mean to use the equality operator == or ===. The former assigns a value while the latter two compare values.
- Be cautious when dealing with conditions that may produce a truthy or falsy value, even when they are not explicitly true or false. For example, an empty string, NaN, null, 0, and undefined are all falsy, while any non-zero number, non-empty string and other objects are truthy in Javascript.
An incorrect example might look like this:
let pie = "blueberry"; if pie = "apple" { console.log("Apple pie is delicious"); }
In this example, the assignment operator = is used incorrectly in place of the comparison operator == . A Javascript error will be thrown here. The correct code will be
let pie = "blueberry"; if (pie == "apple") { console.log("Apple pie is delicious"); }
Avoiding these pitfalls will ensure smoother operations and cleaner, more readable code.
Dive into Nested If Statements in Javascript
Nested If Statements in Javascript add a new layer of complexity and flexibility to your conditional programming. They provide you with the ability to test numerous conditions and handle them effectively, making your program more dynamic. When a condition needs to be checked within another condition, a 'Nested If Statement' is the ideal solution. Let's explore in depth.
An introduction to Nested If Statements in Javascript
Nested If Statements, as the term suggests, involve using If Statements within other If Statements. They come in handy when you need to test multiple conditions that are dependent on each other. In simpler terms, the execution of one If Statement is contingent on the outcome of another.
A Nested If in Javascript is an If Statement residing inside another If Statement's 'true' code block. If the outer condition meets the specified criteria, it triggers the nested If Statement. The nested If Statement then evaluates its condition and executes its code block if the secondary condition is satisfied.
The Syntax for a Nested If Statement in Javascript is as follows:
if (outerCondition) { if (innerCondition) { statement to be executed if both conditions are true } }
Keep in mind that, much like regular If Statements, each nested If Statement can also have an optional 'else' clause. This 'else' clause contains the code that runs when the associated condition is false.
Here is an example:
if (weather === "sunny") { if (temperature > 30) { console.log("It's a hot sunny day"); } else { console.log("Enjoy the sunny day"); } } else { console.log("Looks like it's not sunny today"); }
This example features a Nested If Statement used to check the details of the weather. The Outer If Statement assesses whether it's a sunny day or not. If it is, the Nested If Statement then determines whether the temperature is greater than 30 degrees or less. Each condition directs a different console message.
Real-world Nested If Statements exercises in Javascript
JavaScript Nested If Statements are widely used in real-world programming to deal with complex decision-making scenarios. From input validation to complex algorithms, they're instrumental in numerous use cases. Let's roll up our sleeves and look at some practical exercises.
Imagine you are developing a rewards system for a game. The system rewards users based on the number of hours they play and extra bonuses they acquire. You could set up a Nested If Statement to handle this scenario:
if (hoursPlayed > 10) { if (bonus > 5) { console.log("You earned a Gold Medal!"); } else { console.log("You earned a Silver Medal!"); } } else { console.log("You need to spend more time playing to earn medals"); }
This code determines the type of medal a player receives based on the number of hours they've played and the bonuses they've acquired. If the player has played for more than 10 hours and gathered more than five bonuses, they receive a gold medal. Players who played for over 10 hours but gathered five or fewer bonuses receive a silver medal.
Now consider a more intricate case - a tax calculation engine. It is not as simple as the previous examples but a good illustration of how nested Javascript If Statements are able to handle complicated real-world scenarios. In this case, the taxable amount depends on an individual's income and age.
if (income > 50000) { if (age > 60) { tax = income * 0.1; } else { tax = income * 0.2; } } else { tax = income * 0.05; }
This example calculates the tax payment for an individual. If the individual's income is greater than 50,000, another condition checks their age. If they are over 60, the tax rate is 10%, else it's 20%. If the individual's income is less than or equal to 50,000, the tax rate is 5%, regardless of age.
These examples provide a view of the enduring role Nested If Statements play in Javascript, illustrating their importance in handling complex conditions.
The Javascript If and Statement
The If and Statement in Javascript represents a potent manipulation of the conventional If Statement. It introduces a way to check multiple conditions simultaneously, thereby escalating the complexity and capabilities of your code. This potent tool employs the usage of Javascript's logical AND operator, denoted by '&&'.
Understanding the Javascript If and Statement and its applications
Javascript's If and Statement employs the logical AND operator in managing numerous conditions. An expression including the '&&' operator will return true or 'truthy' only when all the conditions are met. In cases where any condition fails, the entire expression defaults to false or 'falsy'. This means that when using the If and Statement, every single condition you specify must be true for your code block to execute. If any condition isn't met, Javascript skips your code block and moves to the next section of your script.
The structure of an If and Statement in Javascript generally resembles the following:
if (condition1 && condition2 && condition3) { // Code to be executed if all conditions are truthful }
For instance, if you want to print "Welcome, User!" only when both the username and password entered by the user are correct, you can use an If and Statement.
Importantly, the AND operator (&&) acts as a short-circuit operator. It means, if the first condition is false, Javascript won't bother to check the remaining conditions because the entire statement can't be true if the first condition is false. This feature can be an advantage for performance, especially when your conditions involve complex or time-consuming computations.
It is also often used in combination with the 'else' statement for alternative courses of action in case a condition fails to be met. In general, the If and Statement is useful when you need to ensure multiple conditions or circumstances are in place before the execution of specific sections of your code.
Detailed Examples of Javascript If and Statement in action
Let's delve into some specific ways the If and Statement can be employed in Javascript, using detailed examples to illustrate its function and impact on your programming.
Consider a basic If and Statement which combines two conditions. Suppose you own a blog, and you want to display a certain message only to logged in users who are also members of your site. The code might look something like this:
if (loggedIn && isMember) { console.log("Welcome, dear member!"); }
In this example, the message "Welcome, dear member!" will only be printed to the console if both conditions, 'loggedIn' and 'isMember', are true. If either of these conditions isn't met, the message won't be printed.
Now, imagine a more complex scenario. Assume you wish to implement a fitness tracker application that alerts the user to hydrate only if the user has exercised for over an hour, and the current temperature is above 25 degrees Celsius.
The Javascript If and Statement for this case might look like this:
if (exerciseHours > 1 && currentTemperature > 25) { alert("You've been exercising for over an hour and the temperature is above 25C. Time to hydrate!"); }
In this situation, the user sees the hydration alert only when both conditions - 'exerciseHours > 1 ' and 'currentTemperature > 25' - are met. If either condition isn't satisfied, no alert is issued to the user.
In both examples, the If and Statement enhanced the functionality of the programs to deliver an experience better tailored to the user's specific conditions. Whether you wish to personalise experiences or enforce precise conditions before triggering a command, harnessing the power of the If and Statement will accommodate more intricate projects.
Exploring Javascript If Statement Examples
Dabbling with examples is paramount in mastering Javascript If Statement. Whether you're learning to program or honing your Javascript prowess, dissecting various examples is incredibly beneficial. By delving into If Statement examples, you magnify your understanding of Javascript's condition flow control, thereby enhancing your ability to undertake more complex programming tasks.
Common Javascript If Statement Scenarios
Get ready to dive into If Statement, one of the key building blocks in Javascript. Embark on an exciting journey to explore some every day coding scenarios in which an If Statement plays a starring role.
Often, a Javascript If Statement uses comparison or 'relational' operators. Relational operators compare values and return true or false according to the outcome. The operators include:
- Equals to (==)
- Not equal to (!=)
- Greater than (>)
- Less than (<)
- Greater than or equal to (>=)
- Less than or equal to (<=)
If Statements typically follow this syntax:
if (condition) { code to be executed if condition is true }
Take for instance the following case:
var score = 85; if (score >= 60) { console.log("You passed the exam"); }
In this instance, if the variable 'score' is greater than or equal to 60, the message "You passed the exam" will be displayed in the console.
In more complex scenarios, 'else' and 'else if' statements are paired with If Statements. The 'else' statement encompasses the code to be executed when the condition in the If Statement is false, while 'else if' introduces a new condition to be tested if the first condition is false.
Check out this elucidation:
var temperature = 20; if (temperature > 30) { console.log("It's hot outside"); } else if (temperature < 15) { console.log("It's cold outside"); } else { console.log("The weather is mild"); }
Depending on the value of 'temperature', a different message is printed in the console: "It's hot outside" for temperatures above 30; "It's cold outside" for temperatures below 15 and "The weather is mild" for temperatures between 15 and 30.
Complex Javascript If Statement Situations
Javascript If Statement can also deal with much more complicated scenarios that involve multiple conditions and complex logical operations. When dealing with such conditions, you can utilise logical operators like AND (&&) and OR (||). They can help to check multiple conditions in a very effective and efficient way.
Examine this:
var age = 20; var hasDrivingLicense = true; if (age >= 18 && hasDrivingLicense) { console.log("You can drive a car"); } else { console.log("You are not eligible to drive a car"); }
In this code, the message "You can drive a car" is only displayed if both the 'age' is equal to or greater than 18, and 'hasDrivingLicense' is true. This best exemplifies how the AND (&&) operator can be used within an If Statement to check multiple conditions.
In scenarios where you desire one of multiple conditions to hold true, the OR (||) operator is applied. OR operator will return true if at least one of the conditions is true. See the next example:
Here's how it works:
var vegetarian = true; var likesSalad = false; if (vegetarian || likesSalad) { console.log("We recommend our Garden Salad"); } else { console.log("You might prefer our Steak"); }
In this code snippet, the message "We recommend our Garden Salad" will be displayed if at least one of 'vegetarian' or 'likesSalad' is true. If both are false, you will see "You might prefer our Steak" in the console.
Also, Nested If Statements might be applied in more complex scenarios where conditions are based on the results of other conditions. These If Statements sit within other If Statements, and provide further control over how your code runs.
For instance:
var age = 20; var hasTicket = true; if (age >= 18) { if (hasTicket) { console.log("Enjoy the movie!"); } else { console.log("Please buy a ticket first"); } } else { console.log("You're underage for this movie"); }
This case comprises a Nested If Statement. If the 'age' is equal to or greater than 18, Javascript then checks if 'hasTicket' is true. If so, the message "Enjoy the movie" is displayed. If 'hasTicket' is false, "Please buy a ticket first" is shown in the console. If the 'age' is less than 18, the console displays "You're underage for this movie".
Decoding complex Javascript If Statement situations enables you to explore multiple coding scenarios and exhibit creativity in solving problems. The more familiar you become with these applications, the better equipped you'll be at solving intricate coding problems.
Characteristics of If Statement in Javascript
In Javascript, the If Statement is a fundamental conditional statement employed for decision making based on set conditions. This powerful tool impacts the flow of code execution, escalating your script's complexity and functionality. Let's uncover its unique characteristics and how they shape your Javascript programming experience.
Highlighting the unique properties of Javascript If Statements
An If Statement in Javascript is used to execute a block of code, only if a specified condition is fulfilled. The If Statement sets the stage for how instructions run, and its properties are essential in understanding its application. Consider these unique attributes:
1. Conditional Execution: An If Statement controls whether a block of code will execute. If the condition within the statement holds true, then the attached block of code will run. However, if the condition is false, Javascript bypasses the code block and carries on to the next part of the script.
For instance, consider a program that wants to check whether a number is positive:
let number = 5; if (number > 0) { console.log('The number is positive'); }
This block of code will only run if 'number' is greater than \(0\). If 'number' is not greater than \(0\), the console.log command is not executed.
2. Use with Logical and Comparison Operators: If Statements in Javascript commonly use logical and comparison operators. For example, '==' tests for equality, '&&' tests for logical AND, '||' tests for logical OR, etc. These operators help formulate the condition for the If Statement, allowing you to compare values or test multiple conditions.
let userName = 'John'; let userRole = 'Admin'; if (userName == 'John' && userRole == 'Admin') { console.log('Welcome, Admin John'); }
This example demonstrates how logical 'AND' ('&&') and comparison operator '==' are often used in an If Statement. The code specified in the If block is only executed when both conditions, 'userName' equal to 'John' and 'userRole' equal to 'Admin', are true.
3. Pairing with Else or Else If: If Statements can be paired with 'else' or 'else if' statements, which provide alternative flows of execution when the initial If Statement's condition is not met. This forms an 'if-else' or 'if-else if' statement.
let age = 16; if (age >= 18) { console.log('Eligible to vote'); } else { console.log('Not eligible to vote'); }
In this case, the 'else' statement acts as an alternative to the If Statement. If the condition in the If Statement, 'age' is greater than or equal to \(18\), isn't met, then the 'else' block executes and a message "Not eligible to vote" is displayed.
How the characteristics of If Statement shape Javascript programming
The unique characteristics of Javascript If Statements lay the foundation for precise, conditional programming. These characteristics steer the code execution, offering control over what parts of your code will execute and when.
Remember, the Conditional Execution characteristic gives you power over your code's execution. It can be incredibly useful when you want to execute certain blocks of code only under specific circumstances. Whether you need to display personalised user messages or perform an action only when a particular condition is met, If Statements are your go-to method.
The property of using Logical and Comparison Operators is another shaping factor. It amplifies your capacity to write highly specific conditions, enabling you to fine-tune your flow of execution. With these operators, your If Statements become far more flexible and dynamic, catering to a broad array of conditions.
Adding to the flexibility is the characteristic of Pairing with Else or Else If. Complementing the If Statement with Else or Else If leads to more comprehensive and versatile flow control. When dealing with multiple potential scenarios or outcomes, this feature ensures that different blocks of code can be executed depending on various conditions, providing a more holistic response.
In essence, the unique characteristics of Javascript If Statement do more than just introduce conditionality in your code. They constitute a powerful syntax strategy, making your programming experience more efficient, versatile, and creative. Most importantly, they help to build responsive scripts that can adapt to different situations and make decisions, thereby facilitating functionality and smooth user experience.
Javascript If Statement - Key takeaways
- A Javascript If Statement enables one to test conditions, with code executed when the condition is satisfied, which is when the condition returns true.
- If Statements are typically combined with relational operators for comparison, such as 'equals to (==)', 'not equals to (!=)', 'greater than (>)', 'less than (<)', 'greater than or equal to (>=)', and 'less than or equal to (<=)'.
- The '===' operator checks not only values but also types of variables, making it more precise than the '==' operator in Javascript.
- Nested If Statements in Javascript are applied to check conditions within other conditions, with their execution based on the outcome of the outer condition.
- The Javascript If and Statement is used to check multiple conditions simultaneously by using the logical AND operator (&&), requiring all conditions to be true to execute the code block.
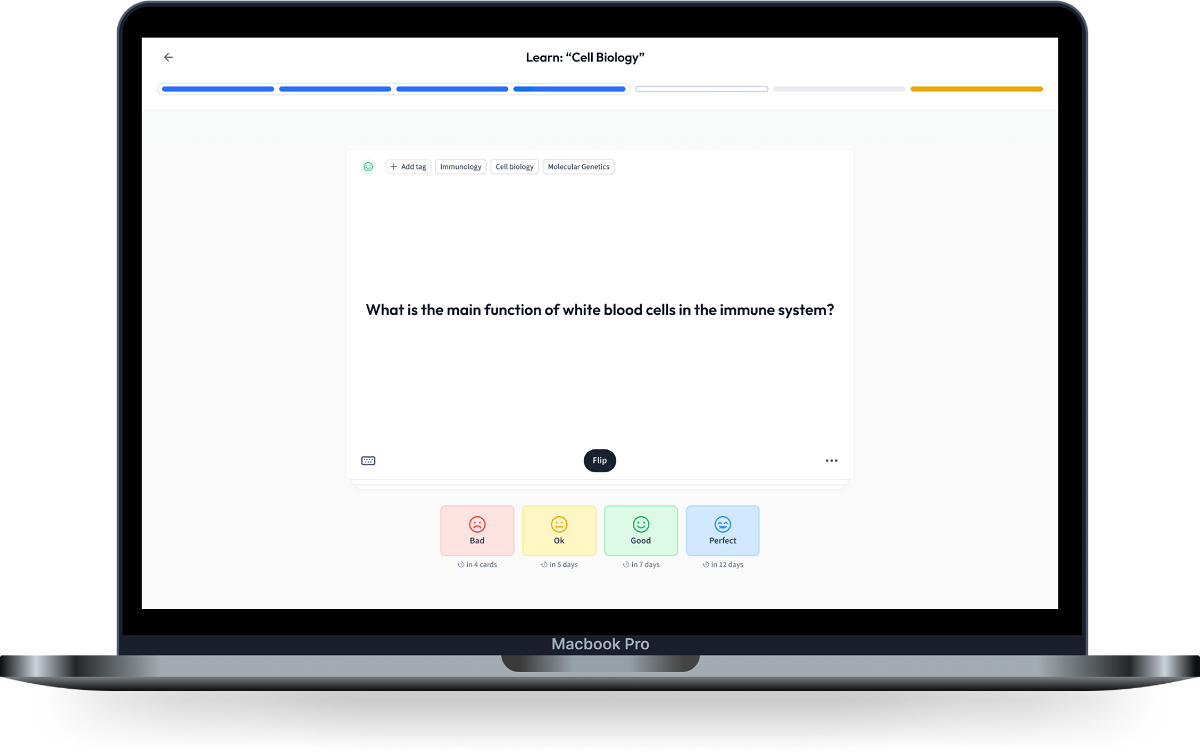
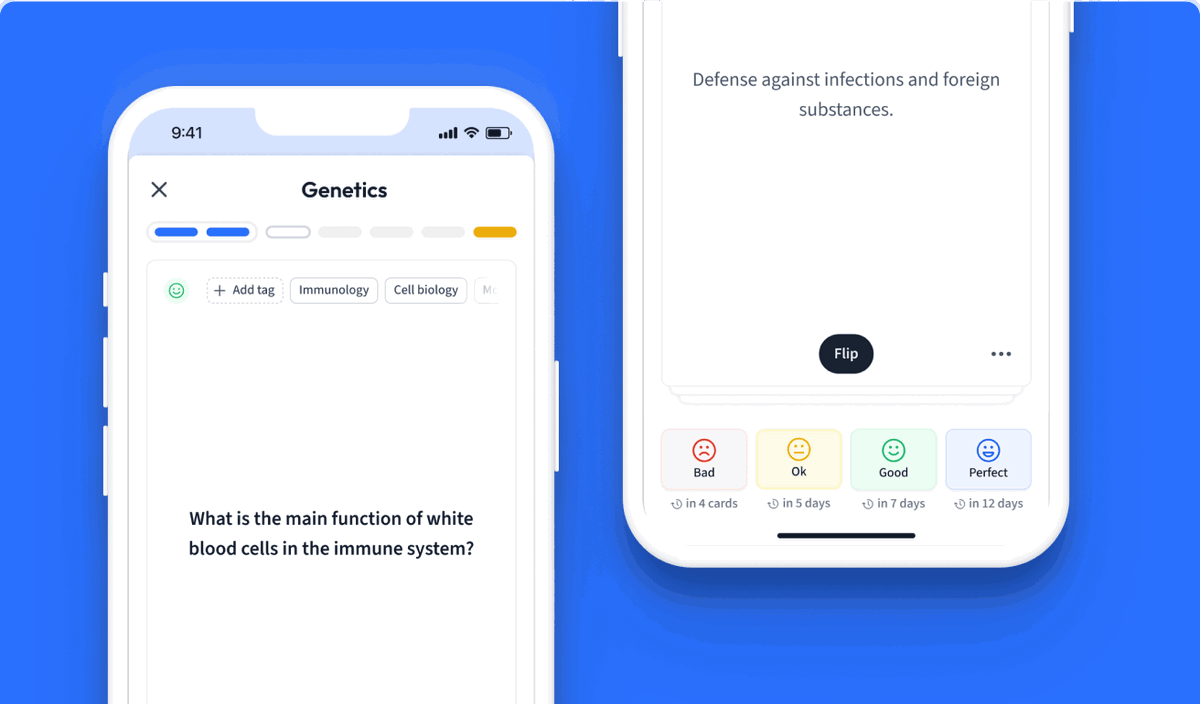
Learn with 12 Javascript If Statement flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Javascript If Statement
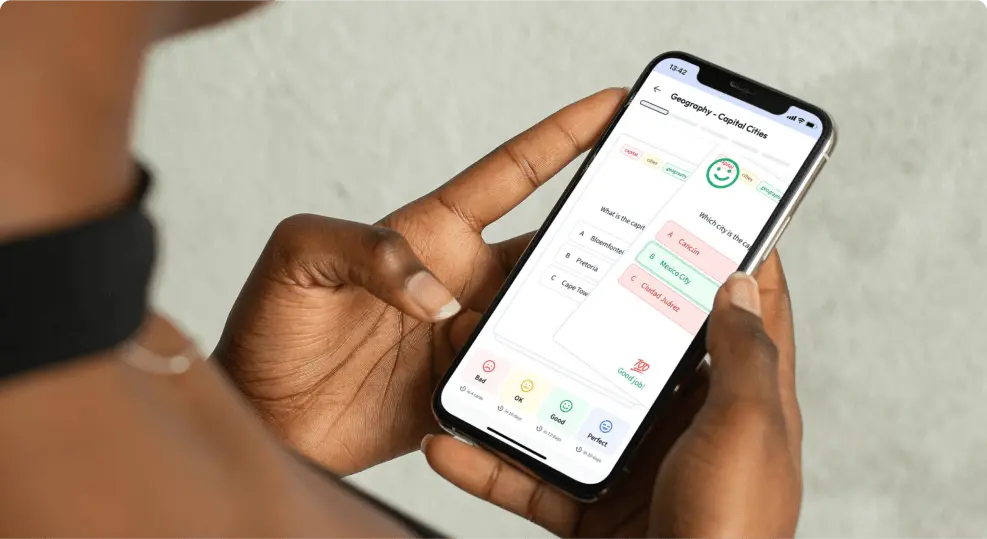
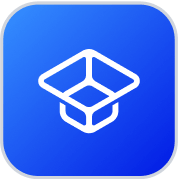
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more