Understanding Functional Programming Concepts
Diving into the realm of Computer Science, you'll come across varied programming paradigms, one of which is Functional Programming. It is a distinct method where computations are treated as mathematical functions, making your code reliable and predictable.Introduction to Core Functional Programming Concepts
Functional Programming pivots on some unique principles which set it apart from other programming methodologies. These core concepts ensure cleaner and more maintainable code, and bolster your program's efficiency.Immutable Data in Functional Programming
Immutable data, as the term suggests, cannot be altered once created. In Functional Programming Concepts, immutability is a fundamental principle. It eliminates side effects because if you cannot alter a data object, you cannot unknowingly affect the rest of the program.
const array1 = [1, 2, 3, 4]; const array2 = array1; array2.push(5);Here, pushing a new value to array2 inadvertently modifies array1 too. This is a side effect that could cause bugs. Immutability could preclude this issue.
Immutable.js, a JavaScript library developed by Facebook, is a widely-used tool that supports immutability in JavaScript applications.
First-Class and Higher-Order Functions
A significant feature of Functional Programming is the treatment of functions as First-Class citizens.First-Class functions imply that functions in that language are treated like any other variable. They can be created dynamically, passed as arguments or used as return values.
Deep Dive into Functional Programming Techniques
Not just theoretical principles, functional programming is about practical techniques to optimise and organise your code structure.Pure Functions in Functional Programming
A function is considered 'pure' if it produces the same output for the same input and has no side-effects. Essentially, a pure function's result solely depends on its input, making the code predictable and easier to test.
function sum(a, b) { return a + b; }The output of this function depends entirely on the input values 'a' and 'b'. No external variables manipulate the function, making it 'pure'.
The Role of Recursion in Functional Programming
The term 'recursion' refers to a function calling itself, providing a way to break down complex problems into simpler ones. Recursive functions are particularly efficient in Functional Programming as they prevent mutation and eliminate the need for loop constructs. Here's an example of a recursive function that calculates the factorial of a number:function factorial(num) { if (num === 0) { return 1; } else { return (num * factorial(num - 1)); } }In the example, the 'factorial' function calls itself to calculate the factorial, adhering to the principle of recursion. Though recursion can be a bit challenging to understand initially, once grasped, it simplifies array or list manipulations.]
Exploring Functional Programming Concepts in JavaScript
JavaScript, one of the world's most popular programming languages, is a multi-paradigm language that supports functional programming. This capability makes it versatile, providing you with the tools to reap the benefits of functional programming concepts in your applications.Understanding JavaScript as a Functional Language
In the realm of computer programming, JavaScript is a powerful and ubiquitous language that resonates with functional programming concepts. With JavaScript missing out on built-in support for immutability, incorporating functional programming practices requires an understanding of the core principles and a set of good practices.How JavaScript Handles Functional Programming
Despite JavaScript not being a "pure" functional language like Haskell or Erlang, it encompasses several elements of functional programming. Notably, JavaScript treats functions as first-class objects, allowing them to be stored in variables, passed as arguments, or used as return values.First-Class Objects, in the context of JavaScript, are entities with properties and methods, capable of being stored in a variable, passed as a function parameter or returned from a function.
String.prototype.concat()
Array.prototype.concat()
Array.prototype.slice()
push()and
splice()are destructive, as they do mutate the original array. Thus, these should be avoided if you aim to adhere firmly to functional programming principles.
For instance, consider this piece of JavaScript code that adheres to functional programming by using the .concat() method instead of .push()
const arr1 = [1, 2, 3]; const arr2 = arr1.concat(4); // arr2 = [1, 2, 3, 4], arr1 remains unchanged
JavaScript and Higher-Order Functions
Higher-Order Functions play a vital role in functional programming. In JavaScript, the name of these gift-bearing "elevated" functions should evoke familiar patterns for those who have worked with arrays.Higher-Order Functions, in JavaScript, are functions that operate on other functions. They can either accept other functions as parameters or return them as a result.
Array.prototype.map()
Array.prototype.filter()
Array.prototype.reduce()
Analysing Functional Programming Concepts Example in JavaScript
The theoretical concepts of functional programming can be elucidated best with examples in JavaScript. Let's consider pure functions and recursion, two cornerstones of functional programming.Examples of Pure Functions in JavaScript
Pure functions, a fundamental tenet of functional programming, dramatically ease debugging and testing in JavaScript.Pure Functions are predictable functions that give the same output for the same set of inputs, irrespective of the number of times they are called. They have no side effects and don't depend on any data not passed into them as input.
function add(x, y) { return x + y; }
Recursion Examples in JavaScript Programming
'Recursion' refers to a process where a function calls itself until it reaches a specified condition — the base case. In JavaScript, the use of recursion aligns well with the principles of functional programming, since it avoids state changes and mutations that iterative processes like loops might introduce. Here's an example to calculate the factorial of a number using recursion in JavaScript:function factorial(n) { if (n === 0) { return 1; } else { return n * factorial(n - 1); } }The factorial function keeps calling itself with a progressively smaller argument until it reaches the base case (\(n = 0\)), where it returns 1. Then it starts returning, multiplying each return value by the current argument. In this way, the function recursively calculates the factorial without mutating any state.
The Connection of Functional Programming Concepts in Computer Science
In the vast and diverse field of Computer Science, Functional Programming has emerged as a building block, issuing a unique style of software construction. This paradigm is not just an academic concept but has influences that stretch into every corner of modern programming.Functional Programming's Influence on Modern Programming
Functional programming seeks not just to make the task of programming easier, but to fundamentally improve the quality, scalability, and maintainability of software. Understanding Functional Programming concepts can significantly change your perspective on programming tasks and problems. In virtually every type of software system–from web applications and mobile apps to machine learning and data science–Functional Programming design techniques can make a difference. Similar to how reading a novel changes one's perspective of the world, understanding functional programming broadens your problem-solving perspective and makes you a better programmer. Consider the influence of Functional Programming concepts on JavaScript, a popular, omnipresent web development language. JavaScript's shift towards Functional Programming has led to the widespread adoption of functional methods such as .map(), .filter(), and .reduce(). Additionally, libraries like React heavily rely on these functional concepts and on the principle of immutability to manage application state predictably and scalably. In a world of Concurrency and Parallel Computing, Functional Programming shines bright with multitasking capabilities. Here's why:- Immutability: Since data can't be changed once created, overlapping processes cannot disrupt each other by unintentionally changing shared data.
- Statelessness: In Functional Programming, functions don't have states, thereby eliminating potential race conditions in multi-threaded environments.
Why Functional Programming Matters in Computer Science
One may wonder why Functional Programming matters so much in Computer Science. Here's why:- Maintainability: Functional Programming is precise and modular. The inherent immutability of the paradigm helps resist bugs, providing a solid basis for future code modifications and extensions.
- Efficiency: Functional Programs are easy to parallelise since functions are independent of each other. The absence of shared state, mutable data and side-effects means that computations can run independently.
- Scalability: Functional Programming paradigms are excellent for managing and manipulating large data sets—a common task in the age of Big Data.
Evaluating the Impact of Functional Programming on Coding Efficiency
Coding efficiency refers to the art of achieving an operation with the least amount of code, time, and effort. Let's analyse how Functional Programming paves the way for high coding efficiency. In Functional Programming, code is data and data is code. Due to HOFs–Higher Order Functions—you can use functions like any other data type. This way, code becomes highly reusable, yielding a substantial increase in efficiency. This code reusability translates into fewer bugs, improved readability, and less time spent on problem-solving.Higher Order Function: A function that takes one or more functions as arguments, returns a function, or both.
const add = (x, y) => x + y; const double = num => add(num, num);In the example above,
addis a reusable function, while
doubleis a Higher Order Function that uses
addto perform its task. When discussing efficiency, it's important to not overlook the impact of Immutable Data. The inability to change data once it's created ensures consistent program behaviour and eliminates side effects, leading to fewer bugs and less troubleshooting time. To summarise, Functional Programming principles, when applied correctly, can tremendously improve coding efficiency by reducing bugs, decreasing development time, and increasing code readability. While the initial learning curve may be steeper, the gains achieved in the long term make this paradigm a valuable asset in any developer's toolkit.
Practical Application of Functional Programming Techniques
The field of functional programming offers an assortment of techniques that can be leveraged to enhance the robustness and efficiency of code. Applying these concepts to practical programming can yield far-reaching benefits, shaping the code to be more maintainble, reusable, and easier to understand.Writing Efficient Code Using Functional Programming
In any programming project, efficiency plays a pivotal role. With regards to functional programming, this paradigm peculiarly focuses on the purity of functions and the minimisation of mutable state—two fundamental aspects that can considerably optimise performance and readability while quashing potential bugs.Strategies for Efficient Code Writing
Efficient coding, in the realm of functional programming, involves utilising programming techniques that optimise problem-solving without compromisng on code clarity or performance.Efficiency, in coding, signifies achieving the desired functionality with the least amount of computer resources, including memory, CPU cycles, and bandwidth.
- Pure Functions: A function is said to be pure if it produces the same output for the same inputs every time, and it has no side effects. Pure functions contribute to the maintainability and predictability of code, thus buzzing a notion of efficiency.
- Immutability: Immutable data remains constant once created. Promoting immutability can help prevent potential bugs related to data changes, aiding in overall code optimisation.
- Recursion: Functional programming often exploits recursion as a primary control structure since it couples well with immutability and stateless functions. Recursive functions can often perform tasks with less code than iterative functions, promoting efficiency.
- Higher Order Functions: In functional programming languages, functions are first-class citizens. Therefore, high order functions, which take other functions as arguments or return a function as a result, are a distinctive strategy utilised to construct efficient code. Higher order functions can simplify the logic and improve code abstraction, thereby enhancing code readability and maintainability.
The code snippet below demonstrates these concepts in a familiar sorting algorithm, bubble sort, using Higher Order Functions and recursion in JavaScript:
const bubbleSort = (arr) => { const sorted = arr.reduce((acc, num, i, origArr) => { return origArr[i + 1] < num ? acc.concat(origArr[i + 1]).concat(num) : acc.concat(num); }, []); if (JSON.stringify(arr) === JSON.stringify(sorted)) { return sorted; } else { return bubbleSort(sorted); } };This example highlights a significant aspect of functional programming—solving problems declaratively rather than imperatively. All the functions—reduce, concat, JSON.stringify—are pure, demonstrating other aspects of Efficiency, Pure Functions, and Immutability.
The Benefit and Challenges of Functional Programming
Functional programming, while being highly beneficial for creating clean, modular, and testable code, does present a set of distinctive challenges. Benefits of functional programming include:- Maintainability: With its principles of immutability and pure functions, functional code is simple and easy to debug, making maintenance easier.
- Modularity: Functional programs are made up of small, testable functions making the code highly modular and thus, reusable.
- Scalability: Because of its ability to handle large and complex tasks using fewer lines of code.
- Learning Curve: Functional programming requires a shift in mindset from the common imperative and object-oriented paradigms. It could be daunting for beginners, thus a steeper learning curve.
- Verbosity: In some cases, functional programming can lead to verbose code, which might be harder to read and understand.
- Performance: Sometimes, functional operations like recursion can be less performant than traditional loops, especially for substantial datasets. However, modern compilers and interpreters have significantly improved, and they now optimise for such operations.
Expanding Knowledge on Core Functional Programming Concepts
A profound understanding of core functional programming principles, such as immutability and pure functions, can yield significant benefits for any programmer. These principles form the foundation of the functional programming paradigm and deliver several advantages when applied, like enhanced code maintainability and predictability.More About Immutable Data and Pure Functions
Functional Programming brings with it a myriad of tools and methodologies that can enhance your coding aptitude immensely. Of these, Immutable Data and Pure Functions stand at the forefront.Pure Functions are functions where the return value is entirely determined by its input values, and do not produce side effects. Side effects are changes made outside the function's scope, like modifying a global variable or changing an input parameter.
Immutable Data forms a cornerstone of functional programming. It refers to data that can't be changed after creation. Any operation on immutable data will return a new piece of data rather than modifying the original data.
Benefits of using Pure Functions in Coding
Pure functions illuminate several advantages that promote clean and efficient code development:- Predictability: The output of a pure function is solely dependent on its input, rendering it highly predictable and easier to test.
- No-side effects: Pure functions do not alter other parts of the system, reducing the fear of accidental alterations.
- Composition: Pure functions can be composed to form complex functionality. This ability to combine functions promotes modularity and code reuse.
let name = 'John Doe'; function greet() { alert('Hello, ' + name); } function changeName(newName) { name = newName; }Here, the order of function calls would determine the name used in the greeting—which is a typical example of temporal coupling. You would not face such issues when using pure functions.
Understanding Immutability in Depth
Immutability is a concept where a variable can't change its value once it's set. In a programming context, immutability can impart several benefits:- Robustness: Variables can't be changed unexpectedly, thus reducing bugs.
- Simplified Reasoning: Since data doesn't change once set, it's easier to reason about the state of a program at any given point.
- Concurrency Safety: One thread can't accidentally modify data used by another thread.
Functional Programming Concepts - Key takeaways
- JavaScript and Functional Programming: JavaScript, while not a 'pure' functional language, adopts several functional programming principles. It treats functions as first-class objects, meaning they can be assigned to variables, passed as function parameters and returned as results.
- Immutability in JavaScript: To maintain immutability in JavaScript, methods such as
String.prototype.concat()
,Array.prototype.concat()
, andArray.prototype.slice()
that don't mutate the data should be preferred. Methods such aspush()
andsplice()
which mutate the original array, contradicting the principle of immutability should be avoided. - Higher-Order Functions: These are functions in JavaScript that can accept or return other functions. Built-in JavaScript Higher-Order Functions include
Array.prototype.map()
,Array.prototype.filter()
andArray.prototype.reduce()
. - Functional Programming concepts in Computer Science: Functional Programming has significant influence on modern programming improving the quality, scalability, and maintainability of software. Concepts such as immutability and statelessness from Functional Programming have applications in various domains including web applications, data science, and parallel computing.
- Functional Programming Techniques: Strategies for efficient code writing in functional programming includes the use of pure functions, immutable data, recursion and Higher Order Functions. These principles enable writing code that uses lesser computer resources, have fewer bugs, improve readability, and thus increasing overall efficiency.
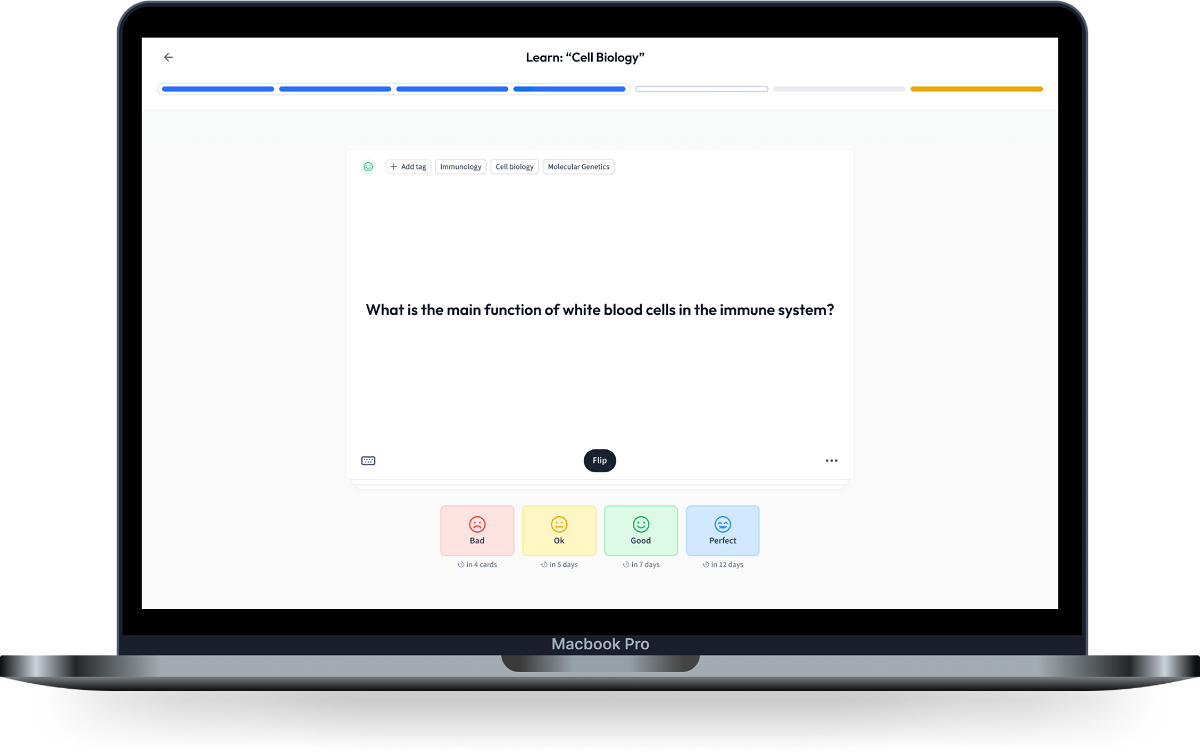
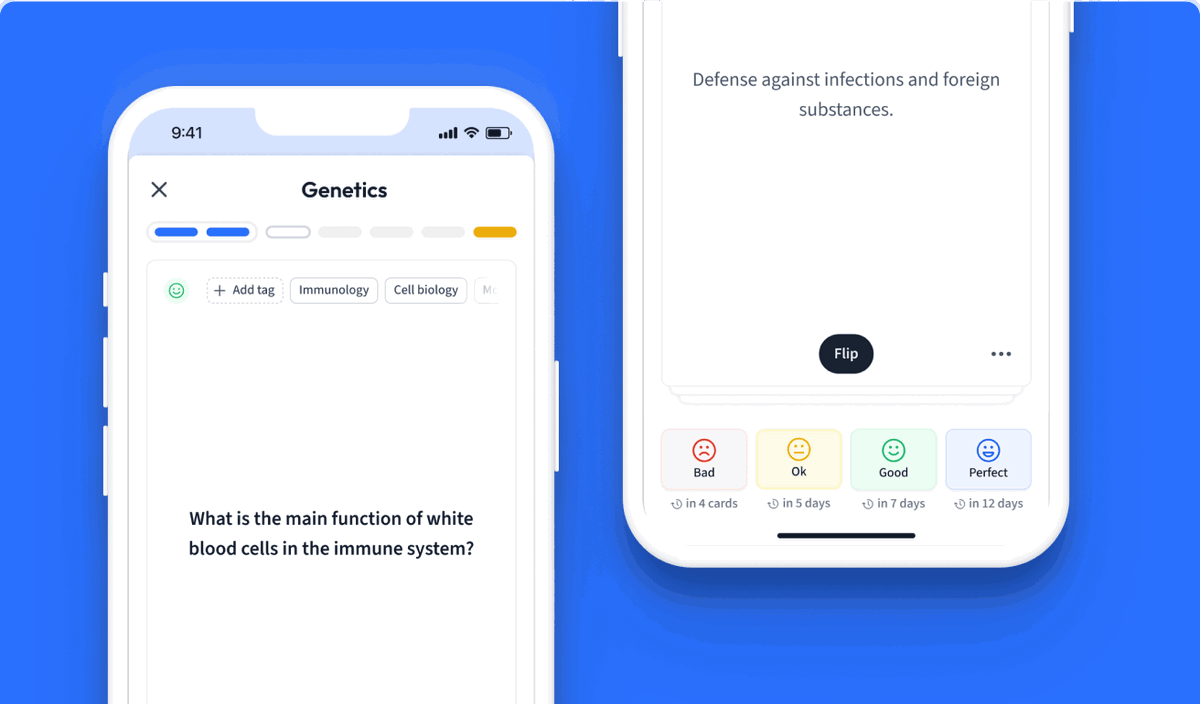
Learn with 132 Functional Programming Concepts flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Functional Programming Concepts
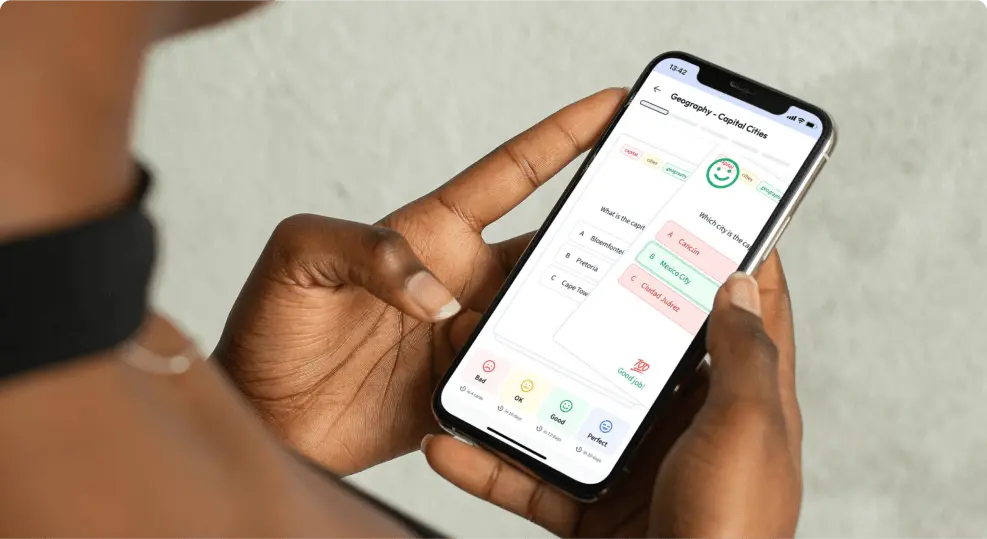
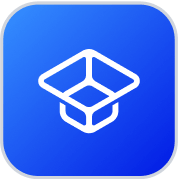
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more