Getting to Grips with the Command Line
For anyone keen on mastering Computer Science, an essential skill to acquire is a comprehensive understanding of the command line. Oft described as intimidating for beginners, the command line is a powerful tool that allows you to interact with your computer in a direct and efficient way using just text commands. The command line is an integral part of operating systems like Linux and MacOS, and still has a strong presence in Windows as well.
Understanding the Basics of the Command Line Interface
At its core, the command line interface (CLI) is a text-based UI used to operate software and operating systems. Unlike graphical user interfaces (GUIs) where you interact with visual elements, the command line requires you to type text commands to perform tasks.
Command Line Interface: A user interface that utilises text commands to interact with a computer system, software or operating system.
Core Principles of the Command Line Interface
There are several principles to grasp when it comes to using the command line, including:
- Commands: These are instructions given to the computer directly from the user. Every command has a specific task associated with it.
- Arguments: These are additional pieces of information that a command might need to run successfully. For example, a 'copy' command needs to know what file to copy and where to copy it to.
- Pipes and Redirection: These concepts are used to control the flow of data between commands or files.
Key Functions of the Command Line Interface
The CLI is renowned for its power, efficiency, and versatility. Its key functions include the ability to:
- Create or manipulate files and directories.
- Process text and data.
- Control running processes.
- Access system-level functionalities.
Command Line Examples and Their Interpretations
With a basic understanding of the command line interface, getting to grips with some common commands is the next step. In this section, we will explore some of these commands and their interpretations.
Decoding Common Command Line Examples
Let's explore some common examples:
Command | Description |
ls | List all files and directories in the current directory. |
cd | Change directory. Used for navigating through the file system. |
echo | Display a line of text. |
Challenges and Solutions in Learning Command Line Examples
Learning the command line can be daunting at first, with its seemingly cryptic commands. However, it is possible to overcome these challenges, and the best approach is through consistent practice.
Many beginners find the switch from a graphical interface to a command line interface intense. However, focus on learning the fundamental commands initially, before moving onto more complex coding commands can ease this transition.
For instance, start with mastering file handling operations like create ('touch'), read ('cat'), update ('nano'), and delete ('rm'). Once comfortable, you may move onto more advanced topics like process management and shell scripting.
Delving Deeper into Command Line Arguments in Python
If you're a student of Computer Science and already have a grip on the basics of the command line and Python programming, it's time to combine these two powerhouses. Let's peek under the hood of command line arguments in Python and understand how these can enhance your coding efficiency.
Comprehensive Overview of Command Line Arguments in Python
A command-line argument is an information that directly follows the program's name on the command line when it is run. These arguments are passed into the program. Python provides a framework to handle command line arguments - the sys module. The arguments stored in the sys.argv list in Python. The name of the script is always the first argument in this list and the rest are the arguments you pass.
sys.argv: A list in Python, which contains the command-line arguments passed to the script. The first item in the list, sys.argv[0], is always the name of the script itself.
Here's a quick visualisation of this concept:
python example_script.py arg1 arg2 arg3
In this scenario, 'example_script.py' is the script that's being executed, and 'arg1', 'arg2', 'arg3' are the command-line arguments.
This lays the groundwork for understanding how Python receives command-line arguments , but how do you use them in Python? This is where the argparse module comes into play.
argparse module: This module makes it easy to write user-friendly command-line interfaces. It parses the defined arguments from the sys.argv and generates help and usage messages.
The argparse module offers brilliant features like positional arguments, optional arguments, and diverse data types, which aids in creating flexible command-line interfaces.
Implementing Command Line Arguments Python in Real-life Scenarios
Wondering how command line arguments are applied in the real world? Let's walk through an example where you need to write a Python program that accepts a text file as a command line argument and counts the number of lines in the file.
import argparse # Create the parser parser = argparse.ArgumentParser() # Add an argument parser.add_argument('filename', help='the file to read') # Parse the argument args = parser.parse_args() # Open the file with open(args.filename, 'r') as f: lines = f.readlines() # Print the number of lines print(f"The file {args.filename} has {len(lines)} lines.")
This script utilises the argparse module to handle the command line argument that represents the file name. The script then reads this file and counts the number of lines it contains using Python's built-in functions.
Overcoming Difficulties with Command Line Arguments Python
While command line arguments in Python provide flexibility and robustness to scripts, they can also pose challenges. Errors often occur when users either provide incorrect argument values or omit necessary arguments. Furthermore, understanding and using the argparse module can initially be tricky due to its extensive features.
To overcome these obstacles:
- Provide clear and concise help messages for each argument, describing its purpose and any required format. This is done using the 'help' parameter in the add_argument() function of the argparse module.
- Set default values for arguments to ensure your script runs even in the absence of some arguments.
- Group related arguments for better organisation and readability of your code.
- Make use of Python's rich documentation and numerous resources available online to bridge any gaps in understanding of the argparse module.
Command line arguments in Python are a deep and exciting area to explore. Remember, the more hands-on practice you gain, the more familiar and comfortable you will become with incorporating command-line arguments into your Python scripts.
Investigating the Uses of Command Line
The command line, also known as a command-line interface (CLI), is more than just a window for typing in commands. Contrary to popular belief, it is an incredibly versatile tool capable of executing a wide array of tasks ranging from basic file management to complex system-level operations.
Common Uses of Command Line in Everyday Tasks
If you're wondering where command line usage comes into play in your day-to-day activities, let's delve deeper. Here are some of the common ways you may use the command line interface for routine tasks:
- File Management: The CLI provides commands to create, delete, copy, move, rename files and directories in the system storage. The commands such as 'ls' to list files, 'mv' to move or rename files, and 'cp' to copy files are extensively used.
- Networking: Whether you want to check internet connectivity, query DNS servers, or establish secure interactive text-oriented connections with other machines via SSH, command line tools got you covered.
- System Monitoring: You can rely on the command line for getting insights into system-level metrics like CPU usage, memory usage, disk usage, running processes, users logged in and much more.
- Software Installation: For operating systems like Linux, many software or package installations occur via the command line.
- Scripting and Automation: The CLI is widely used to automate repetitive tasks by scripting commands together into shell scripts.
Analysing the Advantages and Limitations of the Uses of Command Line
No system is complete without its strengths and weaknesses, and the command line is no exception. Let's take a moment to analyse the pros and cons of the command line interface.
The key strengths of the CLI include:
- Efficiency: Command line operations are generally quicker than graphical interface operations. This is particularly noticeable when dealing with large numbers of files.
- Power: The CLI provides more options and greater flexibility to the user. There isn't much you can't do from a command line.
- Automation: Repeating commands is a fundamental part of programming and system administration. The CLI is perfect for automation as the commands are standardised and predictable.
While the command line is immensely powerful, it also has its limitations:
- Steep Learning Curve: CLI requires a knowledge of commands and its syntax, which could be highly challenging for beginners.
- No Visual Interface: Unlike GUI, there is no graphical representation of the files or the system. Therefore, remembering complex commands and understanding file structures can be difficult.
- Error Prone: A single typo can yield completely different results or even harm your system. Therefore, precision is key.
Practical Demonstrations of the Uses of Command Line
Now that you have gained theoretical knowledge about the command line, practice with a few demonstration examples could be beneficial.
Playing with Files in the Terminaltouch newfile.txt ls mv newfile.txt renamedfile.txt ls
In the code snippet above, four basic file operations are done. 'touch' command creates a file 'newfile.txt'. 'ls' lists the files, 'mv' is used to rename 'newfile.txt' to 'renamedfile.txt’. Running 'ls' again will display 'renamedfile.txt' in the list proving the file renaming was successful.
Checking Network Detailsping google.com
The 'ping' command sends network requests to the domain name (in this case, 'google.com') and checks if responses are received, verifying that the network pathway to the domain exists and is active.
These hands-on examples should help you familiarise with the command line and its wide-ranging capabilities. Remember, consistent practice is the key to getting comfortable with the command line and eventually becoming proficient with it.
Exploring the Application of Command Line in Computer Science
The command line's role in Computer Science is far-reaching and pervasive, extending beyond basic file management and administrative tasks. The practical applications are visible in various thematic structures of Computer Science, including system administration, software development, database management, network configuration, and more.
Role of Command Line in Computer Science Theory
The sphere of computer science theory, which encapsulates topics such as algorithms, data structures, and computational complexity, may not seem a likely place for the application of the command line at first glance. However, it plays an implicit, yet distinct role. }
Understanding command line interfaces and their functioning involves elements of computer system architecture, operating systems, programming languages, and software design - all of which are essential components of Computer Science theory. Learning the command line helps to elucidate these abstract concepts, as it demonstrates how software interacts with the system hardware on a lower level than most modern graphical interfaces.
In addition, the command line serves as a tool for implementing and testing theoretical concepts. Especially in the realm of algorithms, the command line offers an excellent testing ground. Various UNIX-based commands form the backbone of the principle of 'pipes and filters', a software architecture style which employs data transformation through a series of processing steps or stages. This architecture style has roots in mathematical function composition, a concept explored in algorithmic theory.
Furthermore, classroom teaching of Computer Science heavily relies on the command line. Interactive tutorials and programming tasks are often conducted using command line-based systems like the IPython shell or Jupyter notebook, as they offer flexibility and control that a GUI may not.
Literature Review of Command Line in Computer Science Research
A literature review reveals many research papers that highlight the value of command line interfaces in Computer Science. They are primarily focused on three aspects: the usability of command line versus graphical user interfaces, the evolution of command line interfaces, and the educational value of teaching command line.
For example, studies conducted by Nielsen focus on the efficiencies gained when users transition from GUIs to command line interfaces. It was found that while GUIs present a lower learning curve, command line interfaces result in greater long-term productivity due to the faster execution speeds and potential automation.
Research conducted by University of Maryland explores the rich history of command line interfaces, tracing back to early computers like UNIX. This research exemplifies how principles from the command line interfaces form the foundations of modern GUIs. The command line interface's staying power, despite the ubiquitous presence of GUIs, has also been a topic of investigation.
Research on teaching approaches, like the work done at Stanford University, highlights the importance of teaching command line interfaces early on in Computer Science programmes. It strengthens the students' understanding of operating systems, data structures, and algorithms.
Case Studies: Application of Command Line in Computer Science
A deeper understanding of the impact of command line interfaces can be gained by examining practical examples or case studies. Here are two examples:
Firstly, in the realm of advanced software development. SpaceX, the aerospace manufacturer, substantially uses Linux and its command line interfaces for its operations. Their spacecrafts' software runs on Linux and the programmers develop and execute programs using the command line. Falcon 9, the flagship rocket of SpaceX, was programmed using command line tools.
Secondly, the power of command line interfaces is also demonstrated by the creation of powerful software development tools. Git, a version control system used by programmers worldwide, started as a set of command line utilities written by Linus Torvalds. Git's command line interface provides higher speed and more control as compared to its GUI counterparts.
Lastly, well-known universities like MIT and Stanford often involve practical command line tasks in their introductory programming courses. Not only does this help students understand the foundational working principles of computer systems, but also prepares them thoroughly for advanced tasks such as software development and systems administration - areas where command line interfaces are indispensable.
Embracing Command Line: A Key to Success in Computer Systems
The command line, a mighty tool for controlling computers, continues to be integral in the world of Computer Science and Information Technology. Although graphical user interfaces (GUI) often provide more intuitive experiences for everyday computing tasks, the flexibility and control offered by the command line remain unparalleled, marking it as a crucial skill for success in computer systems.'
Fostering Proficiency in Command Line for Computer Systems
Mastering the command line is a key success factor when navigating the realm of computer systems. In the sections that follow, you'll encounter actionable suggestions to bolster your proficiency in this dynamic skill.
Firstly, understanding its potential applications is paramount. The command line has an extensive range of usage that spans from creating and managing files and directories to launching and controlling software applications, from monitoring system performance to automating repetitive tasks via scripts. These are just the crudely ground nuts and bolts, with the possibilities spiralling into more complex realms not limited to database management, system maintenance, network configuration, and much more.
Secondly, acquiring knowledge about the command syntax is critical. In essence, a command line operation compiles a command, followed by one or more spaces, followed by zero or more command-line arguments:
command [argument...]
Each argument provides a distinct context or directive to the command. Notably, the option to utilise wildcards and redirection operators allows command arguments to incorporate pattern matching and to manipulate input and output, respectively.
Lastly, practice is vital. The command line can be unforgiving, with minor typos potentially causing significant harm. Consistent practice across a diverse range of tasks can help recalibrate your approach and mitigate errors, providing you with the confidence to utilise these tools effectively and efficiently.
Adapting to the Growing Importance of Command Line in Computer Systems
The ever-increasing complexity of modern computer systems has rendered command line knowledge significantly important. It is no longer an optional extra, but an essential requirement for anyone seriously considering a career in Computer Science, System Administration, or Network Engineering.
The power of the command line, although more challenging to master, provides notable benefits – including automation and performance efficiency, going beyond what's typically possible with GUIs. This is particularly true when managing large networks of servers, where commands need to be issued from a central point and run on multiple machines simultaneously. This operation can be fine-tuned using scripting capabilities provided by Command Line Interfaces (CLIs).
Moreover, majority of cloud platforms and operating systems, including Linux and macOS, rely heavily on command line interfaces. Having proficiency in command line gives you an edge when working with APIs, automating deployments, managing virtual machines, handling version control systems like Git, or even working with data on a large scale.
The command line also plays a crucial role in security. Many sophisticated attacks on systems are command line-based. Thus, understanding command line interfaces makes you a sharper, more aware practitioner in terms of system vulnerabilities and security.
Underscoring the Value of Command Line Skills for Future Computer Scientists
The path to becoming a proficient computer scientist undoubtedly passes through the domain of the command line. Its importance cannot be overstated in the contemporary digital world.
From an employment perspective, command line skills are highly sought after. Many employers, particularly those operating large scale systems, look for command line proficiency in potential employees because it demonstrates a deeper understanding of computer systems.
Academic institutions globally recognise the significance of the command line and have integrated it into their curricula. Command line interfaces form a crucial part of the pedagogy, right from the introductory stages of computer science programs to advanced levels. Command line exercises, tasks, and projects are common, ensuring students gain a firm grounding in this skill.
For budding computer scientists, understanding and using the command line can lead to many opportunities. Be it software development, system administration, cyber security, data analysis, or research, command line skills help open doors to a wide range of job roles in the industry.
Lastly, the command line can aid in professional development. As most high-performance servers run Linux, understanding the command line equips you with the knowledge to work with high-level computing resources, including supercomputers and cloud-based infrastructure. This can lead to gaining expertise in advanced areas such as computational modelling, data science, and High-Performance Computing (HPC), which are driving technological advancements and breakthroughs.
Command Line - Key takeaways
- A command-line argument is data that immediately follows the name of the program in the command line. These arguments are then passed into the program. The sys module in Python is responsible for handling these arguments, which are stored in the sys.argv list.
- sys.argv is a Python list containing the command-line arguments that are passed into a script. The name of the script is always listed as the first argument.
- The argparse module facilitates the use of command-line arguments in Python by enabling the creation of user-friendly command-line interfaces. It takes the defined arguments from sys.argv and generates relevant help and usage messages.
- Command line, known as the command-line interface (CLI), is a versatile tool that can manage a wide array of tasks, from basic file management to complex system-level operations.
- Learning and using the command line is important in areas of Computer Science such as system administration, software development, and database management. It helps to clarify abstract concepts and offers a method for implementing and testing ideas, especially in the realm of algorithms.
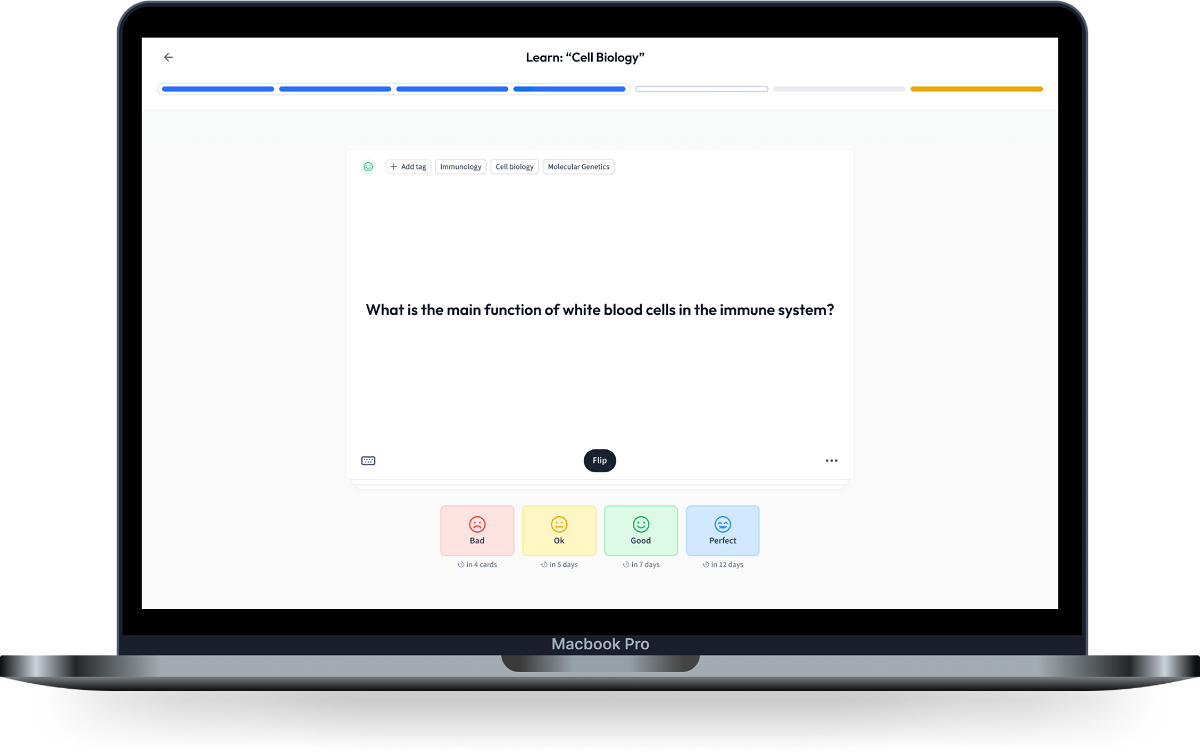
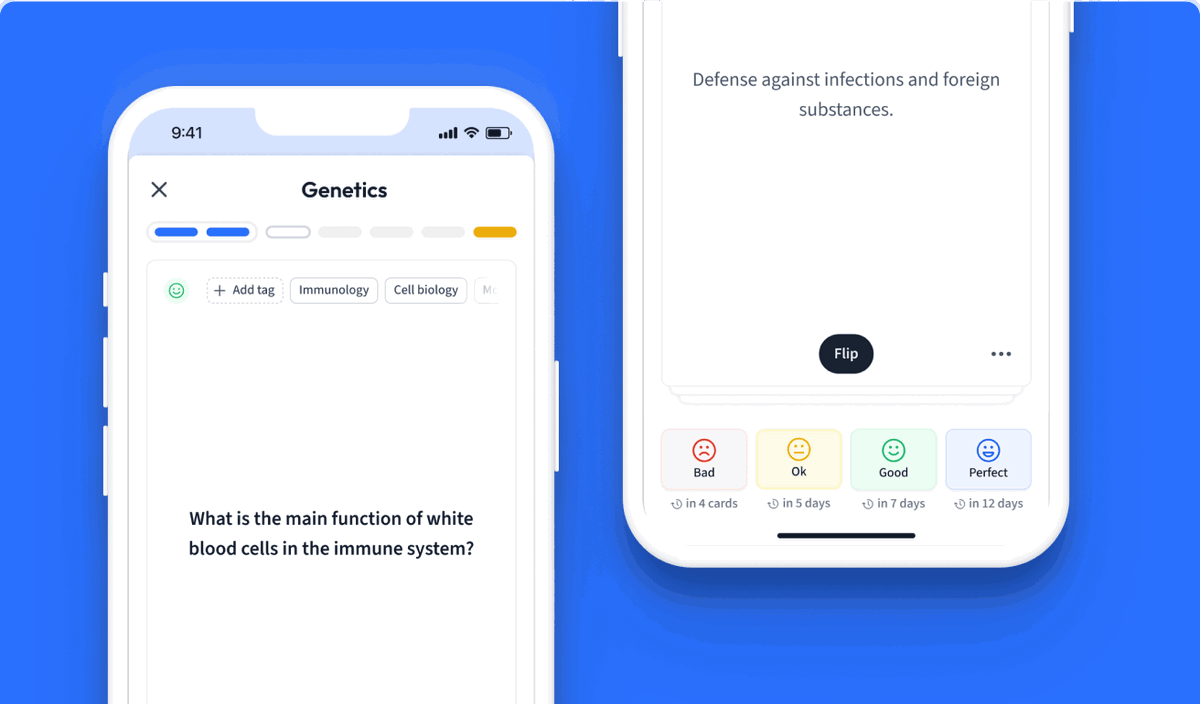
Learn with 15 Command Line flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about Command Line
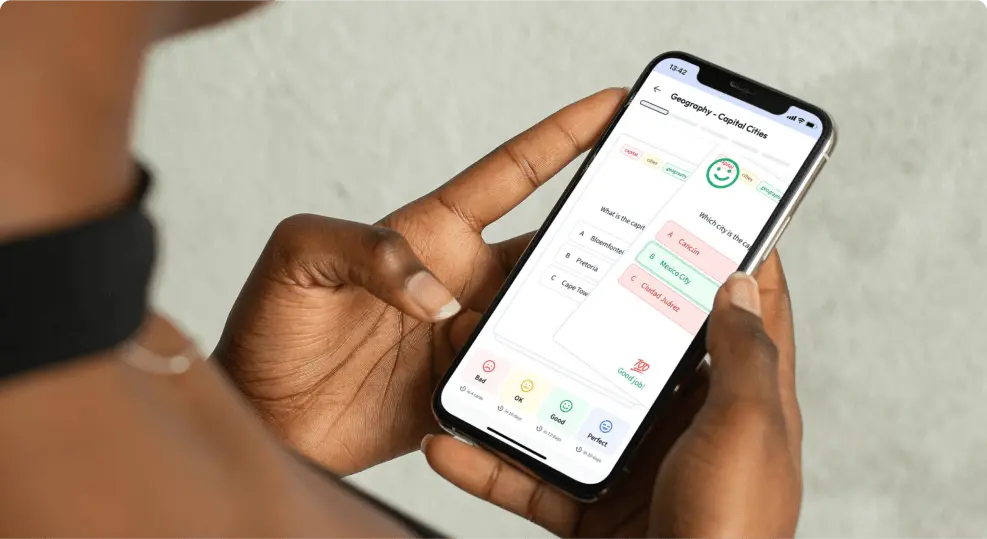
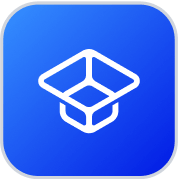
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more