Understanding Lambda Calculus in Computer Science
Lambda Calculus - you may have heard the term, but what does it truly mean and how is it applied in computer science? Let's pull back the curtain and venture into this fascinating subject.
What is Lambda Calculus
Lambda Calculus is a mathematical framework used to describe computations. The basis for functional programming, it focuses on applying functions rather than changing state.
Alonzo Church, with the aid of his students, such as the famous Alan Turing, developed Lambda Calculus in the 1930s. Ironically, it was created before machines could use it!
Origin and Application of Lambda Calculus
Lambda Calculus primed the pump for the computer science revolution. While its birth occurred in the halls of academia, you'll find its influence across the technology landscape today. Here are a few examples of Lambda Calculus' application:- Functional Programming
- Artificial Intelligence
- Machine Learning
Basics of Lambda Calculus Technique
Now you're familiar with what Lambda Calculus is and where it came from. But, how does it work? A lambda function, in simplest terms, is written as follows:\(\lambda x.f(x)\)Where \(\lambda\) is the lambda operator, \(x\) is the argument, and \(f(x)\) is the function applied to x.
As an example, for a lambda function adding 2 to an argument, it would be written as: \(\lambda x. x+2\).
Understanding Lambda Calculus Formulation
To make lambda calculus more understandable, let's divide it into three basic elements:Variables | x, y, z, a, b, c... |
Abstraction | \(\lambda x.F\) where x is a variable, and F is any lambda term |
Application | (M N) where M and N are lambda terms |
abstraction, the variable x is bound in the body F, and in application, a function is applied to an argument.
Grasping Lambda Calculus Principles
Now, as you journey deeper into the realm of Lambda Calculus, you will encounter its core principles, which form the bedrock of this system. Understanding these integral aspects – such as Alpha-Equivalence and Beta-Reduction, as well as the variations in Extended Lambda Calculus – paves the way to a more comprehensive grasp of this subject, offering you valuable insights into this profound area of computer science.
Core Principles of Lambda Calculus
Lambda Calculus operates on a set of principles that guide how computations are performed. Each principle contributes to the overall functionality of the system, shaping the behaviours of lambda functions and their interactions:- Variable Binding: This is the fundamental behaviour of lambda functions. When a lambda function is formed, a variable within that function is 'bound' to the function.
- Alpha-Equivalence: Two lambda expressions are alpha-equivalent if they differ only by the names of their bound variables.
- Beta-Reduction: Also referred to as application, this principle indicates how a lambda function behaves when it's applied to an argument.
- Extended Lambda Calculus: This involves extensions to the core lambda calculus, introducing features that offer extra descriptive power or computational efficiency.
Lambda Calculus: Alpha-Equivalence and Beta-Reduction
Delving into Lambda Calculus, two concepts you'll encounter early on are Alpha-Equivalence and Beta-Reduction. Alpha-Equivalence is a principle that acknowledges the names of variables in lambda functions as being arbitrary. For instance, the function \(\lambda x.x\) is alpha-equivalent to \(\lambda y.y\). Essentially, it states that the name of a bound variable doesn't change the meaning or behaviour of the function. On the other hand, Beta-Reduction encapsulates the core computational process in lambda calculus. When a lambda function is applied to an expression (its argument), it checks if there are any usages of its variable in its body. If so, it replaces these "usages" with the argument. This process is called Beta-Reduction. As an example, \( (\lambda x.x+1) 2 \) reduces to \( 2+1 \), which then evaluates to 3.Exploring Extended Lambda Calculus
Lambda Calculus, while powerful, is quite minimalist. Often, for practical usage or to model more complex computations, you'll see variants or extensions of Lambda Calculus, broadly termed as "Extended" Lambda Calculus. Typically, this extended version includes concepts such as constants, operations (like addition or multiplication), conditional expressions, and recursion. Each of them enables you to perform more sophisticated calculations within the framework of Lambda Calculus. For example, in the extended version, you'd have something like:(\(\lambda x.if~(isZero~x)~then~1~else~(x * factorial~(x - 1)) \) )This function represents the computationally intensive calculation of factorial. Exploring Extended Lambda Calculus can give you a fuller understanding of the various applications and capabilities of this rich computational system in the realm of computer science.
Diving into Lambda Calculus Examples
It's time to put the theory into practice and examine some real-world examples of how Lambda Calculus operates in computer science. The beauty of Lambda Calculus lies in its elegance and simplicity - using a basic set of principles, it's capable of delineating complex computational procedures. In this section, you'll explore both simple and more advanced Lambda Calculus examples to truly cement your understanding of this fascinating paradigm.
Simple Lambda Calculus Examples
Once you've grasped the basic principles of Lambda Calculus as outlined in the previous sections, such as the concept of bound variables, alpha-equivalence, and beta-reduction, you can begin to apply these principles to practical examples. Remember: a simple lambda function is represented as \(\lambda x.f(x)\), where \(\lambda\) is the lambda operator, \(x\) is the argument, and \(f(x)\) is the function applied to \(x\). Let's start applying this knowledge to some simple examples. Example 1: Identity Function The simplest possible lambda function is the Identity Function. It is represented in Lambda Calculus as \(\lambda x.x\), and it simply returns whatever argument is passed into it.\(\lambda x.x\) → This is a function taking an argument \(x\) and returns \(x\). \(\lambda x.x (2\) → When applied with an argument of \(2\), the function returns \(2\).Example 2: Constant Function A slightly more complex concept is the Constant Function, which always returns a constant value, regardless of the input argument.
\(\lambda x.y\) → This function ignores the argument and always returns \(y\). \(\lambda x.y (2)\) → Regardless of the argument \(2\), the function returns \(y\).Example 3: Simple Arithmetic Lambda Calculus, even in its basic form, can represent rudimentary arithmetic. Considering a function that doubles any given integer:
\(\lambda x. 2x\). \(\lambda x. 2x (3)\) → When applied with an argument of \(3\), it outputs \(6\).These examples provide a clear and straightforward overview of how Lambda Calculus utilises simple expressions to compute results.
Advanced Lambda Calculus Examples
Advanced lambda calculus concepts can seem a little daunting, but with your foundational understanding of the basics, you're well-equipped to tackle these higher-level examples. Each of these concepts is a natural extension of the principles you're already familiar with. Example 1: Church Numerals These are a representation of the natural numbers using lambda notation. Introduced by Alonzo Church, the idea behind Church Numerals is to represent each number as a function. For example, the number 1 can be represented as:\( \lambda f.\lambda x.f(x) \)And the number 2 as:
\( \lambda f.\lambda x.f(f(x)) \)While this notation may seem unfamiliar, it actually provides a uniform strategy to represent all the ordinal numbers and enables basic arithmetic's definition in the lambda calculus. Example 2: Higher-Order Functions Lambda calculus' flexibility means you can create Higher-Order Functions. These are functions that can accept other functions as arguments, or return functions as results. A simple example of a higher-order function is a function that takes another function and an argument, applies the function to the argument twice, and returns the result:
\( \lambda f.\lambda x.f(f(x)) \)When the function \( \lambda z.z*2 \) and argument \( 2 \) get passed to this higher-order function, the resulting computation would be \( ((2*2)*2) = 8 \). The examples listed above demonstrate both the simplicity and power of Lambda Calculus. From computing simple arithmetic operations to defining higher-order functions, Lambda Calculus serves as a foundational language for expressing computational logic.
The Role of Lambda Calculus Y Combinator
Moving further into Lambda Calculus, you will encounter a fascinating concept - the Y Combinator. The Y Combinator holds a unique position in the array of tools offered by Lambda Calculus, representing a mechanism for achieving recursion, a key aspect of computational theory. Understanding the role of the Y Combinator can therefore enhance your understanding of not only Lambda Calculus, but also intricate computational procedures.
Understanding The Y Combinator Concept in Lambda Calculus
To genuinely comprehend the concept of the Y Combinator, you must first grasp what a combinator is. In the Lambda Calculus, a combinator is a specific kind of lambda function with no free variables. The Y Combinator, a particular type of combinator, is formulated to facilitate recursion. This concept could seem challenging, but learning it will give you a powerful tool for problem-solving. In the world of Lambda Calculus, recursion appears something like this: \( Y= λf.(λx.f (x x)) λx.f (x x) \). This is the Y Combinator. It’s a fixed-point operator that applies a function to itself until it reaches a solution. Let's break it down:Fixed-point operator: A fixed-point of a function is an input that gives the exact same output. For example, if you have a function f and an input x, then x is a fixed-point of the function if f(x) = x. The Y Combinator allows you to find the fixed point of a function, which helps you implement recursion.
Practical Examples of Lambda Calculus Y Combinator
Getting your head around the Y Combinator in a theoretical sense is one thing, but applying it practically can help illustrate its value. The recursion the Y Combinator provides is used across computer science - from sorting algorithms to data structure traversals. Let's look at a classic example of recursion – computing factorials.Factorial Function: \(factorial = λf.λn. if~(isZero~n)~then~1~else~(n * factorial (n - 1)) \) Apply Y Combinator: \( Y(factorial)\)In the above example, executing \( Y(factorial)\) computes the factorial of a number. Another example of using the Y Combinator is the Fibonacci series, a series of numbers where the next number is found by adding up the two numbers before it.
Fibonacci Function: \(fib = λf.λn. if~(isZero~n)~then~0~else~(if~(equalsTo ~n ~1)~then~1~else~((f~(n - 1)) + (f~(n - 2)) ) ) \) Apply Y Combinator: \( Y(fib)\)In this example, executing \( Y(fib) \) computes the nth number in the Fibonacci series. These practical examples underline the value the Y Combinator brings to the table in performing recursive operations. In Lambda Calculus, it serves as a fundamental component, enabling the formulation of higher-level concepts and computations. This understanding forms an integral part of the toolkit you require for mastering computer science concepts.
Lambda Calculus: Its Significance and Limitations
Lambda Calculus operates as a theoretical framework for practically all functional programming languages, serving as a bedrock for computations and algorithms. At the same time, it's important to understand the limitations this formal system carries in its practical uses. Balancing an understanding of both aspects will not only deepen your knowledge of Lambda Calculus but also offer practical insights into its applications in everyday programming scenarios.
Why Lambda Calculus is Essential in Functional Programming
When it comes to functional programming, Lambda Calculus comprises its essential backbone, offering a solid mathematical basis for encoding and manipulating functions. It's worth exploring why and how Lambda Calculus has such a profound impact on functional programming.
Firstly, Lambda Calculus fosters immutability. Functional programming stresses the absence of changing states, employing immutable data. This principle aligns seamlessly with Lambda calculus, which operates on a similar assumption that functions and variables, once defined, do not change. This feature promotes safer, more reliable code, since functions will always produce the same output given the same input, easing debugging and testing activities. Secondly, higher-order functions are a staple in functional programming, and these are directly supported by Lambda Calculus. Higher-order functions are those that accept other functions as arguments and/or return functions as results. Lambda Calculus, with its simplicity and elegance, represents these functions comfortably, offering a solid theoretical foundation. Another reason is the implementation of lazy evaluation by Lambda Calculus. Lazy evaluation is a tactic where computations are delayed until the result is necessitated. This strategy enhances efficiency, and Lambda Calculus underpins it with a clear mathematical representation. And finally, functional programming employs recursion as the primary control structure instead of the traditional loops used in imperative languages. By utilising the Y Combinator, Lambda Calculus can impeccably depict recursive functions. To summarise, here are the factors that emphasise the importance of Lambda Calculus in functional programming:- Functional programming promotes immutability, mirroring the principle of constantness in Lambda Calculus.
- The support of higher-order functions by Lambda Calculus aligns with the structure of functional programming.
- Lambda Calculus' ability to implement lazy evaluation offers more efficient coding practices.
- The depiction of recursion in functional programming is possible due to the existence of the Y Combinator in Lambda Calculus.
Limitations and Challenges of the Lambda Calculus in Practice
While Lambda Calculus serves as a powerful tool in computer science, particularly within functional programming, it's not without its challenges. A primary challenge of Lambda Calculus is its verbosity. While its mathematical simplicity is a strength, in practical terms, this can result in longer, more complex expressions compared to familiar procedural languages. This verbosity can lead to more difficult readability and maintenance of Lambda calculus expressions, particularly for those new to the paradigm. Efficiency is also a factor, as Lambda Calculus operations can sometimes be slower than their procedural counterparts. This stems from the reliance on recursion, which is inherently more resource-demanding than iteration. Implementation of certain data structures can also be more tedious in Lambda Calculus. Finally, Lambda Calculus heavily leans on the concept of static typing, which can be restrictive compared to dynamic typing languages, posing a limitation to its flexibility. To summarise, while Lambda Calculus serves as a foundational language for expressing computational logic, ultimately, these limitations must be taken into account as they can affect the efficiency and readability of solutions generated using this paradigm. Regardless, Lambda Calculus remains an indispensable tool in the computer science field, serving as the backbone for understanding and implementing functional programming language concepts. An understanding of Lambda Calculus, including its strengths and weaknesses, will significantly advance your understanding of the field.Lambda Calculus - Key takeaways
- Lambda Calculus is a system for expressing computation based on function abstraction and application.
- Three basic elements form the Lambda Calculus formulation: variables (such as x, y, z, a, b, c), abstraction (represented as λx.F where x is a variable, and F is any lambda term), and application (represented as (M N) where M and N are lambda terms).
- Key principles of Lambda Calculus include variable binding, alpha-equivalence, beta-reduction, and the extensions in "Extended" Lambda Calculus. Alpha-equivalence states that the names of variables in lambda functions are arbitrary, while beta-reduction refers to how a lambda function behaves when it's applied to an argument. Extended Lambda Calculus introduces features that enhance computational efficiency.
- The Y Combinator in Lambda Calculus is a powerful tool for achieving recursion, enabling the solution of problems that can be broken down into smaller, identical issues. The Y Combinator is a fixed-point operator that applies a function to itself until finding a solution.
- Lambda Calculus plays a fundamental role in functional programming because it supports key principles such as immutability, higher-order functions, and lazy evaluation. However, it has limitations in practical uses, which should be considered during application.
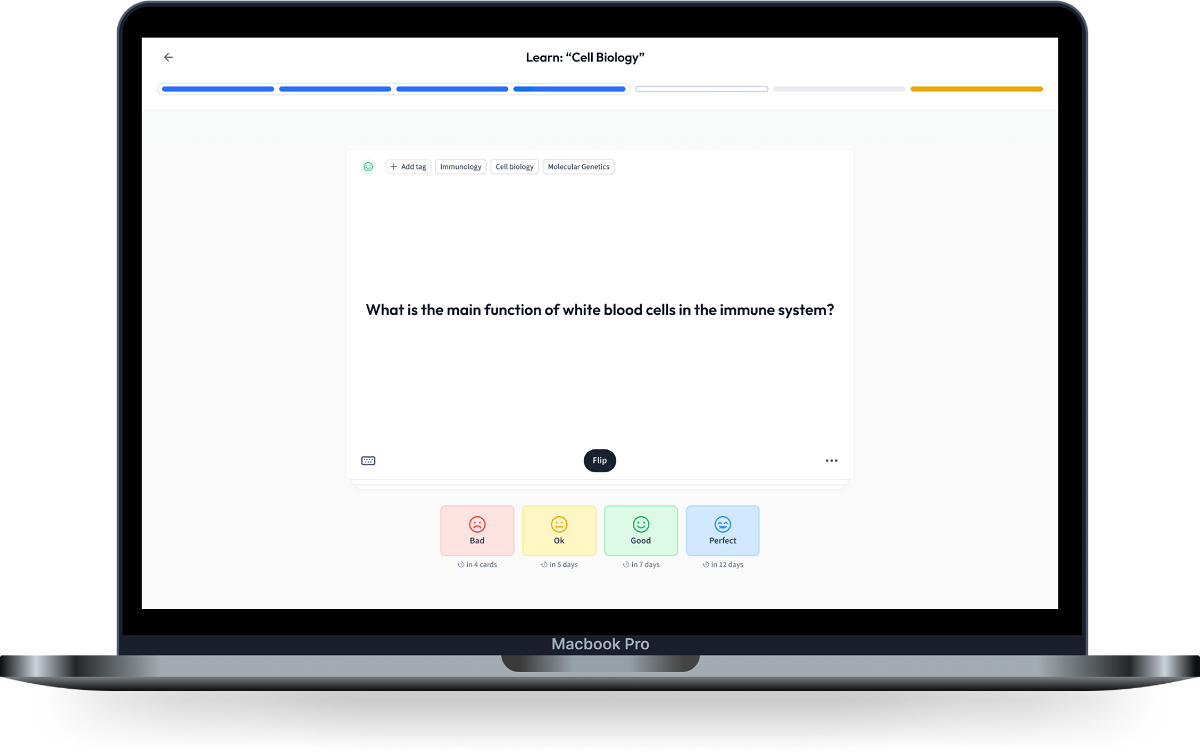
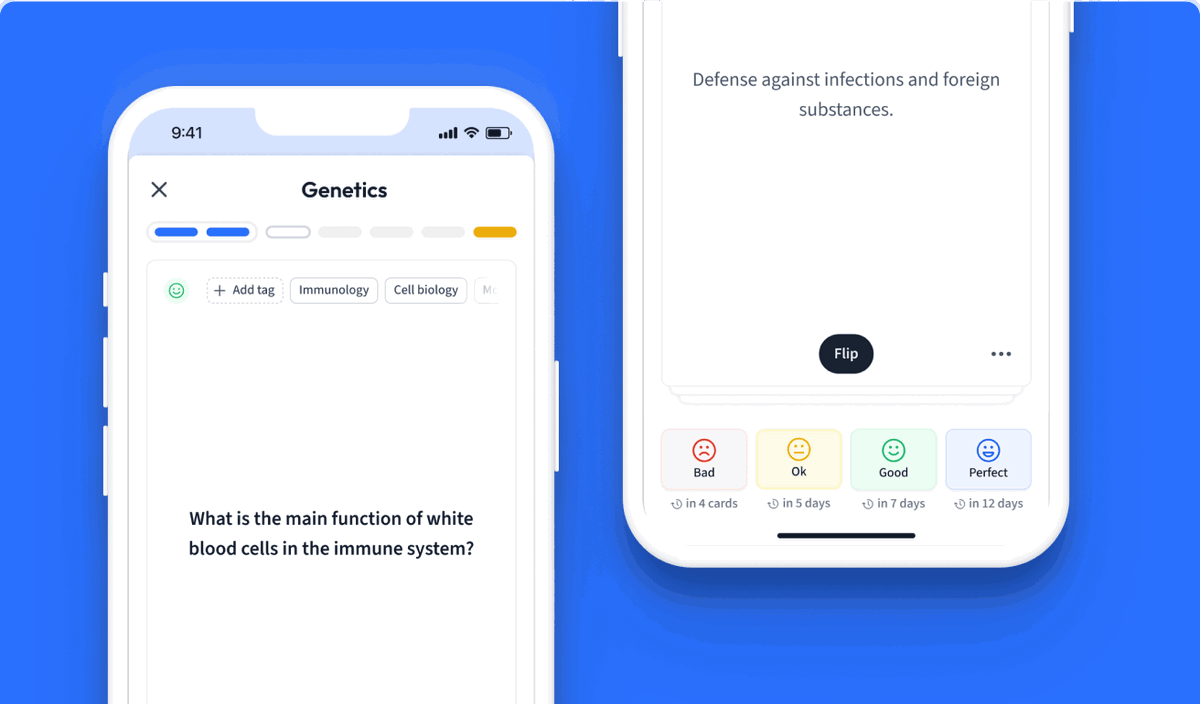
Learn with 42 Lambda Calculus flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Lambda Calculus
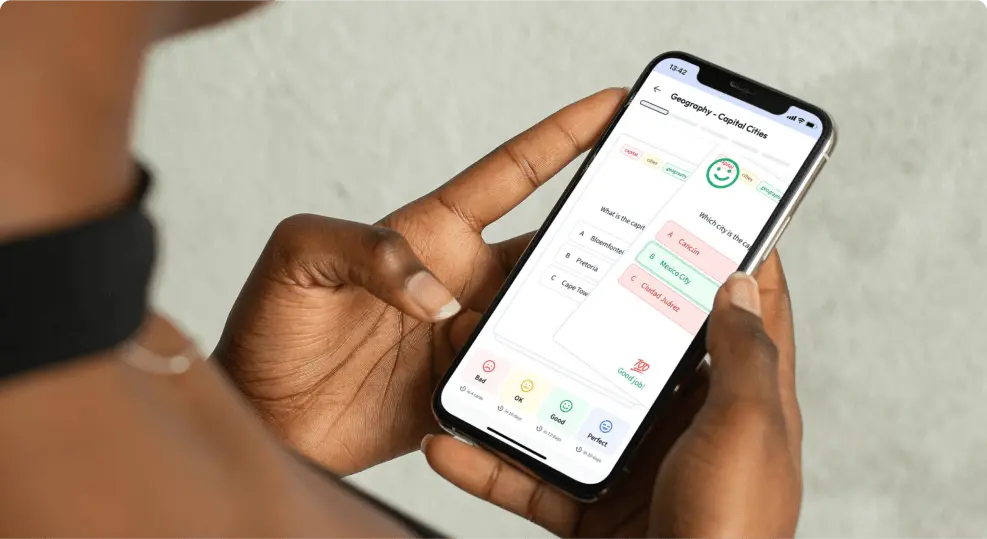
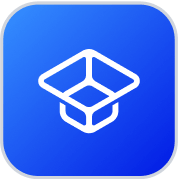
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more