Introduction to the Scala Language
The Scala language is a high-level, modern programming language that is designed to express common programming patterns with a concise, elegant, and type-safe way. It has been gaining popularity for being a language of choice for many types of applications – from small scripting tasks to large systems.A high-level language like Scala is designed to be easy for humans to write, read, and understand. It has a considerable level of abstraction from machine code and is closely related to natural language and programming concepts.
Origin and Design of the Scala Coding Language
Let's take a deep dive into the creation and conceptualization of the Scala language, shall we?
Think of it like a having a car that you can easily modify and upgrade, be it the engine for more speed or adding navigation systems for better driving assistance. That's what Scala is all about in the world of programming languages. It's adaptable, scalable, and versatile!
- It is primarily object-oriented: Every value is an object and every operation is a method-call.
- It is also functional: Functions are first-class values. It supports advanced component architectures through classes and traits.
object HelloWorld {
def main(args: Array[String]) {
println("Hello, world!")
}
}
Understanding the Scala Language Specification
To fully understand the Scala language, you need to explore its specifications, which are technical details describing the behaviour of the Scala platform. One of the key features of Scala is its seamless integration of Object Oriented and Functional programming. The following table provides an overview:Object-Oriented | Functional |
---|---|
Every value is an object | Functions are first-class values |
Types and behavior of objects are described by classes and traits | Operations of calculations and data manipulations are carried out with the use of functions |
Static Type System can help to eliminate potential bugs by detecting an error at compile-time rather than at run-time.
val a = 10
val b = 20
val sum = a.+(b)
This demonstrates an essential aspect of Scala – that every operation is a method call, even the ones that seem to be simple mathematical operations. Conclusively, the Scala language provides a blend of object-oriented and functional programming. It is powerful, adaptable, and scalable, which makes it an appealing choice for your programming needs!Exploring Features of the Scala Language
The Scala language offers a multitude of features that are designed in coherency with the modern needs of programming. By offering the hybrid of functional and object-oriented programming paradigms, the Scala language increases productivity, scalability and coder satisfaction.
Key Components of the Scala Language
To understand the strength and flexibility of the Scala language, let's discuss some of its key components. Object-Oriented Programming: In Scala, every value is an object and every operation is a method. It means, all the traditional structures like integers, booleans, and functions are objects. This characteristic allows programmers to construct robust and concise code snippets. For instance, you can add two numbers, a and b, like this:val a = 10
val b = 20
val sum = a.+(b)
Functional Programming: Scala supports functional programming out of the box. It treats functions as first-class values and allows them to be stored into variables, passed to other functions, returned as values, etc. val closeDoor = () => println("door closed")
Immutable Data:Scala encourages the use of immutable data particularly in functional programming. Immutable data increases code predictability and makes the code simpler for parallel programming.Let’s assume you have an object representing a date and time. In a mutable setting, it could be possible to set the day to 32. The object, in this case, would be in an inconsistent state between the operation of setting the day and correcting it. With Immutable objects, operations either create new objects or fail, without any in-between states.
Did you know? Type information in Scala is used at compile time to check the correctness of programs. This means, you can prevent many runtime errors by catching them at compile time with Scala's robust and expressive static type system.
Advanced Scala Language Features
Scala language is known for its synergistic blend of object-oriented and functional programming. This gives it a powerful set of advanced features that includes: Concurrency & Distribution: Scala provides a practical set of language-level features that allow developers to cope with concurrency, parallelism and distribution. It does this using Futures and Promises, Akka actors, or concurrent data structures. Pattern Matching: Scala has a powerful pattern matching mechanism. It allows you to match on any sort of data with a first-match policy. This functionality is similar to switch statements in other languages like C++, Java etc., but on steroids! For instance, if you have a list of numbers and you want to print out all the zeros in the list, you can do so using pattern matching:numbers.foreach { number =>
number match {
case 0 => println("zero")
case _ => // do nothing
}
}
String Interpolation: Scala makes it simple to embed variable references directly in processed string literals. This can be very handy for debugging or creating dynamic strings. For example, val name = "Scala"
println(s"Hello, $name!")
Traits:Traits in Scala language are a way to share interfaces and fields between classes. They are similar to Java's interfaces, but can also have method implementations and state.Scalability and Performance of the Scala Language
Scala delivers high scalability and performance, which makes it a preferred choice for many developers and businesses around the globe. Scalability: The name Scala originates from the term "Scalable Language". It suggests that it is designed to scale with the growing demands of its users, from small scripting tasks to large systems. One of the ways Scala achieves this scalability is through its functional and object-oriented programming paradigms. It has been designed to express general programming patterns in a concise, elegant, and type-safe way. Which eliminates redundant code and thus, improves development speed, reducing errors. Performance: Scala also prioritizes performance. It uses the Java Virtual Machine (JVM) as the runtime platform, which means it inherits the performance optimisations of the JVM. Also, as a statically typed language, performance is not hindered due to dynamic typing overheads at runtime. In conclusion, Scala, with its scalability and performance capabilities, delivers robust solutions, irrespective of the problem's complexity. It harmoniously combines object-oriented and functional programming paradigms, making it a highly versatile language.Practical Uses of the Scala Language
The Scala language, with its elegant fusion of object-oriented and functional programming, finds a broad spectrum of applications. In the software industry, Scala has become a language of choice due to its ability to simplify complex tasks and provide scalable solutions.Popular Scala Language Uses in the Software Industry
The Scala language's unique combination of object-oriented and functional programming paradigms significantly expands its practical applications in the software industry. Here are some popular uses of Scala: Data Analysis: The availability of distributed computing tools in Scala such as Apache Spark, helps perform data analysis tasks on a massive scale. Whether you need to perform complex computations or manage large databases, Scala offers a reliable solution. Web Development: Scala language also finds use in web development. The Play Framework, which is a high velocity web framework for Java and Scala, makes it easy to build web applications. It provides a productive environment for building scalable web applications, thanks to Scala’s powerful features. Game Development: Several game developers are also turning to Scala language to leverage its strong support for concurrent programming. Machine Learning: In the era of artificial intelligence and machine learning, the Scala language stands as a viable choice due to its ability to handle complex mathematical calculations and distributed computing through libraries like Breeze and Spark MLLib. Distributed Systems: Scala's mature ecosystem incorporates projects like Akka, which simplifies the creation of fault-tolerant and event-driven applications ideal for distributed systems.Scala in Large Scale Systems
Building large scale systems such as microservices or real-time data streaming systems demands robust and efficient programming languages. Scala language, with its expressive syntax and powerful features, makes it possible to design architecturally elegant and scalable solutions in such scenarios. Prominent companies such as Twitter, LinkedIn, and Netflix have incorporated Scala to develop some parts of their large-scale backend systems. Employing Scala has enabled them to handle vast amounts of data, take advantage of multi-core architecture, and even manage system failures gracefully.How Scala Coding Language Simplifies Complex Tasks
Scala language has the power of simplifying complex programming tasks, particularly ones that deal with concurrency, parallelism, and large data processing. Simplified Concurrency & Parallelism: Dealing with multi-threaded code can be a challenging task. However, Scala simplifies this via features like immutability and the actor model provided by libraries such as Akka. The actor model makes it easier to write concurrent and distributed systems by offering high-level abstractions over low-level threading mechanisms. For example, let's consider a banking system with multiple transactions happening simultaneously. Here, Scala's actor model can enable easy and effective communication between various entities(transaction, account, bank) in a thread-safe manner. Ease in Handling Large Data: Scala shines in handling big data with robust tools like Apache Spark. Using Scala, you can easily code big data processing tasks, which allows developers to focus on the problem at hand rather than worrying about handling large datasets. An illustration of the code could be something like this:val data = spark.read.json("example.json")
data.filter(data("age") > 30).show()
This example shows how to read a JSON file and filter the data for ages greater than 30. The code is concise and readable, even for large data processing. Pattern Matching: Pattern matching is another feature where Scala shines. It allows developers to quickly address individual cases for complex, nested data structures. This function is not available in many popular languages such as Java, but it greatly simplifies the development process in Scala. Pattern matching allows you to address each case within a data structure directly, making your code cleaner and more manageable. A simple illustration of pattern matching could be:
list match {
case head :: tail => process(head)
case Nil => println("Empty list")
}
In the example above, depending on whether the list is empty or not, an appropriate action is performed. This makes handling complex data structures significantly more straightforward. To conclude, Scala provides a variety of powerful features that make it easier to solve complex programming tasks. Its flexible and adaptable nature allows developers to express their ideas concisely without sacrificing performance or scalability. This makes Scala a versatile language suitable for a wide array of applications in the software industry.Learning to Code with Scala
Scala is a brilliant choice to learn to code due to its ability to support both object-oriented and functional programming paradigms. Its code is concise, expressive, and equipped with sophisticated features like immutability, pattern matching, and higher-order functions. As a statically-typed language, Scala can help catch potential bugs earlier by checking the type safety at compile time rather than waiting until runtime.Scala Language Example for Beginners
Initially stepping into a new programming language can be daunting. Therefore, let's start with a simple example to help illustrate the basic syntax and structure of the Scala language. A quintessential example for any budding programmer is the classic "Hello, World!" program. In Scala, it would look something like this:object HelloWorld {
def main(args: Array[String]): Unit = {
println("Hello, World!")
}
}
This is a standalone Scala program. Let's break it down: The keyword `object` declares a singleton object, which is a class that has exactly one instance. Here, `HelloWorld` is the object, which is the name of our program. The `main` method is the entry point of a Scala program. The `main` method takes one parameter, `args: Array[String]`, which is an array of command-line arguments. The `Unit` keyword corresponds to `void` in Java or C++. It signifies that the function doesn't return any meaningful result. Finally, `println("Hello, World!")` is a built-in Scala method for printing to the console with a newline after it. It's equivalent to `console.log` in JavaScript or `System.out.println` in Java.This is the most basic Scala program you can write, but it signifies a very important practice implemented in every language — printing to the console. It's these basics that lay the groundwork for more complex programs and concepts.
def add(x: Int, y: Int): Int = {
return x + y
}
println(add(5, 7))
In this example, the `add` method takes two parameters `x` and `y` of type `Int`, and defines their sum. This demonstrates how to define a function and pass parameters in Scala.Advanced Scala Language Example
Moving on to more advanced Scala programming, let's look at higher-order functions and pattern matching. A Higher-order function (HOF) is a function that does at least one of the following: takes one or more functions as arguments, returns a function as its result. In the following example, we define a method `apply()` that takes another function `f` and a value `v` and applies function `f` to `v`.def apply(f: Int => String, v: Int) = f(v)
def layout[A](x: A) = "[" + x.toString() + "]"
println(apply(layout, 10))
In the example above, function `layout` is passed into `apply` function as an argument. This is a basic illustration of a higher-order function. Scala also offers a feature named pattern matching which can be compared to a switch statement in imperatively used languages. It allows you to test the value against a series of patterns. If it finds a match, it executes the associated code.val dayOfWeek = "Saturday"
dayOfWeek match {
case "Monday" => println("It's the start of the work week.")
case "Friday" => println("Almost weekend!")
case "Saturday" | "Sunday" => println("Yay, weekend!")
case otherDay => println("Just another day.")
}
In this code snippet, we're matching the value of `dayOfWeek` against several string patterns. If `dayOfWeek` matches with "Saturday" or "Sunday", the program prints "Yay, weekend!"The Role of Functional Programming in Scala
Functional programming is one of the cornerstones of the Scala language. Scala provides powerful functional programming abstractions that enable developers to write expressive and concise code. In a functional programming paradigm, functions are regarded as first class values. It means that they can be passed as arguments, stored in variables, returned from other functions, etc. Essentially, it provides programmers with flexibility to use functions just like any other data type. For example,val double = (i: Int) => i * 2
println(double(4)) // prints 8
In this code, `double` is a function that takes an integer as input and doubles it. This is an example of a simple lambda function or an anonymous function in Scala. Moreover, functional programming favors immutability, which makes your programs more predictable and easier to understand. Scala language also supports pure functions, i.e., functions that do not produce any side effects. They always produce the same result given the same inputs and can be invoked at any time without affecting other parts of the program. For instance: val x = 10
val addFive = (i: Int) => i + 5
println(addFive(x)) // prints 15
Here, `addFive` is a pure function. No matter how many times you execute this function with the same value of `x`, the result will always be the same. With these powerful functional attributes, Scala instills wise programming practices that culminate in robust, maintainable code that is easier to reason about and debug. Functional programming in Scala is no afterthought. It's an inherent part of the language's design, making Scala an effective tool for tackling complex business logic and developing high-performance applications.Advancing Your Skills in the Scala Language
Having a fundamental understanding of Scala is an excellent start. However, in the ever-evolving landscape of computer science, continuously learning and refining your skills is imperative. Here are several ways in which you can continue your journey with the Scala language and reach advanced level proficiency.Resources for Further Learning in Scala Coding
There are an abundance of resources available to catapult your understanding and usage of Scala language beyond the beginner level. Let's explore some of them. Online Interactive Platforms:Interactive learning platforms provide a hands-on environment making learning engaging and effective. Platforms such as:offer comprehensive courses and exercises which allow you to practice Scala, get real-time feedback, and improve your skills as you progress. Books: A number of high-quality books written by Scala experts are available to deepen your understanding of the language. Some top ones include:- Programming in Scala by Martin Odersky, Lex Spoon, and Bill Venners
- Functional Programming in Scala by Paul Chiusano and Rúnar Bjarnason
- Scala Cookbook by Alvin Alexander
- Participating in forums like Scala Users, can help you get answers to your queries.
- Open source projects on Github allow you to look at real-world applications of Scala and learn from existing codebases.
You can also attend local meetups or global Scala events and conferences to interact with and learn from other developers, share your ideas, and stay up-to-date with the latest advancements in the Scala language.
Deep Diving Into the Advanced Scala Topics
Apart from the resources mentioned above, some advanced topics need your attention to fully master the Scala language. Type System: Scala has a powerful and expressive type system. Understanding this in-depth will provide you with the ability to fully harness the power of Scala's static type-checking. Exploring concepts such as variance annotations, upper and lower type bounds, abstract types, and compound types is vital. Implicit Parameters and Conversions: One of Scala's distinctive features is its use of implicits for parameters and conversions. While being a subject of discussion due to their complexity, they contibute to scala's power and flexibility. Understanding their working, use-cases, and trade-off's is an essential step in becoming an advanced Scala programmer. Macros and Metaprogramming: Scala also provides facilities for metaprogramming, such as macros, which provide the ability to manipulate Scala code within Scala itself. While macros can be complex, they empower the end users with tools to simplify repetitive tasks and yield powerful libraries and DSLs. Concurrency and Distributed Systems (Akka):The principles of constructing concurrent and distributed systems are crucial. Here, the Akka library shines, which implements the Actor Model providing concurrency and fault-tolerance. Exploring Akka and its eco-system can boost your understanding about building scalable and resilient systems.The Actor model in computer science is a mathematical model of concurrent computation that treats "actors" as the universal primitives of concurrent computation: in response to a message that it receives, an actor can make local decisions, create more actors, send more messages, and determine how to respond to the next message received.
Scala language - Key takeaways
The Scala language is a high-level, modern programming language designed to express common programming patterns in a concise, elegant, and type-safe way.
Scala stands for Scalable Language, hinting at its design to grow with the demands of its users.
The Scala coding language merges object-oriented and functional programming paradigms.
One key feature of Scala is its seamless integration of Object Oriented and Functional programming, with every value being an object and every operation being a method-call.
Scala employs a static type system, which means that the type of every variable and expression is predefined and does not change over time.
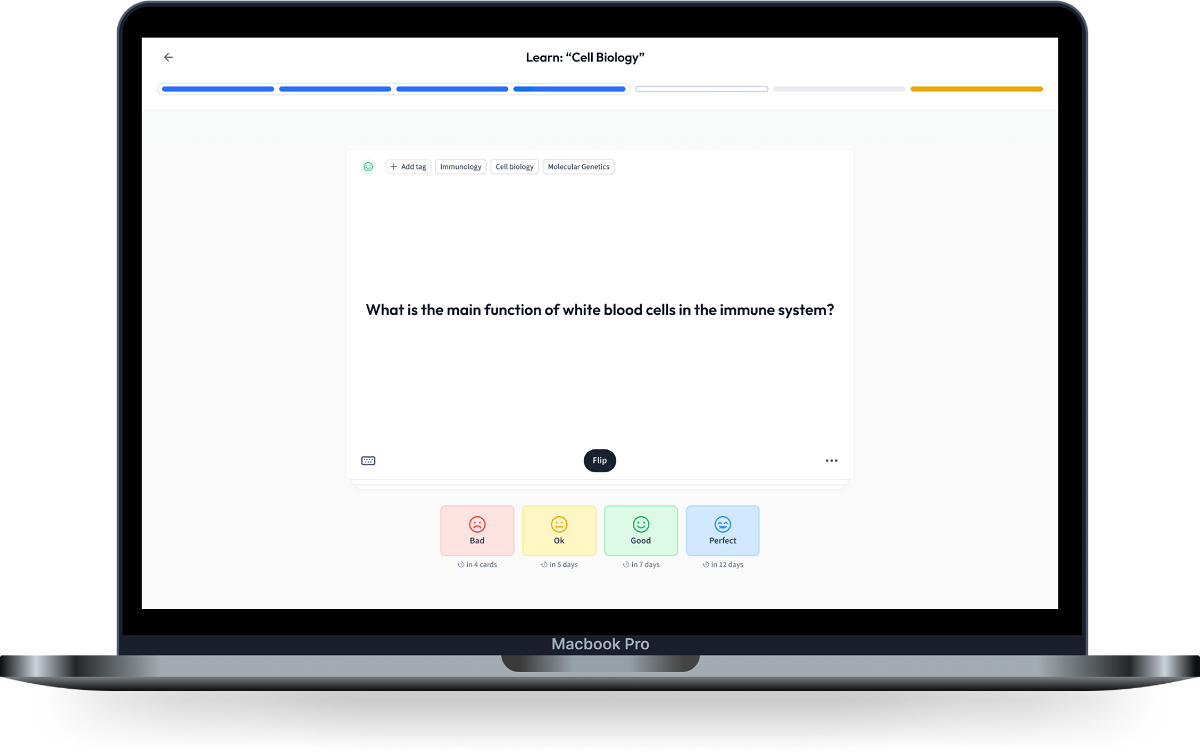
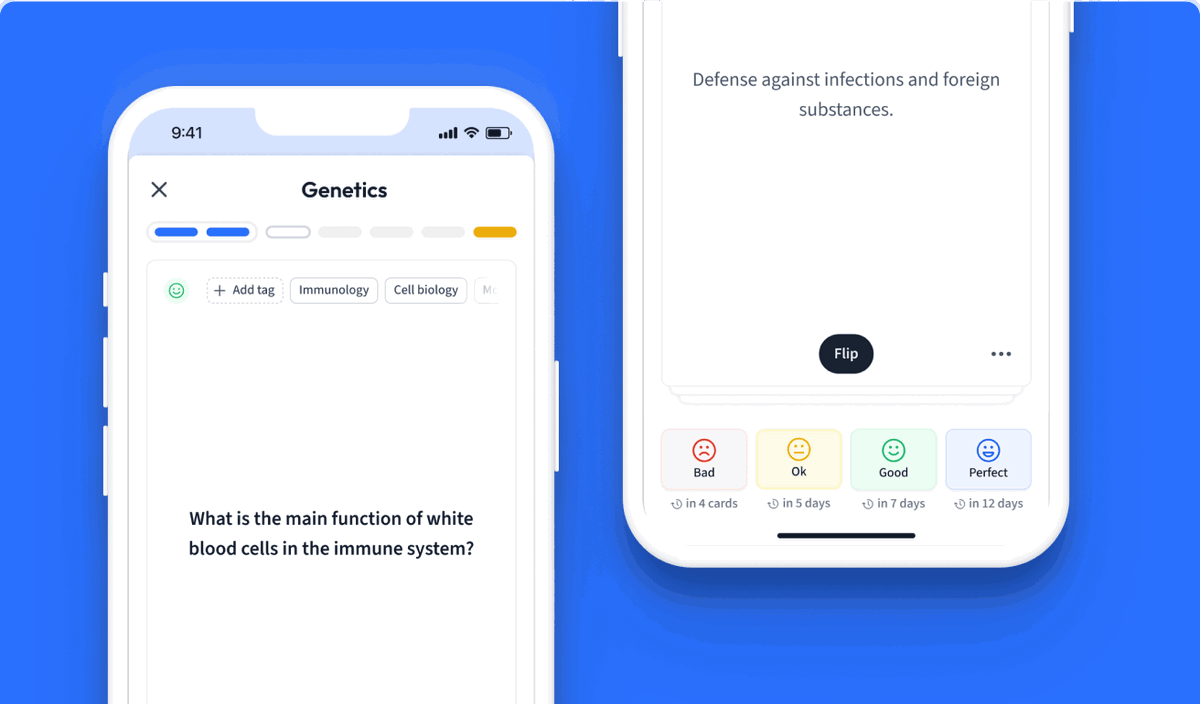
Learn with 15 Scala language flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Scala language
Is scala a functional language?
Is scala a good language?
What is scala language?
What is scala language used for?
Is scala a backend language?
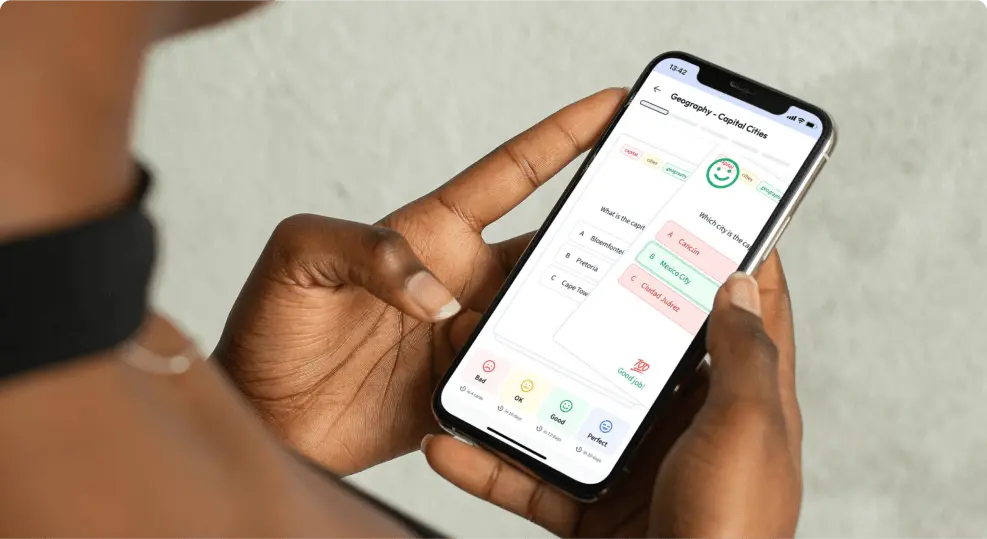
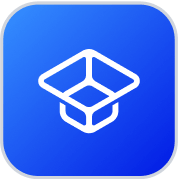
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more